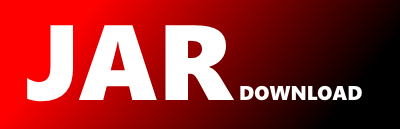
com.amazonaws.services.ec2.AmazonEC2Client Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.ec2;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import java.util.Map.Entry;
import com.amazonaws.*;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.services.ec2.model.*;
import com.amazonaws.services.ec2.model.transform.*;
/**
* Client for accessing AmazonEC2. All service calls made
* using this client are blocking, and will not return until the service call
* completes.
*
* Amazon Elastic Compute Cloud
* Amazon Elastic Compute Cloud (Amazon EC2) provides resizable computing
* capacity in the Amazon Web Services (AWS) cloud. Using Amazon EC2
* eliminates your need to invest in hardware up front, so you can
* develop and deploy applications faster.
*
*/
public class AmazonEC2Client extends AmazonWebServiceClient implements AmazonEC2 {
/** Provider for AWS credentials. */
private AWSCredentialsProvider awsCredentialsProvider;
/**
* List of exception unmarshallers for all AmazonEC2 exceptions.
*/
protected final List> exceptionUnmarshallers
= new ArrayList>();
/**
* Constructs a new client to invoke service methods on
* AmazonEC2. A credentials provider chain will be used
* that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @see DefaultAWSCredentialsProviderChain
*/
public AmazonEC2Client() {
this(new DefaultAWSCredentialsProviderChain(), new ClientConfiguration());
}
/**
* Constructs a new client to invoke service methods on
* AmazonEC2. A credentials provider chain will be used
* that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to AmazonEC2
* (ex: proxy settings, retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
*/
public AmazonEC2Client(ClientConfiguration clientConfiguration) {
this(new DefaultAWSCredentialsProviderChain(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on
* AmazonEC2 using the specified AWS account credentials.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentials The AWS credentials (access key ID and secret key) to use
* when authenticating with AWS services.
*/
public AmazonEC2Client(AWSCredentials awsCredentials) {
this(awsCredentials, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service methods on
* AmazonEC2 using the specified AWS account credentials
* and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentials The AWS credentials (access key ID and secret key) to use
* when authenticating with AWS services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to AmazonEC2
* (ex: proxy settings, retry counts, etc.).
*/
public AmazonEC2Client(AWSCredentials awsCredentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.awsCredentialsProvider = new StaticCredentialsProvider(awsCredentials);
init();
}
/**
* Constructs a new client to invoke service methods on
* AmazonEC2 using the specified AWS account credentials provider.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
*/
public AmazonEC2Client(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service methods on
* AmazonEC2 using the specified AWS account credentials
* provider and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to AmazonEC2
* (ex: proxy settings, retry counts, etc.).
*/
public AmazonEC2Client(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on
* AmazonEC2 using the specified AWS account credentials
* provider, client configuration options, and request metric collector.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to AmazonEC2
* (ex: proxy settings, retry counts, etc.).
* @param requestMetricCollector optional request metric collector
*/
public AmazonEC2Client(AWSCredentialsProvider awsCredentialsProvider,
ClientConfiguration clientConfiguration,
RequestMetricCollector requestMetricCollector) {
super(clientConfiguration, requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
init();
}
private void init() {
exceptionUnmarshallers.add(new LegacyErrorUnmarshaller());
// calling this.setEndPoint(...) will also modify the signer accordingly
this.setEndpoint("ec2.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain(
"/com/amazonaws/services/ec2/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain(
"/com/amazonaws/services/ec2/request.handler2s"));
}
/**
*
* Requests a reboot of one or more instances. This operation is
* asynchronous; it only queues a request to reboot the specified
* instances. The operation succeeds if the instances are valid and
* belong to you. Requests to reboot terminated instances are ignored.
*
*
* If a Linux/Unix instance does not cleanly shut down within four
* minutes, Amazon EC2 performs a hard reboot.
*
*
* @param rebootInstancesRequest Container for the necessary parameters
* to execute the RebootInstances service method on AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void rebootInstances(RebootInstancesRequest rebootInstancesRequest) {
ExecutionContext executionContext = createExecutionContext(rebootInstancesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new RebootInstancesRequestMarshaller().marshall(rebootInstancesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Describes one or more of the Reserved Instances that you purchased.
*
*
* For more information about Reserved Instances, see
* Reserved Instances
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param describeReservedInstancesRequest Container for the necessary
* parameters to execute the DescribeReservedInstances service method on
* AmazonEC2.
*
* @return The response from the DescribeReservedInstances service
* method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeReservedInstancesResult describeReservedInstances(DescribeReservedInstancesRequest describeReservedInstancesRequest) {
ExecutionContext executionContext = createExecutionContext(describeReservedInstancesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeReservedInstancesRequestMarshaller().marshall(describeReservedInstancesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeReservedInstancesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes one or more of the Availability Zones that are available to
* you. The results include zones only for the region you're currently
* using. If there is an event impacting an Availability Zone, you can
* use this request to view the state and any provided message for that
* Availability Zone.
*
*
* For more information, see
* Regions and Availability Zones
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param describeAvailabilityZonesRequest Container for the necessary
* parameters to execute the DescribeAvailabilityZones service method on
* AmazonEC2.
*
* @return The response from the DescribeAvailabilityZones service
* method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeAvailabilityZonesResult describeAvailabilityZones(DescribeAvailabilityZonesRequest describeAvailabilityZonesRequest) {
ExecutionContext executionContext = createExecutionContext(describeAvailabilityZonesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeAvailabilityZonesRequestMarshaller().marshall(describeAvailabilityZonesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeAvailabilityZonesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Detaches an Amazon EBS volume from an instance. Make sure to unmount
* any file systems on the device within your operating system before
* detaching the volume. Failure to do so results in the volume being
* stuck in a busy state while detaching.
*
*
* If an Amazon EBS volume is the root device of an instance, it can't be
* detached while the instance is running. To detach the root volume,
* stop the instance first.
*
*
* If the root volume is detached from an instance with an AWS
* Marketplace product code, then the AWS Marketplace product codes from
* that volume are no longer associated with the instance.
*
*
* For more information, see
* Detaching an Amazon EBS Volume
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param detachVolumeRequest Container for the necessary parameters to
* execute the DetachVolume service method on AmazonEC2.
*
* @return The response from the DetachVolume service method, as returned
* by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DetachVolumeResult detachVolume(DetachVolumeRequest detachVolumeRequest) {
ExecutionContext executionContext = createExecutionContext(detachVolumeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DetachVolumeRequestMarshaller().marshall(detachVolumeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DetachVolumeResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified key pair, by removing the public key from Amazon
* EC2.
*
*
* @param deleteKeyPairRequest Container for the necessary parameters to
* execute the DeleteKeyPair service method on AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteKeyPair(DeleteKeyPairRequest deleteKeyPairRequest) {
ExecutionContext executionContext = createExecutionContext(deleteKeyPairRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteKeyPairRequestMarshaller().marshall(deleteKeyPairRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Disables monitoring for a running instance. For more information about
* monitoring instances, see
* Monitoring Your Instances and Volumes
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param unmonitorInstancesRequest Container for the necessary
* parameters to execute the UnmonitorInstances service method on
* AmazonEC2.
*
* @return The response from the UnmonitorInstances service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public UnmonitorInstancesResult unmonitorInstances(UnmonitorInstancesRequest unmonitorInstancesRequest) {
ExecutionContext executionContext = createExecutionContext(unmonitorInstancesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new UnmonitorInstancesRequestMarshaller().marshall(unmonitorInstancesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new UnmonitorInstancesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Attaches a virtual private gateway to a VPC. For more information, see
* Adding a Hardware Virtual Private Gateway to Your VPC
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param attachVpnGatewayRequest Container for the necessary parameters
* to execute the AttachVpnGateway service method on AmazonEC2.
*
* @return The response from the AttachVpnGateway service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public AttachVpnGatewayResult attachVpnGateway(AttachVpnGatewayRequest attachVpnGatewayRequest) {
ExecutionContext executionContext = createExecutionContext(attachVpnGatewayRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new AttachVpnGatewayRequestMarshaller().marshall(attachVpnGatewayRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new AttachVpnGatewayResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Amazon EBS-backed AMI from an Amazon EBS-backed instance
* that is either running or stopped.
*
*
* If you customized your instance with instance store volumes or EBS
* volumes in addition to the root device volume, the new AMI contains
* block device mapping information for those volumes. When you launch an
* instance from this new AMI, the instance automatically launches with
* those additional volumes.
*
*
* For more information, see
* Creating Amazon EBS-Backed Linux AMIs
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param createImageRequest Container for the necessary parameters to
* execute the CreateImage service method on AmazonEC2.
*
* @return The response from the CreateImage service method, as returned
* by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateImageResult createImage(CreateImageRequest createImageRequest) {
ExecutionContext executionContext = createExecutionContext(createImageRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateImageRequestMarshaller().marshall(createImageRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateImageResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a security group.
*
*
* If you attempt to delete a security group that is associated with an
* instance, or is referenced by another security group, the operation
* fails with InvalidGroup.InUse
in EC2-Classic or
* DependencyViolation
in EC2-VPC.
*
*
* @param deleteSecurityGroupRequest Container for the necessary
* parameters to execute the DeleteSecurityGroup service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteSecurityGroup(DeleteSecurityGroupRequest deleteSecurityGroupRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSecurityGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteSecurityGroupRequestMarshaller().marshall(deleteSecurityGroupRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Exports a running or stopped instance to an Amazon S3 bucket.
*
*
* For information about the supported operating systems, image formats,
* and known limitations for the types of instances you can export, see
* Exporting EC2 Instances
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param createInstanceExportTaskRequest Container for the necessary
* parameters to execute the CreateInstanceExportTask service method on
* AmazonEC2.
*
* @return The response from the CreateInstanceExportTask service method,
* as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateInstanceExportTaskResult createInstanceExportTask(CreateInstanceExportTaskRequest createInstanceExportTaskRequest) {
ExecutionContext executionContext = createExecutionContext(createInstanceExportTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateInstanceExportTaskRequestMarshaller().marshall(createInstanceExportTaskRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateInstanceExportTaskResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the encrypted administrator password for an instance running
* Windows.
*
*
* The Windows password is only generated the first time an AMI is
* launched. It is not generated for rebundled AMIs or after the password
* is changed on an instance.
*
*
* The password is encrypted using the key pair that you specified when
* you launched the instance. You must provide the corresponding key pair
* file.
*
*
* Password generation and encryption takes a few moments. We recommend
* that you wait up to 15 minutes after launching an instance before
* trying to retrieve the generated password.
*
*
* @param getPasswordDataRequest Container for the necessary parameters
* to execute the GetPasswordData service method on AmazonEC2.
*
* @return The response from the GetPasswordData service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public GetPasswordDataResult getPasswordData(GetPasswordDataRequest getPasswordDataRequest) {
ExecutionContext executionContext = createExecutionContext(getPasswordDataRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new GetPasswordDataRequestMarshaller().marshall(getPasswordDataRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new GetPasswordDataResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates a set of DHCP options (that you've previously created) with
* the specified VPC, or associates no DHCP options with the VPC.
*
*
* After you associate the options with the VPC, any existing instances
* and all new instances that you launch in that VPC use the options. You
* don't need to restart or relaunch the instances. They automatically
* pick up the changes within a few hours, depending on how frequently
* the instance renews its DHCP lease. You can explicitly renew the lease
* using the operating system on the instance.
*
*
* For more information, see
* DHCP Options Sets
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param associateDhcpOptionsRequest Container for the necessary
* parameters to execute the AssociateDhcpOptions service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void associateDhcpOptions(AssociateDhcpOptionsRequest associateDhcpOptionsRequest) {
ExecutionContext executionContext = createExecutionContext(associateDhcpOptionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new AssociateDhcpOptionsRequestMarshaller().marshall(associateDhcpOptionsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Adds one or more egress rules to a security group for use with a VPC.
* Specifically, this action permits instances to send traffic to one or
* more CIDR IP address ranges, or to one or more security groups for the
* same VPC.
*
*
* IMPORTANT: You can have up to 50 rules per security group
* (covering both ingress and egress rules).
*
*
* A security group is for use with instances either in the EC2-Classic
* platform or in a specific VPC. This action doesn't apply to security
* groups for use in EC2-Classic. For more information, see
* Security Groups for Your VPC
* in the Amazon Virtual Private Cloud User Guide .
*
*
* Each rule consists of the protocol (for example, TCP), plus either a
* CIDR range or a source group. For the TCP and UDP protocols, you must
* also specify the destination port or port range. For the ICMP
* protocol, you must also specify the ICMP type and code. You can use -1
* for the type or code to mean all types or all codes.
*
*
* Rule changes are propagated to affected instances as quickly as
* possible. However, a small delay might occur.
*
*
* @param authorizeSecurityGroupEgressRequest Container for the necessary
* parameters to execute the AuthorizeSecurityGroupEgress service method
* on AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void authorizeSecurityGroupEgress(AuthorizeSecurityGroupEgressRequest authorizeSecurityGroupEgressRequest) {
ExecutionContext executionContext = createExecutionContext(authorizeSecurityGroupEgressRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new AuthorizeSecurityGroupEgressRequestMarshaller().marshall(authorizeSecurityGroupEgressRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Stops an Amazon EBS-backed instance. Each time you transition an
* instance from stopped to started, Amazon EC2 charges a full instance
* hour, even if transitions happen multiple times within a single hour.
*
*
* You can't start or stop Spot Instances.
*
*
* Instances that use Amazon EBS volumes as their root devices can be
* quickly stopped and started. When an instance is stopped, the compute
* resources are released and you are not billed for hourly instance
* usage. However, your root partition Amazon EBS volume remains,
* continues to persist your data, and you are charged for Amazon EBS
* volume usage. You can restart your instance at any time.
*
*
* Before stopping an instance, make sure it is in a state from which it
* can be restarted. Stopping an instance does not preserve data stored
* in RAM.
*
*
* Performing this operation on an instance that uses an instance store
* as its root device returns an error.
*
*
* You can stop, start, and terminate EBS-backed instances. You can only
* terminate instance store-backed instances. What happens to an instance
* differs if you stop it or terminate it. For example, when you stop an
* instance, the root device and any other devices attached to the
* instance persist. When you terminate an instance, the root device and
* any other devices attached during the instance launch are
* automatically deleted. For more information about the differences
* between stopping and terminating instances, see
* Instance Lifecycle
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param stopInstancesRequest Container for the necessary parameters to
* execute the StopInstances service method on AmazonEC2.
*
* @return The response from the StopInstances service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public StopInstancesResult stopInstances(StopInstancesRequest stopInstancesRequest) {
ExecutionContext executionContext = createExecutionContext(stopInstancesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new StopInstancesRequestMarshaller().marshall(stopInstancesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new StopInstancesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Imports the public key from an RSA key pair that you created with a
* third-party tool. Compare this with CreateKeyPair, in which AWS
* creates the key pair and gives the keys to you (AWS keeps a copy of
* the public key). With ImportKeyPair, you create the key pair and give
* AWS just the public key. The private key is never transferred between
* you and AWS.
*
*
* For more information about key pairs, see
* Key Pairs
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param importKeyPairRequest Container for the necessary parameters to
* execute the ImportKeyPair service method on AmazonEC2.
*
* @return The response from the ImportKeyPair service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public ImportKeyPairResult importKeyPair(ImportKeyPairRequest importKeyPairRequest) {
ExecutionContext executionContext = createExecutionContext(importKeyPairRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ImportKeyPairRequestMarshaller().marshall(importKeyPairRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ImportKeyPairResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified network interface. You must detach the network
* interface before you can delete it.
*
*
* @param deleteNetworkInterfaceRequest Container for the necessary
* parameters to execute the DeleteNetworkInterface service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteNetworkInterface(DeleteNetworkInterfaceRequest deleteNetworkInterfaceRequest) {
ExecutionContext executionContext = createExecutionContext(deleteNetworkInterfaceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteNetworkInterfaceRequestMarshaller().marshall(deleteNetworkInterfaceRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Modifies the specified attribute of the specified VPC.
*
*
* @param modifyVpcAttributeRequest Container for the necessary
* parameters to execute the ModifyVpcAttribute service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void modifyVpcAttribute(ModifyVpcAttributeRequest modifyVpcAttributeRequest) {
ExecutionContext executionContext = createExecutionContext(modifyVpcAttributeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ModifyVpcAttributeRequestMarshaller().marshall(modifyVpcAttributeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Creates a security group.
*
*
* A security group is for use with instances either in the EC2-Classic
* platform or in a specific VPC. For more information, see
* Amazon EC2 Security Groups in the Amazon Elastic Compute Cloud User Guide and Security Groups for Your VPC
* in the Amazon Virtual Private Cloud User Guide .
*
*
* IMPORTANT: EC2-Classic: You can have up to 500 security groups.
* EC2-VPC: You can create up to 100 security groups per VPC.
*
*
* When you create a security group, you specify a friendly name of your
* choice. You can have a security group for use in EC2-Classic with the
* same name as a security group for use in a VPC. However, you can't
* have two security groups for use in EC2-Classic with the same name or
* two security groups for use in a VPC with the same name.
*
*
* You have a default security group for use in EC2-Classic and a default
* security group for use in your VPC. If you don't specify a security
* group when you launch an instance, the instance is launched into the
* appropriate default security group. A default security group includes
* a default rule that grants instances unrestricted network access to
* each other.
*
*
* You can add or remove rules from your security groups using
* AuthorizeSecurityGroupIngress, AuthorizeSecurityGroupEgress,
* RevokeSecurityGroupIngress, and RevokeSecurityGroupEgress.
*
*
* @param createSecurityGroupRequest Container for the necessary
* parameters to execute the CreateSecurityGroup service method on
* AmazonEC2.
*
* @return The response from the CreateSecurityGroup service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateSecurityGroupResult createSecurityGroup(CreateSecurityGroupRequest createSecurityGroupRequest) {
ExecutionContext executionContext = createExecutionContext(createSecurityGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateSecurityGroupRequestMarshaller().marshall(createSecurityGroupRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateSecurityGroupResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the Spot Price history. Spot Instances are instances that
* Amazon EC2 starts on your behalf when the maximum price that you
* specify exceeds the current Spot Price. Amazon EC2 periodically sets
* the Spot Price based on available Spot Instance capacity and current
* Spot Instance requests. For more information about Spot Instances, see
* Spot Instances
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* When you specify an Availability Zone, this operation describes the
* price history for the specified Availability Zone with the most recent
* set of prices listed first. If you don't specify an Availability Zone,
* you get the prices across all Availability Zones, starting with the
* most recent set. However, if you're using an API version earlier than
* 2011-05-15, you get the lowest price across the region for the
* specified time period. The prices returned are listed in chronological
* order, from the oldest to the most recent.
*
*
* @param describeSpotPriceHistoryRequest Container for the necessary
* parameters to execute the DescribeSpotPriceHistory service method on
* AmazonEC2.
*
* @return The response from the DescribeSpotPriceHistory service method,
* as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeSpotPriceHistoryResult describeSpotPriceHistory(DescribeSpotPriceHistoryRequest describeSpotPriceHistoryRequest) {
ExecutionContext executionContext = createExecutionContext(describeSpotPriceHistoryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeSpotPriceHistoryRequestMarshaller().marshall(describeSpotPriceHistoryRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeSpotPriceHistoryResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes one or more of your network interfaces.
*
*
* @param describeNetworkInterfacesRequest Container for the necessary
* parameters to execute the DescribeNetworkInterfaces service method on
* AmazonEC2.
*
* @return The response from the DescribeNetworkInterfaces service
* method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeNetworkInterfacesResult describeNetworkInterfaces(DescribeNetworkInterfacesRequest describeNetworkInterfacesRequest) {
ExecutionContext executionContext = createExecutionContext(describeNetworkInterfacesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeNetworkInterfacesRequestMarshaller().marshall(describeNetworkInterfacesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeNetworkInterfacesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes one or more regions that are currently available to you.
*
*
* For a list of the regions supported by Amazon EC2, see
* Regions and Endpoints
* .
*
*
* @param describeRegionsRequest Container for the necessary parameters
* to execute the DescribeRegions service method on AmazonEC2.
*
* @return The response from the DescribeRegions service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeRegionsResult describeRegions(DescribeRegionsRequest describeRegionsRequest) {
ExecutionContext executionContext = createExecutionContext(describeRegionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeRegionsRequestMarshaller().marshall(describeRegionsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeRegionsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a listing for Amazon EC2 Reserved Instances to be sold in the
* Reserved Instance Marketplace. You can submit one Reserved Instance
* listing at a time.
*
*
* For more information, see
* Reserved Instance Marketplace
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param createReservedInstancesListingRequest Container for the
* necessary parameters to execute the CreateReservedInstancesListing
* service method on AmazonEC2.
*
* @return The response from the CreateReservedInstancesListing service
* method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateReservedInstancesListingResult createReservedInstancesListing(CreateReservedInstancesListingRequest createReservedInstancesListingRequest) {
ExecutionContext executionContext = createExecutionContext(createReservedInstancesListingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateReservedInstancesListingRequestMarshaller().marshall(createReservedInstancesListingRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateReservedInstancesListingResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a set of DHCP options for your VPC. After creating the set,
* you must associate it with the VPC, causing all existing and new
* instances that you launch in the VPC to use this set of DHCP options.
* The following are the individual DHCP options you can specify. For
* more information about the options, see
* RFC 2132
* .
*
*
* For more information about DHCP options, see
* DHCP Options Sets
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param createDhcpOptionsRequest Container for the necessary parameters
* to execute the CreateDhcpOptions service method on AmazonEC2.
*
* @return The response from the CreateDhcpOptions service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateDhcpOptionsResult createDhcpOptions(CreateDhcpOptionsRequest createDhcpOptionsRequest) {
ExecutionContext executionContext = createExecutionContext(createDhcpOptionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateDhcpOptionsRequestMarshaller().marshall(createDhcpOptionsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateDhcpOptionsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Resets permission settings for the specified snapshot.
*
*
* For more information on modifying snapshot permissions, see
* Sharing Snapshots
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param resetSnapshotAttributeRequest Container for the necessary
* parameters to execute the ResetSnapshotAttribute service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void resetSnapshotAttribute(ResetSnapshotAttributeRequest resetSnapshotAttributeRequest) {
ExecutionContext executionContext = createExecutionContext(resetSnapshotAttributeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ResetSnapshotAttributeRequestMarshaller().marshall(resetSnapshotAttributeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Deletes the specified route from the specified route table.
*
*
* @param deleteRouteRequest Container for the necessary parameters to
* execute the DeleteRoute service method on AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteRoute(DeleteRouteRequest deleteRouteRequest) {
ExecutionContext executionContext = createExecutionContext(deleteRouteRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteRouteRequestMarshaller().marshall(deleteRouteRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Describes one or more of your Internet gateways.
*
*
* @param describeInternetGatewaysRequest Container for the necessary
* parameters to execute the DescribeInternetGateways service method on
* AmazonEC2.
*
* @return The response from the DescribeInternetGateways service method,
* as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeInternetGatewaysResult describeInternetGateways(DescribeInternetGatewaysRequest describeInternetGatewaysRequest) {
ExecutionContext executionContext = createExecutionContext(describeInternetGatewaysRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeInternetGatewaysRequestMarshaller().marshall(describeInternetGatewaysRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeInternetGatewaysResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an import volume task using metadata from the specified disk
* image. After importing the image, you then upload it using the
* ec2-upload-disk-image command in the Amazon EC2 command-line interface
* (CLI) tools. For more information, see
* Using the Command Line Tools to Import Your Virtual Machine to Amazon EC2
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param importVolumeRequest Container for the necessary parameters to
* execute the ImportVolume service method on AmazonEC2.
*
* @return The response from the ImportVolume service method, as returned
* by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public ImportVolumeResult importVolume(ImportVolumeRequest importVolumeRequest) {
ExecutionContext executionContext = createExecutionContext(importVolumeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ImportVolumeRequestMarshaller().marshall(importVolumeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ImportVolumeResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes one or more of your security groups.
*
*
* A security group is for use with instances either in the EC2-Classic
* platform or in a specific VPC. For more information, see
* Amazon EC2 Security Groups in the Amazon Elastic Compute Cloud User Guide and Security Groups for Your VPC
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param describeSecurityGroupsRequest Container for the necessary
* parameters to execute the DescribeSecurityGroups service method on
* AmazonEC2.
*
* @return The response from the DescribeSecurityGroups service method,
* as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeSecurityGroupsResult describeSecurityGroups(DescribeSecurityGroupsRequest describeSecurityGroupsRequest) {
ExecutionContext executionContext = createExecutionContext(describeSecurityGroupsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeSecurityGroupsRequestMarshaller().marshall(describeSecurityGroupsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeSecurityGroupsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Rejects a VPC peering connection request. The VPC peering connection
* must be in the pending-acceptance
state. Use the
* DescribeVpcPeeringConnections
request to view your
* outstanding VPC peering connection requests.
*
*
* @param rejectVpcPeeringConnectionRequest Container for the necessary
* parameters to execute the RejectVpcPeeringConnection service method on
* AmazonEC2.
*
* @return The response from the RejectVpcPeeringConnection service
* method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public RejectVpcPeeringConnectionResult rejectVpcPeeringConnection(RejectVpcPeeringConnectionRequest rejectVpcPeeringConnectionRequest) {
ExecutionContext executionContext = createExecutionContext(rejectVpcPeeringConnectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new RejectVpcPeeringConnectionRequestMarshaller().marshall(rejectVpcPeeringConnectionRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new RejectVpcPeeringConnectionResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Detaches a virtual private gateway from a VPC. You do this if you're
* planning to turn off the VPC and not use it anymore. You can confirm a
* virtual private gateway has been completely detached from a VPC by
* describing the virtual private gateway (any attachments to the virtual
* private gateway are also described).
*
*
* You must wait for the attachment's state to switch to
* detached
before you can delete the VPC or attach a
* different VPC to the virtual private gateway.
*
*
* @param detachVpnGatewayRequest Container for the necessary parameters
* to execute the DetachVpnGateway service method on AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void detachVpnGateway(DetachVpnGatewayRequest detachVpnGatewayRequest) {
ExecutionContext executionContext = createExecutionContext(detachVpnGatewayRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DetachVpnGatewayRequestMarshaller().marshall(detachVpnGatewayRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Deregisters the specified AMI. After you deregister an AMI, it can't
* be used to launch new instances.
*
*
* This command does not delete the AMI.
*
*
* @param deregisterImageRequest Container for the necessary parameters
* to execute the DeregisterImage service method on AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deregisterImage(DeregisterImageRequest deregisterImageRequest) {
ExecutionContext executionContext = createExecutionContext(deregisterImageRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeregisterImageRequestMarshaller().marshall(deregisterImageRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Describes the datafeed for Spot Instances. For more information, see
* Spot Instances
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param describeSpotDatafeedSubscriptionRequest Container for the
* necessary parameters to execute the DescribeSpotDatafeedSubscription
* service method on AmazonEC2.
*
* @return The response from the DescribeSpotDatafeedSubscription service
* method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeSpotDatafeedSubscriptionResult describeSpotDatafeedSubscription(DescribeSpotDatafeedSubscriptionRequest describeSpotDatafeedSubscriptionRequest) {
ExecutionContext executionContext = createExecutionContext(describeSpotDatafeedSubscriptionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeSpotDatafeedSubscriptionRequestMarshaller().marshall(describeSpotDatafeedSubscriptionRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeSpotDatafeedSubscriptionResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified set of tags from the specified set of resources.
* This call is designed to follow a DescribeTags
request.
*
*
* For more information about tags, see
* Tagging Your Resources
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param deleteTagsRequest Container for the necessary parameters to
* execute the DeleteTags service method on AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteTags(DeleteTagsRequest deleteTagsRequest) {
ExecutionContext executionContext = createExecutionContext(deleteTagsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteTagsRequestMarshaller().marshall(deleteTagsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Deletes the specified subnet. You must terminate all running instances
* in the subnet before you can delete the subnet.
*
*
* @param deleteSubnetRequest Container for the necessary parameters to
* execute the DeleteSubnet service method on AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteSubnet(DeleteSubnetRequest deleteSubnetRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSubnetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteSubnetRequestMarshaller().marshall(deleteSubnetRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Describes the specified attribute of your AWS account.
*
*
* @param describeAccountAttributesRequest Container for the necessary
* parameters to execute the DescribeAccountAttributes service method on
* AmazonEC2.
*
* @return The response from the DescribeAccountAttributes service
* method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeAccountAttributesResult describeAccountAttributes(DescribeAccountAttributesRequest describeAccountAttributesRequest) {
ExecutionContext executionContext = createExecutionContext(describeAccountAttributesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeAccountAttributesRequestMarshaller().marshall(describeAccountAttributesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeAccountAttributesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a virtual private gateway. A virtual private gateway is the
* endpoint on the VPC side of your VPN connection. You can create a
* virtual private gateway before creating the VPC itself.
*
*
* For more information about virtual private gateways, see
* Adding a Hardware Virtual Private Gateway to Your VPC
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param createVpnGatewayRequest Container for the necessary parameters
* to execute the CreateVpnGateway service method on AmazonEC2.
*
* @return The response from the CreateVpnGateway service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateVpnGatewayResult createVpnGateway(CreateVpnGatewayRequest createVpnGatewayRequest) {
ExecutionContext executionContext = createExecutionContext(createVpnGatewayRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateVpnGatewayRequestMarshaller().marshall(createVpnGatewayRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateVpnGatewayResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Enables I/O operations for a volume that had I/O operations disabled
* because the data on the volume was potentially inconsistent.
*
*
* @param enableVolumeIORequest Container for the necessary parameters to
* execute the EnableVolumeIO service method on AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void enableVolumeIO(EnableVolumeIORequest enableVolumeIORequest) {
ExecutionContext executionContext = createExecutionContext(enableVolumeIORequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new EnableVolumeIORequestMarshaller().marshall(enableVolumeIORequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Deletes the specified virtual private gateway. We recommend that
* before you delete a virtual private gateway, you detach it from the
* VPC and delete the VPN connection. Note that you don't need to delete
* the virtual private gateway if you plan to delete and recreate the VPN
* connection between your VPC and your network.
*
*
* @param deleteVpnGatewayRequest Container for the necessary parameters
* to execute the DeleteVpnGateway service method on AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteVpnGateway(DeleteVpnGatewayRequest deleteVpnGatewayRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVpnGatewayRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteVpnGatewayRequestMarshaller().marshall(deleteVpnGatewayRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Attaches an Amazon EBS volume to a running or stopped instance and
* exposes it to the instance with the specified device name.
*
*
* For a list of supported device names, see
* Attaching an Amazon EBS Volume to an Instance . Any device names that aren't reserved for instance store volumes can be used for Amazon EBS volumes. For more information, see Amazon EC2 Instance Store
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* If a volume has an AWS Marketplace product code:
*
*
*
* - The volume can only be attached as the root device of a stopped
* instance.
* - You must be subscribed to the AWS Marketplace code that is on the
* volume.
* - The configuration (instance type, operating system) of the
* instance must support that specific AWS Marketplace code. For example,
* you cannot take a volume from a Windows instance and attach it to a
* Linux instance.
* - AWS Marketplace product codes are copied from the volume to the
* instance.
*
*
*
* For an overview of the AWS Marketplace, see
* https://aws.amazon.com/marketplace/help/200900000 . For more information about how to use the AWS Marketplace, see AWS Marketplace
* .
*
*
* For more information about Amazon EBS volumes, see
* Attaching Amazon EBS Volumes
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param attachVolumeRequest Container for the necessary parameters to
* execute the AttachVolume service method on AmazonEC2.
*
* @return The response from the AttachVolume service method, as returned
* by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public AttachVolumeResult attachVolume(AttachVolumeRequest attachVolumeRequest) {
ExecutionContext executionContext = createExecutionContext(attachVolumeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new AttachVolumeRequestMarshaller().marshall(attachVolumeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new AttachVolumeResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the status of the specified volumes. Volume status provides
* the result of the checks performed on your volumes to determine events
* that can impair the performance of your volumes. The performance of a
* volume can be affected if an issue occurs on the volume's underlying
* host. If the volume's underlying host experiences a power outage or
* system issue, after the system is restored, there could be data
* inconsistencies on the volume. Volume events notify you if this
* occurs. Volume actions notify you if any action needs to be taken in
* response to the event.
*
*
* The DescribeVolumeStatus
operation provides the following
* information about the specified volumes:
*
*
* Status : Reflects the current status of the volume. The
* possible values are ok
,
* impaired
,
* warning
, or
* insufficient-data
. If all checks pass, the overall
* status of the volume is ok
. If the check fails, the
* overall status is impaired
. If the status is
* insufficient-data
, then the checks may still be taking
* place on your volume at the time. We recommend that you retry the
* request. For more information on volume status, see
* Monitoring the Status of Your Volumes
* .
*
*
* Events : Reflect the cause of a volume status and may require
* you to take action. For example, if your volume returns an
* impaired
status, then the volume event might be
* potential-data-inconsistency
. This means that your
* volume has been affected by an issue with the underlying host, has all
* I/O operations disabled, and may have inconsistent data.
*
*
* Actions : Reflect the actions you may have to take in response
* to an event. For example, if the status of the volume is
* impaired
and the volume event shows
* potential-data-inconsistency
, then the action shows
* enable-volume-io
. This means that you may want to enable
* the I/O operations for the volume by calling the EnableVolumeIO action
* and then check the volume for data consistency.
*
*
* NOTE: Volume status is based on the volume status checks, and
* does not reflect the volume state. Therefore, volume status does not
* indicate volumes in the error state (for example, when a volume is
* incapable of accepting I/O.)
*
*
* @param describeVolumeStatusRequest Container for the necessary
* parameters to execute the DescribeVolumeStatus service method on
* AmazonEC2.
*
* @return The response from the DescribeVolumeStatus service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeVolumeStatusResult describeVolumeStatus(DescribeVolumeStatusRequest describeVolumeStatusRequest) {
ExecutionContext executionContext = createExecutionContext(describeVolumeStatusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeVolumeStatusRequestMarshaller().marshall(describeVolumeStatusRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeVolumeStatusResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Resets an attribute of an AMI to its default value.
*
*
* @param resetImageAttributeRequest Container for the necessary
* parameters to execute the ResetImageAttribute service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void resetImageAttribute(ResetImageAttributeRequest resetImageAttributeRequest) {
ExecutionContext executionContext = createExecutionContext(resetImageAttributeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ResetImageAttributeRequestMarshaller().marshall(resetImageAttributeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Describes one or more of your VPN connections.
*
*
* For more information about VPN connections, see
* Adding a Hardware Virtual Private Gateway to Your VPC
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param describeVpnConnectionsRequest Container for the necessary
* parameters to execute the DescribeVpnConnections service method on
* AmazonEC2.
*
* @return The response from the DescribeVpnConnections service method,
* as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeVpnConnectionsResult describeVpnConnections(DescribeVpnConnectionsRequest describeVpnConnectionsRequest) {
ExecutionContext executionContext = createExecutionContext(describeVpnConnectionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeVpnConnectionsRequestMarshaller().marshall(describeVpnConnectionsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeVpnConnectionsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Enables a virtual private gateway (VGW) to propagate routes to the
* routing tables of a VPC.
*
*
* @param enableVgwRoutePropagationRequest Container for the necessary
* parameters to execute the EnableVgwRoutePropagation service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void enableVgwRoutePropagation(EnableVgwRoutePropagationRequest enableVgwRoutePropagationRequest) {
ExecutionContext executionContext = createExecutionContext(enableVgwRoutePropagationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new EnableVgwRoutePropagationRequestMarshaller().marshall(enableVgwRoutePropagationRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Creates a snapshot of an Amazon EBS volume and stores it in Amazon S3.
* You can use snapshots for backups, to make copies of Amazon EBS
* volumes, and to save data before shutting down an instance.
*
*
* When a snapshot is created, any AWS Marketplace product codes that are
* associated with the source volume are propagated to the snapshot.
*
*
* You can take a snapshot of an attached volume that is in use. However,
* snapshots only capture data that has been written to your Amazon EBS
* volume at the time the snapshot command is issued; this may exclude
* any data that has been cached by any applications or the operating
* system. If you can pause any file writes to the volume long enough to
* take a snapshot, your snapshot should be complete. However, if you
* cannot pause all file writes to the volume, you should unmount the
* volume from within the instance, issue the snapshot command, and then
* remount the volume to ensure a consistent and complete snapshot. You
* may remount and use your volume while the snapshot status is
* pending
.
*
*
* To create a snapshot for Amazon EBS volumes that serve as root
* devices, you should stop the instance before taking the snapshot.
*
*
* For more information, see
* Creating an Amazon EBS Snapshot
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param createSnapshotRequest Container for the necessary parameters to
* execute the CreateSnapshot service method on AmazonEC2.
*
* @return The response from the CreateSnapshot service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateSnapshotResult createSnapshot(CreateSnapshotRequest createSnapshotRequest) {
ExecutionContext executionContext = createExecutionContext(createSnapshotRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateSnapshotRequestMarshaller().marshall(createSnapshotRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateSnapshotResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified Amazon EBS volume. The volume must be in the
* available
state (not attached to an instance).
*
*
* NOTE: The volume remains in the deleting state for several
* minutes.
*
*
* For more information, see
* Deleting an Amazon EBS Volume
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param deleteVolumeRequest Container for the necessary parameters to
* execute the DeleteVolume service method on AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteVolume(DeleteVolumeRequest deleteVolumeRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVolumeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteVolumeRequestMarshaller().marshall(deleteVolumeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Creates a network interface in the specified subnet.
*
*
* For more information about network interfaces, see
* Elastic Network Interfaces
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param createNetworkInterfaceRequest Container for the necessary
* parameters to execute the CreateNetworkInterface service method on
* AmazonEC2.
*
* @return The response from the CreateNetworkInterface service method,
* as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateNetworkInterfaceResult createNetworkInterface(CreateNetworkInterfaceRequest createNetworkInterfaceRequest) {
ExecutionContext executionContext = createExecutionContext(createNetworkInterfaceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateNetworkInterfaceRequestMarshaller().marshall(createNetworkInterfaceRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateNetworkInterfaceResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Modifies the Availability Zone, instance count, instance type, or
* network platform (EC2-Classic or EC2-VPC) of your Reserved Instances.
* The Reserved Instances to be modified must be identical, except for
* Availability Zone, network platform, and instance type.
*
*
* @param modifyReservedInstancesRequest Container for the necessary
* parameters to execute the ModifyReservedInstances service method on
* AmazonEC2.
*
* @return The response from the ModifyReservedInstances service method,
* as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public ModifyReservedInstancesResult modifyReservedInstances(ModifyReservedInstancesRequest modifyReservedInstancesRequest) {
ExecutionContext executionContext = createExecutionContext(modifyReservedInstancesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ModifyReservedInstancesRequestMarshaller().marshall(modifyReservedInstancesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ModifyReservedInstancesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes one or more of your VPCs.
*
*
* @param describeVpcsRequest Container for the necessary parameters to
* execute the DescribeVpcs service method on AmazonEC2.
*
* @return The response from the DescribeVpcs service method, as returned
* by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeVpcsResult describeVpcs(DescribeVpcsRequest describeVpcsRequest) {
ExecutionContext executionContext = createExecutionContext(describeVpcsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeVpcsRequestMarshaller().marshall(describeVpcsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeVpcsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Unassigns one or more secondary private IP addresses from a network
* interface.
*
*
* @param unassignPrivateIpAddressesRequest Container for the necessary
* parameters to execute the UnassignPrivateIpAddresses service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void unassignPrivateIpAddresses(UnassignPrivateIpAddressesRequest unassignPrivateIpAddressesRequest) {
ExecutionContext executionContext = createExecutionContext(unassignPrivateIpAddressesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new UnassignPrivateIpAddressesRequestMarshaller().marshall(unassignPrivateIpAddressesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Cancels an active conversion task. The task can be the import of an
* instance or volume. The action removes all artifacts of the
* conversion, including a partially uploaded volume or instance. If the
* conversion is complete or is in the process of transferring the final
* disk image, the command fails and returns an exception.
*
*
* For more information, see
* Using the Command Line Tools to Import Your Virtual Machine to Amazon EC2
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param cancelConversionTaskRequest Container for the necessary
* parameters to execute the CancelConversionTask service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void cancelConversionTask(CancelConversionTaskRequest cancelConversionTaskRequest) {
ExecutionContext executionContext = createExecutionContext(cancelConversionTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CancelConversionTaskRequestMarshaller().marshall(cancelConversionTaskRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Associates an Elastic IP address with an instance or a network
* interface.
*
*
* An Elastic IP address is for use in either the EC2-Classic platform or
* in a VPC. For more information, see
* Elastic IP Addresses
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* [EC2-Classic, default VPC] If the Elastic IP address is already
* associated with a different instance, it is disassociated from that
* instance and associated with the specified instance.
*
*
* [EC2-VPC] If you don't specify a private IP address, the Elastic IP
* address is associated with the primary IP address. If the Elastic IP
* address is already associated with a different instance or a network
* interface, you get an error unless you allow reassociation.
*
*
* This is an idempotent operation. If you perform the operation more
* than once, Amazon EC2 doesn't return an error.
*
*
* @param associateAddressRequest Container for the necessary parameters
* to execute the AssociateAddress service method on AmazonEC2.
*
* @return The response from the AssociateAddress service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public AssociateAddressResult associateAddress(AssociateAddressRequest associateAddressRequest) {
ExecutionContext executionContext = createExecutionContext(associateAddressRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new AssociateAddressRequestMarshaller().marshall(associateAddressRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new AssociateAddressResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified customer gateway. You must delete the VPN
* connection before you can delete the customer gateway.
*
*
* @param deleteCustomerGatewayRequest Container for the necessary
* parameters to execute the DeleteCustomerGateway service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteCustomerGateway(DeleteCustomerGatewayRequest deleteCustomerGatewayRequest) {
ExecutionContext executionContext = createExecutionContext(deleteCustomerGatewayRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteCustomerGatewayRequestMarshaller().marshall(deleteCustomerGatewayRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Creates an entry (a rule) in a network ACL with the specified rule
* number. Each network ACL has a set of numbered ingress rules and a
* separate set of numbered egress rules. When determining whether a
* packet should be allowed in or out of a subnet associated with the
* ACL, we process the entries in the ACL according to the rule numbers,
* in ascending order. Each network ACL has a set of ingress rules and a
* separate set of egress rules.
*
*
* We recommend that you leave room between the rule numbers (for
* example, 100, 110, 120, ...), and not number them one right after the
* other (for example, 101, 102, 103, ...). This makes it easier to add a
* rule between existing ones without having to renumber the rules.
*
*
* After you add an entry, you can't modify it; you must either replace
* it, or create an entry and delete the old one.
*
*
* For more information about network ACLs, see
* Network ACLs
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param createNetworkAclEntryRequest Container for the necessary
* parameters to execute the CreateNetworkAclEntry service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void createNetworkAclEntry(CreateNetworkAclEntryRequest createNetworkAclEntryRequest) {
ExecutionContext executionContext = createExecutionContext(createNetworkAclEntryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateNetworkAclEntryRequestMarshaller().marshall(createNetworkAclEntryRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Accept a VPC peering connection request. To accept a request, the VPC
* peering connection must be in the pending-acceptance
* state, and you must be the owner of the peer VPC. Use the
* DescribeVpcPeeringConnections
request to view your
* outstanding VPC peering connection requests.
*
*
* @param acceptVpcPeeringConnectionRequest Container for the necessary
* parameters to execute the AcceptVpcPeeringConnection service method on
* AmazonEC2.
*
* @return The response from the AcceptVpcPeeringConnection service
* method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public AcceptVpcPeeringConnectionResult acceptVpcPeeringConnection(AcceptVpcPeeringConnectionRequest acceptVpcPeeringConnectionRequest) {
ExecutionContext executionContext = createExecutionContext(acceptVpcPeeringConnectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new AcceptVpcPeeringConnectionRequestMarshaller().marshall(acceptVpcPeeringConnectionRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new AcceptVpcPeeringConnectionResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes one or more of your export tasks.
*
*
* @param describeExportTasksRequest Container for the necessary
* parameters to execute the DescribeExportTasks service method on
* AmazonEC2.
*
* @return The response from the DescribeExportTasks service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeExportTasksResult describeExportTasks(DescribeExportTasksRequest describeExportTasksRequest) {
ExecutionContext executionContext = createExecutionContext(describeExportTasksRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeExportTasksRequestMarshaller().marshall(describeExportTasksRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeExportTasksResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Detaches an Internet gateway from a VPC, disabling connectivity
* between the Internet and the VPC. The VPC must not contain any running
* instances with Elastic IP addresses.
*
*
* @param detachInternetGatewayRequest Container for the necessary
* parameters to execute the DetachInternetGateway service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void detachInternetGateway(DetachInternetGatewayRequest detachInternetGatewayRequest) {
ExecutionContext executionContext = createExecutionContext(detachInternetGatewayRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DetachInternetGatewayRequestMarshaller().marshall(detachInternetGatewayRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Requests a VPC peering connection between two VPCs: a requester VPC
* that you own and a peer VPC with which to create the connection. The
* peer VPC can belong to another AWS account. The requester VPC and peer
* VPC cannot have overlapping CIDR blocks.
*
*
* The owner of the peer VPC must accept the the peering request to
* activate the peering connection. The VPC peering connection request
* expires after 7 days, after which it cannot be accepted or rejected.
*
*
* A CreateVpcPeeringConnection
request between VPCs with
* overlapping CIDR blocks results in the VPC peering connection having a
* status of failed
.
*
*
* @param createVpcPeeringConnectionRequest Container for the necessary
* parameters to execute the CreateVpcPeeringConnection service method on
* AmazonEC2.
*
* @return The response from the CreateVpcPeeringConnection service
* method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateVpcPeeringConnectionResult createVpcPeeringConnection(CreateVpcPeeringConnectionRequest createVpcPeeringConnectionRequest) {
ExecutionContext executionContext = createExecutionContext(createVpcPeeringConnectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateVpcPeeringConnectionRequestMarshaller().marshall(createVpcPeeringConnectionRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateVpcPeeringConnectionResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a route table for the specified VPC. After you create a route
* table, you can add routes and associate the table with a subnet.
*
*
* For more information about route tables, see
* Route Tables
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param createRouteTableRequest Container for the necessary parameters
* to execute the CreateRouteTable service method on AmazonEC2.
*
* @return The response from the CreateRouteTable service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateRouteTableResult createRouteTable(CreateRouteTableRequest createRouteTableRequest) {
ExecutionContext executionContext = createExecutionContext(createRouteTableRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateRouteTableRequestMarshaller().marshall(createRouteTableRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateRouteTableResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the specified Amazon EBS volumes.
*
*
* For more information about Amazon EBS volumes, see
* Amazon EBS Volumes
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param describeVolumesRequest Container for the necessary parameters
* to execute the DescribeVolumes service method on AmazonEC2.
*
* @return The response from the DescribeVolumes service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeVolumesResult describeVolumes(DescribeVolumesRequest describeVolumesRequest) {
ExecutionContext executionContext = createExecutionContext(describeVolumesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeVolumesRequestMarshaller().marshall(describeVolumesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeVolumesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes your account's Reserved Instance listings in the Reserved
* Instance Marketplace.
*
*
* For more information, see
* Reserved Instance Marketplace
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param describeReservedInstancesListingsRequest Container for the
* necessary parameters to execute the DescribeReservedInstancesListings
* service method on AmazonEC2.
*
* @return The response from the DescribeReservedInstancesListings
* service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeReservedInstancesListingsResult describeReservedInstancesListings(DescribeReservedInstancesListingsRequest describeReservedInstancesListingsRequest) {
ExecutionContext executionContext = createExecutionContext(describeReservedInstancesListingsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeReservedInstancesListingsRequestMarshaller().marshall(describeReservedInstancesListingsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeReservedInstancesListingsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Submits feedback about the status of an instance. The instance must be
* in the running
state. If your experience with the
* instance differs from the instance status returned by
* DescribeInstanceStatus, use ReportInstanceStatus to report your
* experience with the instance. Amazon EC2 collects this information to
* improve the accuracy of status checks.
*
*
* @param reportInstanceStatusRequest Container for the necessary
* parameters to execute the ReportInstanceStatus service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void reportInstanceStatus(ReportInstanceStatusRequest reportInstanceStatusRequest) {
ExecutionContext executionContext = createExecutionContext(reportInstanceStatusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ReportInstanceStatusRequestMarshaller().marshall(reportInstanceStatusRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Describes one or more of your route tables.
*
*
* For more information about route tables, see
* Route Tables
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param describeRouteTablesRequest Container for the necessary
* parameters to execute the DescribeRouteTables service method on
* AmazonEC2.
*
* @return The response from the DescribeRouteTables service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeRouteTablesResult describeRouteTables(DescribeRouteTablesRequest describeRouteTablesRequest) {
ExecutionContext executionContext = createExecutionContext(describeRouteTablesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeRouteTablesRequestMarshaller().marshall(describeRouteTablesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeRouteTablesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes one or more of your DHCP options sets.
*
*
* For more information about DHCP options sets, see
* DHCP Options Sets
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param describeDhcpOptionsRequest Container for the necessary
* parameters to execute the DescribeDhcpOptions service method on
* AmazonEC2.
*
* @return The response from the DescribeDhcpOptions service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeDhcpOptionsResult describeDhcpOptions(DescribeDhcpOptionsRequest describeDhcpOptionsRequest) {
ExecutionContext executionContext = createExecutionContext(describeDhcpOptionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeDhcpOptionsRequestMarshaller().marshall(describeDhcpOptionsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeDhcpOptionsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Enables monitoring for a running instance. For more information about
* monitoring instances, see
* Monitoring Your Instances and Volumes
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param monitorInstancesRequest Container for the necessary parameters
* to execute the MonitorInstances service method on AmazonEC2.
*
* @return The response from the MonitorInstances service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public MonitorInstancesResult monitorInstances(MonitorInstancesRequest monitorInstancesRequest) {
ExecutionContext executionContext = createExecutionContext(monitorInstancesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new MonitorInstancesRequestMarshaller().marshall(monitorInstancesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new MonitorInstancesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes one or more of your network ACLs.
*
*
* For more information about network ACLs, see
* Network ACLs
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param describeNetworkAclsRequest Container for the necessary
* parameters to execute the DescribeNetworkAcls service method on
* AmazonEC2.
*
* @return The response from the DescribeNetworkAcls service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeNetworkAclsResult describeNetworkAcls(DescribeNetworkAclsRequest describeNetworkAclsRequest) {
ExecutionContext executionContext = createExecutionContext(describeNetworkAclsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeNetworkAclsRequestMarshaller().marshall(describeNetworkAclsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeNetworkAclsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes one or more of your bundling tasks.
*
*
* NOTE: Completed bundle tasks are listed for only a limited
* time. If your bundle task is no longer in the list, you can still
* register an AMI from it. Just use RegisterImage with the Amazon S3
* bucket name and image manifest name you provided to the bundle task.
*
*
* @param describeBundleTasksRequest Container for the necessary
* parameters to execute the DescribeBundleTasks service method on
* AmazonEC2.
*
* @return The response from the DescribeBundleTasks service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeBundleTasksResult describeBundleTasks(DescribeBundleTasksRequest describeBundleTasksRequest) {
ExecutionContext executionContext = createExecutionContext(describeBundleTasksRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeBundleTasksRequestMarshaller().marshall(describeBundleTasksRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeBundleTasksResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an import instance task using metadata from the specified disk
* image. After importing the image, you then upload it using the
* ec2-upload-disk-image command in the EC2 command line tools. For more
* information, see Using the Command Line Tools to Import Your Virtual
* Machine to Amazon EC2 in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param importInstanceRequest Container for the necessary parameters to
* execute the ImportInstance service method on AmazonEC2.
*
* @return The response from the ImportInstance service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public ImportInstanceResult importInstance(ImportInstanceRequest importInstanceRequest) {
ExecutionContext executionContext = createExecutionContext(importInstanceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ImportInstanceRequestMarshaller().marshall(importInstanceRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ImportInstanceResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes one or more ingress rules from a security group. The values
* that you specify in the revoke request (for example, ports) must match
* the existing rule's values for the rule to be removed.
*
*
* Each rule consists of the protocol and the CIDR range or source
* security group. For the TCP and UDP protocols, you must also specify
* the destination port or range of ports. For the ICMP protocol, you
* must also specify the ICMP type and code.
*
*
* Rule changes are propagated to instances within the security group as
* quickly as possible. However, a small delay might occur.
*
*
* @param revokeSecurityGroupIngressRequest Container for the necessary
* parameters to execute the RevokeSecurityGroupIngress service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void revokeSecurityGroupIngress(RevokeSecurityGroupIngressRequest revokeSecurityGroupIngressRequest) {
ExecutionContext executionContext = createExecutionContext(revokeSecurityGroupIngressRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new RevokeSecurityGroupIngressRequestMarshaller().marshall(revokeSecurityGroupIngressRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Deletes a VPC peering connection. Either the owner of the requester
* VPC or the owner of the peer VPC can delete the VPC peering connection
* if it's in the active
state. The owner of the requester
* VPC can delete a VPC peering connection in the
* pending-acceptance
state.
*
*
* @param deleteVpcPeeringConnectionRequest Container for the necessary
* parameters to execute the DeleteVpcPeeringConnection service method on
* AmazonEC2.
*
* @return The response from the DeleteVpcPeeringConnection service
* method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DeleteVpcPeeringConnectionResult deleteVpcPeeringConnection(DeleteVpcPeeringConnectionRequest deleteVpcPeeringConnectionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVpcPeeringConnectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteVpcPeeringConnectionRequestMarshaller().marshall(deleteVpcPeeringConnectionRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DeleteVpcPeeringConnectionResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the console output for the specified instance.
*
*
* Instances do not have a physical monitor through which you can view
* their console output. They also lack physical controls that allow you
* to power up, reboot, or shut them down. To allow these actions, we
* provide them through the Amazon EC2 API and command line interface.
*
*
* Instance console output is buffered and posted shortly after instance
* boot, reboot, and termination. Amazon EC2 preserves the most recent 64
* KB output which is available for at least one hour after the most
* recent post.
*
*
* For Linux/Unix instances, the instance console output displays the
* exact console output that would normally be displayed on a physical
* monitor attached to a machine. This output is buffered because the
* instance produces it and then posts it to a store where the instance's
* owner can retrieve it.
*
*
* For Windows instances, the instance console output displays the last
* three system event log errors.
*
*
* @param getConsoleOutputRequest Container for the necessary parameters
* to execute the GetConsoleOutput service method on AmazonEC2.
*
* @return The response from the GetConsoleOutput service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public GetConsoleOutputResult getConsoleOutput(GetConsoleOutputRequest getConsoleOutputRequest) {
ExecutionContext executionContext = createExecutionContext(getConsoleOutputRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new GetConsoleOutputRequestMarshaller().marshall(getConsoleOutputRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new GetConsoleOutputResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Internet gateway for use with a VPC. After creating the
* Internet gateway, you attach it to a VPC using AttachInternetGateway.
*
*
* For more information about your VPC and Internet gateway, see the
* Amazon Virtual Private Cloud User Guide
* .
*
*
* @param createInternetGatewayRequest Container for the necessary
* parameters to execute the CreateInternetGateway service method on
* AmazonEC2.
*
* @return The response from the CreateInternetGateway service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateInternetGatewayResult createInternetGateway(CreateInternetGatewayRequest createInternetGatewayRequest) {
ExecutionContext executionContext = createExecutionContext(createInternetGatewayRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateInternetGatewayRequestMarshaller().marshall(createInternetGatewayRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateInternetGatewayResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified static route associated with a VPN connection
* between an existing virtual private gateway and a VPN customer
* gateway. The static route allows traffic to be routed from the virtual
* private gateway to the VPN customer gateway.
*
*
* @param deleteVpnConnectionRouteRequest Container for the necessary
* parameters to execute the DeleteVpnConnectionRoute service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteVpnConnectionRoute(DeleteVpnConnectionRouteRequest deleteVpnConnectionRouteRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVpnConnectionRouteRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteVpnConnectionRouteRequestMarshaller().marshall(deleteVpnConnectionRouteRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Detaches a network interface from an instance.
*
*
* @param detachNetworkInterfaceRequest Container for the necessary
* parameters to execute the DetachNetworkInterface service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void detachNetworkInterface(DetachNetworkInterfaceRequest detachNetworkInterfaceRequest) {
ExecutionContext executionContext = createExecutionContext(detachNetworkInterfaceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DetachNetworkInterfaceRequestMarshaller().marshall(detachNetworkInterfaceRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Modifies the specified attribute of the specified AMI. You can specify
* only one attribute at a time.
*
*
* NOTE: AWS Marketplace product codes cannot be modified. Images
* with an AWS Marketplace product code cannot be made public.
*
*
* @param modifyImageAttributeRequest Container for the necessary
* parameters to execute the ModifyImageAttribute service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void modifyImageAttribute(ModifyImageAttributeRequest modifyImageAttributeRequest) {
ExecutionContext executionContext = createExecutionContext(modifyImageAttributeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ModifyImageAttributeRequestMarshaller().marshall(modifyImageAttributeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Provides information to AWS about your VPN customer gateway device.
* The customer gateway is the appliance at your end of the VPN
* connection. (The device on the AWS side of the VPN connection is the
* virtual private gateway.) You must provide the Internet-routable IP
* address of the customer gateway's external interface. The IP address
* must be static and can't be behind a device performing network address
* translation (NAT).
*
*
* For devices that use Border Gateway Protocol (BGP), you can also
* provide the device's BGP Autonomous System Number (ASN). You can use
* an existing ASN assigned to your network. If you don't have an ASN
* already, you can use a private ASN (in the 64512 - 65534 range).
*
*
* NOTE: Amazon EC2 supports all 2-byte ASN numbers in the range
* of 1 - 65534, with the exception of 7224, which is reserved in the
* us-east-1 region, and 9059, which is reserved in the eu-west-1 region.
*
*
* For more information about VPN customer gateways, see
* Adding a Hardware Virtual Private Gateway to Your VPC
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param createCustomerGatewayRequest Container for the necessary
* parameters to execute the CreateCustomerGateway service method on
* AmazonEC2.
*
* @return The response from the CreateCustomerGateway service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateCustomerGatewayResult createCustomerGateway(CreateCustomerGatewayRequest createCustomerGatewayRequest) {
ExecutionContext executionContext = createExecutionContext(createCustomerGatewayRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateCustomerGatewayRequestMarshaller().marshall(createCustomerGatewayRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateCustomerGatewayResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a datafeed for Spot Instances, enabling you to view Spot
* Instance usage logs. You can create one data feed per AWS account. For
* more information, see
* Spot Instances
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param createSpotDatafeedSubscriptionRequest Container for the
* necessary parameters to execute the CreateSpotDatafeedSubscription
* service method on AmazonEC2.
*
* @return The response from the CreateSpotDatafeedSubscription service
* method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateSpotDatafeedSubscriptionResult createSpotDatafeedSubscription(CreateSpotDatafeedSubscriptionRequest createSpotDatafeedSubscriptionRequest) {
ExecutionContext executionContext = createExecutionContext(createSpotDatafeedSubscriptionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateSpotDatafeedSubscriptionRequestMarshaller().marshall(createSpotDatafeedSubscriptionRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateSpotDatafeedSubscriptionResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Attaches an Internet gateway to a VPC, enabling connectivity between
* the Internet and the VPC. For more information about your VPC and
* Internet gateway, see the
* Amazon Virtual Private Cloud User Guide
* .
*
*
* @param attachInternetGatewayRequest Container for the necessary
* parameters to execute the AttachInternetGateway service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void attachInternetGateway(AttachInternetGatewayRequest attachInternetGatewayRequest) {
ExecutionContext executionContext = createExecutionContext(attachInternetGatewayRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new AttachInternetGatewayRequestMarshaller().marshall(attachInternetGatewayRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Deletes the specified VPN connection.
*
*
* If you're deleting the VPC and its associated components, we recommend
* that you detach the virtual private gateway from the VPC and delete
* the VPC before deleting the VPN connection.
*
*
* @param deleteVpnConnectionRequest Container for the necessary
* parameters to execute the DeleteVpnConnection service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteVpnConnection(DeleteVpnConnectionRequest deleteVpnConnectionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVpnConnectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteVpnConnectionRequestMarshaller().marshall(deleteVpnConnectionRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Describes one or more of your conversion tasks. For more information,
* see
* Using the Command Line Tools to Import Your Virtual Machine to Amazon EC2
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param describeConversionTasksRequest Container for the necessary
* parameters to execute the DescribeConversionTasks service method on
* AmazonEC2.
*
* @return The response from the DescribeConversionTasks service method,
* as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeConversionTasksResult describeConversionTasks(DescribeConversionTasksRequest describeConversionTasksRequest) {
ExecutionContext executionContext = createExecutionContext(describeConversionTasksRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeConversionTasksRequestMarshaller().marshall(describeConversionTasksRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeConversionTasksResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a VPN connection between an existing virtual private gateway
* and a VPN customer gateway. The only supported connection type is
* ipsec.1
.
*
*
* The response includes information that you need to give to your
* network administrator to configure your customer gateway.
*
*
* IMPORTANT: We strongly recommend that you use HTTPS when
* calling this operation because the response contains sensitive
* cryptographic information for configuring your customer gateway.
*
*
* If you decide to shut down your VPN connection for any reason and
* later create a new VPN connection, you must reconfigure your customer
* gateway with the new information returned from this call.
*
*
* For more information about VPN connections, see
* Adding a Hardware Virtual Private Gateway to Your VPC
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param createVpnConnectionRequest Container for the necessary
* parameters to execute the CreateVpnConnection service method on
* AmazonEC2.
*
* @return The response from the CreateVpnConnection service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateVpnConnectionResult createVpnConnection(CreateVpnConnectionRequest createVpnConnectionRequest) {
ExecutionContext executionContext = createExecutionContext(createVpnConnectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateVpnConnectionRequestMarshaller().marshall(createVpnConnectionRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateVpnConnectionResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the specified attribute of the specified instance. You can
* specify only one attribute at a time.
*
*
* @param describeInstanceAttributeRequest Container for the necessary
* parameters to execute the DescribeInstanceAttribute service method on
* AmazonEC2.
*
* @return The response from the DescribeInstanceAttribute service
* method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeInstanceAttributeResult describeInstanceAttribute(DescribeInstanceAttributeRequest describeInstanceAttributeRequest) {
ExecutionContext executionContext = createExecutionContext(describeInstanceAttributeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeInstanceAttributeRequestMarshaller().marshall(describeInstanceAttributeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeInstanceAttributeResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes one or more of your VPC peering connections.
*
*
* @param describeVpcPeeringConnectionsRequest Container for the
* necessary parameters to execute the DescribeVpcPeeringConnections
* service method on AmazonEC2.
*
* @return The response from the DescribeVpcPeeringConnections service
* method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeVpcPeeringConnectionsResult describeVpcPeeringConnections(DescribeVpcPeeringConnectionsRequest describeVpcPeeringConnectionsRequest) {
ExecutionContext executionContext = createExecutionContext(describeVpcPeeringConnectionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeVpcPeeringConnectionsRequestMarshaller().marshall(describeVpcPeeringConnectionsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeVpcPeeringConnectionsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes one or more of your subnets.
*
*
* For more information about subnets, see
* Your VPC and Subnets
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param describeSubnetsRequest Container for the necessary parameters
* to execute the DescribeSubnets service method on AmazonEC2.
*
* @return The response from the DescribeSubnets service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeSubnetsResult describeSubnets(DescribeSubnetsRequest describeSubnetsRequest) {
ExecutionContext executionContext = createExecutionContext(describeSubnetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeSubnetsRequestMarshaller().marshall(describeSubnetsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeSubnetsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Launches the specified number of instances using an AMI for which you
* have permissions.
*
*
* When you launch an instance, it enters the pending
state.
* After the instance is ready for you, it enters the
* running
state. To check the state of your instance, call
* DescribeInstances.
*
*
* If you don't specify a security group when launching an instance,
* Amazon EC2 uses the default security group. For more information, see
* Security Groups
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* Linux instances have access to the public key of the key pair at boot.
* You can use this key to provide secure access to the instance. Amazon
* EC2 public images use this feature to provide secure access without
* passwords. For more information, see
* Key Pairs
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* You can provide optional user data when launching an instance. For
* more information, see
* Instance Metadata
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* If any of the AMIs have a product code attached for which the user has
* not subscribed, RunInstances
fails.
*
*
* @param runInstancesRequest Container for the necessary parameters to
* execute the RunInstances service method on AmazonEC2.
*
* @return The response from the RunInstances service method, as returned
* by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public RunInstancesResult runInstances(RunInstancesRequest runInstancesRequest) {
ExecutionContext executionContext = createExecutionContext(runInstancesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new RunInstancesRequestMarshaller().marshall(runInstancesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new RunInstancesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes one or more of your placement groups. For more information
* about placement groups and cluster instances, see
* Cluster Instances
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param describePlacementGroupsRequest Container for the necessary
* parameters to execute the DescribePlacementGroups service method on
* AmazonEC2.
*
* @return The response from the DescribePlacementGroups service method,
* as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribePlacementGroupsResult describePlacementGroups(DescribePlacementGroupsRequest describePlacementGroupsRequest) {
ExecutionContext executionContext = createExecutionContext(describePlacementGroupsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribePlacementGroupsRequestMarshaller().marshall(describePlacementGroupsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribePlacementGroupsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates a subnet with a route table. The subnet and route table
* must be in the same VPC. This association causes traffic originating
* from the subnet to be routed according to the routes in the route
* table. The action returns an association ID, which you need in order
* to disassociate the route table from the subnet later. A route table
* can be associated with multiple subnets.
*
*
* For more information about route tables, see
* Route Tables
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param associateRouteTableRequest Container for the necessary
* parameters to execute the AssociateRouteTable service method on
* AmazonEC2.
*
* @return The response from the AssociateRouteTable service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public AssociateRouteTableResult associateRouteTable(AssociateRouteTableRequest associateRouteTableRequest) {
ExecutionContext executionContext = createExecutionContext(associateRouteTableRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new AssociateRouteTableRequestMarshaller().marshall(associateRouteTableRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new AssociateRouteTableResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes one or more of your instances.
*
*
* If you specify one or more instance IDs, Amazon EC2 returns
* information for those instances. If you do not specify instance IDs,
* Amazon EC2 returns information for all relevant instances. If you
* specify an instance ID that is not valid, an error is returned. If you
* specify an instance that you do not own, it is not included in the
* returned results.
*
*
* Recently terminated instances might appear in the returned results.
* This interval is usually less than one hour.
*
*
* @param describeInstancesRequest Container for the necessary parameters
* to execute the DescribeInstances service method on AmazonEC2.
*
* @return The response from the DescribeInstances service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeInstancesResult describeInstances(DescribeInstancesRequest describeInstancesRequest) {
ExecutionContext executionContext = createExecutionContext(describeInstancesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeInstancesRequestMarshaller().marshall(describeInstancesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeInstancesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified network ACL. You can't delete the ACL if it's
* associated with any subnets. You can't delete the default network ACL.
*
*
* @param deleteNetworkAclRequest Container for the necessary parameters
* to execute the DeleteNetworkAcl service method on AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteNetworkAcl(DeleteNetworkAclRequest deleteNetworkAclRequest) {
ExecutionContext executionContext = createExecutionContext(deleteNetworkAclRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteNetworkAclRequestMarshaller().marshall(deleteNetworkAclRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Modifies a volume attribute.
*
*
* By default, all I/O operations for the volume are suspended when the
* data on the volume is determined to be potentially inconsistent, to
* prevent undetectable, latent data corruption. The I/O access to the
* volume can be resumed by first enabling I/O access and then checking
* the data consistency on your volume.
*
*
* You can change the default behavior to resume I/O operations. We
* recommend that you change this only for boot volumes or for volumes
* that are stateless or disposable.
*
*
* @param modifyVolumeAttributeRequest Container for the necessary
* parameters to execute the ModifyVolumeAttribute service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void modifyVolumeAttribute(ModifyVolumeAttributeRequest modifyVolumeAttributeRequest) {
ExecutionContext executionContext = createExecutionContext(modifyVolumeAttributeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ModifyVolumeAttributeRequestMarshaller().marshall(modifyVolumeAttributeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Describes one or more of the images (AMIs, AKIs, and ARIs) available
* to you. Images available to you include public images, private images
* that you own, and private images owned by other AWS accounts but for
* which you have explicit launch permissions.
*
*
* NOTE: Deregistered images are included in the returned results
* for an unspecified interval after deregistration.
*
*
* @param describeImagesRequest Container for the necessary parameters to
* execute the DescribeImages service method on AmazonEC2.
*
* @return The response from the DescribeImages service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeImagesResult describeImages(DescribeImagesRequest describeImagesRequest) {
ExecutionContext executionContext = createExecutionContext(describeImagesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeImagesRequestMarshaller().marshall(describeImagesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeImagesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Starts an Amazon EBS-backed AMI that you've previously stopped.
*
*
* Instances that use Amazon EBS volumes as their root devices can be
* quickly stopped and started. When an instance is stopped, the compute
* resources are released and you are not billed for hourly instance
* usage. However, your root partition Amazon EBS volume remains,
* continues to persist your data, and you are charged for Amazon EBS
* volume usage. You can restart your instance at any time. Each time you
* transition an instance from stopped to started, Amazon EC2 charges a
* full instance hour, even if transitions happen multiple times within a
* single hour.
*
*
* Before stopping an instance, make sure it is in a state from which it
* can be restarted. Stopping an instance does not preserve data stored
* in RAM.
*
*
* Performing this operation on an instance that uses an instance store
* as its root device returns an error.
*
*
* For more information, see
* Stopping Instances
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param startInstancesRequest Container for the necessary parameters to
* execute the StartInstances service method on AmazonEC2.
*
* @return The response from the StartInstances service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public StartInstancesResult startInstances(StartInstancesRequest startInstancesRequest) {
ExecutionContext executionContext = createExecutionContext(startInstancesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new StartInstancesRequestMarshaller().marshall(startInstancesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new StartInstancesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Cancels the specified Reserved Instance listing in the Reserved
* Instance Marketplace.
*
*
* For more information, see
* Reserved Instance Marketplace
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param cancelReservedInstancesListingRequest Container for the
* necessary parameters to execute the CancelReservedInstancesListing
* service method on AmazonEC2.
*
* @return The response from the CancelReservedInstancesListing service
* method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public CancelReservedInstancesListingResult cancelReservedInstancesListing(CancelReservedInstancesListingRequest cancelReservedInstancesListingRequest) {
ExecutionContext executionContext = createExecutionContext(cancelReservedInstancesListingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CancelReservedInstancesListingRequestMarshaller().marshall(cancelReservedInstancesListingRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CancelReservedInstancesListingResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Modifies the specified attribute of the specified instance. You can
* specify only one attribute at a time.
*
*
* To modify some attributes, the instance must be stopped. For more
* information, see
* Modifying Attributes of a Stopped Instance
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param modifyInstanceAttributeRequest Container for the necessary
* parameters to execute the ModifyInstanceAttribute service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void modifyInstanceAttribute(ModifyInstanceAttributeRequest modifyInstanceAttributeRequest) {
ExecutionContext executionContext = createExecutionContext(modifyInstanceAttributeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ModifyInstanceAttributeRequestMarshaller().marshall(modifyInstanceAttributeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Deletes the specified set of DHCP options. You must disassociate the
* set of DHCP options before you can delete it. You can disassociate the
* set of DHCP options by associating either a new set of options or the
* default set of options with the VPC.
*
*
* @param deleteDhcpOptionsRequest Container for the necessary parameters
* to execute the DeleteDhcpOptions service method on AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteDhcpOptions(DeleteDhcpOptionsRequest deleteDhcpOptionsRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDhcpOptionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteDhcpOptionsRequestMarshaller().marshall(deleteDhcpOptionsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Adds one or more ingress rules to a security group.
*
*
* IMPORTANT: EC2-Classic: You can have up to 100 rules per group.
* EC2-VPC: You can have up to 50 rules per group (covering both ingress
* and egress rules).
*
*
* Rule changes are propagated to instances within the security group as
* quickly as possible. However, a small delay might occur.
*
*
* [EC2-Classic] This action gives one or more CIDR IP address ranges
* permission to access a security group in your account, or gives one or
* more security groups (called the source groups ) permission to
* access a security group for your account. A source group can be for
* your own AWS account, or another.
*
*
* [EC2-VPC] This action gives one or more CIDR IP address ranges
* permission to access a security group in your VPC, or gives one or
* more other security groups (called the source groups )
* permission to access a security group for your VPC. The security
* groups must all be for the same VPC.
*
*
* @param authorizeSecurityGroupIngressRequest Container for the
* necessary parameters to execute the AuthorizeSecurityGroupIngress
* service method on AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void authorizeSecurityGroupIngress(AuthorizeSecurityGroupIngressRequest authorizeSecurityGroupIngressRequest) {
ExecutionContext executionContext = createExecutionContext(authorizeSecurityGroupIngressRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new AuthorizeSecurityGroupIngressRequestMarshaller().marshall(authorizeSecurityGroupIngressRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Describes the Spot Instance requests that belong to your account. Spot
* Instances are instances that Amazon EC2 starts on your behalf when the
* maximum price that you specify exceeds the current Spot Price. Amazon
* EC2 periodically sets the Spot Price based on available Spot Instance
* capacity and current Spot Instance requests. For more information
* about Spot Instances, see
* Spot Instances
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* You can use DescribeSpotInstanceRequests
to find a
* running Spot Instance by examining the response. If the status of the
* Spot Instance is fulfilled
, the instance ID appears in
* the response and contains the identifier of the instance.
* Alternatively, you can use DescribeInstances with a filter to look for
* instances where the instance lifecycle is spot
.
*
*
* @param describeSpotInstanceRequestsRequest Container for the necessary
* parameters to execute the DescribeSpotInstanceRequests service method
* on AmazonEC2.
*
* @return The response from the DescribeSpotInstanceRequests service
* method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeSpotInstanceRequestsResult describeSpotInstanceRequests(DescribeSpotInstanceRequestsRequest describeSpotInstanceRequestsRequest) {
ExecutionContext executionContext = createExecutionContext(describeSpotInstanceRequestsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeSpotInstanceRequestsRequestMarshaller().marshall(describeSpotInstanceRequestsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeSpotInstanceRequestsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a VPC with the specified CIDR block.
*
*
* The smallest VPC you can create uses a /28 netmask (16 IP addresses),
* and the largest uses a /16 netmask (65,536 IP addresses). To help you
* decide how big to make your VPC, see
* Your VPC and Subnets
* in the Amazon Virtual Private Cloud User Guide .
*
*
* By default, each instance you launch in the VPC has the default DHCP
* options, which includes only a default DNS server that we provide
* (AmazonProvidedDNS). For more information about DHCP options, see
* DHCP Options Sets
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param createVpcRequest Container for the necessary parameters to
* execute the CreateVpc service method on AmazonEC2.
*
* @return The response from the CreateVpc service method, as returned by
* AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateVpcResult createVpc(CreateVpcRequest createVpcRequest) {
ExecutionContext executionContext = createExecutionContext(createVpcRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateVpcRequestMarshaller().marshall(createVpcRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateVpcResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes one or more of your VPN customer gateways.
*
*
* For more information about VPN customer gateways, see
* Adding a Hardware Virtual Private Gateway to Your VPC
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param describeCustomerGatewaysRequest Container for the necessary
* parameters to execute the DescribeCustomerGateways service method on
* AmazonEC2.
*
* @return The response from the DescribeCustomerGateways service method,
* as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeCustomerGatewaysResult describeCustomerGateways(DescribeCustomerGatewaysRequest describeCustomerGatewaysRequest) {
ExecutionContext executionContext = createExecutionContext(describeCustomerGatewaysRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeCustomerGatewaysRequestMarshaller().marshall(describeCustomerGatewaysRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeCustomerGatewaysResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Cancels an active export task. The request removes all artifacts of
* the export, including any partially-created Amazon S3 objects. If the
* export task is complete or is in the process of transferring the final
* disk image, the command fails and returns an error.
*
*
* @param cancelExportTaskRequest Container for the necessary parameters
* to execute the CancelExportTask service method on AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void cancelExportTask(CancelExportTaskRequest cancelExportTaskRequest) {
ExecutionContext executionContext = createExecutionContext(cancelExportTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CancelExportTaskRequestMarshaller().marshall(cancelExportTaskRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Creates a route in a route table within a VPC.
*
*
* You must specify one of the following targets: Internet gateway, NAT
* instance, VPC peering connection, or network interface.
*
*
* When determining how to route traffic, we use the route with the most
* specific match. For example, let's say the traffic is destined for
* 192.0.2.3
, and the route table includes the following
* two routes:
*
*
*
* -
* 192.0.2.0/24
(goes to some target A)
*
*
* -
* 192.0.2.0/28
(goes to some target B)
*
*
*
*
*
* Both routes apply to the traffic destined for 192.0.2.3
.
* However, the second route in the list covers a smaller number of IP
* addresses and is therefore more specific, so we use that route to
* determine where to target the traffic.
*
*
* For more information about route tables, see
* Route Tables
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param createRouteRequest Container for the necessary parameters to
* execute the CreateRoute service method on AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void createRoute(CreateRouteRequest createRouteRequest) {
ExecutionContext executionContext = createExecutionContext(createRouteRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateRouteRequestMarshaller().marshall(createRouteRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Initiates the copy of an AMI from the specified source region to the
* region in which the request was made.
*
*
* For more information, see
* Copying AMIs
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param copyImageRequest Container for the necessary parameters to
* execute the CopyImage service method on AmazonEC2.
*
* @return The response from the CopyImage service method, as returned by
* AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public CopyImageResult copyImage(CopyImageRequest copyImageRequest) {
ExecutionContext executionContext = createExecutionContext(copyImageRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CopyImageRequestMarshaller().marshall(copyImageRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CopyImageResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Modifies the specified network interface attribute. You can specify
* only one attribute at a time.
*
*
* @param modifyNetworkInterfaceAttributeRequest Container for the
* necessary parameters to execute the ModifyNetworkInterfaceAttribute
* service method on AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void modifyNetworkInterfaceAttribute(ModifyNetworkInterfaceAttributeRequest modifyNetworkInterfaceAttributeRequest) {
ExecutionContext executionContext = createExecutionContext(modifyNetworkInterfaceAttributeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ModifyNetworkInterfaceAttributeRequestMarshaller().marshall(modifyNetworkInterfaceAttributeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Deletes the specified route table. You must disassociate the route
* table from any subnets before you can delete it. You can't delete the
* main route table.
*
*
* @param deleteRouteTableRequest Container for the necessary parameters
* to execute the DeleteRouteTable service method on AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteRouteTable(DeleteRouteTableRequest deleteRouteTableRequest) {
ExecutionContext executionContext = createExecutionContext(deleteRouteTableRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteRouteTableRequestMarshaller().marshall(deleteRouteTableRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Describes a network interface attribute. You can specify only one
* attribute at a time.
*
*
* @param describeNetworkInterfaceAttributeRequest Container for the
* necessary parameters to execute the DescribeNetworkInterfaceAttribute
* service method on AmazonEC2.
*
* @return The response from the DescribeNetworkInterfaceAttribute
* service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeNetworkInterfaceAttributeResult describeNetworkInterfaceAttribute(DescribeNetworkInterfaceAttributeRequest describeNetworkInterfaceAttributeRequest) {
ExecutionContext executionContext = createExecutionContext(describeNetworkInterfaceAttributeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeNetworkInterfaceAttributeRequestMarshaller().marshall(describeNetworkInterfaceAttributeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeNetworkInterfaceAttributeResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a Spot Instance request. Spot Instances are instances that
* Amazon EC2 starts on your behalf when the maximum price that you
* specify exceeds the current Spot Price. Amazon EC2 periodically sets
* the Spot Price based on available Spot Instance capacity and current
* Spot Instance requests. For more information about Spot Instances, see
* Spot Instances
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param requestSpotInstancesRequest Container for the necessary
* parameters to execute the RequestSpotInstances service method on
* AmazonEC2.
*
* @return The response from the RequestSpotInstances service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public RequestSpotInstancesResult requestSpotInstances(RequestSpotInstancesRequest requestSpotInstancesRequest) {
ExecutionContext executionContext = createExecutionContext(requestSpotInstancesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new RequestSpotInstancesRequestMarshaller().marshall(requestSpotInstancesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new RequestSpotInstancesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds or overwrites one or more tags for the specified EC2 resource or
* resources. Each resource can have a maximum of 10 tags. Each tag
* consists of a key and optional value. Tag keys must be unique per
* resource.
*
*
* For more information about tags, see
* Tagging Your Resources
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param createTagsRequest Container for the necessary parameters to
* execute the CreateTags service method on AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void createTags(CreateTagsRequest createTagsRequest) {
ExecutionContext executionContext = createExecutionContext(createTagsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateTagsRequestMarshaller().marshall(createTagsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Describes the specified attribute of the specified volume. You can
* specify only one attribute at a time.
*
*
* For more information about Amazon EBS volumes, see
* Amazon EBS Volumes
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param describeVolumeAttributeRequest Container for the necessary
* parameters to execute the DescribeVolumeAttribute service method on
* AmazonEC2.
*
* @return The response from the DescribeVolumeAttribute service method,
* as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeVolumeAttributeResult describeVolumeAttribute(DescribeVolumeAttributeRequest describeVolumeAttributeRequest) {
ExecutionContext executionContext = createExecutionContext(describeVolumeAttributeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeVolumeAttributeRequestMarshaller().marshall(describeVolumeAttributeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeVolumeAttributeResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Attaches a network interface to an instance.
*
*
* @param attachNetworkInterfaceRequest Container for the necessary
* parameters to execute the AttachNetworkInterface service method on
* AmazonEC2.
*
* @return The response from the AttachNetworkInterface service method,
* as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public AttachNetworkInterfaceResult attachNetworkInterface(AttachNetworkInterfaceRequest attachNetworkInterfaceRequest) {
ExecutionContext executionContext = createExecutionContext(attachNetworkInterfaceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new AttachNetworkInterfaceRequestMarshaller().marshall(attachNetworkInterfaceRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new AttachNetworkInterfaceResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Replaces an existing route within a route table in a VPC. You must
* provide only one of the following: Internet gateway, NAT instance, VPC
* peering connection, or network interface.
*
*
* For more information about route tables, see
* Route Tables
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param replaceRouteRequest Container for the necessary parameters to
* execute the ReplaceRoute service method on AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void replaceRoute(ReplaceRouteRequest replaceRouteRequest) {
ExecutionContext executionContext = createExecutionContext(replaceRouteRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ReplaceRouteRequestMarshaller().marshall(replaceRouteRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Describes one or more of the tags for your EC2 resources.
*
*
* For more information about tags, see
* Tagging Your Resources
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param describeTagsRequest Container for the necessary parameters to
* execute the DescribeTags service method on AmazonEC2.
*
* @return The response from the DescribeTags service method, as returned
* by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeTagsResult describeTags(DescribeTagsRequest describeTagsRequest) {
ExecutionContext executionContext = createExecutionContext(describeTagsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeTagsRequestMarshaller().marshall(describeTagsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeTagsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Cancels a bundling operation for an instance store-backed Windows
* instance.
*
*
* @param cancelBundleTaskRequest Container for the necessary parameters
* to execute the CancelBundleTask service method on AmazonEC2.
*
* @return The response from the CancelBundleTask service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public CancelBundleTaskResult cancelBundleTask(CancelBundleTaskRequest cancelBundleTaskRequest) {
ExecutionContext executionContext = createExecutionContext(cancelBundleTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CancelBundleTaskRequestMarshaller().marshall(cancelBundleTaskRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CancelBundleTaskResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disables a virtual private gateway (VGW) from propagating routes to
* the routing tables of a VPC.
*
*
* @param disableVgwRoutePropagationRequest Container for the necessary
* parameters to execute the DisableVgwRoutePropagation service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void disableVgwRoutePropagation(DisableVgwRoutePropagationRequest disableVgwRoutePropagationRequest) {
ExecutionContext executionContext = createExecutionContext(disableVgwRoutePropagationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DisableVgwRoutePropagationRequestMarshaller().marshall(disableVgwRoutePropagationRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Cancels one or more Spot Instance requests. Spot Instances are
* instances that Amazon EC2 starts on your behalf when the maximum price
* that you specify exceeds the current Spot Price. Amazon EC2
* periodically sets the Spot Price based on available Spot Instance
* capacity and current Spot Instance requests. For more information
* about Spot Instances, see
* Spot Instances
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* IMPORTANT: Canceling a Spot Instance request does not terminate
* running Spot Instances associated with the request.
*
*
* @param cancelSpotInstanceRequestsRequest Container for the necessary
* parameters to execute the CancelSpotInstanceRequests service method on
* AmazonEC2.
*
* @return The response from the CancelSpotInstanceRequests service
* method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public CancelSpotInstanceRequestsResult cancelSpotInstanceRequests(CancelSpotInstanceRequestsRequest cancelSpotInstanceRequestsRequest) {
ExecutionContext executionContext = createExecutionContext(cancelSpotInstanceRequestsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CancelSpotInstanceRequestsRequestMarshaller().marshall(cancelSpotInstanceRequestsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CancelSpotInstanceRequestsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Purchases a Reserved Instance for use with your account. With Amazon
* EC2 Reserved Instances, you obtain a capacity reservation for a
* certain instance configuration over a specified period of time. You
* pay a lower usage rate than with On-Demand instances for the time that
* you actually use the capacity reservation.
*
*
* For more information, see
* Reserved Instance Marketplace
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param purchaseReservedInstancesOfferingRequest Container for the
* necessary parameters to execute the PurchaseReservedInstancesOffering
* service method on AmazonEC2.
*
* @return The response from the PurchaseReservedInstancesOffering
* service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public PurchaseReservedInstancesOfferingResult purchaseReservedInstancesOffering(PurchaseReservedInstancesOfferingRequest purchaseReservedInstancesOfferingRequest) {
ExecutionContext executionContext = createExecutionContext(purchaseReservedInstancesOfferingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new PurchaseReservedInstancesOfferingRequestMarshaller().marshall(purchaseReservedInstancesOfferingRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new PurchaseReservedInstancesOfferingResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds or removes permission settings for the specified snapshot.
*
*
* For more information on modifying snapshot permissions, see
* Sharing Snapshots
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* NOTE: Snapshots with AWS Marketplace product codes cannot be
* made public.
*
*
* @param modifySnapshotAttributeRequest Container for the necessary
* parameters to execute the ModifySnapshotAttribute service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void modifySnapshotAttribute(ModifySnapshotAttributeRequest modifySnapshotAttributeRequest) {
ExecutionContext executionContext = createExecutionContext(modifySnapshotAttributeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ModifySnapshotAttributeRequestMarshaller().marshall(modifySnapshotAttributeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Describes the modifications made to your Reserved Instances. If no
* parameter is specified, information about all your Reserved Instances
* modification requests is returned. If a modification ID is specified,
* only information about the specific modification is returned.
*
*
* @param describeReservedInstancesModificationsRequest Container for the
* necessary parameters to execute the
* DescribeReservedInstancesModifications service method on AmazonEC2.
*
* @return The response from the DescribeReservedInstancesModifications
* service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeReservedInstancesModificationsResult describeReservedInstancesModifications(DescribeReservedInstancesModificationsRequest describeReservedInstancesModificationsRequest) {
ExecutionContext executionContext = createExecutionContext(describeReservedInstancesModificationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeReservedInstancesModificationsRequestMarshaller().marshall(describeReservedInstancesModificationsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeReservedInstancesModificationsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Shuts down one or more instances. This operation is idempotent; if you
* terminate an instance more than once, each call succeeds.
*
*
* Terminated instances remain visible after termination (for
* approximately one hour).
*
*
* By default, Amazon EC2 deletes all Amazon EBS volumes that were
* attached when the instance launched. Volumes attached after instance
* launch continue running.
*
*
* You can stop, start, and terminate EBS-backed instances. You can only
* terminate instance store-backed instances. What happens to an instance
* differs if you stop it or terminate it. For example, when you stop an
* instance, the root device and any other devices attached to the
* instance persist. When you terminate an instance, the root device and
* any other devices attached during the instance launch are
* automatically deleted. For more information about the differences
* between stopping and terminating instances, see
* Instance Lifecycle
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param terminateInstancesRequest Container for the necessary
* parameters to execute the TerminateInstances service method on
* AmazonEC2.
*
* @return The response from the TerminateInstances service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public TerminateInstancesResult terminateInstances(TerminateInstancesRequest terminateInstancesRequest) {
ExecutionContext executionContext = createExecutionContext(terminateInstancesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new TerminateInstancesRequestMarshaller().marshall(terminateInstancesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new TerminateInstancesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the datafeed for Spot Instances. For more information, see
* Spot Instances
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param deleteSpotDatafeedSubscriptionRequest Container for the
* necessary parameters to execute the DeleteSpotDatafeedSubscription
* service method on AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteSpotDatafeedSubscription(DeleteSpotDatafeedSubscriptionRequest deleteSpotDatafeedSubscriptionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSpotDatafeedSubscriptionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteSpotDatafeedSubscriptionRequestMarshaller().marshall(deleteSpotDatafeedSubscriptionRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Deletes the specified Internet gateway. You must detach the Internet
* gateway from the VPC before you can delete it.
*
*
* @param deleteInternetGatewayRequest Container for the necessary
* parameters to execute the DeleteInternetGateway service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteInternetGateway(DeleteInternetGatewayRequest deleteInternetGatewayRequest) {
ExecutionContext executionContext = createExecutionContext(deleteInternetGatewayRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteInternetGatewayRequestMarshaller().marshall(deleteInternetGatewayRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Describes the specified attribute of the specified snapshot. You can
* specify only one attribute at a time.
*
*
* For more information about Amazon EBS snapshots, see
* Amazon EBS Snapshots
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param describeSnapshotAttributeRequest Container for the necessary
* parameters to execute the DescribeSnapshotAttribute service method on
* AmazonEC2.
*
* @return The response from the DescribeSnapshotAttribute service
* method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeSnapshotAttributeResult describeSnapshotAttribute(DescribeSnapshotAttributeRequest describeSnapshotAttributeRequest) {
ExecutionContext executionContext = createExecutionContext(describeSnapshotAttributeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeSnapshotAttributeRequestMarshaller().marshall(describeSnapshotAttributeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeSnapshotAttributeResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Changes the route table associated with a given subnet in a VPC. After
* the operation completes, the subnet uses the routes in the new route
* table it's associated with. For more information about route tables,
* see
* Route Tables
* in the Amazon Virtual Private Cloud User Guide .
*
*
* You can also use ReplaceRouteTableAssociation to change which table is
* the main route table in the VPC. You just specify the main route
* table's association ID and the route table to be the new main route
* table.
*
*
* @param replaceRouteTableAssociationRequest Container for the necessary
* parameters to execute the ReplaceRouteTableAssociation service method
* on AmazonEC2.
*
* @return The response from the ReplaceRouteTableAssociation service
* method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public ReplaceRouteTableAssociationResult replaceRouteTableAssociation(ReplaceRouteTableAssociationRequest replaceRouteTableAssociationRequest) {
ExecutionContext executionContext = createExecutionContext(replaceRouteTableAssociationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ReplaceRouteTableAssociationRequestMarshaller().marshall(replaceRouteTableAssociationRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ReplaceRouteTableAssociationResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes one or more of your Elastic IP addresses.
*
*
* An Elastic IP address is for use in either the EC2-Classic platform or
* in a VPC. For more information, see
* Elastic IP Addresses
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param describeAddressesRequest Container for the necessary parameters
* to execute the DescribeAddresses service method on AmazonEC2.
*
* @return The response from the DescribeAddresses service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeAddressesResult describeAddresses(DescribeAddressesRequest describeAddressesRequest) {
ExecutionContext executionContext = createExecutionContext(describeAddressesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeAddressesRequestMarshaller().marshall(describeAddressesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeAddressesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the specified attribute of the specified AMI. You can
* specify only one attribute at a time.
*
*
* @param describeImageAttributeRequest Container for the necessary
* parameters to execute the DescribeImageAttribute service method on
* AmazonEC2.
*
* @return The response from the DescribeImageAttribute service method,
* as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeImageAttributeResult describeImageAttribute(DescribeImageAttributeRequest describeImageAttributeRequest) {
ExecutionContext executionContext = createExecutionContext(describeImageAttributeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeImageAttributeRequestMarshaller().marshall(describeImageAttributeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeImageAttributeResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes one or more of your key pairs.
*
*
* For more information about key pairs, see
* Key Pairs
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param describeKeyPairsRequest Container for the necessary parameters
* to execute the DescribeKeyPairs service method on AmazonEC2.
*
* @return The response from the DescribeKeyPairs service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeKeyPairsResult describeKeyPairs(DescribeKeyPairsRequest describeKeyPairsRequest) {
ExecutionContext executionContext = createExecutionContext(describeKeyPairsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeKeyPairsRequestMarshaller().marshall(describeKeyPairsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeKeyPairsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Determines whether a product code is associated with an instance. This
* action can only be used by the owner of the product code. It is useful
* when a product code owner needs to verify whether another user's
* instance is eligible for support.
*
*
* @param confirmProductInstanceRequest Container for the necessary
* parameters to execute the ConfirmProductInstance service method on
* AmazonEC2.
*
* @return The response from the ConfirmProductInstance service method,
* as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public ConfirmProductInstanceResult confirmProductInstance(ConfirmProductInstanceRequest confirmProductInstanceRequest) {
ExecutionContext executionContext = createExecutionContext(confirmProductInstanceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ConfirmProductInstanceRequestMarshaller().marshall(confirmProductInstanceRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ConfirmProductInstanceResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates a subnet from a route table.
*
*
* After you perform this action, the subnet no longer uses the routes in
* the route table. Instead, it uses the routes in the VPC's main route
* table. For more information about route tables, see
* Route Tables
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param disassociateRouteTableRequest Container for the necessary
* parameters to execute the DisassociateRouteTable service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void disassociateRouteTable(DisassociateRouteTableRequest disassociateRouteTableRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateRouteTableRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DisassociateRouteTableRequestMarshaller().marshall(disassociateRouteTableRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Describes the specified attribute of the specified VPC. You can
* specify only one attribute at a time.
*
*
* @param describeVpcAttributeRequest Container for the necessary
* parameters to execute the DescribeVpcAttribute service method on
* AmazonEC2.
*
* @return The response from the DescribeVpcAttribute service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeVpcAttributeResult describeVpcAttribute(DescribeVpcAttributeRequest describeVpcAttributeRequest) {
ExecutionContext executionContext = createExecutionContext(describeVpcAttributeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeVpcAttributeRequestMarshaller().marshall(describeVpcAttributeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeVpcAttributeResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes one or more egress rules from a security group for EC2-VPC.
* The values that you specify in the revoke request (for example, ports)
* must match the existing rule's values for the rule to be revoked.
*
*
* Each rule consists of the protocol and the CIDR range or source
* security group. For the TCP and UDP protocols, you must also specify
* the destination port or range of ports. For the ICMP protocol, you
* must also specify the ICMP type and code.
*
*
* Rule changes are propagated to instances within the security group as
* quickly as possible. However, a small delay might occur.
*
*
* @param revokeSecurityGroupEgressRequest Container for the necessary
* parameters to execute the RevokeSecurityGroupEgress service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void revokeSecurityGroupEgress(RevokeSecurityGroupEgressRequest revokeSecurityGroupEgressRequest) {
ExecutionContext executionContext = createExecutionContext(revokeSecurityGroupEgressRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new RevokeSecurityGroupEgressRequestMarshaller().marshall(revokeSecurityGroupEgressRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Deletes the specified ingress or egress entry (rule) from the
* specified network ACL.
*
*
* @param deleteNetworkAclEntryRequest Container for the necessary
* parameters to execute the DeleteNetworkAclEntry service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteNetworkAclEntry(DeleteNetworkAclEntryRequest deleteNetworkAclEntryRequest) {
ExecutionContext executionContext = createExecutionContext(deleteNetworkAclEntryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteNetworkAclEntryRequestMarshaller().marshall(deleteNetworkAclEntryRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Creates an Amazon EBS volume that can be attached to any instance in
* the same Availability Zone.
*
*
* Any AWS Marketplace product codes from the snapshot are propagated to
* the volume.
*
*
* For more information, see
* Creating or Restoring an Amazon EBS Volume
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param createVolumeRequest Container for the necessary parameters to
* execute the CreateVolume service method on AmazonEC2.
*
* @return The response from the CreateVolume service method, as returned
* by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateVolumeResult createVolume(CreateVolumeRequest createVolumeRequest) {
ExecutionContext executionContext = createExecutionContext(createVolumeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateVolumeRequestMarshaller().marshall(createVolumeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateVolumeResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the status of one or more instances, including any scheduled
* events.
*
*
* Instance status has two main components:
*
*
*
* -
* System Status reports impaired functionality that stems from issues
* related to the systems that support an instance, such as such as
* hardware failures and network connectivity problems. This call reports
* such problems as impaired reachability.
*
*
* -
* Instance Status reports impaired functionality that arises from
* problems internal to the instance. This call reports such problems as
* impaired reachability.
*
*
*
*
*
* Instance status provides information about four types of scheduled
* events for an instance that may require your attention:
*
*
*
* -
* Scheduled Reboot: When Amazon EC2 determines that an instance must be
* rebooted, the instances status returns one of two event codes:
* system-reboot
or instance-reboot
. System
* reboot commonly occurs if certain maintenance or upgrade operations
* require a reboot of the underlying host that supports an instance.
* Instance reboot commonly occurs if the instance must be rebooted,
* rather than the underlying host. Rebooting events include a scheduled
* start and end time.
*
*
* -
* System Maintenance: When Amazon EC2 determines that an instance
* requires maintenance that requires power or network impact, the
* instance status is the event code system-maintenance
.
* System maintenance is either power maintenance or network maintenance.
* For power maintenance, your instance will be unavailable for a brief
* period of time and then rebooted. For network maintenance, your
* instance will experience a brief loss of network connectivity. System
* maintenance events include a scheduled start and end time. You will
* also be notified by email if one of your instances is set for system
* maintenance. The email message indicates when your instance is
* scheduled for maintenance.
*
*
* -
* Scheduled Retirement: When Amazon EC2 determines that an instance must
* be shut down, the instance status is the event code
* instance-retirement
. Retirement commonly occurs when the
* underlying host is degraded and must be replaced. Retirement events
* include a scheduled start and end time. You will also be notified by
* email if one of your instances is set to retiring. The email message
* indicates when your instance will be permanently retired.
*
*
* -
* Scheduled Stop: When Amazon EC2 determines that an instance must be
* shut down, the instances status returns an event code called
* instance-stop
.
* Stop events include a scheduled start and end time. You will
* also be notified by email if one of your instances is set to stop. The
* email message indicates when your instance will be stopped.
*
*
*
*
*
* When your instance is retired, it will either be terminated (if its
* root device type is the instance-store) or stopped (if its root device
* type is an EBS volume). Instances stopped due to retirement will not
* be restarted, but you can do so manually. You can also avoid
* retirement of EBS-backed instances by manually restarting your
* instance when its event code is instance-retirement
.
* This ensures that your instance is started on a different underlying
* host.
*
*
* @param describeInstanceStatusRequest Container for the necessary
* parameters to execute the DescribeInstanceStatus service method on
* AmazonEC2.
*
* @return The response from the DescribeInstanceStatus service method,
* as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeInstanceStatusResult describeInstanceStatus(DescribeInstanceStatusRequest describeInstanceStatusRequest) {
ExecutionContext executionContext = createExecutionContext(describeInstanceStatusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeInstanceStatusRequestMarshaller().marshall(describeInstanceStatusRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeInstanceStatusResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes one or more of your virtual private gateways.
*
*
* For more information about virtual private gateways, see
* Adding an IPsec Hardware VPN to Your VPC
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param describeVpnGatewaysRequest Container for the necessary
* parameters to execute the DescribeVpnGateways service method on
* AmazonEC2.
*
* @return The response from the DescribeVpnGateways service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeVpnGatewaysResult describeVpnGateways(DescribeVpnGatewaysRequest describeVpnGatewaysRequest) {
ExecutionContext executionContext = createExecutionContext(describeVpnGatewaysRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeVpnGatewaysRequestMarshaller().marshall(describeVpnGatewaysRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeVpnGatewaysResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a subnet in an existing VPC.
*
*
* When you create each subnet, you provide the VPC ID and the CIDR block
* you want for the subnet. After you create a subnet, you can't change
* its CIDR block. The subnet's CIDR block can be the same as the VPC's
* CIDR block (assuming you want only a single subnet in the VPC), or a
* subset of the VPC's CIDR block. If you create more than one subnet in
* a VPC, the subnets' CIDR blocks must not overlap. The smallest subnet
* (and VPC) you can create uses a /28 netmask (16 IP addresses), and the
* largest uses a /16 netmask (65,536 IP addresses).
*
*
* IMPORTANT: AWS reserves both the first four and the last IP
* address in each subnet's CIDR block. They're not available for use.
*
*
* If you add more than one subnet to a VPC, they're set up in a star
* topology with a logical router in the middle.
*
*
* For more information about subnets, see
* Your VPC and Subnets
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param createSubnetRequest Container for the necessary parameters to
* execute the CreateSubnet service method on AmazonEC2.
*
* @return The response from the CreateSubnet service method, as returned
* by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateSubnetResult createSubnet(CreateSubnetRequest createSubnetRequest) {
ExecutionContext executionContext = createExecutionContext(createSubnetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateSubnetRequestMarshaller().marshall(createSubnetRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateSubnetResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes Reserved Instance offerings that are available for purchase.
* With Reserved Instances, you purchase the right to launch instances
* for a period of time. During that time period, you do not receive
* insufficient capacity errors, and you pay a lower usage rate than the
* rate charged for On-Demand instances for the actual time used.
*
*
* For more information, see
* Reserved Instance Marketplace
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param describeReservedInstancesOfferingsRequest Container for the
* necessary parameters to execute the DescribeReservedInstancesOfferings
* service method on AmazonEC2.
*
* @return The response from the DescribeReservedInstancesOfferings
* service method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeReservedInstancesOfferingsResult describeReservedInstancesOfferings(DescribeReservedInstancesOfferingsRequest describeReservedInstancesOfferingsRequest) {
ExecutionContext executionContext = createExecutionContext(describeReservedInstancesOfferingsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeReservedInstancesOfferingsRequestMarshaller().marshall(describeReservedInstancesOfferingsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeReservedInstancesOfferingsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Assigns one or more secondary private IP addresses to the specified
* network interface. You can specify one or more specific secondary IP
* addresses, or you can specify the number of secondary IP addresses to
* be automatically assigned within the subnet's CIDR block range. The
* number of secondary IP addresses that you can assign to an instance
* varies by instance type. For information about instance types, see
* Instance Types in the Amazon Elastic Compute Cloud User Guide . For more information about Elastic IP addresses, see Elastic IP Addresses
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* AssignPrivateIpAddresses is available only in EC2-VPC.
*
*
* @param assignPrivateIpAddressesRequest Container for the necessary
* parameters to execute the AssignPrivateIpAddresses service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void assignPrivateIpAddresses(AssignPrivateIpAddressesRequest assignPrivateIpAddressesRequest) {
ExecutionContext executionContext = createExecutionContext(assignPrivateIpAddressesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new AssignPrivateIpAddressesRequestMarshaller().marshall(assignPrivateIpAddressesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Deletes the specified snapshot.
*
*
* When you make periodic snapshots of a volume, the snapshots are
* incremental, and only the blocks on the device that have changed since
* your last snapshot are saved in the new snapshot. When you delete a
* snapshot, only the data not needed for any other snapshot is removed.
* So regardless of which prior snapshots have been deleted, all active
* snapshots will have access to all the information needed to restore
* the volume.
*
*
* You cannot delete a snapshot of the root device of an Amazon EBS
* volume used by a registered AMI. You must first de-register the AMI
* before you can delete the snapshot.
*
*
* For more information, see
* Deleting an Amazon EBS Snapshot
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param deleteSnapshotRequest Container for the necessary parameters to
* execute the DeleteSnapshot service method on AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteSnapshot(DeleteSnapshotRequest deleteSnapshotRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSnapshotRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteSnapshotRequestMarshaller().marshall(deleteSnapshotRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Changes which network ACL a subnet is associated with. By default when
* you create a subnet, it's automatically associated with the default
* network ACL. For more information about network ACLs, see
* Network ACLs
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param replaceNetworkAclAssociationRequest Container for the necessary
* parameters to execute the ReplaceNetworkAclAssociation service method
* on AmazonEC2.
*
* @return The response from the ReplaceNetworkAclAssociation service
* method, as returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public ReplaceNetworkAclAssociationResult replaceNetworkAclAssociation(ReplaceNetworkAclAssociationRequest replaceNetworkAclAssociationRequest) {
ExecutionContext executionContext = createExecutionContext(replaceNetworkAclAssociationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ReplaceNetworkAclAssociationRequestMarshaller().marshall(replaceNetworkAclAssociationRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ReplaceNetworkAclAssociationResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates an Elastic IP address from the instance or network
* interface it's associated with.
*
*
* This is an idempotent operation. If you perform the operation more
* than once, Amazon EC2 doesn't return an error.
*
*
* @param disassociateAddressRequest Container for the necessary
* parameters to execute the DisassociateAddress service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void disassociateAddress(DisassociateAddressRequest disassociateAddressRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateAddressRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DisassociateAddressRequestMarshaller().marshall(disassociateAddressRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Creates a placement group that you launch cluster instances into. You
* must give the group a name that's unique within the scope of your
* account.
*
*
* For more information about placement groups and cluster instances, see
* Cluster Instances
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param createPlacementGroupRequest Container for the necessary
* parameters to execute the CreatePlacementGroup service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void createPlacementGroup(CreatePlacementGroupRequest createPlacementGroupRequest) {
ExecutionContext executionContext = createExecutionContext(createPlacementGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreatePlacementGroupRequestMarshaller().marshall(createPlacementGroupRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Bundles an Amazon instance store-backed Windows instance.
*
*
* During bundling, only the root device volume (C:\) is bundled. Data on
* other instance store volumes is not preserved.
*
*
* NOTE: This procedure is not applicable for Linux/Unix instances
* or Windows instances that are backed by Amazon EBS.
*
*
* For more information, see
* Creating an Instance Store-Backed Windows AMI
* .
*
*
* @param bundleInstanceRequest Container for the necessary parameters to
* execute the BundleInstance service method on AmazonEC2.
*
* @return The response from the BundleInstance service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public BundleInstanceResult bundleInstance(BundleInstanceRequest bundleInstanceRequest) {
ExecutionContext executionContext = createExecutionContext(bundleInstanceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new BundleInstanceRequestMarshaller().marshall(bundleInstanceRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new BundleInstanceResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified placement group. You must terminate all
* instances in the placement group before you can delete the placement
* group. For more information about placement groups and cluster
* instances, see
* Cluster Instances
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param deletePlacementGroupRequest Container for the necessary
* parameters to execute the DeletePlacementGroup service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deletePlacementGroup(DeletePlacementGroupRequest deletePlacementGroupRequest) {
ExecutionContext executionContext = createExecutionContext(deletePlacementGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeletePlacementGroupRequestMarshaller().marshall(deletePlacementGroupRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Deletes the specified VPC. You must detach or delete all gateways and
* resources that are associated with the VPC before you can delete it.
* For example, you must terminate all instances running in the VPC,
* delete all security groups associated with the VPC (except the default
* one), delete all route tables associated with the VPC (except the
* default one), and so on.
*
*
* @param deleteVpcRequest Container for the necessary parameters to
* execute the DeleteVpc service method on AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteVpc(DeleteVpcRequest deleteVpcRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVpcRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteVpcRequestMarshaller().marshall(deleteVpcRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Copies a point-in-time snapshot of an Amazon EBS volume and stores it
* in Amazon S3. You can copy the snapshot within the same region or from
* one region to another. You can use the snapshot to create Amazon EBS
* volumes or Amazon Machine Images (AMIs).
*
*
* For more information, see
* Copying an Amazon EBS Snapshot
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param copySnapshotRequest Container for the necessary parameters to
* execute the CopySnapshot service method on AmazonEC2.
*
* @return The response from the CopySnapshot service method, as returned
* by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public CopySnapshotResult copySnapshot(CopySnapshotRequest copySnapshotRequest) {
ExecutionContext executionContext = createExecutionContext(copySnapshotRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CopySnapshotRequestMarshaller().marshall(copySnapshotRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CopySnapshotResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Acquires an Elastic IP address.
*
*
* An Elastic IP address is for use either in the EC2-Classic platform or
* in a VPC. For more information, see
* Elastic IP Addresses
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param allocateAddressRequest Container for the necessary parameters
* to execute the AllocateAddress service method on AmazonEC2.
*
* @return The response from the AllocateAddress service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public AllocateAddressResult allocateAddress(AllocateAddressRequest allocateAddressRequest) {
ExecutionContext executionContext = createExecutionContext(allocateAddressRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new AllocateAddressRequestMarshaller().marshall(allocateAddressRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new AllocateAddressResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Releases the specified Elastic IP address.
*
*
* After releasing an Elastic IP address, it is released to the IP
* address pool and might be unavailable to you. Be sure to update your
* DNS records and any servers or devices that communicate with the
* address. If you attempt to release an Elastic IP address that you
* already released, you'll get an AuthFailure
error if the
* address is already allocated to another AWS account.
*
*
* [EC2-Classic, default VPC] Releasing an Elastic IP address
* automatically disassociates it from any instance that it's associated
* with. To disassociate an Elastic IP address without releasing it, use
* DisassociateAddress.
*
*
* [Nondefault VPC] You must use the DisassociateAddress to disassociate
* the Elastic IP address before you try to release it. Otherwise, Amazon
* EC2 returns an error ( InvalidIPAddress.InUse
).
*
*
* @param releaseAddressRequest Container for the necessary parameters to
* execute the ReleaseAddress service method on AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void releaseAddress(ReleaseAddressRequest releaseAddressRequest) {
ExecutionContext executionContext = createExecutionContext(releaseAddressRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ReleaseAddressRequestMarshaller().marshall(releaseAddressRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Resets an attribute of an instance to its default value. To reset the
* kernel or RAM disk, the instance must be in a stopped state. To reset
* the SourceDestCheck
, the instance can be either running
* or stopped.
*
*
* The SourceDestCheck
attribute controls whether
* source/destination checking is enabled. The default value is
* true
, which means checking is enabled. This value must
* be false
for a NAT instance to perform NAT. For more
* information, see
* NAT Instances
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param resetInstanceAttributeRequest Container for the necessary
* parameters to execute the ResetInstanceAttribute service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void resetInstanceAttribute(ResetInstanceAttributeRequest resetInstanceAttributeRequest) {
ExecutionContext executionContext = createExecutionContext(resetInstanceAttributeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ResetInstanceAttributeRequestMarshaller().marshall(resetInstanceAttributeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Creates a 2048-bit RSA key pair with the specified name. Amazon EC2
* stores the public key and displays the private key for you to save to
* a file. The private key is returned as an unencrypted PEM encoded
* PKCS#8 private key. If a key with the specified name already exists,
* Amazon EC2 returns an error.
*
*
* You can have up to five thousand key pairs per region.
*
*
* For more information about key pairs, see
* Key Pairs
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param createKeyPairRequest Container for the necessary parameters to
* execute the CreateKeyPair service method on AmazonEC2.
*
* @return The response from the CreateKeyPair service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateKeyPairResult createKeyPair(CreateKeyPairRequest createKeyPairRequest) {
ExecutionContext executionContext = createExecutionContext(createKeyPairRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateKeyPairRequestMarshaller().marshall(createKeyPairRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateKeyPairResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Replaces an entry (rule) in a network ACL. For more information about
* network ACLs, see
* Network ACLs
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param replaceNetworkAclEntryRequest Container for the necessary
* parameters to execute the ReplaceNetworkAclEntry service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void replaceNetworkAclEntry(ReplaceNetworkAclEntryRequest replaceNetworkAclEntryRequest) {
ExecutionContext executionContext = createExecutionContext(replaceNetworkAclEntryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ReplaceNetworkAclEntryRequestMarshaller().marshall(replaceNetworkAclEntryRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Describes one or more of the Amazon EBS snapshots available to you.
* Available snapshots include public snapshots available for any AWS
* account to launch, private snapshots that you own, and private
* snapshots owned by another AWS account but for which you've been given
* explicit create volume permissions.
*
*
* The create volume permissions fall into the following categories:
*
*
*
* - public : The owner of the snapshot granted create volume
* permissions for the snapshot to the
all
group. All AWS
* accounts have create volume permissions for these snapshots.
* - explicit : The owner of the snapshot granted create
* volume permissions to a specific AWS account.
* - implicit : An AWS account has implicit create volume
* permissions for all snapshots it owns.
*
*
*
* The list of snapshots returned can be modified by specifying snapshot
* IDs, snapshot owners, or AWS accounts with create volume permissions.
* If no options are specified, Amazon EC2 returns all snapshots for
* which you have create volume permissions.
*
*
* If you specify one or more snapshot IDs, only snapshots that have the
* specified IDs are returned. If you specify an invalid snapshot ID, an
* error is returned. If you specify a snapshot ID for which you do not
* have access, it is not included in the returned results.
*
*
* If you specify one or more snapshot owners, only snapshots from the
* specified owners and for which you have access are returned. The
* results can include the AWS account IDs of the specified owners,
* amazon
for snapshots owned by Amazon, or
* self
for snapshots that you own.
*
*
* If you specify a list of restorable users, only snapshots with create
* snapshot permissions for those users are returned. You can specify AWS
* account IDs (if you own the snapshots), self
for
* snapshots for which you own or have explicit permissions, or
* all
for public snapshots.
*
*
* For more information about Amazon EBS snapshots, see
* Amazon EBS Snapshots
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* @param describeSnapshotsRequest Container for the necessary parameters
* to execute the DescribeSnapshots service method on AmazonEC2.
*
* @return The response from the DescribeSnapshots service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeSnapshotsResult describeSnapshots(DescribeSnapshotsRequest describeSnapshotsRequest) {
ExecutionContext executionContext = createExecutionContext(describeSnapshotsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeSnapshotsRequestMarshaller().marshall(describeSnapshotsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeSnapshotsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a network ACL in a VPC. Network ACLs provide an optional layer
* of security (in addition to security groups) for the instances in your
* VPC.
*
*
* For more information about network ACLs, see
* Network ACLs
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param createNetworkAclRequest Container for the necessary parameters
* to execute the CreateNetworkAcl service method on AmazonEC2.
*
* @return The response from the CreateNetworkAcl service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateNetworkAclResult createNetworkAcl(CreateNetworkAclRequest createNetworkAclRequest) {
ExecutionContext executionContext = createExecutionContext(createNetworkAclRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateNetworkAclRequestMarshaller().marshall(createNetworkAclRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateNetworkAclResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Registers an AMI. When you're creating an AMI, this is the final step
* you must complete before you can launch an instance from the AMI. For
* more information about creating AMIs, see
* Creating Your Own AMIs
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* NOTE: For Amazon EBS-backed instances, CreateImage creates and
* registers the AMI in a single request, so you don't have to register
* the AMI yourself.
*
*
* You can also use RegisterImage
to create an Amazon
* EBS-backed AMI from a snapshot of a root device volume. For more
* information, see
* Launching an Instance from a Snapshot
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* If needed, you can deregister an AMI at any time. Any modifications
* you make to an AMI backed by an instance store volume invalidates its
* registration. If you make changes to an image, deregister the previous
* image and register the new image.
*
*
* NOTE: You can't register an image where a secondary (non-root)
* snapshot has AWS Marketplace product codes.
*
*
* @param registerImageRequest Container for the necessary parameters to
* execute the RegisterImage service method on AmazonEC2.
*
* @return The response from the RegisterImage service method, as
* returned by AmazonEC2.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public RegisterImageResult registerImage(RegisterImageRequest registerImageRequest) {
ExecutionContext executionContext = createExecutionContext(registerImageRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new RegisterImageRequestMarshaller().marshall(registerImageRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new RegisterImageResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Resets a network interface attribute. You can specify only one
* attribute at a time.
*
*
* @param resetNetworkInterfaceAttributeRequest Container for the
* necessary parameters to execute the ResetNetworkInterfaceAttribute
* service method on AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void resetNetworkInterfaceAttribute(ResetNetworkInterfaceAttributeRequest resetNetworkInterfaceAttributeRequest) {
ExecutionContext executionContext = createExecutionContext(resetNetworkInterfaceAttributeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ResetNetworkInterfaceAttributeRequestMarshaller().marshall(resetNetworkInterfaceAttributeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Creates a static route associated with a VPN connection between an
* existing virtual private gateway and a VPN customer gateway. The
* static route allows traffic to be routed from the virtual private
* gateway to the VPN customer gateway.
*
*
* For more information about VPN connections, see
* Adding a Hardware Virtual Private Gateway to Your VPC
* in the Amazon Virtual Private Cloud User Guide .
*
*
* @param createVpnConnectionRouteRequest Container for the necessary
* parameters to execute the CreateVpnConnectionRoute service method on
* AmazonEC2.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonEC2 indicating
* either a problem with the data in the request, or a server side issue.
*/
public void createVpnConnectionRoute(CreateVpnConnectionRouteRequest createVpnConnectionRouteRequest) {
ExecutionContext executionContext = createExecutionContext(createVpnConnectionRouteRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateVpnConnectionRouteRequestMarshaller().marshall(createVpnConnectionRouteRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Describes one or more of the Reserved Instances that you purchased.
*
*
* For more information about Reserved Instances, see
*