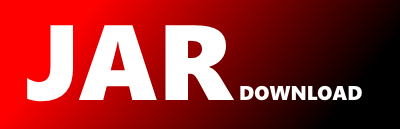
com.amazonaws.services.ec2.model.DescribeNetworkInterfacesRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.ec2.model;
import java.io.Serializable;
import com.amazonaws.AmazonWebServiceRequest;
import com.amazonaws.Request;
import com.amazonaws.services.ec2.model.transform.DescribeNetworkInterfacesRequestMarshaller;
/**
* Container for the parameters to the {@link com.amazonaws.services.ec2.AmazonEC2#describeNetworkInterfaces(DescribeNetworkInterfacesRequest) DescribeNetworkInterfaces operation}.
*
* Describes one or more of your network interfaces.
*
*
* @see com.amazonaws.services.ec2.AmazonEC2#describeNetworkInterfaces(DescribeNetworkInterfacesRequest)
*/
public class DescribeNetworkInterfacesRequest extends AmazonWebServiceRequest implements Serializable, DryRunSupportedRequest {
/**
* One or more network interface IDs. Default: Describes all your
* network interfaces.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag networkInterfaceIds;
/**
* One or more filters. -
*
addresses.private-ip-address
- The private IP
* addresses associated with the network interface.
-
*
addresses.primary
- Whether the private IP address is
* the primary IP address associated with the network interface.
* -
addresses.association.public-ip
- The association
* ID returned when the network interface was associated with the Elastic
* IP address.
-
addresses.association.owner-id
* - The owner ID of the addresses associated with the network interface.
*
-
association.association-id
- The
* association ID returned when the network interface was associated with
* an IP address.
-
association.allocation-id
-
* The allocation ID returned when you allocated the Elastic IP address
* for your network interface.
-
*
association.ip-owner-id
- The owner of the Elastic IP
* address associated with the network interface.
-
*
association.public-ip
- The address of the Elastic IP
* address bound to the network interface.
-
*
attachment.attachment-id
- The ID of the interface
* attachment.
-
attachment.instance-id
- The ID
* of the instance to which the network interface is attached.
-
*
attachment.instance-owner-id
- The owner ID of the
* instance to which the network interface is attached.
-
*
attachment.device-index
- The device index to which
* the network interface is attached.
-
*
attachment.status
- The status of the attachment
* (attaching
| attached
|
* detaching
| detached
).
-
*
attachment.attach.time
- The time that the network
* interface was attached to an instance.
-
*
attachment.delete-on-termination
- Indicates whether
* the attachment is deleted when an instance is terminated.
-
*
availability-zone
- The Availability Zone of the
* network interface.
-
description
- The
* description of the network interface.
-
*
group-id
- The ID of a security group associated with
* the network interface.
-
group-name
- The
* name of a security group associated with the network interface.
* -
mac-address
- The MAC address of the network
* interface.
-
network-interface-id
- The ID of
* the network interface.
-
owner-id
- The AWS
* account ID of the network interface owner.
-
*
private-ip-address
- The private IP address or
* addresses of the network interface.
-
*
private-dns-name
- The private DNS name of the network
* interface.
-
requester-id
- The ID of the
* entity that launched the instance on your behalf (for example, AWS
* Management Console, Auto Scaling, and so on).
-
*
requester-managed
- Indicates whether the network
* interface is being managed by an AWS service (for example, AWS
* Management Console, Auto Scaling, and so on).
-
*
source-desk-check
- Indicates whether the network
* interface performs source/destination checking. A value of
* true
means checking is enabled, and false
* means checking is disabled. The value must be false
for
* the network interface to perform Network Address Translation (NAT) in
* your VPC.
-
status
- The status of the
* network interface. If the network interface is not attached to an
* instance, the status is available
; if a network interface
* is attached to an instance the status is in-use
.
* -
subnet-id
- The ID of the subnet for the network
* interface.
-
tag
:key=value -
* The key/value combination of a tag assigned to the resource.
* -
tag-key
- The key of a tag assigned to the
* resource. This filter is independent of the tag-value
* filter. For example, if you use both the filter "tag-key=Purpose" and
* the filter "tag-value=X", you get any resources assigned both the tag
* key Purpose (regardless of what the tag's value is), and the tag value
* X (regardless of what the tag's key is). If you want to list only
* resources where Purpose is X, see the
* tag
:key=value filter.
-
*
tag-value
- The value of a tag assigned to the
* resource. This filter is independent of the tag-key
* filter.
-
vpc-id
- The ID of the VPC for the
* network interface.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag filters;
/**
* One or more network interface IDs. Default: Describes all your
* network interfaces.
*
* @return One or more network interface IDs.
Default: Describes all your
* network interfaces.
*/
public java.util.List getNetworkInterfaceIds() {
if (networkInterfaceIds == null) {
networkInterfaceIds = new com.amazonaws.internal.ListWithAutoConstructFlag();
networkInterfaceIds.setAutoConstruct(true);
}
return networkInterfaceIds;
}
/**
* One or more network interface IDs. Default: Describes all your
* network interfaces.
*
* @param networkInterfaceIds One or more network interface IDs.
Default: Describes all your
* network interfaces.
*/
public void setNetworkInterfaceIds(java.util.Collection networkInterfaceIds) {
if (networkInterfaceIds == null) {
this.networkInterfaceIds = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag networkInterfaceIdsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(networkInterfaceIds.size());
networkInterfaceIdsCopy.addAll(networkInterfaceIds);
this.networkInterfaceIds = networkInterfaceIdsCopy;
}
/**
* One or more network interface IDs. Default: Describes all your
* network interfaces.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param networkInterfaceIds One or more network interface IDs.
Default: Describes all your
* network interfaces.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public DescribeNetworkInterfacesRequest withNetworkInterfaceIds(String... networkInterfaceIds) {
if (getNetworkInterfaceIds() == null) setNetworkInterfaceIds(new java.util.ArrayList(networkInterfaceIds.length));
for (String value : networkInterfaceIds) {
getNetworkInterfaceIds().add(value);
}
return this;
}
/**
* One or more network interface IDs. Default: Describes all your
* network interfaces.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param networkInterfaceIds One or more network interface IDs.
Default: Describes all your
* network interfaces.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public DescribeNetworkInterfacesRequest withNetworkInterfaceIds(java.util.Collection networkInterfaceIds) {
if (networkInterfaceIds == null) {
this.networkInterfaceIds = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag networkInterfaceIdsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(networkInterfaceIds.size());
networkInterfaceIdsCopy.addAll(networkInterfaceIds);
this.networkInterfaceIds = networkInterfaceIdsCopy;
}
return this;
}
/**
* One or more filters. -
*
addresses.private-ip-address
- The private IP
* addresses associated with the network interface.
-
*
addresses.primary
- Whether the private IP address is
* the primary IP address associated with the network interface.
* -
addresses.association.public-ip
- The association
* ID returned when the network interface was associated with the Elastic
* IP address.
-
addresses.association.owner-id
* - The owner ID of the addresses associated with the network interface.
*
-
association.association-id
- The
* association ID returned when the network interface was associated with
* an IP address.
-
association.allocation-id
-
* The allocation ID returned when you allocated the Elastic IP address
* for your network interface.
-
*
association.ip-owner-id
- The owner of the Elastic IP
* address associated with the network interface.
-
*
association.public-ip
- The address of the Elastic IP
* address bound to the network interface.
-
*
attachment.attachment-id
- The ID of the interface
* attachment.
-
attachment.instance-id
- The ID
* of the instance to which the network interface is attached.
-
*
attachment.instance-owner-id
- The owner ID of the
* instance to which the network interface is attached.
-
*
attachment.device-index
- The device index to which
* the network interface is attached.
-
*
attachment.status
- The status of the attachment
* (attaching
| attached
|
* detaching
| detached
).
-
*
attachment.attach.time
- The time that the network
* interface was attached to an instance.
-
*
attachment.delete-on-termination
- Indicates whether
* the attachment is deleted when an instance is terminated.
-
*
availability-zone
- The Availability Zone of the
* network interface.
-
description
- The
* description of the network interface.
-
*
group-id
- The ID of a security group associated with
* the network interface.
-
group-name
- The
* name of a security group associated with the network interface.
* -
mac-address
- The MAC address of the network
* interface.
-
network-interface-id
- The ID of
* the network interface.
-
owner-id
- The AWS
* account ID of the network interface owner.
-
*
private-ip-address
- The private IP address or
* addresses of the network interface.
-
*
private-dns-name
- The private DNS name of the network
* interface.
-
requester-id
- The ID of the
* entity that launched the instance on your behalf (for example, AWS
* Management Console, Auto Scaling, and so on).
-
*
requester-managed
- Indicates whether the network
* interface is being managed by an AWS service (for example, AWS
* Management Console, Auto Scaling, and so on).
-
*
source-desk-check
- Indicates whether the network
* interface performs source/destination checking. A value of
* true
means checking is enabled, and false
* means checking is disabled. The value must be false
for
* the network interface to perform Network Address Translation (NAT) in
* your VPC.
-
status
- The status of the
* network interface. If the network interface is not attached to an
* instance, the status is available
; if a network interface
* is attached to an instance the status is in-use
.
* -
subnet-id
- The ID of the subnet for the network
* interface.
-
tag
:key=value -
* The key/value combination of a tag assigned to the resource.
* -
tag-key
- The key of a tag assigned to the
* resource. This filter is independent of the tag-value
* filter. For example, if you use both the filter "tag-key=Purpose" and
* the filter "tag-value=X", you get any resources assigned both the tag
* key Purpose (regardless of what the tag's value is), and the tag value
* X (regardless of what the tag's key is). If you want to list only
* resources where Purpose is X, see the
* tag
:key=value filter.
-
*
tag-value
- The value of a tag assigned to the
* resource. This filter is independent of the tag-key
* filter.
-
vpc-id
- The ID of the VPC for the
* network interface.
*
* @return One or more filters. -
*
addresses.private-ip-address
- The private IP
* addresses associated with the network interface.
-
*
addresses.primary
- Whether the private IP address is
* the primary IP address associated with the network interface.
* -
addresses.association.public-ip
- The association
* ID returned when the network interface was associated with the Elastic
* IP address.
-
addresses.association.owner-id
* - The owner ID of the addresses associated with the network interface.
*
-
association.association-id
- The
* association ID returned when the network interface was associated with
* an IP address.
-
association.allocation-id
-
* The allocation ID returned when you allocated the Elastic IP address
* for your network interface.
-
*
association.ip-owner-id
- The owner of the Elastic IP
* address associated with the network interface.
-
*
association.public-ip
- The address of the Elastic IP
* address bound to the network interface.
-
*
attachment.attachment-id
- The ID of the interface
* attachment.
-
attachment.instance-id
- The ID
* of the instance to which the network interface is attached.
-
*
attachment.instance-owner-id
- The owner ID of the
* instance to which the network interface is attached.
-
*
attachment.device-index
- The device index to which
* the network interface is attached.
-
*
attachment.status
- The status of the attachment
* (attaching
| attached
|
* detaching
| detached
).
-
*
attachment.attach.time
- The time that the network
* interface was attached to an instance.
-
*
attachment.delete-on-termination
- Indicates whether
* the attachment is deleted when an instance is terminated.
-
*
availability-zone
- The Availability Zone of the
* network interface.
-
description
- The
* description of the network interface.
-
*
group-id
- The ID of a security group associated with
* the network interface.
-
group-name
- The
* name of a security group associated with the network interface.
* -
mac-address
- The MAC address of the network
* interface.
-
network-interface-id
- The ID of
* the network interface.
-
owner-id
- The AWS
* account ID of the network interface owner.
-
*
private-ip-address
- The private IP address or
* addresses of the network interface.
-
*
private-dns-name
- The private DNS name of the network
* interface.
-
requester-id
- The ID of the
* entity that launched the instance on your behalf (for example, AWS
* Management Console, Auto Scaling, and so on).
-
*
requester-managed
- Indicates whether the network
* interface is being managed by an AWS service (for example, AWS
* Management Console, Auto Scaling, and so on).
-
*
source-desk-check
- Indicates whether the network
* interface performs source/destination checking. A value of
* true
means checking is enabled, and false
* means checking is disabled. The value must be false
for
* the network interface to perform Network Address Translation (NAT) in
* your VPC.
-
status
- The status of the
* network interface. If the network interface is not attached to an
* instance, the status is available
; if a network interface
* is attached to an instance the status is in-use
.
* -
subnet-id
- The ID of the subnet for the network
* interface.
-
tag
:key=value -
* The key/value combination of a tag assigned to the resource.
* -
tag-key
- The key of a tag assigned to the
* resource. This filter is independent of the tag-value
* filter. For example, if you use both the filter "tag-key=Purpose" and
* the filter "tag-value=X", you get any resources assigned both the tag
* key Purpose (regardless of what the tag's value is), and the tag value
* X (regardless of what the tag's key is). If you want to list only
* resources where Purpose is X, see the
* tag
:key=value filter.
-
*
tag-value
- The value of a tag assigned to the
* resource. This filter is independent of the tag-key
* filter.
-
vpc-id
- The ID of the VPC for the
* network interface.
*/
public java.util.List getFilters() {
if (filters == null) {
filters = new com.amazonaws.internal.ListWithAutoConstructFlag();
filters.setAutoConstruct(true);
}
return filters;
}
/**
* One or more filters. -
*
addresses.private-ip-address
- The private IP
* addresses associated with the network interface.
-
*
addresses.primary
- Whether the private IP address is
* the primary IP address associated with the network interface.
* -
addresses.association.public-ip
- The association
* ID returned when the network interface was associated with the Elastic
* IP address.
-
addresses.association.owner-id
* - The owner ID of the addresses associated with the network interface.
*
-
association.association-id
- The
* association ID returned when the network interface was associated with
* an IP address.
-
association.allocation-id
-
* The allocation ID returned when you allocated the Elastic IP address
* for your network interface.
-
*
association.ip-owner-id
- The owner of the Elastic IP
* address associated with the network interface.
-
*
association.public-ip
- The address of the Elastic IP
* address bound to the network interface.
-
*
attachment.attachment-id
- The ID of the interface
* attachment.
-
attachment.instance-id
- The ID
* of the instance to which the network interface is attached.
-
*
attachment.instance-owner-id
- The owner ID of the
* instance to which the network interface is attached.
-
*
attachment.device-index
- The device index to which
* the network interface is attached.
-
*
attachment.status
- The status of the attachment
* (attaching
| attached
|
* detaching
| detached
).
-
*
attachment.attach.time
- The time that the network
* interface was attached to an instance.
-
*
attachment.delete-on-termination
- Indicates whether
* the attachment is deleted when an instance is terminated.
-
*
availability-zone
- The Availability Zone of the
* network interface.
-
description
- The
* description of the network interface.
-
*
group-id
- The ID of a security group associated with
* the network interface.
-
group-name
- The
* name of a security group associated with the network interface.
* -
mac-address
- The MAC address of the network
* interface.
-
network-interface-id
- The ID of
* the network interface.
-
owner-id
- The AWS
* account ID of the network interface owner.
-
*
private-ip-address
- The private IP address or
* addresses of the network interface.
-
*
private-dns-name
- The private DNS name of the network
* interface.
-
requester-id
- The ID of the
* entity that launched the instance on your behalf (for example, AWS
* Management Console, Auto Scaling, and so on).
-
*
requester-managed
- Indicates whether the network
* interface is being managed by an AWS service (for example, AWS
* Management Console, Auto Scaling, and so on).
-
*
source-desk-check
- Indicates whether the network
* interface performs source/destination checking. A value of
* true
means checking is enabled, and false
* means checking is disabled. The value must be false
for
* the network interface to perform Network Address Translation (NAT) in
* your VPC.
-
status
- The status of the
* network interface. If the network interface is not attached to an
* instance, the status is available
; if a network interface
* is attached to an instance the status is in-use
.
* -
subnet-id
- The ID of the subnet for the network
* interface.
-
tag
:key=value -
* The key/value combination of a tag assigned to the resource.
* -
tag-key
- The key of a tag assigned to the
* resource. This filter is independent of the tag-value
* filter. For example, if you use both the filter "tag-key=Purpose" and
* the filter "tag-value=X", you get any resources assigned both the tag
* key Purpose (regardless of what the tag's value is), and the tag value
* X (regardless of what the tag's key is). If you want to list only
* resources where Purpose is X, see the
* tag
:key=value filter.
-
*
tag-value
- The value of a tag assigned to the
* resource. This filter is independent of the tag-key
* filter.
-
vpc-id
- The ID of the VPC for the
* network interface.
*
* @param filters One or more filters. -
*
addresses.private-ip-address
- The private IP
* addresses associated with the network interface.
-
*
addresses.primary
- Whether the private IP address is
* the primary IP address associated with the network interface.
* -
addresses.association.public-ip
- The association
* ID returned when the network interface was associated with the Elastic
* IP address.
-
addresses.association.owner-id
* - The owner ID of the addresses associated with the network interface.
*
-
association.association-id
- The
* association ID returned when the network interface was associated with
* an IP address.
-
association.allocation-id
-
* The allocation ID returned when you allocated the Elastic IP address
* for your network interface.
-
*
association.ip-owner-id
- The owner of the Elastic IP
* address associated with the network interface.
-
*
association.public-ip
- The address of the Elastic IP
* address bound to the network interface.
-
*
attachment.attachment-id
- The ID of the interface
* attachment.
-
attachment.instance-id
- The ID
* of the instance to which the network interface is attached.
-
*
attachment.instance-owner-id
- The owner ID of the
* instance to which the network interface is attached.
-
*
attachment.device-index
- The device index to which
* the network interface is attached.
-
*
attachment.status
- The status of the attachment
* (attaching
| attached
|
* detaching
| detached
).
-
*
attachment.attach.time
- The time that the network
* interface was attached to an instance.
-
*
attachment.delete-on-termination
- Indicates whether
* the attachment is deleted when an instance is terminated.
-
*
availability-zone
- The Availability Zone of the
* network interface.
-
description
- The
* description of the network interface.
-
*
group-id
- The ID of a security group associated with
* the network interface.
-
group-name
- The
* name of a security group associated with the network interface.
* -
mac-address
- The MAC address of the network
* interface.
-
network-interface-id
- The ID of
* the network interface.
-
owner-id
- The AWS
* account ID of the network interface owner.
-
*
private-ip-address
- The private IP address or
* addresses of the network interface.
-
*
private-dns-name
- The private DNS name of the network
* interface.
-
requester-id
- The ID of the
* entity that launched the instance on your behalf (for example, AWS
* Management Console, Auto Scaling, and so on).
-
*
requester-managed
- Indicates whether the network
* interface is being managed by an AWS service (for example, AWS
* Management Console, Auto Scaling, and so on).
-
*
source-desk-check
- Indicates whether the network
* interface performs source/destination checking. A value of
* true
means checking is enabled, and false
* means checking is disabled. The value must be false
for
* the network interface to perform Network Address Translation (NAT) in
* your VPC.
-
status
- The status of the
* network interface. If the network interface is not attached to an
* instance, the status is available
; if a network interface
* is attached to an instance the status is in-use
.
* -
subnet-id
- The ID of the subnet for the network
* interface.
-
tag
:key=value -
* The key/value combination of a tag assigned to the resource.
* -
tag-key
- The key of a tag assigned to the
* resource. This filter is independent of the tag-value
* filter. For example, if you use both the filter "tag-key=Purpose" and
* the filter "tag-value=X", you get any resources assigned both the tag
* key Purpose (regardless of what the tag's value is), and the tag value
* X (regardless of what the tag's key is). If you want to list only
* resources where Purpose is X, see the
* tag
:key=value filter.
-
*
tag-value
- The value of a tag assigned to the
* resource. This filter is independent of the tag-key
* filter.
-
vpc-id
- The ID of the VPC for the
* network interface.
*/
public void setFilters(java.util.Collection filters) {
if (filters == null) {
this.filters = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag filtersCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(filters.size());
filtersCopy.addAll(filters);
this.filters = filtersCopy;
}
/**
* One or more filters. -
*
addresses.private-ip-address
- The private IP
* addresses associated with the network interface.
-
*
addresses.primary
- Whether the private IP address is
* the primary IP address associated with the network interface.
* -
addresses.association.public-ip
- The association
* ID returned when the network interface was associated with the Elastic
* IP address.
-
addresses.association.owner-id
* - The owner ID of the addresses associated with the network interface.
*
-
association.association-id
- The
* association ID returned when the network interface was associated with
* an IP address.
-
association.allocation-id
-
* The allocation ID returned when you allocated the Elastic IP address
* for your network interface.
-
*
association.ip-owner-id
- The owner of the Elastic IP
* address associated with the network interface.
-
*
association.public-ip
- The address of the Elastic IP
* address bound to the network interface.
-
*
attachment.attachment-id
- The ID of the interface
* attachment.
-
attachment.instance-id
- The ID
* of the instance to which the network interface is attached.
-
*
attachment.instance-owner-id
- The owner ID of the
* instance to which the network interface is attached.
-
*
attachment.device-index
- The device index to which
* the network interface is attached.
-
*
attachment.status
- The status of the attachment
* (attaching
| attached
|
* detaching
| detached
).
-
*
attachment.attach.time
- The time that the network
* interface was attached to an instance.
-
*
attachment.delete-on-termination
- Indicates whether
* the attachment is deleted when an instance is terminated.
-
*
availability-zone
- The Availability Zone of the
* network interface.
-
description
- The
* description of the network interface.
-
*
group-id
- The ID of a security group associated with
* the network interface.
-
group-name
- The
* name of a security group associated with the network interface.
* -
mac-address
- The MAC address of the network
* interface.
-
network-interface-id
- The ID of
* the network interface.
-
owner-id
- The AWS
* account ID of the network interface owner.
-
*
private-ip-address
- The private IP address or
* addresses of the network interface.
-
*
private-dns-name
- The private DNS name of the network
* interface.
-
requester-id
- The ID of the
* entity that launched the instance on your behalf (for example, AWS
* Management Console, Auto Scaling, and so on).
-
*
requester-managed
- Indicates whether the network
* interface is being managed by an AWS service (for example, AWS
* Management Console, Auto Scaling, and so on).
-
*
source-desk-check
- Indicates whether the network
* interface performs source/destination checking. A value of
* true
means checking is enabled, and false
* means checking is disabled. The value must be false
for
* the network interface to perform Network Address Translation (NAT) in
* your VPC.
-
status
- The status of the
* network interface. If the network interface is not attached to an
* instance, the status is available
; if a network interface
* is attached to an instance the status is in-use
.
* -
subnet-id
- The ID of the subnet for the network
* interface.
-
tag
:key=value -
* The key/value combination of a tag assigned to the resource.
* -
tag-key
- The key of a tag assigned to the
* resource. This filter is independent of the tag-value
* filter. For example, if you use both the filter "tag-key=Purpose" and
* the filter "tag-value=X", you get any resources assigned both the tag
* key Purpose (regardless of what the tag's value is), and the tag value
* X (regardless of what the tag's key is). If you want to list only
* resources where Purpose is X, see the
* tag
:key=value filter.
-
*
tag-value
- The value of a tag assigned to the
* resource. This filter is independent of the tag-key
* filter.
-
vpc-id
- The ID of the VPC for the
* network interface.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param filters One or more filters.
-
*
addresses.private-ip-address
- The private IP
* addresses associated with the network interface.
-
*
addresses.primary
- Whether the private IP address is
* the primary IP address associated with the network interface.
* -
addresses.association.public-ip
- The association
* ID returned when the network interface was associated with the Elastic
* IP address.
-
addresses.association.owner-id
* - The owner ID of the addresses associated with the network interface.
*
-
association.association-id
- The
* association ID returned when the network interface was associated with
* an IP address.
-
association.allocation-id
-
* The allocation ID returned when you allocated the Elastic IP address
* for your network interface.
-
*
association.ip-owner-id
- The owner of the Elastic IP
* address associated with the network interface.
-
*
association.public-ip
- The address of the Elastic IP
* address bound to the network interface.
-
*
attachment.attachment-id
- The ID of the interface
* attachment.
-
attachment.instance-id
- The ID
* of the instance to which the network interface is attached.
-
*
attachment.instance-owner-id
- The owner ID of the
* instance to which the network interface is attached.
-
*
attachment.device-index
- The device index to which
* the network interface is attached.
-
*
attachment.status
- The status of the attachment
* (attaching
| attached
|
* detaching
| detached
).
-
*
attachment.attach.time
- The time that the network
* interface was attached to an instance.
-
*
attachment.delete-on-termination
- Indicates whether
* the attachment is deleted when an instance is terminated.
-
*
availability-zone
- The Availability Zone of the
* network interface.
-
description
- The
* description of the network interface.
-
*
group-id
- The ID of a security group associated with
* the network interface.
-
group-name
- The
* name of a security group associated with the network interface.
* -
mac-address
- The MAC address of the network
* interface.
-
network-interface-id
- The ID of
* the network interface.
-
owner-id
- The AWS
* account ID of the network interface owner.
-
*
private-ip-address
- The private IP address or
* addresses of the network interface.
-
*
private-dns-name
- The private DNS name of the network
* interface.
-
requester-id
- The ID of the
* entity that launched the instance on your behalf (for example, AWS
* Management Console, Auto Scaling, and so on).
-
*
requester-managed
- Indicates whether the network
* interface is being managed by an AWS service (for example, AWS
* Management Console, Auto Scaling, and so on).
-
*
source-desk-check
- Indicates whether the network
* interface performs source/destination checking. A value of
* true
means checking is enabled, and false
* means checking is disabled. The value must be false
for
* the network interface to perform Network Address Translation (NAT) in
* your VPC.
-
status
- The status of the
* network interface. If the network interface is not attached to an
* instance, the status is available
; if a network interface
* is attached to an instance the status is in-use
.
* -
subnet-id
- The ID of the subnet for the network
* interface.
-
tag
:key=value -
* The key/value combination of a tag assigned to the resource.
* -
tag-key
- The key of a tag assigned to the
* resource. This filter is independent of the tag-value
* filter. For example, if you use both the filter "tag-key=Purpose" and
* the filter "tag-value=X", you get any resources assigned both the tag
* key Purpose (regardless of what the tag's value is), and the tag value
* X (regardless of what the tag's key is). If you want to list only
* resources where Purpose is X, see the
* tag
:key=value filter.
-
*
tag-value
- The value of a tag assigned to the
* resource. This filter is independent of the tag-key
* filter.
-
vpc-id
- The ID of the VPC for the
* network interface.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public DescribeNetworkInterfacesRequest withFilters(Filter... filters) {
if (getFilters() == null) setFilters(new java.util.ArrayList(filters.length));
for (Filter value : filters) {
getFilters().add(value);
}
return this;
}
/**
* One or more filters. -
*
addresses.private-ip-address
- The private IP
* addresses associated with the network interface.
-
*
addresses.primary
- Whether the private IP address is
* the primary IP address associated with the network interface.
* -
addresses.association.public-ip
- The association
* ID returned when the network interface was associated with the Elastic
* IP address.
-
addresses.association.owner-id
* - The owner ID of the addresses associated with the network interface.
*
-
association.association-id
- The
* association ID returned when the network interface was associated with
* an IP address.
-
association.allocation-id
-
* The allocation ID returned when you allocated the Elastic IP address
* for your network interface.
-
*
association.ip-owner-id
- The owner of the Elastic IP
* address associated with the network interface.
-
*
association.public-ip
- The address of the Elastic IP
* address bound to the network interface.
-
*
attachment.attachment-id
- The ID of the interface
* attachment.
-
attachment.instance-id
- The ID
* of the instance to which the network interface is attached.
-
*
attachment.instance-owner-id
- The owner ID of the
* instance to which the network interface is attached.
-
*
attachment.device-index
- The device index to which
* the network interface is attached.
-
*
attachment.status
- The status of the attachment
* (attaching
| attached
|
* detaching
| detached
).
-
*
attachment.attach.time
- The time that the network
* interface was attached to an instance.
-
*
attachment.delete-on-termination
- Indicates whether
* the attachment is deleted when an instance is terminated.
-
*
availability-zone
- The Availability Zone of the
* network interface.
-
description
- The
* description of the network interface.
-
*
group-id
- The ID of a security group associated with
* the network interface.
-
group-name
- The
* name of a security group associated with the network interface.
* -
mac-address
- The MAC address of the network
* interface.
-
network-interface-id
- The ID of
* the network interface.
-
owner-id
- The AWS
* account ID of the network interface owner.
-
*
private-ip-address
- The private IP address or
* addresses of the network interface.
-
*
private-dns-name
- The private DNS name of the network
* interface.
-
requester-id
- The ID of the
* entity that launched the instance on your behalf (for example, AWS
* Management Console, Auto Scaling, and so on).
-
*
requester-managed
- Indicates whether the network
* interface is being managed by an AWS service (for example, AWS
* Management Console, Auto Scaling, and so on).
-
*
source-desk-check
- Indicates whether the network
* interface performs source/destination checking. A value of
* true
means checking is enabled, and false
* means checking is disabled. The value must be false
for
* the network interface to perform Network Address Translation (NAT) in
* your VPC.
-
status
- The status of the
* network interface. If the network interface is not attached to an
* instance, the status is available
; if a network interface
* is attached to an instance the status is in-use
.
* -
subnet-id
- The ID of the subnet for the network
* interface.
-
tag
:key=value -
* The key/value combination of a tag assigned to the resource.
* -
tag-key
- The key of a tag assigned to the
* resource. This filter is independent of the tag-value
* filter. For example, if you use both the filter "tag-key=Purpose" and
* the filter "tag-value=X", you get any resources assigned both the tag
* key Purpose (regardless of what the tag's value is), and the tag value
* X (regardless of what the tag's key is). If you want to list only
* resources where Purpose is X, see the
* tag
:key=value filter.
-
*
tag-value
- The value of a tag assigned to the
* resource. This filter is independent of the tag-key
* filter.
-
vpc-id
- The ID of the VPC for the
* network interface.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param filters One or more filters.
-
*
addresses.private-ip-address
- The private IP
* addresses associated with the network interface.
-
*
addresses.primary
- Whether the private IP address is
* the primary IP address associated with the network interface.
* -
addresses.association.public-ip
- The association
* ID returned when the network interface was associated with the Elastic
* IP address.
-
addresses.association.owner-id
* - The owner ID of the addresses associated with the network interface.
*
-
association.association-id
- The
* association ID returned when the network interface was associated with
* an IP address.
-
association.allocation-id
-
* The allocation ID returned when you allocated the Elastic IP address
* for your network interface.
-
*
association.ip-owner-id
- The owner of the Elastic IP
* address associated with the network interface.
-
*
association.public-ip
- The address of the Elastic IP
* address bound to the network interface.
-
*
attachment.attachment-id
- The ID of the interface
* attachment.
-
attachment.instance-id
- The ID
* of the instance to which the network interface is attached.
-
*
attachment.instance-owner-id
- The owner ID of the
* instance to which the network interface is attached.
-
*
attachment.device-index
- The device index to which
* the network interface is attached.
-
*
attachment.status
- The status of the attachment
* (attaching
| attached
|
* detaching
| detached
).
-
*
attachment.attach.time
- The time that the network
* interface was attached to an instance.
-
*
attachment.delete-on-termination
- Indicates whether
* the attachment is deleted when an instance is terminated.
-
*
availability-zone
- The Availability Zone of the
* network interface.
-
description
- The
* description of the network interface.
-
*
group-id
- The ID of a security group associated with
* the network interface.
-
group-name
- The
* name of a security group associated with the network interface.
* -
mac-address
- The MAC address of the network
* interface.
-
network-interface-id
- The ID of
* the network interface.
-
owner-id
- The AWS
* account ID of the network interface owner.
-
*
private-ip-address
- The private IP address or
* addresses of the network interface.
-
*
private-dns-name
- The private DNS name of the network
* interface.
-
requester-id
- The ID of the
* entity that launched the instance on your behalf (for example, AWS
* Management Console, Auto Scaling, and so on).
-
*
requester-managed
- Indicates whether the network
* interface is being managed by an AWS service (for example, AWS
* Management Console, Auto Scaling, and so on).
-
*
source-desk-check
- Indicates whether the network
* interface performs source/destination checking. A value of
* true
means checking is enabled, and false
* means checking is disabled. The value must be false
for
* the network interface to perform Network Address Translation (NAT) in
* your VPC.
-
status
- The status of the
* network interface. If the network interface is not attached to an
* instance, the status is available
; if a network interface
* is attached to an instance the status is in-use
.
* -
subnet-id
- The ID of the subnet for the network
* interface.
-
tag
:key=value -
* The key/value combination of a tag assigned to the resource.
* -
tag-key
- The key of a tag assigned to the
* resource. This filter is independent of the tag-value
* filter. For example, if you use both the filter "tag-key=Purpose" and
* the filter "tag-value=X", you get any resources assigned both the tag
* key Purpose (regardless of what the tag's value is), and the tag value
* X (regardless of what the tag's key is). If you want to list only
* resources where Purpose is X, see the
* tag
:key=value filter.
-
*
tag-value
- The value of a tag assigned to the
* resource. This filter is independent of the tag-key
* filter.
-
vpc-id
- The ID of the VPC for the
* network interface.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public DescribeNetworkInterfacesRequest withFilters(java.util.Collection filters) {
if (filters == null) {
this.filters = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag filtersCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(filters.size());
filtersCopy.addAll(filters);
this.filters = filtersCopy;
}
return this;
}
/**
* This method is intended for internal use only.
* Returns the marshaled request configured with additional parameters to
* enable operation dry-run.
*/
@Override
public Request getDryRunRequest() {
Request request = new DescribeNetworkInterfacesRequestMarshaller().marshall(this);
request.addParameter("DryRun", Boolean.toString(true));
return request;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getNetworkInterfaceIds() != null) sb.append("NetworkInterfaceIds: " + getNetworkInterfaceIds() + ",");
if (getFilters() != null) sb.append("Filters: " + getFilters() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getNetworkInterfaceIds() == null) ? 0 : getNetworkInterfaceIds().hashCode());
hashCode = prime * hashCode + ((getFilters() == null) ? 0 : getFilters().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof DescribeNetworkInterfacesRequest == false) return false;
DescribeNetworkInterfacesRequest other = (DescribeNetworkInterfacesRequest)obj;
if (other.getNetworkInterfaceIds() == null ^ this.getNetworkInterfaceIds() == null) return false;
if (other.getNetworkInterfaceIds() != null && other.getNetworkInterfaceIds().equals(this.getNetworkInterfaceIds()) == false) return false;
if (other.getFilters() == null ^ this.getFilters() == null) return false;
if (other.getFilters() != null && other.getFilters().equals(this.getFilters()) == false) return false;
return true;
}
}