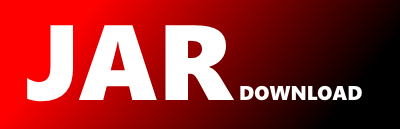
com.amazonaws.services.autoscaling.model.CreateAutoScalingGroupRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.autoscaling.model;
import java.io.Serializable;
import com.amazonaws.AmazonWebServiceRequest;
/**
* Container for the parameters to the {@link com.amazonaws.services.autoscaling.AmazonAutoScaling#createAutoScalingGroup(CreateAutoScalingGroupRequest) CreateAutoScalingGroup operation}.
*
* Creates a new Auto Scaling group with the specified name and other
* attributes. When the creation request is completed, the Auto Scaling
* group is ready to be used in other calls.
*
*
* NOTE: The Auto Scaling group name must be unique within the
* scope of your AWS account.
*
*
* @see com.amazonaws.services.autoscaling.AmazonAutoScaling#createAutoScalingGroup(CreateAutoScalingGroupRequest)
*/
public class CreateAutoScalingGroupRequest extends AmazonWebServiceRequest implements Serializable {
/**
* The name of the Auto Scaling group.
*
* Constraints:
* Length: 1 - 255
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*/
private String autoScalingGroupName;
/**
* The name of an existing launch configuration to use to launch new
* instances. Use this attribute if you want to create an Auto Scaling
* group using an existing launch configuration instead of an EC2
* instance.
*
* Constraints:
* Length: 1 - 1600
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*/
private String launchConfigurationName;
/**
* The ID of the Amazon EC2 instance you want to use to create the Auto
* Scaling group. Use this attribute if you want to create an Auto
* Scaling group using an EC2 instance instead of a launch configuration.
*
When you use an instance to create an Auto Scaling group, a new
* launch configuration is first created and then associated with the
* Auto Scaling group. The new launch configuration derives all its
* attributes from the instance that is used to create the Auto Scaling
* group, with the exception of BlockDeviceMapping
.
For
* more information, see Create
* an Auto Scaling Group Using EC2 Instance in the Auto Scaling
* Developer Guide.
*
* Constraints:
* Length: 1 - 16
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*/
private String instanceId;
/**
* The minimum size of the Auto Scaling group.
*/
private Integer minSize;
/**
* The maximum size of the Auto Scaling group.
*/
private Integer maxSize;
/**
* The number of Amazon EC2 instances that should be running in the
* group. The desired capacity must be greater than or equal to the
* minimum size and less than or equal to the maximum size specified for
* the Auto Scaling group.
*/
private Integer desiredCapacity;
/**
* The amount of time, in seconds, between a successful scaling activity
* and the succeeding scaling activity.
If a
* DefaultCooldown
period is not specified, Auto Scaling
* uses the default value of 300 as the default cool down period for the
* Auto Scaling group. For more information, see Cooldown
* Period
*/
private Integer defaultCooldown;
/**
* A list of Availability Zones for the Auto Scaling group. This is
* required unless you have specified subnets.
*
* Constraints:
* Length: 1 -
*/
private com.amazonaws.internal.ListWithAutoConstructFlag availabilityZones;
/**
* A list of existing Elastic Load Balancing load balancers to use. The
* load balancers must be associated with the AWS account. For
* information on using load balancers, see Use
* Load Balancer to Load Balance Your Auto Scaling Group in the
* Auto Scaling Developer Guide.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag loadBalancerNames;
/**
* The service you want the health checks from, Amazon EC2 or Elastic
* Load Balancer. Valid values are EC2
or ELB
.
* By default, the Auto Scaling health check uses the results of
* Amazon EC2 instance status checks to determine the health of an
* instance. For more information, see Health
* Check.
*
* Constraints:
* Length: 1 - 32
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*/
private String healthCheckType;
/**
* Length of time in seconds after a new Amazon EC2 instance comes into
* service that Auto Scaling starts checking its health. During this time
* any health check failure for the that instance is ignored.
This is
* required if you are adding ELB
health check. Frequently,
* new instances need to warm up, briefly, before they can pass a health
* check. To provide ample warm-up time, set the health check grace
* period of the group to match the expected startup period of your
* application.
*/
private Integer healthCheckGracePeriod;
/**
* Physical location of an existing cluster placement group into which
* you want to launch your instances. For information about cluster
* placement group, see Using
* Cluster Instances
*
* Constraints:
* Length: 1 - 255
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*/
private String placementGroup;
/**
* A comma-separated list of subnet identifiers of Amazon Virtual Private
* Clouds (Amazon VPCs).
If you specify subnets and Availability Zones
* with this call, ensure that the subnets' Availability Zones match the
* Availability Zones specified.
For information on launching your
* Auto Scaling group into Amazon VPC subnets, see Launch
* Auto Scaling Instances into Amazon VPC in the Auto Scaling
* Developer Guide .
*
* Constraints:
* Length: 1 - 255
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*/
private String vPCZoneIdentifier;
/**
* A standalone termination policy or a list of termination policies used
* to select the instance to terminate. The policies are executed in the
* order that they are listed.
For more information on configuring a
* termination policy for your Auto Scaling group, see Instance
* Termination Policy for Your Auto Scaling Group in the Auto
* Scaling Developer Guide.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag terminationPolicies;
/**
* The tag to be created or updated. Each tag should be defined by its
* resource type, resource ID, key, value, and a propagate flag. Valid
* values: key=value, value=value, propagate=true or
* false. Value and propagate are optional parameters. For
* information about using tags, see Tag
* Your Auto Scaling Groups and Amazon EC2 Instances in the Auto
* Scaling Developer Guide.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag tags;
/**
* The name of the Auto Scaling group.
*
* Constraints:
* Length: 1 - 255
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @return The name of the Auto Scaling group.
*/
public String getAutoScalingGroupName() {
return autoScalingGroupName;
}
/**
* The name of the Auto Scaling group.
*
* Constraints:
* Length: 1 - 255
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @param autoScalingGroupName The name of the Auto Scaling group.
*/
public void setAutoScalingGroupName(String autoScalingGroupName) {
this.autoScalingGroupName = autoScalingGroupName;
}
/**
* The name of the Auto Scaling group.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 255
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @param autoScalingGroupName The name of the Auto Scaling group.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateAutoScalingGroupRequest withAutoScalingGroupName(String autoScalingGroupName) {
this.autoScalingGroupName = autoScalingGroupName;
return this;
}
/**
* The name of an existing launch configuration to use to launch new
* instances. Use this attribute if you want to create an Auto Scaling
* group using an existing launch configuration instead of an EC2
* instance.
*
* Constraints:
* Length: 1 - 1600
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @return The name of an existing launch configuration to use to launch new
* instances. Use this attribute if you want to create an Auto Scaling
* group using an existing launch configuration instead of an EC2
* instance.
*/
public String getLaunchConfigurationName() {
return launchConfigurationName;
}
/**
* The name of an existing launch configuration to use to launch new
* instances. Use this attribute if you want to create an Auto Scaling
* group using an existing launch configuration instead of an EC2
* instance.
*
* Constraints:
* Length: 1 - 1600
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @param launchConfigurationName The name of an existing launch configuration to use to launch new
* instances. Use this attribute if you want to create an Auto Scaling
* group using an existing launch configuration instead of an EC2
* instance.
*/
public void setLaunchConfigurationName(String launchConfigurationName) {
this.launchConfigurationName = launchConfigurationName;
}
/**
* The name of an existing launch configuration to use to launch new
* instances. Use this attribute if you want to create an Auto Scaling
* group using an existing launch configuration instead of an EC2
* instance.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 1600
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @param launchConfigurationName The name of an existing launch configuration to use to launch new
* instances. Use this attribute if you want to create an Auto Scaling
* group using an existing launch configuration instead of an EC2
* instance.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateAutoScalingGroupRequest withLaunchConfigurationName(String launchConfigurationName) {
this.launchConfigurationName = launchConfigurationName;
return this;
}
/**
* The ID of the Amazon EC2 instance you want to use to create the Auto
* Scaling group. Use this attribute if you want to create an Auto
* Scaling group using an EC2 instance instead of a launch configuration.
*
When you use an instance to create an Auto Scaling group, a new
* launch configuration is first created and then associated with the
* Auto Scaling group. The new launch configuration derives all its
* attributes from the instance that is used to create the Auto Scaling
* group, with the exception of BlockDeviceMapping
.
For
* more information, see Create
* an Auto Scaling Group Using EC2 Instance in the Auto Scaling
* Developer Guide.
*
* Constraints:
* Length: 1 - 16
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @return The ID of the Amazon EC2 instance you want to use to create the Auto
* Scaling group. Use this attribute if you want to create an Auto
* Scaling group using an EC2 instance instead of a launch configuration.
*
When you use an instance to create an Auto Scaling group, a new
* launch configuration is first created and then associated with the
* Auto Scaling group. The new launch configuration derives all its
* attributes from the instance that is used to create the Auto Scaling
* group, with the exception of BlockDeviceMapping
.
For
* more information, see Create
* an Auto Scaling Group Using EC2 Instance in the Auto Scaling
* Developer Guide.
*/
public String getInstanceId() {
return instanceId;
}
/**
* The ID of the Amazon EC2 instance you want to use to create the Auto
* Scaling group. Use this attribute if you want to create an Auto
* Scaling group using an EC2 instance instead of a launch configuration.
*
When you use an instance to create an Auto Scaling group, a new
* launch configuration is first created and then associated with the
* Auto Scaling group. The new launch configuration derives all its
* attributes from the instance that is used to create the Auto Scaling
* group, with the exception of BlockDeviceMapping
.
For
* more information, see Create
* an Auto Scaling Group Using EC2 Instance in the Auto Scaling
* Developer Guide.
*
* Constraints:
* Length: 1 - 16
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @param instanceId The ID of the Amazon EC2 instance you want to use to create the Auto
* Scaling group. Use this attribute if you want to create an Auto
* Scaling group using an EC2 instance instead of a launch configuration.
*
When you use an instance to create an Auto Scaling group, a new
* launch configuration is first created and then associated with the
* Auto Scaling group. The new launch configuration derives all its
* attributes from the instance that is used to create the Auto Scaling
* group, with the exception of BlockDeviceMapping
.
For
* more information, see Create
* an Auto Scaling Group Using EC2 Instance in the Auto Scaling
* Developer Guide.
*/
public void setInstanceId(String instanceId) {
this.instanceId = instanceId;
}
/**
* The ID of the Amazon EC2 instance you want to use to create the Auto
* Scaling group. Use this attribute if you want to create an Auto
* Scaling group using an EC2 instance instead of a launch configuration.
*
When you use an instance to create an Auto Scaling group, a new
* launch configuration is first created and then associated with the
* Auto Scaling group. The new launch configuration derives all its
* attributes from the instance that is used to create the Auto Scaling
* group, with the exception of BlockDeviceMapping
.
For
* more information, see Create
* an Auto Scaling Group Using EC2 Instance in the Auto Scaling
* Developer Guide.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 16
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @param instanceId The ID of the Amazon EC2 instance you want to use to create the Auto
* Scaling group. Use this attribute if you want to create an Auto
* Scaling group using an EC2 instance instead of a launch configuration.
*
When you use an instance to create an Auto Scaling group, a new
* launch configuration is first created and then associated with the
* Auto Scaling group. The new launch configuration derives all its
* attributes from the instance that is used to create the Auto Scaling
* group, with the exception of BlockDeviceMapping
.
For
* more information, see Create
* an Auto Scaling Group Using EC2 Instance in the Auto Scaling
* Developer Guide.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateAutoScalingGroupRequest withInstanceId(String instanceId) {
this.instanceId = instanceId;
return this;
}
/**
* The minimum size of the Auto Scaling group.
*
* @return The minimum size of the Auto Scaling group.
*/
public Integer getMinSize() {
return minSize;
}
/**
* The minimum size of the Auto Scaling group.
*
* @param minSize The minimum size of the Auto Scaling group.
*/
public void setMinSize(Integer minSize) {
this.minSize = minSize;
}
/**
* The minimum size of the Auto Scaling group.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param minSize The minimum size of the Auto Scaling group.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateAutoScalingGroupRequest withMinSize(Integer minSize) {
this.minSize = minSize;
return this;
}
/**
* The maximum size of the Auto Scaling group.
*
* @return The maximum size of the Auto Scaling group.
*/
public Integer getMaxSize() {
return maxSize;
}
/**
* The maximum size of the Auto Scaling group.
*
* @param maxSize The maximum size of the Auto Scaling group.
*/
public void setMaxSize(Integer maxSize) {
this.maxSize = maxSize;
}
/**
* The maximum size of the Auto Scaling group.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param maxSize The maximum size of the Auto Scaling group.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateAutoScalingGroupRequest withMaxSize(Integer maxSize) {
this.maxSize = maxSize;
return this;
}
/**
* The number of Amazon EC2 instances that should be running in the
* group. The desired capacity must be greater than or equal to the
* minimum size and less than or equal to the maximum size specified for
* the Auto Scaling group.
*
* @return The number of Amazon EC2 instances that should be running in the
* group. The desired capacity must be greater than or equal to the
* minimum size and less than or equal to the maximum size specified for
* the Auto Scaling group.
*/
public Integer getDesiredCapacity() {
return desiredCapacity;
}
/**
* The number of Amazon EC2 instances that should be running in the
* group. The desired capacity must be greater than or equal to the
* minimum size and less than or equal to the maximum size specified for
* the Auto Scaling group.
*
* @param desiredCapacity The number of Amazon EC2 instances that should be running in the
* group. The desired capacity must be greater than or equal to the
* minimum size and less than or equal to the maximum size specified for
* the Auto Scaling group.
*/
public void setDesiredCapacity(Integer desiredCapacity) {
this.desiredCapacity = desiredCapacity;
}
/**
* The number of Amazon EC2 instances that should be running in the
* group. The desired capacity must be greater than or equal to the
* minimum size and less than or equal to the maximum size specified for
* the Auto Scaling group.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param desiredCapacity The number of Amazon EC2 instances that should be running in the
* group. The desired capacity must be greater than or equal to the
* minimum size and less than or equal to the maximum size specified for
* the Auto Scaling group.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateAutoScalingGroupRequest withDesiredCapacity(Integer desiredCapacity) {
this.desiredCapacity = desiredCapacity;
return this;
}
/**
* The amount of time, in seconds, between a successful scaling activity
* and the succeeding scaling activity.
If a
* DefaultCooldown
period is not specified, Auto Scaling
* uses the default value of 300 as the default cool down period for the
* Auto Scaling group. For more information, see Cooldown
* Period
*
* @return The amount of time, in seconds, between a successful scaling activity
* and the succeeding scaling activity.
If a
* DefaultCooldown
period is not specified, Auto Scaling
* uses the default value of 300 as the default cool down period for the
* Auto Scaling group. For more information, see Cooldown
* Period
*/
public Integer getDefaultCooldown() {
return defaultCooldown;
}
/**
* The amount of time, in seconds, between a successful scaling activity
* and the succeeding scaling activity.
If a
* DefaultCooldown
period is not specified, Auto Scaling
* uses the default value of 300 as the default cool down period for the
* Auto Scaling group. For more information, see Cooldown
* Period
*
* @param defaultCooldown The amount of time, in seconds, between a successful scaling activity
* and the succeeding scaling activity.
If a
* DefaultCooldown
period is not specified, Auto Scaling
* uses the default value of 300 as the default cool down period for the
* Auto Scaling group. For more information, see Cooldown
* Period
*/
public void setDefaultCooldown(Integer defaultCooldown) {
this.defaultCooldown = defaultCooldown;
}
/**
* The amount of time, in seconds, between a successful scaling activity
* and the succeeding scaling activity.
If a
* DefaultCooldown
period is not specified, Auto Scaling
* uses the default value of 300 as the default cool down period for the
* Auto Scaling group. For more information, see Cooldown
* Period
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param defaultCooldown The amount of time, in seconds, between a successful scaling activity
* and the succeeding scaling activity.
If a
* DefaultCooldown
period is not specified, Auto Scaling
* uses the default value of 300 as the default cool down period for the
* Auto Scaling group. For more information, see Cooldown
* Period
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateAutoScalingGroupRequest withDefaultCooldown(Integer defaultCooldown) {
this.defaultCooldown = defaultCooldown;
return this;
}
/**
* A list of Availability Zones for the Auto Scaling group. This is
* required unless you have specified subnets.
*
* Constraints:
* Length: 1 -
*
* @return A list of Availability Zones for the Auto Scaling group. This is
* required unless you have specified subnets.
*/
public java.util.List getAvailabilityZones() {
if (availabilityZones == null) {
availabilityZones = new com.amazonaws.internal.ListWithAutoConstructFlag();
availabilityZones.setAutoConstruct(true);
}
return availabilityZones;
}
/**
* A list of Availability Zones for the Auto Scaling group. This is
* required unless you have specified subnets.
*
* Constraints:
* Length: 1 -
*
* @param availabilityZones A list of Availability Zones for the Auto Scaling group. This is
* required unless you have specified subnets.
*/
public void setAvailabilityZones(java.util.Collection availabilityZones) {
if (availabilityZones == null) {
this.availabilityZones = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag availabilityZonesCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(availabilityZones.size());
availabilityZonesCopy.addAll(availabilityZones);
this.availabilityZones = availabilityZonesCopy;
}
/**
* A list of Availability Zones for the Auto Scaling group. This is
* required unless you have specified subnets.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 -
*
* @param availabilityZones A list of Availability Zones for the Auto Scaling group. This is
* required unless you have specified subnets.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateAutoScalingGroupRequest withAvailabilityZones(String... availabilityZones) {
if (getAvailabilityZones() == null) setAvailabilityZones(new java.util.ArrayList(availabilityZones.length));
for (String value : availabilityZones) {
getAvailabilityZones().add(value);
}
return this;
}
/**
* A list of Availability Zones for the Auto Scaling group. This is
* required unless you have specified subnets.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 -
*
* @param availabilityZones A list of Availability Zones for the Auto Scaling group. This is
* required unless you have specified subnets.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateAutoScalingGroupRequest withAvailabilityZones(java.util.Collection availabilityZones) {
if (availabilityZones == null) {
this.availabilityZones = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag availabilityZonesCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(availabilityZones.size());
availabilityZonesCopy.addAll(availabilityZones);
this.availabilityZones = availabilityZonesCopy;
}
return this;
}
/**
* A list of existing Elastic Load Balancing load balancers to use. The
* load balancers must be associated with the AWS account. For
* information on using load balancers, see Use
* Load Balancer to Load Balance Your Auto Scaling Group in the
* Auto Scaling Developer Guide.
*
* @return A list of existing Elastic Load Balancing load balancers to use. The
* load balancers must be associated with the AWS account.
For
* information on using load balancers, see Use
* Load Balancer to Load Balance Your Auto Scaling Group in the
* Auto Scaling Developer Guide.
*/
public java.util.List getLoadBalancerNames() {
if (loadBalancerNames == null) {
loadBalancerNames = new com.amazonaws.internal.ListWithAutoConstructFlag();
loadBalancerNames.setAutoConstruct(true);
}
return loadBalancerNames;
}
/**
* A list of existing Elastic Load Balancing load balancers to use. The
* load balancers must be associated with the AWS account. For
* information on using load balancers, see Use
* Load Balancer to Load Balance Your Auto Scaling Group in the
* Auto Scaling Developer Guide.
*
* @param loadBalancerNames A list of existing Elastic Load Balancing load balancers to use. The
* load balancers must be associated with the AWS account.
For
* information on using load balancers, see Use
* Load Balancer to Load Balance Your Auto Scaling Group in the
* Auto Scaling Developer Guide.
*/
public void setLoadBalancerNames(java.util.Collection loadBalancerNames) {
if (loadBalancerNames == null) {
this.loadBalancerNames = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag loadBalancerNamesCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(loadBalancerNames.size());
loadBalancerNamesCopy.addAll(loadBalancerNames);
this.loadBalancerNames = loadBalancerNamesCopy;
}
/**
* A list of existing Elastic Load Balancing load balancers to use. The
* load balancers must be associated with the AWS account. For
* information on using load balancers, see Use
* Load Balancer to Load Balance Your Auto Scaling Group in the
* Auto Scaling Developer Guide.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param loadBalancerNames A list of existing Elastic Load Balancing load balancers to use. The
* load balancers must be associated with the AWS account.
For
* information on using load balancers, see Use
* Load Balancer to Load Balance Your Auto Scaling Group in the
* Auto Scaling Developer Guide.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateAutoScalingGroupRequest withLoadBalancerNames(String... loadBalancerNames) {
if (getLoadBalancerNames() == null) setLoadBalancerNames(new java.util.ArrayList(loadBalancerNames.length));
for (String value : loadBalancerNames) {
getLoadBalancerNames().add(value);
}
return this;
}
/**
* A list of existing Elastic Load Balancing load balancers to use. The
* load balancers must be associated with the AWS account. For
* information on using load balancers, see Use
* Load Balancer to Load Balance Your Auto Scaling Group in the
* Auto Scaling Developer Guide.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param loadBalancerNames A list of existing Elastic Load Balancing load balancers to use. The
* load balancers must be associated with the AWS account.
For
* information on using load balancers, see Use
* Load Balancer to Load Balance Your Auto Scaling Group in the
* Auto Scaling Developer Guide.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateAutoScalingGroupRequest withLoadBalancerNames(java.util.Collection loadBalancerNames) {
if (loadBalancerNames == null) {
this.loadBalancerNames = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag loadBalancerNamesCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(loadBalancerNames.size());
loadBalancerNamesCopy.addAll(loadBalancerNames);
this.loadBalancerNames = loadBalancerNamesCopy;
}
return this;
}
/**
* The service you want the health checks from, Amazon EC2 or Elastic
* Load Balancer. Valid values are EC2
or ELB
.
* By default, the Auto Scaling health check uses the results of
* Amazon EC2 instance status checks to determine the health of an
* instance. For more information, see Health
* Check.
*
* Constraints:
* Length: 1 - 32
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @return The service you want the health checks from, Amazon EC2 or Elastic
* Load Balancer. Valid values are EC2
or ELB
.
*
By default, the Auto Scaling health check uses the results of
* Amazon EC2 instance status checks to determine the health of an
* instance. For more information, see Health
* Check.
*/
public String getHealthCheckType() {
return healthCheckType;
}
/**
* The service you want the health checks from, Amazon EC2 or Elastic
* Load Balancer. Valid values are EC2
or ELB
.
*
By default, the Auto Scaling health check uses the results of
* Amazon EC2 instance status checks to determine the health of an
* instance. For more information, see Health
* Check.
*
* Constraints:
* Length: 1 - 32
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @param healthCheckType The service you want the health checks from, Amazon EC2 or Elastic
* Load Balancer. Valid values are EC2
or ELB
.
*
By default, the Auto Scaling health check uses the results of
* Amazon EC2 instance status checks to determine the health of an
* instance. For more information, see Health
* Check.
*/
public void setHealthCheckType(String healthCheckType) {
this.healthCheckType = healthCheckType;
}
/**
* The service you want the health checks from, Amazon EC2 or Elastic
* Load Balancer. Valid values are EC2
or ELB
.
*
By default, the Auto Scaling health check uses the results of
* Amazon EC2 instance status checks to determine the health of an
* instance. For more information, see Health
* Check.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 32
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @param healthCheckType The service you want the health checks from, Amazon EC2 or Elastic
* Load Balancer. Valid values are EC2
or ELB
.
*
By default, the Auto Scaling health check uses the results of
* Amazon EC2 instance status checks to determine the health of an
* instance. For more information, see Health
* Check.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateAutoScalingGroupRequest withHealthCheckType(String healthCheckType) {
this.healthCheckType = healthCheckType;
return this;
}
/**
* Length of time in seconds after a new Amazon EC2 instance comes into
* service that Auto Scaling starts checking its health. During this time
* any health check failure for the that instance is ignored.
This is
* required if you are adding ELB
health check. Frequently,
* new instances need to warm up, briefly, before they can pass a health
* check. To provide ample warm-up time, set the health check grace
* period of the group to match the expected startup period of your
* application.
*
* @return Length of time in seconds after a new Amazon EC2 instance comes into
* service that Auto Scaling starts checking its health. During this time
* any health check failure for the that instance is ignored.
This is
* required if you are adding ELB
health check. Frequently,
* new instances need to warm up, briefly, before they can pass a health
* check. To provide ample warm-up time, set the health check grace
* period of the group to match the expected startup period of your
* application.
*/
public Integer getHealthCheckGracePeriod() {
return healthCheckGracePeriod;
}
/**
* Length of time in seconds after a new Amazon EC2 instance comes into
* service that Auto Scaling starts checking its health. During this time
* any health check failure for the that instance is ignored.
This is
* required if you are adding ELB
health check. Frequently,
* new instances need to warm up, briefly, before they can pass a health
* check. To provide ample warm-up time, set the health check grace
* period of the group to match the expected startup period of your
* application.
*
* @param healthCheckGracePeriod Length of time in seconds after a new Amazon EC2 instance comes into
* service that Auto Scaling starts checking its health. During this time
* any health check failure for the that instance is ignored.
This is
* required if you are adding ELB
health check. Frequently,
* new instances need to warm up, briefly, before they can pass a health
* check. To provide ample warm-up time, set the health check grace
* period of the group to match the expected startup period of your
* application.
*/
public void setHealthCheckGracePeriod(Integer healthCheckGracePeriod) {
this.healthCheckGracePeriod = healthCheckGracePeriod;
}
/**
* Length of time in seconds after a new Amazon EC2 instance comes into
* service that Auto Scaling starts checking its health. During this time
* any health check failure for the that instance is ignored.
This is
* required if you are adding ELB
health check. Frequently,
* new instances need to warm up, briefly, before they can pass a health
* check. To provide ample warm-up time, set the health check grace
* period of the group to match the expected startup period of your
* application.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param healthCheckGracePeriod Length of time in seconds after a new Amazon EC2 instance comes into
* service that Auto Scaling starts checking its health. During this time
* any health check failure for the that instance is ignored.
This is
* required if you are adding ELB
health check. Frequently,
* new instances need to warm up, briefly, before they can pass a health
* check. To provide ample warm-up time, set the health check grace
* period of the group to match the expected startup period of your
* application.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateAutoScalingGroupRequest withHealthCheckGracePeriod(Integer healthCheckGracePeriod) {
this.healthCheckGracePeriod = healthCheckGracePeriod;
return this;
}
/**
* Physical location of an existing cluster placement group into which
* you want to launch your instances. For information about cluster
* placement group, see Using
* Cluster Instances
*
* Constraints:
* Length: 1 - 255
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @return Physical location of an existing cluster placement group into which
* you want to launch your instances. For information about cluster
* placement group, see Using
* Cluster Instances
*/
public String getPlacementGroup() {
return placementGroup;
}
/**
* Physical location of an existing cluster placement group into which
* you want to launch your instances. For information about cluster
* placement group, see Using
* Cluster Instances
*
* Constraints:
* Length: 1 - 255
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @param placementGroup Physical location of an existing cluster placement group into which
* you want to launch your instances. For information about cluster
* placement group, see Using
* Cluster Instances
*/
public void setPlacementGroup(String placementGroup) {
this.placementGroup = placementGroup;
}
/**
* Physical location of an existing cluster placement group into which
* you want to launch your instances. For information about cluster
* placement group, see Using
* Cluster Instances
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 255
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @param placementGroup Physical location of an existing cluster placement group into which
* you want to launch your instances. For information about cluster
* placement group, see Using
* Cluster Instances
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateAutoScalingGroupRequest withPlacementGroup(String placementGroup) {
this.placementGroup = placementGroup;
return this;
}
/**
* A comma-separated list of subnet identifiers of Amazon Virtual Private
* Clouds (Amazon VPCs).
If you specify subnets and Availability Zones
* with this call, ensure that the subnets' Availability Zones match the
* Availability Zones specified.
For information on launching your
* Auto Scaling group into Amazon VPC subnets, see Launch
* Auto Scaling Instances into Amazon VPC in the Auto Scaling
* Developer Guide .
*
* Constraints:
* Length: 1 - 255
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @return A comma-separated list of subnet identifiers of Amazon Virtual Private
* Clouds (Amazon VPCs).
If you specify subnets and Availability Zones
* with this call, ensure that the subnets' Availability Zones match the
* Availability Zones specified.
For information on launching your
* Auto Scaling group into Amazon VPC subnets, see Launch
* Auto Scaling Instances into Amazon VPC in the Auto Scaling
* Developer Guide .
*/
public String getVPCZoneIdentifier() {
return vPCZoneIdentifier;
}
/**
* A comma-separated list of subnet identifiers of Amazon Virtual Private
* Clouds (Amazon VPCs).
If you specify subnets and Availability Zones
* with this call, ensure that the subnets' Availability Zones match the
* Availability Zones specified.
For information on launching your
* Auto Scaling group into Amazon VPC subnets, see Launch
* Auto Scaling Instances into Amazon VPC in the Auto Scaling
* Developer Guide .
*
* Constraints:
* Length: 1 - 255
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @param vPCZoneIdentifier A comma-separated list of subnet identifiers of Amazon Virtual Private
* Clouds (Amazon VPCs).
If you specify subnets and Availability Zones
* with this call, ensure that the subnets' Availability Zones match the
* Availability Zones specified.
For information on launching your
* Auto Scaling group into Amazon VPC subnets, see Launch
* Auto Scaling Instances into Amazon VPC in the Auto Scaling
* Developer Guide .
*/
public void setVPCZoneIdentifier(String vPCZoneIdentifier) {
this.vPCZoneIdentifier = vPCZoneIdentifier;
}
/**
* A comma-separated list of subnet identifiers of Amazon Virtual Private
* Clouds (Amazon VPCs).
If you specify subnets and Availability Zones
* with this call, ensure that the subnets' Availability Zones match the
* Availability Zones specified.
For information on launching your
* Auto Scaling group into Amazon VPC subnets, see Launch
* Auto Scaling Instances into Amazon VPC in the Auto Scaling
* Developer Guide .
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 255
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @param vPCZoneIdentifier A comma-separated list of subnet identifiers of Amazon Virtual Private
* Clouds (Amazon VPCs).
If you specify subnets and Availability Zones
* with this call, ensure that the subnets' Availability Zones match the
* Availability Zones specified.
For information on launching your
* Auto Scaling group into Amazon VPC subnets, see Launch
* Auto Scaling Instances into Amazon VPC in the Auto Scaling
* Developer Guide .
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateAutoScalingGroupRequest withVPCZoneIdentifier(String vPCZoneIdentifier) {
this.vPCZoneIdentifier = vPCZoneIdentifier;
return this;
}
/**
* A standalone termination policy or a list of termination policies used
* to select the instance to terminate. The policies are executed in the
* order that they are listed.
For more information on configuring a
* termination policy for your Auto Scaling group, see Instance
* Termination Policy for Your Auto Scaling Group in the Auto
* Scaling Developer Guide.
*
* @return A standalone termination policy or a list of termination policies used
* to select the instance to terminate. The policies are executed in the
* order that they are listed.
For more information on configuring a
* termination policy for your Auto Scaling group, see Instance
* Termination Policy for Your Auto Scaling Group in the Auto
* Scaling Developer Guide.
*/
public java.util.List getTerminationPolicies() {
if (terminationPolicies == null) {
terminationPolicies = new com.amazonaws.internal.ListWithAutoConstructFlag();
terminationPolicies.setAutoConstruct(true);
}
return terminationPolicies;
}
/**
* A standalone termination policy or a list of termination policies used
* to select the instance to terminate. The policies are executed in the
* order that they are listed. For more information on configuring a
* termination policy for your Auto Scaling group, see Instance
* Termination Policy for Your Auto Scaling Group in the Auto
* Scaling Developer Guide.
*
* @param terminationPolicies A standalone termination policy or a list of termination policies used
* to select the instance to terminate. The policies are executed in the
* order that they are listed.
For more information on configuring a
* termination policy for your Auto Scaling group, see Instance
* Termination Policy for Your Auto Scaling Group in the Auto
* Scaling Developer Guide.
*/
public void setTerminationPolicies(java.util.Collection terminationPolicies) {
if (terminationPolicies == null) {
this.terminationPolicies = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag terminationPoliciesCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(terminationPolicies.size());
terminationPoliciesCopy.addAll(terminationPolicies);
this.terminationPolicies = terminationPoliciesCopy;
}
/**
* A standalone termination policy or a list of termination policies used
* to select the instance to terminate. The policies are executed in the
* order that they are listed. For more information on configuring a
* termination policy for your Auto Scaling group, see Instance
* Termination Policy for Your Auto Scaling Group in the Auto
* Scaling Developer Guide.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param terminationPolicies A standalone termination policy or a list of termination policies used
* to select the instance to terminate. The policies are executed in the
* order that they are listed.
For more information on configuring a
* termination policy for your Auto Scaling group, see Instance
* Termination Policy for Your Auto Scaling Group in the Auto
* Scaling Developer Guide.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateAutoScalingGroupRequest withTerminationPolicies(String... terminationPolicies) {
if (getTerminationPolicies() == null) setTerminationPolicies(new java.util.ArrayList(terminationPolicies.length));
for (String value : terminationPolicies) {
getTerminationPolicies().add(value);
}
return this;
}
/**
* A standalone termination policy or a list of termination policies used
* to select the instance to terminate. The policies are executed in the
* order that they are listed. For more information on configuring a
* termination policy for your Auto Scaling group, see Instance
* Termination Policy for Your Auto Scaling Group in the Auto
* Scaling Developer Guide.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param terminationPolicies A standalone termination policy or a list of termination policies used
* to select the instance to terminate. The policies are executed in the
* order that they are listed.
For more information on configuring a
* termination policy for your Auto Scaling group, see Instance
* Termination Policy for Your Auto Scaling Group in the Auto
* Scaling Developer Guide.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateAutoScalingGroupRequest withTerminationPolicies(java.util.Collection terminationPolicies) {
if (terminationPolicies == null) {
this.terminationPolicies = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag terminationPoliciesCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(terminationPolicies.size());
terminationPoliciesCopy.addAll(terminationPolicies);
this.terminationPolicies = terminationPoliciesCopy;
}
return this;
}
/**
* The tag to be created or updated. Each tag should be defined by its
* resource type, resource ID, key, value, and a propagate flag. Valid
* values: key=value, value=value, propagate=true or
* false. Value and propagate are optional parameters. For
* information about using tags, see Tag
* Your Auto Scaling Groups and Amazon EC2 Instances in the Auto
* Scaling Developer Guide.
*
* @return The tag to be created or updated. Each tag should be defined by its
* resource type, resource ID, key, value, and a propagate flag. Valid
* values: key=value, value=value, propagate=true or
* false. Value and propagate are optional parameters.
For
* information about using tags, see Tag
* Your Auto Scaling Groups and Amazon EC2 Instances in the Auto
* Scaling Developer Guide.
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.ListWithAutoConstructFlag();
tags.setAutoConstruct(true);
}
return tags;
}
/**
* The tag to be created or updated. Each tag should be defined by its
* resource type, resource ID, key, value, and a propagate flag. Valid
* values: key=value, value=value, propagate=true or
* false. Value and propagate are optional parameters. For
* information about using tags, see Tag
* Your Auto Scaling Groups and Amazon EC2 Instances in the Auto
* Scaling Developer Guide.
*
* @param tags The tag to be created or updated. Each tag should be defined by its
* resource type, resource ID, key, value, and a propagate flag. Valid
* values: key=value, value=value, propagate=true or
* false. Value and propagate are optional parameters.
For
* information about using tags, see Tag
* Your Auto Scaling Groups and Amazon EC2 Instances in the Auto
* Scaling Developer Guide.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag tagsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(tags.size());
tagsCopy.addAll(tags);
this.tags = tagsCopy;
}
/**
* The tag to be created or updated. Each tag should be defined by its
* resource type, resource ID, key, value, and a propagate flag. Valid
* values: key=value, value=value, propagate=true or
* false. Value and propagate are optional parameters. For
* information about using tags, see Tag
* Your Auto Scaling Groups and Amazon EC2 Instances in the Auto
* Scaling Developer Guide.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param tags The tag to be created or updated. Each tag should be defined by its
* resource type, resource ID, key, value, and a propagate flag. Valid
* values: key=value, value=value, propagate=true or
* false. Value and propagate are optional parameters.
For
* information about using tags, see Tag
* Your Auto Scaling Groups and Amazon EC2 Instances in the Auto
* Scaling Developer Guide.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateAutoScalingGroupRequest withTags(Tag... tags) {
if (getTags() == null) setTags(new java.util.ArrayList(tags.length));
for (Tag value : tags) {
getTags().add(value);
}
return this;
}
/**
* The tag to be created or updated. Each tag should be defined by its
* resource type, resource ID, key, value, and a propagate flag. Valid
* values: key=value, value=value, propagate=true or
* false. Value and propagate are optional parameters. For
* information about using tags, see Tag
* Your Auto Scaling Groups and Amazon EC2 Instances in the Auto
* Scaling Developer Guide.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param tags The tag to be created or updated. Each tag should be defined by its
* resource type, resource ID, key, value, and a propagate flag. Valid
* values: key=value, value=value, propagate=true or
* false. Value and propagate are optional parameters.
For
* information about using tags, see Tag
* Your Auto Scaling Groups and Amazon EC2 Instances in the Auto
* Scaling Developer Guide.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateAutoScalingGroupRequest withTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag tagsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(tags.size());
tagsCopy.addAll(tags);
this.tags = tagsCopy;
}
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAutoScalingGroupName() != null) sb.append("AutoScalingGroupName: " + getAutoScalingGroupName() + ",");
if (getLaunchConfigurationName() != null) sb.append("LaunchConfigurationName: " + getLaunchConfigurationName() + ",");
if (getInstanceId() != null) sb.append("InstanceId: " + getInstanceId() + ",");
if (getMinSize() != null) sb.append("MinSize: " + getMinSize() + ",");
if (getMaxSize() != null) sb.append("MaxSize: " + getMaxSize() + ",");
if (getDesiredCapacity() != null) sb.append("DesiredCapacity: " + getDesiredCapacity() + ",");
if (getDefaultCooldown() != null) sb.append("DefaultCooldown: " + getDefaultCooldown() + ",");
if (getAvailabilityZones() != null) sb.append("AvailabilityZones: " + getAvailabilityZones() + ",");
if (getLoadBalancerNames() != null) sb.append("LoadBalancerNames: " + getLoadBalancerNames() + ",");
if (getHealthCheckType() != null) sb.append("HealthCheckType: " + getHealthCheckType() + ",");
if (getHealthCheckGracePeriod() != null) sb.append("HealthCheckGracePeriod: " + getHealthCheckGracePeriod() + ",");
if (getPlacementGroup() != null) sb.append("PlacementGroup: " + getPlacementGroup() + ",");
if (getVPCZoneIdentifier() != null) sb.append("VPCZoneIdentifier: " + getVPCZoneIdentifier() + ",");
if (getTerminationPolicies() != null) sb.append("TerminationPolicies: " + getTerminationPolicies() + ",");
if (getTags() != null) sb.append("Tags: " + getTags() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAutoScalingGroupName() == null) ? 0 : getAutoScalingGroupName().hashCode());
hashCode = prime * hashCode + ((getLaunchConfigurationName() == null) ? 0 : getLaunchConfigurationName().hashCode());
hashCode = prime * hashCode + ((getInstanceId() == null) ? 0 : getInstanceId().hashCode());
hashCode = prime * hashCode + ((getMinSize() == null) ? 0 : getMinSize().hashCode());
hashCode = prime * hashCode + ((getMaxSize() == null) ? 0 : getMaxSize().hashCode());
hashCode = prime * hashCode + ((getDesiredCapacity() == null) ? 0 : getDesiredCapacity().hashCode());
hashCode = prime * hashCode + ((getDefaultCooldown() == null) ? 0 : getDefaultCooldown().hashCode());
hashCode = prime * hashCode + ((getAvailabilityZones() == null) ? 0 : getAvailabilityZones().hashCode());
hashCode = prime * hashCode + ((getLoadBalancerNames() == null) ? 0 : getLoadBalancerNames().hashCode());
hashCode = prime * hashCode + ((getHealthCheckType() == null) ? 0 : getHealthCheckType().hashCode());
hashCode = prime * hashCode + ((getHealthCheckGracePeriod() == null) ? 0 : getHealthCheckGracePeriod().hashCode());
hashCode = prime * hashCode + ((getPlacementGroup() == null) ? 0 : getPlacementGroup().hashCode());
hashCode = prime * hashCode + ((getVPCZoneIdentifier() == null) ? 0 : getVPCZoneIdentifier().hashCode());
hashCode = prime * hashCode + ((getTerminationPolicies() == null) ? 0 : getTerminationPolicies().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof CreateAutoScalingGroupRequest == false) return false;
CreateAutoScalingGroupRequest other = (CreateAutoScalingGroupRequest)obj;
if (other.getAutoScalingGroupName() == null ^ this.getAutoScalingGroupName() == null) return false;
if (other.getAutoScalingGroupName() != null && other.getAutoScalingGroupName().equals(this.getAutoScalingGroupName()) == false) return false;
if (other.getLaunchConfigurationName() == null ^ this.getLaunchConfigurationName() == null) return false;
if (other.getLaunchConfigurationName() != null && other.getLaunchConfigurationName().equals(this.getLaunchConfigurationName()) == false) return false;
if (other.getInstanceId() == null ^ this.getInstanceId() == null) return false;
if (other.getInstanceId() != null && other.getInstanceId().equals(this.getInstanceId()) == false) return false;
if (other.getMinSize() == null ^ this.getMinSize() == null) return false;
if (other.getMinSize() != null && other.getMinSize().equals(this.getMinSize()) == false) return false;
if (other.getMaxSize() == null ^ this.getMaxSize() == null) return false;
if (other.getMaxSize() != null && other.getMaxSize().equals(this.getMaxSize()) == false) return false;
if (other.getDesiredCapacity() == null ^ this.getDesiredCapacity() == null) return false;
if (other.getDesiredCapacity() != null && other.getDesiredCapacity().equals(this.getDesiredCapacity()) == false) return false;
if (other.getDefaultCooldown() == null ^ this.getDefaultCooldown() == null) return false;
if (other.getDefaultCooldown() != null && other.getDefaultCooldown().equals(this.getDefaultCooldown()) == false) return false;
if (other.getAvailabilityZones() == null ^ this.getAvailabilityZones() == null) return false;
if (other.getAvailabilityZones() != null && other.getAvailabilityZones().equals(this.getAvailabilityZones()) == false) return false;
if (other.getLoadBalancerNames() == null ^ this.getLoadBalancerNames() == null) return false;
if (other.getLoadBalancerNames() != null && other.getLoadBalancerNames().equals(this.getLoadBalancerNames()) == false) return false;
if (other.getHealthCheckType() == null ^ this.getHealthCheckType() == null) return false;
if (other.getHealthCheckType() != null && other.getHealthCheckType().equals(this.getHealthCheckType()) == false) return false;
if (other.getHealthCheckGracePeriod() == null ^ this.getHealthCheckGracePeriod() == null) return false;
if (other.getHealthCheckGracePeriod() != null && other.getHealthCheckGracePeriod().equals(this.getHealthCheckGracePeriod()) == false) return false;
if (other.getPlacementGroup() == null ^ this.getPlacementGroup() == null) return false;
if (other.getPlacementGroup() != null && other.getPlacementGroup().equals(this.getPlacementGroup()) == false) return false;
if (other.getVPCZoneIdentifier() == null ^ this.getVPCZoneIdentifier() == null) return false;
if (other.getVPCZoneIdentifier() != null && other.getVPCZoneIdentifier().equals(this.getVPCZoneIdentifier()) == false) return false;
if (other.getTerminationPolicies() == null ^ this.getTerminationPolicies() == null) return false;
if (other.getTerminationPolicies() != null && other.getTerminationPolicies().equals(this.getTerminationPolicies()) == false) return false;
if (other.getTags() == null ^ this.getTags() == null) return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false) return false;
return true;
}
}