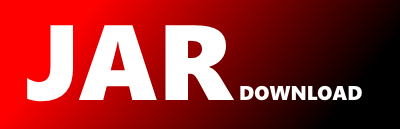
com.amazonaws.services.dynamodb.model.ListTablesRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.dynamodb.model;
import com.amazonaws.AmazonWebServiceRequest;
import java.io.Serializable;
/**
* Container for the parameters to the {@link com.amazonaws.services.dynamodb.AmazonDynamoDB#listTables(ListTablesRequest) ListTables operation}.
*
* Retrieves a paginated list of table names created by the AWS Account of the caller in the AWS Region (e.g. us-east-1
).
*
*
* @see com.amazonaws.services.dynamodb.AmazonDynamoDB#listTables(ListTablesRequest)
*
* @deprecated Use {@link com.amazonaws.services.dynamodbv2.model.ListTablesRequest} instead.
*/
@Deprecated
public class ListTablesRequest extends AmazonWebServiceRequest implements Serializable {
/**
* The name of the table that starts the list. If you already ran a
* ListTables
operation and received a
* LastEvaluatedTableName
value in the response, use that
* value here to continue the list.
*
* Constraints:
* Length: 3 - 255
* Pattern: [a-zA-Z0-9_.-]+
*/
private String exclusiveStartTableName;
/**
* A number of maximum table names to return.
*
* Constraints:
* Range: 1 - 100
*/
private Integer limit;
/**
* The name of the table that starts the list. If you already ran a
* ListTables
operation and received a
* LastEvaluatedTableName
value in the response, use that
* value here to continue the list.
*
* Constraints:
* Length: 3 - 255
* Pattern: [a-zA-Z0-9_.-]+
*
* @return The name of the table that starts the list. If you already ran a
* ListTables
operation and received a
* LastEvaluatedTableName
value in the response, use that
* value here to continue the list.
*/
public String getExclusiveStartTableName() {
return exclusiveStartTableName;
}
/**
* The name of the table that starts the list. If you already ran a
* ListTables
operation and received a
* LastEvaluatedTableName
value in the response, use that
* value here to continue the list.
*
* Constraints:
* Length: 3 - 255
* Pattern: [a-zA-Z0-9_.-]+
*
* @param exclusiveStartTableName The name of the table that starts the list. If you already ran a
* ListTables
operation and received a
* LastEvaluatedTableName
value in the response, use that
* value here to continue the list.
*/
public void setExclusiveStartTableName(String exclusiveStartTableName) {
this.exclusiveStartTableName = exclusiveStartTableName;
}
/**
* The name of the table that starts the list. If you already ran a
* ListTables
operation and received a
* LastEvaluatedTableName
value in the response, use that
* value here to continue the list.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 3 - 255
* Pattern: [a-zA-Z0-9_.-]+
*
* @param exclusiveStartTableName The name of the table that starts the list. If you already ran a
* ListTables
operation and received a
* LastEvaluatedTableName
value in the response, use that
* value here to continue the list.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public ListTablesRequest withExclusiveStartTableName(String exclusiveStartTableName) {
this.exclusiveStartTableName = exclusiveStartTableName;
return this;
}
/**
* A number of maximum table names to return.
*
* Constraints:
* Range: 1 - 100
*
* @return A number of maximum table names to return.
*/
public Integer getLimit() {
return limit;
}
/**
* A number of maximum table names to return.
*
* Constraints:
* Range: 1 - 100
*
* @param limit A number of maximum table names to return.
*/
public void setLimit(Integer limit) {
this.limit = limit;
}
/**
* A number of maximum table names to return.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Range: 1 - 100
*
* @param limit A number of maximum table names to return.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public ListTablesRequest withLimit(Integer limit) {
this.limit = limit;
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getExclusiveStartTableName() != null) sb.append("ExclusiveStartTableName: " + getExclusiveStartTableName() + ", ");
if (getLimit() != null) sb.append("Limit: " + getLimit() + ", ");
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getExclusiveStartTableName() == null) ? 0 : getExclusiveStartTableName().hashCode());
hashCode = prime * hashCode + ((getLimit() == null) ? 0 : getLimit().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof ListTablesRequest == false) return false;
ListTablesRequest other = (ListTablesRequest)obj;
if (other.getExclusiveStartTableName() == null ^ this.getExclusiveStartTableName() == null) return false;
if (other.getExclusiveStartTableName() != null && other.getExclusiveStartTableName().equals(this.getExclusiveStartTableName()) == false) return false;
if (other.getLimit() == null ^ this.getLimit() == null) return false;
if (other.getLimit() != null && other.getLimit().equals(this.getLimit()) == false) return false;
return true;
}
}