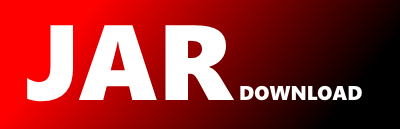
com.amazonaws.services.dynamodbv2.model.PutItemRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.dynamodbv2.model;
import java.io.Serializable;
import com.amazonaws.AmazonWebServiceRequest;
/**
* Container for the parameters to the {@link com.amazonaws.services.dynamodbv2.AmazonDynamoDB#putItem(PutItemRequest) PutItem operation}.
*
* Creates a new item, or replaces an old item with a new item. If an
* item already exists in the specified table with the same primary key,
* the new item completely replaces the existing item. You can perform a
* conditional put (insert a new item if one with the specified primary
* key doesn't exist), or replace an existing item if it has certain
* attribute values.
*
*
* In addition to putting an item, you can also return the item's
* attribute values in the same operation, using the ReturnValues
* parameter.
*
*
* When you add an item, the primary key attribute(s) are the only
* required attributes. Attribute values cannot be null. String and
* binary type attributes must have lengths greater than zero. Set type
* attributes cannot be empty. Requests with empty values will be
* rejected with a ValidationException .
*
*
* You can request that PutItem return either a copy of the old
* item (before the update) or a copy of the new item (after the update).
* For more information, see the ReturnValues description.
*
*
* NOTE: To prevent a new item from replacing an existing item,
* use a conditional put operation with Exists set to false for the
* primary key attribute, or attributes.
*
*
* For more information about using this API, see
* Working with Items
* in the Amazon DynamoDB Developer Guide.
*
*
* @see com.amazonaws.services.dynamodbv2.AmazonDynamoDB#putItem(PutItemRequest)
*/
public class PutItemRequest extends AmazonWebServiceRequest implements Serializable {
/**
* The name of the table to contain the item.
*
* Constraints:
* Length: 3 - 255
* Pattern: [a-zA-Z0-9_.-]+
*/
private String tableName;
/**
* A map of attribute name/value pairs, one for each attribute. Only the
* primary key attributes are required; you can optionally provide other
* attribute name-value pairs for the item.
If you specify any
* attributes that are part of an index key, then the data types for
* those attributes must match those of the schema in the table's
* attribute definition.
For more information about primary keys, see
* Primary
* Key in the Amazon DynamoDB Developer Guide.
Each element in the
* Item map is an AttributeValue object.
*/
private java.util.Map item;
/**
* A map of attribute/condition pairs. This is the conditional block for
* the PutItem operation. All the conditions must be met for the
* operation to succeed. Expected allows you to provide an
* attribute name, and whether or not DynamoDB should check to see if the
* attribute value already exists; or if the attribute value exists and
* has a particular value before changing it.
Each item in
* Expected represents an attribute name for DynamoDB to check,
* along with the following:
-
Value - A value for
* DynamoDB to compare with an attribute. When performing the comparison,
* strongly consistent reads are used.
-
Exists -
* Causes DynamoDB to evaluate the value before attempting a conditional
* operation:
-
If Exists is true
,
* DynamoDB will check to see if that attribute value already exists in
* the table. If it is found, then the operation succeeds. If it is not
* found, the operation fails with a
* ConditionalCheckFailedException.
-
If Exists
* is false
, DynamoDB assumes that the attribute value does
* not exist in the table. If in fact the value does not exist,
* then the assumption is valid and the operation succeeds. If the value
* is found, despite the assumption that it does not exist, the operation
* fails with a ConditionalCheckFailedException.
* The default setting for Exists is true
. If you
* supply a Value all by itself, DynamoDB assumes the attribute
* exists: You don't have to set Exists to true
,
* because it is implied.
DynamoDB returns a
* ValidationException if:
-
Exists is
* true
but there is no Value to check. (You expect a
* value to exist, but don't specify what that value is.)
-
*
Exists is false
but you also specify a
* Value. (You cannot expect an attribute to have a value, while
* also expecting it not to exist.)
If you
* specify more than one condition for Exists, then all of the
* conditions must evaluate to true. (In other words, the conditions are
* ANDed together.) Otherwise, the conditional operation will fail.
*/
private java.util.Map expected;
/**
* Use ReturnValues if you want to get the item attributes as they
* appeared before they were updated with the PutItem request. For
* PutItem, the valid values are: -
NONE
-
* If ReturnValues is not specified, or if its value is
* NONE
, then nothing is returned. (This is the default for
* ReturnValues.)
-
ALL_OLD
- If
* PutItem overwrote an attribute name-value pair, then the
* content of the old item is returned.
*
* Constraints:
* Allowed Values: NONE, ALL_OLD, UPDATED_OLD, ALL_NEW, UPDATED_NEW
*/
private String returnValues;
/**
* If set to TOTAL
, the response includes
* ConsumedCapacity data for tables and indexes. If set to
* INDEXES
, the response includes ConsumedCapacity
* for indexes. If set to NONE
(the default),
* ConsumedCapacity is not included in the response.
*
* Constraints:
* Allowed Values: INDEXES, TOTAL, NONE
*/
private String returnConsumedCapacity;
/**
* If set to SIZE
, statistics about item collections, if
* any, that were modified during the operation are returned in the
* response. If set to NONE
(the default), no statistics are
* returned.
*
* Constraints:
* Allowed Values: SIZE, NONE
*/
private String returnItemCollectionMetrics;
/**
* Default constructor for a new PutItemRequest object. Callers should use the
* setter or fluent setter (with...) methods to initialize this object after creating it.
*/
public PutItemRequest() {}
/**
* Constructs a new PutItemRequest object.
* Callers should use the setter or fluent setter (with...) methods to
* initialize any additional object members.
*
* @param tableName The name of the table to contain the item.
* @param item A map of attribute name/value pairs, one for each
* attribute. Only the primary key attributes are required; you can
* optionally provide other attribute name-value pairs for the item.
*
If you specify any attributes that are part of an index key, then
* the data types for those attributes must match those of the schema in
* the table's attribute definition.
For more information about
* primary keys, see Primary
* Key in the Amazon DynamoDB Developer Guide.
Each element in the
* Item map is an AttributeValue object.
*/
public PutItemRequest(String tableName, java.util.Map item) {
setTableName(tableName);
setItem(item);
}
/**
* Constructs a new PutItemRequest object.
* Callers should use the setter or fluent setter (with...) methods to
* initialize any additional object members.
*
* @param tableName The name of the table to contain the item.
* @param item A map of attribute name/value pairs, one for each
* attribute. Only the primary key attributes are required; you can
* optionally provide other attribute name-value pairs for the item.
* If you specify any attributes that are part of an index key, then
* the data types for those attributes must match those of the schema in
* the table's attribute definition.
For more information about
* primary keys, see Primary
* Key in the Amazon DynamoDB Developer Guide.
Each element in the
* Item map is an AttributeValue object.
* @param returnValues Use ReturnValues if you want to get the
* item attributes as they appeared before they were updated with the
* PutItem request. For PutItem, the valid values are:
* -
NONE
- If ReturnValues is not specified,
* or if its value is NONE
, then nothing is returned. (This
* is the default for ReturnValues.)
-
*
ALL_OLD
- If PutItem overwrote an attribute
* name-value pair, then the content of the old item is returned.
*
*/
public PutItemRequest(String tableName, java.util.Map item, String returnValues) {
setTableName(tableName);
setItem(item);
setReturnValues(returnValues);
}
/**
* Constructs a new PutItemRequest object.
* Callers should use the setter or fluent setter (with...) methods to
* initialize any additional object members.
*
* @param tableName The name of the table to contain the item.
* @param item A map of attribute name/value pairs, one for each
* attribute. Only the primary key attributes are required; you can
* optionally provide other attribute name-value pairs for the item.
* If you specify any attributes that are part of an index key, then
* the data types for those attributes must match those of the schema in
* the table's attribute definition.
For more information about
* primary keys, see Primary
* Key in the Amazon DynamoDB Developer Guide.
Each element in the
* Item map is an AttributeValue object.
* @param returnValues Use ReturnValues if you want to get the
* item attributes as they appeared before they were updated with the
* PutItem request. For PutItem, the valid values are:
* -
NONE
- If ReturnValues is not specified,
* or if its value is NONE
, then nothing is returned. (This
* is the default for ReturnValues.)
-
*
ALL_OLD
- If PutItem overwrote an attribute
* name-value pair, then the content of the old item is returned.
*
*/
public PutItemRequest(String tableName, java.util.Map item, ReturnValue returnValues) {
this.tableName = tableName;
this.item = item;
this.returnValues = returnValues.toString();
}
/**
* The name of the table to contain the item.
*
* Constraints:
* Length: 3 - 255
* Pattern: [a-zA-Z0-9_.-]+
*
* @return The name of the table to contain the item.
*/
public String getTableName() {
return tableName;
}
/**
* The name of the table to contain the item.
*
* Constraints:
* Length: 3 - 255
* Pattern: [a-zA-Z0-9_.-]+
*
* @param tableName The name of the table to contain the item.
*/
public void setTableName(String tableName) {
this.tableName = tableName;
}
/**
* The name of the table to contain the item.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 3 - 255
* Pattern: [a-zA-Z0-9_.-]+
*
* @param tableName The name of the table to contain the item.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public PutItemRequest withTableName(String tableName) {
this.tableName = tableName;
return this;
}
/**
* A map of attribute name/value pairs, one for each attribute. Only the
* primary key attributes are required; you can optionally provide other
* attribute name-value pairs for the item.
If you specify any
* attributes that are part of an index key, then the data types for
* those attributes must match those of the schema in the table's
* attribute definition.
For more information about primary keys, see
* Primary
* Key in the Amazon DynamoDB Developer Guide.
Each element in the
* Item map is an AttributeValue object.
*
* @return A map of attribute name/value pairs, one for each attribute. Only the
* primary key attributes are required; you can optionally provide other
* attribute name-value pairs for the item.
If you specify any
* attributes that are part of an index key, then the data types for
* those attributes must match those of the schema in the table's
* attribute definition.
For more information about primary keys, see
* Primary
* Key in the Amazon DynamoDB Developer Guide.
Each element in the
* Item map is an AttributeValue object.
*/
public java.util.Map getItem() {
return item;
}
/**
* A map of attribute name/value pairs, one for each attribute. Only the
* primary key attributes are required; you can optionally provide other
* attribute name-value pairs for the item. If you specify any
* attributes that are part of an index key, then the data types for
* those attributes must match those of the schema in the table's
* attribute definition.
For more information about primary keys, see
* Primary
* Key in the Amazon DynamoDB Developer Guide.
Each element in the
* Item map is an AttributeValue object.
*
* @param item A map of attribute name/value pairs, one for each attribute. Only the
* primary key attributes are required; you can optionally provide other
* attribute name-value pairs for the item.
If you specify any
* attributes that are part of an index key, then the data types for
* those attributes must match those of the schema in the table's
* attribute definition.
For more information about primary keys, see
* Primary
* Key in the Amazon DynamoDB Developer Guide.
Each element in the
* Item map is an AttributeValue object.
*/
public void setItem(java.util.Map item) {
this.item = item;
}
/**
* A map of attribute name/value pairs, one for each attribute. Only the
* primary key attributes are required; you can optionally provide other
* attribute name-value pairs for the item. If you specify any
* attributes that are part of an index key, then the data types for
* those attributes must match those of the schema in the table's
* attribute definition.
For more information about primary keys, see
* Primary
* Key in the Amazon DynamoDB Developer Guide.
Each element in the
* Item map is an AttributeValue object.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param item A map of attribute name/value pairs, one for each attribute. Only the
* primary key attributes are required; you can optionally provide other
* attribute name-value pairs for the item.
If you specify any
* attributes that are part of an index key, then the data types for
* those attributes must match those of the schema in the table's
* attribute definition.
For more information about primary keys, see
* Primary
* Key in the Amazon DynamoDB Developer Guide.
Each element in the
* Item map is an AttributeValue object.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public PutItemRequest withItem(java.util.Map item) {
setItem(item);
return this;
}
/**
* A map of attribute name/value pairs, one for each attribute. Only the
* primary key attributes are required; you can optionally provide other
* attribute name-value pairs for the item. If you specify any
* attributes that are part of an index key, then the data types for
* those attributes must match those of the schema in the table's
* attribute definition.
For more information about primary keys, see
* Primary
* Key in the Amazon DynamoDB Developer Guide.
Each element in the
* Item map is an AttributeValue object.
*
* The method adds a new key-value pair into Item parameter, and returns
* a reference to this object so that method calls can be chained
* together.
*
* @param key The key of the entry to be added into Item.
* @param value The corresponding value of the entry to be added into Item.
*/
public PutItemRequest addItemEntry(String key, AttributeValue value) {
if (null == this.item) {
this.item = new java.util.HashMap();
}
if (this.item.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.item.put(key, value);
return this;
}
/**
* Removes all the entries added into Item.
*
* Returns a reference to this object so that method calls can be chained together.
*/
public PutItemRequest clearItemEntries() {
this.item = null;
return this;
}
/**
* A map of attribute/condition pairs. This is the conditional block for
* the PutItem operation. All the conditions must be met for the
* operation to succeed.
Expected allows you to provide an
* attribute name, and whether or not DynamoDB should check to see if the
* attribute value already exists; or if the attribute value exists and
* has a particular value before changing it.
Each item in
* Expected represents an attribute name for DynamoDB to check,
* along with the following:
-
Value - A value for
* DynamoDB to compare with an attribute. When performing the comparison,
* strongly consistent reads are used.
-
Exists -
* Causes DynamoDB to evaluate the value before attempting a conditional
* operation:
-
If Exists is true
,
* DynamoDB will check to see if that attribute value already exists in
* the table. If it is found, then the operation succeeds. If it is not
* found, the operation fails with a
* ConditionalCheckFailedException.
-
If Exists
* is false
, DynamoDB assumes that the attribute value does
* not exist in the table. If in fact the value does not exist,
* then the assumption is valid and the operation succeeds. If the value
* is found, despite the assumption that it does not exist, the operation
* fails with a ConditionalCheckFailedException.
* The default setting for Exists is true
. If you
* supply a Value all by itself, DynamoDB assumes the attribute
* exists: You don't have to set Exists to true
,
* because it is implied.
DynamoDB returns a
* ValidationException if:
-
Exists is
* true
but there is no Value to check. (You expect a
* value to exist, but don't specify what that value is.)
-
*
Exists is false
but you also specify a
* Value. (You cannot expect an attribute to have a value, while
* also expecting it not to exist.)
If you
* specify more than one condition for Exists, then all of the
* conditions must evaluate to true. (In other words, the conditions are
* ANDed together.) Otherwise, the conditional operation will fail.
*
* @return A map of attribute/condition pairs. This is the conditional block for
* the PutItem operation. All the conditions must be met for the
* operation to succeed.
Expected allows you to provide an
* attribute name, and whether or not DynamoDB should check to see if the
* attribute value already exists; or if the attribute value exists and
* has a particular value before changing it.
Each item in
* Expected represents an attribute name for DynamoDB to check,
* along with the following:
-
Value - A value for
* DynamoDB to compare with an attribute. When performing the comparison,
* strongly consistent reads are used.
-
Exists -
* Causes DynamoDB to evaluate the value before attempting a conditional
* operation:
-
If Exists is true
,
* DynamoDB will check to see if that attribute value already exists in
* the table. If it is found, then the operation succeeds. If it is not
* found, the operation fails with a
* ConditionalCheckFailedException.
-
If Exists
* is false
, DynamoDB assumes that the attribute value does
* not exist in the table. If in fact the value does not exist,
* then the assumption is valid and the operation succeeds. If the value
* is found, despite the assumption that it does not exist, the operation
* fails with a ConditionalCheckFailedException.
* The default setting for Exists is true
. If you
* supply a Value all by itself, DynamoDB assumes the attribute
* exists: You don't have to set Exists to true
,
* because it is implied.
DynamoDB returns a
* ValidationException if:
-
Exists is
* true
but there is no Value to check. (You expect a
* value to exist, but don't specify what that value is.)
-
*
Exists is false
but you also specify a
* Value. (You cannot expect an attribute to have a value, while
* also expecting it not to exist.)
If you
* specify more than one condition for Exists, then all of the
* conditions must evaluate to true. (In other words, the conditions are
* ANDed together.) Otherwise, the conditional operation will fail.
*/
public java.util.Map getExpected() {
return expected;
}
/**
* A map of attribute/condition pairs. This is the conditional block for
* the PutItem operation. All the conditions must be met for the
* operation to succeed. Expected allows you to provide an
* attribute name, and whether or not DynamoDB should check to see if the
* attribute value already exists; or if the attribute value exists and
* has a particular value before changing it.
Each item in
* Expected represents an attribute name for DynamoDB to check,
* along with the following:
-
Value - A value for
* DynamoDB to compare with an attribute. When performing the comparison,
* strongly consistent reads are used.
-
Exists -
* Causes DynamoDB to evaluate the value before attempting a conditional
* operation:
-
If Exists is true
,
* DynamoDB will check to see if that attribute value already exists in
* the table. If it is found, then the operation succeeds. If it is not
* found, the operation fails with a
* ConditionalCheckFailedException.
-
If Exists
* is false
, DynamoDB assumes that the attribute value does
* not exist in the table. If in fact the value does not exist,
* then the assumption is valid and the operation succeeds. If the value
* is found, despite the assumption that it does not exist, the operation
* fails with a ConditionalCheckFailedException.
* The default setting for Exists is true
. If you
* supply a Value all by itself, DynamoDB assumes the attribute
* exists: You don't have to set Exists to true
,
* because it is implied.
DynamoDB returns a
* ValidationException if:
-
Exists is
* true
but there is no Value to check. (You expect a
* value to exist, but don't specify what that value is.)
-
*
Exists is false
but you also specify a
* Value. (You cannot expect an attribute to have a value, while
* also expecting it not to exist.)
If you
* specify more than one condition for Exists, then all of the
* conditions must evaluate to true. (In other words, the conditions are
* ANDed together.) Otherwise, the conditional operation will fail.
*
* @param expected A map of attribute/condition pairs. This is the conditional block for
* the PutItem operation. All the conditions must be met for the
* operation to succeed.
Expected allows you to provide an
* attribute name, and whether or not DynamoDB should check to see if the
* attribute value already exists; or if the attribute value exists and
* has a particular value before changing it.
Each item in
* Expected represents an attribute name for DynamoDB to check,
* along with the following:
-
Value - A value for
* DynamoDB to compare with an attribute. When performing the comparison,
* strongly consistent reads are used.
-
Exists -
* Causes DynamoDB to evaluate the value before attempting a conditional
* operation:
-
If Exists is true
,
* DynamoDB will check to see if that attribute value already exists in
* the table. If it is found, then the operation succeeds. If it is not
* found, the operation fails with a
* ConditionalCheckFailedException.
-
If Exists
* is false
, DynamoDB assumes that the attribute value does
* not exist in the table. If in fact the value does not exist,
* then the assumption is valid and the operation succeeds. If the value
* is found, despite the assumption that it does not exist, the operation
* fails with a ConditionalCheckFailedException.
* The default setting for Exists is true
. If you
* supply a Value all by itself, DynamoDB assumes the attribute
* exists: You don't have to set Exists to true
,
* because it is implied.
DynamoDB returns a
* ValidationException if:
-
Exists is
* true
but there is no Value to check. (You expect a
* value to exist, but don't specify what that value is.)
-
*
Exists is false
but you also specify a
* Value. (You cannot expect an attribute to have a value, while
* also expecting it not to exist.)
If you
* specify more than one condition for Exists, then all of the
* conditions must evaluate to true. (In other words, the conditions are
* ANDed together.) Otherwise, the conditional operation will fail.
*/
public void setExpected(java.util.Map expected) {
this.expected = expected;
}
/**
* A map of attribute/condition pairs. This is the conditional block for
* the PutItem operation. All the conditions must be met for the
* operation to succeed. Expected allows you to provide an
* attribute name, and whether or not DynamoDB should check to see if the
* attribute value already exists; or if the attribute value exists and
* has a particular value before changing it.
Each item in
* Expected represents an attribute name for DynamoDB to check,
* along with the following:
-
Value - A value for
* DynamoDB to compare with an attribute. When performing the comparison,
* strongly consistent reads are used.
-
Exists -
* Causes DynamoDB to evaluate the value before attempting a conditional
* operation:
-
If Exists is true
,
* DynamoDB will check to see if that attribute value already exists in
* the table. If it is found, then the operation succeeds. If it is not
* found, the operation fails with a
* ConditionalCheckFailedException.
-
If Exists
* is false
, DynamoDB assumes that the attribute value does
* not exist in the table. If in fact the value does not exist,
* then the assumption is valid and the operation succeeds. If the value
* is found, despite the assumption that it does not exist, the operation
* fails with a ConditionalCheckFailedException.
* The default setting for Exists is true
. If you
* supply a Value all by itself, DynamoDB assumes the attribute
* exists: You don't have to set Exists to true
,
* because it is implied.
DynamoDB returns a
* ValidationException if:
-
Exists is
* true
but there is no Value to check. (You expect a
* value to exist, but don't specify what that value is.)
-
*
Exists is false
but you also specify a
* Value. (You cannot expect an attribute to have a value, while
* also expecting it not to exist.)
If you
* specify more than one condition for Exists, then all of the
* conditions must evaluate to true. (In other words, the conditions are
* ANDed together.) Otherwise, the conditional operation will fail.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param expected A map of attribute/condition pairs. This is the conditional block for
* the PutItem operation. All the conditions must be met for the
* operation to succeed.
Expected allows you to provide an
* attribute name, and whether or not DynamoDB should check to see if the
* attribute value already exists; or if the attribute value exists and
* has a particular value before changing it.
Each item in
* Expected represents an attribute name for DynamoDB to check,
* along with the following:
-
Value - A value for
* DynamoDB to compare with an attribute. When performing the comparison,
* strongly consistent reads are used.
-
Exists -
* Causes DynamoDB to evaluate the value before attempting a conditional
* operation:
-
If Exists is true
,
* DynamoDB will check to see if that attribute value already exists in
* the table. If it is found, then the operation succeeds. If it is not
* found, the operation fails with a
* ConditionalCheckFailedException.
-
If Exists
* is false
, DynamoDB assumes that the attribute value does
* not exist in the table. If in fact the value does not exist,
* then the assumption is valid and the operation succeeds. If the value
* is found, despite the assumption that it does not exist, the operation
* fails with a ConditionalCheckFailedException.
* The default setting for Exists is true
. If you
* supply a Value all by itself, DynamoDB assumes the attribute
* exists: You don't have to set Exists to true
,
* because it is implied.
DynamoDB returns a
* ValidationException if:
-
Exists is
* true
but there is no Value to check. (You expect a
* value to exist, but don't specify what that value is.)
-
*
Exists is false
but you also specify a
* Value. (You cannot expect an attribute to have a value, while
* also expecting it not to exist.)
If you
* specify more than one condition for Exists, then all of the
* conditions must evaluate to true. (In other words, the conditions are
* ANDed together.) Otherwise, the conditional operation will fail.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public PutItemRequest withExpected(java.util.Map expected) {
setExpected(expected);
return this;
}
/**
* A map of attribute/condition pairs. This is the conditional block for
* the PutItem operation. All the conditions must be met for the
* operation to succeed. Expected allows you to provide an
* attribute name, and whether or not DynamoDB should check to see if the
* attribute value already exists; or if the attribute value exists and
* has a particular value before changing it.
Each item in
* Expected represents an attribute name for DynamoDB to check,
* along with the following:
-
Value - A value for
* DynamoDB to compare with an attribute. When performing the comparison,
* strongly consistent reads are used.
-
Exists -
* Causes DynamoDB to evaluate the value before attempting a conditional
* operation:
-
If Exists is true
,
* DynamoDB will check to see if that attribute value already exists in
* the table. If it is found, then the operation succeeds. If it is not
* found, the operation fails with a
* ConditionalCheckFailedException.
-
If Exists
* is false
, DynamoDB assumes that the attribute value does
* not exist in the table. If in fact the value does not exist,
* then the assumption is valid and the operation succeeds. If the value
* is found, despite the assumption that it does not exist, the operation
* fails with a ConditionalCheckFailedException.
* The default setting for Exists is true
. If you
* supply a Value all by itself, DynamoDB assumes the attribute
* exists: You don't have to set Exists to true
,
* because it is implied.
DynamoDB returns a
* ValidationException if:
-
Exists is
* true
but there is no Value to check. (You expect a
* value to exist, but don't specify what that value is.)
-
*
Exists is false
but you also specify a
* Value. (You cannot expect an attribute to have a value, while
* also expecting it not to exist.)
If you
* specify more than one condition for Exists, then all of the
* conditions must evaluate to true. (In other words, the conditions are
* ANDed together.) Otherwise, the conditional operation will fail.
*
* The method adds a new key-value pair into Expected parameter, and
* returns a reference to this object so that method calls can be chained
* together.
*
* @param key The key of the entry to be added into Expected.
* @param value The corresponding value of the entry to be added into Expected.
*/
public PutItemRequest addExpectedEntry(String key, ExpectedAttributeValue value) {
if (null == this.expected) {
this.expected = new java.util.HashMap();
}
if (this.expected.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.expected.put(key, value);
return this;
}
/**
* Removes all the entries added into Expected.
*
* Returns a reference to this object so that method calls can be chained together.
*/
public PutItemRequest clearExpectedEntries() {
this.expected = null;
return this;
}
/**
* Use ReturnValues if you want to get the item attributes as they
* appeared before they were updated with the PutItem request. For
* PutItem, the valid values are:
-
NONE
-
* If ReturnValues is not specified, or if its value is
* NONE
, then nothing is returned. (This is the default for
* ReturnValues.)
-
ALL_OLD
- If
* PutItem overwrote an attribute name-value pair, then the
* content of the old item is returned.
*
* Constraints:
* Allowed Values: NONE, ALL_OLD, UPDATED_OLD, ALL_NEW, UPDATED_NEW
*
* @return Use ReturnValues if you want to get the item attributes as they
* appeared before they were updated with the PutItem request. For
* PutItem, the valid values are:
-
NONE
-
* If ReturnValues is not specified, or if its value is
* NONE
, then nothing is returned. (This is the default for
* ReturnValues.)
-
ALL_OLD
- If
* PutItem overwrote an attribute name-value pair, then the
* content of the old item is returned.
*
* @see ReturnValue
*/
public String getReturnValues() {
return returnValues;
}
/**
* Use ReturnValues if you want to get the item attributes as they
* appeared before they were updated with the PutItem request. For
* PutItem, the valid values are: -
NONE
-
* If ReturnValues is not specified, or if its value is
* NONE
, then nothing is returned. (This is the default for
* ReturnValues.)
-
ALL_OLD
- If
* PutItem overwrote an attribute name-value pair, then the
* content of the old item is returned.
*
* Constraints:
* Allowed Values: NONE, ALL_OLD, UPDATED_OLD, ALL_NEW, UPDATED_NEW
*
* @param returnValues Use ReturnValues if you want to get the item attributes as they
* appeared before they were updated with the PutItem request. For
* PutItem, the valid values are:
-
NONE
-
* If ReturnValues is not specified, or if its value is
* NONE
, then nothing is returned. (This is the default for
* ReturnValues.)
-
ALL_OLD
- If
* PutItem overwrote an attribute name-value pair, then the
* content of the old item is returned.
*
* @see ReturnValue
*/
public void setReturnValues(String returnValues) {
this.returnValues = returnValues;
}
/**
* Use ReturnValues if you want to get the item attributes as they
* appeared before they were updated with the PutItem request. For
* PutItem, the valid values are: -
NONE
-
* If ReturnValues is not specified, or if its value is
* NONE
, then nothing is returned. (This is the default for
* ReturnValues.)
-
ALL_OLD
- If
* PutItem overwrote an attribute name-value pair, then the
* content of the old item is returned.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: NONE, ALL_OLD, UPDATED_OLD, ALL_NEW, UPDATED_NEW
*
* @param returnValues Use ReturnValues if you want to get the item attributes as they
* appeared before they were updated with the PutItem request. For
* PutItem, the valid values are:
-
NONE
-
* If ReturnValues is not specified, or if its value is
* NONE
, then nothing is returned. (This is the default for
* ReturnValues.)
-
ALL_OLD
- If
* PutItem overwrote an attribute name-value pair, then the
* content of the old item is returned.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see ReturnValue
*/
public PutItemRequest withReturnValues(String returnValues) {
this.returnValues = returnValues;
return this;
}
/**
* Use ReturnValues if you want to get the item attributes as they
* appeared before they were updated with the PutItem request. For
* PutItem, the valid values are: -
NONE
-
* If ReturnValues is not specified, or if its value is
* NONE
, then nothing is returned. (This is the default for
* ReturnValues.)
-
ALL_OLD
- If
* PutItem overwrote an attribute name-value pair, then the
* content of the old item is returned.
*
* Constraints:
* Allowed Values: NONE, ALL_OLD, UPDATED_OLD, ALL_NEW, UPDATED_NEW
*
* @param returnValues Use ReturnValues if you want to get the item attributes as they
* appeared before they were updated with the PutItem request. For
* PutItem, the valid values are:
-
NONE
-
* If ReturnValues is not specified, or if its value is
* NONE
, then nothing is returned. (This is the default for
* ReturnValues.)
-
ALL_OLD
- If
* PutItem overwrote an attribute name-value pair, then the
* content of the old item is returned.
*
* @see ReturnValue
*/
public void setReturnValues(ReturnValue returnValues) {
this.returnValues = returnValues.toString();
}
/**
* Use ReturnValues if you want to get the item attributes as they
* appeared before they were updated with the PutItem request. For
* PutItem, the valid values are: -
NONE
-
* If ReturnValues is not specified, or if its value is
* NONE
, then nothing is returned. (This is the default for
* ReturnValues.)
-
ALL_OLD
- If
* PutItem overwrote an attribute name-value pair, then the
* content of the old item is returned.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: NONE, ALL_OLD, UPDATED_OLD, ALL_NEW, UPDATED_NEW
*
* @param returnValues Use ReturnValues if you want to get the item attributes as they
* appeared before they were updated with the PutItem request. For
* PutItem, the valid values are:
-
NONE
-
* If ReturnValues is not specified, or if its value is
* NONE
, then nothing is returned. (This is the default for
* ReturnValues.)
-
ALL_OLD
- If
* PutItem overwrote an attribute name-value pair, then the
* content of the old item is returned.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see ReturnValue
*/
public PutItemRequest withReturnValues(ReturnValue returnValues) {
this.returnValues = returnValues.toString();
return this;
}
/**
* If set to TOTAL
, the response includes
* ConsumedCapacity data for tables and indexes. If set to
* INDEXES
, the response includes ConsumedCapacity
* for indexes. If set to NONE
(the default),
* ConsumedCapacity is not included in the response.
*
* Constraints:
* Allowed Values: INDEXES, TOTAL, NONE
*
* @return If set to TOTAL
, the response includes
* ConsumedCapacity data for tables and indexes. If set to
* INDEXES
, the response includes ConsumedCapacity
* for indexes. If set to NONE
(the default),
* ConsumedCapacity is not included in the response.
*
* @see ReturnConsumedCapacity
*/
public String getReturnConsumedCapacity() {
return returnConsumedCapacity;
}
/**
* If set to TOTAL
, the response includes
* ConsumedCapacity data for tables and indexes. If set to
* INDEXES
, the response includes ConsumedCapacity
* for indexes. If set to NONE
(the default),
* ConsumedCapacity is not included in the response.
*
* Constraints:
* Allowed Values: INDEXES, TOTAL, NONE
*
* @param returnConsumedCapacity If set to TOTAL
, the response includes
* ConsumedCapacity data for tables and indexes. If set to
* INDEXES
, the response includes ConsumedCapacity
* for indexes. If set to NONE
(the default),
* ConsumedCapacity is not included in the response.
*
* @see ReturnConsumedCapacity
*/
public void setReturnConsumedCapacity(String returnConsumedCapacity) {
this.returnConsumedCapacity = returnConsumedCapacity;
}
/**
* If set to TOTAL
, the response includes
* ConsumedCapacity data for tables and indexes. If set to
* INDEXES
, the response includes ConsumedCapacity
* for indexes. If set to NONE
(the default),
* ConsumedCapacity is not included in the response.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: INDEXES, TOTAL, NONE
*
* @param returnConsumedCapacity If set to TOTAL
, the response includes
* ConsumedCapacity data for tables and indexes. If set to
* INDEXES
, the response includes ConsumedCapacity
* for indexes. If set to NONE
(the default),
* ConsumedCapacity is not included in the response.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see ReturnConsumedCapacity
*/
public PutItemRequest withReturnConsumedCapacity(String returnConsumedCapacity) {
this.returnConsumedCapacity = returnConsumedCapacity;
return this;
}
/**
* If set to TOTAL
, the response includes
* ConsumedCapacity data for tables and indexes. If set to
* INDEXES
, the response includes ConsumedCapacity
* for indexes. If set to NONE
(the default),
* ConsumedCapacity is not included in the response.
*
* Constraints:
* Allowed Values: INDEXES, TOTAL, NONE
*
* @param returnConsumedCapacity If set to TOTAL
, the response includes
* ConsumedCapacity data for tables and indexes. If set to
* INDEXES
, the response includes ConsumedCapacity
* for indexes. If set to NONE
(the default),
* ConsumedCapacity is not included in the response.
*
* @see ReturnConsumedCapacity
*/
public void setReturnConsumedCapacity(ReturnConsumedCapacity returnConsumedCapacity) {
this.returnConsumedCapacity = returnConsumedCapacity.toString();
}
/**
* If set to TOTAL
, the response includes
* ConsumedCapacity data for tables and indexes. If set to
* INDEXES
, the response includes ConsumedCapacity
* for indexes. If set to NONE
(the default),
* ConsumedCapacity is not included in the response.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: INDEXES, TOTAL, NONE
*
* @param returnConsumedCapacity If set to TOTAL
, the response includes
* ConsumedCapacity data for tables and indexes. If set to
* INDEXES
, the response includes ConsumedCapacity
* for indexes. If set to NONE
(the default),
* ConsumedCapacity is not included in the response.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see ReturnConsumedCapacity
*/
public PutItemRequest withReturnConsumedCapacity(ReturnConsumedCapacity returnConsumedCapacity) {
this.returnConsumedCapacity = returnConsumedCapacity.toString();
return this;
}
/**
* If set to SIZE
, statistics about item collections, if
* any, that were modified during the operation are returned in the
* response. If set to NONE
(the default), no statistics are
* returned.
*
* Constraints:
* Allowed Values: SIZE, NONE
*
* @return If set to SIZE
, statistics about item collections, if
* any, that were modified during the operation are returned in the
* response. If set to NONE
(the default), no statistics are
* returned.
*
* @see ReturnItemCollectionMetrics
*/
public String getReturnItemCollectionMetrics() {
return returnItemCollectionMetrics;
}
/**
* If set to SIZE
, statistics about item collections, if
* any, that were modified during the operation are returned in the
* response. If set to NONE
(the default), no statistics are
* returned.
*
* Constraints:
* Allowed Values: SIZE, NONE
*
* @param returnItemCollectionMetrics If set to SIZE
, statistics about item collections, if
* any, that were modified during the operation are returned in the
* response. If set to NONE
(the default), no statistics are
* returned.
*
* @see ReturnItemCollectionMetrics
*/
public void setReturnItemCollectionMetrics(String returnItemCollectionMetrics) {
this.returnItemCollectionMetrics = returnItemCollectionMetrics;
}
/**
* If set to SIZE
, statistics about item collections, if
* any, that were modified during the operation are returned in the
* response. If set to NONE
(the default), no statistics are
* returned.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: SIZE, NONE
*
* @param returnItemCollectionMetrics If set to SIZE
, statistics about item collections, if
* any, that were modified during the operation are returned in the
* response. If set to NONE
(the default), no statistics are
* returned.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see ReturnItemCollectionMetrics
*/
public PutItemRequest withReturnItemCollectionMetrics(String returnItemCollectionMetrics) {
this.returnItemCollectionMetrics = returnItemCollectionMetrics;
return this;
}
/**
* If set to SIZE
, statistics about item collections, if
* any, that were modified during the operation are returned in the
* response. If set to NONE
(the default), no statistics are
* returned.
*
* Constraints:
* Allowed Values: SIZE, NONE
*
* @param returnItemCollectionMetrics If set to SIZE
, statistics about item collections, if
* any, that were modified during the operation are returned in the
* response. If set to NONE
(the default), no statistics are
* returned.
*
* @see ReturnItemCollectionMetrics
*/
public void setReturnItemCollectionMetrics(ReturnItemCollectionMetrics returnItemCollectionMetrics) {
this.returnItemCollectionMetrics = returnItemCollectionMetrics.toString();
}
/**
* If set to SIZE
, statistics about item collections, if
* any, that were modified during the operation are returned in the
* response. If set to NONE
(the default), no statistics are
* returned.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: SIZE, NONE
*
* @param returnItemCollectionMetrics If set to SIZE
, statistics about item collections, if
* any, that were modified during the operation are returned in the
* response. If set to NONE
(the default), no statistics are
* returned.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see ReturnItemCollectionMetrics
*/
public PutItemRequest withReturnItemCollectionMetrics(ReturnItemCollectionMetrics returnItemCollectionMetrics) {
this.returnItemCollectionMetrics = returnItemCollectionMetrics.toString();
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTableName() != null) sb.append("TableName: " + getTableName() + ",");
if (getItem() != null) sb.append("Item: " + getItem() + ",");
if (getExpected() != null) sb.append("Expected: " + getExpected() + ",");
if (getReturnValues() != null) sb.append("ReturnValues: " + getReturnValues() + ",");
if (getReturnConsumedCapacity() != null) sb.append("ReturnConsumedCapacity: " + getReturnConsumedCapacity() + ",");
if (getReturnItemCollectionMetrics() != null) sb.append("ReturnItemCollectionMetrics: " + getReturnItemCollectionMetrics() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTableName() == null) ? 0 : getTableName().hashCode());
hashCode = prime * hashCode + ((getItem() == null) ? 0 : getItem().hashCode());
hashCode = prime * hashCode + ((getExpected() == null) ? 0 : getExpected().hashCode());
hashCode = prime * hashCode + ((getReturnValues() == null) ? 0 : getReturnValues().hashCode());
hashCode = prime * hashCode + ((getReturnConsumedCapacity() == null) ? 0 : getReturnConsumedCapacity().hashCode());
hashCode = prime * hashCode + ((getReturnItemCollectionMetrics() == null) ? 0 : getReturnItemCollectionMetrics().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof PutItemRequest == false) return false;
PutItemRequest other = (PutItemRequest)obj;
if (other.getTableName() == null ^ this.getTableName() == null) return false;
if (other.getTableName() != null && other.getTableName().equals(this.getTableName()) == false) return false;
if (other.getItem() == null ^ this.getItem() == null) return false;
if (other.getItem() != null && other.getItem().equals(this.getItem()) == false) return false;
if (other.getExpected() == null ^ this.getExpected() == null) return false;
if (other.getExpected() != null && other.getExpected().equals(this.getExpected()) == false) return false;
if (other.getReturnValues() == null ^ this.getReturnValues() == null) return false;
if (other.getReturnValues() != null && other.getReturnValues().equals(this.getReturnValues()) == false) return false;
if (other.getReturnConsumedCapacity() == null ^ this.getReturnConsumedCapacity() == null) return false;
if (other.getReturnConsumedCapacity() != null && other.getReturnConsumedCapacity().equals(this.getReturnConsumedCapacity()) == false) return false;
if (other.getReturnItemCollectionMetrics() == null ^ this.getReturnItemCollectionMetrics() == null) return false;
if (other.getReturnItemCollectionMetrics() != null && other.getReturnItemCollectionMetrics().equals(this.getReturnItemCollectionMetrics()) == false) return false;
return true;
}
}