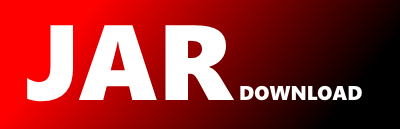
com.emc.ecs.nfsclient.network.NetMgr Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nfs-client Show documentation
Show all versions of nfs-client Show documentation
NFS Client for Java - provides read/write access to data on NFS servers. The current implementation supports only NFS version 3.
/**
* Copyright 2016 EMC Corporation. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://www.apache.org/licenses/LICENSE-2.0.txt
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.emc.ecs.nfsclient.network;
import com.emc.ecs.nfsclient.rpc.RpcException;
import com.emc.ecs.nfsclient.rpc.Xdr;
import org.jboss.netty.channel.ChannelFactory;
import org.jboss.netty.channel.socket.nio.NioClientSocketChannelFactory;
import java.net.InetSocketAddress;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.Executors;
/**
* Singleton class to manage all Connection instances
*
* @author seibed
*/
public class NetMgr {
/**
* The single instance.
*/
private static final NetMgr _instance = new NetMgr();
/**
* @return The instance.
*/
public static NetMgr getInstance() {
return _instance;
}
/**
* Construct the private instance.
*/
private NetMgr() {
super();
}
/**
* connection tracking map
*/
private ConcurrentHashMap _connectionMap = new ConcurrentHashMap();
/**
* privileged connection tracking map
*/
private ConcurrentHashMap _privilegedConnectionMap = new ConcurrentHashMap();
/**
* Netty helper instance.
*/
private ChannelFactory _factory = new NioClientSocketChannelFactory(Executors.newCachedThreadPool(),
Executors.newCachedThreadPool());
/**
* Basic RPC call functionality only. Send the request, creating a new
* connection as necessary, and return the raw Xdr returned.
*
* @param serverIP
* The endpoint of the server being called.
* @param port
* The remote host port being called for this operation.
* @param usePrivilegedPort
*
* - If
true
, use a privileged local port (below
* 1024) for RPC communication.
* - If
false
, use any non-privileged local port
* for RPC communication.
*
* @param xdrRequest
* The Xdr data for the request.
* @param timeout
* The timeout in seconds.
* @return The Xdr data for the response.
* @throws RpcException
*/
public Xdr sendAndWait(String serverIP, int port, boolean usePrivilegedPort, Xdr xdrRequest, int timeout) throws RpcException {
InetSocketAddress key = InetSocketAddress.createUnresolved(serverIP, port);
Map connectionMap = usePrivilegedPort ? _privilegedConnectionMap : _connectionMap;
Connection connection = connectionMap.get(key);
if (connection == null) {
connection = new Connection(serverIP, port, usePrivilegedPort);
connectionMap.put(key, connection);
connection.connect();
}
return connection.sendAndWait(timeout, xdrRequest);
}
/**
* Remove a dropped connection from the map.
*
* @param key
* The key
*/
public void dropConnection(InetSocketAddress key) {
_connectionMap.remove(key);
_privilegedConnectionMap.remove(key);
}
/**
* Called when the application is being shut down.
*/
public void shutdown() {
for (Connection connection : _connectionMap.values()) {
connection.shutdown();
}
for (Connection connection : _privilegedConnectionMap.values()) {
connection.shutdown();
}
_factory.releaseExternalResources();
}
/**
* Getter method for Factory access.
*
* @return The factory.
*/
public ChannelFactory getFactory() {
return _factory;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy