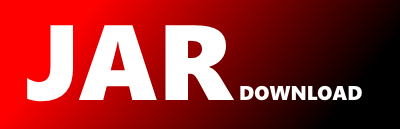
com.amazonaws.services.autoscaling.model.AutoScalingInstanceDetails Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.autoscaling.model;
import java.io.Serializable;
/**
*
* The AutoScalingInstanceDetails
data type.
*
*/
public class AutoScalingInstanceDetails implements Serializable {
/**
* The instance ID of the Amazon EC2 instance.
*
* Constraints:
* Length: 1 - 16
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*/
private String instanceId;
/**
* The name of the Auto Scaling group associated with this instance.
*
* Constraints:
* Length: 1 - 255
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*/
private String autoScalingGroupName;
/**
* The Availability Zone in which this instance resides.
*
* Constraints:
* Length: 1 - 255
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*/
private String availabilityZone;
/**
* The life cycle state of this instance. for more information, see Instance
* Lifecycle State in the Auto Scaling Developer Guide.
*
* Constraints:
* Length: 1 - 32
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*/
private String lifecycleState;
/**
* The health status of this instance. "Healthy" means that the instance
* is healthy and should remain in service. "Unhealthy" means that the
* instance is unhealthy. Auto Scaling should terminate and replace it.
*
* Constraints:
* Length: 1 - 32
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*/
private String healthStatus;
/**
* The launch configuration associated with this instance.
*
* Constraints:
* Length: 1 - 255
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*/
private String launchConfigurationName;
/**
* The instance ID of the Amazon EC2 instance.
*
* Constraints:
* Length: 1 - 16
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @return The instance ID of the Amazon EC2 instance.
*/
public String getInstanceId() {
return instanceId;
}
/**
* The instance ID of the Amazon EC2 instance.
*
* Constraints:
* Length: 1 - 16
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @param instanceId The instance ID of the Amazon EC2 instance.
*/
public void setInstanceId(String instanceId) {
this.instanceId = instanceId;
}
/**
* The instance ID of the Amazon EC2 instance.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 16
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @param instanceId The instance ID of the Amazon EC2 instance.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public AutoScalingInstanceDetails withInstanceId(String instanceId) {
this.instanceId = instanceId;
return this;
}
/**
* The name of the Auto Scaling group associated with this instance.
*
* Constraints:
* Length: 1 - 255
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @return The name of the Auto Scaling group associated with this instance.
*/
public String getAutoScalingGroupName() {
return autoScalingGroupName;
}
/**
* The name of the Auto Scaling group associated with this instance.
*
* Constraints:
* Length: 1 - 255
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @param autoScalingGroupName The name of the Auto Scaling group associated with this instance.
*/
public void setAutoScalingGroupName(String autoScalingGroupName) {
this.autoScalingGroupName = autoScalingGroupName;
}
/**
* The name of the Auto Scaling group associated with this instance.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 255
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @param autoScalingGroupName The name of the Auto Scaling group associated with this instance.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public AutoScalingInstanceDetails withAutoScalingGroupName(String autoScalingGroupName) {
this.autoScalingGroupName = autoScalingGroupName;
return this;
}
/**
* The Availability Zone in which this instance resides.
*
* Constraints:
* Length: 1 - 255
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @return The Availability Zone in which this instance resides.
*/
public String getAvailabilityZone() {
return availabilityZone;
}
/**
* The Availability Zone in which this instance resides.
*
* Constraints:
* Length: 1 - 255
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @param availabilityZone The Availability Zone in which this instance resides.
*/
public void setAvailabilityZone(String availabilityZone) {
this.availabilityZone = availabilityZone;
}
/**
* The Availability Zone in which this instance resides.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 255
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @param availabilityZone The Availability Zone in which this instance resides.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public AutoScalingInstanceDetails withAvailabilityZone(String availabilityZone) {
this.availabilityZone = availabilityZone;
return this;
}
/**
* The life cycle state of this instance. for more information, see Instance
* Lifecycle State in the Auto Scaling Developer Guide.
*
* Constraints:
* Length: 1 - 32
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @return The life cycle state of this instance. for more information, see Instance
* Lifecycle State in the Auto Scaling Developer Guide.
*/
public String getLifecycleState() {
return lifecycleState;
}
/**
* The life cycle state of this instance. for more information, see Instance
* Lifecycle State in the Auto Scaling Developer Guide.
*
* Constraints:
* Length: 1 - 32
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @param lifecycleState The life cycle state of this instance. for more information, see Instance
* Lifecycle State in the Auto Scaling Developer Guide.
*/
public void setLifecycleState(String lifecycleState) {
this.lifecycleState = lifecycleState;
}
/**
* The life cycle state of this instance. for more information, see Instance
* Lifecycle State in the Auto Scaling Developer Guide.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 32
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @param lifecycleState The life cycle state of this instance. for more information, see Instance
* Lifecycle State in the Auto Scaling Developer Guide.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public AutoScalingInstanceDetails withLifecycleState(String lifecycleState) {
this.lifecycleState = lifecycleState;
return this;
}
/**
* The health status of this instance. "Healthy" means that the instance
* is healthy and should remain in service. "Unhealthy" means that the
* instance is unhealthy. Auto Scaling should terminate and replace it.
*
* Constraints:
* Length: 1 - 32
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @return The health status of this instance. "Healthy" means that the instance
* is healthy and should remain in service. "Unhealthy" means that the
* instance is unhealthy. Auto Scaling should terminate and replace it.
*/
public String getHealthStatus() {
return healthStatus;
}
/**
* The health status of this instance. "Healthy" means that the instance
* is healthy and should remain in service. "Unhealthy" means that the
* instance is unhealthy. Auto Scaling should terminate and replace it.
*
* Constraints:
* Length: 1 - 32
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @param healthStatus The health status of this instance. "Healthy" means that the instance
* is healthy and should remain in service. "Unhealthy" means that the
* instance is unhealthy. Auto Scaling should terminate and replace it.
*/
public void setHealthStatus(String healthStatus) {
this.healthStatus = healthStatus;
}
/**
* The health status of this instance. "Healthy" means that the instance
* is healthy and should remain in service. "Unhealthy" means that the
* instance is unhealthy. Auto Scaling should terminate and replace it.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 32
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @param healthStatus The health status of this instance. "Healthy" means that the instance
* is healthy and should remain in service. "Unhealthy" means that the
* instance is unhealthy. Auto Scaling should terminate and replace it.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public AutoScalingInstanceDetails withHealthStatus(String healthStatus) {
this.healthStatus = healthStatus;
return this;
}
/**
* The launch configuration associated with this instance.
*
* Constraints:
* Length: 1 - 255
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @return The launch configuration associated with this instance.
*/
public String getLaunchConfigurationName() {
return launchConfigurationName;
}
/**
* The launch configuration associated with this instance.
*
* Constraints:
* Length: 1 - 255
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @param launchConfigurationName The launch configuration associated with this instance.
*/
public void setLaunchConfigurationName(String launchConfigurationName) {
this.launchConfigurationName = launchConfigurationName;
}
/**
* The launch configuration associated with this instance.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 255
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @param launchConfigurationName The launch configuration associated with this instance.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public AutoScalingInstanceDetails withLaunchConfigurationName(String launchConfigurationName) {
this.launchConfigurationName = launchConfigurationName;
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getInstanceId() != null) sb.append("InstanceId: " + getInstanceId() + ",");
if (getAutoScalingGroupName() != null) sb.append("AutoScalingGroupName: " + getAutoScalingGroupName() + ",");
if (getAvailabilityZone() != null) sb.append("AvailabilityZone: " + getAvailabilityZone() + ",");
if (getLifecycleState() != null) sb.append("LifecycleState: " + getLifecycleState() + ",");
if (getHealthStatus() != null) sb.append("HealthStatus: " + getHealthStatus() + ",");
if (getLaunchConfigurationName() != null) sb.append("LaunchConfigurationName: " + getLaunchConfigurationName() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getInstanceId() == null) ? 0 : getInstanceId().hashCode());
hashCode = prime * hashCode + ((getAutoScalingGroupName() == null) ? 0 : getAutoScalingGroupName().hashCode());
hashCode = prime * hashCode + ((getAvailabilityZone() == null) ? 0 : getAvailabilityZone().hashCode());
hashCode = prime * hashCode + ((getLifecycleState() == null) ? 0 : getLifecycleState().hashCode());
hashCode = prime * hashCode + ((getHealthStatus() == null) ? 0 : getHealthStatus().hashCode());
hashCode = prime * hashCode + ((getLaunchConfigurationName() == null) ? 0 : getLaunchConfigurationName().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof AutoScalingInstanceDetails == false) return false;
AutoScalingInstanceDetails other = (AutoScalingInstanceDetails)obj;
if (other.getInstanceId() == null ^ this.getInstanceId() == null) return false;
if (other.getInstanceId() != null && other.getInstanceId().equals(this.getInstanceId()) == false) return false;
if (other.getAutoScalingGroupName() == null ^ this.getAutoScalingGroupName() == null) return false;
if (other.getAutoScalingGroupName() != null && other.getAutoScalingGroupName().equals(this.getAutoScalingGroupName()) == false) return false;
if (other.getAvailabilityZone() == null ^ this.getAvailabilityZone() == null) return false;
if (other.getAvailabilityZone() != null && other.getAvailabilityZone().equals(this.getAvailabilityZone()) == false) return false;
if (other.getLifecycleState() == null ^ this.getLifecycleState() == null) return false;
if (other.getLifecycleState() != null && other.getLifecycleState().equals(this.getLifecycleState()) == false) return false;
if (other.getHealthStatus() == null ^ this.getHealthStatus() == null) return false;
if (other.getHealthStatus() != null && other.getHealthStatus().equals(this.getHealthStatus()) == false) return false;
if (other.getLaunchConfigurationName() == null ^ this.getLaunchConfigurationName() == null) return false;
if (other.getLaunchConfigurationName() != null && other.getLaunchConfigurationName().equals(this.getLaunchConfigurationName()) == false) return false;
return true;
}
}