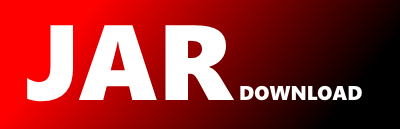
com.amazonaws.services.dynamodbv2.model.QueryRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.dynamodbv2.model;
import java.io.Serializable;
import com.amazonaws.AmazonWebServiceRequest;
/**
* Container for the parameters to the {@link com.amazonaws.services.dynamodbv2.AmazonDynamoDB#query(QueryRequest) Query operation}.
*
* A Query operation directly accesses items from a table using
* the table primary key, or from an index using the index key. You must
* provide a specific hash key value. You can narrow the scope of the
* query by using comparison operators on the range key value, or on the
* index key. You can use the ScanIndexForward parameter to get
* results in forward or reverse order, by range key or by index key.
*
*
* Queries that do not return results consume the minimum read capacity
* units according to the type of read.
*
*
* If the total number of items meeting the query criteria exceeds the
* result set size limit of 1 MB, the query stops and results are
* returned to the user with a LastEvaluatedKey to continue the
* query in a subsequent operation. Unlike a Scan operation, a
* Query operation never returns an empty result set and a
* LastEvaluatedKey . The
* LastEvaluatedKey is only provided if the results exceed 1 MB,
* or if you have used Limit .
*
*
* You can query a table, a local secondary index (LSI), or a global
* secondary index (GSI). For a query on a table or on an LSI, you can
* set ConsistentRead to true and obtain a strongly consistent
* result. GSIs support eventually consistent reads only, so do not
* specify ConsistentRead when querying a GSI.
*
*
* @see com.amazonaws.services.dynamodbv2.AmazonDynamoDB#query(QueryRequest)
*/
public class QueryRequest extends AmazonWebServiceRequest implements Serializable {
/**
* The name of the table containing the requested items.
*
* Constraints:
* Length: 3 - 255
* Pattern: [a-zA-Z0-9_.-]+
*/
private String tableName;
/**
* The name of an index to query. This can be any local secondary index
* or global secondary index on the table.
*
* Constraints:
* Length: 3 - 255
* Pattern: [a-zA-Z0-9_.-]+
*/
private String indexName;
/**
* The attributes to be returned in the result. You can retrieve all item
* attributes, specific item attributes, the count of matching items, or
* in the case of an index, some or all of the attributes projected into
* the index.
-
ALL_ATTRIBUTES
: Returns all of
* the item attributes from the specified table or index. If you are
* querying a local secondary index, then for each matching item in the
* index DynamoDB will fetch the entire item from the parent table. If
* the index is configured to project all item attributes, then all of
* the data can be obtained from the local secondary index, and no
* fetching is required..
-
*
ALL_PROJECTED_ATTRIBUTES
: Allowed only when querying
* an index. Retrieves all attributes which have been projected into the
* index. If the index is configured to project all attributes, this is
* equivalent to specifying ALL_ATTRIBUTES
.
-
*
COUNT
: Returns the number of matching items, rather
* than the matching items themselves.
-
* SPECIFIC_ATTRIBUTES
: Returns only the attributes listed
* in AttributesToGet. This is equivalent to specifying
* AttributesToGet without specifying any value for Select.
*
If you are querying a local secondary index and request only
* attributes that are projected into that index, the operation will read
* only the index and not the table. If any of the requested attributes
* are not projected into the local secondary index, DynamoDB will fetch
* each of these attributes from the parent table. This extra fetching
* incurs additional throughput cost and latency.
If you are querying
* a global secondary index, you can only request attributes that are
* projected into the index. Global secondary index queries cannot fetch
* attributes from the parent table.
If neither
* Select nor AttributesToGet are specified, DynamoDB
* defaults to ALL_ATTRIBUTES
when accessing a table, and
* ALL_PROJECTED_ATTRIBUTES
when accessing an index. You
* cannot use both Select and AttributesToGet together in a
* single request, unless the value for Select is
* SPECIFIC_ATTRIBUTES
. (This usage is equivalent to
* specifying AttributesToGet without any value for
* Select.)
*
* Constraints:
* Allowed Values: ALL_ATTRIBUTES, ALL_PROJECTED_ATTRIBUTES, SPECIFIC_ATTRIBUTES, COUNT
*/
private String select;
/**
* The names of one or more attributes to retrieve. If no attribute names
* are specified, then all attributes will be returned. If any of the
* requested attributes are not found, they will not appear in the
* result.
You cannot use both AttributesToGet and
* Select together in a Query request, unless the
* value for Select is SPECIFIC_ATTRIBUTES
. (This
* usage is equivalent to specifying AttributesToGet without any
* value for Select.)
If you are querying a local secondary
* index and request only attributes that are projected into that index,
* the operation will read only the index and not the table. If any of
* the requested attributes are not projected into the local secondary
* index, DynamoDB will fetch each of these attributes from the parent
* table. This extra fetching incurs additional throughput cost and
* latency.
If you are querying a global secondary index, you can only
* request attributes that are projected into the index. Global secondary
* index queries cannot fetch attributes from the parent table.
*
* Constraints:
* Length: 1 -
*/
private com.amazonaws.internal.ListWithAutoConstructFlag attributesToGet;
/**
* The maximum number of items to evaluate (not necessarily the number of
* matching items). If DynamoDB processes the number of items up to the
* limit while processing the results, it stops the operation and returns
* the matching values up to that point, and a LastEvaluatedKey to
* apply in a subsequent operation, so that you can pick up where you
* left off. Also, if the processed data set size exceeds 1 MB before
* DynamoDB reaches this limit, it stops the operation and returns the
* matching values up to the limit, and a LastEvaluatedKey to
* apply in a subsequent operation to continue the operation. For more
* information see Query
* and Scan in the Amazon DynamoDB Developer Guide.
*
* Constraints:
* Range: 1 -
*/
private Integer limit;
/**
* If set to true
, then the operation uses strongly
* consistent reads; otherwise, eventually consistent reads are used.
*
Strongly consistent reads are not supported on global secondary
* indexes. If you query a global secondary index with
* ConsistentRead set to true
, you will receive an
* error message.
*/
private Boolean consistentRead;
/**
* The selection criteria for the query.
For a query on a table, you
* can only have conditions on the table primary key attributes. You must
* specify the hash key attribute name and value as an EQ
* condition. You can optionally specify a second condition, referring to
* the range key attribute.
For a query on an index, you can only have
* conditions on the index key attributes. You must specify the index
* hash attribute name and value as an EQ condition. You can optionally
* specify a second condition, referring to the index key range
* attribute.
Multiple conditions are evaluated using "AND"; in other
* words, all of the conditions must be met in order for an item to
* appear in the results results.
Each KeyConditions element
* consists of an attribute name to compare, along with the following:
*
AttributeValueList - One or more values to evaluate
* against the supplied attribute. This list contains exactly one value,
* except for a BETWEEN
comparison, in which case the list
* contains two values. For type Number, value comparisons are
* numeric.
String value comparisons for greater than, equals, or less
* than are based on ASCII character code values. For example,
* a
is greater than A
, and aa
is
* greater than B
. For a list of code values, see http://en.wikipedia.org/wiki/ASCII#ASCII_printable_characters.
*
For Binary, DynamoDB treats each byte of the binary data as
* unsigned when it compares binary values, for example when evaluating
* query expressions.
ComparisonOperator - A
* comparator for evaluating attributes. For example, equals, greater
* than, less than, etc.
Valid comparison operators for Query:
*
EQ | LE | LT | GE | GT | BEGINS_WITH | BETWEEN
For
* information on specifying data types in JSON, see JSON
* Data Format in the Amazon DynamoDB Developer Guide.
The
* following are descriptions of each comparison operator.
-
*
EQ
: Equal.
AttributeValueList can contain
* only one AttributeValue of type String, Number, or Binary (not
* a set). If an item contains an AttributeValue of a different
* type than the one specified in the request, the value does not match.
* For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not equal
* {"NS":["6", "2", "1"]}
.
-
*
LE
: Less than or equal.
AttributeValueList
* can contain only one AttributeValue of type String, Number, or
* Binary (not a set). If an item contains an AttributeValue of a
* different type than the one specified in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
LT
: Less than.
AttributeValueList can
* contain only one AttributeValue of type String, Number, or
* Binary (not a set). If an item contains an AttributeValue of a
* different type than the one specified in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
GE
: Greater than or equal.
*
AttributeValueList can contain only one
* AttributeValue of type String, Number, or Binary (not a set).
* If an item contains an AttributeValue of a different type than
* the one specified in the request, the value does not match. For
* example, {"S":"6"}
does not equal {"N":"6"}
.
* Also, {"N":"6"}
does not compare to {"NS":["6",
* "2", "1"]}
.
-
GT
: Greater than.
*
AttributeValueList can contain only one
* AttributeValue of type String, Number, or Binary (not a set).
* If an item contains an AttributeValue of a different type than
* the one specified in the request, the value does not match. For
* example, {"S":"6"}
does not equal {"N":"6"}
.
* Also, {"N":"6"}
does not compare to {"NS":["6",
* "2", "1"]}
.
-
BEGINS_WITH
:
* checks for a prefix.
AttributeValueList can contain only one
* AttributeValue of type String or Binary (not a Number or a
* set). The target attribute of the comparison must be a String or
* Binary (not a Number or a set).
-
*
BETWEEN
: Greater than or equal to the first value,
* and less than or equal to the second value.
*
AttributeValueList must contain two AttributeValue
* elements of the same type, either String, Number, or Binary (not a
* set). A target attribute matches if the target value is greater than,
* or equal to, the first element and less than, or equal to, the second
* element. If an item contains an AttributeValue of a different
* type than the one specified in the request, the value does not match.
* For example, {"S":"6"}
does not compare to
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
*/
private java.util.Map keyConditions;
/**
* Specifies ascending (true) or descending (false) traversal of the
* index. DynamoDB returns results reflecting the requested order
* determined by the range key. If the data type is Number, the results
* are returned in numeric order. For String, the results are returned in
* order of ASCII character code values. For Binary, Amazon DynamoDB
* treats each byte of the binary data as unsigned when it compares
* binary values. If ScanIndexForward is not specified, the
* results are returned in ascending order.
*/
private Boolean scanIndexForward;
/**
* The primary key of the first item that this operation will evaluate.
* Use the value that was returned for LastEvaluatedKey in the
* previous operation.
The data type for ExclusiveStartKey must
* be String, Number or Binary. No set data types are allowed.
*/
private java.util.Map exclusiveStartKey;
/**
* If set to TOTAL
, the response includes
* ConsumedCapacity data for tables and indexes. If set to
* INDEXES
, the response includes ConsumedCapacity
* for indexes. If set to NONE
(the default),
* ConsumedCapacity is not included in the response.
*
* Constraints:
* Allowed Values: INDEXES, TOTAL, NONE
*/
private String returnConsumedCapacity;
/**
* Default constructor for a new QueryRequest object. Callers should use the
* setter or fluent setter (with...) methods to initialize this object after creating it.
*/
public QueryRequest() {}
/**
* Constructs a new QueryRequest object.
* Callers should use the setter or fluent setter (with...) methods to
* initialize any additional object members.
*
* @param tableName The name of the table containing the requested items.
*/
public QueryRequest(String tableName) {
setTableName(tableName);
}
/**
* The name of the table containing the requested items.
*
* Constraints:
* Length: 3 - 255
* Pattern: [a-zA-Z0-9_.-]+
*
* @return The name of the table containing the requested items.
*/
public String getTableName() {
return tableName;
}
/**
* The name of the table containing the requested items.
*
* Constraints:
* Length: 3 - 255
* Pattern: [a-zA-Z0-9_.-]+
*
* @param tableName The name of the table containing the requested items.
*/
public void setTableName(String tableName) {
this.tableName = tableName;
}
/**
* The name of the table containing the requested items.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 3 - 255
* Pattern: [a-zA-Z0-9_.-]+
*
* @param tableName The name of the table containing the requested items.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public QueryRequest withTableName(String tableName) {
this.tableName = tableName;
return this;
}
/**
* The name of an index to query. This can be any local secondary index
* or global secondary index on the table.
*
* Constraints:
* Length: 3 - 255
* Pattern: [a-zA-Z0-9_.-]+
*
* @return The name of an index to query. This can be any local secondary index
* or global secondary index on the table.
*/
public String getIndexName() {
return indexName;
}
/**
* The name of an index to query. This can be any local secondary index
* or global secondary index on the table.
*
* Constraints:
* Length: 3 - 255
* Pattern: [a-zA-Z0-9_.-]+
*
* @param indexName The name of an index to query. This can be any local secondary index
* or global secondary index on the table.
*/
public void setIndexName(String indexName) {
this.indexName = indexName;
}
/**
* The name of an index to query. This can be any local secondary index
* or global secondary index on the table.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 3 - 255
* Pattern: [a-zA-Z0-9_.-]+
*
* @param indexName The name of an index to query. This can be any local secondary index
* or global secondary index on the table.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public QueryRequest withIndexName(String indexName) {
this.indexName = indexName;
return this;
}
/**
* The attributes to be returned in the result. You can retrieve all item
* attributes, specific item attributes, the count of matching items, or
* in the case of an index, some or all of the attributes projected into
* the index.
-
ALL_ATTRIBUTES
: Returns all of
* the item attributes from the specified table or index. If you are
* querying a local secondary index, then for each matching item in the
* index DynamoDB will fetch the entire item from the parent table. If
* the index is configured to project all item attributes, then all of
* the data can be obtained from the local secondary index, and no
* fetching is required..
-
*
ALL_PROJECTED_ATTRIBUTES
: Allowed only when querying
* an index. Retrieves all attributes which have been projected into the
* index. If the index is configured to project all attributes, this is
* equivalent to specifying ALL_ATTRIBUTES
.
-
*
COUNT
: Returns the number of matching items, rather
* than the matching items themselves.
-
* SPECIFIC_ATTRIBUTES
: Returns only the attributes listed
* in AttributesToGet. This is equivalent to specifying
* AttributesToGet without specifying any value for Select.
*
If you are querying a local secondary index and request only
* attributes that are projected into that index, the operation will read
* only the index and not the table. If any of the requested attributes
* are not projected into the local secondary index, DynamoDB will fetch
* each of these attributes from the parent table. This extra fetching
* incurs additional throughput cost and latency.
If you are querying
* a global secondary index, you can only request attributes that are
* projected into the index. Global secondary index queries cannot fetch
* attributes from the parent table.
If neither
* Select nor AttributesToGet are specified, DynamoDB
* defaults to ALL_ATTRIBUTES
when accessing a table, and
* ALL_PROJECTED_ATTRIBUTES
when accessing an index. You
* cannot use both Select and AttributesToGet together in a
* single request, unless the value for Select is
* SPECIFIC_ATTRIBUTES
. (This usage is equivalent to
* specifying AttributesToGet without any value for
* Select.)
*
* Constraints:
* Allowed Values: ALL_ATTRIBUTES, ALL_PROJECTED_ATTRIBUTES, SPECIFIC_ATTRIBUTES, COUNT
*
* @return The attributes to be returned in the result. You can retrieve all item
* attributes, specific item attributes, the count of matching items, or
* in the case of an index, some or all of the attributes projected into
* the index.
-
ALL_ATTRIBUTES
: Returns all of
* the item attributes from the specified table or index. If you are
* querying a local secondary index, then for each matching item in the
* index DynamoDB will fetch the entire item from the parent table. If
* the index is configured to project all item attributes, then all of
* the data can be obtained from the local secondary index, and no
* fetching is required..
-
*
ALL_PROJECTED_ATTRIBUTES
: Allowed only when querying
* an index. Retrieves all attributes which have been projected into the
* index. If the index is configured to project all attributes, this is
* equivalent to specifying ALL_ATTRIBUTES
.
-
*
COUNT
: Returns the number of matching items, rather
* than the matching items themselves.
-
* SPECIFIC_ATTRIBUTES
: Returns only the attributes listed
* in AttributesToGet. This is equivalent to specifying
* AttributesToGet without specifying any value for Select.
*
If you are querying a local secondary index and request only
* attributes that are projected into that index, the operation will read
* only the index and not the table. If any of the requested attributes
* are not projected into the local secondary index, DynamoDB will fetch
* each of these attributes from the parent table. This extra fetching
* incurs additional throughput cost and latency.
If you are querying
* a global secondary index, you can only request attributes that are
* projected into the index. Global secondary index queries cannot fetch
* attributes from the parent table.
If neither
* Select nor AttributesToGet are specified, DynamoDB
* defaults to ALL_ATTRIBUTES
when accessing a table, and
* ALL_PROJECTED_ATTRIBUTES
when accessing an index. You
* cannot use both Select and AttributesToGet together in a
* single request, unless the value for Select is
* SPECIFIC_ATTRIBUTES
. (This usage is equivalent to
* specifying AttributesToGet without any value for
* Select.)
*
* @see Select
*/
public String getSelect() {
return select;
}
/**
* The attributes to be returned in the result. You can retrieve all item
* attributes, specific item attributes, the count of matching items, or
* in the case of an index, some or all of the attributes projected into
* the index.
-
ALL_ATTRIBUTES
: Returns all of
* the item attributes from the specified table or index. If you are
* querying a local secondary index, then for each matching item in the
* index DynamoDB will fetch the entire item from the parent table. If
* the index is configured to project all item attributes, then all of
* the data can be obtained from the local secondary index, and no
* fetching is required..
-
*
ALL_PROJECTED_ATTRIBUTES
: Allowed only when querying
* an index. Retrieves all attributes which have been projected into the
* index. If the index is configured to project all attributes, this is
* equivalent to specifying ALL_ATTRIBUTES
.
-
*
COUNT
: Returns the number of matching items, rather
* than the matching items themselves.
-
* SPECIFIC_ATTRIBUTES
: Returns only the attributes listed
* in AttributesToGet. This is equivalent to specifying
* AttributesToGet without specifying any value for Select.
*
If you are querying a local secondary index and request only
* attributes that are projected into that index, the operation will read
* only the index and not the table. If any of the requested attributes
* are not projected into the local secondary index, DynamoDB will fetch
* each of these attributes from the parent table. This extra fetching
* incurs additional throughput cost and latency.
If you are querying
* a global secondary index, you can only request attributes that are
* projected into the index. Global secondary index queries cannot fetch
* attributes from the parent table.
If neither
* Select nor AttributesToGet are specified, DynamoDB
* defaults to ALL_ATTRIBUTES
when accessing a table, and
* ALL_PROJECTED_ATTRIBUTES
when accessing an index. You
* cannot use both Select and AttributesToGet together in a
* single request, unless the value for Select is
* SPECIFIC_ATTRIBUTES
. (This usage is equivalent to
* specifying AttributesToGet without any value for
* Select.)
*
* Constraints:
* Allowed Values: ALL_ATTRIBUTES, ALL_PROJECTED_ATTRIBUTES, SPECIFIC_ATTRIBUTES, COUNT
*
* @param select The attributes to be returned in the result. You can retrieve all item
* attributes, specific item attributes, the count of matching items, or
* in the case of an index, some or all of the attributes projected into
* the index.
-
ALL_ATTRIBUTES
: Returns all of
* the item attributes from the specified table or index. If you are
* querying a local secondary index, then for each matching item in the
* index DynamoDB will fetch the entire item from the parent table. If
* the index is configured to project all item attributes, then all of
* the data can be obtained from the local secondary index, and no
* fetching is required..
-
*
ALL_PROJECTED_ATTRIBUTES
: Allowed only when querying
* an index. Retrieves all attributes which have been projected into the
* index. If the index is configured to project all attributes, this is
* equivalent to specifying ALL_ATTRIBUTES
.
-
*
COUNT
: Returns the number of matching items, rather
* than the matching items themselves.
-
* SPECIFIC_ATTRIBUTES
: Returns only the attributes listed
* in AttributesToGet. This is equivalent to specifying
* AttributesToGet without specifying any value for Select.
*
If you are querying a local secondary index and request only
* attributes that are projected into that index, the operation will read
* only the index and not the table. If any of the requested attributes
* are not projected into the local secondary index, DynamoDB will fetch
* each of these attributes from the parent table. This extra fetching
* incurs additional throughput cost and latency.
If you are querying
* a global secondary index, you can only request attributes that are
* projected into the index. Global secondary index queries cannot fetch
* attributes from the parent table.
If neither
* Select nor AttributesToGet are specified, DynamoDB
* defaults to ALL_ATTRIBUTES
when accessing a table, and
* ALL_PROJECTED_ATTRIBUTES
when accessing an index. You
* cannot use both Select and AttributesToGet together in a
* single request, unless the value for Select is
* SPECIFIC_ATTRIBUTES
. (This usage is equivalent to
* specifying AttributesToGet without any value for
* Select.)
*
* @see Select
*/
public void setSelect(String select) {
this.select = select;
}
/**
* The attributes to be returned in the result. You can retrieve all item
* attributes, specific item attributes, the count of matching items, or
* in the case of an index, some or all of the attributes projected into
* the index.
-
ALL_ATTRIBUTES
: Returns all of
* the item attributes from the specified table or index. If you are
* querying a local secondary index, then for each matching item in the
* index DynamoDB will fetch the entire item from the parent table. If
* the index is configured to project all item attributes, then all of
* the data can be obtained from the local secondary index, and no
* fetching is required..
-
*
ALL_PROJECTED_ATTRIBUTES
: Allowed only when querying
* an index. Retrieves all attributes which have been projected into the
* index. If the index is configured to project all attributes, this is
* equivalent to specifying ALL_ATTRIBUTES
.
-
*
COUNT
: Returns the number of matching items, rather
* than the matching items themselves.
-
* SPECIFIC_ATTRIBUTES
: Returns only the attributes listed
* in AttributesToGet. This is equivalent to specifying
* AttributesToGet without specifying any value for Select.
*
If you are querying a local secondary index and request only
* attributes that are projected into that index, the operation will read
* only the index and not the table. If any of the requested attributes
* are not projected into the local secondary index, DynamoDB will fetch
* each of these attributes from the parent table. This extra fetching
* incurs additional throughput cost and latency.
If you are querying
* a global secondary index, you can only request attributes that are
* projected into the index. Global secondary index queries cannot fetch
* attributes from the parent table.
If neither
* Select nor AttributesToGet are specified, DynamoDB
* defaults to ALL_ATTRIBUTES
when accessing a table, and
* ALL_PROJECTED_ATTRIBUTES
when accessing an index. You
* cannot use both Select and AttributesToGet together in a
* single request, unless the value for Select is
* SPECIFIC_ATTRIBUTES
. (This usage is equivalent to
* specifying AttributesToGet without any value for
* Select.)
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: ALL_ATTRIBUTES, ALL_PROJECTED_ATTRIBUTES, SPECIFIC_ATTRIBUTES, COUNT
*
* @param select The attributes to be returned in the result. You can retrieve all item
* attributes, specific item attributes, the count of matching items, or
* in the case of an index, some or all of the attributes projected into
* the index.
-
ALL_ATTRIBUTES
: Returns all of
* the item attributes from the specified table or index. If you are
* querying a local secondary index, then for each matching item in the
* index DynamoDB will fetch the entire item from the parent table. If
* the index is configured to project all item attributes, then all of
* the data can be obtained from the local secondary index, and no
* fetching is required..
-
*
ALL_PROJECTED_ATTRIBUTES
: Allowed only when querying
* an index. Retrieves all attributes which have been projected into the
* index. If the index is configured to project all attributes, this is
* equivalent to specifying ALL_ATTRIBUTES
.
-
*
COUNT
: Returns the number of matching items, rather
* than the matching items themselves.
-
* SPECIFIC_ATTRIBUTES
: Returns only the attributes listed
* in AttributesToGet. This is equivalent to specifying
* AttributesToGet without specifying any value for Select.
*
If you are querying a local secondary index and request only
* attributes that are projected into that index, the operation will read
* only the index and not the table. If any of the requested attributes
* are not projected into the local secondary index, DynamoDB will fetch
* each of these attributes from the parent table. This extra fetching
* incurs additional throughput cost and latency.
If you are querying
* a global secondary index, you can only request attributes that are
* projected into the index. Global secondary index queries cannot fetch
* attributes from the parent table.
If neither
* Select nor AttributesToGet are specified, DynamoDB
* defaults to ALL_ATTRIBUTES
when accessing a table, and
* ALL_PROJECTED_ATTRIBUTES
when accessing an index. You
* cannot use both Select and AttributesToGet together in a
* single request, unless the value for Select is
* SPECIFIC_ATTRIBUTES
. (This usage is equivalent to
* specifying AttributesToGet without any value for
* Select.)
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see Select
*/
public QueryRequest withSelect(String select) {
this.select = select;
return this;
}
/**
* The attributes to be returned in the result. You can retrieve all item
* attributes, specific item attributes, the count of matching items, or
* in the case of an index, some or all of the attributes projected into
* the index.
-
ALL_ATTRIBUTES
: Returns all of
* the item attributes from the specified table or index. If you are
* querying a local secondary index, then for each matching item in the
* index DynamoDB will fetch the entire item from the parent table. If
* the index is configured to project all item attributes, then all of
* the data can be obtained from the local secondary index, and no
* fetching is required..
-
*
ALL_PROJECTED_ATTRIBUTES
: Allowed only when querying
* an index. Retrieves all attributes which have been projected into the
* index. If the index is configured to project all attributes, this is
* equivalent to specifying ALL_ATTRIBUTES
.
-
*
COUNT
: Returns the number of matching items, rather
* than the matching items themselves.
-
* SPECIFIC_ATTRIBUTES
: Returns only the attributes listed
* in AttributesToGet. This is equivalent to specifying
* AttributesToGet without specifying any value for Select.
*
If you are querying a local secondary index and request only
* attributes that are projected into that index, the operation will read
* only the index and not the table. If any of the requested attributes
* are not projected into the local secondary index, DynamoDB will fetch
* each of these attributes from the parent table. This extra fetching
* incurs additional throughput cost and latency.
If you are querying
* a global secondary index, you can only request attributes that are
* projected into the index. Global secondary index queries cannot fetch
* attributes from the parent table.
If neither
* Select nor AttributesToGet are specified, DynamoDB
* defaults to ALL_ATTRIBUTES
when accessing a table, and
* ALL_PROJECTED_ATTRIBUTES
when accessing an index. You
* cannot use both Select and AttributesToGet together in a
* single request, unless the value for Select is
* SPECIFIC_ATTRIBUTES
. (This usage is equivalent to
* specifying AttributesToGet without any value for
* Select.)
*
* Constraints:
* Allowed Values: ALL_ATTRIBUTES, ALL_PROJECTED_ATTRIBUTES, SPECIFIC_ATTRIBUTES, COUNT
*
* @param select The attributes to be returned in the result. You can retrieve all item
* attributes, specific item attributes, the count of matching items, or
* in the case of an index, some or all of the attributes projected into
* the index.
-
ALL_ATTRIBUTES
: Returns all of
* the item attributes from the specified table or index. If you are
* querying a local secondary index, then for each matching item in the
* index DynamoDB will fetch the entire item from the parent table. If
* the index is configured to project all item attributes, then all of
* the data can be obtained from the local secondary index, and no
* fetching is required..
-
*
ALL_PROJECTED_ATTRIBUTES
: Allowed only when querying
* an index. Retrieves all attributes which have been projected into the
* index. If the index is configured to project all attributes, this is
* equivalent to specifying ALL_ATTRIBUTES
.
-
*
COUNT
: Returns the number of matching items, rather
* than the matching items themselves.
-
* SPECIFIC_ATTRIBUTES
: Returns only the attributes listed
* in AttributesToGet. This is equivalent to specifying
* AttributesToGet without specifying any value for Select.
*
If you are querying a local secondary index and request only
* attributes that are projected into that index, the operation will read
* only the index and not the table. If any of the requested attributes
* are not projected into the local secondary index, DynamoDB will fetch
* each of these attributes from the parent table. This extra fetching
* incurs additional throughput cost and latency.
If you are querying
* a global secondary index, you can only request attributes that are
* projected into the index. Global secondary index queries cannot fetch
* attributes from the parent table.
If neither
* Select nor AttributesToGet are specified, DynamoDB
* defaults to ALL_ATTRIBUTES
when accessing a table, and
* ALL_PROJECTED_ATTRIBUTES
when accessing an index. You
* cannot use both Select and AttributesToGet together in a
* single request, unless the value for Select is
* SPECIFIC_ATTRIBUTES
. (This usage is equivalent to
* specifying AttributesToGet without any value for
* Select.)
*
* @see Select
*/
public void setSelect(Select select) {
this.select = select.toString();
}
/**
* The attributes to be returned in the result. You can retrieve all item
* attributes, specific item attributes, the count of matching items, or
* in the case of an index, some or all of the attributes projected into
* the index.
-
ALL_ATTRIBUTES
: Returns all of
* the item attributes from the specified table or index. If you are
* querying a local secondary index, then for each matching item in the
* index DynamoDB will fetch the entire item from the parent table. If
* the index is configured to project all item attributes, then all of
* the data can be obtained from the local secondary index, and no
* fetching is required..
-
*
ALL_PROJECTED_ATTRIBUTES
: Allowed only when querying
* an index. Retrieves all attributes which have been projected into the
* index. If the index is configured to project all attributes, this is
* equivalent to specifying ALL_ATTRIBUTES
.
-
*
COUNT
: Returns the number of matching items, rather
* than the matching items themselves.
-
* SPECIFIC_ATTRIBUTES
: Returns only the attributes listed
* in AttributesToGet. This is equivalent to specifying
* AttributesToGet without specifying any value for Select.
*
If you are querying a local secondary index and request only
* attributes that are projected into that index, the operation will read
* only the index and not the table. If any of the requested attributes
* are not projected into the local secondary index, DynamoDB will fetch
* each of these attributes from the parent table. This extra fetching
* incurs additional throughput cost and latency.
If you are querying
* a global secondary index, you can only request attributes that are
* projected into the index. Global secondary index queries cannot fetch
* attributes from the parent table.
If neither
* Select nor AttributesToGet are specified, DynamoDB
* defaults to ALL_ATTRIBUTES
when accessing a table, and
* ALL_PROJECTED_ATTRIBUTES
when accessing an index. You
* cannot use both Select and AttributesToGet together in a
* single request, unless the value for Select is
* SPECIFIC_ATTRIBUTES
. (This usage is equivalent to
* specifying AttributesToGet without any value for
* Select.)
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: ALL_ATTRIBUTES, ALL_PROJECTED_ATTRIBUTES, SPECIFIC_ATTRIBUTES, COUNT
*
* @param select The attributes to be returned in the result. You can retrieve all item
* attributes, specific item attributes, the count of matching items, or
* in the case of an index, some or all of the attributes projected into
* the index.
-
ALL_ATTRIBUTES
: Returns all of
* the item attributes from the specified table or index. If you are
* querying a local secondary index, then for each matching item in the
* index DynamoDB will fetch the entire item from the parent table. If
* the index is configured to project all item attributes, then all of
* the data can be obtained from the local secondary index, and no
* fetching is required..
-
*
ALL_PROJECTED_ATTRIBUTES
: Allowed only when querying
* an index. Retrieves all attributes which have been projected into the
* index. If the index is configured to project all attributes, this is
* equivalent to specifying ALL_ATTRIBUTES
.
-
*
COUNT
: Returns the number of matching items, rather
* than the matching items themselves.
-
* SPECIFIC_ATTRIBUTES
: Returns only the attributes listed
* in AttributesToGet. This is equivalent to specifying
* AttributesToGet without specifying any value for Select.
*
If you are querying a local secondary index and request only
* attributes that are projected into that index, the operation will read
* only the index and not the table. If any of the requested attributes
* are not projected into the local secondary index, DynamoDB will fetch
* each of these attributes from the parent table. This extra fetching
* incurs additional throughput cost and latency.
If you are querying
* a global secondary index, you can only request attributes that are
* projected into the index. Global secondary index queries cannot fetch
* attributes from the parent table.
If neither
* Select nor AttributesToGet are specified, DynamoDB
* defaults to ALL_ATTRIBUTES
when accessing a table, and
* ALL_PROJECTED_ATTRIBUTES
when accessing an index. You
* cannot use both Select and AttributesToGet together in a
* single request, unless the value for Select is
* SPECIFIC_ATTRIBUTES
. (This usage is equivalent to
* specifying AttributesToGet without any value for
* Select.)
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see Select
*/
public QueryRequest withSelect(Select select) {
this.select = select.toString();
return this;
}
/**
* The names of one or more attributes to retrieve. If no attribute names
* are specified, then all attributes will be returned. If any of the
* requested attributes are not found, they will not appear in the
* result.
You cannot use both AttributesToGet and
* Select together in a Query request, unless the
* value for Select is SPECIFIC_ATTRIBUTES
. (This
* usage is equivalent to specifying AttributesToGet without any
* value for Select.)
If you are querying a local secondary
* index and request only attributes that are projected into that index,
* the operation will read only the index and not the table. If any of
* the requested attributes are not projected into the local secondary
* index, DynamoDB will fetch each of these attributes from the parent
* table. This extra fetching incurs additional throughput cost and
* latency.
If you are querying a global secondary index, you can only
* request attributes that are projected into the index. Global secondary
* index queries cannot fetch attributes from the parent table.
*
* Constraints:
* Length: 1 -
*
* @return The names of one or more attributes to retrieve. If no attribute names
* are specified, then all attributes will be returned. If any of the
* requested attributes are not found, they will not appear in the
* result.
You cannot use both AttributesToGet and
* Select together in a Query request, unless the
* value for Select is SPECIFIC_ATTRIBUTES
. (This
* usage is equivalent to specifying AttributesToGet without any
* value for Select.)
If you are querying a local secondary
* index and request only attributes that are projected into that index,
* the operation will read only the index and not the table. If any of
* the requested attributes are not projected into the local secondary
* index, DynamoDB will fetch each of these attributes from the parent
* table. This extra fetching incurs additional throughput cost and
* latency.
If you are querying a global secondary index, you can only
* request attributes that are projected into the index. Global secondary
* index queries cannot fetch attributes from the parent table.
*/
public java.util.List getAttributesToGet() {
return attributesToGet;
}
/**
* The names of one or more attributes to retrieve. If no attribute names
* are specified, then all attributes will be returned. If any of the
* requested attributes are not found, they will not appear in the
* result. You cannot use both AttributesToGet and
* Select together in a Query request, unless the
* value for Select is SPECIFIC_ATTRIBUTES
. (This
* usage is equivalent to specifying AttributesToGet without any
* value for Select.)
If you are querying a local secondary
* index and request only attributes that are projected into that index,
* the operation will read only the index and not the table. If any of
* the requested attributes are not projected into the local secondary
* index, DynamoDB will fetch each of these attributes from the parent
* table. This extra fetching incurs additional throughput cost and
* latency.
If you are querying a global secondary index, you can only
* request attributes that are projected into the index. Global secondary
* index queries cannot fetch attributes from the parent table.
*
* Constraints:
* Length: 1 -
*
* @param attributesToGet The names of one or more attributes to retrieve. If no attribute names
* are specified, then all attributes will be returned. If any of the
* requested attributes are not found, they will not appear in the
* result.
You cannot use both AttributesToGet and
* Select together in a Query request, unless the
* value for Select is SPECIFIC_ATTRIBUTES
. (This
* usage is equivalent to specifying AttributesToGet without any
* value for Select.)
If you are querying a local secondary
* index and request only attributes that are projected into that index,
* the operation will read only the index and not the table. If any of
* the requested attributes are not projected into the local secondary
* index, DynamoDB will fetch each of these attributes from the parent
* table. This extra fetching incurs additional throughput cost and
* latency.
If you are querying a global secondary index, you can only
* request attributes that are projected into the index. Global secondary
* index queries cannot fetch attributes from the parent table.
*/
public void setAttributesToGet(java.util.Collection attributesToGet) {
if (attributesToGet == null) {
this.attributesToGet = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag attributesToGetCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(attributesToGet.size());
attributesToGetCopy.addAll(attributesToGet);
this.attributesToGet = attributesToGetCopy;
}
/**
* The names of one or more attributes to retrieve. If no attribute names
* are specified, then all attributes will be returned. If any of the
* requested attributes are not found, they will not appear in the
* result. You cannot use both AttributesToGet and
* Select together in a Query request, unless the
* value for Select is SPECIFIC_ATTRIBUTES
. (This
* usage is equivalent to specifying AttributesToGet without any
* value for Select.)
If you are querying a local secondary
* index and request only attributes that are projected into that index,
* the operation will read only the index and not the table. If any of
* the requested attributes are not projected into the local secondary
* index, DynamoDB will fetch each of these attributes from the parent
* table. This extra fetching incurs additional throughput cost and
* latency.
If you are querying a global secondary index, you can only
* request attributes that are projected into the index. Global secondary
* index queries cannot fetch attributes from the parent table.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 -
*
* @param attributesToGet The names of one or more attributes to retrieve. If no attribute names
* are specified, then all attributes will be returned. If any of the
* requested attributes are not found, they will not appear in the
* result.
You cannot use both AttributesToGet and
* Select together in a Query request, unless the
* value for Select is SPECIFIC_ATTRIBUTES
. (This
* usage is equivalent to specifying AttributesToGet without any
* value for Select.)
If you are querying a local secondary
* index and request only attributes that are projected into that index,
* the operation will read only the index and not the table. If any of
* the requested attributes are not projected into the local secondary
* index, DynamoDB will fetch each of these attributes from the parent
* table. This extra fetching incurs additional throughput cost and
* latency.
If you are querying a global secondary index, you can only
* request attributes that are projected into the index. Global secondary
* index queries cannot fetch attributes from the parent table.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public QueryRequest withAttributesToGet(String... attributesToGet) {
if (getAttributesToGet() == null) setAttributesToGet(new java.util.ArrayList(attributesToGet.length));
for (String value : attributesToGet) {
getAttributesToGet().add(value);
}
return this;
}
/**
* The names of one or more attributes to retrieve. If no attribute names
* are specified, then all attributes will be returned. If any of the
* requested attributes are not found, they will not appear in the
* result. You cannot use both AttributesToGet and
* Select together in a Query request, unless the
* value for Select is SPECIFIC_ATTRIBUTES
. (This
* usage is equivalent to specifying AttributesToGet without any
* value for Select.)
If you are querying a local secondary
* index and request only attributes that are projected into that index,
* the operation will read only the index and not the table. If any of
* the requested attributes are not projected into the local secondary
* index, DynamoDB will fetch each of these attributes from the parent
* table. This extra fetching incurs additional throughput cost and
* latency.
If you are querying a global secondary index, you can only
* request attributes that are projected into the index. Global secondary
* index queries cannot fetch attributes from the parent table.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 -
*
* @param attributesToGet The names of one or more attributes to retrieve. If no attribute names
* are specified, then all attributes will be returned. If any of the
* requested attributes are not found, they will not appear in the
* result.
You cannot use both AttributesToGet and
* Select together in a Query request, unless the
* value for Select is SPECIFIC_ATTRIBUTES
. (This
* usage is equivalent to specifying AttributesToGet without any
* value for Select.)
If you are querying a local secondary
* index and request only attributes that are projected into that index,
* the operation will read only the index and not the table. If any of
* the requested attributes are not projected into the local secondary
* index, DynamoDB will fetch each of these attributes from the parent
* table. This extra fetching incurs additional throughput cost and
* latency.
If you are querying a global secondary index, you can only
* request attributes that are projected into the index. Global secondary
* index queries cannot fetch attributes from the parent table.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public QueryRequest withAttributesToGet(java.util.Collection attributesToGet) {
if (attributesToGet == null) {
this.attributesToGet = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag attributesToGetCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(attributesToGet.size());
attributesToGetCopy.addAll(attributesToGet);
this.attributesToGet = attributesToGetCopy;
}
return this;
}
/**
* The maximum number of items to evaluate (not necessarily the number of
* matching items). If DynamoDB processes the number of items up to the
* limit while processing the results, it stops the operation and returns
* the matching values up to that point, and a LastEvaluatedKey to
* apply in a subsequent operation, so that you can pick up where you
* left off. Also, if the processed data set size exceeds 1 MB before
* DynamoDB reaches this limit, it stops the operation and returns the
* matching values up to the limit, and a LastEvaluatedKey to
* apply in a subsequent operation to continue the operation. For more
* information see Query
* and Scan in the Amazon DynamoDB Developer Guide.
*
* Constraints:
* Range: 1 -
*
* @return The maximum number of items to evaluate (not necessarily the number of
* matching items). If DynamoDB processes the number of items up to the
* limit while processing the results, it stops the operation and returns
* the matching values up to that point, and a LastEvaluatedKey to
* apply in a subsequent operation, so that you can pick up where you
* left off. Also, if the processed data set size exceeds 1 MB before
* DynamoDB reaches this limit, it stops the operation and returns the
* matching values up to the limit, and a LastEvaluatedKey to
* apply in a subsequent operation to continue the operation. For more
* information see Query
* and Scan in the Amazon DynamoDB Developer Guide.
*/
public Integer getLimit() {
return limit;
}
/**
* The maximum number of items to evaluate (not necessarily the number of
* matching items). If DynamoDB processes the number of items up to the
* limit while processing the results, it stops the operation and returns
* the matching values up to that point, and a LastEvaluatedKey to
* apply in a subsequent operation, so that you can pick up where you
* left off. Also, if the processed data set size exceeds 1 MB before
* DynamoDB reaches this limit, it stops the operation and returns the
* matching values up to the limit, and a LastEvaluatedKey to
* apply in a subsequent operation to continue the operation. For more
* information see Query
* and Scan in the Amazon DynamoDB Developer Guide.
*
* Constraints:
* Range: 1 -
*
* @param limit The maximum number of items to evaluate (not necessarily the number of
* matching items). If DynamoDB processes the number of items up to the
* limit while processing the results, it stops the operation and returns
* the matching values up to that point, and a LastEvaluatedKey to
* apply in a subsequent operation, so that you can pick up where you
* left off. Also, if the processed data set size exceeds 1 MB before
* DynamoDB reaches this limit, it stops the operation and returns the
* matching values up to the limit, and a LastEvaluatedKey to
* apply in a subsequent operation to continue the operation. For more
* information see Query
* and Scan in the Amazon DynamoDB Developer Guide.
*/
public void setLimit(Integer limit) {
this.limit = limit;
}
/**
* The maximum number of items to evaluate (not necessarily the number of
* matching items). If DynamoDB processes the number of items up to the
* limit while processing the results, it stops the operation and returns
* the matching values up to that point, and a LastEvaluatedKey to
* apply in a subsequent operation, so that you can pick up where you
* left off. Also, if the processed data set size exceeds 1 MB before
* DynamoDB reaches this limit, it stops the operation and returns the
* matching values up to the limit, and a LastEvaluatedKey to
* apply in a subsequent operation to continue the operation. For more
* information see Query
* and Scan in the Amazon DynamoDB Developer Guide.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Range: 1 -
*
* @param limit The maximum number of items to evaluate (not necessarily the number of
* matching items). If DynamoDB processes the number of items up to the
* limit while processing the results, it stops the operation and returns
* the matching values up to that point, and a LastEvaluatedKey to
* apply in a subsequent operation, so that you can pick up where you
* left off. Also, if the processed data set size exceeds 1 MB before
* DynamoDB reaches this limit, it stops the operation and returns the
* matching values up to the limit, and a LastEvaluatedKey to
* apply in a subsequent operation to continue the operation. For more
* information see Query
* and Scan in the Amazon DynamoDB Developer Guide.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public QueryRequest withLimit(Integer limit) {
this.limit = limit;
return this;
}
/**
* If set to true
, then the operation uses strongly
* consistent reads; otherwise, eventually consistent reads are used.
*
Strongly consistent reads are not supported on global secondary
* indexes. If you query a global secondary index with
* ConsistentRead set to true
, you will receive an
* error message.
*
* @return If set to true
, then the operation uses strongly
* consistent reads; otherwise, eventually consistent reads are used.
*
Strongly consistent reads are not supported on global secondary
* indexes. If you query a global secondary index with
* ConsistentRead set to true
, you will receive an
* error message.
*/
public Boolean isConsistentRead() {
return consistentRead;
}
/**
* If set to true
, then the operation uses strongly
* consistent reads; otherwise, eventually consistent reads are used.
*
Strongly consistent reads are not supported on global secondary
* indexes. If you query a global secondary index with
* ConsistentRead set to true
, you will receive an
* error message.
*
* @param consistentRead If set to true
, then the operation uses strongly
* consistent reads; otherwise, eventually consistent reads are used.
*
Strongly consistent reads are not supported on global secondary
* indexes. If you query a global secondary index with
* ConsistentRead set to true
, you will receive an
* error message.
*/
public void setConsistentRead(Boolean consistentRead) {
this.consistentRead = consistentRead;
}
/**
* If set to true
, then the operation uses strongly
* consistent reads; otherwise, eventually consistent reads are used.
*
Strongly consistent reads are not supported on global secondary
* indexes. If you query a global secondary index with
* ConsistentRead set to true
, you will receive an
* error message.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param consistentRead If set to true
, then the operation uses strongly
* consistent reads; otherwise, eventually consistent reads are used.
*
Strongly consistent reads are not supported on global secondary
* indexes. If you query a global secondary index with
* ConsistentRead set to true
, you will receive an
* error message.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public QueryRequest withConsistentRead(Boolean consistentRead) {
this.consistentRead = consistentRead;
return this;
}
/**
* If set to true
, then the operation uses strongly
* consistent reads; otherwise, eventually consistent reads are used.
*
Strongly consistent reads are not supported on global secondary
* indexes. If you query a global secondary index with
* ConsistentRead set to true
, you will receive an
* error message.
*
* @return If set to true
, then the operation uses strongly
* consistent reads; otherwise, eventually consistent reads are used.
*
Strongly consistent reads are not supported on global secondary
* indexes. If you query a global secondary index with
* ConsistentRead set to true
, you will receive an
* error message.
*/
public Boolean getConsistentRead() {
return consistentRead;
}
/**
* The selection criteria for the query.
For a query on a table, you
* can only have conditions on the table primary key attributes. You must
* specify the hash key attribute name and value as an EQ
* condition. You can optionally specify a second condition, referring to
* the range key attribute.
For a query on an index, you can only have
* conditions on the index key attributes. You must specify the index
* hash attribute name and value as an EQ condition. You can optionally
* specify a second condition, referring to the index key range
* attribute.
Multiple conditions are evaluated using "AND"; in other
* words, all of the conditions must be met in order for an item to
* appear in the results results.
Each KeyConditions element
* consists of an attribute name to compare, along with the following:
*
AttributeValueList - One or more values to evaluate
* against the supplied attribute. This list contains exactly one value,
* except for a BETWEEN
comparison, in which case the list
* contains two values. For type Number, value comparisons are
* numeric.
String value comparisons for greater than, equals, or less
* than are based on ASCII character code values. For example,
* a
is greater than A
, and aa
is
* greater than B
. For a list of code values, see http://en.wikipedia.org/wiki/ASCII#ASCII_printable_characters.
*
For Binary, DynamoDB treats each byte of the binary data as
* unsigned when it compares binary values, for example when evaluating
* query expressions.
ComparisonOperator - A
* comparator for evaluating attributes. For example, equals, greater
* than, less than, etc.
Valid comparison operators for Query:
*
EQ | LE | LT | GE | GT | BEGINS_WITH | BETWEEN
For
* information on specifying data types in JSON, see JSON
* Data Format in the Amazon DynamoDB Developer Guide.
The
* following are descriptions of each comparison operator.
-
*
EQ
: Equal.
AttributeValueList can contain
* only one AttributeValue of type String, Number, or Binary (not
* a set). If an item contains an AttributeValue of a different
* type than the one specified in the request, the value does not match.
* For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not equal
* {"NS":["6", "2", "1"]}
.
-
*
LE
: Less than or equal.
AttributeValueList
* can contain only one AttributeValue of type String, Number, or
* Binary (not a set). If an item contains an AttributeValue of a
* different type than the one specified in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
LT
: Less than.
AttributeValueList can
* contain only one AttributeValue of type String, Number, or
* Binary (not a set). If an item contains an AttributeValue of a
* different type than the one specified in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
GE
: Greater than or equal.
*
AttributeValueList can contain only one
* AttributeValue of type String, Number, or Binary (not a set).
* If an item contains an AttributeValue of a different type than
* the one specified in the request, the value does not match. For
* example, {"S":"6"}
does not equal {"N":"6"}
.
* Also, {"N":"6"}
does not compare to {"NS":["6",
* "2", "1"]}
.
-
GT
: Greater than.
*
AttributeValueList can contain only one
* AttributeValue of type String, Number, or Binary (not a set).
* If an item contains an AttributeValue of a different type than
* the one specified in the request, the value does not match. For
* example, {"S":"6"}
does not equal {"N":"6"}
.
* Also, {"N":"6"}
does not compare to {"NS":["6",
* "2", "1"]}
.
-
BEGINS_WITH
:
* checks for a prefix.
AttributeValueList can contain only one
* AttributeValue of type String or Binary (not a Number or a
* set). The target attribute of the comparison must be a String or
* Binary (not a Number or a set).
-
*
BETWEEN
: Greater than or equal to the first value,
* and less than or equal to the second value.
*
AttributeValueList must contain two AttributeValue
* elements of the same type, either String, Number, or Binary (not a
* set). A target attribute matches if the target value is greater than,
* or equal to, the first element and less than, or equal to, the second
* element. If an item contains an AttributeValue of a different
* type than the one specified in the request, the value does not match.
* For example, {"S":"6"}
does not compare to
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
*
* @return The selection criteria for the query. For a query on a table, you
* can only have conditions on the table primary key attributes. You must
* specify the hash key attribute name and value as an EQ
* condition. You can optionally specify a second condition, referring to
* the range key attribute.
For a query on an index, you can only have
* conditions on the index key attributes. You must specify the index
* hash attribute name and value as an EQ condition. You can optionally
* specify a second condition, referring to the index key range
* attribute.
Multiple conditions are evaluated using "AND"; in other
* words, all of the conditions must be met in order for an item to
* appear in the results results.
Each KeyConditions element
* consists of an attribute name to compare, along with the following:
*
AttributeValueList - One or more values to evaluate
* against the supplied attribute. This list contains exactly one value,
* except for a BETWEEN
comparison, in which case the list
* contains two values. For type Number, value comparisons are
* numeric.
String value comparisons for greater than, equals, or less
* than are based on ASCII character code values. For example,
* a
is greater than A
, and aa
is
* greater than B
. For a list of code values, see http://en.wikipedia.org/wiki/ASCII#ASCII_printable_characters.
*
For Binary, DynamoDB treats each byte of the binary data as
* unsigned when it compares binary values, for example when evaluating
* query expressions.
ComparisonOperator - A
* comparator for evaluating attributes. For example, equals, greater
* than, less than, etc.
Valid comparison operators for Query:
*
EQ | LE | LT | GE | GT | BEGINS_WITH | BETWEEN
For
* information on specifying data types in JSON, see JSON
* Data Format in the Amazon DynamoDB Developer Guide.
The
* following are descriptions of each comparison operator.
-
*
EQ
: Equal.
AttributeValueList can contain
* only one AttributeValue of type String, Number, or Binary (not
* a set). If an item contains an AttributeValue of a different
* type than the one specified in the request, the value does not match.
* For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not equal
* {"NS":["6", "2", "1"]}
.
-
*
LE
: Less than or equal.
AttributeValueList
* can contain only one AttributeValue of type String, Number, or
* Binary (not a set). If an item contains an AttributeValue of a
* different type than the one specified in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
LT
: Less than.
AttributeValueList can
* contain only one AttributeValue of type String, Number, or
* Binary (not a set). If an item contains an AttributeValue of a
* different type than the one specified in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
GE
: Greater than or equal.
*
AttributeValueList can contain only one
* AttributeValue of type String, Number, or Binary (not a set).
* If an item contains an AttributeValue of a different type than
* the one specified in the request, the value does not match. For
* example, {"S":"6"}
does not equal {"N":"6"}
.
* Also, {"N":"6"}
does not compare to {"NS":["6",
* "2", "1"]}
.
-
GT
: Greater than.
*
AttributeValueList can contain only one
* AttributeValue of type String, Number, or Binary (not a set).
* If an item contains an AttributeValue of a different type than
* the one specified in the request, the value does not match. For
* example, {"S":"6"}
does not equal {"N":"6"}
.
* Also, {"N":"6"}
does not compare to {"NS":["6",
* "2", "1"]}
.
-
BEGINS_WITH
:
* checks for a prefix.
AttributeValueList can contain only one
* AttributeValue of type String or Binary (not a Number or a
* set). The target attribute of the comparison must be a String or
* Binary (not a Number or a set).
-
*
BETWEEN
: Greater than or equal to the first value,
* and less than or equal to the second value.
*
AttributeValueList must contain two AttributeValue
* elements of the same type, either String, Number, or Binary (not a
* set). A target attribute matches if the target value is greater than,
* or equal to, the first element and less than, or equal to, the second
* element. If an item contains an AttributeValue of a different
* type than the one specified in the request, the value does not match.
* For example, {"S":"6"}
does not compare to
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
*/
public java.util.Map getKeyConditions() {
return keyConditions;
}
/**
* The selection criteria for the query. For a query on a table, you
* can only have conditions on the table primary key attributes. You must
* specify the hash key attribute name and value as an EQ
* condition. You can optionally specify a second condition, referring to
* the range key attribute.
For a query on an index, you can only have
* conditions on the index key attributes. You must specify the index
* hash attribute name and value as an EQ condition. You can optionally
* specify a second condition, referring to the index key range
* attribute.
Multiple conditions are evaluated using "AND"; in other
* words, all of the conditions must be met in order for an item to
* appear in the results results.
Each KeyConditions element
* consists of an attribute name to compare, along with the following:
*
AttributeValueList - One or more values to evaluate
* against the supplied attribute. This list contains exactly one value,
* except for a BETWEEN
comparison, in which case the list
* contains two values. For type Number, value comparisons are
* numeric.
String value comparisons for greater than, equals, or less
* than are based on ASCII character code values. For example,
* a
is greater than A
, and aa
is
* greater than B
. For a list of code values, see http://en.wikipedia.org/wiki/ASCII#ASCII_printable_characters.
*
For Binary, DynamoDB treats each byte of the binary data as
* unsigned when it compares binary values, for example when evaluating
* query expressions.
ComparisonOperator - A
* comparator for evaluating attributes. For example, equals, greater
* than, less than, etc.
Valid comparison operators for Query:
*
EQ | LE | LT | GE | GT | BEGINS_WITH | BETWEEN
For
* information on specifying data types in JSON, see JSON
* Data Format in the Amazon DynamoDB Developer Guide.
The
* following are descriptions of each comparison operator.
-
*
EQ
: Equal.
AttributeValueList can contain
* only one AttributeValue of type String, Number, or Binary (not
* a set). If an item contains an AttributeValue of a different
* type than the one specified in the request, the value does not match.
* For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not equal
* {"NS":["6", "2", "1"]}
.
-
*
LE
: Less than or equal.
AttributeValueList
* can contain only one AttributeValue of type String, Number, or
* Binary (not a set). If an item contains an AttributeValue of a
* different type than the one specified in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
LT
: Less than.
AttributeValueList can
* contain only one AttributeValue of type String, Number, or
* Binary (not a set). If an item contains an AttributeValue of a
* different type than the one specified in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
GE
: Greater than or equal.
*
AttributeValueList can contain only one
* AttributeValue of type String, Number, or Binary (not a set).
* If an item contains an AttributeValue of a different type than
* the one specified in the request, the value does not match. For
* example, {"S":"6"}
does not equal {"N":"6"}
.
* Also, {"N":"6"}
does not compare to {"NS":["6",
* "2", "1"]}
.
-
GT
: Greater than.
*
AttributeValueList can contain only one
* AttributeValue of type String, Number, or Binary (not a set).
* If an item contains an AttributeValue of a different type than
* the one specified in the request, the value does not match. For
* example, {"S":"6"}
does not equal {"N":"6"}
.
* Also, {"N":"6"}
does not compare to {"NS":["6",
* "2", "1"]}
.
-
BEGINS_WITH
:
* checks for a prefix.
AttributeValueList can contain only one
* AttributeValue of type String or Binary (not a Number or a
* set). The target attribute of the comparison must be a String or
* Binary (not a Number or a set).
-
*
BETWEEN
: Greater than or equal to the first value,
* and less than or equal to the second value.
*
AttributeValueList must contain two AttributeValue
* elements of the same type, either String, Number, or Binary (not a
* set). A target attribute matches if the target value is greater than,
* or equal to, the first element and less than, or equal to, the second
* element. If an item contains an AttributeValue of a different
* type than the one specified in the request, the value does not match.
* For example, {"S":"6"}
does not compare to
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
*
* @param keyConditions The selection criteria for the query. For a query on a table, you
* can only have conditions on the table primary key attributes. You must
* specify the hash key attribute name and value as an EQ
* condition. You can optionally specify a second condition, referring to
* the range key attribute.
For a query on an index, you can only have
* conditions on the index key attributes. You must specify the index
* hash attribute name and value as an EQ condition. You can optionally
* specify a second condition, referring to the index key range
* attribute.
Multiple conditions are evaluated using "AND"; in other
* words, all of the conditions must be met in order for an item to
* appear in the results results.
Each KeyConditions element
* consists of an attribute name to compare, along with the following:
*
AttributeValueList - One or more values to evaluate
* against the supplied attribute. This list contains exactly one value,
* except for a BETWEEN
comparison, in which case the list
* contains two values. For type Number, value comparisons are
* numeric.
String value comparisons for greater than, equals, or less
* than are based on ASCII character code values. For example,
* a
is greater than A
, and aa
is
* greater than B
. For a list of code values, see http://en.wikipedia.org/wiki/ASCII#ASCII_printable_characters.
*
For Binary, DynamoDB treats each byte of the binary data as
* unsigned when it compares binary values, for example when evaluating
* query expressions.
ComparisonOperator - A
* comparator for evaluating attributes. For example, equals, greater
* than, less than, etc.
Valid comparison operators for Query:
*
EQ | LE | LT | GE | GT | BEGINS_WITH | BETWEEN
For
* information on specifying data types in JSON, see JSON
* Data Format in the Amazon DynamoDB Developer Guide.
The
* following are descriptions of each comparison operator.
-
*
EQ
: Equal.
AttributeValueList can contain
* only one AttributeValue of type String, Number, or Binary (not
* a set). If an item contains an AttributeValue of a different
* type than the one specified in the request, the value does not match.
* For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not equal
* {"NS":["6", "2", "1"]}
.
-
*
LE
: Less than or equal.
AttributeValueList
* can contain only one AttributeValue of type String, Number, or
* Binary (not a set). If an item contains an AttributeValue of a
* different type than the one specified in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
LT
: Less than.
AttributeValueList can
* contain only one AttributeValue of type String, Number, or
* Binary (not a set). If an item contains an AttributeValue of a
* different type than the one specified in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
GE
: Greater than or equal.
*
AttributeValueList can contain only one
* AttributeValue of type String, Number, or Binary (not a set).
* If an item contains an AttributeValue of a different type than
* the one specified in the request, the value does not match. For
* example, {"S":"6"}
does not equal {"N":"6"}
.
* Also, {"N":"6"}
does not compare to {"NS":["6",
* "2", "1"]}
.
-
GT
: Greater than.
*
AttributeValueList can contain only one
* AttributeValue of type String, Number, or Binary (not a set).
* If an item contains an AttributeValue of a different type than
* the one specified in the request, the value does not match. For
* example, {"S":"6"}
does not equal {"N":"6"}
.
* Also, {"N":"6"}
does not compare to {"NS":["6",
* "2", "1"]}
.
-
BEGINS_WITH
:
* checks for a prefix.
AttributeValueList can contain only one
* AttributeValue of type String or Binary (not a Number or a
* set). The target attribute of the comparison must be a String or
* Binary (not a Number or a set).
-
*
BETWEEN
: Greater than or equal to the first value,
* and less than or equal to the second value.
*
AttributeValueList must contain two AttributeValue
* elements of the same type, either String, Number, or Binary (not a
* set). A target attribute matches if the target value is greater than,
* or equal to, the first element and less than, or equal to, the second
* element. If an item contains an AttributeValue of a different
* type than the one specified in the request, the value does not match.
* For example, {"S":"6"}
does not compare to
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
*/
public void setKeyConditions(java.util.Map keyConditions) {
this.keyConditions = keyConditions;
}
/**
* The selection criteria for the query. For a query on a table, you
* can only have conditions on the table primary key attributes. You must
* specify the hash key attribute name and value as an EQ
* condition. You can optionally specify a second condition, referring to
* the range key attribute.
For a query on an index, you can only have
* conditions on the index key attributes. You must specify the index
* hash attribute name and value as an EQ condition. You can optionally
* specify a second condition, referring to the index key range
* attribute.
Multiple conditions are evaluated using "AND"; in other
* words, all of the conditions must be met in order for an item to
* appear in the results results.
Each KeyConditions element
* consists of an attribute name to compare, along with the following:
*
AttributeValueList - One or more values to evaluate
* against the supplied attribute. This list contains exactly one value,
* except for a BETWEEN
comparison, in which case the list
* contains two values. For type Number, value comparisons are
* numeric.
String value comparisons for greater than, equals, or less
* than are based on ASCII character code values. For example,
* a
is greater than A
, and aa
is
* greater than B
. For a list of code values, see http://en.wikipedia.org/wiki/ASCII#ASCII_printable_characters.
*
For Binary, DynamoDB treats each byte of the binary data as
* unsigned when it compares binary values, for example when evaluating
* query expressions.
ComparisonOperator - A
* comparator for evaluating attributes. For example, equals, greater
* than, less than, etc.
Valid comparison operators for Query:
*
EQ | LE | LT | GE | GT | BEGINS_WITH | BETWEEN
For
* information on specifying data types in JSON, see JSON
* Data Format in the Amazon DynamoDB Developer Guide.
The
* following are descriptions of each comparison operator.
-
*
EQ
: Equal.
AttributeValueList can contain
* only one AttributeValue of type String, Number, or Binary (not
* a set). If an item contains an AttributeValue of a different
* type than the one specified in the request, the value does not match.
* For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not equal
* {"NS":["6", "2", "1"]}
.
-
*
LE
: Less than or equal.
AttributeValueList
* can contain only one AttributeValue of type String, Number, or
* Binary (not a set). If an item contains an AttributeValue of a
* different type than the one specified in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
LT
: Less than.
AttributeValueList can
* contain only one AttributeValue of type String, Number, or
* Binary (not a set). If an item contains an AttributeValue of a
* different type than the one specified in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
GE
: Greater than or equal.
*
AttributeValueList can contain only one
* AttributeValue of type String, Number, or Binary (not a set).
* If an item contains an AttributeValue of a different type than
* the one specified in the request, the value does not match. For
* example, {"S":"6"}
does not equal {"N":"6"}
.
* Also, {"N":"6"}
does not compare to {"NS":["6",
* "2", "1"]}
.
-
GT
: Greater than.
*
AttributeValueList can contain only one
* AttributeValue of type String, Number, or Binary (not a set).
* If an item contains an AttributeValue of a different type than
* the one specified in the request, the value does not match. For
* example, {"S":"6"}
does not equal {"N":"6"}
.
* Also, {"N":"6"}
does not compare to {"NS":["6",
* "2", "1"]}
.
-
BEGINS_WITH
:
* checks for a prefix.
AttributeValueList can contain only one
* AttributeValue of type String or Binary (not a Number or a
* set). The target attribute of the comparison must be a String or
* Binary (not a Number or a set).
-
*
BETWEEN
: Greater than or equal to the first value,
* and less than or equal to the second value.
*
AttributeValueList must contain two AttributeValue
* elements of the same type, either String, Number, or Binary (not a
* set). A target attribute matches if the target value is greater than,
* or equal to, the first element and less than, or equal to, the second
* element. If an item contains an AttributeValue of a different
* type than the one specified in the request, the value does not match.
* For example, {"S":"6"}
does not compare to
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param keyConditions The selection criteria for the query.
For a query on a table, you
* can only have conditions on the table primary key attributes. You must
* specify the hash key attribute name and value as an EQ
* condition. You can optionally specify a second condition, referring to
* the range key attribute.
For a query on an index, you can only have
* conditions on the index key attributes. You must specify the index
* hash attribute name and value as an EQ condition. You can optionally
* specify a second condition, referring to the index key range
* attribute.
Multiple conditions are evaluated using "AND"; in other
* words, all of the conditions must be met in order for an item to
* appear in the results results.
Each KeyConditions element
* consists of an attribute name to compare, along with the following:
*
AttributeValueList - One or more values to evaluate
* against the supplied attribute. This list contains exactly one value,
* except for a BETWEEN
comparison, in which case the list
* contains two values. For type Number, value comparisons are
* numeric.
String value comparisons for greater than, equals, or less
* than are based on ASCII character code values. For example,
* a
is greater than A
, and aa
is
* greater than B
. For a list of code values, see http://en.wikipedia.org/wiki/ASCII#ASCII_printable_characters.
*
For Binary, DynamoDB treats each byte of the binary data as
* unsigned when it compares binary values, for example when evaluating
* query expressions.
ComparisonOperator - A
* comparator for evaluating attributes. For example, equals, greater
* than, less than, etc.
Valid comparison operators for Query:
*
EQ | LE | LT | GE | GT | BEGINS_WITH | BETWEEN
For
* information on specifying data types in JSON, see JSON
* Data Format in the Amazon DynamoDB Developer Guide.
The
* following are descriptions of each comparison operator.
-
*
EQ
: Equal.
AttributeValueList can contain
* only one AttributeValue of type String, Number, or Binary (not
* a set). If an item contains an AttributeValue of a different
* type than the one specified in the request, the value does not match.
* For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not equal
* {"NS":["6", "2", "1"]}
.
-
*
LE
: Less than or equal.
AttributeValueList
* can contain only one AttributeValue of type String, Number, or
* Binary (not a set). If an item contains an AttributeValue of a
* different type than the one specified in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
LT
: Less than.
AttributeValueList can
* contain only one AttributeValue of type String, Number, or
* Binary (not a set). If an item contains an AttributeValue of a
* different type than the one specified in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
GE
: Greater than or equal.
*
AttributeValueList can contain only one
* AttributeValue of type String, Number, or Binary (not a set).
* If an item contains an AttributeValue of a different type than
* the one specified in the request, the value does not match. For
* example, {"S":"6"}
does not equal {"N":"6"}
.
* Also, {"N":"6"}
does not compare to {"NS":["6",
* "2", "1"]}
.
-
GT
: Greater than.
*
AttributeValueList can contain only one
* AttributeValue of type String, Number, or Binary (not a set).
* If an item contains an AttributeValue of a different type than
* the one specified in the request, the value does not match. For
* example, {"S":"6"}
does not equal {"N":"6"}
.
* Also, {"N":"6"}
does not compare to {"NS":["6",
* "2", "1"]}
.
-
BEGINS_WITH
:
* checks for a prefix.
AttributeValueList can contain only one
* AttributeValue of type String or Binary (not a Number or a
* set). The target attribute of the comparison must be a String or
* Binary (not a Number or a set).
-
*
BETWEEN
: Greater than or equal to the first value,
* and less than or equal to the second value.
*
AttributeValueList must contain two AttributeValue
* elements of the same type, either String, Number, or Binary (not a
* set). A target attribute matches if the target value is greater than,
* or equal to, the first element and less than, or equal to, the second
* element. If an item contains an AttributeValue of a different
* type than the one specified in the request, the value does not match.
* For example, {"S":"6"}
does not compare to
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public QueryRequest withKeyConditions(java.util.Map keyConditions) {
setKeyConditions(keyConditions);
return this;
}
/**
* The selection criteria for the query. For a query on a table, you
* can only have conditions on the table primary key attributes. You must
* specify the hash key attribute name and value as an EQ
* condition. You can optionally specify a second condition, referring to
* the range key attribute.
For a query on an index, you can only have
* conditions on the index key attributes. You must specify the index
* hash attribute name and value as an EQ condition. You can optionally
* specify a second condition, referring to the index key range
* attribute.
Multiple conditions are evaluated using "AND"; in other
* words, all of the conditions must be met in order for an item to
* appear in the results results.
Each KeyConditions element
* consists of an attribute name to compare, along with the following:
*
AttributeValueList - One or more values to evaluate
* against the supplied attribute. This list contains exactly one value,
* except for a BETWEEN
comparison, in which case the list
* contains two values. For type Number, value comparisons are
* numeric.
String value comparisons for greater than, equals, or less
* than are based on ASCII character code values. For example,
* a
is greater than A
, and aa
is
* greater than B
. For a list of code values, see http://en.wikipedia.org/wiki/ASCII#ASCII_printable_characters.
*
For Binary, DynamoDB treats each byte of the binary data as
* unsigned when it compares binary values, for example when evaluating
* query expressions.
ComparisonOperator - A
* comparator for evaluating attributes. For example, equals, greater
* than, less than, etc.
Valid comparison operators for Query:
*
EQ | LE | LT | GE | GT | BEGINS_WITH | BETWEEN
For
* information on specifying data types in JSON, see JSON
* Data Format in the Amazon DynamoDB Developer Guide.
The
* following are descriptions of each comparison operator.
-
*
EQ
: Equal.
AttributeValueList can contain
* only one AttributeValue of type String, Number, or Binary (not
* a set). If an item contains an AttributeValue of a different
* type than the one specified in the request, the value does not match.
* For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not equal
* {"NS":["6", "2", "1"]}
.
-
*
LE
: Less than or equal.
AttributeValueList
* can contain only one AttributeValue of type String, Number, or
* Binary (not a set). If an item contains an AttributeValue of a
* different type than the one specified in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
LT
: Less than.
AttributeValueList can
* contain only one AttributeValue of type String, Number, or
* Binary (not a set). If an item contains an AttributeValue of a
* different type than the one specified in the request, the value does
* not match. For example, {"S":"6"}
does not equal
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
.
-
*
GE
: Greater than or equal.
*
AttributeValueList can contain only one
* AttributeValue of type String, Number, or Binary (not a set).
* If an item contains an AttributeValue of a different type than
* the one specified in the request, the value does not match. For
* example, {"S":"6"}
does not equal {"N":"6"}
.
* Also, {"N":"6"}
does not compare to {"NS":["6",
* "2", "1"]}
.
-
GT
: Greater than.
*
AttributeValueList can contain only one
* AttributeValue of type String, Number, or Binary (not a set).
* If an item contains an AttributeValue of a different type than
* the one specified in the request, the value does not match. For
* example, {"S":"6"}
does not equal {"N":"6"}
.
* Also, {"N":"6"}
does not compare to {"NS":["6",
* "2", "1"]}
.
-
BEGINS_WITH
:
* checks for a prefix.
AttributeValueList can contain only one
* AttributeValue of type String or Binary (not a Number or a
* set). The target attribute of the comparison must be a String or
* Binary (not a Number or a set).
-
*
BETWEEN
: Greater than or equal to the first value,
* and less than or equal to the second value.
*
AttributeValueList must contain two AttributeValue
* elements of the same type, either String, Number, or Binary (not a
* set). A target attribute matches if the target value is greater than,
* or equal to, the first element and less than, or equal to, the second
* element. If an item contains an AttributeValue of a different
* type than the one specified in the request, the value does not match.
* For example, {"S":"6"}
does not compare to
* {"N":"6"}
. Also, {"N":"6"}
does not compare
* to {"NS":["6", "2", "1"]}
*
* The method adds a new key-value pair into KeyConditions parameter, and
* returns a reference to this object so that method calls can be chained
* together.
*
* @param key The key of the entry to be added into KeyConditions.
* @param value The corresponding value of the entry to be added into KeyConditions.
*/
public QueryRequest addKeyConditionsEntry(String key, Condition value) {
if (null == this.keyConditions) {
this.keyConditions = new java.util.HashMap();
}
if (this.keyConditions.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.keyConditions.put(key, value);
return this;
}
/**
* Removes all the entries added into KeyConditions.
*
* Returns a reference to this object so that method calls can be chained together.
*/
public QueryRequest clearKeyConditionsEntries() {
this.keyConditions = null;
return this;
}
/**
* Specifies ascending (true) or descending (false) traversal of the
* index. DynamoDB returns results reflecting the requested order
* determined by the range key. If the data type is Number, the results
* are returned in numeric order. For String, the results are returned in
* order of ASCII character code values. For Binary, Amazon DynamoDB
* treats each byte of the binary data as unsigned when it compares
* binary values.
If ScanIndexForward is not specified, the
* results are returned in ascending order.
*
* @return Specifies ascending (true) or descending (false) traversal of the
* index. DynamoDB returns results reflecting the requested order
* determined by the range key. If the data type is Number, the results
* are returned in numeric order. For String, the results are returned in
* order of ASCII character code values. For Binary, Amazon DynamoDB
* treats each byte of the binary data as unsigned when it compares
* binary values.
If ScanIndexForward is not specified, the
* results are returned in ascending order.
*/
public Boolean isScanIndexForward() {
return scanIndexForward;
}
/**
* Specifies ascending (true) or descending (false) traversal of the
* index. DynamoDB returns results reflecting the requested order
* determined by the range key. If the data type is Number, the results
* are returned in numeric order. For String, the results are returned in
* order of ASCII character code values. For Binary, Amazon DynamoDB
* treats each byte of the binary data as unsigned when it compares
* binary values.
If ScanIndexForward is not specified, the
* results are returned in ascending order.
*
* @param scanIndexForward Specifies ascending (true) or descending (false) traversal of the
* index. DynamoDB returns results reflecting the requested order
* determined by the range key. If the data type is Number, the results
* are returned in numeric order. For String, the results are returned in
* order of ASCII character code values. For Binary, Amazon DynamoDB
* treats each byte of the binary data as unsigned when it compares
* binary values.
If ScanIndexForward is not specified, the
* results are returned in ascending order.
*/
public void setScanIndexForward(Boolean scanIndexForward) {
this.scanIndexForward = scanIndexForward;
}
/**
* Specifies ascending (true) or descending (false) traversal of the
* index. DynamoDB returns results reflecting the requested order
* determined by the range key. If the data type is Number, the results
* are returned in numeric order. For String, the results are returned in
* order of ASCII character code values. For Binary, Amazon DynamoDB
* treats each byte of the binary data as unsigned when it compares
* binary values.
If ScanIndexForward is not specified, the
* results are returned in ascending order.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param scanIndexForward Specifies ascending (true) or descending (false) traversal of the
* index. DynamoDB returns results reflecting the requested order
* determined by the range key. If the data type is Number, the results
* are returned in numeric order. For String, the results are returned in
* order of ASCII character code values. For Binary, Amazon DynamoDB
* treats each byte of the binary data as unsigned when it compares
* binary values.
If ScanIndexForward is not specified, the
* results are returned in ascending order.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public QueryRequest withScanIndexForward(Boolean scanIndexForward) {
this.scanIndexForward = scanIndexForward;
return this;
}
/**
* Specifies ascending (true) or descending (false) traversal of the
* index. DynamoDB returns results reflecting the requested order
* determined by the range key. If the data type is Number, the results
* are returned in numeric order. For String, the results are returned in
* order of ASCII character code values. For Binary, Amazon DynamoDB
* treats each byte of the binary data as unsigned when it compares
* binary values.
If ScanIndexForward is not specified, the
* results are returned in ascending order.
*
* @return Specifies ascending (true) or descending (false) traversal of the
* index. DynamoDB returns results reflecting the requested order
* determined by the range key. If the data type is Number, the results
* are returned in numeric order. For String, the results are returned in
* order of ASCII character code values. For Binary, Amazon DynamoDB
* treats each byte of the binary data as unsigned when it compares
* binary values.
If ScanIndexForward is not specified, the
* results are returned in ascending order.
*/
public Boolean getScanIndexForward() {
return scanIndexForward;
}
/**
* The primary key of the first item that this operation will evaluate.
* Use the value that was returned for LastEvaluatedKey in the
* previous operation.
The data type for ExclusiveStartKey must
* be String, Number or Binary. No set data types are allowed.
*
* @return The primary key of the first item that this operation will evaluate.
* Use the value that was returned for LastEvaluatedKey in the
* previous operation.
The data type for ExclusiveStartKey must
* be String, Number or Binary. No set data types are allowed.
*/
public java.util.Map getExclusiveStartKey() {
return exclusiveStartKey;
}
/**
* The primary key of the first item that this operation will evaluate.
* Use the value that was returned for LastEvaluatedKey in the
* previous operation. The data type for ExclusiveStartKey must
* be String, Number or Binary. No set data types are allowed.
*
* @param exclusiveStartKey The primary key of the first item that this operation will evaluate.
* Use the value that was returned for LastEvaluatedKey in the
* previous operation.
The data type for ExclusiveStartKey must
* be String, Number or Binary. No set data types are allowed.
*/
public void setExclusiveStartKey(java.util.Map exclusiveStartKey) {
this.exclusiveStartKey = exclusiveStartKey;
}
/**
* The primary key of the first item that this operation will evaluate.
* Use the value that was returned for LastEvaluatedKey in the
* previous operation. The data type for ExclusiveStartKey must
* be String, Number or Binary. No set data types are allowed.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param exclusiveStartKey The primary key of the first item that this operation will evaluate.
* Use the value that was returned for LastEvaluatedKey in the
* previous operation.
The data type for ExclusiveStartKey must
* be String, Number or Binary. No set data types are allowed.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public QueryRequest withExclusiveStartKey(java.util.Map exclusiveStartKey) {
setExclusiveStartKey(exclusiveStartKey);
return this;
}
/**
* The primary key of the first item that this operation will evaluate.
* Use the value that was returned for LastEvaluatedKey in the
* previous operation. The data type for ExclusiveStartKey must
* be String, Number or Binary. No set data types are allowed.
*
* This method accepts the hashKey, rangeKey of ExclusiveStartKey as
* java.util.Map.Entry objects.
*
* @param hashKey Primary hash key.
* @param rangeKey Primary range key. (null if it a hash-only table)
*/
public void setExclusiveStartKey(java.util.Map.Entry hashKey, java.util.Map.Entry rangeKey) throws IllegalArgumentException {
java.util.HashMap exclusiveStartKey = new java.util.HashMap();
if (hashKey != null) {
exclusiveStartKey.put(hashKey.getKey(), hashKey.getValue());
} else
throw new IllegalArgumentException("hashKey must be non-null object.");
if (rangeKey != null) {
exclusiveStartKey.put(rangeKey.getKey(), rangeKey.getValue());
}
setExclusiveStartKey(exclusiveStartKey);
}
/**
* The primary key of the first item that this operation will evaluate.
* Use the value that was returned for LastEvaluatedKey in the
* previous operation. The data type for ExclusiveStartKey must
* be String, Number or Binary. No set data types are allowed.
*
* This method accepts the hashKey, rangeKey of ExclusiveStartKey as
* java.util.Map.Entry objects.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param hashKey Primary hash key.
* @param rangeKey Primary range key. (null if it a hash-only table)
*/
public QueryRequest withExclusiveStartKey(java.util.Map.Entry hashKey, java.util.Map.Entry rangeKey) throws IllegalArgumentException {
setExclusiveStartKey(hashKey, rangeKey);
return this;
}
/**
* The primary key of the first item that this operation will evaluate.
* Use the value that was returned for LastEvaluatedKey in the
* previous operation. The data type for ExclusiveStartKey must
* be String, Number or Binary. No set data types are allowed.
*
* The method adds a new key-value pair into ExclusiveStartKey parameter,
* and returns a reference to this object so that method calls can be
* chained together.
*
* @param key The key of the entry to be added into ExclusiveStartKey.
* @param value The corresponding value of the entry to be added into ExclusiveStartKey.
*/
public QueryRequest addExclusiveStartKeyEntry(String key, AttributeValue value) {
if (null == this.exclusiveStartKey) {
this.exclusiveStartKey = new java.util.HashMap();
}
if (this.exclusiveStartKey.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.exclusiveStartKey.put(key, value);
return this;
}
/**
* Removes all the entries added into ExclusiveStartKey.
*
* Returns a reference to this object so that method calls can be chained together.
*/
public QueryRequest clearExclusiveStartKeyEntries() {
this.exclusiveStartKey = null;
return this;
}
/**
* If set to TOTAL
, the response includes
* ConsumedCapacity data for tables and indexes. If set to
* INDEXES
, the response includes ConsumedCapacity
* for indexes. If set to NONE
(the default),
* ConsumedCapacity is not included in the response.
*
* Constraints:
* Allowed Values: INDEXES, TOTAL, NONE
*
* @return If set to TOTAL
, the response includes
* ConsumedCapacity data for tables and indexes. If set to
* INDEXES
, the response includes ConsumedCapacity
* for indexes. If set to NONE
(the default),
* ConsumedCapacity is not included in the response.
*
* @see ReturnConsumedCapacity
*/
public String getReturnConsumedCapacity() {
return returnConsumedCapacity;
}
/**
* If set to TOTAL
, the response includes
* ConsumedCapacity data for tables and indexes. If set to
* INDEXES
, the response includes ConsumedCapacity
* for indexes. If set to NONE
(the default),
* ConsumedCapacity is not included in the response.
*
* Constraints:
* Allowed Values: INDEXES, TOTAL, NONE
*
* @param returnConsumedCapacity If set to TOTAL
, the response includes
* ConsumedCapacity data for tables and indexes. If set to
* INDEXES
, the response includes ConsumedCapacity
* for indexes. If set to NONE
(the default),
* ConsumedCapacity is not included in the response.
*
* @see ReturnConsumedCapacity
*/
public void setReturnConsumedCapacity(String returnConsumedCapacity) {
this.returnConsumedCapacity = returnConsumedCapacity;
}
/**
* If set to TOTAL
, the response includes
* ConsumedCapacity data for tables and indexes. If set to
* INDEXES
, the response includes ConsumedCapacity
* for indexes. If set to NONE
(the default),
* ConsumedCapacity is not included in the response.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: INDEXES, TOTAL, NONE
*
* @param returnConsumedCapacity If set to TOTAL
, the response includes
* ConsumedCapacity data for tables and indexes. If set to
* INDEXES
, the response includes ConsumedCapacity
* for indexes. If set to NONE
(the default),
* ConsumedCapacity is not included in the response.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see ReturnConsumedCapacity
*/
public QueryRequest withReturnConsumedCapacity(String returnConsumedCapacity) {
this.returnConsumedCapacity = returnConsumedCapacity;
return this;
}
/**
* If set to TOTAL
, the response includes
* ConsumedCapacity data for tables and indexes. If set to
* INDEXES
, the response includes ConsumedCapacity
* for indexes. If set to NONE
(the default),
* ConsumedCapacity is not included in the response.
*
* Constraints:
* Allowed Values: INDEXES, TOTAL, NONE
*
* @param returnConsumedCapacity If set to TOTAL
, the response includes
* ConsumedCapacity data for tables and indexes. If set to
* INDEXES
, the response includes ConsumedCapacity
* for indexes. If set to NONE
(the default),
* ConsumedCapacity is not included in the response.
*
* @see ReturnConsumedCapacity
*/
public void setReturnConsumedCapacity(ReturnConsumedCapacity returnConsumedCapacity) {
this.returnConsumedCapacity = returnConsumedCapacity.toString();
}
/**
* If set to TOTAL
, the response includes
* ConsumedCapacity data for tables and indexes. If set to
* INDEXES
, the response includes ConsumedCapacity
* for indexes. If set to NONE
(the default),
* ConsumedCapacity is not included in the response.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: INDEXES, TOTAL, NONE
*
* @param returnConsumedCapacity If set to TOTAL
, the response includes
* ConsumedCapacity data for tables and indexes. If set to
* INDEXES
, the response includes ConsumedCapacity
* for indexes. If set to NONE
(the default),
* ConsumedCapacity is not included in the response.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see ReturnConsumedCapacity
*/
public QueryRequest withReturnConsumedCapacity(ReturnConsumedCapacity returnConsumedCapacity) {
this.returnConsumedCapacity = returnConsumedCapacity.toString();
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTableName() != null) sb.append("TableName: " + getTableName() + ",");
if (getIndexName() != null) sb.append("IndexName: " + getIndexName() + ",");
if (getSelect() != null) sb.append("Select: " + getSelect() + ",");
if (getAttributesToGet() != null) sb.append("AttributesToGet: " + getAttributesToGet() + ",");
if (getLimit() != null) sb.append("Limit: " + getLimit() + ",");
if (isConsistentRead() != null) sb.append("ConsistentRead: " + isConsistentRead() + ",");
if (getKeyConditions() != null) sb.append("KeyConditions: " + getKeyConditions() + ",");
if (isScanIndexForward() != null) sb.append("ScanIndexForward: " + isScanIndexForward() + ",");
if (getExclusiveStartKey() != null) sb.append("ExclusiveStartKey: " + getExclusiveStartKey() + ",");
if (getReturnConsumedCapacity() != null) sb.append("ReturnConsumedCapacity: " + getReturnConsumedCapacity() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTableName() == null) ? 0 : getTableName().hashCode());
hashCode = prime * hashCode + ((getIndexName() == null) ? 0 : getIndexName().hashCode());
hashCode = prime * hashCode + ((getSelect() == null) ? 0 : getSelect().hashCode());
hashCode = prime * hashCode + ((getAttributesToGet() == null) ? 0 : getAttributesToGet().hashCode());
hashCode = prime * hashCode + ((getLimit() == null) ? 0 : getLimit().hashCode());
hashCode = prime * hashCode + ((isConsistentRead() == null) ? 0 : isConsistentRead().hashCode());
hashCode = prime * hashCode + ((getKeyConditions() == null) ? 0 : getKeyConditions().hashCode());
hashCode = prime * hashCode + ((isScanIndexForward() == null) ? 0 : isScanIndexForward().hashCode());
hashCode = prime * hashCode + ((getExclusiveStartKey() == null) ? 0 : getExclusiveStartKey().hashCode());
hashCode = prime * hashCode + ((getReturnConsumedCapacity() == null) ? 0 : getReturnConsumedCapacity().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof QueryRequest == false) return false;
QueryRequest other = (QueryRequest)obj;
if (other.getTableName() == null ^ this.getTableName() == null) return false;
if (other.getTableName() != null && other.getTableName().equals(this.getTableName()) == false) return false;
if (other.getIndexName() == null ^ this.getIndexName() == null) return false;
if (other.getIndexName() != null && other.getIndexName().equals(this.getIndexName()) == false) return false;
if (other.getSelect() == null ^ this.getSelect() == null) return false;
if (other.getSelect() != null && other.getSelect().equals(this.getSelect()) == false) return false;
if (other.getAttributesToGet() == null ^ this.getAttributesToGet() == null) return false;
if (other.getAttributesToGet() != null && other.getAttributesToGet().equals(this.getAttributesToGet()) == false) return false;
if (other.getLimit() == null ^ this.getLimit() == null) return false;
if (other.getLimit() != null && other.getLimit().equals(this.getLimit()) == false) return false;
if (other.isConsistentRead() == null ^ this.isConsistentRead() == null) return false;
if (other.isConsistentRead() != null && other.isConsistentRead().equals(this.isConsistentRead()) == false) return false;
if (other.getKeyConditions() == null ^ this.getKeyConditions() == null) return false;
if (other.getKeyConditions() != null && other.getKeyConditions().equals(this.getKeyConditions()) == false) return false;
if (other.isScanIndexForward() == null ^ this.isScanIndexForward() == null) return false;
if (other.isScanIndexForward() != null && other.isScanIndexForward().equals(this.isScanIndexForward()) == false) return false;
if (other.getExclusiveStartKey() == null ^ this.getExclusiveStartKey() == null) return false;
if (other.getExclusiveStartKey() != null && other.getExclusiveStartKey().equals(this.getExclusiveStartKey()) == false) return false;
if (other.getReturnConsumedCapacity() == null ^ this.getReturnConsumedCapacity() == null) return false;
if (other.getReturnConsumedCapacity() != null && other.getReturnConsumedCapacity().equals(this.getReturnConsumedCapacity()) == false) return false;
return true;
}
}