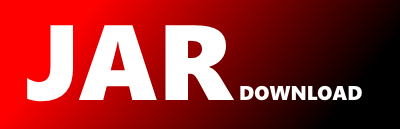
com.amazonaws.services.cloudformation.AmazonCloudFormationAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.cloudformation;
import java.util.concurrent.Future;
import com.amazonaws.AmazonClientException;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.handlers.AsyncHandler;
import com.amazonaws.services.cloudformation.model.*;
/**
* Interface for accessing AmazonCloudFormation asynchronously.
* Each asynchronous method will return a Java Future object, and users are also allowed
* to provide a callback handler.
* AWS CloudFormation
* AWS CloudFormation enables you to create and manage AWS infrastructure
* deployments predictably and repeatedly. AWS CloudFormation helps you
* leverage AWS products such as Amazon EC2, EBS, Amazon SNS, ELB, and
* Auto Scaling to build highly-reliable, highly scalable, cost effective
* applications without worrying about creating and configuring the
* underlying AWS infrastructure.
*
*
* With AWS CloudFormation, you declare all of your resources and
* dependencies in a template file. The template defines a collection of
* resources as a single unit called a stack. AWS CloudFormation creates
* and deletes all member resources of the stack together and manages all
* dependencies between the resources for you.
*
*
* For more information about this product, go to the
* CloudFormation Product Page
* .
*
*
* Amazon CloudFormation makes use of other AWS products. If you need
* additional technical information about a specific AWS product, you can
* find the product's technical documentation at
* http://aws.amazon.com/documentation/
* .
*
*/
public interface AmazonCloudFormationAsync extends AmazonCloudFormation {
/**
*
* Validates a specified template.
*
*
* @param validateTemplateRequest Container for the necessary parameters
* to execute the ValidateTemplate operation on AmazonCloudFormation.
*
* @return A Java Future object containing the response from the
* ValidateTemplate service method, as returned by AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future validateTemplateAsync(ValidateTemplateRequest validateTemplateRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Validates a specified template.
*
*
* @param validateTemplateRequest Container for the necessary parameters
* to execute the ValidateTemplate operation on AmazonCloudFormation.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ValidateTemplate service method, as returned by AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future validateTemplateAsync(ValidateTemplateRequest validateTemplateRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns the description for the specified stack; if no stack name was
* specified, then it returns the description for all the stacks created.
*
*
* @param describeStacksRequest Container for the necessary parameters to
* execute the DescribeStacks operation on AmazonCloudFormation.
*
* @return A Java Future object containing the response from the
* DescribeStacks service method, as returned by AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeStacksAsync(DescribeStacksRequest describeStacksRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns the description for the specified stack; if no stack name was
* specified, then it returns the description for all the stacks created.
*
*
* @param describeStacksRequest Container for the necessary parameters to
* execute the DescribeStacks operation on AmazonCloudFormation.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeStacks service method, as returned by AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeStacksAsync(DescribeStacksRequest describeStacksRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns the template body for a specified stack. You can get the
* template for running or deleted stacks.
*
*
* For deleted stacks, GetTemplate returns the template for up to 90 days
* after the stack has been deleted.
*
*
* NOTE: If the template does not exist, a ValidationError is
* returned.
*
*
* @param getTemplateRequest Container for the necessary parameters to
* execute the GetTemplate operation on AmazonCloudFormation.
*
* @return A Java Future object containing the response from the
* GetTemplate service method, as returned by AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getTemplateAsync(GetTemplateRequest getTemplateRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns the template body for a specified stack. You can get the
* template for running or deleted stacks.
*
*
* For deleted stacks, GetTemplate returns the template for up to 90 days
* after the stack has been deleted.
*
*
* NOTE: If the template does not exist, a ValidationError is
* returned.
*
*
* @param getTemplateRequest Container for the necessary parameters to
* execute the GetTemplate operation on AmazonCloudFormation.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* GetTemplate service method, as returned by AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getTemplateAsync(GetTemplateRequest getTemplateRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns the stack policy for a specified stack. If a stack doesn't
* have a policy, a null value is returned.
*
*
* @param getStackPolicyRequest Container for the necessary parameters to
* execute the GetStackPolicy operation on AmazonCloudFormation.
*
* @return A Java Future object containing the response from the
* GetStackPolicy service method, as returned by AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getStackPolicyAsync(GetStackPolicyRequest getStackPolicyRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns the stack policy for a specified stack. If a stack doesn't
* have a policy, a null value is returned.
*
*
* @param getStackPolicyRequest Container for the necessary parameters to
* execute the GetStackPolicy operation on AmazonCloudFormation.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* GetStackPolicy service method, as returned by AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getStackPolicyAsync(GetStackPolicyRequest getStackPolicyRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns the summary information for stacks whose status matches the
* specified StackStatusFilter. Summary information for stacks that have
* been deleted is kept for 90 days after the stack is deleted. If no
* StackStatusFilter is specified, summary information for all stacks is
* returned (including existing stacks and stacks that have been
* deleted).
*
*
* @param listStacksRequest Container for the necessary parameters to
* execute the ListStacks operation on AmazonCloudFormation.
*
* @return A Java Future object containing the response from the
* ListStacks service method, as returned by AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listStacksAsync(ListStacksRequest listStacksRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns the summary information for stacks whose status matches the
* specified StackStatusFilter. Summary information for stacks that have
* been deleted is kept for 90 days after the stack is deleted. If no
* StackStatusFilter is specified, summary information for all stacks is
* returned (including existing stacks and stacks that have been
* deleted).
*
*
* @param listStacksRequest Container for the necessary parameters to
* execute the ListStacks operation on AmazonCloudFormation.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ListStacks service method, as returned by AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listStacksAsync(ListStacksRequest listStacksRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates a stack as specified in the template. After the call completes
* successfully, the stack creation starts. You can check the status of
* the stack via the DescribeStacks API.
*
*
* @param createStackRequest Container for the necessary parameters to
* execute the CreateStack operation on AmazonCloudFormation.
*
* @return A Java Future object containing the response from the
* CreateStack service method, as returned by AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createStackAsync(CreateStackRequest createStackRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates a stack as specified in the template. After the call completes
* successfully, the stack creation starts. You can check the status of
* the stack via the DescribeStacks API.
*
*
* @param createStackRequest Container for the necessary parameters to
* execute the CreateStack operation on AmazonCloudFormation.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateStack service method, as returned by AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createStackAsync(CreateStackRequest createStackRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Sets a stack policy for a specified stack.
*
*
* @param setStackPolicyRequest Container for the necessary parameters to
* execute the SetStackPolicy operation on AmazonCloudFormation.
*
* @return A Java Future object containing the response from the
* SetStackPolicy service method, as returned by AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future setStackPolicyAsync(SetStackPolicyRequest setStackPolicyRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Sets a stack policy for a specified stack.
*
*
* @param setStackPolicyRequest Container for the necessary parameters to
* execute the SetStackPolicy operation on AmazonCloudFormation.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* SetStackPolicy service method, as returned by AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future setStackPolicyAsync(SetStackPolicyRequest setStackPolicyRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns the estimated monthly cost of a template. The return value is
* an AWS Simple Monthly Calculator URL with a query string that
* describes the resources required to run the template.
*
*
* @param estimateTemplateCostRequest Container for the necessary
* parameters to execute the EstimateTemplateCost operation on
* AmazonCloudFormation.
*
* @return A Java Future object containing the response from the
* EstimateTemplateCost service method, as returned by
* AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future estimateTemplateCostAsync(EstimateTemplateCostRequest estimateTemplateCostRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns the estimated monthly cost of a template. The return value is
* an AWS Simple Monthly Calculator URL with a query string that
* describes the resources required to run the template.
*
*
* @param estimateTemplateCostRequest Container for the necessary
* parameters to execute the EstimateTemplateCost operation on
* AmazonCloudFormation.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* EstimateTemplateCost service method, as returned by
* AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future estimateTemplateCostAsync(EstimateTemplateCostRequest estimateTemplateCostRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns all stack related events for a specified stack. For more
* information about a stack's event history, go to
* Stacks
* in the AWS CloudFormation User Guide.
*
*
* NOTE:Events are returned, even if the stack never existed or
* has been successfully deleted.
*
*
* @param describeStackEventsRequest Container for the necessary
* parameters to execute the DescribeStackEvents operation on
* AmazonCloudFormation.
*
* @return A Java Future object containing the response from the
* DescribeStackEvents service method, as returned by
* AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeStackEventsAsync(DescribeStackEventsRequest describeStackEventsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns all stack related events for a specified stack. For more
* information about a stack's event history, go to
* Stacks
* in the AWS CloudFormation User Guide.
*
*
* NOTE:Events are returned, even if the stack never existed or
* has been successfully deleted.
*
*
* @param describeStackEventsRequest Container for the necessary
* parameters to execute the DescribeStackEvents operation on
* AmazonCloudFormation.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeStackEvents service method, as returned by
* AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeStackEventsAsync(DescribeStackEventsRequest describeStackEventsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a description of the specified resource in the specified
* stack.
*
*
* For deleted stacks, DescribeStackResource returns resource information
* for up to 90 days after the stack has been deleted.
*
*
* @param describeStackResourceRequest Container for the necessary
* parameters to execute the DescribeStackResource operation on
* AmazonCloudFormation.
*
* @return A Java Future object containing the response from the
* DescribeStackResource service method, as returned by
* AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeStackResourceAsync(DescribeStackResourceRequest describeStackResourceRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a description of the specified resource in the specified
* stack.
*
*
* For deleted stacks, DescribeStackResource returns resource information
* for up to 90 days after the stack has been deleted.
*
*
* @param describeStackResourceRequest Container for the necessary
* parameters to execute the DescribeStackResource operation on
* AmazonCloudFormation.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeStackResource service method, as returned by
* AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeStackResourceAsync(DescribeStackResourceRequest describeStackResourceRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Cancels an update on the specified stack. If the call completes
* successfully, the stack will roll back the update and revert to the
* previous stack configuration.
*
*
* NOTE:Only stacks that are in the UPDATE_IN_PROGRESS state can
* be canceled.
*
*
* @param cancelUpdateStackRequest Container for the necessary parameters
* to execute the CancelUpdateStack operation on AmazonCloudFormation.
*
* @return A Java Future object containing the response from the
* CancelUpdateStack service method, as returned by AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future cancelUpdateStackAsync(CancelUpdateStackRequest cancelUpdateStackRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Cancels an update on the specified stack. If the call completes
* successfully, the stack will roll back the update and revert to the
* previous stack configuration.
*
*
* NOTE:Only stacks that are in the UPDATE_IN_PROGRESS state can
* be canceled.
*
*
* @param cancelUpdateStackRequest Container for the necessary parameters
* to execute the CancelUpdateStack operation on AmazonCloudFormation.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CancelUpdateStack service method, as returned by AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future cancelUpdateStackAsync(CancelUpdateStackRequest cancelUpdateStackRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Updates a stack as specified in the template. After the call completes
* successfully, the stack update starts. You can check the status of the
* stack via the DescribeStacks action.
*
*
* To get a copy of the template for an existing stack, you can use the
* GetTemplate action.
*
*
* Tags that were associated with this stack during creation time will
* still be associated with the stack after an UpdateStack
* operation.
*
*
* For more information about creating an update template, updating a
* stack, and monitoring the progress of the update, see
* Updating a Stack
* .
*
*
* @param updateStackRequest Container for the necessary parameters to
* execute the UpdateStack operation on AmazonCloudFormation.
*
* @return A Java Future object containing the response from the
* UpdateStack service method, as returned by AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateStackAsync(UpdateStackRequest updateStackRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Updates a stack as specified in the template. After the call completes
* successfully, the stack update starts. You can check the status of the
* stack via the DescribeStacks action.
*
*
* To get a copy of the template for an existing stack, you can use the
* GetTemplate action.
*
*
* Tags that were associated with this stack during creation time will
* still be associated with the stack after an UpdateStack
* operation.
*
*
* For more information about creating an update template, updating a
* stack, and monitoring the progress of the update, see
* Updating a Stack
* .
*
*
* @param updateStackRequest Container for the necessary parameters to
* execute the UpdateStack operation on AmazonCloudFormation.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* UpdateStack service method, as returned by AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateStackAsync(UpdateStackRequest updateStackRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns AWS resource descriptions for running and deleted stacks. If
* StackName
is specified, all the associated resources that
* are part of the stack are returned. If PhysicalResourceId
* is specified, the associated resources of the stack that the resource
* belongs to are returned.
*
*
* NOTE:Only the first 100 resources will be returned. If your
* stack has more resources than this, you should use ListStackResources
* instead.
*
*
* For deleted stacks, DescribeStackResources
returns
* resource information for up to 90 days after the stack has been
* deleted.
*
*
* You must specify either StackName
or
* PhysicalResourceId
, but not both. In addition, you can
* specify LogicalResourceId
to filter the returned result.
* For more information about resources, the
* LogicalResourceId
and PhysicalResourceId
,
* go to the
* AWS CloudFormation User Guide
* .
*
*
* NOTE:A ValidationError is returned if you specify both
* StackName and PhysicalResourceId in the same request.
*
*
* @param describeStackResourcesRequest Container for the necessary
* parameters to execute the DescribeStackResources operation on
* AmazonCloudFormation.
*
* @return A Java Future object containing the response from the
* DescribeStackResources service method, as returned by
* AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeStackResourcesAsync(DescribeStackResourcesRequest describeStackResourcesRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns AWS resource descriptions for running and deleted stacks. If
* StackName
is specified, all the associated resources that
* are part of the stack are returned. If PhysicalResourceId
* is specified, the associated resources of the stack that the resource
* belongs to are returned.
*
*
* NOTE:Only the first 100 resources will be returned. If your
* stack has more resources than this, you should use ListStackResources
* instead.
*
*
* For deleted stacks, DescribeStackResources
returns
* resource information for up to 90 days after the stack has been
* deleted.
*
*
* You must specify either StackName
or
* PhysicalResourceId
, but not both. In addition, you can
* specify LogicalResourceId
to filter the returned result.
* For more information about resources, the
* LogicalResourceId
and PhysicalResourceId
,
* go to the
* AWS CloudFormation User Guide
* .
*
*
* NOTE:A ValidationError is returned if you specify both
* StackName and PhysicalResourceId in the same request.
*
*
* @param describeStackResourcesRequest Container for the necessary
* parameters to execute the DescribeStackResources operation on
* AmazonCloudFormation.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeStackResources service method, as returned by
* AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeStackResourcesAsync(DescribeStackResourcesRequest describeStackResourcesRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes a specified stack. Once the call completes successfully, stack
* deletion starts. Deleted stacks do not show up in the DescribeStacks
* API if the deletion has been completed successfully.
*
*
* @param deleteStackRequest Container for the necessary parameters to
* execute the DeleteStack operation on AmazonCloudFormation.
*
* @return A Java Future object containing the response from the
* DeleteStack service method, as returned by AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteStackAsync(DeleteStackRequest deleteStackRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes a specified stack. Once the call completes successfully, stack
* deletion starts. Deleted stacks do not show up in the DescribeStacks
* API if the deletion has been completed successfully.
*
*
* @param deleteStackRequest Container for the necessary parameters to
* execute the DeleteStack operation on AmazonCloudFormation.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteStack service method, as returned by AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteStackAsync(DeleteStackRequest deleteStackRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns descriptions of all resources of the specified stack.
*
*
* For deleted stacks, ListStackResources returns resource information
* for up to 90 days after the stack has been deleted.
*
*
* @param listStackResourcesRequest Container for the necessary
* parameters to execute the ListStackResources operation on
* AmazonCloudFormation.
*
* @return A Java Future object containing the response from the
* ListStackResources service method, as returned by
* AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listStackResourcesAsync(ListStackResourcesRequest listStackResourcesRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns descriptions of all resources of the specified stack.
*
*
* For deleted stacks, ListStackResources returns resource information
* for up to 90 days after the stack has been deleted.
*
*
* @param listStackResourcesRequest Container for the necessary
* parameters to execute the ListStackResources operation on
* AmazonCloudFormation.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ListStackResources service method, as returned by
* AmazonCloudFormation.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudFormation indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listStackResourcesAsync(ListStackResourcesRequest listStackResourcesRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
}