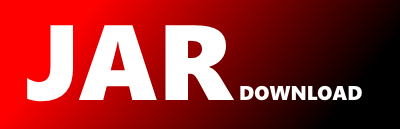
com.amazonaws.services.cloudsearchv2.AmazonCloudSearchClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.cloudsearchv2;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import java.util.Map.Entry;
import com.amazonaws.*;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.services.cloudsearchv2.model.*;
import com.amazonaws.services.cloudsearchv2.model.transform.*;
/**
* Client for accessing AmazonCloudSearchv2. All service calls made
* using this client are blocking, and will not return until the service call
* completes.
*
* Amazon CloudSearch Configuration Service
* You use the Amazon CloudSearch configuration service to create,
* configure, and manage search domains. Configuration service requests
* are submitted using the AWS Query protocol. AWS Query requests are
* HTTP or HTTPS requests submitted via HTTP GET or POST with a query
* parameter named Action.
*
*
* The endpoint for configuration service requests is region-specific:
* cloudsearch. region .amazonaws.com. For example,
* cloudsearch.us-east-1.amazonaws.com. For a current list of supported
* regions and endpoints, see
* Regions and Endpoints
* .
*
*/
public class AmazonCloudSearchClient extends AmazonWebServiceClient implements AmazonCloudSearch {
/** Provider for AWS credentials. */
private AWSCredentialsProvider awsCredentialsProvider;
/**
* List of exception unmarshallers for all AmazonCloudSearchv2 exceptions.
*/
protected final List> exceptionUnmarshallers
= new ArrayList>();
/**
* Constructs a new client to invoke service methods on
* AmazonCloudSearchv2. A credentials provider chain will be used
* that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @see DefaultAWSCredentialsProviderChain
*/
public AmazonCloudSearchClient() {
this(new DefaultAWSCredentialsProviderChain(), new ClientConfiguration());
}
/**
* Constructs a new client to invoke service methods on
* AmazonCloudSearchv2. A credentials provider chain will be used
* that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to AmazonCloudSearchv2
* (ex: proxy settings, retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
*/
public AmazonCloudSearchClient(ClientConfiguration clientConfiguration) {
this(new DefaultAWSCredentialsProviderChain(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on
* AmazonCloudSearchv2 using the specified AWS account credentials.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentials The AWS credentials (access key ID and secret key) to use
* when authenticating with AWS services.
*/
public AmazonCloudSearchClient(AWSCredentials awsCredentials) {
this(awsCredentials, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service methods on
* AmazonCloudSearchv2 using the specified AWS account credentials
* and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentials The AWS credentials (access key ID and secret key) to use
* when authenticating with AWS services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to AmazonCloudSearchv2
* (ex: proxy settings, retry counts, etc.).
*/
public AmazonCloudSearchClient(AWSCredentials awsCredentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.awsCredentialsProvider = new StaticCredentialsProvider(awsCredentials);
init();
}
/**
* Constructs a new client to invoke service methods on
* AmazonCloudSearchv2 using the specified AWS account credentials provider.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
*/
public AmazonCloudSearchClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service methods on
* AmazonCloudSearchv2 using the specified AWS account credentials
* provider and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to AmazonCloudSearchv2
* (ex: proxy settings, retry counts, etc.).
*/
public AmazonCloudSearchClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on
* AmazonCloudSearchv2 using the specified AWS account credentials
* provider, client configuration options, and request metric collector.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to AmazonCloudSearchv2
* (ex: proxy settings, retry counts, etc.).
* @param requestMetricCollector optional request metric collector
*/
public AmazonCloudSearchClient(AWSCredentialsProvider awsCredentialsProvider,
ClientConfiguration clientConfiguration,
RequestMetricCollector requestMetricCollector) {
super(clientConfiguration, requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
init();
}
private void init() {
exceptionUnmarshallers.add(new InvalidTypeExceptionUnmarshaller());
exceptionUnmarshallers.add(new DisabledOperationExceptionUnmarshaller());
exceptionUnmarshallers.add(new BaseExceptionUnmarshaller());
exceptionUnmarshallers.add(new ResourceNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new LimitExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new InternalExceptionUnmarshaller());
exceptionUnmarshallers.add(new StandardErrorUnmarshaller());
// calling this.setEndPoint(...) will also modify the signer accordingly
this.setEndpoint("cloudsearch.us-east-1.amazonaws.com/");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain(
"/com/amazonaws/services/cloudsearchv2/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain(
"/com/amazonaws/services/cloudsearchv2/request.handler2s"));
}
/**
*
* Gets the analysis schemes configured for a domain. An analysis scheme
* defines language-specific text processing options for a
* text
field. Can be limited to specific analysis schemes
* by name. By default, shows all analysis schemes and includes any
* pending changes to the configuration. Set the Deployed
* option to true
to show the active configuration and
* exclude pending changes. For more information, see
* Configuring Analysis Schemes
* in the Amazon CloudSearch Developer Guide .
*
*
* @param describeAnalysisSchemesRequest Container for the necessary
* parameters to execute the DescribeAnalysisSchemes service method on
* AmazonCloudSearchv2.
*
* @return The response from the DescribeAnalysisSchemes service method,
* as returned by AmazonCloudSearchv2.
*
* @throws InternalException
* @throws ResourceNotFoundException
* @throws BaseException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearchv2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeAnalysisSchemesResult describeAnalysisSchemes(DescribeAnalysisSchemesRequest describeAnalysisSchemesRequest) {
ExecutionContext executionContext = createExecutionContext(describeAnalysisSchemesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeAnalysisSchemesRequestMarshaller().marshall(describeAnalysisSchemesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeAnalysisSchemesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes an IndexField
from the search domain. For more
* information, see
* Configuring Index Fields
* in the Amazon CloudSearch Developer Guide .
*
*
* @param deleteIndexFieldRequest Container for the necessary parameters
* to execute the DeleteIndexField service method on AmazonCloudSearchv2.
*
* @return The response from the DeleteIndexField service method, as
* returned by AmazonCloudSearchv2.
*
* @throws InternalException
* @throws InvalidTypeException
* @throws ResourceNotFoundException
* @throws BaseException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearchv2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DeleteIndexFieldResult deleteIndexField(DeleteIndexFieldRequest deleteIndexFieldRequest) {
ExecutionContext executionContext = createExecutionContext(deleteIndexFieldRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteIndexFieldRequestMarshaller().marshall(deleteIndexFieldRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DeleteIndexFieldResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Configures the availability options for a domain. Enabling the
* Multi-AZ option expands an Amazon CloudSearch domain to an additional
* Availability Zone in the same Region to increase fault tolerance in
* the event of a service disruption. Changes to the Multi-AZ option can
* take about half an hour to become active. For more information, see
* Configuring Availability Options
* in the Amazon CloudSearch Developer Guide .
*
*
* @param updateAvailabilityOptionsRequest Container for the necessary
* parameters to execute the UpdateAvailabilityOptions service method on
* AmazonCloudSearchv2.
*
* @return The response from the UpdateAvailabilityOptions service
* method, as returned by AmazonCloudSearchv2.
*
* @throws InternalException
* @throws InvalidTypeException
* @throws DisabledOperationException
* @throws ResourceNotFoundException
* @throws LimitExceededException
* @throws BaseException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearchv2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public UpdateAvailabilityOptionsResult updateAvailabilityOptions(UpdateAvailabilityOptionsRequest updateAvailabilityOptionsRequest) {
ExecutionContext executionContext = createExecutionContext(updateAvailabilityOptionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new UpdateAvailabilityOptionsRequestMarshaller().marshall(updateAvailabilityOptionsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new UpdateAvailabilityOptionsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about the index fields configured for the search
* domain. Can be limited to specific fields by name. By default, shows
* all fields and includes any pending changes to the configuration. Set
* the Deployed
option to true
to show the
* active configuration and exclude pending changes. For more
* information, see
* Getting Domain Information
* in the Amazon CloudSearch Developer Guide .
*
*
* @param describeIndexFieldsRequest Container for the necessary
* parameters to execute the DescribeIndexFields service method on
* AmazonCloudSearchv2.
*
* @return The response from the DescribeIndexFields service method, as
* returned by AmazonCloudSearchv2.
*
* @throws InternalException
* @throws ResourceNotFoundException
* @throws BaseException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearchv2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeIndexFieldsResult describeIndexFields(DescribeIndexFieldsRequest describeIndexFieldsRequest) {
ExecutionContext executionContext = createExecutionContext(describeIndexFieldsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeIndexFieldsRequestMarshaller().marshall(describeIndexFieldsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeIndexFieldsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Configures an Expression
for the search domain. Used to
* create new expressions and modify existing ones. If the expression
* exists, the new configuration replaces the old one. For more
* information, see
* Configuring Expressions
* in the Amazon CloudSearch Developer Guide .
*
*
* @param defineExpressionRequest Container for the necessary parameters
* to execute the DefineExpression service method on AmazonCloudSearchv2.
*
* @return The response from the DefineExpression service method, as
* returned by AmazonCloudSearchv2.
*
* @throws InternalException
* @throws InvalidTypeException
* @throws ResourceNotFoundException
* @throws LimitExceededException
* @throws BaseException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearchv2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DefineExpressionResult defineExpression(DefineExpressionRequest defineExpressionRequest) {
ExecutionContext executionContext = createExecutionContext(defineExpressionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DefineExpressionRequestMarshaller().marshall(defineExpressionRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DefineExpressionResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Configures the access rules that control access to the domain's
* document and search endpoints. For more information, see
* Configuring Access for an Amazon CloudSearch Domain
* .
*
*
* @param updateServiceAccessPoliciesRequest Container for the necessary
* parameters to execute the UpdateServiceAccessPolicies service method
* on AmazonCloudSearchv2.
*
* @return The response from the UpdateServiceAccessPolicies service
* method, as returned by AmazonCloudSearchv2.
*
* @throws InternalException
* @throws InvalidTypeException
* @throws ResourceNotFoundException
* @throws LimitExceededException
* @throws BaseException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearchv2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public UpdateServiceAccessPoliciesResult updateServiceAccessPolicies(UpdateServiceAccessPoliciesRequest updateServiceAccessPoliciesRequest) {
ExecutionContext executionContext = createExecutionContext(updateServiceAccessPoliciesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new UpdateServiceAccessPoliciesRequestMarshaller().marshall(updateServiceAccessPoliciesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new UpdateServiceAccessPoliciesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Configures a suggester for a domain. A suggester enables you to
* display possible matches before users finish typing their queries.
* When you configure a suggester, you must specify the name of the text
* field you want to search for possible matches and a unique name for
* the suggester. For more information, see
* Getting Search Suggestions
* in the Amazon CloudSearch Developer Guide .
*
*
* @param defineSuggesterRequest Container for the necessary parameters
* to execute the DefineSuggester service method on AmazonCloudSearchv2.
*
* @return The response from the DefineSuggester service method, as
* returned by AmazonCloudSearchv2.
*
* @throws InternalException
* @throws InvalidTypeException
* @throws ResourceNotFoundException
* @throws LimitExceededException
* @throws BaseException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearchv2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DefineSuggesterResult defineSuggester(DefineSuggesterRequest defineSuggesterRequest) {
ExecutionContext executionContext = createExecutionContext(defineSuggesterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DefineSuggesterRequestMarshaller().marshall(defineSuggesterRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DefineSuggesterResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an analysis scheme. For more information, see
* Configuring Analysis Schemes
* in the Amazon CloudSearch Developer Guide .
*
*
* @param deleteAnalysisSchemeRequest Container for the necessary
* parameters to execute the DeleteAnalysisScheme service method on
* AmazonCloudSearchv2.
*
* @return The response from the DeleteAnalysisScheme service method, as
* returned by AmazonCloudSearchv2.
*
* @throws InternalException
* @throws InvalidTypeException
* @throws ResourceNotFoundException
* @throws BaseException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearchv2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DeleteAnalysisSchemeResult deleteAnalysisScheme(DeleteAnalysisSchemeRequest deleteAnalysisSchemeRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAnalysisSchemeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteAnalysisSchemeRequestMarshaller().marshall(deleteAnalysisSchemeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DeleteAnalysisSchemeResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Tells the search domain to start indexing its documents using the
* latest indexing options. This operation must be invoked to activate
* options whose OptionStatus is RequiresIndexDocuments
.
*
*
* @param indexDocumentsRequest Container for the necessary parameters to
* execute the IndexDocuments service method on AmazonCloudSearchv2.
*
* @return The response from the IndexDocuments service method, as
* returned by AmazonCloudSearchv2.
*
* @throws InternalException
* @throws ResourceNotFoundException
* @throws BaseException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearchv2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public IndexDocumentsResult indexDocuments(IndexDocumentsRequest indexDocumentsRequest) {
ExecutionContext executionContext = createExecutionContext(indexDocumentsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new IndexDocumentsRequestMarshaller().marshall(indexDocumentsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new IndexDocumentsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the suggesters configured for a domain. A suggester enables you
* to display possible matches before users finish typing their queries.
* Can be limited to specific suggesters by name. By default, shows all
* suggesters and includes any pending changes to the configuration. Set
* the Deployed
option to true
to show the
* active configuration and exclude pending changes. For more
* information, see
* Getting Search Suggestions
* in the Amazon CloudSearch Developer Guide .
*
*
* @param describeSuggestersRequest Container for the necessary
* parameters to execute the DescribeSuggesters service method on
* AmazonCloudSearchv2.
*
* @return The response from the DescribeSuggesters service method, as
* returned by AmazonCloudSearchv2.
*
* @throws InternalException
* @throws ResourceNotFoundException
* @throws BaseException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearchv2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeSuggestersResult describeSuggesters(DescribeSuggestersRequest describeSuggestersRequest) {
ExecutionContext executionContext = createExecutionContext(describeSuggestersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeSuggestersRequestMarshaller().marshall(describeSuggestersRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeSuggestersResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Configures scaling parameters for a domain. A domain's scaling
* parameters specify the desired search instance type and replication
* count. Amazon CloudSearch will still automatically scale your domain
* based on the volume of data and traffic, but not below the desired
* instance type and replication count. If the Multi-AZ option is
* enabled, these values control the resources used per Availability
* Zone. For more information, see
* Configuring Scaling Options
* in the Amazon CloudSearch Developer Guide .
*
*
* @param updateScalingParametersRequest Container for the necessary
* parameters to execute the UpdateScalingParameters service method on
* AmazonCloudSearchv2.
*
* @return The response from the UpdateScalingParameters service method,
* as returned by AmazonCloudSearchv2.
*
* @throws InternalException
* @throws InvalidTypeException
* @throws ResourceNotFoundException
* @throws LimitExceededException
* @throws BaseException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearchv2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public UpdateScalingParametersResult updateScalingParameters(UpdateScalingParametersRequest updateScalingParametersRequest) {
ExecutionContext executionContext = createExecutionContext(updateScalingParametersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new UpdateScalingParametersRequestMarshaller().marshall(updateScalingParametersRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new UpdateScalingParametersResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all search domains owned by an account.
*
*
* @param listDomainNamesRequest Container for the necessary parameters
* to execute the ListDomainNames service method on AmazonCloudSearchv2.
*
* @return The response from the ListDomainNames service method, as
* returned by AmazonCloudSearchv2.
*
* @throws BaseException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearchv2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListDomainNamesResult listDomainNames(ListDomainNamesRequest listDomainNamesRequest) {
ExecutionContext executionContext = createExecutionContext(listDomainNamesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ListDomainNamesRequestMarshaller().marshall(listDomainNamesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ListDomainNamesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Configures an IndexField
for the search domain. Used to
* create new fields and modify existing ones. You must specify the name
* of the domain you are configuring and an index field configuration.
* The index field configuration specifies a unique name, the index field
* type, and the options you want to configure for the field. The options
* you can specify depend on the IndexFieldType
. If the
* field exists, the new configuration replaces the old one. For more
* information, see
* Configuring Index Fields
* in the Amazon CloudSearch Developer Guide .
*
*
* @param defineIndexFieldRequest Container for the necessary parameters
* to execute the DefineIndexField service method on AmazonCloudSearchv2.
*
* @return The response from the DefineIndexField service method, as
* returned by AmazonCloudSearchv2.
*
* @throws InternalException
* @throws InvalidTypeException
* @throws ResourceNotFoundException
* @throws LimitExceededException
* @throws BaseException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearchv2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DefineIndexFieldResult defineIndexField(DefineIndexFieldRequest defineIndexFieldRequest) {
ExecutionContext executionContext = createExecutionContext(defineIndexFieldRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DefineIndexFieldRequestMarshaller().marshall(defineIndexFieldRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DefineIndexFieldResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new search domain. For more information, see
* Creating a Search Domain
* in the Amazon CloudSearch Developer Guide .
*
*
* @param createDomainRequest Container for the necessary parameters to
* execute the CreateDomain service method on AmazonCloudSearchv2.
*
* @return The response from the CreateDomain service method, as returned
* by AmazonCloudSearchv2.
*
* @throws InternalException
* @throws LimitExceededException
* @throws BaseException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearchv2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateDomainResult createDomain(CreateDomainRequest createDomainRequest) {
ExecutionContext executionContext = createExecutionContext(createDomainRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateDomainRequestMarshaller().marshall(createDomainRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateDomainResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a suggester. For more information, see
* Getting Search Suggestions
* in the Amazon CloudSearch Developer Guide .
*
*
* @param deleteSuggesterRequest Container for the necessary parameters
* to execute the DeleteSuggester service method on AmazonCloudSearchv2.
*
* @return The response from the DeleteSuggester service method, as
* returned by AmazonCloudSearchv2.
*
* @throws InternalException
* @throws InvalidTypeException
* @throws ResourceNotFoundException
* @throws BaseException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearchv2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DeleteSuggesterResult deleteSuggester(DeleteSuggesterRequest deleteSuggesterRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSuggesterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteSuggesterRequestMarshaller().marshall(deleteSuggesterRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DeleteSuggesterResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes an Expression
from the search domain. For more
* information, see
* Configuring Expressions
* in the Amazon CloudSearch Developer Guide .
*
*
* @param deleteExpressionRequest Container for the necessary parameters
* to execute the DeleteExpression service method on AmazonCloudSearchv2.
*
* @return The response from the DeleteExpression service method, as
* returned by AmazonCloudSearchv2.
*
* @throws InternalException
* @throws InvalidTypeException
* @throws ResourceNotFoundException
* @throws BaseException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearchv2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DeleteExpressionResult deleteExpression(DeleteExpressionRequest deleteExpressionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteExpressionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteExpressionRequestMarshaller().marshall(deleteExpressionRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DeleteExpressionResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the availability options configured for a domain. By default,
* shows the configuration with any pending changes. Set the
* Deployed
option to true
to show the active
* configuration and exclude pending changes. For more information, see
* Configuring Availability Options
* in the Amazon CloudSearch Developer Guide .
*
*
* @param describeAvailabilityOptionsRequest Container for the necessary
* parameters to execute the DescribeAvailabilityOptions service method
* on AmazonCloudSearchv2.
*
* @return The response from the DescribeAvailabilityOptions service
* method, as returned by AmazonCloudSearchv2.
*
* @throws InternalException
* @throws InvalidTypeException
* @throws DisabledOperationException
* @throws ResourceNotFoundException
* @throws LimitExceededException
* @throws BaseException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearchv2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeAvailabilityOptionsResult describeAvailabilityOptions(DescribeAvailabilityOptionsRequest describeAvailabilityOptionsRequest) {
ExecutionContext executionContext = createExecutionContext(describeAvailabilityOptionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeAvailabilityOptionsRequestMarshaller().marshall(describeAvailabilityOptionsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeAvailabilityOptionsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Configures an analysis scheme for a domain. An analysis scheme defines
* language-specific text processing options for a text
* field. For more information, see
* Configuring Analysis Schemes
* in the Amazon CloudSearch Developer Guide .
*
*
* @param defineAnalysisSchemeRequest Container for the necessary
* parameters to execute the DefineAnalysisScheme service method on
* AmazonCloudSearchv2.
*
* @return The response from the DefineAnalysisScheme service method, as
* returned by AmazonCloudSearchv2.
*
* @throws InternalException
* @throws InvalidTypeException
* @throws ResourceNotFoundException
* @throws LimitExceededException
* @throws BaseException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearchv2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DefineAnalysisSchemeResult defineAnalysisScheme(DefineAnalysisSchemeRequest defineAnalysisSchemeRequest) {
ExecutionContext executionContext = createExecutionContext(defineAnalysisSchemeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DefineAnalysisSchemeRequestMarshaller().marshall(defineAnalysisSchemeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DefineAnalysisSchemeResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Indexes the search suggestions.
*
*
* @param buildSuggestersRequest Container for the necessary parameters
* to execute the BuildSuggesters service method on AmazonCloudSearchv2.
*
* @return The response from the BuildSuggesters service method, as
* returned by AmazonCloudSearchv2.
*
* @throws InternalException
* @throws ResourceNotFoundException
* @throws BaseException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearchv2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public BuildSuggestersResult buildSuggesters(BuildSuggestersRequest buildSuggestersRequest) {
ExecutionContext executionContext = createExecutionContext(buildSuggestersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new BuildSuggestersRequestMarshaller().marshall(buildSuggestersRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new BuildSuggestersResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about the access policies that control access to the
* domain's document and search endpoints. By default, shows the
* configuration with any pending changes. Set the Deployed
* option to true
to show the active configuration and
* exclude pending changes. For more information, see
* Configuring Access for a Search Domain
* in the Amazon CloudSearch Developer Guide .
*
*
* @param describeServiceAccessPoliciesRequest Container for the
* necessary parameters to execute the DescribeServiceAccessPolicies
* service method on AmazonCloudSearchv2.
*
* @return The response from the DescribeServiceAccessPolicies service
* method, as returned by AmazonCloudSearchv2.
*
* @throws InternalException
* @throws ResourceNotFoundException
* @throws BaseException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearchv2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeServiceAccessPoliciesResult describeServiceAccessPolicies(DescribeServiceAccessPoliciesRequest describeServiceAccessPoliciesRequest) {
ExecutionContext executionContext = createExecutionContext(describeServiceAccessPoliciesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeServiceAccessPoliciesRequestMarshaller().marshall(describeServiceAccessPoliciesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeServiceAccessPoliciesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Permanently deletes a search domain and all of its data. Once a domain
* has been deleted, it cannot be recovered. For more information, see
* Deleting a Search Domain
* in the Amazon CloudSearch Developer Guide .
*
*
* @param deleteDomainRequest Container for the necessary parameters to
* execute the DeleteDomain service method on AmazonCloudSearchv2.
*
* @return The response from the DeleteDomain service method, as returned
* by AmazonCloudSearchv2.
*
* @throws InternalException
* @throws BaseException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearchv2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DeleteDomainResult deleteDomain(DeleteDomainRequest deleteDomainRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDomainRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteDomainRequestMarshaller().marshall(deleteDomainRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DeleteDomainResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the expressions configured for the search domain. Can be limited
* to specific expressions by name. By default, shows all expressions and
* includes any pending changes to the configuration. Set the
* Deployed
option to true
to show the active
* configuration and exclude pending changes. For more information, see
* Configuring Expressions
* in the Amazon CloudSearch Developer Guide .
*
*
* @param describeExpressionsRequest Container for the necessary
* parameters to execute the DescribeExpressions service method on
* AmazonCloudSearchv2.
*
* @return The response from the DescribeExpressions service method, as
* returned by AmazonCloudSearchv2.
*
* @throws InternalException
* @throws ResourceNotFoundException
* @throws BaseException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearchv2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeExpressionsResult describeExpressions(DescribeExpressionsRequest describeExpressionsRequest) {
ExecutionContext executionContext = createExecutionContext(describeExpressionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeExpressionsRequestMarshaller().marshall(describeExpressionsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeExpressionsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets information about the search domains owned by this account. Can
* be limited to specific domains. Shows all domains by default. For more
* information, see
* Getting Information about a Search Domain
* in the Amazon CloudSearch Developer Guide .
*
*
* @param describeDomainsRequest Container for the necessary parameters
* to execute the DescribeDomains service method on AmazonCloudSearchv2.
*
* @return The response from the DescribeDomains service method, as
* returned by AmazonCloudSearchv2.
*
* @throws InternalException
* @throws BaseException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearchv2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeDomainsResult describeDomains(DescribeDomainsRequest describeDomainsRequest) {
ExecutionContext executionContext = createExecutionContext(describeDomainsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeDomainsRequestMarshaller().marshall(describeDomainsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeDomainsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the scaling parameters configured for a domain. A domain's
* scaling parameters specify the desired search instance type and
* replication count. For more information, see
* Configuring Scaling Options
* in the Amazon CloudSearch Developer Guide .
*
*
* @param describeScalingParametersRequest Container for the necessary
* parameters to execute the DescribeScalingParameters service method on
* AmazonCloudSearchv2.
*
* @return The response from the DescribeScalingParameters service
* method, as returned by AmazonCloudSearchv2.
*
* @throws InternalException
* @throws ResourceNotFoundException
* @throws BaseException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearchv2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeScalingParametersResult describeScalingParameters(DescribeScalingParametersRequest describeScalingParametersRequest) {
ExecutionContext executionContext = createExecutionContext(describeScalingParametersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeScalingParametersRequestMarshaller().marshall(describeScalingParametersRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeScalingParametersResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all search domains owned by an account.
*
*
* @return The response from the ListDomainNames service method, as
* returned by AmazonCloudSearchv2.
*
* @throws BaseException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearchv2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListDomainNamesResult listDomainNames() throws AmazonServiceException, AmazonClientException {
return listDomainNames(new ListDomainNamesRequest());
}
/**
*
* Gets information about the search domains owned by this account. Can
* be limited to specific domains. Shows all domains by default. For more
* information, see
* Getting Information about a Search Domain
* in the Amazon CloudSearch Developer Guide .
*
*
* @return The response from the DescribeDomains service method, as
* returned by AmazonCloudSearchv2.
*
* @throws InternalException
* @throws BaseException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonCloudSearchv2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeDomainsResult describeDomains() throws AmazonServiceException, AmazonClientException {
return describeDomains(new DescribeDomainsRequest());
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for
* debugging issues where a service isn't acting as expected. This data isn't considered part
* of the result data returned by an operation, so it's available through this separate,
* diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access
* this extra diagnostic information for an executed request, you should use this method
* to retrieve it as soon as possible after executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none
* is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
private Response invoke(Request request,
Unmarshaller unmarshaller,
ExecutionContext executionContext)
{
request.setEndpoint(endpoint);
request.setTimeOffset(timeOffset);
AmazonWebServiceRequest originalRequest = request.getOriginalRequest();
for (Entry entry : originalRequest.copyPrivateRequestParameters().entrySet()) {
request.addParameter(entry.getKey(), entry.getValue());
}
AWSCredentials credentials = awsCredentialsProvider.getCredentials();
if (originalRequest.getRequestCredentials() != null) {
credentials = originalRequest.getRequestCredentials();
}
executionContext.setCredentials(credentials);
StaxResponseHandler responseHandler = new StaxResponseHandler(unmarshaller);
DefaultErrorResponseHandler errorResponseHandler = new DefaultErrorResponseHandler(exceptionUnmarshallers);
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
}