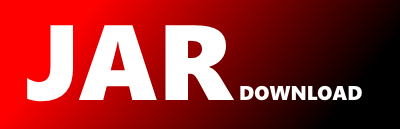
com.amazonaws.services.dynamodbv2.model.CreateTableRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.dynamodbv2.model;
import java.io.Serializable;
import com.amazonaws.AmazonWebServiceRequest;
/**
* Container for the parameters to the {@link com.amazonaws.services.dynamodbv2.AmazonDynamoDB#createTable(CreateTableRequest) CreateTable operation}.
*
* The CreateTable operation adds a new table to your account. In
* an AWS account, table names must be unique within each region. That
* is, you can have two tables with same name if you create the tables in
* different regions.
*
*
* CreateTable is an asynchronous operation. Upon receiving a
* CreateTable request, DynamoDB immediately returns a response
* with a TableStatus of CREATING
. After the table
* is created, DynamoDB sets the TableStatus to
* ACTIVE
. You can perform read and write operations only
* on an ACTIVE
table.
*
*
* If you want to create multiple tables with secondary indexes on them,
* you must create them sequentially. Only one table with secondary
* indexes can be in the CREATING
state at any given time.
*
*
* You can use the DescribeTable API to check the table status.
*
*
* @see com.amazonaws.services.dynamodbv2.AmazonDynamoDB#createTable(CreateTableRequest)
*/
public class CreateTableRequest extends AmazonWebServiceRequest implements Serializable {
/**
* An array of attributes that describe the key schema for the table and
* indexes.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag attributeDefinitions;
/**
* The name of the table to create.
*
* Constraints:
* Length: 3 - 255
* Pattern: [a-zA-Z0-9_.-]+
*/
private String tableName;
/**
* Specifies the attributes that make up the primary key for a table or
* an index. The attributes in KeySchema must also be defined in
* the AttributeDefinitions array. For more information, see Data
* Model in the Amazon DynamoDB Developer Guide.
Each
* KeySchemaElement in the array is composed of:
-
*
AttributeName - The name of this key attribute.
-
*
KeyType - Determines whether the key attribute is
* HASH
or RANGE
.
For a primary
* key that consists of a hash attribute, you must specify exactly one
* element with a KeyType of HASH
.
For a primary
* key that consists of hash and range attributes, you must specify
* exactly two elements, in this order: The first element must have a
* KeyType of HASH
, and the second element must have
* a KeyType of RANGE
.
For more information, see
* Specifying
* the Primary Key in the Amazon DynamoDB Developer Guide.
*
* Constraints:
* Length: 1 - 2
*/
private com.amazonaws.internal.ListWithAutoConstructFlag keySchema;
/**
* One or more local secondary indexes (the maximum is five) to be
* created on the table. Each index is scoped to a given hash key value.
* There is a 10 GB size limit per hash key; otherwise, the size of a
* local secondary index is unconstrained. Each local secondary index
* in the array includes the following:
-
IndexName -
* The name of the local secondary index. Must be unique only for this
* table.
-
KeySchema - Specifies the key schema
* for the local secondary index. The key schema must begin with the same
* hash key attribute as the table.
-
Projection -
* Specifies attributes that are copied (projected) from the table into
* the index. These are in addition to the primary key attributes and
* index key attributes, which are automatically projected. Each
* attribute specification is composed of:
-
*
ProjectionType - One of the following:
-
*
KEYS_ONLY
- Only the index and primary keys are
* projected into the index.
-
INCLUDE
- Only
* the specified table attributes are projected into the index. The list
* of projected attributes are in NonKeyAttributes.
-
*
ALL
- All of the table attributes are projected into
* the index.
-
NonKeyAttributes - A list
* of one or more non-key attribute names that are projected into the
* secondary index. The total count of attributes specified in
* NonKeyAttributes, summed across all of the secondary indexes,
* must not exceed 20. If you project the same attribute into two
* different indexes, this counts as two distinct attributes when
* determining the total.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag localSecondaryIndexes;
/**
* One or more global secondary indexes (the maximum is five) to be
* created on the table. Each global secondary index in the array
* includes the following: -
IndexName - The name of
* the global secondary index. Must be unique only for this table.
* -
KeySchema - Specifies the key schema for the
* global secondary index.
-
Projection - Specifies
* attributes that are copied (projected) from the table into the index.
* These are in addition to the primary key attributes and index key
* attributes, which are automatically projected. Each attribute
* specification is composed of:
-
ProjectionType - One
* of the following:
-
KEYS_ONLY
- Only the index
* and primary keys are projected into the index.
-
*
INCLUDE
- Only the specified table attributes are
* projected into the index. The list of projected attributes are in
* NonKeyAttributes.
-
ALL
- All of the
* table attributes are projected into the index.
-
*
NonKeyAttributes - A list of one or more non-key attribute
* names that are projected into the secondary index. The total count of
* attributes specified in NonKeyAttributes, summed across all of
* the secondary indexes, must not exceed 20. If you project the same
* attribute into two different indexes, this counts as two distinct
* attributes when determining the total.
-
*
ProvisionedThroughput - The provisioned throughput settings
* for the global secondary index, consisting of read and write capacity
* units.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag globalSecondaryIndexes;
/**
* Represents the provisioned throughput settings for a specified table
* or index. The settings can be modified using the UpdateTable
* operation. For current minimum and maximum provisioned throughput
* values, see Limits
* in the Amazon DynamoDB Developer Guide.
*/
private ProvisionedThroughput provisionedThroughput;
/**
* Default constructor for a new CreateTableRequest object. Callers should use the
* setter or fluent setter (with...) methods to initialize this object after creating it.
*/
public CreateTableRequest() {}
/**
* Constructs a new CreateTableRequest object.
* Callers should use the setter or fluent setter (with...) methods to
* initialize any additional object members.
*
* @param tableName The name of the table to create.
* @param keySchema Specifies the attributes that make up the primary key
* for a table or an index. The attributes in KeySchema must also
* be defined in the AttributeDefinitions array. For more
* information, see Data
* Model in the Amazon DynamoDB Developer Guide.
Each
* KeySchemaElement in the array is composed of:
-
*
AttributeName - The name of this key attribute.
-
*
KeyType - Determines whether the key attribute is
* HASH
or RANGE
.
For a primary
* key that consists of a hash attribute, you must specify exactly one
* element with a KeyType of HASH
.
For a primary
* key that consists of hash and range attributes, you must specify
* exactly two elements, in this order: The first element must have a
* KeyType of HASH
, and the second element must have
* a KeyType of RANGE
.
For more information, see
* Specifying
* the Primary Key in the Amazon DynamoDB Developer Guide.
*/
public CreateTableRequest(String tableName, java.util.List keySchema) {
setTableName(tableName);
setKeySchema(keySchema);
}
/**
* Constructs a new CreateTableRequest object.
* Callers should use the setter or fluent setter (with...) methods to
* initialize any additional object members.
*
* @param attributeDefinitions An array of attributes that describe the
* key schema for the table and indexes.
* @param tableName The name of the table to create.
* @param keySchema Specifies the attributes that make up the primary key
* for a table or an index. The attributes in KeySchema must also
* be defined in the AttributeDefinitions array. For more
* information, see Data
* Model in the Amazon DynamoDB Developer Guide. Each
* KeySchemaElement in the array is composed of:
-
*
AttributeName - The name of this key attribute.
-
*
KeyType - Determines whether the key attribute is
* HASH
or RANGE
.
For a primary
* key that consists of a hash attribute, you must specify exactly one
* element with a KeyType of HASH
.
For a primary
* key that consists of hash and range attributes, you must specify
* exactly two elements, in this order: The first element must have a
* KeyType of HASH
, and the second element must have
* a KeyType of RANGE
.
For more information, see
* Specifying
* the Primary Key in the Amazon DynamoDB Developer Guide.
* @param provisionedThroughput Represents the provisioned throughput
* settings for a specified table or index. The settings can be modified
* using the UpdateTable operation.
For current minimum and
* maximum provisioned throughput values, see Limits
* in the Amazon DynamoDB Developer Guide.
*/
public CreateTableRequest(java.util.List attributeDefinitions, String tableName, java.util.List keySchema, ProvisionedThroughput provisionedThroughput) {
setAttributeDefinitions(attributeDefinitions);
setTableName(tableName);
setKeySchema(keySchema);
setProvisionedThroughput(provisionedThroughput);
}
/**
* An array of attributes that describe the key schema for the table and
* indexes.
*
* @return An array of attributes that describe the key schema for the table and
* indexes.
*/
public java.util.List getAttributeDefinitions() {
return attributeDefinitions;
}
/**
* An array of attributes that describe the key schema for the table and
* indexes.
*
* @param attributeDefinitions An array of attributes that describe the key schema for the table and
* indexes.
*/
public void setAttributeDefinitions(java.util.Collection attributeDefinitions) {
if (attributeDefinitions == null) {
this.attributeDefinitions = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag attributeDefinitionsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(attributeDefinitions.size());
attributeDefinitionsCopy.addAll(attributeDefinitions);
this.attributeDefinitions = attributeDefinitionsCopy;
}
/**
* An array of attributes that describe the key schema for the table and
* indexes.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param attributeDefinitions An array of attributes that describe the key schema for the table and
* indexes.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateTableRequest withAttributeDefinitions(AttributeDefinition... attributeDefinitions) {
if (getAttributeDefinitions() == null) setAttributeDefinitions(new java.util.ArrayList(attributeDefinitions.length));
for (AttributeDefinition value : attributeDefinitions) {
getAttributeDefinitions().add(value);
}
return this;
}
/**
* An array of attributes that describe the key schema for the table and
* indexes.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param attributeDefinitions An array of attributes that describe the key schema for the table and
* indexes.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateTableRequest withAttributeDefinitions(java.util.Collection attributeDefinitions) {
if (attributeDefinitions == null) {
this.attributeDefinitions = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag attributeDefinitionsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(attributeDefinitions.size());
attributeDefinitionsCopy.addAll(attributeDefinitions);
this.attributeDefinitions = attributeDefinitionsCopy;
}
return this;
}
/**
* The name of the table to create.
*
* Constraints:
* Length: 3 - 255
* Pattern: [a-zA-Z0-9_.-]+
*
* @return The name of the table to create.
*/
public String getTableName() {
return tableName;
}
/**
* The name of the table to create.
*
* Constraints:
* Length: 3 - 255
* Pattern: [a-zA-Z0-9_.-]+
*
* @param tableName The name of the table to create.
*/
public void setTableName(String tableName) {
this.tableName = tableName;
}
/**
* The name of the table to create.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 3 - 255
* Pattern: [a-zA-Z0-9_.-]+
*
* @param tableName The name of the table to create.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateTableRequest withTableName(String tableName) {
this.tableName = tableName;
return this;
}
/**
* Specifies the attributes that make up the primary key for a table or
* an index. The attributes in KeySchema must also be defined in
* the AttributeDefinitions array. For more information, see Data
* Model in the Amazon DynamoDB Developer Guide.
Each
* KeySchemaElement in the array is composed of:
-
*
AttributeName - The name of this key attribute.
-
*
KeyType - Determines whether the key attribute is
* HASH
or RANGE
.
For a primary
* key that consists of a hash attribute, you must specify exactly one
* element with a KeyType of HASH
.
For a primary
* key that consists of hash and range attributes, you must specify
* exactly two elements, in this order: The first element must have a
* KeyType of HASH
, and the second element must have
* a KeyType of RANGE
.
For more information, see
* Specifying
* the Primary Key in the Amazon DynamoDB Developer Guide.
*
* Constraints:
* Length: 1 - 2
*
* @return Specifies the attributes that make up the primary key for a table or
* an index. The attributes in KeySchema must also be defined in
* the AttributeDefinitions array. For more information, see Data
* Model in the Amazon DynamoDB Developer Guide.
Each
* KeySchemaElement in the array is composed of:
-
*
AttributeName - The name of this key attribute.
-
*
KeyType - Determines whether the key attribute is
* HASH
or RANGE
.
For a primary
* key that consists of a hash attribute, you must specify exactly one
* element with a KeyType of HASH
.
For a primary
* key that consists of hash and range attributes, you must specify
* exactly two elements, in this order: The first element must have a
* KeyType of HASH
, and the second element must have
* a KeyType of RANGE
.
For more information, see
* Specifying
* the Primary Key in the Amazon DynamoDB Developer Guide.
*/
public java.util.List getKeySchema() {
return keySchema;
}
/**
* Specifies the attributes that make up the primary key for a table or
* an index. The attributes in KeySchema must also be defined in
* the AttributeDefinitions array. For more information, see Data
* Model in the Amazon DynamoDB Developer Guide. Each
* KeySchemaElement in the array is composed of:
-
*
AttributeName - The name of this key attribute.
-
*
KeyType - Determines whether the key attribute is
* HASH
or RANGE
.
For a primary
* key that consists of a hash attribute, you must specify exactly one
* element with a KeyType of HASH
.
For a primary
* key that consists of hash and range attributes, you must specify
* exactly two elements, in this order: The first element must have a
* KeyType of HASH
, and the second element must have
* a KeyType of RANGE
.
For more information, see
* Specifying
* the Primary Key in the Amazon DynamoDB Developer Guide.
*
* Constraints:
* Length: 1 - 2
*
* @param keySchema Specifies the attributes that make up the primary key for a table or
* an index. The attributes in KeySchema must also be defined in
* the AttributeDefinitions array. For more information, see Data
* Model in the Amazon DynamoDB Developer Guide.
Each
* KeySchemaElement in the array is composed of:
-
*
AttributeName - The name of this key attribute.
-
*
KeyType - Determines whether the key attribute is
* HASH
or RANGE
.
For a primary
* key that consists of a hash attribute, you must specify exactly one
* element with a KeyType of HASH
.
For a primary
* key that consists of hash and range attributes, you must specify
* exactly two elements, in this order: The first element must have a
* KeyType of HASH
, and the second element must have
* a KeyType of RANGE
.
For more information, see
* Specifying
* the Primary Key in the Amazon DynamoDB Developer Guide.
*/
public void setKeySchema(java.util.Collection keySchema) {
if (keySchema == null) {
this.keySchema = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag keySchemaCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(keySchema.size());
keySchemaCopy.addAll(keySchema);
this.keySchema = keySchemaCopy;
}
/**
* Specifies the attributes that make up the primary key for a table or
* an index. The attributes in KeySchema must also be defined in
* the AttributeDefinitions array. For more information, see Data
* Model in the Amazon DynamoDB Developer Guide. Each
* KeySchemaElement in the array is composed of:
-
*
AttributeName - The name of this key attribute.
-
*
KeyType - Determines whether the key attribute is
* HASH
or RANGE
.
For a primary
* key that consists of a hash attribute, you must specify exactly one
* element with a KeyType of HASH
.
For a primary
* key that consists of hash and range attributes, you must specify
* exactly two elements, in this order: The first element must have a
* KeyType of HASH
, and the second element must have
* a KeyType of RANGE
.
For more information, see
* Specifying
* the Primary Key in the Amazon DynamoDB Developer Guide.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 2
*
* @param keySchema Specifies the attributes that make up the primary key for a table or
* an index. The attributes in KeySchema must also be defined in
* the AttributeDefinitions array. For more information, see Data
* Model in the Amazon DynamoDB Developer Guide.
Each
* KeySchemaElement in the array is composed of:
-
*
AttributeName - The name of this key attribute.
-
*
KeyType - Determines whether the key attribute is
* HASH
or RANGE
.
For a primary
* key that consists of a hash attribute, you must specify exactly one
* element with a KeyType of HASH
.
For a primary
* key that consists of hash and range attributes, you must specify
* exactly two elements, in this order: The first element must have a
* KeyType of HASH
, and the second element must have
* a KeyType of RANGE
.
For more information, see
* Specifying
* the Primary Key in the Amazon DynamoDB Developer Guide.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateTableRequest withKeySchema(KeySchemaElement... keySchema) {
if (getKeySchema() == null) setKeySchema(new java.util.ArrayList(keySchema.length));
for (KeySchemaElement value : keySchema) {
getKeySchema().add(value);
}
return this;
}
/**
* Specifies the attributes that make up the primary key for a table or
* an index. The attributes in KeySchema must also be defined in
* the AttributeDefinitions array. For more information, see Data
* Model in the Amazon DynamoDB Developer Guide. Each
* KeySchemaElement in the array is composed of:
-
*
AttributeName - The name of this key attribute.
-
*
KeyType - Determines whether the key attribute is
* HASH
or RANGE
.
For a primary
* key that consists of a hash attribute, you must specify exactly one
* element with a KeyType of HASH
.
For a primary
* key that consists of hash and range attributes, you must specify
* exactly two elements, in this order: The first element must have a
* KeyType of HASH
, and the second element must have
* a KeyType of RANGE
.
For more information, see
* Specifying
* the Primary Key in the Amazon DynamoDB Developer Guide.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 2
*
* @param keySchema Specifies the attributes that make up the primary key for a table or
* an index. The attributes in KeySchema must also be defined in
* the AttributeDefinitions array. For more information, see Data
* Model in the Amazon DynamoDB Developer Guide.
Each
* KeySchemaElement in the array is composed of:
-
*
AttributeName - The name of this key attribute.
-
*
KeyType - Determines whether the key attribute is
* HASH
or RANGE
.
For a primary
* key that consists of a hash attribute, you must specify exactly one
* element with a KeyType of HASH
.
For a primary
* key that consists of hash and range attributes, you must specify
* exactly two elements, in this order: The first element must have a
* KeyType of HASH
, and the second element must have
* a KeyType of RANGE
.
For more information, see
* Specifying
* the Primary Key in the Amazon DynamoDB Developer Guide.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateTableRequest withKeySchema(java.util.Collection keySchema) {
if (keySchema == null) {
this.keySchema = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag keySchemaCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(keySchema.size());
keySchemaCopy.addAll(keySchema);
this.keySchema = keySchemaCopy;
}
return this;
}
/**
* One or more local secondary indexes (the maximum is five) to be
* created on the table. Each index is scoped to a given hash key value.
* There is a 10 GB size limit per hash key; otherwise, the size of a
* local secondary index is unconstrained. Each local secondary index
* in the array includes the following:
-
IndexName -
* The name of the local secondary index. Must be unique only for this
* table.
-
KeySchema - Specifies the key schema
* for the local secondary index. The key schema must begin with the same
* hash key attribute as the table.
-
Projection -
* Specifies attributes that are copied (projected) from the table into
* the index. These are in addition to the primary key attributes and
* index key attributes, which are automatically projected. Each
* attribute specification is composed of:
-
*
ProjectionType - One of the following:
-
*
KEYS_ONLY
- Only the index and primary keys are
* projected into the index.
-
INCLUDE
- Only
* the specified table attributes are projected into the index. The list
* of projected attributes are in NonKeyAttributes.
-
*
ALL
- All of the table attributes are projected into
* the index.
-
NonKeyAttributes - A list
* of one or more non-key attribute names that are projected into the
* secondary index. The total count of attributes specified in
* NonKeyAttributes, summed across all of the secondary indexes,
* must not exceed 20. If you project the same attribute into two
* different indexes, this counts as two distinct attributes when
* determining the total.
*
* @return One or more local secondary indexes (the maximum is five) to be
* created on the table. Each index is scoped to a given hash key value.
* There is a 10 GB size limit per hash key; otherwise, the size of a
* local secondary index is unconstrained. Each local secondary index
* in the array includes the following:
-
IndexName -
* The name of the local secondary index. Must be unique only for this
* table.
-
KeySchema - Specifies the key schema
* for the local secondary index. The key schema must begin with the same
* hash key attribute as the table.
-
Projection -
* Specifies attributes that are copied (projected) from the table into
* the index. These are in addition to the primary key attributes and
* index key attributes, which are automatically projected. Each
* attribute specification is composed of:
-
*
ProjectionType - One of the following:
-
*
KEYS_ONLY
- Only the index and primary keys are
* projected into the index.
-
INCLUDE
- Only
* the specified table attributes are projected into the index. The list
* of projected attributes are in NonKeyAttributes.
-
*
ALL
- All of the table attributes are projected into
* the index.
-
NonKeyAttributes - A list
* of one or more non-key attribute names that are projected into the
* secondary index. The total count of attributes specified in
* NonKeyAttributes, summed across all of the secondary indexes,
* must not exceed 20. If you project the same attribute into two
* different indexes, this counts as two distinct attributes when
* determining the total.
*/
public java.util.List getLocalSecondaryIndexes() {
return localSecondaryIndexes;
}
/**
* One or more local secondary indexes (the maximum is five) to be
* created on the table. Each index is scoped to a given hash key value.
* There is a 10 GB size limit per hash key; otherwise, the size of a
* local secondary index is unconstrained. Each local secondary index
* in the array includes the following:
-
IndexName -
* The name of the local secondary index. Must be unique only for this
* table.
-
KeySchema - Specifies the key schema
* for the local secondary index. The key schema must begin with the same
* hash key attribute as the table.
-
Projection -
* Specifies attributes that are copied (projected) from the table into
* the index. These are in addition to the primary key attributes and
* index key attributes, which are automatically projected. Each
* attribute specification is composed of:
-
*
ProjectionType - One of the following:
-
*
KEYS_ONLY
- Only the index and primary keys are
* projected into the index.
-
INCLUDE
- Only
* the specified table attributes are projected into the index. The list
* of projected attributes are in NonKeyAttributes.
-
*
ALL
- All of the table attributes are projected into
* the index.
-
NonKeyAttributes - A list
* of one or more non-key attribute names that are projected into the
* secondary index. The total count of attributes specified in
* NonKeyAttributes, summed across all of the secondary indexes,
* must not exceed 20. If you project the same attribute into two
* different indexes, this counts as two distinct attributes when
* determining the total.
*
* @param localSecondaryIndexes One or more local secondary indexes (the maximum is five) to be
* created on the table. Each index is scoped to a given hash key value.
* There is a 10 GB size limit per hash key; otherwise, the size of a
* local secondary index is unconstrained. Each local secondary index
* in the array includes the following:
-
IndexName -
* The name of the local secondary index. Must be unique only for this
* table.
-
KeySchema - Specifies the key schema
* for the local secondary index. The key schema must begin with the same
* hash key attribute as the table.
-
Projection -
* Specifies attributes that are copied (projected) from the table into
* the index. These are in addition to the primary key attributes and
* index key attributes, which are automatically projected. Each
* attribute specification is composed of:
-
*
ProjectionType - One of the following:
-
*
KEYS_ONLY
- Only the index and primary keys are
* projected into the index.
-
INCLUDE
- Only
* the specified table attributes are projected into the index. The list
* of projected attributes are in NonKeyAttributes.
-
*
ALL
- All of the table attributes are projected into
* the index.
-
NonKeyAttributes - A list
* of one or more non-key attribute names that are projected into the
* secondary index. The total count of attributes specified in
* NonKeyAttributes, summed across all of the secondary indexes,
* must not exceed 20. If you project the same attribute into two
* different indexes, this counts as two distinct attributes when
* determining the total.
*/
public void setLocalSecondaryIndexes(java.util.Collection localSecondaryIndexes) {
if (localSecondaryIndexes == null) {
this.localSecondaryIndexes = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag localSecondaryIndexesCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(localSecondaryIndexes.size());
localSecondaryIndexesCopy.addAll(localSecondaryIndexes);
this.localSecondaryIndexes = localSecondaryIndexesCopy;
}
/**
* One or more local secondary indexes (the maximum is five) to be
* created on the table. Each index is scoped to a given hash key value.
* There is a 10 GB size limit per hash key; otherwise, the size of a
* local secondary index is unconstrained. Each local secondary index
* in the array includes the following:
-
IndexName -
* The name of the local secondary index. Must be unique only for this
* table.
-
KeySchema - Specifies the key schema
* for the local secondary index. The key schema must begin with the same
* hash key attribute as the table.
-
Projection -
* Specifies attributes that are copied (projected) from the table into
* the index. These are in addition to the primary key attributes and
* index key attributes, which are automatically projected. Each
* attribute specification is composed of:
-
*
ProjectionType - One of the following:
-
*
KEYS_ONLY
- Only the index and primary keys are
* projected into the index.
-
INCLUDE
- Only
* the specified table attributes are projected into the index. The list
* of projected attributes are in NonKeyAttributes.
-
*
ALL
- All of the table attributes are projected into
* the index.
-
NonKeyAttributes - A list
* of one or more non-key attribute names that are projected into the
* secondary index. The total count of attributes specified in
* NonKeyAttributes, summed across all of the secondary indexes,
* must not exceed 20. If you project the same attribute into two
* different indexes, this counts as two distinct attributes when
* determining the total.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param localSecondaryIndexes One or more local secondary indexes (the maximum is five) to be
* created on the table. Each index is scoped to a given hash key value.
* There is a 10 GB size limit per hash key; otherwise, the size of a
* local secondary index is unconstrained.
Each local secondary index
* in the array includes the following:
-
IndexName -
* The name of the local secondary index. Must be unique only for this
* table.
-
KeySchema - Specifies the key schema
* for the local secondary index. The key schema must begin with the same
* hash key attribute as the table.
-
Projection -
* Specifies attributes that are copied (projected) from the table into
* the index. These are in addition to the primary key attributes and
* index key attributes, which are automatically projected. Each
* attribute specification is composed of:
-
*
ProjectionType - One of the following:
-
*
KEYS_ONLY
- Only the index and primary keys are
* projected into the index.
-
INCLUDE
- Only
* the specified table attributes are projected into the index. The list
* of projected attributes are in NonKeyAttributes.
-
*
ALL
- All of the table attributes are projected into
* the index.
-
NonKeyAttributes - A list
* of one or more non-key attribute names that are projected into the
* secondary index. The total count of attributes specified in
* NonKeyAttributes, summed across all of the secondary indexes,
* must not exceed 20. If you project the same attribute into two
* different indexes, this counts as two distinct attributes when
* determining the total.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateTableRequest withLocalSecondaryIndexes(LocalSecondaryIndex... localSecondaryIndexes) {
if (getLocalSecondaryIndexes() == null) setLocalSecondaryIndexes(new java.util.ArrayList(localSecondaryIndexes.length));
for (LocalSecondaryIndex value : localSecondaryIndexes) {
getLocalSecondaryIndexes().add(value);
}
return this;
}
/**
* One or more local secondary indexes (the maximum is five) to be
* created on the table. Each index is scoped to a given hash key value.
* There is a 10 GB size limit per hash key; otherwise, the size of a
* local secondary index is unconstrained. Each local secondary index
* in the array includes the following:
-
IndexName -
* The name of the local secondary index. Must be unique only for this
* table.
-
KeySchema - Specifies the key schema
* for the local secondary index. The key schema must begin with the same
* hash key attribute as the table.
-
Projection -
* Specifies attributes that are copied (projected) from the table into
* the index. These are in addition to the primary key attributes and
* index key attributes, which are automatically projected. Each
* attribute specification is composed of:
-
*
ProjectionType - One of the following:
-
*
KEYS_ONLY
- Only the index and primary keys are
* projected into the index.
-
INCLUDE
- Only
* the specified table attributes are projected into the index. The list
* of projected attributes are in NonKeyAttributes.
-
*
ALL
- All of the table attributes are projected into
* the index.
-
NonKeyAttributes - A list
* of one or more non-key attribute names that are projected into the
* secondary index. The total count of attributes specified in
* NonKeyAttributes, summed across all of the secondary indexes,
* must not exceed 20. If you project the same attribute into two
* different indexes, this counts as two distinct attributes when
* determining the total.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param localSecondaryIndexes One or more local secondary indexes (the maximum is five) to be
* created on the table. Each index is scoped to a given hash key value.
* There is a 10 GB size limit per hash key; otherwise, the size of a
* local secondary index is unconstrained.
Each local secondary index
* in the array includes the following:
-
IndexName -
* The name of the local secondary index. Must be unique only for this
* table.
-
KeySchema - Specifies the key schema
* for the local secondary index. The key schema must begin with the same
* hash key attribute as the table.
-
Projection -
* Specifies attributes that are copied (projected) from the table into
* the index. These are in addition to the primary key attributes and
* index key attributes, which are automatically projected. Each
* attribute specification is composed of:
-
*
ProjectionType - One of the following:
-
*
KEYS_ONLY
- Only the index and primary keys are
* projected into the index.
-
INCLUDE
- Only
* the specified table attributes are projected into the index. The list
* of projected attributes are in NonKeyAttributes.
-
*
ALL
- All of the table attributes are projected into
* the index.
-
NonKeyAttributes - A list
* of one or more non-key attribute names that are projected into the
* secondary index. The total count of attributes specified in
* NonKeyAttributes, summed across all of the secondary indexes,
* must not exceed 20. If you project the same attribute into two
* different indexes, this counts as two distinct attributes when
* determining the total.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateTableRequest withLocalSecondaryIndexes(java.util.Collection localSecondaryIndexes) {
if (localSecondaryIndexes == null) {
this.localSecondaryIndexes = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag localSecondaryIndexesCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(localSecondaryIndexes.size());
localSecondaryIndexesCopy.addAll(localSecondaryIndexes);
this.localSecondaryIndexes = localSecondaryIndexesCopy;
}
return this;
}
/**
* One or more global secondary indexes (the maximum is five) to be
* created on the table. Each global secondary index in the array
* includes the following: -
IndexName - The name of
* the global secondary index. Must be unique only for this table.
* -
KeySchema - Specifies the key schema for the
* global secondary index.
-
Projection - Specifies
* attributes that are copied (projected) from the table into the index.
* These are in addition to the primary key attributes and index key
* attributes, which are automatically projected. Each attribute
* specification is composed of:
-
ProjectionType - One
* of the following:
-
KEYS_ONLY
- Only the index
* and primary keys are projected into the index.
-
*
INCLUDE
- Only the specified table attributes are
* projected into the index. The list of projected attributes are in
* NonKeyAttributes.
-
ALL
- All of the
* table attributes are projected into the index.
-
*
NonKeyAttributes - A list of one or more non-key attribute
* names that are projected into the secondary index. The total count of
* attributes specified in NonKeyAttributes, summed across all of
* the secondary indexes, must not exceed 20. If you project the same
* attribute into two different indexes, this counts as two distinct
* attributes when determining the total.
-
*
ProvisionedThroughput - The provisioned throughput settings
* for the global secondary index, consisting of read and write capacity
* units.
*
* @return One or more global secondary indexes (the maximum is five) to be
* created on the table. Each global secondary index in the array
* includes the following: -
IndexName - The name of
* the global secondary index. Must be unique only for this table.
* -
KeySchema - Specifies the key schema for the
* global secondary index.
-
Projection - Specifies
* attributes that are copied (projected) from the table into the index.
* These are in addition to the primary key attributes and index key
* attributes, which are automatically projected. Each attribute
* specification is composed of:
-
ProjectionType - One
* of the following:
-
KEYS_ONLY
- Only the index
* and primary keys are projected into the index.
-
*
INCLUDE
- Only the specified table attributes are
* projected into the index. The list of projected attributes are in
* NonKeyAttributes.
-
ALL
- All of the
* table attributes are projected into the index.
-
*
NonKeyAttributes - A list of one or more non-key attribute
* names that are projected into the secondary index. The total count of
* attributes specified in NonKeyAttributes, summed across all of
* the secondary indexes, must not exceed 20. If you project the same
* attribute into two different indexes, this counts as two distinct
* attributes when determining the total.
-
*
ProvisionedThroughput - The provisioned throughput settings
* for the global secondary index, consisting of read and write capacity
* units.
*/
public java.util.List getGlobalSecondaryIndexes() {
return globalSecondaryIndexes;
}
/**
* One or more global secondary indexes (the maximum is five) to be
* created on the table. Each global secondary index in the array
* includes the following: -
IndexName - The name of
* the global secondary index. Must be unique only for this table.
* -
KeySchema - Specifies the key schema for the
* global secondary index.
-
Projection - Specifies
* attributes that are copied (projected) from the table into the index.
* These are in addition to the primary key attributes and index key
* attributes, which are automatically projected. Each attribute
* specification is composed of:
-
ProjectionType - One
* of the following:
-
KEYS_ONLY
- Only the index
* and primary keys are projected into the index.
-
*
INCLUDE
- Only the specified table attributes are
* projected into the index. The list of projected attributes are in
* NonKeyAttributes.
-
ALL
- All of the
* table attributes are projected into the index.
-
*
NonKeyAttributes - A list of one or more non-key attribute
* names that are projected into the secondary index. The total count of
* attributes specified in NonKeyAttributes, summed across all of
* the secondary indexes, must not exceed 20. If you project the same
* attribute into two different indexes, this counts as two distinct
* attributes when determining the total.
-
*
ProvisionedThroughput - The provisioned throughput settings
* for the global secondary index, consisting of read and write capacity
* units.
*
* @param globalSecondaryIndexes One or more global secondary indexes (the maximum is five) to be
* created on the table. Each global secondary index in the array
* includes the following: -
IndexName - The name of
* the global secondary index. Must be unique only for this table.
* -
KeySchema - Specifies the key schema for the
* global secondary index.
-
Projection - Specifies
* attributes that are copied (projected) from the table into the index.
* These are in addition to the primary key attributes and index key
* attributes, which are automatically projected. Each attribute
* specification is composed of:
-
ProjectionType - One
* of the following:
-
KEYS_ONLY
- Only the index
* and primary keys are projected into the index.
-
*
INCLUDE
- Only the specified table attributes are
* projected into the index. The list of projected attributes are in
* NonKeyAttributes.
-
ALL
- All of the
* table attributes are projected into the index.
-
*
NonKeyAttributes - A list of one or more non-key attribute
* names that are projected into the secondary index. The total count of
* attributes specified in NonKeyAttributes, summed across all of
* the secondary indexes, must not exceed 20. If you project the same
* attribute into two different indexes, this counts as two distinct
* attributes when determining the total.
-
*
ProvisionedThroughput - The provisioned throughput settings
* for the global secondary index, consisting of read and write capacity
* units.
*/
public void setGlobalSecondaryIndexes(java.util.Collection globalSecondaryIndexes) {
if (globalSecondaryIndexes == null) {
this.globalSecondaryIndexes = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag globalSecondaryIndexesCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(globalSecondaryIndexes.size());
globalSecondaryIndexesCopy.addAll(globalSecondaryIndexes);
this.globalSecondaryIndexes = globalSecondaryIndexesCopy;
}
/**
* One or more global secondary indexes (the maximum is five) to be
* created on the table. Each global secondary index in the array
* includes the following: -
IndexName - The name of
* the global secondary index. Must be unique only for this table.
* -
KeySchema - Specifies the key schema for the
* global secondary index.
-
Projection - Specifies
* attributes that are copied (projected) from the table into the index.
* These are in addition to the primary key attributes and index key
* attributes, which are automatically projected. Each attribute
* specification is composed of:
-
ProjectionType - One
* of the following:
-
KEYS_ONLY
- Only the index
* and primary keys are projected into the index.
-
*
INCLUDE
- Only the specified table attributes are
* projected into the index. The list of projected attributes are in
* NonKeyAttributes.
-
ALL
- All of the
* table attributes are projected into the index.
-
*
NonKeyAttributes - A list of one or more non-key attribute
* names that are projected into the secondary index. The total count of
* attributes specified in NonKeyAttributes, summed across all of
* the secondary indexes, must not exceed 20. If you project the same
* attribute into two different indexes, this counts as two distinct
* attributes when determining the total.
-
*
ProvisionedThroughput - The provisioned throughput settings
* for the global secondary index, consisting of read and write capacity
* units.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param globalSecondaryIndexes One or more global secondary indexes (the maximum is five) to be
* created on the table. Each global secondary index in the array
* includes the following:
-
IndexName - The name of
* the global secondary index. Must be unique only for this table.
* -
KeySchema - Specifies the key schema for the
* global secondary index.
-
Projection - Specifies
* attributes that are copied (projected) from the table into the index.
* These are in addition to the primary key attributes and index key
* attributes, which are automatically projected. Each attribute
* specification is composed of:
-
ProjectionType - One
* of the following:
-
KEYS_ONLY
- Only the index
* and primary keys are projected into the index.
-
*
INCLUDE
- Only the specified table attributes are
* projected into the index. The list of projected attributes are in
* NonKeyAttributes.
-
ALL
- All of the
* table attributes are projected into the index.
-
*
NonKeyAttributes - A list of one or more non-key attribute
* names that are projected into the secondary index. The total count of
* attributes specified in NonKeyAttributes, summed across all of
* the secondary indexes, must not exceed 20. If you project the same
* attribute into two different indexes, this counts as two distinct
* attributes when determining the total.
-
*
ProvisionedThroughput - The provisioned throughput settings
* for the global secondary index, consisting of read and write capacity
* units.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateTableRequest withGlobalSecondaryIndexes(GlobalSecondaryIndex... globalSecondaryIndexes) {
if (getGlobalSecondaryIndexes() == null) setGlobalSecondaryIndexes(new java.util.ArrayList(globalSecondaryIndexes.length));
for (GlobalSecondaryIndex value : globalSecondaryIndexes) {
getGlobalSecondaryIndexes().add(value);
}
return this;
}
/**
* One or more global secondary indexes (the maximum is five) to be
* created on the table. Each global secondary index in the array
* includes the following: -
IndexName - The name of
* the global secondary index. Must be unique only for this table.
* -
KeySchema - Specifies the key schema for the
* global secondary index.
-
Projection - Specifies
* attributes that are copied (projected) from the table into the index.
* These are in addition to the primary key attributes and index key
* attributes, which are automatically projected. Each attribute
* specification is composed of:
-
ProjectionType - One
* of the following:
-
KEYS_ONLY
- Only the index
* and primary keys are projected into the index.
-
*
INCLUDE
- Only the specified table attributes are
* projected into the index. The list of projected attributes are in
* NonKeyAttributes.
-
ALL
- All of the
* table attributes are projected into the index.
-
*
NonKeyAttributes - A list of one or more non-key attribute
* names that are projected into the secondary index. The total count of
* attributes specified in NonKeyAttributes, summed across all of
* the secondary indexes, must not exceed 20. If you project the same
* attribute into two different indexes, this counts as two distinct
* attributes when determining the total.
-
*
ProvisionedThroughput - The provisioned throughput settings
* for the global secondary index, consisting of read and write capacity
* units.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param globalSecondaryIndexes One or more global secondary indexes (the maximum is five) to be
* created on the table. Each global secondary index in the array
* includes the following:
-
IndexName - The name of
* the global secondary index. Must be unique only for this table.
* -
KeySchema - Specifies the key schema for the
* global secondary index.
-
Projection - Specifies
* attributes that are copied (projected) from the table into the index.
* These are in addition to the primary key attributes and index key
* attributes, which are automatically projected. Each attribute
* specification is composed of:
-
ProjectionType - One
* of the following:
-
KEYS_ONLY
- Only the index
* and primary keys are projected into the index.
-
*
INCLUDE
- Only the specified table attributes are
* projected into the index. The list of projected attributes are in
* NonKeyAttributes.
-
ALL
- All of the
* table attributes are projected into the index.
-
*
NonKeyAttributes - A list of one or more non-key attribute
* names that are projected into the secondary index. The total count of
* attributes specified in NonKeyAttributes, summed across all of
* the secondary indexes, must not exceed 20. If you project the same
* attribute into two different indexes, this counts as two distinct
* attributes when determining the total.
-
*
ProvisionedThroughput - The provisioned throughput settings
* for the global secondary index, consisting of read and write capacity
* units.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateTableRequest withGlobalSecondaryIndexes(java.util.Collection globalSecondaryIndexes) {
if (globalSecondaryIndexes == null) {
this.globalSecondaryIndexes = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag globalSecondaryIndexesCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(globalSecondaryIndexes.size());
globalSecondaryIndexesCopy.addAll(globalSecondaryIndexes);
this.globalSecondaryIndexes = globalSecondaryIndexesCopy;
}
return this;
}
/**
* Represents the provisioned throughput settings for a specified table
* or index. The settings can be modified using the UpdateTable
* operation. For current minimum and maximum provisioned throughput
* values, see Limits
* in the Amazon DynamoDB Developer Guide.
*
* @return Represents the provisioned throughput settings for a specified table
* or index. The settings can be modified using the UpdateTable
* operation.
For current minimum and maximum provisioned throughput
* values, see Limits
* in the Amazon DynamoDB Developer Guide.
*/
public ProvisionedThroughput getProvisionedThroughput() {
return provisionedThroughput;
}
/**
* Represents the provisioned throughput settings for a specified table
* or index. The settings can be modified using the UpdateTable
* operation.
For current minimum and maximum provisioned throughput
* values, see Limits
* in the Amazon DynamoDB Developer Guide.
*
* @param provisionedThroughput Represents the provisioned throughput settings for a specified table
* or index. The settings can be modified using the UpdateTable
* operation.
For current minimum and maximum provisioned throughput
* values, see Limits
* in the Amazon DynamoDB Developer Guide.
*/
public void setProvisionedThroughput(ProvisionedThroughput provisionedThroughput) {
this.provisionedThroughput = provisionedThroughput;
}
/**
* Represents the provisioned throughput settings for a specified table
* or index. The settings can be modified using the UpdateTable
* operation.
For current minimum and maximum provisioned throughput
* values, see Limits
* in the Amazon DynamoDB Developer Guide.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param provisionedThroughput Represents the provisioned throughput settings for a specified table
* or index. The settings can be modified using the UpdateTable
* operation.
For current minimum and maximum provisioned throughput
* values, see Limits
* in the Amazon DynamoDB Developer Guide.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateTableRequest withProvisionedThroughput(ProvisionedThroughput provisionedThroughput) {
this.provisionedThroughput = provisionedThroughput;
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAttributeDefinitions() != null) sb.append("AttributeDefinitions: " + getAttributeDefinitions() + ",");
if (getTableName() != null) sb.append("TableName: " + getTableName() + ",");
if (getKeySchema() != null) sb.append("KeySchema: " + getKeySchema() + ",");
if (getLocalSecondaryIndexes() != null) sb.append("LocalSecondaryIndexes: " + getLocalSecondaryIndexes() + ",");
if (getGlobalSecondaryIndexes() != null) sb.append("GlobalSecondaryIndexes: " + getGlobalSecondaryIndexes() + ",");
if (getProvisionedThroughput() != null) sb.append("ProvisionedThroughput: " + getProvisionedThroughput() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAttributeDefinitions() == null) ? 0 : getAttributeDefinitions().hashCode());
hashCode = prime * hashCode + ((getTableName() == null) ? 0 : getTableName().hashCode());
hashCode = prime * hashCode + ((getKeySchema() == null) ? 0 : getKeySchema().hashCode());
hashCode = prime * hashCode + ((getLocalSecondaryIndexes() == null) ? 0 : getLocalSecondaryIndexes().hashCode());
hashCode = prime * hashCode + ((getGlobalSecondaryIndexes() == null) ? 0 : getGlobalSecondaryIndexes().hashCode());
hashCode = prime * hashCode + ((getProvisionedThroughput() == null) ? 0 : getProvisionedThroughput().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof CreateTableRequest == false) return false;
CreateTableRequest other = (CreateTableRequest)obj;
if (other.getAttributeDefinitions() == null ^ this.getAttributeDefinitions() == null) return false;
if (other.getAttributeDefinitions() != null && other.getAttributeDefinitions().equals(this.getAttributeDefinitions()) == false) return false;
if (other.getTableName() == null ^ this.getTableName() == null) return false;
if (other.getTableName() != null && other.getTableName().equals(this.getTableName()) == false) return false;
if (other.getKeySchema() == null ^ this.getKeySchema() == null) return false;
if (other.getKeySchema() != null && other.getKeySchema().equals(this.getKeySchema()) == false) return false;
if (other.getLocalSecondaryIndexes() == null ^ this.getLocalSecondaryIndexes() == null) return false;
if (other.getLocalSecondaryIndexes() != null && other.getLocalSecondaryIndexes().equals(this.getLocalSecondaryIndexes()) == false) return false;
if (other.getGlobalSecondaryIndexes() == null ^ this.getGlobalSecondaryIndexes() == null) return false;
if (other.getGlobalSecondaryIndexes() != null && other.getGlobalSecondaryIndexes().equals(this.getGlobalSecondaryIndexes()) == false) return false;
if (other.getProvisionedThroughput() == null ^ this.getProvisionedThroughput() == null) return false;
if (other.getProvisionedThroughput() != null && other.getProvisionedThroughput().equals(this.getProvisionedThroughput()) == false) return false;
return true;
}
}