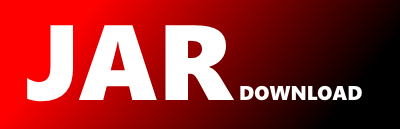
com.amazonaws.services.cloudformation.model.UpdateStackRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.cloudformation.model;
import java.io.Serializable;
import com.amazonaws.AmazonWebServiceRequest;
/**
* Container for the parameters to the {@link com.amazonaws.services.cloudformation.AmazonCloudFormation#updateStack(UpdateStackRequest) UpdateStack operation}.
*
* Updates a stack as specified in the template. After the call completes
* successfully, the stack update starts. You can check the status of the
* stack via the DescribeStacks action.
*
*
* To get a copy of the template for an existing stack, you can use the
* GetTemplate action.
*
*
* Tags that were associated with this stack during creation time will
* still be associated with the stack after an UpdateStack
* operation.
*
*
* For more information about creating an update template, updating a
* stack, and monitoring the progress of the update, see
* Updating a Stack
* .
*
*
* @see com.amazonaws.services.cloudformation.AmazonCloudFormation#updateStack(UpdateStackRequest)
*/
public class UpdateStackRequest extends AmazonWebServiceRequest implements Serializable {
/**
* The name or stack ID of the stack to update. Must contain only
* alphanumeric characters (case sensitive) and start with an alpha
* character. Maximum length of the name is 255 characters.
*/
private String stackName;
/**
* Structure containing the template body with a minimum length of 1 byte
* and a maximum length of 51,200 bytes. (For more information, go to Template
* Anatomy in the AWS CloudFormation User Guide.) Conditional: You
* must specify either the TemplateBody
or the
* TemplateURL
parameter, but not both.
*
* Constraints:
* Length: 1 -
*/
private String templateBody;
/**
* Location of file containing the template body. The URL must point to a
* template located in an S3 bucket in the same region as the stack. For
* more information, go to Template
* Anatomy in the AWS CloudFormation User Guide.
Conditional: You
* must specify either the TemplateBody
or the
* TemplateURL
parameter, but not both.
*
* Constraints:
* Length: 1 - 1024
*/
private String templateURL;
/**
* Structure containing the temporary overriding stack policy body. You
* can specify either the StackPolicyDuringUpdateBody
or the
* StackPolicyDuringUpdateURL
parameter, but not both.
If
* you want to update protected resources, specify a temporary overriding
* stack policy during this update. If you do not specify a stack policy,
* the current policy that is associated with the stack will be used.
*
* Constraints:
* Length: 1 - 16384
*/
private String stackPolicyDuringUpdateBody;
/**
* Location of a file containing the temporary overriding stack policy.
* The URL must point to a policy (max size: 16KB) located in an S3
* bucket in the same region as the stack. You can specify either the
* StackPolicyDuringUpdateBody
or the
* StackPolicyDuringUpdateURL
parameter, but not both.
If
* you want to update protected resources, specify a temporary overriding
* stack policy during this update. If you do not specify a stack policy,
* the current policy that is associated with the stack will be used.
*
* Constraints:
* Length: 1 - 1350
*/
private String stackPolicyDuringUpdateURL;
/**
* A list of Parameter
structures that specify input
* parameters for the stack.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag parameters;
/**
* The list of capabilities that you want to allow in the stack. If your
* stack contains IAM resources, you must specify the CAPABILITY_IAM
* value for this parameter; otherwise, this action returns an
* InsufficientCapabilities error. IAM resources are the following: AWS::IAM::AccessKey,
* AWS::IAM::Group,
* AWS::IAM::Policy,
* AWS::IAM::User,
* and AWS::IAM::UserToGroupAddition.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag capabilities;
/**
* Structure containing a new stack policy body. You can specify either
* the StackPolicyBody
or the StackPolicyURL
* parameter, but not both. You might update the stack policy, for
* example, in order to protect a new resource that you created during a
* stack update. If you do not specify a stack policy, the current policy
* that is associated with the stack is unchanged.
*
* Constraints:
* Length: 1 - 16384
*/
private String stackPolicyBody;
/**
* Location of a file containing the updated stack policy. The URL must
* point to a policy (max size: 16KB) located in an S3 bucket in the same
* region as the stack. You can specify either the
* StackPolicyBody
or the StackPolicyURL
* parameter, but not both.
You might update the stack policy, for
* example, in order to protect a new resource that you created during a
* stack update. If you do not specify a stack policy, the current policy
* that is associated with the stack is unchanged.
*
* Constraints:
* Length: 1 - 1350
*/
private String stackPolicyURL;
/**
* The name or stack ID of the stack to update. Must contain only
* alphanumeric characters (case sensitive) and start with an alpha
* character. Maximum length of the name is 255 characters.
*
* @return The name or stack ID of the stack to update. Must contain only
* alphanumeric characters (case sensitive) and start with an alpha
* character. Maximum length of the name is 255 characters.
*/
public String getStackName() {
return stackName;
}
/**
* The name or stack ID of the stack to update. Must contain only
* alphanumeric characters (case sensitive) and start with an alpha
* character. Maximum length of the name is 255 characters.
*
* @param stackName The name or stack ID of the stack to update. Must contain only
* alphanumeric characters (case sensitive) and start with an alpha
* character. Maximum length of the name is 255 characters.
*/
public void setStackName(String stackName) {
this.stackName = stackName;
}
/**
* The name or stack ID of the stack to update. Must contain only
* alphanumeric characters (case sensitive) and start with an alpha
* character. Maximum length of the name is 255 characters.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param stackName The name or stack ID of the stack to update. Must contain only
* alphanumeric characters (case sensitive) and start with an alpha
* character. Maximum length of the name is 255 characters.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public UpdateStackRequest withStackName(String stackName) {
this.stackName = stackName;
return this;
}
/**
* Structure containing the template body with a minimum length of 1 byte
* and a maximum length of 51,200 bytes. (For more information, go to Template
* Anatomy in the AWS CloudFormation User Guide.)
Conditional: You
* must specify either the TemplateBody
or the
* TemplateURL
parameter, but not both.
*
* Constraints:
* Length: 1 -
*
* @return Structure containing the template body with a minimum length of 1 byte
* and a maximum length of 51,200 bytes. (For more information, go to Template
* Anatomy in the AWS CloudFormation User Guide.)
Conditional: You
* must specify either the TemplateBody
or the
* TemplateURL
parameter, but not both.
*/
public String getTemplateBody() {
return templateBody;
}
/**
* Structure containing the template body with a minimum length of 1 byte
* and a maximum length of 51,200 bytes. (For more information, go to Template
* Anatomy in the AWS CloudFormation User Guide.)
Conditional: You
* must specify either the TemplateBody
or the
* TemplateURL
parameter, but not both.
*
* Constraints:
* Length: 1 -
*
* @param templateBody Structure containing the template body with a minimum length of 1 byte
* and a maximum length of 51,200 bytes. (For more information, go to Template
* Anatomy in the AWS CloudFormation User Guide.)
Conditional: You
* must specify either the TemplateBody
or the
* TemplateURL
parameter, but not both.
*/
public void setTemplateBody(String templateBody) {
this.templateBody = templateBody;
}
/**
* Structure containing the template body with a minimum length of 1 byte
* and a maximum length of 51,200 bytes. (For more information, go to Template
* Anatomy in the AWS CloudFormation User Guide.)
Conditional: You
* must specify either the TemplateBody
or the
* TemplateURL
parameter, but not both.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 -
*
* @param templateBody Structure containing the template body with a minimum length of 1 byte
* and a maximum length of 51,200 bytes. (For more information, go to Template
* Anatomy in the AWS CloudFormation User Guide.)
Conditional: You
* must specify either the TemplateBody
or the
* TemplateURL
parameter, but not both.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public UpdateStackRequest withTemplateBody(String templateBody) {
this.templateBody = templateBody;
return this;
}
/**
* Location of file containing the template body. The URL must point to a
* template located in an S3 bucket in the same region as the stack. For
* more information, go to Template
* Anatomy in the AWS CloudFormation User Guide.
Conditional: You
* must specify either the TemplateBody
or the
* TemplateURL
parameter, but not both.
*
* Constraints:
* Length: 1 - 1024
*
* @return Location of file containing the template body. The URL must point to a
* template located in an S3 bucket in the same region as the stack. For
* more information, go to Template
* Anatomy in the AWS CloudFormation User Guide.
Conditional: You
* must specify either the TemplateBody
or the
* TemplateURL
parameter, but not both.
*/
public String getTemplateURL() {
return templateURL;
}
/**
* Location of file containing the template body. The URL must point to a
* template located in an S3 bucket in the same region as the stack. For
* more information, go to Template
* Anatomy in the AWS CloudFormation User Guide.
Conditional: You
* must specify either the TemplateBody
or the
* TemplateURL
parameter, but not both.
*
* Constraints:
* Length: 1 - 1024
*
* @param templateURL Location of file containing the template body. The URL must point to a
* template located in an S3 bucket in the same region as the stack. For
* more information, go to Template
* Anatomy in the AWS CloudFormation User Guide.
Conditional: You
* must specify either the TemplateBody
or the
* TemplateURL
parameter, but not both.
*/
public void setTemplateURL(String templateURL) {
this.templateURL = templateURL;
}
/**
* Location of file containing the template body. The URL must point to a
* template located in an S3 bucket in the same region as the stack. For
* more information, go to Template
* Anatomy in the AWS CloudFormation User Guide.
Conditional: You
* must specify either the TemplateBody
or the
* TemplateURL
parameter, but not both.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 1024
*
* @param templateURL Location of file containing the template body. The URL must point to a
* template located in an S3 bucket in the same region as the stack. For
* more information, go to Template
* Anatomy in the AWS CloudFormation User Guide.
Conditional: You
* must specify either the TemplateBody
or the
* TemplateURL
parameter, but not both.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public UpdateStackRequest withTemplateURL(String templateURL) {
this.templateURL = templateURL;
return this;
}
/**
* Structure containing the temporary overriding stack policy body. You
* can specify either the StackPolicyDuringUpdateBody
or the
* StackPolicyDuringUpdateURL
parameter, but not both.
If
* you want to update protected resources, specify a temporary overriding
* stack policy during this update. If you do not specify a stack policy,
* the current policy that is associated with the stack will be used.
*
* Constraints:
* Length: 1 - 16384
*
* @return Structure containing the temporary overriding stack policy body. You
* can specify either the StackPolicyDuringUpdateBody
or the
* StackPolicyDuringUpdateURL
parameter, but not both.
If
* you want to update protected resources, specify a temporary overriding
* stack policy during this update. If you do not specify a stack policy,
* the current policy that is associated with the stack will be used.
*/
public String getStackPolicyDuringUpdateBody() {
return stackPolicyDuringUpdateBody;
}
/**
* Structure containing the temporary overriding stack policy body. You
* can specify either the StackPolicyDuringUpdateBody
or the
* StackPolicyDuringUpdateURL
parameter, but not both.
If
* you want to update protected resources, specify a temporary overriding
* stack policy during this update. If you do not specify a stack policy,
* the current policy that is associated with the stack will be used.
*
* Constraints:
* Length: 1 - 16384
*
* @param stackPolicyDuringUpdateBody Structure containing the temporary overriding stack policy body. You
* can specify either the StackPolicyDuringUpdateBody
or the
* StackPolicyDuringUpdateURL
parameter, but not both.
If
* you want to update protected resources, specify a temporary overriding
* stack policy during this update. If you do not specify a stack policy,
* the current policy that is associated with the stack will be used.
*/
public void setStackPolicyDuringUpdateBody(String stackPolicyDuringUpdateBody) {
this.stackPolicyDuringUpdateBody = stackPolicyDuringUpdateBody;
}
/**
* Structure containing the temporary overriding stack policy body. You
* can specify either the StackPolicyDuringUpdateBody
or the
* StackPolicyDuringUpdateURL
parameter, but not both.
If
* you want to update protected resources, specify a temporary overriding
* stack policy during this update. If you do not specify a stack policy,
* the current policy that is associated with the stack will be used.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 16384
*
* @param stackPolicyDuringUpdateBody Structure containing the temporary overriding stack policy body. You
* can specify either the StackPolicyDuringUpdateBody
or the
* StackPolicyDuringUpdateURL
parameter, but not both.
If
* you want to update protected resources, specify a temporary overriding
* stack policy during this update. If you do not specify a stack policy,
* the current policy that is associated with the stack will be used.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public UpdateStackRequest withStackPolicyDuringUpdateBody(String stackPolicyDuringUpdateBody) {
this.stackPolicyDuringUpdateBody = stackPolicyDuringUpdateBody;
return this;
}
/**
* Location of a file containing the temporary overriding stack policy.
* The URL must point to a policy (max size: 16KB) located in an S3
* bucket in the same region as the stack. You can specify either the
* StackPolicyDuringUpdateBody
or the
* StackPolicyDuringUpdateURL
parameter, but not both.
If
* you want to update protected resources, specify a temporary overriding
* stack policy during this update. If you do not specify a stack policy,
* the current policy that is associated with the stack will be used.
*
* Constraints:
* Length: 1 - 1350
*
* @return Location of a file containing the temporary overriding stack policy.
* The URL must point to a policy (max size: 16KB) located in an S3
* bucket in the same region as the stack. You can specify either the
* StackPolicyDuringUpdateBody
or the
* StackPolicyDuringUpdateURL
parameter, but not both.
If
* you want to update protected resources, specify a temporary overriding
* stack policy during this update. If you do not specify a stack policy,
* the current policy that is associated with the stack will be used.
*/
public String getStackPolicyDuringUpdateURL() {
return stackPolicyDuringUpdateURL;
}
/**
* Location of a file containing the temporary overriding stack policy.
* The URL must point to a policy (max size: 16KB) located in an S3
* bucket in the same region as the stack. You can specify either the
* StackPolicyDuringUpdateBody
or the
* StackPolicyDuringUpdateURL
parameter, but not both.
If
* you want to update protected resources, specify a temporary overriding
* stack policy during this update. If you do not specify a stack policy,
* the current policy that is associated with the stack will be used.
*
* Constraints:
* Length: 1 - 1350
*
* @param stackPolicyDuringUpdateURL Location of a file containing the temporary overriding stack policy.
* The URL must point to a policy (max size: 16KB) located in an S3
* bucket in the same region as the stack. You can specify either the
* StackPolicyDuringUpdateBody
or the
* StackPolicyDuringUpdateURL
parameter, but not both.
If
* you want to update protected resources, specify a temporary overriding
* stack policy during this update. If you do not specify a stack policy,
* the current policy that is associated with the stack will be used.
*/
public void setStackPolicyDuringUpdateURL(String stackPolicyDuringUpdateURL) {
this.stackPolicyDuringUpdateURL = stackPolicyDuringUpdateURL;
}
/**
* Location of a file containing the temporary overriding stack policy.
* The URL must point to a policy (max size: 16KB) located in an S3
* bucket in the same region as the stack. You can specify either the
* StackPolicyDuringUpdateBody
or the
* StackPolicyDuringUpdateURL
parameter, but not both.
If
* you want to update protected resources, specify a temporary overriding
* stack policy during this update. If you do not specify a stack policy,
* the current policy that is associated with the stack will be used.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 1350
*
* @param stackPolicyDuringUpdateURL Location of a file containing the temporary overriding stack policy.
* The URL must point to a policy (max size: 16KB) located in an S3
* bucket in the same region as the stack. You can specify either the
* StackPolicyDuringUpdateBody
or the
* StackPolicyDuringUpdateURL
parameter, but not both.
If
* you want to update protected resources, specify a temporary overriding
* stack policy during this update. If you do not specify a stack policy,
* the current policy that is associated with the stack will be used.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public UpdateStackRequest withStackPolicyDuringUpdateURL(String stackPolicyDuringUpdateURL) {
this.stackPolicyDuringUpdateURL = stackPolicyDuringUpdateURL;
return this;
}
/**
* A list of Parameter
structures that specify input
* parameters for the stack.
*
* @return A list of Parameter
structures that specify input
* parameters for the stack.
*/
public java.util.List getParameters() {
if (parameters == null) {
parameters = new com.amazonaws.internal.ListWithAutoConstructFlag();
parameters.setAutoConstruct(true);
}
return parameters;
}
/**
* A list of Parameter
structures that specify input
* parameters for the stack.
*
* @param parameters A list of Parameter
structures that specify input
* parameters for the stack.
*/
public void setParameters(java.util.Collection parameters) {
if (parameters == null) {
this.parameters = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag parametersCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(parameters.size());
parametersCopy.addAll(parameters);
this.parameters = parametersCopy;
}
/**
* A list of Parameter
structures that specify input
* parameters for the stack.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param parameters A list of Parameter
structures that specify input
* parameters for the stack.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public UpdateStackRequest withParameters(Parameter... parameters) {
if (getParameters() == null) setParameters(new java.util.ArrayList(parameters.length));
for (Parameter value : parameters) {
getParameters().add(value);
}
return this;
}
/**
* A list of Parameter
structures that specify input
* parameters for the stack.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param parameters A list of Parameter
structures that specify input
* parameters for the stack.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public UpdateStackRequest withParameters(java.util.Collection parameters) {
if (parameters == null) {
this.parameters = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag parametersCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(parameters.size());
parametersCopy.addAll(parameters);
this.parameters = parametersCopy;
}
return this;
}
/**
* The list of capabilities that you want to allow in the stack. If your
* stack contains IAM resources, you must specify the CAPABILITY_IAM
* value for this parameter; otherwise, this action returns an
* InsufficientCapabilities error. IAM resources are the following: AWS::IAM::AccessKey,
* AWS::IAM::Group,
* AWS::IAM::Policy,
* AWS::IAM::User,
* and AWS::IAM::UserToGroupAddition.
*
* @return The list of capabilities that you want to allow in the stack. If your
* stack contains IAM resources, you must specify the CAPABILITY_IAM
* value for this parameter; otherwise, this action returns an
* InsufficientCapabilities error. IAM resources are the following: AWS::IAM::AccessKey,
* AWS::IAM::Group,
* AWS::IAM::Policy,
* AWS::IAM::User,
* and AWS::IAM::UserToGroupAddition.
*/
public java.util.List getCapabilities() {
if (capabilities == null) {
capabilities = new com.amazonaws.internal.ListWithAutoConstructFlag();
capabilities.setAutoConstruct(true);
}
return capabilities;
}
/**
* The list of capabilities that you want to allow in the stack. If your
* stack contains IAM resources, you must specify the CAPABILITY_IAM
* value for this parameter; otherwise, this action returns an
* InsufficientCapabilities error. IAM resources are the following: AWS::IAM::AccessKey,
* AWS::IAM::Group,
* AWS::IAM::Policy,
* AWS::IAM::User,
* and AWS::IAM::UserToGroupAddition.
*
* @param capabilities The list of capabilities that you want to allow in the stack. If your
* stack contains IAM resources, you must specify the CAPABILITY_IAM
* value for this parameter; otherwise, this action returns an
* InsufficientCapabilities error. IAM resources are the following: AWS::IAM::AccessKey,
* AWS::IAM::Group,
* AWS::IAM::Policy,
* AWS::IAM::User,
* and AWS::IAM::UserToGroupAddition.
*/
public void setCapabilities(java.util.Collection capabilities) {
if (capabilities == null) {
this.capabilities = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag capabilitiesCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(capabilities.size());
capabilitiesCopy.addAll(capabilities);
this.capabilities = capabilitiesCopy;
}
/**
* The list of capabilities that you want to allow in the stack. If your
* stack contains IAM resources, you must specify the CAPABILITY_IAM
* value for this parameter; otherwise, this action returns an
* InsufficientCapabilities error. IAM resources are the following: AWS::IAM::AccessKey,
* AWS::IAM::Group,
* AWS::IAM::Policy,
* AWS::IAM::User,
* and AWS::IAM::UserToGroupAddition.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param capabilities The list of capabilities that you want to allow in the stack. If your
* stack contains IAM resources, you must specify the CAPABILITY_IAM
* value for this parameter; otherwise, this action returns an
* InsufficientCapabilities error. IAM resources are the following: AWS::IAM::AccessKey,
* AWS::IAM::Group,
* AWS::IAM::Policy,
* AWS::IAM::User,
* and AWS::IAM::UserToGroupAddition.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public UpdateStackRequest withCapabilities(String... capabilities) {
if (getCapabilities() == null) setCapabilities(new java.util.ArrayList(capabilities.length));
for (String value : capabilities) {
getCapabilities().add(value);
}
return this;
}
/**
* The list of capabilities that you want to allow in the stack. If your
* stack contains IAM resources, you must specify the CAPABILITY_IAM
* value for this parameter; otherwise, this action returns an
* InsufficientCapabilities error. IAM resources are the following: AWS::IAM::AccessKey,
* AWS::IAM::Group,
* AWS::IAM::Policy,
* AWS::IAM::User,
* and AWS::IAM::UserToGroupAddition.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param capabilities The list of capabilities that you want to allow in the stack. If your
* stack contains IAM resources, you must specify the CAPABILITY_IAM
* value for this parameter; otherwise, this action returns an
* InsufficientCapabilities error. IAM resources are the following: AWS::IAM::AccessKey,
* AWS::IAM::Group,
* AWS::IAM::Policy,
* AWS::IAM::User,
* and AWS::IAM::UserToGroupAddition.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public UpdateStackRequest withCapabilities(java.util.Collection capabilities) {
if (capabilities == null) {
this.capabilities = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag capabilitiesCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(capabilities.size());
capabilitiesCopy.addAll(capabilities);
this.capabilities = capabilitiesCopy;
}
return this;
}
/**
* The list of capabilities that you want to allow in the stack. If your
* stack contains IAM resources, you must specify the CAPABILITY_IAM
* value for this parameter; otherwise, this action returns an
* InsufficientCapabilities error. IAM resources are the following: AWS::IAM::AccessKey,
* AWS::IAM::Group,
* AWS::IAM::Policy,
* AWS::IAM::User,
* and AWS::IAM::UserToGroupAddition.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param capabilities The list of capabilities that you want to allow in the stack. If your
* stack contains IAM resources, you must specify the CAPABILITY_IAM
* value for this parameter; otherwise, this action returns an
* InsufficientCapabilities error. IAM resources are the following: AWS::IAM::AccessKey,
* AWS::IAM::Group,
* AWS::IAM::Policy,
* AWS::IAM::User,
* and AWS::IAM::UserToGroupAddition.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public UpdateStackRequest withCapabilities(Capability... capabilities) {
java.util.ArrayList capabilitiesCopy = new java.util.ArrayList(capabilities.length);
for (Capability member : capabilities) {
capabilitiesCopy.add(member.toString());
}
if (getCapabilities() == null) {
setCapabilities(capabilitiesCopy);
} else {
getCapabilities().addAll(capabilitiesCopy);
}
return this;
}
/**
* Structure containing a new stack policy body. You can specify either
* the StackPolicyBody
or the StackPolicyURL
* parameter, but not both. You might update the stack policy, for
* example, in order to protect a new resource that you created during a
* stack update. If you do not specify a stack policy, the current policy
* that is associated with the stack is unchanged.
*
* Constraints:
* Length: 1 - 16384
*
* @return Structure containing a new stack policy body. You can specify either
* the StackPolicyBody
or the StackPolicyURL
* parameter, but not both.
You might update the stack policy, for
* example, in order to protect a new resource that you created during a
* stack update. If you do not specify a stack policy, the current policy
* that is associated with the stack is unchanged.
*/
public String getStackPolicyBody() {
return stackPolicyBody;
}
/**
* Structure containing a new stack policy body. You can specify either
* the StackPolicyBody
or the StackPolicyURL
* parameter, but not both.
You might update the stack policy, for
* example, in order to protect a new resource that you created during a
* stack update. If you do not specify a stack policy, the current policy
* that is associated with the stack is unchanged.
*
* Constraints:
* Length: 1 - 16384
*
* @param stackPolicyBody Structure containing a new stack policy body. You can specify either
* the StackPolicyBody
or the StackPolicyURL
* parameter, but not both.
You might update the stack policy, for
* example, in order to protect a new resource that you created during a
* stack update. If you do not specify a stack policy, the current policy
* that is associated with the stack is unchanged.
*/
public void setStackPolicyBody(String stackPolicyBody) {
this.stackPolicyBody = stackPolicyBody;
}
/**
* Structure containing a new stack policy body. You can specify either
* the StackPolicyBody
or the StackPolicyURL
* parameter, but not both.
You might update the stack policy, for
* example, in order to protect a new resource that you created during a
* stack update. If you do not specify a stack policy, the current policy
* that is associated with the stack is unchanged.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 16384
*
* @param stackPolicyBody Structure containing a new stack policy body. You can specify either
* the StackPolicyBody
or the StackPolicyURL
* parameter, but not both.
You might update the stack policy, for
* example, in order to protect a new resource that you created during a
* stack update. If you do not specify a stack policy, the current policy
* that is associated with the stack is unchanged.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public UpdateStackRequest withStackPolicyBody(String stackPolicyBody) {
this.stackPolicyBody = stackPolicyBody;
return this;
}
/**
* Location of a file containing the updated stack policy. The URL must
* point to a policy (max size: 16KB) located in an S3 bucket in the same
* region as the stack. You can specify either the
* StackPolicyBody
or the StackPolicyURL
* parameter, but not both.
You might update the stack policy, for
* example, in order to protect a new resource that you created during a
* stack update. If you do not specify a stack policy, the current policy
* that is associated with the stack is unchanged.
*
* Constraints:
* Length: 1 - 1350
*
* @return Location of a file containing the updated stack policy. The URL must
* point to a policy (max size: 16KB) located in an S3 bucket in the same
* region as the stack. You can specify either the
* StackPolicyBody
or the StackPolicyURL
* parameter, but not both.
You might update the stack policy, for
* example, in order to protect a new resource that you created during a
* stack update. If you do not specify a stack policy, the current policy
* that is associated with the stack is unchanged.
*/
public String getStackPolicyURL() {
return stackPolicyURL;
}
/**
* Location of a file containing the updated stack policy. The URL must
* point to a policy (max size: 16KB) located in an S3 bucket in the same
* region as the stack. You can specify either the
* StackPolicyBody
or the StackPolicyURL
* parameter, but not both.
You might update the stack policy, for
* example, in order to protect a new resource that you created during a
* stack update. If you do not specify a stack policy, the current policy
* that is associated with the stack is unchanged.
*
* Constraints:
* Length: 1 - 1350
*
* @param stackPolicyURL Location of a file containing the updated stack policy. The URL must
* point to a policy (max size: 16KB) located in an S3 bucket in the same
* region as the stack. You can specify either the
* StackPolicyBody
or the StackPolicyURL
* parameter, but not both.
You might update the stack policy, for
* example, in order to protect a new resource that you created during a
* stack update. If you do not specify a stack policy, the current policy
* that is associated with the stack is unchanged.
*/
public void setStackPolicyURL(String stackPolicyURL) {
this.stackPolicyURL = stackPolicyURL;
}
/**
* Location of a file containing the updated stack policy. The URL must
* point to a policy (max size: 16KB) located in an S3 bucket in the same
* region as the stack. You can specify either the
* StackPolicyBody
or the StackPolicyURL
* parameter, but not both.
You might update the stack policy, for
* example, in order to protect a new resource that you created during a
* stack update. If you do not specify a stack policy, the current policy
* that is associated with the stack is unchanged.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 1350
*
* @param stackPolicyURL Location of a file containing the updated stack policy. The URL must
* point to a policy (max size: 16KB) located in an S3 bucket in the same
* region as the stack. You can specify either the
* StackPolicyBody
or the StackPolicyURL
* parameter, but not both.
You might update the stack policy, for
* example, in order to protect a new resource that you created during a
* stack update. If you do not specify a stack policy, the current policy
* that is associated with the stack is unchanged.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public UpdateStackRequest withStackPolicyURL(String stackPolicyURL) {
this.stackPolicyURL = stackPolicyURL;
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getStackName() != null) sb.append("StackName: " + getStackName() + ",");
if (getTemplateBody() != null) sb.append("TemplateBody: " + getTemplateBody() + ",");
if (getTemplateURL() != null) sb.append("TemplateURL: " + getTemplateURL() + ",");
if (getStackPolicyDuringUpdateBody() != null) sb.append("StackPolicyDuringUpdateBody: " + getStackPolicyDuringUpdateBody() + ",");
if (getStackPolicyDuringUpdateURL() != null) sb.append("StackPolicyDuringUpdateURL: " + getStackPolicyDuringUpdateURL() + ",");
if (getParameters() != null) sb.append("Parameters: " + getParameters() + ",");
if (getCapabilities() != null) sb.append("Capabilities: " + getCapabilities() + ",");
if (getStackPolicyBody() != null) sb.append("StackPolicyBody: " + getStackPolicyBody() + ",");
if (getStackPolicyURL() != null) sb.append("StackPolicyURL: " + getStackPolicyURL() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getStackName() == null) ? 0 : getStackName().hashCode());
hashCode = prime * hashCode + ((getTemplateBody() == null) ? 0 : getTemplateBody().hashCode());
hashCode = prime * hashCode + ((getTemplateURL() == null) ? 0 : getTemplateURL().hashCode());
hashCode = prime * hashCode + ((getStackPolicyDuringUpdateBody() == null) ? 0 : getStackPolicyDuringUpdateBody().hashCode());
hashCode = prime * hashCode + ((getStackPolicyDuringUpdateURL() == null) ? 0 : getStackPolicyDuringUpdateURL().hashCode());
hashCode = prime * hashCode + ((getParameters() == null) ? 0 : getParameters().hashCode());
hashCode = prime * hashCode + ((getCapabilities() == null) ? 0 : getCapabilities().hashCode());
hashCode = prime * hashCode + ((getStackPolicyBody() == null) ? 0 : getStackPolicyBody().hashCode());
hashCode = prime * hashCode + ((getStackPolicyURL() == null) ? 0 : getStackPolicyURL().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof UpdateStackRequest == false) return false;
UpdateStackRequest other = (UpdateStackRequest)obj;
if (other.getStackName() == null ^ this.getStackName() == null) return false;
if (other.getStackName() != null && other.getStackName().equals(this.getStackName()) == false) return false;
if (other.getTemplateBody() == null ^ this.getTemplateBody() == null) return false;
if (other.getTemplateBody() != null && other.getTemplateBody().equals(this.getTemplateBody()) == false) return false;
if (other.getTemplateURL() == null ^ this.getTemplateURL() == null) return false;
if (other.getTemplateURL() != null && other.getTemplateURL().equals(this.getTemplateURL()) == false) return false;
if (other.getStackPolicyDuringUpdateBody() == null ^ this.getStackPolicyDuringUpdateBody() == null) return false;
if (other.getStackPolicyDuringUpdateBody() != null && other.getStackPolicyDuringUpdateBody().equals(this.getStackPolicyDuringUpdateBody()) == false) return false;
if (other.getStackPolicyDuringUpdateURL() == null ^ this.getStackPolicyDuringUpdateURL() == null) return false;
if (other.getStackPolicyDuringUpdateURL() != null && other.getStackPolicyDuringUpdateURL().equals(this.getStackPolicyDuringUpdateURL()) == false) return false;
if (other.getParameters() == null ^ this.getParameters() == null) return false;
if (other.getParameters() != null && other.getParameters().equals(this.getParameters()) == false) return false;
if (other.getCapabilities() == null ^ this.getCapabilities() == null) return false;
if (other.getCapabilities() != null && other.getCapabilities().equals(this.getCapabilities()) == false) return false;
if (other.getStackPolicyBody() == null ^ this.getStackPolicyBody() == null) return false;
if (other.getStackPolicyBody() != null && other.getStackPolicyBody().equals(this.getStackPolicyBody()) == false) return false;
if (other.getStackPolicyURL() == null ^ this.getStackPolicyURL() == null) return false;
if (other.getStackPolicyURL() != null && other.getStackPolicyURL().equals(this.getStackPolicyURL()) == false) return false;
return true;
}
}