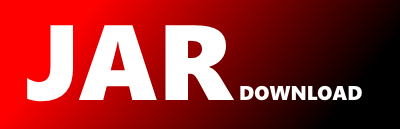
com.amazonaws.AmazonWebServiceRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws-java-sdk Show documentation
Show all versions of aws-java-sdk Show documentation
The Amazon Web Services SDK for Java provides Java APIs for building software on AWS' cost-effective, scalable, and reliable infrastructure products. The AWS Java SDK allows developers to code against APIs for all of Amazon's infrastructure web services (Amazon S3, Amazon EC2, Amazon SQS, Amazon Relational Database Service, Amazon AutoScaling, etc).
The newest version!
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws;
import java.util.HashMap;
import java.util.Map;
import org.apache.http.annotation.NotThreadSafe;
import com.amazonaws.auth.AWSCredentials;
import com.amazonaws.metrics.RequestMetricCollector;;
/**
* Base class for all user facing web service request objects.
*/
@NotThreadSafe
public abstract class AmazonWebServiceRequest {
/**
* Arbitrary options storage for individual {@link AmazonWebServiceRequest}s. This
* field is not intended to be used by clients.
*/
private final RequestClientOptions requestClientOptions = new RequestClientOptions();
/**
* A request metric collector used for this specific service request; or
* null if there is none. This collector always takes precedence over the
* ones specified at the http client level and AWS SDK level.
*/
private RequestMetricCollector requestMetricCollector;
/**
* The optional credentials to use for this request - overrides the
* default credentials set at the client level.
*/
private AWSCredentials credentials;
/**
* Sets the optional credentials to use for this request, overriding the
* default credentials set at the client level.
*
* @param credentials
* The optional AWS security credentials to use for this request,
* overriding the default credentials set at the client level.
*/
public void setRequestCredentials(AWSCredentials credentials) {
this.credentials = credentials;
}
/**
* Returns the optional credentials to use to sign this request, overriding
* the default credentials set at the client level.
*
* @return The optional credentials to use to sign this request, overriding
* the default credentials set at the client level.
*/
public AWSCredentials getRequestCredentials() {
return credentials;
}
/**
* Internal only method for accessing private, internal request parameters.
* Not intended for direct use by callers.
*
* @return private, internal request parameter information.
*/
public Map copyPrivateRequestParameters() {
return new HashMap();
}
/**
* Gets the options stored with this request object. Intended for internal
* use only.
*/
public RequestClientOptions getRequestClientOptions() {
return requestClientOptions;
}
/**
* Returns a request level metric collector; or null if not specified.
*/
public RequestMetricCollector getRequestMetricCollector() {
return requestMetricCollector;
}
/**
* Sets a request level request metric collector which takes precedence over
* the ones at the http client level and AWS SDK level.
*/
public void setRequestMetricCollector(RequestMetricCollector requestMetricCollector) {
this.requestMetricCollector = requestMetricCollector;
}
/**
* Specifies a request level metric collector which takes precedence over
* the ones at the http client level and AWS SDK level.
*/
public T withRequestMetricCollector(RequestMetricCollector metricCollector) {
setRequestMetricCollector(metricCollector);
@SuppressWarnings("unchecked") T t = (T)this;
return t;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy