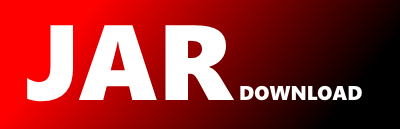
com.emc.codec.util.CodecUtil Maven / Gradle / Ivy
/*
* Copyright (c) 2015, EMC Corporation.
* Redistribution and use in source and binary forms, with or without modification,
* are permitted provided that the following conditions are met:
*
* + Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
* + Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* + The name of EMC Corporation may not be used to endorse or promote
* products derived from this software without specific prior written
* permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED
* TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
* PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS
* BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*
*/
package com.emc.codec.util;
import java.util.Map;
public final class CodecUtil {
public static String getEncodeType(String encodeSpec) {
if (encodeSpec.contains(":")) return encodeSpec.substring(0, encodeSpec.indexOf(":"));
return encodeSpec;
}
public static String getEncodeAlgorithm(String encodeSpec) {
if (encodeSpec.contains(":")) {
String algorithm = encodeSpec.substring(encodeSpec.indexOf(":") + 1);
if (algorithm.trim().length() > 0) return algorithm;
}
return null;
}
public static String getEncodeSpec(String type, String algorithm) {
if (algorithm == null || algorithm.trim().length() == 0) return type;
return type + ":" + algorithm;
}
/**
* Note: you can only search system properties if defaultValue is one of [String, Integer, Long, Float, Double]
*/
@SuppressWarnings("unchecked")
public static T getCodecProperty(String propertyName, Map codecProperties, T defaultValue) {
if (codecProperties != null && codecProperties.containsKey(propertyName))
return (T) codecProperties.get(propertyName);
String systemValue = System.getProperty(propertyName);
if (systemValue != null) {
if (defaultValue.getClass() == String.class) return (T) systemValue;
else if (defaultValue.getClass() == Integer.class) return (T) Integer.valueOf(systemValue);
else if (defaultValue.getClass() == Long.class) return (T) Long.valueOf(systemValue);
else if (defaultValue.getClass() == Float.class) return (T) Float.valueOf(systemValue);
else if (defaultValue.getClass() == Double.class) return (T) Double.valueOf(systemValue);
}
return defaultValue;
}
private CodecUtil() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy