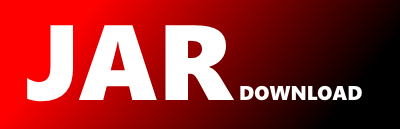
com.embeddedunveiled.serial.SerialComByteStream Maven / Gradle / Ivy
Show all versions of scm Show documentation
/**
* Author : Rishi Gupta
*
* This file is part of 'serial communication manager' library.
*
* The 'serial communication manager' is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by the Free Software
* Foundation, either version 3 of the License, or (at your option) any later version.
*
* The 'serial communication manager' is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A
* PARTICULAR PURPOSE. See the GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with serial communication manager. If not, see .
*/
package com.embeddedunveiled.serial;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
/**
* This class represents an output stream of bytes that gets sent over to serial port for transmission.
*/
class SerialComOutputStream extends OutputStream {
private SerialComManager scm = null;
private long handle = 0;
public SerialComOutputStream(SerialComManager scm, long handle) {
this.scm = scm;
this.handle = handle;
}
/**
* Writes the specified byte to this output stream (eight low-order bits of the argument data).
* @param integer data to be written to serial port
* @throws IOException if write fails
*/
@Override
public void write(int data) throws IOException {
try {
scm.writeSingleByte(handle, (byte)data);
} catch (SerialComException e) {
throw new IOException(e);
}
}
/**
* Writes data.length bytes from the specified byte array to this output stream.
* @param byte type array of data to be written to serial port
* @throws IOException if write fails
*/
@Override
public void write(byte[] data) throws IOException {
try {
scm.writeBytes(handle, data, 0);
} catch (SerialComException e) {
throw new IOException(e);
}
}
/**
* Writes len bytes from the specified byte array starting at offset off to this output stream.
* If b is null, a NullPointerException is thrown.
* If off is negative, or len is negative, or off+len is greater than the length of the array data,
* then an IndexOutOfBoundsException is thrown.
* @param data byte type array of data to be written to serial port
* @param off offset from where to start sending data
* @param len length of data to be written
* @throws IOException if write fails
*/
@Override
public void write(byte[] data, int off, int len) throws IOException, IndexOutOfBoundsException {
if(data == null) {
throw new NullPointerException("write(), " + SerialComErrorMapper.ERR_WRITE_NULL_DATA_PASSED);
}
if((off < 0) || (len < 0) || ((off+len) < data.length)) {
throw new IndexOutOfBoundsException("write(), " + SerialComErrorMapper.ERR_WRITE_INDEX_VIOLATION);
}
byte[] buf = new byte[len];
System.arraycopy(data, off, buf, 0, len);
try {
scm.writeBytes(handle, buf, 0);
} catch (SerialComException e) {
throw new IOException(e);
}
}
/**
*
The flush method of OutputStream does nothing. Kept in source code just to specify developer
* about behaviour of this library when flush is called.
*/
@Override
public void flush() throws IOException {
}
}
/**
* This class represents an input stream of bytes that gets sent over to serial port for transmission.
*/
class SerialComInputStream extends InputStream {
private SerialComManager scm = null;
private long handle = 0;
public SerialComInputStream(SerialComManager scm, long handle) {
this.scm = scm;
this.handle = handle;
}
/**
* Returns an estimate of the minimum number of bytes that can be read from this input stream
* without blocking by the next invocation of a method for this input stream.
* @return an estimate of the minimum number of bytes that can be read from this input stream without blocking
* @throws IOException - if an I/O error occurs.
*/
@Override
public int available() throws IOException {
int[] numBytesAvailable = new int[2];
try {
numBytesAvailable = scm.getByteCountInPortIOBuffer(handle);
} catch (SerialComException e) {
throw new IOException(e);
}
return numBytesAvailable[0];
}
/**
* scm does not support mark and reset of input stream. If required, it can be developed at application level.
* @return always returns false
*/
@Override
public boolean markSupported() {
return false;
}
/**
* Reads the next byte of data from the input stream. The value byte is returned as an int in
* the range 0 to 255. If no byte is available because the end of the stream has been reached,
* the value -1 is returned.
*
* From the perspective of serial port communication, it should be noted that when this method
* returns 0 which indicate that there was no data, data might have arrived just after the instant
* this method returned.
*
* @return the next byte of data, or 0 no byte is read.
* @throws IOException - if an I/O error occurs.
*/
@Override
public int read() throws IOException {
int x = 0;
byte data[] = new byte[1];
try {
data = scm.readBytes(handle, 1);
if(data == null) {
x = -1;
}
} catch (SerialComException e) {
throw new IOException(e);
}
return x;
}
/**
* scm does not support skip. If required, it can be developed at application level.
* @param number of bytes to skip
* @return always returns 0
*/
@Override
public long skip(long n) {
return 0;
}
}
/**
* Note that scm is non-blocking I/O.
*/
public final class SerialComByteStream {
private SerialComManager scm = null;
private long handle = 0;
private SerialComOutputStream outStream = null;
private SerialComInputStream inStream = null;
/**
*
*/
public SerialComByteStream(SerialComManager scm, long handle) {
this.scm = scm;
this.handle = handle;
}
public SerialComOutputStream getSerialComOutputStream() {
if(outStream == null) {
outStream = new SerialComOutputStream(scm, handle);
}
return outStream;
}
public SerialComInputStream getSerialComInputStream() {
if(inStream == null) {
inStream = new SerialComInputStream(scm, handle);
}
return inStream;
}
}