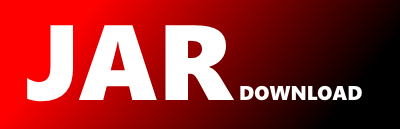
com.amazonaws.services.ec2.model.DescribeSpotInstanceRequestsRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.ec2.model;
import java.io.Serializable;
import com.amazonaws.AmazonWebServiceRequest;
import com.amazonaws.Request;
import com.amazonaws.services.ec2.model.transform.DescribeSpotInstanceRequestsRequestMarshaller;
/**
* Container for the parameters to the {@link com.amazonaws.services.ec2.AmazonEC2#describeSpotInstanceRequests(DescribeSpotInstanceRequestsRequest) DescribeSpotInstanceRequests operation}.
*
* Describes the Spot Instance requests that belong to your account. Spot
* Instances are instances that Amazon EC2 starts on your behalf when the
* maximum price that you specify exceeds the current Spot Price. Amazon
* EC2 periodically sets the Spot Price based on available Spot Instance
* capacity and current Spot Instance requests. For more information
* about Spot Instances, see
* Spot Instances
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* You can use DescribeSpotInstanceRequests
to find a
* running Spot Instance by examining the response. If the status of the
* Spot Instance is fulfilled
, the instance ID appears in
* the response and contains the identifier of the instance.
* Alternatively, you can use DescribeInstances with a filter to look for
* instances where the instance lifecycle is spot
.
*
*
* @see com.amazonaws.services.ec2.AmazonEC2#describeSpotInstanceRequests(DescribeSpotInstanceRequestsRequest)
*/
public class DescribeSpotInstanceRequestsRequest extends AmazonWebServiceRequest implements Serializable, DryRunSupportedRequest {
/**
* One or more Spot Instance request IDs.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag spotInstanceRequestIds;
/**
* One or more filters. -
availability-zone-group
* - The Availability Zone group.
-
create-time
* - The time stamp when the Spot Instance request was created.
* -
fault-code
- The fault code related to the
* request.
-
fault-message
- The fault message
* related to the request.
-
instance-id
- The
* ID of the instance that fulfilled the request.
-
*
launch-group
- The Spot Instance launch group.
* -
launch.block-device-mapping.delete-on-termination
* - Indicates whether the Amazon EBS volume is deleted on instance
* termination.
-
*
launch.block-device-mapping.device-name
- The device
* name for the Amazon EBS volume (for example, /dev/sdh
).
*
-
launch.block-device-mapping.snapshot-id
-
* The ID of the snapshot used for the Amazon EBS volume.
-
*
launch.block-device-mapping.volume-size
- The size of
* the Amazon EBS volume, in GiB.
-
*
launch.block-device-mapping.volume-type
- The type of
* the Amazon EBS volume (standard
| io1
).
*
-
launch.group-id
- The security group for
* the instance.
-
launch.image-id
- The ID of
* the AMI.
-
launch.instance-type
- The type of
* instance (for example, m1.small
).
-
*
launch.kernel-id
- The kernel ID.
-
*
launch.key-name
- The name of the key pair the
* instance launched with.
-
*
launch.monitoring-enabled
- Whether monitoring is
* enabled for the Spot Instance.
-
*
launch.ramdisk-id
- The RAM disk ID.
-
*
launch.network-interface.network-interface-id
- The ID
* of the network interface.
-
*
launch.network-interface.device-index
- The index of
* the device for the network interface attachment on the instance.
* -
launch.network-interface.subnet-id
- The ID of
* the subnet for the instance.
-
*
launch.network-interface.description
- A description
* of the network interface.
-
*
launch.network-interface.private-ip-address
- The
* primary private IP address of the network interface.
-
*
launch.network-interface.delete-on-termination
-
* Indicates whether the network interface is deleted when the instance
* is terminated.
-
*
launch.network-interface.group-id
- The ID of the
* security group associated with the network interface.
-
*
launch.network-interface.group-name
- The name of the
* security group associated with the network interface.
-
*
launch.network-interface.addresses.primary
- Indicates
* whether the IP address is the primary private IP address.
-
*
product-description
- The product description
* associated with the instance (Linux/UNIX
|
* Windows
).
-
*
spot-instance-request-id
- The Spot Instance request
* ID.
-
spot-price
- The maximum hourly price
* for any Spot Instance launched to fulfill the request.
-
*
state
- The state of the Spot Instance request
* (open
| active
| closed
|
* cancelled
| failed
).
-
*
status-code
- The short code describing the most
* recent evaluation of your Spot Instance request.
-
*
status-message
- The message explaining the status of
* the Spot Instance request.
-
*
tag
:key=value - The key/value
* combination of a tag assigned to the resource.
-
*
tag-key
- The key of a tag assigned to the resource.
* This filter is independent of the tag-value
filter. For
* example, if you use both the filter "tag-key=Purpose" and the filter
* "tag-value=X", you get any resources assigned both the tag key Purpose
* (regardless of what the tag's value is), and the tag value X
* (regardless of what the tag's key is). If you want to list only
* resources where Purpose is X, see the
* tag
:key=value filter.
-
*
tag-value
- The value of a tag assigned to the
* resource. This filter is independent of the tag-key
* filter.
-
type
- The type of Spot Instance
* request (one-time
| persistent
).
-
*
launched-availability-zone
- The Availability Zone in
* which the bid is launched.
-
valid-from
- The
* start date of the request.
-
valid-until
-
* The end date of the request.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag filters;
/**
* One or more Spot Instance request IDs.
*
* @return One or more Spot Instance request IDs.
*/
public java.util.List getSpotInstanceRequestIds() {
if (spotInstanceRequestIds == null) {
spotInstanceRequestIds = new com.amazonaws.internal.ListWithAutoConstructFlag();
spotInstanceRequestIds.setAutoConstruct(true);
}
return spotInstanceRequestIds;
}
/**
* One or more Spot Instance request IDs.
*
* @param spotInstanceRequestIds One or more Spot Instance request IDs.
*/
public void setSpotInstanceRequestIds(java.util.Collection spotInstanceRequestIds) {
if (spotInstanceRequestIds == null) {
this.spotInstanceRequestIds = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag spotInstanceRequestIdsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(spotInstanceRequestIds.size());
spotInstanceRequestIdsCopy.addAll(spotInstanceRequestIds);
this.spotInstanceRequestIds = spotInstanceRequestIdsCopy;
}
/**
* One or more Spot Instance request IDs.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param spotInstanceRequestIds One or more Spot Instance request IDs.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public DescribeSpotInstanceRequestsRequest withSpotInstanceRequestIds(String... spotInstanceRequestIds) {
if (getSpotInstanceRequestIds() == null) setSpotInstanceRequestIds(new java.util.ArrayList(spotInstanceRequestIds.length));
for (String value : spotInstanceRequestIds) {
getSpotInstanceRequestIds().add(value);
}
return this;
}
/**
* One or more Spot Instance request IDs.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param spotInstanceRequestIds One or more Spot Instance request IDs.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public DescribeSpotInstanceRequestsRequest withSpotInstanceRequestIds(java.util.Collection spotInstanceRequestIds) {
if (spotInstanceRequestIds == null) {
this.spotInstanceRequestIds = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag spotInstanceRequestIdsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(spotInstanceRequestIds.size());
spotInstanceRequestIdsCopy.addAll(spotInstanceRequestIds);
this.spotInstanceRequestIds = spotInstanceRequestIdsCopy;
}
return this;
}
/**
* One or more filters. -
availability-zone-group
* - The Availability Zone group.
-
create-time
* - The time stamp when the Spot Instance request was created.
* -
fault-code
- The fault code related to the
* request.
-
fault-message
- The fault message
* related to the request.
-
instance-id
- The
* ID of the instance that fulfilled the request.
-
*
launch-group
- The Spot Instance launch group.
* -
launch.block-device-mapping.delete-on-termination
* - Indicates whether the Amazon EBS volume is deleted on instance
* termination.
-
*
launch.block-device-mapping.device-name
- The device
* name for the Amazon EBS volume (for example, /dev/sdh
).
*
-
launch.block-device-mapping.snapshot-id
-
* The ID of the snapshot used for the Amazon EBS volume.
-
*
launch.block-device-mapping.volume-size
- The size of
* the Amazon EBS volume, in GiB.
-
*
launch.block-device-mapping.volume-type
- The type of
* the Amazon EBS volume (standard
| io1
).
*
-
launch.group-id
- The security group for
* the instance.
-
launch.image-id
- The ID of
* the AMI.
-
launch.instance-type
- The type of
* instance (for example, m1.small
).
-
*
launch.kernel-id
- The kernel ID.
-
*
launch.key-name
- The name of the key pair the
* instance launched with.
-
*
launch.monitoring-enabled
- Whether monitoring is
* enabled for the Spot Instance.
-
*
launch.ramdisk-id
- The RAM disk ID.
-
*
launch.network-interface.network-interface-id
- The ID
* of the network interface.
-
*
launch.network-interface.device-index
- The index of
* the device for the network interface attachment on the instance.
* -
launch.network-interface.subnet-id
- The ID of
* the subnet for the instance.
-
*
launch.network-interface.description
- A description
* of the network interface.
-
*
launch.network-interface.private-ip-address
- The
* primary private IP address of the network interface.
-
*
launch.network-interface.delete-on-termination
-
* Indicates whether the network interface is deleted when the instance
* is terminated.
-
*
launch.network-interface.group-id
- The ID of the
* security group associated with the network interface.
-
*
launch.network-interface.group-name
- The name of the
* security group associated with the network interface.
-
*
launch.network-interface.addresses.primary
- Indicates
* whether the IP address is the primary private IP address.
-
*
product-description
- The product description
* associated with the instance (Linux/UNIX
|
* Windows
).
-
*
spot-instance-request-id
- The Spot Instance request
* ID.
-
spot-price
- The maximum hourly price
* for any Spot Instance launched to fulfill the request.
-
*
state
- The state of the Spot Instance request
* (open
| active
| closed
|
* cancelled
| failed
).
-
*
status-code
- The short code describing the most
* recent evaluation of your Spot Instance request.
-
*
status-message
- The message explaining the status of
* the Spot Instance request.
-
*
tag
:key=value - The key/value
* combination of a tag assigned to the resource.
-
*
tag-key
- The key of a tag assigned to the resource.
* This filter is independent of the tag-value
filter. For
* example, if you use both the filter "tag-key=Purpose" and the filter
* "tag-value=X", you get any resources assigned both the tag key Purpose
* (regardless of what the tag's value is), and the tag value X
* (regardless of what the tag's key is). If you want to list only
* resources where Purpose is X, see the
* tag
:key=value filter.
-
*
tag-value
- The value of a tag assigned to the
* resource. This filter is independent of the tag-key
* filter.
-
type
- The type of Spot Instance
* request (one-time
| persistent
).
-
*
launched-availability-zone
- The Availability Zone in
* which the bid is launched.
-
valid-from
- The
* start date of the request.
-
valid-until
-
* The end date of the request.
*
* @return One or more filters. -
availability-zone-group
* - The Availability Zone group.
-
create-time
* - The time stamp when the Spot Instance request was created.
* -
fault-code
- The fault code related to the
* request.
-
fault-message
- The fault message
* related to the request.
-
instance-id
- The
* ID of the instance that fulfilled the request.
-
*
launch-group
- The Spot Instance launch group.
* -
launch.block-device-mapping.delete-on-termination
* - Indicates whether the Amazon EBS volume is deleted on instance
* termination.
-
*
launch.block-device-mapping.device-name
- The device
* name for the Amazon EBS volume (for example, /dev/sdh
).
*
-
launch.block-device-mapping.snapshot-id
-
* The ID of the snapshot used for the Amazon EBS volume.
-
*
launch.block-device-mapping.volume-size
- The size of
* the Amazon EBS volume, in GiB.
-
*
launch.block-device-mapping.volume-type
- The type of
* the Amazon EBS volume (standard
| io1
).
*
-
launch.group-id
- The security group for
* the instance.
-
launch.image-id
- The ID of
* the AMI.
-
launch.instance-type
- The type of
* instance (for example, m1.small
).
-
*
launch.kernel-id
- The kernel ID.
-
*
launch.key-name
- The name of the key pair the
* instance launched with.
-
*
launch.monitoring-enabled
- Whether monitoring is
* enabled for the Spot Instance.
-
*
launch.ramdisk-id
- The RAM disk ID.
-
*
launch.network-interface.network-interface-id
- The ID
* of the network interface.
-
*
launch.network-interface.device-index
- The index of
* the device for the network interface attachment on the instance.
* -
launch.network-interface.subnet-id
- The ID of
* the subnet for the instance.
-
*
launch.network-interface.description
- A description
* of the network interface.
-
*
launch.network-interface.private-ip-address
- The
* primary private IP address of the network interface.
-
*
launch.network-interface.delete-on-termination
-
* Indicates whether the network interface is deleted when the instance
* is terminated.
-
*
launch.network-interface.group-id
- The ID of the
* security group associated with the network interface.
-
*
launch.network-interface.group-name
- The name of the
* security group associated with the network interface.
-
*
launch.network-interface.addresses.primary
- Indicates
* whether the IP address is the primary private IP address.
-
*
product-description
- The product description
* associated with the instance (Linux/UNIX
|
* Windows
).
-
*
spot-instance-request-id
- The Spot Instance request
* ID.
-
spot-price
- The maximum hourly price
* for any Spot Instance launched to fulfill the request.
-
*
state
- The state of the Spot Instance request
* (open
| active
| closed
|
* cancelled
| failed
).
-
*
status-code
- The short code describing the most
* recent evaluation of your Spot Instance request.
-
*
status-message
- The message explaining the status of
* the Spot Instance request.
-
*
tag
:key=value - The key/value
* combination of a tag assigned to the resource.
-
*
tag-key
- The key of a tag assigned to the resource.
* This filter is independent of the tag-value
filter. For
* example, if you use both the filter "tag-key=Purpose" and the filter
* "tag-value=X", you get any resources assigned both the tag key Purpose
* (regardless of what the tag's value is), and the tag value X
* (regardless of what the tag's key is). If you want to list only
* resources where Purpose is X, see the
* tag
:key=value filter.
-
*
tag-value
- The value of a tag assigned to the
* resource. This filter is independent of the tag-key
* filter.
-
type
- The type of Spot Instance
* request (one-time
| persistent
).
-
*
launched-availability-zone
- The Availability Zone in
* which the bid is launched.
-
valid-from
- The
* start date of the request.
-
valid-until
-
* The end date of the request.
*/
public java.util.List getFilters() {
if (filters == null) {
filters = new com.amazonaws.internal.ListWithAutoConstructFlag();
filters.setAutoConstruct(true);
}
return filters;
}
/**
* One or more filters. -
availability-zone-group
* - The Availability Zone group.
-
create-time
* - The time stamp when the Spot Instance request was created.
* -
fault-code
- The fault code related to the
* request.
-
fault-message
- The fault message
* related to the request.
-
instance-id
- The
* ID of the instance that fulfilled the request.
-
*
launch-group
- The Spot Instance launch group.
* -
launch.block-device-mapping.delete-on-termination
* - Indicates whether the Amazon EBS volume is deleted on instance
* termination.
-
*
launch.block-device-mapping.device-name
- The device
* name for the Amazon EBS volume (for example, /dev/sdh
).
*
-
launch.block-device-mapping.snapshot-id
-
* The ID of the snapshot used for the Amazon EBS volume.
-
*
launch.block-device-mapping.volume-size
- The size of
* the Amazon EBS volume, in GiB.
-
*
launch.block-device-mapping.volume-type
- The type of
* the Amazon EBS volume (standard
| io1
).
*
-
launch.group-id
- The security group for
* the instance.
-
launch.image-id
- The ID of
* the AMI.
-
launch.instance-type
- The type of
* instance (for example, m1.small
).
-
*
launch.kernel-id
- The kernel ID.
-
*
launch.key-name
- The name of the key pair the
* instance launched with.
-
*
launch.monitoring-enabled
- Whether monitoring is
* enabled for the Spot Instance.
-
*
launch.ramdisk-id
- The RAM disk ID.
-
*
launch.network-interface.network-interface-id
- The ID
* of the network interface.
-
*
launch.network-interface.device-index
- The index of
* the device for the network interface attachment on the instance.
* -
launch.network-interface.subnet-id
- The ID of
* the subnet for the instance.
-
*
launch.network-interface.description
- A description
* of the network interface.
-
*
launch.network-interface.private-ip-address
- The
* primary private IP address of the network interface.
-
*
launch.network-interface.delete-on-termination
-
* Indicates whether the network interface is deleted when the instance
* is terminated.
-
*
launch.network-interface.group-id
- The ID of the
* security group associated with the network interface.
-
*
launch.network-interface.group-name
- The name of the
* security group associated with the network interface.
-
*
launch.network-interface.addresses.primary
- Indicates
* whether the IP address is the primary private IP address.
-
*
product-description
- The product description
* associated with the instance (Linux/UNIX
|
* Windows
).
-
*
spot-instance-request-id
- The Spot Instance request
* ID.
-
spot-price
- The maximum hourly price
* for any Spot Instance launched to fulfill the request.
-
*
state
- The state of the Spot Instance request
* (open
| active
| closed
|
* cancelled
| failed
).
-
*
status-code
- The short code describing the most
* recent evaluation of your Spot Instance request.
-
*
status-message
- The message explaining the status of
* the Spot Instance request.
-
*
tag
:key=value - The key/value
* combination of a tag assigned to the resource.
-
*
tag-key
- The key of a tag assigned to the resource.
* This filter is independent of the tag-value
filter. For
* example, if you use both the filter "tag-key=Purpose" and the filter
* "tag-value=X", you get any resources assigned both the tag key Purpose
* (regardless of what the tag's value is), and the tag value X
* (regardless of what the tag's key is). If you want to list only
* resources where Purpose is X, see the
* tag
:key=value filter.
-
*
tag-value
- The value of a tag assigned to the
* resource. This filter is independent of the tag-key
* filter.
-
type
- The type of Spot Instance
* request (one-time
| persistent
).
-
*
launched-availability-zone
- The Availability Zone in
* which the bid is launched.
-
valid-from
- The
* start date of the request.
-
valid-until
-
* The end date of the request.
*
* @param filters One or more filters. -
availability-zone-group
* - The Availability Zone group.
-
create-time
* - The time stamp when the Spot Instance request was created.
* -
fault-code
- The fault code related to the
* request.
-
fault-message
- The fault message
* related to the request.
-
instance-id
- The
* ID of the instance that fulfilled the request.
-
*
launch-group
- The Spot Instance launch group.
* -
launch.block-device-mapping.delete-on-termination
* - Indicates whether the Amazon EBS volume is deleted on instance
* termination.
-
*
launch.block-device-mapping.device-name
- The device
* name for the Amazon EBS volume (for example, /dev/sdh
).
*
-
launch.block-device-mapping.snapshot-id
-
* The ID of the snapshot used for the Amazon EBS volume.
-
*
launch.block-device-mapping.volume-size
- The size of
* the Amazon EBS volume, in GiB.
-
*
launch.block-device-mapping.volume-type
- The type of
* the Amazon EBS volume (standard
| io1
).
*
-
launch.group-id
- The security group for
* the instance.
-
launch.image-id
- The ID of
* the AMI.
-
launch.instance-type
- The type of
* instance (for example, m1.small
).
-
*
launch.kernel-id
- The kernel ID.
-
*
launch.key-name
- The name of the key pair the
* instance launched with.
-
*
launch.monitoring-enabled
- Whether monitoring is
* enabled for the Spot Instance.
-
*
launch.ramdisk-id
- The RAM disk ID.
-
*
launch.network-interface.network-interface-id
- The ID
* of the network interface.
-
*
launch.network-interface.device-index
- The index of
* the device for the network interface attachment on the instance.
* -
launch.network-interface.subnet-id
- The ID of
* the subnet for the instance.
-
*
launch.network-interface.description
- A description
* of the network interface.
-
*
launch.network-interface.private-ip-address
- The
* primary private IP address of the network interface.
-
*
launch.network-interface.delete-on-termination
-
* Indicates whether the network interface is deleted when the instance
* is terminated.
-
*
launch.network-interface.group-id
- The ID of the
* security group associated with the network interface.
-
*
launch.network-interface.group-name
- The name of the
* security group associated with the network interface.
-
*
launch.network-interface.addresses.primary
- Indicates
* whether the IP address is the primary private IP address.
-
*
product-description
- The product description
* associated with the instance (Linux/UNIX
|
* Windows
).
-
*
spot-instance-request-id
- The Spot Instance request
* ID.
-
spot-price
- The maximum hourly price
* for any Spot Instance launched to fulfill the request.
-
*
state
- The state of the Spot Instance request
* (open
| active
| closed
|
* cancelled
| failed
).
-
*
status-code
- The short code describing the most
* recent evaluation of your Spot Instance request.
-
*
status-message
- The message explaining the status of
* the Spot Instance request.
-
*
tag
:key=value - The key/value
* combination of a tag assigned to the resource.
-
*
tag-key
- The key of a tag assigned to the resource.
* This filter is independent of the tag-value
filter. For
* example, if you use both the filter "tag-key=Purpose" and the filter
* "tag-value=X", you get any resources assigned both the tag key Purpose
* (regardless of what the tag's value is), and the tag value X
* (regardless of what the tag's key is). If you want to list only
* resources where Purpose is X, see the
* tag
:key=value filter.
-
*
tag-value
- The value of a tag assigned to the
* resource. This filter is independent of the tag-key
* filter.
-
type
- The type of Spot Instance
* request (one-time
| persistent
).
-
*
launched-availability-zone
- The Availability Zone in
* which the bid is launched.
-
valid-from
- The
* start date of the request.
-
valid-until
-
* The end date of the request.
*/
public void setFilters(java.util.Collection filters) {
if (filters == null) {
this.filters = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag filtersCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(filters.size());
filtersCopy.addAll(filters);
this.filters = filtersCopy;
}
/**
* One or more filters. -
availability-zone-group
* - The Availability Zone group.
-
create-time
* - The time stamp when the Spot Instance request was created.
* -
fault-code
- The fault code related to the
* request.
-
fault-message
- The fault message
* related to the request.
-
instance-id
- The
* ID of the instance that fulfilled the request.
-
*
launch-group
- The Spot Instance launch group.
* -
launch.block-device-mapping.delete-on-termination
* - Indicates whether the Amazon EBS volume is deleted on instance
* termination.
-
*
launch.block-device-mapping.device-name
- The device
* name for the Amazon EBS volume (for example, /dev/sdh
).
*
-
launch.block-device-mapping.snapshot-id
-
* The ID of the snapshot used for the Amazon EBS volume.
-
*
launch.block-device-mapping.volume-size
- The size of
* the Amazon EBS volume, in GiB.
-
*
launch.block-device-mapping.volume-type
- The type of
* the Amazon EBS volume (standard
| io1
).
*
-
launch.group-id
- The security group for
* the instance.
-
launch.image-id
- The ID of
* the AMI.
-
launch.instance-type
- The type of
* instance (for example, m1.small
).
-
*
launch.kernel-id
- The kernel ID.
-
*
launch.key-name
- The name of the key pair the
* instance launched with.
-
*
launch.monitoring-enabled
- Whether monitoring is
* enabled for the Spot Instance.
-
*
launch.ramdisk-id
- The RAM disk ID.
-
*
launch.network-interface.network-interface-id
- The ID
* of the network interface.
-
*
launch.network-interface.device-index
- The index of
* the device for the network interface attachment on the instance.
* -
launch.network-interface.subnet-id
- The ID of
* the subnet for the instance.
-
*
launch.network-interface.description
- A description
* of the network interface.
-
*
launch.network-interface.private-ip-address
- The
* primary private IP address of the network interface.
-
*
launch.network-interface.delete-on-termination
-
* Indicates whether the network interface is deleted when the instance
* is terminated.
-
*
launch.network-interface.group-id
- The ID of the
* security group associated with the network interface.
-
*
launch.network-interface.group-name
- The name of the
* security group associated with the network interface.
-
*
launch.network-interface.addresses.primary
- Indicates
* whether the IP address is the primary private IP address.
-
*
product-description
- The product description
* associated with the instance (Linux/UNIX
|
* Windows
).
-
*
spot-instance-request-id
- The Spot Instance request
* ID.
-
spot-price
- The maximum hourly price
* for any Spot Instance launched to fulfill the request.
-
*
state
- The state of the Spot Instance request
* (open
| active
| closed
|
* cancelled
| failed
).
-
*
status-code
- The short code describing the most
* recent evaluation of your Spot Instance request.
-
*
status-message
- The message explaining the status of
* the Spot Instance request.
-
*
tag
:key=value - The key/value
* combination of a tag assigned to the resource.
-
*
tag-key
- The key of a tag assigned to the resource.
* This filter is independent of the tag-value
filter. For
* example, if you use both the filter "tag-key=Purpose" and the filter
* "tag-value=X", you get any resources assigned both the tag key Purpose
* (regardless of what the tag's value is), and the tag value X
* (regardless of what the tag's key is). If you want to list only
* resources where Purpose is X, see the
* tag
:key=value filter.
-
*
tag-value
- The value of a tag assigned to the
* resource. This filter is independent of the tag-key
* filter.
-
type
- The type of Spot Instance
* request (one-time
| persistent
).
-
*
launched-availability-zone
- The Availability Zone in
* which the bid is launched.
-
valid-from
- The
* start date of the request.
-
valid-until
-
* The end date of the request.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param filters One or more filters.
-
availability-zone-group
* - The Availability Zone group.
-
create-time
* - The time stamp when the Spot Instance request was created.
* -
fault-code
- The fault code related to the
* request.
-
fault-message
- The fault message
* related to the request.
-
instance-id
- The
* ID of the instance that fulfilled the request.
-
*
launch-group
- The Spot Instance launch group.
* -
launch.block-device-mapping.delete-on-termination
* - Indicates whether the Amazon EBS volume is deleted on instance
* termination.
-
*
launch.block-device-mapping.device-name
- The device
* name for the Amazon EBS volume (for example, /dev/sdh
).
*
-
launch.block-device-mapping.snapshot-id
-
* The ID of the snapshot used for the Amazon EBS volume.
-
*
launch.block-device-mapping.volume-size
- The size of
* the Amazon EBS volume, in GiB.
-
*
launch.block-device-mapping.volume-type
- The type of
* the Amazon EBS volume (standard
| io1
).
*
-
launch.group-id
- The security group for
* the instance.
-
launch.image-id
- The ID of
* the AMI.
-
launch.instance-type
- The type of
* instance (for example, m1.small
).
-
*
launch.kernel-id
- The kernel ID.
-
*
launch.key-name
- The name of the key pair the
* instance launched with.
-
*
launch.monitoring-enabled
- Whether monitoring is
* enabled for the Spot Instance.
-
*
launch.ramdisk-id
- The RAM disk ID.
-
*
launch.network-interface.network-interface-id
- The ID
* of the network interface.
-
*
launch.network-interface.device-index
- The index of
* the device for the network interface attachment on the instance.
* -
launch.network-interface.subnet-id
- The ID of
* the subnet for the instance.
-
*
launch.network-interface.description
- A description
* of the network interface.
-
*
launch.network-interface.private-ip-address
- The
* primary private IP address of the network interface.
-
*
launch.network-interface.delete-on-termination
-
* Indicates whether the network interface is deleted when the instance
* is terminated.
-
*
launch.network-interface.group-id
- The ID of the
* security group associated with the network interface.
-
*
launch.network-interface.group-name
- The name of the
* security group associated with the network interface.
-
*
launch.network-interface.addresses.primary
- Indicates
* whether the IP address is the primary private IP address.
-
*
product-description
- The product description
* associated with the instance (Linux/UNIX
|
* Windows
).
-
*
spot-instance-request-id
- The Spot Instance request
* ID.
-
spot-price
- The maximum hourly price
* for any Spot Instance launched to fulfill the request.
-
*
state
- The state of the Spot Instance request
* (open
| active
| closed
|
* cancelled
| failed
).
-
*
status-code
- The short code describing the most
* recent evaluation of your Spot Instance request.
-
*
status-message
- The message explaining the status of
* the Spot Instance request.
-
*
tag
:key=value - The key/value
* combination of a tag assigned to the resource.
-
*
tag-key
- The key of a tag assigned to the resource.
* This filter is independent of the tag-value
filter. For
* example, if you use both the filter "tag-key=Purpose" and the filter
* "tag-value=X", you get any resources assigned both the tag key Purpose
* (regardless of what the tag's value is), and the tag value X
* (regardless of what the tag's key is). If you want to list only
* resources where Purpose is X, see the
* tag
:key=value filter.
-
*
tag-value
- The value of a tag assigned to the
* resource. This filter is independent of the tag-key
* filter.
-
type
- The type of Spot Instance
* request (one-time
| persistent
).
-
*
launched-availability-zone
- The Availability Zone in
* which the bid is launched.
-
valid-from
- The
* start date of the request.
-
valid-until
-
* The end date of the request.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public DescribeSpotInstanceRequestsRequest withFilters(Filter... filters) {
if (getFilters() == null) setFilters(new java.util.ArrayList(filters.length));
for (Filter value : filters) {
getFilters().add(value);
}
return this;
}
/**
* One or more filters. -
availability-zone-group
* - The Availability Zone group.
-
create-time
* - The time stamp when the Spot Instance request was created.
* -
fault-code
- The fault code related to the
* request.
-
fault-message
- The fault message
* related to the request.
-
instance-id
- The
* ID of the instance that fulfilled the request.
-
*
launch-group
- The Spot Instance launch group.
* -
launch.block-device-mapping.delete-on-termination
* - Indicates whether the Amazon EBS volume is deleted on instance
* termination.
-
*
launch.block-device-mapping.device-name
- The device
* name for the Amazon EBS volume (for example, /dev/sdh
).
*
-
launch.block-device-mapping.snapshot-id
-
* The ID of the snapshot used for the Amazon EBS volume.
-
*
launch.block-device-mapping.volume-size
- The size of
* the Amazon EBS volume, in GiB.
-
*
launch.block-device-mapping.volume-type
- The type of
* the Amazon EBS volume (standard
| io1
).
*
-
launch.group-id
- The security group for
* the instance.
-
launch.image-id
- The ID of
* the AMI.
-
launch.instance-type
- The type of
* instance (for example, m1.small
).
-
*
launch.kernel-id
- The kernel ID.
-
*
launch.key-name
- The name of the key pair the
* instance launched with.
-
*
launch.monitoring-enabled
- Whether monitoring is
* enabled for the Spot Instance.
-
*
launch.ramdisk-id
- The RAM disk ID.
-
*
launch.network-interface.network-interface-id
- The ID
* of the network interface.
-
*
launch.network-interface.device-index
- The index of
* the device for the network interface attachment on the instance.
* -
launch.network-interface.subnet-id
- The ID of
* the subnet for the instance.
-
*
launch.network-interface.description
- A description
* of the network interface.
-
*
launch.network-interface.private-ip-address
- The
* primary private IP address of the network interface.
-
*
launch.network-interface.delete-on-termination
-
* Indicates whether the network interface is deleted when the instance
* is terminated.
-
*
launch.network-interface.group-id
- The ID of the
* security group associated with the network interface.
-
*
launch.network-interface.group-name
- The name of the
* security group associated with the network interface.
-
*
launch.network-interface.addresses.primary
- Indicates
* whether the IP address is the primary private IP address.
-
*
product-description
- The product description
* associated with the instance (Linux/UNIX
|
* Windows
).
-
*
spot-instance-request-id
- The Spot Instance request
* ID.
-
spot-price
- The maximum hourly price
* for any Spot Instance launched to fulfill the request.
-
*
state
- The state of the Spot Instance request
* (open
| active
| closed
|
* cancelled
| failed
).
-
*
status-code
- The short code describing the most
* recent evaluation of your Spot Instance request.
-
*
status-message
- The message explaining the status of
* the Spot Instance request.
-
*
tag
:key=value - The key/value
* combination of a tag assigned to the resource.
-
*
tag-key
- The key of a tag assigned to the resource.
* This filter is independent of the tag-value
filter. For
* example, if you use both the filter "tag-key=Purpose" and the filter
* "tag-value=X", you get any resources assigned both the tag key Purpose
* (regardless of what the tag's value is), and the tag value X
* (regardless of what the tag's key is). If you want to list only
* resources where Purpose is X, see the
* tag
:key=value filter.
-
*
tag-value
- The value of a tag assigned to the
* resource. This filter is independent of the tag-key
* filter.
-
type
- The type of Spot Instance
* request (one-time
| persistent
).
-
*
launched-availability-zone
- The Availability Zone in
* which the bid is launched.
-
valid-from
- The
* start date of the request.
-
valid-until
-
* The end date of the request.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param filters One or more filters.
-
availability-zone-group
* - The Availability Zone group.
-
create-time
* - The time stamp when the Spot Instance request was created.
* -
fault-code
- The fault code related to the
* request.
-
fault-message
- The fault message
* related to the request.
-
instance-id
- The
* ID of the instance that fulfilled the request.
-
*
launch-group
- The Spot Instance launch group.
* -
launch.block-device-mapping.delete-on-termination
* - Indicates whether the Amazon EBS volume is deleted on instance
* termination.
-
*
launch.block-device-mapping.device-name
- The device
* name for the Amazon EBS volume (for example, /dev/sdh
).
*
-
launch.block-device-mapping.snapshot-id
-
* The ID of the snapshot used for the Amazon EBS volume.
-
*
launch.block-device-mapping.volume-size
- The size of
* the Amazon EBS volume, in GiB.
-
*
launch.block-device-mapping.volume-type
- The type of
* the Amazon EBS volume (standard
| io1
).
*
-
launch.group-id
- The security group for
* the instance.
-
launch.image-id
- The ID of
* the AMI.
-
launch.instance-type
- The type of
* instance (for example, m1.small
).
-
*
launch.kernel-id
- The kernel ID.
-
*
launch.key-name
- The name of the key pair the
* instance launched with.
-
*
launch.monitoring-enabled
- Whether monitoring is
* enabled for the Spot Instance.
-
*
launch.ramdisk-id
- The RAM disk ID.
-
*
launch.network-interface.network-interface-id
- The ID
* of the network interface.
-
*
launch.network-interface.device-index
- The index of
* the device for the network interface attachment on the instance.
* -
launch.network-interface.subnet-id
- The ID of
* the subnet for the instance.
-
*
launch.network-interface.description
- A description
* of the network interface.
-
*
launch.network-interface.private-ip-address
- The
* primary private IP address of the network interface.
-
*
launch.network-interface.delete-on-termination
-
* Indicates whether the network interface is deleted when the instance
* is terminated.
-
*
launch.network-interface.group-id
- The ID of the
* security group associated with the network interface.
-
*
launch.network-interface.group-name
- The name of the
* security group associated with the network interface.
-
*
launch.network-interface.addresses.primary
- Indicates
* whether the IP address is the primary private IP address.
-
*
product-description
- The product description
* associated with the instance (Linux/UNIX
|
* Windows
).
-
*
spot-instance-request-id
- The Spot Instance request
* ID.
-
spot-price
- The maximum hourly price
* for any Spot Instance launched to fulfill the request.
-
*
state
- The state of the Spot Instance request
* (open
| active
| closed
|
* cancelled
| failed
).
-
*
status-code
- The short code describing the most
* recent evaluation of your Spot Instance request.
-
*
status-message
- The message explaining the status of
* the Spot Instance request.
-
*
tag
:key=value - The key/value
* combination of a tag assigned to the resource.
-
*
tag-key
- The key of a tag assigned to the resource.
* This filter is independent of the tag-value
filter. For
* example, if you use both the filter "tag-key=Purpose" and the filter
* "tag-value=X", you get any resources assigned both the tag key Purpose
* (regardless of what the tag's value is), and the tag value X
* (regardless of what the tag's key is). If you want to list only
* resources where Purpose is X, see the
* tag
:key=value filter.
-
*
tag-value
- The value of a tag assigned to the
* resource. This filter is independent of the tag-key
* filter.
-
type
- The type of Spot Instance
* request (one-time
| persistent
).
-
*
launched-availability-zone
- The Availability Zone in
* which the bid is launched.
-
valid-from
- The
* start date of the request.
-
valid-until
-
* The end date of the request.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public DescribeSpotInstanceRequestsRequest withFilters(java.util.Collection filters) {
if (filters == null) {
this.filters = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag filtersCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(filters.size());
filtersCopy.addAll(filters);
this.filters = filtersCopy;
}
return this;
}
/**
* This method is intended for internal use only.
* Returns the marshaled request configured with additional parameters to
* enable operation dry-run.
*/
@Override
public Request getDryRunRequest() {
Request request = new DescribeSpotInstanceRequestsRequestMarshaller().marshall(this);
request.addParameter("DryRun", Boolean.toString(true));
return request;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getSpotInstanceRequestIds() != null) sb.append("SpotInstanceRequestIds: " + getSpotInstanceRequestIds() + ",");
if (getFilters() != null) sb.append("Filters: " + getFilters() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getSpotInstanceRequestIds() == null) ? 0 : getSpotInstanceRequestIds().hashCode());
hashCode = prime * hashCode + ((getFilters() == null) ? 0 : getFilters().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof DescribeSpotInstanceRequestsRequest == false) return false;
DescribeSpotInstanceRequestsRequest other = (DescribeSpotInstanceRequestsRequest)obj;
if (other.getSpotInstanceRequestIds() == null ^ this.getSpotInstanceRequestIds() == null) return false;
if (other.getSpotInstanceRequestIds() != null && other.getSpotInstanceRequestIds().equals(this.getSpotInstanceRequestIds()) == false) return false;
if (other.getFilters() == null ^ this.getFilters() == null) return false;
if (other.getFilters() != null && other.getFilters().equals(this.getFilters()) == false) return false;
return true;
}
}