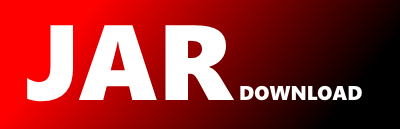
com.amazonaws.services.autoscaling.model.Ebs Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.autoscaling.model;
import java.io.Serializable;
/**
*
* The Ebs data type.
*
*/
public class Ebs implements Serializable {
/**
* The snapshot ID.
*
* Constraints:
* Length: 1 - 255
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*/
private String snapshotId;
/**
* The volume size, in gigabytes.
Valid values: If the volume type is
* io1
, the minimum size of the volume is 10.
Default: If
* you're creating the volume from a snapshot, and you don't specify a
* volume size, the default is the snapshot size.
Required: Required
* when the volume type is io1
.
*
* Constraints:
* Range: 1 - 1024
*/
private Integer volumeSize;
/**
* The volume type.
Valid values: standard | io1
*
Default: standard
*
* Constraints:
* Length: 1 - 255
*/
private String volumeType;
/**
* Indicates whether to delete the volume on instance termination.
*
Default: true
*/
private Boolean deleteOnTermination;
/**
* The number of I/O operations per second (IOPS) that the volume
* supports.
The maximum ratio of IOPS to volume size is 30.0
Valid
* Values: Range is 100 to 4000.
Default: None.
*
* Constraints:
* Range: 100 - 4000
*/
private Integer iops;
/**
* The snapshot ID.
*
* Constraints:
* Length: 1 - 255
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @return The snapshot ID.
*/
public String getSnapshotId() {
return snapshotId;
}
/**
* The snapshot ID.
*
* Constraints:
* Length: 1 - 255
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @param snapshotId The snapshot ID.
*/
public void setSnapshotId(String snapshotId) {
this.snapshotId = snapshotId;
}
/**
* The snapshot ID.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 255
* Pattern: [\u0020-\uD7FF\uE000-\uFFFD\uD800\uDC00-\uDBFF\uDFFF\r\n\t]*
*
* @param snapshotId The snapshot ID.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Ebs withSnapshotId(String snapshotId) {
this.snapshotId = snapshotId;
return this;
}
/**
* The volume size, in gigabytes.
Valid values: If the volume type is
* io1
, the minimum size of the volume is 10.
Default: If
* you're creating the volume from a snapshot, and you don't specify a
* volume size, the default is the snapshot size.
Required: Required
* when the volume type is io1
.
*
* Constraints:
* Range: 1 - 1024
*
* @return The volume size, in gigabytes.
Valid values: If the volume type is
* io1
, the minimum size of the volume is 10.
Default: If
* you're creating the volume from a snapshot, and you don't specify a
* volume size, the default is the snapshot size.
Required: Required
* when the volume type is io1
.
*/
public Integer getVolumeSize() {
return volumeSize;
}
/**
* The volume size, in gigabytes.
Valid values: If the volume type is
* io1
, the minimum size of the volume is 10.
Default: If
* you're creating the volume from a snapshot, and you don't specify a
* volume size, the default is the snapshot size.
Required: Required
* when the volume type is io1
.
*
* Constraints:
* Range: 1 - 1024
*
* @param volumeSize The volume size, in gigabytes.
Valid values: If the volume type is
* io1
, the minimum size of the volume is 10.
Default: If
* you're creating the volume from a snapshot, and you don't specify a
* volume size, the default is the snapshot size.
Required: Required
* when the volume type is io1
.
*/
public void setVolumeSize(Integer volumeSize) {
this.volumeSize = volumeSize;
}
/**
* The volume size, in gigabytes.
Valid values: If the volume type is
* io1
, the minimum size of the volume is 10.
Default: If
* you're creating the volume from a snapshot, and you don't specify a
* volume size, the default is the snapshot size.
Required: Required
* when the volume type is io1
.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Range: 1 - 1024
*
* @param volumeSize The volume size, in gigabytes.
Valid values: If the volume type is
* io1
, the minimum size of the volume is 10.
Default: If
* you're creating the volume from a snapshot, and you don't specify a
* volume size, the default is the snapshot size.
Required: Required
* when the volume type is io1
.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Ebs withVolumeSize(Integer volumeSize) {
this.volumeSize = volumeSize;
return this;
}
/**
* The volume type.
Valid values: standard | io1
*
Default: standard
*
* Constraints:
* Length: 1 - 255
*
* @return The volume type.
Valid values: standard | io1
*
Default: standard
*/
public String getVolumeType() {
return volumeType;
}
/**
* The volume type.
Valid values: standard | io1
*
Default: standard
*
* Constraints:
* Length: 1 - 255
*
* @param volumeType The volume type.
Valid values: standard | io1
*
Default: standard
*/
public void setVolumeType(String volumeType) {
this.volumeType = volumeType;
}
/**
* The volume type.
Valid values: standard | io1
*
Default: standard
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 255
*
* @param volumeType The volume type.
Valid values: standard | io1
*
Default: standard
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Ebs withVolumeType(String volumeType) {
this.volumeType = volumeType;
return this;
}
/**
* Indicates whether to delete the volume on instance termination.
*
Default: true
*
* @return Indicates whether to delete the volume on instance termination.
*
Default: true
*/
public Boolean isDeleteOnTermination() {
return deleteOnTermination;
}
/**
* Indicates whether to delete the volume on instance termination.
*
Default: true
*
* @param deleteOnTermination Indicates whether to delete the volume on instance termination.
*
Default: true
*/
public void setDeleteOnTermination(Boolean deleteOnTermination) {
this.deleteOnTermination = deleteOnTermination;
}
/**
* Indicates whether to delete the volume on instance termination.
*
Default: true
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param deleteOnTermination Indicates whether to delete the volume on instance termination.
*
Default: true
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Ebs withDeleteOnTermination(Boolean deleteOnTermination) {
this.deleteOnTermination = deleteOnTermination;
return this;
}
/**
* Indicates whether to delete the volume on instance termination.
*
Default: true
*
* @return Indicates whether to delete the volume on instance termination.
*
Default: true
*/
public Boolean getDeleteOnTermination() {
return deleteOnTermination;
}
/**
* The number of I/O operations per second (IOPS) that the volume
* supports.
The maximum ratio of IOPS to volume size is 30.0
Valid
* Values: Range is 100 to 4000.
Default: None.
*
* Constraints:
* Range: 100 - 4000
*
* @return The number of I/O operations per second (IOPS) that the volume
* supports.
The maximum ratio of IOPS to volume size is 30.0
Valid
* Values: Range is 100 to 4000.
Default: None.
*/
public Integer getIops() {
return iops;
}
/**
* The number of I/O operations per second (IOPS) that the volume
* supports.
The maximum ratio of IOPS to volume size is 30.0
Valid
* Values: Range is 100 to 4000.
Default: None.
*
* Constraints:
* Range: 100 - 4000
*
* @param iops The number of I/O operations per second (IOPS) that the volume
* supports.
The maximum ratio of IOPS to volume size is 30.0
Valid
* Values: Range is 100 to 4000.
Default: None.
*/
public void setIops(Integer iops) {
this.iops = iops;
}
/**
* The number of I/O operations per second (IOPS) that the volume
* supports.
The maximum ratio of IOPS to volume size is 30.0
Valid
* Values: Range is 100 to 4000.
Default: None.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Range: 100 - 4000
*
* @param iops The number of I/O operations per second (IOPS) that the volume
* supports.
The maximum ratio of IOPS to volume size is 30.0
Valid
* Values: Range is 100 to 4000.
Default: None.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Ebs withIops(Integer iops) {
this.iops = iops;
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getSnapshotId() != null) sb.append("SnapshotId: " + getSnapshotId() + ",");
if (getVolumeSize() != null) sb.append("VolumeSize: " + getVolumeSize() + ",");
if (getVolumeType() != null) sb.append("VolumeType: " + getVolumeType() + ",");
if (isDeleteOnTermination() != null) sb.append("DeleteOnTermination: " + isDeleteOnTermination() + ",");
if (getIops() != null) sb.append("Iops: " + getIops() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getSnapshotId() == null) ? 0 : getSnapshotId().hashCode());
hashCode = prime * hashCode + ((getVolumeSize() == null) ? 0 : getVolumeSize().hashCode());
hashCode = prime * hashCode + ((getVolumeType() == null) ? 0 : getVolumeType().hashCode());
hashCode = prime * hashCode + ((isDeleteOnTermination() == null) ? 0 : isDeleteOnTermination().hashCode());
hashCode = prime * hashCode + ((getIops() == null) ? 0 : getIops().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof Ebs == false) return false;
Ebs other = (Ebs)obj;
if (other.getSnapshotId() == null ^ this.getSnapshotId() == null) return false;
if (other.getSnapshotId() != null && other.getSnapshotId().equals(this.getSnapshotId()) == false) return false;
if (other.getVolumeSize() == null ^ this.getVolumeSize() == null) return false;
if (other.getVolumeSize() != null && other.getVolumeSize().equals(this.getVolumeSize()) == false) return false;
if (other.getVolumeType() == null ^ this.getVolumeType() == null) return false;
if (other.getVolumeType() != null && other.getVolumeType().equals(this.getVolumeType()) == false) return false;
if (other.isDeleteOnTermination() == null ^ this.isDeleteOnTermination() == null) return false;
if (other.isDeleteOnTermination() != null && other.isDeleteOnTermination().equals(this.isDeleteOnTermination()) == false) return false;
if (other.getIops() == null ^ this.getIops() == null) return false;
if (other.getIops() != null && other.getIops().equals(this.getIops()) == false) return false;
return true;
}
}