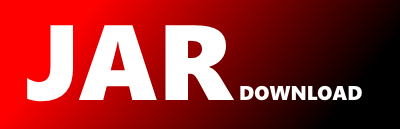
com.amazonaws.services.dynamodbv2.model.TableDescription Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.dynamodbv2.model;
import java.io.Serializable;
/**
*
* Represents the properties of a table.
*
*/
public class TableDescription implements Serializable {
/**
* An array of AttributeDefinition objects. Each of these objects
* describes one attribute in the table and index key schema. Each
* AttributeDefinition object in this array is composed of:
* -
AttributeName - The name of the attribute.
-
*
AttributeType - The data type for the attribute.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag attributeDefinitions;
/**
* The name of the table.
*
* Constraints:
* Length: 3 - 255
* Pattern: [a-zA-Z0-9_.-]+
*/
private String tableName;
/**
* The primary key structure for the table. Each KeySchemaElement
* consists of:
-
AttributeName - The name of the
* attribute.
-
KeyType - The key type for the
* attribute. Can be either HASH
or RANGE
.
*
For more information about primary keys, see Primary
* Key in the Amazon DynamoDB Developer Guide.
*
* Constraints:
* Length: 1 - 2
*/
private com.amazonaws.internal.ListWithAutoConstructFlag keySchema;
/**
* The current state of the table: -
CREATING - The
* table is being created, as the result of a CreateTable
* operation.
-
UPDATING - The table is being updated,
* as the result of an UpdateTable operation.
-
*
DELETING - The table is being deleted, as the result of a
* DeleteTable operation.
-
ACTIVE - The table
* is ready for use.
*
* Constraints:
* Allowed Values: CREATING, UPDATING, DELETING, ACTIVE
*/
private String tableStatus;
/**
* The date and time when the table was created, in UNIX epoch time format.
*/
private java.util.Date creationDateTime;
/**
* The provisioned throughput settings for the table, consisting of read
* and write capacity units, along with data about increases and
* decreases.
*/
private ProvisionedThroughputDescription provisionedThroughput;
/**
* The total size of the specified table, in bytes. DynamoDB updates this
* value approximately every six hours. Recent changes might not be
* reflected in this value.
*/
private Long tableSizeBytes;
/**
* The number of items in the specified table. DynamoDB updates this
* value approximately every six hours. Recent changes might not be
* reflected in this value.
*/
private Long itemCount;
/**
* Represents one or more local secondary indexes on the table. Each
* index is scoped to a given hash key value. Tables with one or more
* local secondary indexes are subject to an item collection size limit,
* where the amount of data within a given item collection cannot exceed
* 10 GB. Each element is composed of:
-
IndexName -
* The name of the local secondary index.
-
KeySchema
* - Specifies the complete index key schema. The attribute names in the
* key schema must be between 1 and 255 characters (inclusive). The key
* schema must begin with the same hash key attribute as the table.
* -
Projection - Specifies attributes that are copied
* (projected) from the table into the index. These are in addition to
* the primary key attributes and index key attributes, which are
* automatically projected. Each attribute specification is composed of:
*
-
ProjectionType - One of the following:
-
*
KEYS_ONLY
- Only the index and primary keys are
* projected into the index.
-
INCLUDE
- Only
* the specified table attributes are projected into the index. The list
* of projected attributes are in NonKeyAttributes.
-
*
ALL
- All of the table attributes are projected into
* the index.
-
NonKeyAttributes - A list
* of one or more non-key attribute names that are projected into the
* secondary index. The total count of attributes specified in
* NonKeyAttributes, summed across all of the secondary indexes,
* must not exceed 20. If you project the same attribute into two
* different indexes, this counts as two distinct attributes when
* determining the total.
-
IndexSizeBytes
* - Represents the total size of the index, in bytes. DynamoDB updates
* this value approximately every six hours. Recent changes might not be
* reflected in this value.
-
ItemCount - Represents
* the number of items in the index. DynamoDB updates this value
* approximately every six hours. Recent changes might not be reflected
* in this value.
If the table is in the
* DELETING
state, no information about indexes will be
* returned.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag localSecondaryIndexes;
/**
* The global secondary indexes, if any, on the table. Each index is
* scoped to a given hash key value. Each element is composed of:
* -
IndexName - The name of the global secondary index.
*
-
IndexSizeBytes - The total size of the global
* secondary index, in bytes. DynamoDB updates this value approximately
* every six hours. Recent changes might not be reflected in this value.
*
-
IndexStatus - The current status of the global
* secondary index:
-
CREATING - The index is being
* created.
-
UPDATING - The index is being updated.
*
-
DELETING - The index is being deleted.
-
*
ACTIVE - The index is ready for use.
-
*
ItemCount - The number of items in the global secondary
* index. DynamoDB updates this value approximately every six hours.
* Recent changes might not be reflected in this value.
-
*
KeySchema - Specifies the complete index key schema. The
* attribute names in the key schema must be between 1 and 255 characters
* (inclusive). The key schema must begin with the same hash key
* attribute as the table.
-
Projection - Specifies
* attributes that are copied (projected) from the table into the index.
* These are in addition to the primary key attributes and index key
* attributes, which are automatically projected. Each attribute
* specification is composed of:
-
ProjectionType - One
* of the following:
-
KEYS_ONLY
- Only the index
* and primary keys are projected into the index.
-
*
INCLUDE
- Only the specified table attributes are
* projected into the index. The list of projected attributes are in
* NonKeyAttributes.
-
ALL
- All of the
* table attributes are projected into the index.
-
*
NonKeyAttributes - A list of one or more non-key attribute
* names that are projected into the secondary index. The total count of
* attributes specified in NonKeyAttributes, summed across all of
* the secondary indexes, must not exceed 20. If you project the same
* attribute into two different indexes, this counts as two distinct
* attributes when determining the total.
-
*
ProvisionedThroughput - The provisioned throughput settings
* for the global secondary index, consisting of read and write capacity
* units, along with data about increases and decreases.
* If the table is in the DELETING
state, no information
* about indexes will be returned.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag globalSecondaryIndexes;
/**
* Default constructor for a new TableDescription object. Callers should use the
* setter or fluent setter (with...) methods to initialize this object after creating it.
*/
public TableDescription() {}
/**
* An array of AttributeDefinition objects. Each of these objects
* describes one attribute in the table and index key schema. Each
* AttributeDefinition object in this array is composed of:
* -
AttributeName - The name of the attribute.
-
*
AttributeType - The data type for the attribute.
*
* @return An array of AttributeDefinition objects. Each of these objects
* describes one attribute in the table and index key schema. Each
* AttributeDefinition object in this array is composed of:
* -
AttributeName - The name of the attribute.
-
*
AttributeType - The data type for the attribute.
*/
public java.util.List getAttributeDefinitions() {
return attributeDefinitions;
}
/**
* An array of AttributeDefinition objects. Each of these objects
* describes one attribute in the table and index key schema. Each
* AttributeDefinition object in this array is composed of:
* -
AttributeName - The name of the attribute.
-
*
AttributeType - The data type for the attribute.
*
* @param attributeDefinitions An array of AttributeDefinition objects. Each of these objects
* describes one attribute in the table and index key schema. Each
* AttributeDefinition object in this array is composed of:
* -
AttributeName - The name of the attribute.
-
*
AttributeType - The data type for the attribute.
*/
public void setAttributeDefinitions(java.util.Collection attributeDefinitions) {
if (attributeDefinitions == null) {
this.attributeDefinitions = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag attributeDefinitionsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(attributeDefinitions.size());
attributeDefinitionsCopy.addAll(attributeDefinitions);
this.attributeDefinitions = attributeDefinitionsCopy;
}
/**
* An array of AttributeDefinition objects. Each of these objects
* describes one attribute in the table and index key schema. Each
* AttributeDefinition object in this array is composed of:
* -
AttributeName - The name of the attribute.
-
*
AttributeType - The data type for the attribute.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param attributeDefinitions An array of AttributeDefinition objects. Each of these objects
* describes one attribute in the table and index key schema.
Each
* AttributeDefinition object in this array is composed of:
* -
AttributeName - The name of the attribute.
-
*
AttributeType - The data type for the attribute.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public TableDescription withAttributeDefinitions(AttributeDefinition... attributeDefinitions) {
if (getAttributeDefinitions() == null) setAttributeDefinitions(new java.util.ArrayList(attributeDefinitions.length));
for (AttributeDefinition value : attributeDefinitions) {
getAttributeDefinitions().add(value);
}
return this;
}
/**
* An array of AttributeDefinition objects. Each of these objects
* describes one attribute in the table and index key schema. Each
* AttributeDefinition object in this array is composed of:
* -
AttributeName - The name of the attribute.
-
*
AttributeType - The data type for the attribute.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param attributeDefinitions An array of AttributeDefinition objects. Each of these objects
* describes one attribute in the table and index key schema.
Each
* AttributeDefinition object in this array is composed of:
* -
AttributeName - The name of the attribute.
-
*
AttributeType - The data type for the attribute.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public TableDescription withAttributeDefinitions(java.util.Collection attributeDefinitions) {
if (attributeDefinitions == null) {
this.attributeDefinitions = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag attributeDefinitionsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(attributeDefinitions.size());
attributeDefinitionsCopy.addAll(attributeDefinitions);
this.attributeDefinitions = attributeDefinitionsCopy;
}
return this;
}
/**
* The name of the table.
*
* Constraints:
* Length: 3 - 255
* Pattern: [a-zA-Z0-9_.-]+
*
* @return The name of the table.
*/
public String getTableName() {
return tableName;
}
/**
* The name of the table.
*
* Constraints:
* Length: 3 - 255
* Pattern: [a-zA-Z0-9_.-]+
*
* @param tableName The name of the table.
*/
public void setTableName(String tableName) {
this.tableName = tableName;
}
/**
* The name of the table.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 3 - 255
* Pattern: [a-zA-Z0-9_.-]+
*
* @param tableName The name of the table.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public TableDescription withTableName(String tableName) {
this.tableName = tableName;
return this;
}
/**
* The primary key structure for the table. Each KeySchemaElement
* consists of:
-
AttributeName - The name of the
* attribute.
-
KeyType - The key type for the
* attribute. Can be either HASH
or RANGE
.
*
For more information about primary keys, see Primary
* Key in the Amazon DynamoDB Developer Guide.
*
* Constraints:
* Length: 1 - 2
*
* @return The primary key structure for the table. Each KeySchemaElement
* consists of:
-
AttributeName - The name of the
* attribute.
-
KeyType - The key type for the
* attribute. Can be either HASH
or RANGE
.
*
For more information about primary keys, see Primary
* Key in the Amazon DynamoDB Developer Guide.
*/
public java.util.List getKeySchema() {
return keySchema;
}
/**
* The primary key structure for the table. Each KeySchemaElement
* consists of: -
AttributeName - The name of the
* attribute.
-
KeyType - The key type for the
* attribute. Can be either HASH
or RANGE
.
*
For more information about primary keys, see Primary
* Key in the Amazon DynamoDB Developer Guide.
*
* Constraints:
* Length: 1 - 2
*
* @param keySchema The primary key structure for the table. Each KeySchemaElement
* consists of:
-
AttributeName - The name of the
* attribute.
-
KeyType - The key type for the
* attribute. Can be either HASH
or RANGE
.
*
For more information about primary keys, see Primary
* Key in the Amazon DynamoDB Developer Guide.
*/
public void setKeySchema(java.util.Collection keySchema) {
if (keySchema == null) {
this.keySchema = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag keySchemaCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(keySchema.size());
keySchemaCopy.addAll(keySchema);
this.keySchema = keySchemaCopy;
}
/**
* The primary key structure for the table. Each KeySchemaElement
* consists of: -
AttributeName - The name of the
* attribute.
-
KeyType - The key type for the
* attribute. Can be either HASH
or RANGE
.
*
For more information about primary keys, see Primary
* Key in the Amazon DynamoDB Developer Guide.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 2
*
* @param keySchema The primary key structure for the table. Each KeySchemaElement
* consists of:
-
AttributeName - The name of the
* attribute.
-
KeyType - The key type for the
* attribute. Can be either HASH
or RANGE
.
*
For more information about primary keys, see Primary
* Key in the Amazon DynamoDB Developer Guide.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public TableDescription withKeySchema(KeySchemaElement... keySchema) {
if (getKeySchema() == null) setKeySchema(new java.util.ArrayList(keySchema.length));
for (KeySchemaElement value : keySchema) {
getKeySchema().add(value);
}
return this;
}
/**
* The primary key structure for the table. Each KeySchemaElement
* consists of: -
AttributeName - The name of the
* attribute.
-
KeyType - The key type for the
* attribute. Can be either HASH
or RANGE
.
*
For more information about primary keys, see Primary
* Key in the Amazon DynamoDB Developer Guide.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 2
*
* @param keySchema The primary key structure for the table. Each KeySchemaElement
* consists of:
-
AttributeName - The name of the
* attribute.
-
KeyType - The key type for the
* attribute. Can be either HASH
or RANGE
.
*
For more information about primary keys, see Primary
* Key in the Amazon DynamoDB Developer Guide.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public TableDescription withKeySchema(java.util.Collection keySchema) {
if (keySchema == null) {
this.keySchema = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag keySchemaCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(keySchema.size());
keySchemaCopy.addAll(keySchema);
this.keySchema = keySchemaCopy;
}
return this;
}
/**
* The current state of the table: -
CREATING - The
* table is being created, as the result of a CreateTable
* operation.
-
UPDATING - The table is being updated,
* as the result of an UpdateTable operation.
-
*
DELETING - The table is being deleted, as the result of a
* DeleteTable operation.
-
ACTIVE - The table
* is ready for use.
*
* Constraints:
* Allowed Values: CREATING, UPDATING, DELETING, ACTIVE
*
* @return The current state of the table:
-
CREATING - The
* table is being created, as the result of a CreateTable
* operation.
-
UPDATING - The table is being updated,
* as the result of an UpdateTable operation.
-
*
DELETING - The table is being deleted, as the result of a
* DeleteTable operation.
-
ACTIVE - The table
* is ready for use.
*
* @see TableStatus
*/
public String getTableStatus() {
return tableStatus;
}
/**
* The current state of the table: -
CREATING - The
* table is being created, as the result of a CreateTable
* operation.
-
UPDATING - The table is being updated,
* as the result of an UpdateTable operation.
-
*
DELETING - The table is being deleted, as the result of a
* DeleteTable operation.
-
ACTIVE - The table
* is ready for use.
*
* Constraints:
* Allowed Values: CREATING, UPDATING, DELETING, ACTIVE
*
* @param tableStatus The current state of the table:
-
CREATING - The
* table is being created, as the result of a CreateTable
* operation.
-
UPDATING - The table is being updated,
* as the result of an UpdateTable operation.
-
*
DELETING - The table is being deleted, as the result of a
* DeleteTable operation.
-
ACTIVE - The table
* is ready for use.
*
* @see TableStatus
*/
public void setTableStatus(String tableStatus) {
this.tableStatus = tableStatus;
}
/**
* The current state of the table: -
CREATING - The
* table is being created, as the result of a CreateTable
* operation.
-
UPDATING - The table is being updated,
* as the result of an UpdateTable operation.
-
*
DELETING - The table is being deleted, as the result of a
* DeleteTable operation.
-
ACTIVE - The table
* is ready for use.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: CREATING, UPDATING, DELETING, ACTIVE
*
* @param tableStatus The current state of the table:
-
CREATING - The
* table is being created, as the result of a CreateTable
* operation.
-
UPDATING - The table is being updated,
* as the result of an UpdateTable operation.
-
*
DELETING - The table is being deleted, as the result of a
* DeleteTable operation.
-
ACTIVE - The table
* is ready for use.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see TableStatus
*/
public TableDescription withTableStatus(String tableStatus) {
this.tableStatus = tableStatus;
return this;
}
/**
* The current state of the table: -
CREATING - The
* table is being created, as the result of a CreateTable
* operation.
-
UPDATING - The table is being updated,
* as the result of an UpdateTable operation.
-
*
DELETING - The table is being deleted, as the result of a
* DeleteTable operation.
-
ACTIVE - The table
* is ready for use.
*
* Constraints:
* Allowed Values: CREATING, UPDATING, DELETING, ACTIVE
*
* @param tableStatus The current state of the table:
-
CREATING - The
* table is being created, as the result of a CreateTable
* operation.
-
UPDATING - The table is being updated,
* as the result of an UpdateTable operation.
-
*
DELETING - The table is being deleted, as the result of a
* DeleteTable operation.
-
ACTIVE - The table
* is ready for use.
*
* @see TableStatus
*/
public void setTableStatus(TableStatus tableStatus) {
this.tableStatus = tableStatus.toString();
}
/**
* The current state of the table: -
CREATING - The
* table is being created, as the result of a CreateTable
* operation.
-
UPDATING - The table is being updated,
* as the result of an UpdateTable operation.
-
*
DELETING - The table is being deleted, as the result of a
* DeleteTable operation.
-
ACTIVE - The table
* is ready for use.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: CREATING, UPDATING, DELETING, ACTIVE
*
* @param tableStatus The current state of the table:
-
CREATING - The
* table is being created, as the result of a CreateTable
* operation.
-
UPDATING - The table is being updated,
* as the result of an UpdateTable operation.
-
*
DELETING - The table is being deleted, as the result of a
* DeleteTable operation.
-
ACTIVE - The table
* is ready for use.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see TableStatus
*/
public TableDescription withTableStatus(TableStatus tableStatus) {
this.tableStatus = tableStatus.toString();
return this;
}
/**
* The date and time when the table was created, in UNIX epoch time format.
*
* @return The date and time when the table was created, in UNIX epoch time format.
*/
public java.util.Date getCreationDateTime() {
return creationDateTime;
}
/**
* The date and time when the table was created, in UNIX epoch time format.
*
* @param creationDateTime The date and time when the table was created, in UNIX epoch time format.
*/
public void setCreationDateTime(java.util.Date creationDateTime) {
this.creationDateTime = creationDateTime;
}
/**
* The date and time when the table was created, in UNIX epoch time format.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param creationDateTime The date and time when the table was created, in UNIX epoch time format.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public TableDescription withCreationDateTime(java.util.Date creationDateTime) {
this.creationDateTime = creationDateTime;
return this;
}
/**
* The provisioned throughput settings for the table, consisting of read
* and write capacity units, along with data about increases and
* decreases.
*
* @return The provisioned throughput settings for the table, consisting of read
* and write capacity units, along with data about increases and
* decreases.
*/
public ProvisionedThroughputDescription getProvisionedThroughput() {
return provisionedThroughput;
}
/**
* The provisioned throughput settings for the table, consisting of read
* and write capacity units, along with data about increases and
* decreases.
*
* @param provisionedThroughput The provisioned throughput settings for the table, consisting of read
* and write capacity units, along with data about increases and
* decreases.
*/
public void setProvisionedThroughput(ProvisionedThroughputDescription provisionedThroughput) {
this.provisionedThroughput = provisionedThroughput;
}
/**
* The provisioned throughput settings for the table, consisting of read
* and write capacity units, along with data about increases and
* decreases.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param provisionedThroughput The provisioned throughput settings for the table, consisting of read
* and write capacity units, along with data about increases and
* decreases.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public TableDescription withProvisionedThroughput(ProvisionedThroughputDescription provisionedThroughput) {
this.provisionedThroughput = provisionedThroughput;
return this;
}
/**
* The total size of the specified table, in bytes. DynamoDB updates this
* value approximately every six hours. Recent changes might not be
* reflected in this value.
*
* @return The total size of the specified table, in bytes. DynamoDB updates this
* value approximately every six hours. Recent changes might not be
* reflected in this value.
*/
public Long getTableSizeBytes() {
return tableSizeBytes;
}
/**
* The total size of the specified table, in bytes. DynamoDB updates this
* value approximately every six hours. Recent changes might not be
* reflected in this value.
*
* @param tableSizeBytes The total size of the specified table, in bytes. DynamoDB updates this
* value approximately every six hours. Recent changes might not be
* reflected in this value.
*/
public void setTableSizeBytes(Long tableSizeBytes) {
this.tableSizeBytes = tableSizeBytes;
}
/**
* The total size of the specified table, in bytes. DynamoDB updates this
* value approximately every six hours. Recent changes might not be
* reflected in this value.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param tableSizeBytes The total size of the specified table, in bytes. DynamoDB updates this
* value approximately every six hours. Recent changes might not be
* reflected in this value.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public TableDescription withTableSizeBytes(Long tableSizeBytes) {
this.tableSizeBytes = tableSizeBytes;
return this;
}
/**
* The number of items in the specified table. DynamoDB updates this
* value approximately every six hours. Recent changes might not be
* reflected in this value.
*
* @return The number of items in the specified table. DynamoDB updates this
* value approximately every six hours. Recent changes might not be
* reflected in this value.
*/
public Long getItemCount() {
return itemCount;
}
/**
* The number of items in the specified table. DynamoDB updates this
* value approximately every six hours. Recent changes might not be
* reflected in this value.
*
* @param itemCount The number of items in the specified table. DynamoDB updates this
* value approximately every six hours. Recent changes might not be
* reflected in this value.
*/
public void setItemCount(Long itemCount) {
this.itemCount = itemCount;
}
/**
* The number of items in the specified table. DynamoDB updates this
* value approximately every six hours. Recent changes might not be
* reflected in this value.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param itemCount The number of items in the specified table. DynamoDB updates this
* value approximately every six hours. Recent changes might not be
* reflected in this value.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public TableDescription withItemCount(Long itemCount) {
this.itemCount = itemCount;
return this;
}
/**
* Represents one or more local secondary indexes on the table. Each
* index is scoped to a given hash key value. Tables with one or more
* local secondary indexes are subject to an item collection size limit,
* where the amount of data within a given item collection cannot exceed
* 10 GB. Each element is composed of:
-
IndexName -
* The name of the local secondary index.
-
KeySchema
* - Specifies the complete index key schema. The attribute names in the
* key schema must be between 1 and 255 characters (inclusive). The key
* schema must begin with the same hash key attribute as the table.
* -
Projection - Specifies attributes that are copied
* (projected) from the table into the index. These are in addition to
* the primary key attributes and index key attributes, which are
* automatically projected. Each attribute specification is composed of:
*
-
ProjectionType - One of the following:
-
*
KEYS_ONLY
- Only the index and primary keys are
* projected into the index.
-
INCLUDE
- Only
* the specified table attributes are projected into the index. The list
* of projected attributes are in NonKeyAttributes.
-
*
ALL
- All of the table attributes are projected into
* the index.
-
NonKeyAttributes - A list
* of one or more non-key attribute names that are projected into the
* secondary index. The total count of attributes specified in
* NonKeyAttributes, summed across all of the secondary indexes,
* must not exceed 20. If you project the same attribute into two
* different indexes, this counts as two distinct attributes when
* determining the total.
-
IndexSizeBytes
* - Represents the total size of the index, in bytes. DynamoDB updates
* this value approximately every six hours. Recent changes might not be
* reflected in this value.
-
ItemCount - Represents
* the number of items in the index. DynamoDB updates this value
* approximately every six hours. Recent changes might not be reflected
* in this value.
If the table is in the
* DELETING
state, no information about indexes will be
* returned.
*
* @return Represents one or more local secondary indexes on the table. Each
* index is scoped to a given hash key value. Tables with one or more
* local secondary indexes are subject to an item collection size limit,
* where the amount of data within a given item collection cannot exceed
* 10 GB. Each element is composed of:
-
IndexName -
* The name of the local secondary index.
-
KeySchema
* - Specifies the complete index key schema. The attribute names in the
* key schema must be between 1 and 255 characters (inclusive). The key
* schema must begin with the same hash key attribute as the table.
* -
Projection - Specifies attributes that are copied
* (projected) from the table into the index. These are in addition to
* the primary key attributes and index key attributes, which are
* automatically projected. Each attribute specification is composed of:
*
-
ProjectionType - One of the following:
-
*
KEYS_ONLY
- Only the index and primary keys are
* projected into the index.
-
INCLUDE
- Only
* the specified table attributes are projected into the index. The list
* of projected attributes are in NonKeyAttributes.
-
*
ALL
- All of the table attributes are projected into
* the index.
-
NonKeyAttributes - A list
* of one or more non-key attribute names that are projected into the
* secondary index. The total count of attributes specified in
* NonKeyAttributes, summed across all of the secondary indexes,
* must not exceed 20. If you project the same attribute into two
* different indexes, this counts as two distinct attributes when
* determining the total.
-
IndexSizeBytes
* - Represents the total size of the index, in bytes. DynamoDB updates
* this value approximately every six hours. Recent changes might not be
* reflected in this value.
-
ItemCount - Represents
* the number of items in the index. DynamoDB updates this value
* approximately every six hours. Recent changes might not be reflected
* in this value.
If the table is in the
* DELETING
state, no information about indexes will be
* returned.
*/
public java.util.List getLocalSecondaryIndexes() {
return localSecondaryIndexes;
}
/**
* Represents one or more local secondary indexes on the table. Each
* index is scoped to a given hash key value. Tables with one or more
* local secondary indexes are subject to an item collection size limit,
* where the amount of data within a given item collection cannot exceed
* 10 GB. Each element is composed of: -
IndexName -
* The name of the local secondary index.
-
KeySchema
* - Specifies the complete index key schema. The attribute names in the
* key schema must be between 1 and 255 characters (inclusive). The key
* schema must begin with the same hash key attribute as the table.
* -
Projection - Specifies attributes that are copied
* (projected) from the table into the index. These are in addition to
* the primary key attributes and index key attributes, which are
* automatically projected. Each attribute specification is composed of:
*
-
ProjectionType - One of the following:
-
*
KEYS_ONLY
- Only the index and primary keys are
* projected into the index.
-
INCLUDE
- Only
* the specified table attributes are projected into the index. The list
* of projected attributes are in NonKeyAttributes.
-
*
ALL
- All of the table attributes are projected into
* the index.
-
NonKeyAttributes - A list
* of one or more non-key attribute names that are projected into the
* secondary index. The total count of attributes specified in
* NonKeyAttributes, summed across all of the secondary indexes,
* must not exceed 20. If you project the same attribute into two
* different indexes, this counts as two distinct attributes when
* determining the total.
-
IndexSizeBytes
* - Represents the total size of the index, in bytes. DynamoDB updates
* this value approximately every six hours. Recent changes might not be
* reflected in this value.
-
ItemCount - Represents
* the number of items in the index. DynamoDB updates this value
* approximately every six hours. Recent changes might not be reflected
* in this value.
If the table is in the
* DELETING
state, no information about indexes will be
* returned.
*
* @param localSecondaryIndexes Represents one or more local secondary indexes on the table. Each
* index is scoped to a given hash key value. Tables with one or more
* local secondary indexes are subject to an item collection size limit,
* where the amount of data within a given item collection cannot exceed
* 10 GB. Each element is composed of:
-
IndexName -
* The name of the local secondary index.
-
KeySchema
* - Specifies the complete index key schema. The attribute names in the
* key schema must be between 1 and 255 characters (inclusive). The key
* schema must begin with the same hash key attribute as the table.
* -
Projection - Specifies attributes that are copied
* (projected) from the table into the index. These are in addition to
* the primary key attributes and index key attributes, which are
* automatically projected. Each attribute specification is composed of:
*
-
ProjectionType - One of the following:
-
*
KEYS_ONLY
- Only the index and primary keys are
* projected into the index.
-
INCLUDE
- Only
* the specified table attributes are projected into the index. The list
* of projected attributes are in NonKeyAttributes.
-
*
ALL
- All of the table attributes are projected into
* the index.
-
NonKeyAttributes - A list
* of one or more non-key attribute names that are projected into the
* secondary index. The total count of attributes specified in
* NonKeyAttributes, summed across all of the secondary indexes,
* must not exceed 20. If you project the same attribute into two
* different indexes, this counts as two distinct attributes when
* determining the total.
-
IndexSizeBytes
* - Represents the total size of the index, in bytes. DynamoDB updates
* this value approximately every six hours. Recent changes might not be
* reflected in this value.
-
ItemCount - Represents
* the number of items in the index. DynamoDB updates this value
* approximately every six hours. Recent changes might not be reflected
* in this value.
If the table is in the
* DELETING
state, no information about indexes will be
* returned.
*/
public void setLocalSecondaryIndexes(java.util.Collection localSecondaryIndexes) {
if (localSecondaryIndexes == null) {
this.localSecondaryIndexes = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag localSecondaryIndexesCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(localSecondaryIndexes.size());
localSecondaryIndexesCopy.addAll(localSecondaryIndexes);
this.localSecondaryIndexes = localSecondaryIndexesCopy;
}
/**
* Represents one or more local secondary indexes on the table. Each
* index is scoped to a given hash key value. Tables with one or more
* local secondary indexes are subject to an item collection size limit,
* where the amount of data within a given item collection cannot exceed
* 10 GB. Each element is composed of: -
IndexName -
* The name of the local secondary index.
-
KeySchema
* - Specifies the complete index key schema. The attribute names in the
* key schema must be between 1 and 255 characters (inclusive). The key
* schema must begin with the same hash key attribute as the table.
* -
Projection - Specifies attributes that are copied
* (projected) from the table into the index. These are in addition to
* the primary key attributes and index key attributes, which are
* automatically projected. Each attribute specification is composed of:
*
-
ProjectionType - One of the following:
-
*
KEYS_ONLY
- Only the index and primary keys are
* projected into the index.
-
INCLUDE
- Only
* the specified table attributes are projected into the index. The list
* of projected attributes are in NonKeyAttributes.
-
*
ALL
- All of the table attributes are projected into
* the index.
-
NonKeyAttributes - A list
* of one or more non-key attribute names that are projected into the
* secondary index. The total count of attributes specified in
* NonKeyAttributes, summed across all of the secondary indexes,
* must not exceed 20. If you project the same attribute into two
* different indexes, this counts as two distinct attributes when
* determining the total.
-
IndexSizeBytes
* - Represents the total size of the index, in bytes. DynamoDB updates
* this value approximately every six hours. Recent changes might not be
* reflected in this value.
-
ItemCount - Represents
* the number of items in the index. DynamoDB updates this value
* approximately every six hours. Recent changes might not be reflected
* in this value.
If the table is in the
* DELETING
state, no information about indexes will be
* returned.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param localSecondaryIndexes Represents one or more local secondary indexes on the table. Each
* index is scoped to a given hash key value. Tables with one or more
* local secondary indexes are subject to an item collection size limit,
* where the amount of data within a given item collection cannot exceed
* 10 GB. Each element is composed of:
-
IndexName -
* The name of the local secondary index.
-
KeySchema
* - Specifies the complete index key schema. The attribute names in the
* key schema must be between 1 and 255 characters (inclusive). The key
* schema must begin with the same hash key attribute as the table.
* -
Projection - Specifies attributes that are copied
* (projected) from the table into the index. These are in addition to
* the primary key attributes and index key attributes, which are
* automatically projected. Each attribute specification is composed of:
*
-
ProjectionType - One of the following:
-
*
KEYS_ONLY
- Only the index and primary keys are
* projected into the index.
-
INCLUDE
- Only
* the specified table attributes are projected into the index. The list
* of projected attributes are in NonKeyAttributes.
-
*
ALL
- All of the table attributes are projected into
* the index.
-
NonKeyAttributes - A list
* of one or more non-key attribute names that are projected into the
* secondary index. The total count of attributes specified in
* NonKeyAttributes, summed across all of the secondary indexes,
* must not exceed 20. If you project the same attribute into two
* different indexes, this counts as two distinct attributes when
* determining the total.
-
IndexSizeBytes
* - Represents the total size of the index, in bytes. DynamoDB updates
* this value approximately every six hours. Recent changes might not be
* reflected in this value.
-
ItemCount - Represents
* the number of items in the index. DynamoDB updates this value
* approximately every six hours. Recent changes might not be reflected
* in this value.
If the table is in the
* DELETING
state, no information about indexes will be
* returned.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public TableDescription withLocalSecondaryIndexes(LocalSecondaryIndexDescription... localSecondaryIndexes) {
if (getLocalSecondaryIndexes() == null) setLocalSecondaryIndexes(new java.util.ArrayList(localSecondaryIndexes.length));
for (LocalSecondaryIndexDescription value : localSecondaryIndexes) {
getLocalSecondaryIndexes().add(value);
}
return this;
}
/**
* Represents one or more local secondary indexes on the table. Each
* index is scoped to a given hash key value. Tables with one or more
* local secondary indexes are subject to an item collection size limit,
* where the amount of data within a given item collection cannot exceed
* 10 GB. Each element is composed of: -
IndexName -
* The name of the local secondary index.
-
KeySchema
* - Specifies the complete index key schema. The attribute names in the
* key schema must be between 1 and 255 characters (inclusive). The key
* schema must begin with the same hash key attribute as the table.
* -
Projection - Specifies attributes that are copied
* (projected) from the table into the index. These are in addition to
* the primary key attributes and index key attributes, which are
* automatically projected. Each attribute specification is composed of:
*
-
ProjectionType - One of the following:
-
*
KEYS_ONLY
- Only the index and primary keys are
* projected into the index.
-
INCLUDE
- Only
* the specified table attributes are projected into the index. The list
* of projected attributes are in NonKeyAttributes.
-
*
ALL
- All of the table attributes are projected into
* the index.
-
NonKeyAttributes - A list
* of one or more non-key attribute names that are projected into the
* secondary index. The total count of attributes specified in
* NonKeyAttributes, summed across all of the secondary indexes,
* must not exceed 20. If you project the same attribute into two
* different indexes, this counts as two distinct attributes when
* determining the total.
-
IndexSizeBytes
* - Represents the total size of the index, in bytes. DynamoDB updates
* this value approximately every six hours. Recent changes might not be
* reflected in this value.
-
ItemCount - Represents
* the number of items in the index. DynamoDB updates this value
* approximately every six hours. Recent changes might not be reflected
* in this value.
If the table is in the
* DELETING
state, no information about indexes will be
* returned.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param localSecondaryIndexes Represents one or more local secondary indexes on the table. Each
* index is scoped to a given hash key value. Tables with one or more
* local secondary indexes are subject to an item collection size limit,
* where the amount of data within a given item collection cannot exceed
* 10 GB. Each element is composed of:
-
IndexName -
* The name of the local secondary index.
-
KeySchema
* - Specifies the complete index key schema. The attribute names in the
* key schema must be between 1 and 255 characters (inclusive). The key
* schema must begin with the same hash key attribute as the table.
* -
Projection - Specifies attributes that are copied
* (projected) from the table into the index. These are in addition to
* the primary key attributes and index key attributes, which are
* automatically projected. Each attribute specification is composed of:
*
-
ProjectionType - One of the following:
-
*
KEYS_ONLY
- Only the index and primary keys are
* projected into the index.
-
INCLUDE
- Only
* the specified table attributes are projected into the index. The list
* of projected attributes are in NonKeyAttributes.
-
*
ALL
- All of the table attributes are projected into
* the index.
-
NonKeyAttributes - A list
* of one or more non-key attribute names that are projected into the
* secondary index. The total count of attributes specified in
* NonKeyAttributes, summed across all of the secondary indexes,
* must not exceed 20. If you project the same attribute into two
* different indexes, this counts as two distinct attributes when
* determining the total.
-
IndexSizeBytes
* - Represents the total size of the index, in bytes. DynamoDB updates
* this value approximately every six hours. Recent changes might not be
* reflected in this value.
-
ItemCount - Represents
* the number of items in the index. DynamoDB updates this value
* approximately every six hours. Recent changes might not be reflected
* in this value.
If the table is in the
* DELETING
state, no information about indexes will be
* returned.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public TableDescription withLocalSecondaryIndexes(java.util.Collection localSecondaryIndexes) {
if (localSecondaryIndexes == null) {
this.localSecondaryIndexes = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag localSecondaryIndexesCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(localSecondaryIndexes.size());
localSecondaryIndexesCopy.addAll(localSecondaryIndexes);
this.localSecondaryIndexes = localSecondaryIndexesCopy;
}
return this;
}
/**
* The global secondary indexes, if any, on the table. Each index is
* scoped to a given hash key value. Each element is composed of:
* -
IndexName - The name of the global secondary index.
*
-
IndexSizeBytes - The total size of the global
* secondary index, in bytes. DynamoDB updates this value approximately
* every six hours. Recent changes might not be reflected in this value.
*
-
IndexStatus - The current status of the global
* secondary index:
-
CREATING - The index is being
* created.
-
UPDATING - The index is being updated.
*
-
DELETING - The index is being deleted.
-
*
ACTIVE - The index is ready for use.
-
*
ItemCount - The number of items in the global secondary
* index. DynamoDB updates this value approximately every six hours.
* Recent changes might not be reflected in this value.
-
*
KeySchema - Specifies the complete index key schema. The
* attribute names in the key schema must be between 1 and 255 characters
* (inclusive). The key schema must begin with the same hash key
* attribute as the table.
-
Projection - Specifies
* attributes that are copied (projected) from the table into the index.
* These are in addition to the primary key attributes and index key
* attributes, which are automatically projected. Each attribute
* specification is composed of:
-
ProjectionType - One
* of the following:
-
KEYS_ONLY
- Only the index
* and primary keys are projected into the index.
-
*
INCLUDE
- Only the specified table attributes are
* projected into the index. The list of projected attributes are in
* NonKeyAttributes.
-
ALL
- All of the
* table attributes are projected into the index.
-
*
NonKeyAttributes - A list of one or more non-key attribute
* names that are projected into the secondary index. The total count of
* attributes specified in NonKeyAttributes, summed across all of
* the secondary indexes, must not exceed 20. If you project the same
* attribute into two different indexes, this counts as two distinct
* attributes when determining the total.
-
*
ProvisionedThroughput - The provisioned throughput settings
* for the global secondary index, consisting of read and write capacity
* units, along with data about increases and decreases.
* If the table is in the DELETING
state, no information
* about indexes will be returned.
*
* @return The global secondary indexes, if any, on the table. Each index is
* scoped to a given hash key value. Each element is composed of:
* -
IndexName - The name of the global secondary index.
*
-
IndexSizeBytes - The total size of the global
* secondary index, in bytes. DynamoDB updates this value approximately
* every six hours. Recent changes might not be reflected in this value.
*
-
IndexStatus - The current status of the global
* secondary index:
-
CREATING - The index is being
* created.
-
UPDATING - The index is being updated.
*
-
DELETING - The index is being deleted.
-
*
ACTIVE - The index is ready for use.
-
*
ItemCount - The number of items in the global secondary
* index. DynamoDB updates this value approximately every six hours.
* Recent changes might not be reflected in this value.
-
*
KeySchema - Specifies the complete index key schema. The
* attribute names in the key schema must be between 1 and 255 characters
* (inclusive). The key schema must begin with the same hash key
* attribute as the table.
-
Projection - Specifies
* attributes that are copied (projected) from the table into the index.
* These are in addition to the primary key attributes and index key
* attributes, which are automatically projected. Each attribute
* specification is composed of:
-
ProjectionType - One
* of the following:
-
KEYS_ONLY
- Only the index
* and primary keys are projected into the index.
-
*
INCLUDE
- Only the specified table attributes are
* projected into the index. The list of projected attributes are in
* NonKeyAttributes.
-
ALL
- All of the
* table attributes are projected into the index.
-
*
NonKeyAttributes - A list of one or more non-key attribute
* names that are projected into the secondary index. The total count of
* attributes specified in NonKeyAttributes, summed across all of
* the secondary indexes, must not exceed 20. If you project the same
* attribute into two different indexes, this counts as two distinct
* attributes when determining the total.
-
*
ProvisionedThroughput - The provisioned throughput settings
* for the global secondary index, consisting of read and write capacity
* units, along with data about increases and decreases.
* If the table is in the DELETING
state, no information
* about indexes will be returned.
*/
public java.util.List getGlobalSecondaryIndexes() {
return globalSecondaryIndexes;
}
/**
* The global secondary indexes, if any, on the table. Each index is
* scoped to a given hash key value. Each element is composed of:
* -
IndexName - The name of the global secondary index.
*
-
IndexSizeBytes - The total size of the global
* secondary index, in bytes. DynamoDB updates this value approximately
* every six hours. Recent changes might not be reflected in this value.
*
-
IndexStatus - The current status of the global
* secondary index:
-
CREATING - The index is being
* created.
-
UPDATING - The index is being updated.
*
-
DELETING - The index is being deleted.
-
*
ACTIVE - The index is ready for use.
-
*
ItemCount - The number of items in the global secondary
* index. DynamoDB updates this value approximately every six hours.
* Recent changes might not be reflected in this value.
-
*
KeySchema - Specifies the complete index key schema. The
* attribute names in the key schema must be between 1 and 255 characters
* (inclusive). The key schema must begin with the same hash key
* attribute as the table.
-
Projection - Specifies
* attributes that are copied (projected) from the table into the index.
* These are in addition to the primary key attributes and index key
* attributes, which are automatically projected. Each attribute
* specification is composed of:
-
ProjectionType - One
* of the following:
-
KEYS_ONLY
- Only the index
* and primary keys are projected into the index.
-
*
INCLUDE
- Only the specified table attributes are
* projected into the index. The list of projected attributes are in
* NonKeyAttributes.
-
ALL
- All of the
* table attributes are projected into the index.
-
*
NonKeyAttributes - A list of one or more non-key attribute
* names that are projected into the secondary index. The total count of
* attributes specified in NonKeyAttributes, summed across all of
* the secondary indexes, must not exceed 20. If you project the same
* attribute into two different indexes, this counts as two distinct
* attributes when determining the total.
-
*
ProvisionedThroughput - The provisioned throughput settings
* for the global secondary index, consisting of read and write capacity
* units, along with data about increases and decreases.
* If the table is in the DELETING
state, no information
* about indexes will be returned.
*
* @param globalSecondaryIndexes The global secondary indexes, if any, on the table. Each index is
* scoped to a given hash key value. Each element is composed of:
* -
IndexName - The name of the global secondary index.
*
-
IndexSizeBytes - The total size of the global
* secondary index, in bytes. DynamoDB updates this value approximately
* every six hours. Recent changes might not be reflected in this value.
*
-
IndexStatus - The current status of the global
* secondary index:
-
CREATING - The index is being
* created.
-
UPDATING - The index is being updated.
*
-
DELETING - The index is being deleted.
-
*
ACTIVE - The index is ready for use.
-
*
ItemCount - The number of items in the global secondary
* index. DynamoDB updates this value approximately every six hours.
* Recent changes might not be reflected in this value.
-
*
KeySchema - Specifies the complete index key schema. The
* attribute names in the key schema must be between 1 and 255 characters
* (inclusive). The key schema must begin with the same hash key
* attribute as the table.
-
Projection - Specifies
* attributes that are copied (projected) from the table into the index.
* These are in addition to the primary key attributes and index key
* attributes, which are automatically projected. Each attribute
* specification is composed of:
-
ProjectionType - One
* of the following:
-
KEYS_ONLY
- Only the index
* and primary keys are projected into the index.
-
*
INCLUDE
- Only the specified table attributes are
* projected into the index. The list of projected attributes are in
* NonKeyAttributes.
-
ALL
- All of the
* table attributes are projected into the index.
-
*
NonKeyAttributes - A list of one or more non-key attribute
* names that are projected into the secondary index. The total count of
* attributes specified in NonKeyAttributes, summed across all of
* the secondary indexes, must not exceed 20. If you project the same
* attribute into two different indexes, this counts as two distinct
* attributes when determining the total.
-
*
ProvisionedThroughput - The provisioned throughput settings
* for the global secondary index, consisting of read and write capacity
* units, along with data about increases and decreases.
* If the table is in the DELETING
state, no information
* about indexes will be returned.
*/
public void setGlobalSecondaryIndexes(java.util.Collection globalSecondaryIndexes) {
if (globalSecondaryIndexes == null) {
this.globalSecondaryIndexes = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag globalSecondaryIndexesCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(globalSecondaryIndexes.size());
globalSecondaryIndexesCopy.addAll(globalSecondaryIndexes);
this.globalSecondaryIndexes = globalSecondaryIndexesCopy;
}
/**
* The global secondary indexes, if any, on the table. Each index is
* scoped to a given hash key value. Each element is composed of:
* -
IndexName - The name of the global secondary index.
*
-
IndexSizeBytes - The total size of the global
* secondary index, in bytes. DynamoDB updates this value approximately
* every six hours. Recent changes might not be reflected in this value.
*
-
IndexStatus - The current status of the global
* secondary index:
-
CREATING - The index is being
* created.
-
UPDATING - The index is being updated.
*
-
DELETING - The index is being deleted.
-
*
ACTIVE - The index is ready for use.
-
*
ItemCount - The number of items in the global secondary
* index. DynamoDB updates this value approximately every six hours.
* Recent changes might not be reflected in this value.
-
*
KeySchema - Specifies the complete index key schema. The
* attribute names in the key schema must be between 1 and 255 characters
* (inclusive). The key schema must begin with the same hash key
* attribute as the table.
-
Projection - Specifies
* attributes that are copied (projected) from the table into the index.
* These are in addition to the primary key attributes and index key
* attributes, which are automatically projected. Each attribute
* specification is composed of:
-
ProjectionType - One
* of the following:
-
KEYS_ONLY
- Only the index
* and primary keys are projected into the index.
-
*
INCLUDE
- Only the specified table attributes are
* projected into the index. The list of projected attributes are in
* NonKeyAttributes.
-
ALL
- All of the
* table attributes are projected into the index.
-
*
NonKeyAttributes - A list of one or more non-key attribute
* names that are projected into the secondary index. The total count of
* attributes specified in NonKeyAttributes, summed across all of
* the secondary indexes, must not exceed 20. If you project the same
* attribute into two different indexes, this counts as two distinct
* attributes when determining the total.
-
*
ProvisionedThroughput - The provisioned throughput settings
* for the global secondary index, consisting of read and write capacity
* units, along with data about increases and decreases.
* If the table is in the DELETING
state, no information
* about indexes will be returned.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param globalSecondaryIndexes The global secondary indexes, if any, on the table. Each index is
* scoped to a given hash key value. Each element is composed of:
* -
IndexName - The name of the global secondary index.
*
-
IndexSizeBytes - The total size of the global
* secondary index, in bytes. DynamoDB updates this value approximately
* every six hours. Recent changes might not be reflected in this value.
*
-
IndexStatus - The current status of the global
* secondary index:
-
CREATING - The index is being
* created.
-
UPDATING - The index is being updated.
*
-
DELETING - The index is being deleted.
-
*
ACTIVE - The index is ready for use.
-
*
ItemCount - The number of items in the global secondary
* index. DynamoDB updates this value approximately every six hours.
* Recent changes might not be reflected in this value.
-
*
KeySchema - Specifies the complete index key schema. The
* attribute names in the key schema must be between 1 and 255 characters
* (inclusive). The key schema must begin with the same hash key
* attribute as the table.
-
Projection - Specifies
* attributes that are copied (projected) from the table into the index.
* These are in addition to the primary key attributes and index key
* attributes, which are automatically projected. Each attribute
* specification is composed of:
-
ProjectionType - One
* of the following:
-
KEYS_ONLY
- Only the index
* and primary keys are projected into the index.
-
*
INCLUDE
- Only the specified table attributes are
* projected into the index. The list of projected attributes are in
* NonKeyAttributes.
-
ALL
- All of the
* table attributes are projected into the index.
-
*
NonKeyAttributes - A list of one or more non-key attribute
* names that are projected into the secondary index. The total count of
* attributes specified in NonKeyAttributes, summed across all of
* the secondary indexes, must not exceed 20. If you project the same
* attribute into two different indexes, this counts as two distinct
* attributes when determining the total.
-
*
ProvisionedThroughput - The provisioned throughput settings
* for the global secondary index, consisting of read and write capacity
* units, along with data about increases and decreases.
* If the table is in the DELETING
state, no information
* about indexes will be returned.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public TableDescription withGlobalSecondaryIndexes(GlobalSecondaryIndexDescription... globalSecondaryIndexes) {
if (getGlobalSecondaryIndexes() == null) setGlobalSecondaryIndexes(new java.util.ArrayList(globalSecondaryIndexes.length));
for (GlobalSecondaryIndexDescription value : globalSecondaryIndexes) {
getGlobalSecondaryIndexes().add(value);
}
return this;
}
/**
* The global secondary indexes, if any, on the table. Each index is
* scoped to a given hash key value. Each element is composed of:
* -
IndexName - The name of the global secondary index.
*
-
IndexSizeBytes - The total size of the global
* secondary index, in bytes. DynamoDB updates this value approximately
* every six hours. Recent changes might not be reflected in this value.
*
-
IndexStatus - The current status of the global
* secondary index:
-
CREATING - The index is being
* created.
-
UPDATING - The index is being updated.
*
-
DELETING - The index is being deleted.
-
*
ACTIVE - The index is ready for use.
-
*
ItemCount - The number of items in the global secondary
* index. DynamoDB updates this value approximately every six hours.
* Recent changes might not be reflected in this value.
-
*
KeySchema - Specifies the complete index key schema. The
* attribute names in the key schema must be between 1 and 255 characters
* (inclusive). The key schema must begin with the same hash key
* attribute as the table.
-
Projection - Specifies
* attributes that are copied (projected) from the table into the index.
* These are in addition to the primary key attributes and index key
* attributes, which are automatically projected. Each attribute
* specification is composed of:
-
ProjectionType - One
* of the following:
-
KEYS_ONLY
- Only the index
* and primary keys are projected into the index.
-
*
INCLUDE
- Only the specified table attributes are
* projected into the index. The list of projected attributes are in
* NonKeyAttributes.
-
ALL
- All of the
* table attributes are projected into the index.
-
*
NonKeyAttributes - A list of one or more non-key attribute
* names that are projected into the secondary index. The total count of
* attributes specified in NonKeyAttributes, summed across all of
* the secondary indexes, must not exceed 20. If you project the same
* attribute into two different indexes, this counts as two distinct
* attributes when determining the total.
-
*
ProvisionedThroughput - The provisioned throughput settings
* for the global secondary index, consisting of read and write capacity
* units, along with data about increases and decreases.
* If the table is in the DELETING
state, no information
* about indexes will be returned.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param globalSecondaryIndexes The global secondary indexes, if any, on the table. Each index is
* scoped to a given hash key value. Each element is composed of:
* -
IndexName - The name of the global secondary index.
*
-
IndexSizeBytes - The total size of the global
* secondary index, in bytes. DynamoDB updates this value approximately
* every six hours. Recent changes might not be reflected in this value.
*
-
IndexStatus - The current status of the global
* secondary index:
-
CREATING - The index is being
* created.
-
UPDATING - The index is being updated.
*
-
DELETING - The index is being deleted.
-
*
ACTIVE - The index is ready for use.
-
*
ItemCount - The number of items in the global secondary
* index. DynamoDB updates this value approximately every six hours.
* Recent changes might not be reflected in this value.
-
*
KeySchema - Specifies the complete index key schema. The
* attribute names in the key schema must be between 1 and 255 characters
* (inclusive). The key schema must begin with the same hash key
* attribute as the table.
-
Projection - Specifies
* attributes that are copied (projected) from the table into the index.
* These are in addition to the primary key attributes and index key
* attributes, which are automatically projected. Each attribute
* specification is composed of:
-
ProjectionType - One
* of the following:
-
KEYS_ONLY
- Only the index
* and primary keys are projected into the index.
-
*
INCLUDE
- Only the specified table attributes are
* projected into the index. The list of projected attributes are in
* NonKeyAttributes.
-
ALL
- All of the
* table attributes are projected into the index.
-
*
NonKeyAttributes - A list of one or more non-key attribute
* names that are projected into the secondary index. The total count of
* attributes specified in NonKeyAttributes, summed across all of
* the secondary indexes, must not exceed 20. If you project the same
* attribute into two different indexes, this counts as two distinct
* attributes when determining the total.
-
*
ProvisionedThroughput - The provisioned throughput settings
* for the global secondary index, consisting of read and write capacity
* units, along with data about increases and decreases.
* If the table is in the DELETING
state, no information
* about indexes will be returned.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public TableDescription withGlobalSecondaryIndexes(java.util.Collection globalSecondaryIndexes) {
if (globalSecondaryIndexes == null) {
this.globalSecondaryIndexes = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag globalSecondaryIndexesCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(globalSecondaryIndexes.size());
globalSecondaryIndexesCopy.addAll(globalSecondaryIndexes);
this.globalSecondaryIndexes = globalSecondaryIndexesCopy;
}
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAttributeDefinitions() != null) sb.append("AttributeDefinitions: " + getAttributeDefinitions() + ",");
if (getTableName() != null) sb.append("TableName: " + getTableName() + ",");
if (getKeySchema() != null) sb.append("KeySchema: " + getKeySchema() + ",");
if (getTableStatus() != null) sb.append("TableStatus: " + getTableStatus() + ",");
if (getCreationDateTime() != null) sb.append("CreationDateTime: " + getCreationDateTime() + ",");
if (getProvisionedThroughput() != null) sb.append("ProvisionedThroughput: " + getProvisionedThroughput() + ",");
if (getTableSizeBytes() != null) sb.append("TableSizeBytes: " + getTableSizeBytes() + ",");
if (getItemCount() != null) sb.append("ItemCount: " + getItemCount() + ",");
if (getLocalSecondaryIndexes() != null) sb.append("LocalSecondaryIndexes: " + getLocalSecondaryIndexes() + ",");
if (getGlobalSecondaryIndexes() != null) sb.append("GlobalSecondaryIndexes: " + getGlobalSecondaryIndexes() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAttributeDefinitions() == null) ? 0 : getAttributeDefinitions().hashCode());
hashCode = prime * hashCode + ((getTableName() == null) ? 0 : getTableName().hashCode());
hashCode = prime * hashCode + ((getKeySchema() == null) ? 0 : getKeySchema().hashCode());
hashCode = prime * hashCode + ((getTableStatus() == null) ? 0 : getTableStatus().hashCode());
hashCode = prime * hashCode + ((getCreationDateTime() == null) ? 0 : getCreationDateTime().hashCode());
hashCode = prime * hashCode + ((getProvisionedThroughput() == null) ? 0 : getProvisionedThroughput().hashCode());
hashCode = prime * hashCode + ((getTableSizeBytes() == null) ? 0 : getTableSizeBytes().hashCode());
hashCode = prime * hashCode + ((getItemCount() == null) ? 0 : getItemCount().hashCode());
hashCode = prime * hashCode + ((getLocalSecondaryIndexes() == null) ? 0 : getLocalSecondaryIndexes().hashCode());
hashCode = prime * hashCode + ((getGlobalSecondaryIndexes() == null) ? 0 : getGlobalSecondaryIndexes().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof TableDescription == false) return false;
TableDescription other = (TableDescription)obj;
if (other.getAttributeDefinitions() == null ^ this.getAttributeDefinitions() == null) return false;
if (other.getAttributeDefinitions() != null && other.getAttributeDefinitions().equals(this.getAttributeDefinitions()) == false) return false;
if (other.getTableName() == null ^ this.getTableName() == null) return false;
if (other.getTableName() != null && other.getTableName().equals(this.getTableName()) == false) return false;
if (other.getKeySchema() == null ^ this.getKeySchema() == null) return false;
if (other.getKeySchema() != null && other.getKeySchema().equals(this.getKeySchema()) == false) return false;
if (other.getTableStatus() == null ^ this.getTableStatus() == null) return false;
if (other.getTableStatus() != null && other.getTableStatus().equals(this.getTableStatus()) == false) return false;
if (other.getCreationDateTime() == null ^ this.getCreationDateTime() == null) return false;
if (other.getCreationDateTime() != null && other.getCreationDateTime().equals(this.getCreationDateTime()) == false) return false;
if (other.getProvisionedThroughput() == null ^ this.getProvisionedThroughput() == null) return false;
if (other.getProvisionedThroughput() != null && other.getProvisionedThroughput().equals(this.getProvisionedThroughput()) == false) return false;
if (other.getTableSizeBytes() == null ^ this.getTableSizeBytes() == null) return false;
if (other.getTableSizeBytes() != null && other.getTableSizeBytes().equals(this.getTableSizeBytes()) == false) return false;
if (other.getItemCount() == null ^ this.getItemCount() == null) return false;
if (other.getItemCount() != null && other.getItemCount().equals(this.getItemCount()) == false) return false;
if (other.getLocalSecondaryIndexes() == null ^ this.getLocalSecondaryIndexes() == null) return false;
if (other.getLocalSecondaryIndexes() != null && other.getLocalSecondaryIndexes().equals(this.getLocalSecondaryIndexes()) == false) return false;
if (other.getGlobalSecondaryIndexes() == null ^ this.getGlobalSecondaryIndexes() == null) return false;
if (other.getGlobalSecondaryIndexes() != null && other.getGlobalSecondaryIndexes().equals(this.getGlobalSecondaryIndexes()) == false) return false;
return true;
}
}