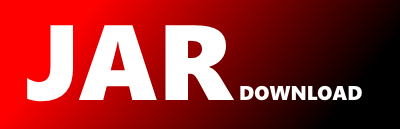
com.amazonaws.services.dynamodbv2.model.BatchWriteItemResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.dynamodbv2.model;
import java.io.Serializable;
/**
*
* Represents the output of a BatchWriteItem operation.
*
*/
public class BatchWriteItemResult implements Serializable {
/**
* A map of tables and requests against those tables that were not
* processed. The UnprocessedItems value is in the same form as
* RequestItems, so you can provide this value directly to a
* subsequent BatchGetItem operation. For more information, see
* RequestItems in the Request Parameters section. Each
* UnprocessedItems entry consists of a table name and, for that
* table, a list of operations to perform (DeleteRequest or
* PutRequest).
-
DeleteRequest - Perform a
* DeleteItem operation on the specified item. The item to be
* deleted is identified by a Key subelement:
-
*
Key - A map of primary key attribute values that uniquely
* identify the item. Each entry in this map consists of an attribute
* name and an attribute value.
-
*
PutRequest - Perform a PutItem operation on the
* specified item. The item to be put is identified by an Item
* subelement:
-
Item - A map of attributes and their
* values. Each entry in this map consists of an attribute name and an
* attribute value. Attribute values must not be null; string and binary
* type attributes must have lengths greater than zero; and set type
* attributes must not be empty. Requests that contain empty values will
* be rejected with a ValidationException.
If you specify any
* attributes that are part of an index key, then the data types for
* those attributes must match those of the schema in the table's
* attribute definition.
If there are no
* unprocessed items remaining, the response contains an empty
* UnprocessedItems map.
*
* Constraints:
* Length: 1 - 25
*/
private java.util.Map> unprocessedItems;
/**
* A list of tables that were processed by BatchWriteItem and, for
* each table, information about any item collections that were affected
* by individual DeleteItem or PutItem operations. Each
* entry consists of the following subelements:
-
*
ItemCollectionKey - The hash key value of the item
* collection. This is the same as the hash key of the item.
-
*
SizeEstimateRange - An estimate of item collection size,
* expressed in GB. This is a two-element array containing a lower bound
* and an upper bound for the estimate. The estimate includes the size of
* all the items in the table, plus the size of all attributes projected
* into all of the local secondary indexes on the table. Use this
* estimate to measure whether a local secondary index is approaching its
* size limit.
The estimate is subject to change over time; therefore,
* do not rely on the precision or accuracy of the estimate.
*/
private java.util.Map> itemCollectionMetrics;
/**
* The capacity units consumed by the operation. Each element consists
* of:
-
TableName - The table that consumed the
* provisioned throughput.
-
CapacityUnits - The total
* number of capacity units consumed.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag consumedCapacity;
/**
* A map of tables and requests against those tables that were not
* processed. The UnprocessedItems value is in the same form as
* RequestItems, so you can provide this value directly to a
* subsequent BatchGetItem operation. For more information, see
* RequestItems in the Request Parameters section. Each
* UnprocessedItems entry consists of a table name and, for that
* table, a list of operations to perform (DeleteRequest or
* PutRequest).
-
DeleteRequest - Perform a
* DeleteItem operation on the specified item. The item to be
* deleted is identified by a Key subelement:
-
*
Key - A map of primary key attribute values that uniquely
* identify the item. Each entry in this map consists of an attribute
* name and an attribute value.
-
*
PutRequest - Perform a PutItem operation on the
* specified item. The item to be put is identified by an Item
* subelement:
-
Item - A map of attributes and their
* values. Each entry in this map consists of an attribute name and an
* attribute value. Attribute values must not be null; string and binary
* type attributes must have lengths greater than zero; and set type
* attributes must not be empty. Requests that contain empty values will
* be rejected with a ValidationException.
If you specify any
* attributes that are part of an index key, then the data types for
* those attributes must match those of the schema in the table's
* attribute definition.
If there are no
* unprocessed items remaining, the response contains an empty
* UnprocessedItems map.
*
* Constraints:
* Length: 1 - 25
*
* @return A map of tables and requests against those tables that were not
* processed. The UnprocessedItems value is in the same form as
* RequestItems, so you can provide this value directly to a
* subsequent BatchGetItem operation. For more information, see
* RequestItems in the Request Parameters section.
Each
* UnprocessedItems entry consists of a table name and, for that
* table, a list of operations to perform (DeleteRequest or
* PutRequest).
-
DeleteRequest - Perform a
* DeleteItem operation on the specified item. The item to be
* deleted is identified by a Key subelement:
-
*
Key - A map of primary key attribute values that uniquely
* identify the item. Each entry in this map consists of an attribute
* name and an attribute value.
-
*
PutRequest - Perform a PutItem operation on the
* specified item. The item to be put is identified by an Item
* subelement:
-
Item - A map of attributes and their
* values. Each entry in this map consists of an attribute name and an
* attribute value. Attribute values must not be null; string and binary
* type attributes must have lengths greater than zero; and set type
* attributes must not be empty. Requests that contain empty values will
* be rejected with a ValidationException.
If you specify any
* attributes that are part of an index key, then the data types for
* those attributes must match those of the schema in the table's
* attribute definition.
If there are no
* unprocessed items remaining, the response contains an empty
* UnprocessedItems map.
*/
public java.util.Map> getUnprocessedItems() {
return unprocessedItems;
}
/**
* A map of tables and requests against those tables that were not
* processed. The UnprocessedItems value is in the same form as
* RequestItems, so you can provide this value directly to a
* subsequent BatchGetItem operation. For more information, see
* RequestItems in the Request Parameters section. Each
* UnprocessedItems entry consists of a table name and, for that
* table, a list of operations to perform (DeleteRequest or
* PutRequest).
-
DeleteRequest - Perform a
* DeleteItem operation on the specified item. The item to be
* deleted is identified by a Key subelement:
-
*
Key - A map of primary key attribute values that uniquely
* identify the item. Each entry in this map consists of an attribute
* name and an attribute value.
-
*
PutRequest - Perform a PutItem operation on the
* specified item. The item to be put is identified by an Item
* subelement:
-
Item - A map of attributes and their
* values. Each entry in this map consists of an attribute name and an
* attribute value. Attribute values must not be null; string and binary
* type attributes must have lengths greater than zero; and set type
* attributes must not be empty. Requests that contain empty values will
* be rejected with a ValidationException.
If you specify any
* attributes that are part of an index key, then the data types for
* those attributes must match those of the schema in the table's
* attribute definition.
If there are no
* unprocessed items remaining, the response contains an empty
* UnprocessedItems map.
*
* Constraints:
* Length: 1 - 25
*
* @param unprocessedItems A map of tables and requests against those tables that were not
* processed. The UnprocessedItems value is in the same form as
* RequestItems, so you can provide this value directly to a
* subsequent BatchGetItem operation. For more information, see
* RequestItems in the Request Parameters section.
Each
* UnprocessedItems entry consists of a table name and, for that
* table, a list of operations to perform (DeleteRequest or
* PutRequest).
-
DeleteRequest - Perform a
* DeleteItem operation on the specified item. The item to be
* deleted is identified by a Key subelement:
-
*
Key - A map of primary key attribute values that uniquely
* identify the item. Each entry in this map consists of an attribute
* name and an attribute value.
-
*
PutRequest - Perform a PutItem operation on the
* specified item. The item to be put is identified by an Item
* subelement:
-
Item - A map of attributes and their
* values. Each entry in this map consists of an attribute name and an
* attribute value. Attribute values must not be null; string and binary
* type attributes must have lengths greater than zero; and set type
* attributes must not be empty. Requests that contain empty values will
* be rejected with a ValidationException.
If you specify any
* attributes that are part of an index key, then the data types for
* those attributes must match those of the schema in the table's
* attribute definition.
If there are no
* unprocessed items remaining, the response contains an empty
* UnprocessedItems map.
*/
public void setUnprocessedItems(java.util.Map> unprocessedItems) {
this.unprocessedItems = unprocessedItems;
}
/**
* A map of tables and requests against those tables that were not
* processed. The UnprocessedItems value is in the same form as
* RequestItems, so you can provide this value directly to a
* subsequent BatchGetItem operation. For more information, see
* RequestItems in the Request Parameters section. Each
* UnprocessedItems entry consists of a table name and, for that
* table, a list of operations to perform (DeleteRequest or
* PutRequest).
-
DeleteRequest - Perform a
* DeleteItem operation on the specified item. The item to be
* deleted is identified by a Key subelement:
-
*
Key - A map of primary key attribute values that uniquely
* identify the item. Each entry in this map consists of an attribute
* name and an attribute value.
-
*
PutRequest - Perform a PutItem operation on the
* specified item. The item to be put is identified by an Item
* subelement:
-
Item - A map of attributes and their
* values. Each entry in this map consists of an attribute name and an
* attribute value. Attribute values must not be null; string and binary
* type attributes must have lengths greater than zero; and set type
* attributes must not be empty. Requests that contain empty values will
* be rejected with a ValidationException.
If you specify any
* attributes that are part of an index key, then the data types for
* those attributes must match those of the schema in the table's
* attribute definition.
If there are no
* unprocessed items remaining, the response contains an empty
* UnprocessedItems map.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 25
*
* @param unprocessedItems A map of tables and requests against those tables that were not
* processed. The UnprocessedItems value is in the same form as
* RequestItems, so you can provide this value directly to a
* subsequent BatchGetItem operation. For more information, see
* RequestItems in the Request Parameters section.
Each
* UnprocessedItems entry consists of a table name and, for that
* table, a list of operations to perform (DeleteRequest or
* PutRequest).
-
DeleteRequest - Perform a
* DeleteItem operation on the specified item. The item to be
* deleted is identified by a Key subelement:
-
*
Key - A map of primary key attribute values that uniquely
* identify the item. Each entry in this map consists of an attribute
* name and an attribute value.
-
*
PutRequest - Perform a PutItem operation on the
* specified item. The item to be put is identified by an Item
* subelement:
-
Item - A map of attributes and their
* values. Each entry in this map consists of an attribute name and an
* attribute value. Attribute values must not be null; string and binary
* type attributes must have lengths greater than zero; and set type
* attributes must not be empty. Requests that contain empty values will
* be rejected with a ValidationException.
If you specify any
* attributes that are part of an index key, then the data types for
* those attributes must match those of the schema in the table's
* attribute definition.
If there are no
* unprocessed items remaining, the response contains an empty
* UnprocessedItems map.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public BatchWriteItemResult withUnprocessedItems(java.util.Map> unprocessedItems) {
setUnprocessedItems(unprocessedItems);
return this;
}
/**
* A map of tables and requests against those tables that were not
* processed. The UnprocessedItems value is in the same form as
* RequestItems, so you can provide this value directly to a
* subsequent BatchGetItem operation. For more information, see
* RequestItems in the Request Parameters section. Each
* UnprocessedItems entry consists of a table name and, for that
* table, a list of operations to perform (DeleteRequest or
* PutRequest).
-
DeleteRequest - Perform a
* DeleteItem operation on the specified item. The item to be
* deleted is identified by a Key subelement:
-
*
Key - A map of primary key attribute values that uniquely
* identify the item. Each entry in this map consists of an attribute
* name and an attribute value.
-
*
PutRequest - Perform a PutItem operation on the
* specified item. The item to be put is identified by an Item
* subelement:
-
Item - A map of attributes and their
* values. Each entry in this map consists of an attribute name and an
* attribute value. Attribute values must not be null; string and binary
* type attributes must have lengths greater than zero; and set type
* attributes must not be empty. Requests that contain empty values will
* be rejected with a ValidationException.
If you specify any
* attributes that are part of an index key, then the data types for
* those attributes must match those of the schema in the table's
* attribute definition.
If there are no
* unprocessed items remaining, the response contains an empty
* UnprocessedItems map.
*
* The method adds a new key-value pair into UnprocessedItems parameter,
* and returns a reference to this object so that method calls can be
* chained together.
*
* Constraints:
* Length: 1 - 25
*
* @param key The key of the entry to be added into UnprocessedItems.
* @param value The corresponding value of the entry to be added into UnprocessedItems.
*/
public BatchWriteItemResult addUnprocessedItemsEntry(String key, java.util.List value) {
if (null == this.unprocessedItems) {
this.unprocessedItems = new java.util.HashMap>();
}
if (this.unprocessedItems.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.unprocessedItems.put(key, value);
return this;
}
/**
* Removes all the entries added into UnprocessedItems.
*
* Returns a reference to this object so that method calls can be chained together.
*/
public BatchWriteItemResult clearUnprocessedItemsEntries() {
this.unprocessedItems = null;
return this;
}
/**
* A list of tables that were processed by BatchWriteItem and, for
* each table, information about any item collections that were affected
* by individual DeleteItem or PutItem operations.
Each
* entry consists of the following subelements:
-
*
ItemCollectionKey - The hash key value of the item
* collection. This is the same as the hash key of the item.
-
*
SizeEstimateRange - An estimate of item collection size,
* expressed in GB. This is a two-element array containing a lower bound
* and an upper bound for the estimate. The estimate includes the size of
* all the items in the table, plus the size of all attributes projected
* into all of the local secondary indexes on the table. Use this
* estimate to measure whether a local secondary index is approaching its
* size limit.
The estimate is subject to change over time; therefore,
* do not rely on the precision or accuracy of the estimate.
*
* @return A list of tables that were processed by BatchWriteItem and, for
* each table, information about any item collections that were affected
* by individual DeleteItem or PutItem operations. Each
* entry consists of the following subelements:
-
*
ItemCollectionKey - The hash key value of the item
* collection. This is the same as the hash key of the item.
-
*
SizeEstimateRange - An estimate of item collection size,
* expressed in GB. This is a two-element array containing a lower bound
* and an upper bound for the estimate. The estimate includes the size of
* all the items in the table, plus the size of all attributes projected
* into all of the local secondary indexes on the table. Use this
* estimate to measure whether a local secondary index is approaching its
* size limit.
The estimate is subject to change over time; therefore,
* do not rely on the precision or accuracy of the estimate.
*/
public java.util.Map> getItemCollectionMetrics() {
return itemCollectionMetrics;
}
/**
* A list of tables that were processed by BatchWriteItem and, for
* each table, information about any item collections that were affected
* by individual DeleteItem or PutItem operations. Each
* entry consists of the following subelements:
-
*
ItemCollectionKey - The hash key value of the item
* collection. This is the same as the hash key of the item.
-
*
SizeEstimateRange - An estimate of item collection size,
* expressed in GB. This is a two-element array containing a lower bound
* and an upper bound for the estimate. The estimate includes the size of
* all the items in the table, plus the size of all attributes projected
* into all of the local secondary indexes on the table. Use this
* estimate to measure whether a local secondary index is approaching its
* size limit.
The estimate is subject to change over time; therefore,
* do not rely on the precision or accuracy of the estimate.
*
* @param itemCollectionMetrics A list of tables that were processed by BatchWriteItem and, for
* each table, information about any item collections that were affected
* by individual DeleteItem or PutItem operations. Each
* entry consists of the following subelements:
-
*
ItemCollectionKey - The hash key value of the item
* collection. This is the same as the hash key of the item.
-
*
SizeEstimateRange - An estimate of item collection size,
* expressed in GB. This is a two-element array containing a lower bound
* and an upper bound for the estimate. The estimate includes the size of
* all the items in the table, plus the size of all attributes projected
* into all of the local secondary indexes on the table. Use this
* estimate to measure whether a local secondary index is approaching its
* size limit.
The estimate is subject to change over time; therefore,
* do not rely on the precision or accuracy of the estimate.
*/
public void setItemCollectionMetrics(java.util.Map> itemCollectionMetrics) {
this.itemCollectionMetrics = itemCollectionMetrics;
}
/**
* A list of tables that were processed by BatchWriteItem and, for
* each table, information about any item collections that were affected
* by individual DeleteItem or PutItem operations. Each
* entry consists of the following subelements:
-
*
ItemCollectionKey - The hash key value of the item
* collection. This is the same as the hash key of the item.
-
*
SizeEstimateRange - An estimate of item collection size,
* expressed in GB. This is a two-element array containing a lower bound
* and an upper bound for the estimate. The estimate includes the size of
* all the items in the table, plus the size of all attributes projected
* into all of the local secondary indexes on the table. Use this
* estimate to measure whether a local secondary index is approaching its
* size limit.
The estimate is subject to change over time; therefore,
* do not rely on the precision or accuracy of the estimate.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param itemCollectionMetrics A list of tables that were processed by BatchWriteItem and, for
* each table, information about any item collections that were affected
* by individual DeleteItem or PutItem operations.
Each
* entry consists of the following subelements:
-
*
ItemCollectionKey - The hash key value of the item
* collection. This is the same as the hash key of the item.
-
*
SizeEstimateRange - An estimate of item collection size,
* expressed in GB. This is a two-element array containing a lower bound
* and an upper bound for the estimate. The estimate includes the size of
* all the items in the table, plus the size of all attributes projected
* into all of the local secondary indexes on the table. Use this
* estimate to measure whether a local secondary index is approaching its
* size limit.
The estimate is subject to change over time; therefore,
* do not rely on the precision or accuracy of the estimate.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public BatchWriteItemResult withItemCollectionMetrics(java.util.Map> itemCollectionMetrics) {
setItemCollectionMetrics(itemCollectionMetrics);
return this;
}
/**
* A list of tables that were processed by BatchWriteItem and, for
* each table, information about any item collections that were affected
* by individual DeleteItem or PutItem operations. Each
* entry consists of the following subelements:
-
*
ItemCollectionKey - The hash key value of the item
* collection. This is the same as the hash key of the item.
-
*
SizeEstimateRange - An estimate of item collection size,
* expressed in GB. This is a two-element array containing a lower bound
* and an upper bound for the estimate. The estimate includes the size of
* all the items in the table, plus the size of all attributes projected
* into all of the local secondary indexes on the table. Use this
* estimate to measure whether a local secondary index is approaching its
* size limit.
The estimate is subject to change over time; therefore,
* do not rely on the precision or accuracy of the estimate.
*
* The method adds a new key-value pair into ItemCollectionMetrics
* parameter, and returns a reference to this object so that method calls
* can be chained together.
*
* @param key The key of the entry to be added into ItemCollectionMetrics.
* @param value The corresponding value of the entry to be added into ItemCollectionMetrics.
*/
public BatchWriteItemResult addItemCollectionMetricsEntry(String key, java.util.List value) {
if (null == this.itemCollectionMetrics) {
this.itemCollectionMetrics = new java.util.HashMap>();
}
if (this.itemCollectionMetrics.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.itemCollectionMetrics.put(key, value);
return this;
}
/**
* Removes all the entries added into ItemCollectionMetrics.
*
* Returns a reference to this object so that method calls can be chained together.
*/
public BatchWriteItemResult clearItemCollectionMetricsEntries() {
this.itemCollectionMetrics = null;
return this;
}
/**
* The capacity units consumed by the operation.
Each element consists
* of:
-
TableName - The table that consumed the
* provisioned throughput.
-
CapacityUnits - The total
* number of capacity units consumed.
*
* @return The capacity units consumed by the operation. Each element consists
* of:
-
TableName - The table that consumed the
* provisioned throughput.
-
CapacityUnits - The total
* number of capacity units consumed.
*/
public java.util.List getConsumedCapacity() {
return consumedCapacity;
}
/**
* The capacity units consumed by the operation. Each element consists
* of:
-
TableName - The table that consumed the
* provisioned throughput.
-
CapacityUnits - The total
* number of capacity units consumed.
*
* @param consumedCapacity The capacity units consumed by the operation. Each element consists
* of:
-
TableName - The table that consumed the
* provisioned throughput.
-
CapacityUnits - The total
* number of capacity units consumed.
*/
public void setConsumedCapacity(java.util.Collection consumedCapacity) {
if (consumedCapacity == null) {
this.consumedCapacity = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag consumedCapacityCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(consumedCapacity.size());
consumedCapacityCopy.addAll(consumedCapacity);
this.consumedCapacity = consumedCapacityCopy;
}
/**
* The capacity units consumed by the operation. Each element consists
* of:
-
TableName - The table that consumed the
* provisioned throughput.
-
CapacityUnits - The total
* number of capacity units consumed.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param consumedCapacity The capacity units consumed by the operation.
Each element consists
* of:
-
TableName - The table that consumed the
* provisioned throughput.
-
CapacityUnits - The total
* number of capacity units consumed.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public BatchWriteItemResult withConsumedCapacity(ConsumedCapacity... consumedCapacity) {
if (getConsumedCapacity() == null) setConsumedCapacity(new java.util.ArrayList(consumedCapacity.length));
for (ConsumedCapacity value : consumedCapacity) {
getConsumedCapacity().add(value);
}
return this;
}
/**
* The capacity units consumed by the operation. Each element consists
* of:
-
TableName - The table that consumed the
* provisioned throughput.
-
CapacityUnits - The total
* number of capacity units consumed.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param consumedCapacity The capacity units consumed by the operation.
Each element consists
* of:
-
TableName - The table that consumed the
* provisioned throughput.
-
CapacityUnits - The total
* number of capacity units consumed.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public BatchWriteItemResult withConsumedCapacity(java.util.Collection consumedCapacity) {
if (consumedCapacity == null) {
this.consumedCapacity = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag consumedCapacityCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(consumedCapacity.size());
consumedCapacityCopy.addAll(consumedCapacity);
this.consumedCapacity = consumedCapacityCopy;
}
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getUnprocessedItems() != null) sb.append("UnprocessedItems: " + getUnprocessedItems() + ",");
if (getItemCollectionMetrics() != null) sb.append("ItemCollectionMetrics: " + getItemCollectionMetrics() + ",");
if (getConsumedCapacity() != null) sb.append("ConsumedCapacity: " + getConsumedCapacity() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getUnprocessedItems() == null) ? 0 : getUnprocessedItems().hashCode());
hashCode = prime * hashCode + ((getItemCollectionMetrics() == null) ? 0 : getItemCollectionMetrics().hashCode());
hashCode = prime * hashCode + ((getConsumedCapacity() == null) ? 0 : getConsumedCapacity().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof BatchWriteItemResult == false) return false;
BatchWriteItemResult other = (BatchWriteItemResult)obj;
if (other.getUnprocessedItems() == null ^ this.getUnprocessedItems() == null) return false;
if (other.getUnprocessedItems() != null && other.getUnprocessedItems().equals(this.getUnprocessedItems()) == false) return false;
if (other.getItemCollectionMetrics() == null ^ this.getItemCollectionMetrics() == null) return false;
if (other.getItemCollectionMetrics() != null && other.getItemCollectionMetrics().equals(this.getItemCollectionMetrics()) == false) return false;
if (other.getConsumedCapacity() == null ^ this.getConsumedCapacity() == null) return false;
if (other.getConsumedCapacity() != null && other.getConsumedCapacity().equals(this.getConsumedCapacity()) == false) return false;
return true;
}
}