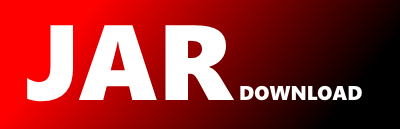
com.amazonaws.services.ec2.model.RunInstancesRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.ec2.model;
import java.io.Serializable;
import com.amazonaws.AmazonWebServiceRequest;
import com.amazonaws.Request;
import com.amazonaws.services.ec2.model.transform.RunInstancesRequestMarshaller;
/**
* Container for the parameters to the {@link com.amazonaws.services.ec2.AmazonEC2#runInstances(RunInstancesRequest) RunInstances operation}.
*
* Launches the specified number of instances using an AMI for which you
* have permissions.
*
*
* When you launch an instance, it enters the pending
state.
* After the instance is ready for you, it enters the
* running
state. To check the state of your instance, call
* DescribeInstances.
*
*
* If you don't specify a security group when launching an instance,
* Amazon EC2 uses the default security group. For more information, see
* Security Groups
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* Linux instances have access to the public key of the key pair at boot.
* You can use this key to provide secure access to the instance. Amazon
* EC2 public images use this feature to provide secure access without
* passwords. For more information, see
* Key Pairs
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* You can provide optional user data when launching an instance. For
* more information, see
* Instance Metadata
* in the Amazon Elastic Compute Cloud User Guide .
*
*
* If any of the AMIs have a product code attached for which the user has
* not subscribed, RunInstances
fails.
*
*
* @see com.amazonaws.services.ec2.AmazonEC2#runInstances(RunInstancesRequest)
*/
public class RunInstancesRequest extends AmazonWebServiceRequest implements Serializable, DryRunSupportedRequest {
/**
* The ID of the AMI, which you can get by calling DescribeImages.
*/
private String imageId;
/**
* The minimum number of instances to launch. If you specify a minimum
* that is more instances than Amazon EC2 can launch in the target
* Availability Zone, Amazon EC2 launches no instances. Constraints:
* Between 1 and the maximum number you're allowed for the specified
* instance type. For more information about the default limits, and how
* to request an increase, see How
* many instances can I run in Amazon EC2 in the Amazon EC2 General
* FAQ.
*/
private Integer minCount;
/**
* The maximum number of instances to launch. If you specify more
* instances than Amazon EC2 can launch in the target Availability Zone,
* Amazon EC2 launches the largest possible number of instances above
* MinCount
.
Constraints: Between 1 and the maximum
* number you're allowed for the specified instance type. For more
* information about the default limits, and how to request an increase,
* see How
* many instances can I run in Amazon EC2 in the Amazon EC2 General
* FAQ.
*/
private Integer maxCount;
/**
* The name of the key pair. You can create a key pair using
* CreateKeyPair or ImportKeyPair. If you
* launch an instance without specifying a key pair, you can't connect to
* the instance.
*/
private String keyName;
/**
* [EC2-Classic, default VPC] One or more security group names. For a
* nondefault VPC, you must use security group IDs instead.
Default:
* Amazon EC2 uses the default security group.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag securityGroups;
/**
* One or more security group IDs. You can create a security group using
* CreateSecurityGroup. Default: Amazon EC2 uses the default
* security group.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag securityGroupIds;
/**
* The Base64-encoded MIME user data for the instances.
*/
private String userData;
/**
* The instance type. For more information, see Instance
* Types in the Amazon Elastic Compute Cloud User Guide.
* Default: m1.small
*
* Constraints:
* Allowed Values: t1.micro, m1.small, m1.medium, m1.large, m1.xlarge, m3.medium, m3.large, m3.xlarge, m3.2xlarge, m2.xlarge, m2.2xlarge, m2.4xlarge, cr1.8xlarge, i2.xlarge, i2.2xlarge, i2.4xlarge, i2.8xlarge, hi1.4xlarge, hs1.8xlarge, c1.medium, c1.xlarge, c3.large, c3.xlarge, c3.2xlarge, c3.4xlarge, c3.8xlarge, cc1.4xlarge, cc2.8xlarge, g2.2xlarge, cg1.4xlarge
*/
private String instanceType;
/**
* The placement for the instance.
*/
private Placement placement;
/**
* The ID of the kernel. We recommend that you use PV-GRUB
* instead of kernels and RAM disks. For more information, see
* PV-GRUB: A New Amazon Kernel Image in the Amazon Elastic
* Compute Cloud User Guide.
*/
private String kernelId;
/**
* The ID of the RAM disk.
*/
private String ramdiskId;
/**
* The block device mapping.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag blockDeviceMappings;
/**
* The monitoring for the instance.
*/
private Boolean monitoring;
/**
* [EC2-VPC] The ID of the subnet to launch the instance into.
*/
private String subnetId;
/**
* If you set this parameter to true
, you can't terminate
* the instance using the Amazon EC2 console, CLI, or API; otherwise, you
* can. If you set this parameter to true
and then later
* want to be able to terminate the instance, you must first change the
* value of the disableApiTermination
attribute to
* false
using ModifyInstanceAttribute.
* Alternatively, if you set
* InstanceInitiatedShutdownBehavior
to
* terminate
, you can terminate the instance by running the
* shutdown command from the instance. Default: false
*/
private Boolean disableApiTermination;
/**
* Indicates whether an instance stops or terminates when you initiate
* shutdown from the instance (using the operating system command for
* system shutdown).
Default: stop
*
* Constraints:
* Allowed Values: stop, terminate
*/
private String instanceInitiatedShutdownBehavior;
/**
* [EC2-VPC] The primary IP address. You must specify a value from the IP
* address range of the subnet.
Only one private IP address can be
* designated as primary. Therefore, you can't specify this parameter if
* PrivateIpAddresses.n.Primary
is set to true
* and PrivateIpAddresses.n.PrivateIpAddress
is set to an IP
* address.
Default: We select an IP address from the IP address range
* of the subnet.
*/
private String privateIpAddress;
/**
* Unique, case-sensitive identifier you provide to ensure the
* idempotency of the request. For more information, see How
* to Ensure Idempotency in the Amazon Elastic Compute Cloud User
* Guide.
Constraints: Maximum 64 ASCII characters
*/
private String clientToken;
/**
*
*/
private String additionalInfo;
/**
* One or more network interfaces.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag networkInterfaces;
/**
* The IAM instance profile.
*/
private IamInstanceProfileSpecification iamInstanceProfile;
/**
* Indicates whether the instance is optimized for EBS I/O. This
* optimization provides dedicated throughput to Amazon EBS and an
* optimized configuration stack to provide optimal Amazon EBS I/O
* performance. This optimization isn't available with all instance
* types. Additional usage charges apply when using an EBS-optimized
* instance. Default: false
*/
private Boolean ebsOptimized;
/**
* Default constructor for a new RunInstancesRequest object. Callers should use the
* setter or fluent setter (with...) methods to initialize this object after creating it.
*/
public RunInstancesRequest() {}
/**
* Constructs a new RunInstancesRequest object.
* Callers should use the setter or fluent setter (with...) methods to
* initialize any additional object members.
*
* @param imageId The ID of the AMI, which you can get by calling
* DescribeImages.
* @param minCount The minimum number of instances to launch. If you
* specify a minimum that is more instances than Amazon EC2 can launch in
* the target Availability Zone, Amazon EC2 launches no instances.
*
Constraints: Between 1 and the maximum number you're allowed for
* the specified instance type. For more information about the default
* limits, and how to request an increase, see How
* many instances can I run in Amazon EC2 in the Amazon EC2 General
* FAQ.
* @param maxCount The maximum number of instances to launch. If you
* specify more instances than Amazon EC2 can launch in the target
* Availability Zone, Amazon EC2 launches the largest possible number of
* instances above MinCount
.
Constraints: Between 1 and
* the maximum number you're allowed for the specified instance type. For
* more information about the default limits, and how to request an
* increase, see How
* many instances can I run in Amazon EC2 in the Amazon EC2 General
* FAQ.
*/
public RunInstancesRequest(String imageId, Integer minCount, Integer maxCount) {
setImageId(imageId);
setMinCount(minCount);
setMaxCount(maxCount);
}
/**
* The ID of the AMI, which you can get by calling DescribeImages.
*
* @return The ID of the AMI, which you can get by calling DescribeImages.
*/
public String getImageId() {
return imageId;
}
/**
* The ID of the AMI, which you can get by calling DescribeImages.
*
* @param imageId The ID of the AMI, which you can get by calling DescribeImages.
*/
public void setImageId(String imageId) {
this.imageId = imageId;
}
/**
* The ID of the AMI, which you can get by calling DescribeImages.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param imageId The ID of the AMI, which you can get by calling DescribeImages.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RunInstancesRequest withImageId(String imageId) {
this.imageId = imageId;
return this;
}
/**
* The minimum number of instances to launch. If you specify a minimum
* that is more instances than Amazon EC2 can launch in the target
* Availability Zone, Amazon EC2 launches no instances.
Constraints:
* Between 1 and the maximum number you're allowed for the specified
* instance type. For more information about the default limits, and how
* to request an increase, see How
* many instances can I run in Amazon EC2 in the Amazon EC2 General
* FAQ.
*
* @return The minimum number of instances to launch. If you specify a minimum
* that is more instances than Amazon EC2 can launch in the target
* Availability Zone, Amazon EC2 launches no instances.
Constraints:
* Between 1 and the maximum number you're allowed for the specified
* instance type. For more information about the default limits, and how
* to request an increase, see How
* many instances can I run in Amazon EC2 in the Amazon EC2 General
* FAQ.
*/
public Integer getMinCount() {
return minCount;
}
/**
* The minimum number of instances to launch. If you specify a minimum
* that is more instances than Amazon EC2 can launch in the target
* Availability Zone, Amazon EC2 launches no instances.
Constraints:
* Between 1 and the maximum number you're allowed for the specified
* instance type. For more information about the default limits, and how
* to request an increase, see How
* many instances can I run in Amazon EC2 in the Amazon EC2 General
* FAQ.
*
* @param minCount The minimum number of instances to launch. If you specify a minimum
* that is more instances than Amazon EC2 can launch in the target
* Availability Zone, Amazon EC2 launches no instances.
Constraints:
* Between 1 and the maximum number you're allowed for the specified
* instance type. For more information about the default limits, and how
* to request an increase, see How
* many instances can I run in Amazon EC2 in the Amazon EC2 General
* FAQ.
*/
public void setMinCount(Integer minCount) {
this.minCount = minCount;
}
/**
* The minimum number of instances to launch. If you specify a minimum
* that is more instances than Amazon EC2 can launch in the target
* Availability Zone, Amazon EC2 launches no instances.
Constraints:
* Between 1 and the maximum number you're allowed for the specified
* instance type. For more information about the default limits, and how
* to request an increase, see How
* many instances can I run in Amazon EC2 in the Amazon EC2 General
* FAQ.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param minCount The minimum number of instances to launch. If you specify a minimum
* that is more instances than Amazon EC2 can launch in the target
* Availability Zone, Amazon EC2 launches no instances.
Constraints:
* Between 1 and the maximum number you're allowed for the specified
* instance type. For more information about the default limits, and how
* to request an increase, see How
* many instances can I run in Amazon EC2 in the Amazon EC2 General
* FAQ.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RunInstancesRequest withMinCount(Integer minCount) {
this.minCount = minCount;
return this;
}
/**
* The maximum number of instances to launch. If you specify more
* instances than Amazon EC2 can launch in the target Availability Zone,
* Amazon EC2 launches the largest possible number of instances above
* MinCount
.
Constraints: Between 1 and the maximum
* number you're allowed for the specified instance type. For more
* information about the default limits, and how to request an increase,
* see How
* many instances can I run in Amazon EC2 in the Amazon EC2 General
* FAQ.
*
* @return The maximum number of instances to launch. If you specify more
* instances than Amazon EC2 can launch in the target Availability Zone,
* Amazon EC2 launches the largest possible number of instances above
* MinCount
.
Constraints: Between 1 and the maximum
* number you're allowed for the specified instance type. For more
* information about the default limits, and how to request an increase,
* see How
* many instances can I run in Amazon EC2 in the Amazon EC2 General
* FAQ.
*/
public Integer getMaxCount() {
return maxCount;
}
/**
* The maximum number of instances to launch. If you specify more
* instances than Amazon EC2 can launch in the target Availability Zone,
* Amazon EC2 launches the largest possible number of instances above
* MinCount
.
Constraints: Between 1 and the maximum
* number you're allowed for the specified instance type. For more
* information about the default limits, and how to request an increase,
* see How
* many instances can I run in Amazon EC2 in the Amazon EC2 General
* FAQ.
*
* @param maxCount The maximum number of instances to launch. If you specify more
* instances than Amazon EC2 can launch in the target Availability Zone,
* Amazon EC2 launches the largest possible number of instances above
* MinCount
.
Constraints: Between 1 and the maximum
* number you're allowed for the specified instance type. For more
* information about the default limits, and how to request an increase,
* see How
* many instances can I run in Amazon EC2 in the Amazon EC2 General
* FAQ.
*/
public void setMaxCount(Integer maxCount) {
this.maxCount = maxCount;
}
/**
* The maximum number of instances to launch. If you specify more
* instances than Amazon EC2 can launch in the target Availability Zone,
* Amazon EC2 launches the largest possible number of instances above
* MinCount
.
Constraints: Between 1 and the maximum
* number you're allowed for the specified instance type. For more
* information about the default limits, and how to request an increase,
* see How
* many instances can I run in Amazon EC2 in the Amazon EC2 General
* FAQ.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param maxCount The maximum number of instances to launch. If you specify more
* instances than Amazon EC2 can launch in the target Availability Zone,
* Amazon EC2 launches the largest possible number of instances above
* MinCount
.
Constraints: Between 1 and the maximum
* number you're allowed for the specified instance type. For more
* information about the default limits, and how to request an increase,
* see How
* many instances can I run in Amazon EC2 in the Amazon EC2 General
* FAQ.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RunInstancesRequest withMaxCount(Integer maxCount) {
this.maxCount = maxCount;
return this;
}
/**
* The name of the key pair. You can create a key pair using
* CreateKeyPair or ImportKeyPair. If you
* launch an instance without specifying a key pair, you can't connect to
* the instance.
*
* @return The name of the key pair. You can create a key pair using
* CreateKeyPair or ImportKeyPair. If you
* launch an instance without specifying a key pair, you can't connect to
* the instance.
*/
public String getKeyName() {
return keyName;
}
/**
* The name of the key pair. You can create a key pair using
* CreateKeyPair or ImportKeyPair. If you
* launch an instance without specifying a key pair, you can't connect to
* the instance.
*
* @param keyName The name of the key pair. You can create a key pair using
* CreateKeyPair or ImportKeyPair. If you
* launch an instance without specifying a key pair, you can't connect to
* the instance.
*/
public void setKeyName(String keyName) {
this.keyName = keyName;
}
/**
* The name of the key pair. You can create a key pair using
* CreateKeyPair or ImportKeyPair. If you
* launch an instance without specifying a key pair, you can't connect to
* the instance.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param keyName The name of the key pair. You can create a key pair using
* CreateKeyPair or ImportKeyPair. If you
* launch an instance without specifying a key pair, you can't connect to
* the instance.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RunInstancesRequest withKeyName(String keyName) {
this.keyName = keyName;
return this;
}
/**
* [EC2-Classic, default VPC] One or more security group names. For a
* nondefault VPC, you must use security group IDs instead.
Default:
* Amazon EC2 uses the default security group.
*
* @return [EC2-Classic, default VPC] One or more security group names. For a
* nondefault VPC, you must use security group IDs instead.
Default:
* Amazon EC2 uses the default security group.
*/
public java.util.List getSecurityGroups() {
if (securityGroups == null) {
securityGroups = new com.amazonaws.internal.ListWithAutoConstructFlag();
securityGroups.setAutoConstruct(true);
}
return securityGroups;
}
/**
* [EC2-Classic, default VPC] One or more security group names. For a
* nondefault VPC, you must use security group IDs instead. Default:
* Amazon EC2 uses the default security group.
*
* @param securityGroups [EC2-Classic, default VPC] One or more security group names. For a
* nondefault VPC, you must use security group IDs instead.
Default:
* Amazon EC2 uses the default security group.
*/
public void setSecurityGroups(java.util.Collection securityGroups) {
if (securityGroups == null) {
this.securityGroups = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag securityGroupsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(securityGroups.size());
securityGroupsCopy.addAll(securityGroups);
this.securityGroups = securityGroupsCopy;
}
/**
* [EC2-Classic, default VPC] One or more security group names. For a
* nondefault VPC, you must use security group IDs instead. Default:
* Amazon EC2 uses the default security group.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param securityGroups [EC2-Classic, default VPC] One or more security group names. For a
* nondefault VPC, you must use security group IDs instead.
Default:
* Amazon EC2 uses the default security group.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RunInstancesRequest withSecurityGroups(String... securityGroups) {
if (getSecurityGroups() == null) setSecurityGroups(new java.util.ArrayList(securityGroups.length));
for (String value : securityGroups) {
getSecurityGroups().add(value);
}
return this;
}
/**
* [EC2-Classic, default VPC] One or more security group names. For a
* nondefault VPC, you must use security group IDs instead. Default:
* Amazon EC2 uses the default security group.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param securityGroups [EC2-Classic, default VPC] One or more security group names. For a
* nondefault VPC, you must use security group IDs instead.
Default:
* Amazon EC2 uses the default security group.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RunInstancesRequest withSecurityGroups(java.util.Collection securityGroups) {
if (securityGroups == null) {
this.securityGroups = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag securityGroupsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(securityGroups.size());
securityGroupsCopy.addAll(securityGroups);
this.securityGroups = securityGroupsCopy;
}
return this;
}
/**
* One or more security group IDs. You can create a security group using
* CreateSecurityGroup. Default: Amazon EC2 uses the default
* security group.
*
* @return One or more security group IDs. You can create a security group using
* CreateSecurityGroup.
Default: Amazon EC2 uses the default
* security group.
*/
public java.util.List getSecurityGroupIds() {
if (securityGroupIds == null) {
securityGroupIds = new com.amazonaws.internal.ListWithAutoConstructFlag();
securityGroupIds.setAutoConstruct(true);
}
return securityGroupIds;
}
/**
* One or more security group IDs. You can create a security group using
* CreateSecurityGroup. Default: Amazon EC2 uses the default
* security group.
*
* @param securityGroupIds One or more security group IDs. You can create a security group using
* CreateSecurityGroup.
Default: Amazon EC2 uses the default
* security group.
*/
public void setSecurityGroupIds(java.util.Collection securityGroupIds) {
if (securityGroupIds == null) {
this.securityGroupIds = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag securityGroupIdsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(securityGroupIds.size());
securityGroupIdsCopy.addAll(securityGroupIds);
this.securityGroupIds = securityGroupIdsCopy;
}
/**
* One or more security group IDs. You can create a security group using
* CreateSecurityGroup. Default: Amazon EC2 uses the default
* security group.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param securityGroupIds One or more security group IDs. You can create a security group using
* CreateSecurityGroup.
Default: Amazon EC2 uses the default
* security group.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RunInstancesRequest withSecurityGroupIds(String... securityGroupIds) {
if (getSecurityGroupIds() == null) setSecurityGroupIds(new java.util.ArrayList(securityGroupIds.length));
for (String value : securityGroupIds) {
getSecurityGroupIds().add(value);
}
return this;
}
/**
* One or more security group IDs. You can create a security group using
* CreateSecurityGroup. Default: Amazon EC2 uses the default
* security group.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param securityGroupIds One or more security group IDs. You can create a security group using
* CreateSecurityGroup.
Default: Amazon EC2 uses the default
* security group.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RunInstancesRequest withSecurityGroupIds(java.util.Collection securityGroupIds) {
if (securityGroupIds == null) {
this.securityGroupIds = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag securityGroupIdsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(securityGroupIds.size());
securityGroupIdsCopy.addAll(securityGroupIds);
this.securityGroupIds = securityGroupIdsCopy;
}
return this;
}
/**
* The Base64-encoded MIME user data for the instances.
*
* @return The Base64-encoded MIME user data for the instances.
*/
public String getUserData() {
return userData;
}
/**
* The Base64-encoded MIME user data for the instances.
*
* @param userData The Base64-encoded MIME user data for the instances.
*/
public void setUserData(String userData) {
this.userData = userData;
}
/**
* The Base64-encoded MIME user data for the instances.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param userData The Base64-encoded MIME user data for the instances.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RunInstancesRequest withUserData(String userData) {
this.userData = userData;
return this;
}
/**
* The instance type. For more information, see Instance
* Types in the Amazon Elastic Compute Cloud User Guide.
*
Default: m1.small
*
* Constraints:
* Allowed Values: t1.micro, m1.small, m1.medium, m1.large, m1.xlarge, m3.medium, m3.large, m3.xlarge, m3.2xlarge, m2.xlarge, m2.2xlarge, m2.4xlarge, cr1.8xlarge, i2.xlarge, i2.2xlarge, i2.4xlarge, i2.8xlarge, hi1.4xlarge, hs1.8xlarge, c1.medium, c1.xlarge, c3.large, c3.xlarge, c3.2xlarge, c3.4xlarge, c3.8xlarge, cc1.4xlarge, cc2.8xlarge, g2.2xlarge, cg1.4xlarge
*
* @return The instance type. For more information, see Instance
* Types in the Amazon Elastic Compute Cloud User Guide.
*
Default: m1.small
*
* @see InstanceType
*/
public String getInstanceType() {
return instanceType;
}
/**
* The instance type. For more information, see Instance
* Types in the Amazon Elastic Compute Cloud User Guide.
*
Default: m1.small
*
* Constraints:
* Allowed Values: t1.micro, m1.small, m1.medium, m1.large, m1.xlarge, m3.medium, m3.large, m3.xlarge, m3.2xlarge, m2.xlarge, m2.2xlarge, m2.4xlarge, cr1.8xlarge, i2.xlarge, i2.2xlarge, i2.4xlarge, i2.8xlarge, hi1.4xlarge, hs1.8xlarge, c1.medium, c1.xlarge, c3.large, c3.xlarge, c3.2xlarge, c3.4xlarge, c3.8xlarge, cc1.4xlarge, cc2.8xlarge, g2.2xlarge, cg1.4xlarge
*
* @param instanceType The instance type. For more information, see Instance
* Types in the Amazon Elastic Compute Cloud User Guide.
*
Default: m1.small
*
* @see InstanceType
*/
public void setInstanceType(String instanceType) {
this.instanceType = instanceType;
}
/**
* The instance type. For more information, see Instance
* Types in the Amazon Elastic Compute Cloud User Guide.
*
Default: m1.small
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: t1.micro, m1.small, m1.medium, m1.large, m1.xlarge, m3.medium, m3.large, m3.xlarge, m3.2xlarge, m2.xlarge, m2.2xlarge, m2.4xlarge, cr1.8xlarge, i2.xlarge, i2.2xlarge, i2.4xlarge, i2.8xlarge, hi1.4xlarge, hs1.8xlarge, c1.medium, c1.xlarge, c3.large, c3.xlarge, c3.2xlarge, c3.4xlarge, c3.8xlarge, cc1.4xlarge, cc2.8xlarge, g2.2xlarge, cg1.4xlarge
*
* @param instanceType The instance type. For more information, see Instance
* Types in the Amazon Elastic Compute Cloud User Guide.
*
Default: m1.small
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see InstanceType
*/
public RunInstancesRequest withInstanceType(String instanceType) {
this.instanceType = instanceType;
return this;
}
/**
* The instance type. For more information, see Instance
* Types in the Amazon Elastic Compute Cloud User Guide.
*
Default: m1.small
*
* Constraints:
* Allowed Values: t1.micro, m1.small, m1.medium, m1.large, m1.xlarge, m3.medium, m3.large, m3.xlarge, m3.2xlarge, m2.xlarge, m2.2xlarge, m2.4xlarge, cr1.8xlarge, i2.xlarge, i2.2xlarge, i2.4xlarge, i2.8xlarge, hi1.4xlarge, hs1.8xlarge, c1.medium, c1.xlarge, c3.large, c3.xlarge, c3.2xlarge, c3.4xlarge, c3.8xlarge, cc1.4xlarge, cc2.8xlarge, g2.2xlarge, cg1.4xlarge
*
* @param instanceType The instance type. For more information, see Instance
* Types in the Amazon Elastic Compute Cloud User Guide.
*
Default: m1.small
*
* @see InstanceType
*/
public void setInstanceType(InstanceType instanceType) {
this.instanceType = instanceType.toString();
}
/**
* The instance type. For more information, see Instance
* Types in the Amazon Elastic Compute Cloud User Guide.
*
Default: m1.small
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: t1.micro, m1.small, m1.medium, m1.large, m1.xlarge, m3.medium, m3.large, m3.xlarge, m3.2xlarge, m2.xlarge, m2.2xlarge, m2.4xlarge, cr1.8xlarge, i2.xlarge, i2.2xlarge, i2.4xlarge, i2.8xlarge, hi1.4xlarge, hs1.8xlarge, c1.medium, c1.xlarge, c3.large, c3.xlarge, c3.2xlarge, c3.4xlarge, c3.8xlarge, cc1.4xlarge, cc2.8xlarge, g2.2xlarge, cg1.4xlarge
*
* @param instanceType The instance type. For more information, see Instance
* Types in the Amazon Elastic Compute Cloud User Guide.
*
Default: m1.small
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see InstanceType
*/
public RunInstancesRequest withInstanceType(InstanceType instanceType) {
this.instanceType = instanceType.toString();
return this;
}
/**
* The placement for the instance.
*
* @return The placement for the instance.
*/
public Placement getPlacement() {
return placement;
}
/**
* The placement for the instance.
*
* @param placement The placement for the instance.
*/
public void setPlacement(Placement placement) {
this.placement = placement;
}
/**
* The placement for the instance.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param placement The placement for the instance.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RunInstancesRequest withPlacement(Placement placement) {
this.placement = placement;
return this;
}
/**
* The ID of the kernel. We recommend that you use PV-GRUB
* instead of kernels and RAM disks. For more information, see
* PV-GRUB: A New Amazon Kernel Image in the Amazon Elastic
* Compute Cloud User Guide.
*
* @return The ID of the kernel. We recommend that you use PV-GRUB
* instead of kernels and RAM disks. For more information, see
* PV-GRUB: A New Amazon Kernel Image in the Amazon Elastic
* Compute Cloud User Guide.
*/
public String getKernelId() {
return kernelId;
}
/**
* The ID of the kernel. We recommend that you use PV-GRUB
* instead of kernels and RAM disks. For more information, see
* PV-GRUB: A New Amazon Kernel Image in the Amazon Elastic
* Compute Cloud User Guide.
*
* @param kernelId The ID of the kernel. We recommend that you use PV-GRUB
* instead of kernels and RAM disks. For more information, see
* PV-GRUB: A New Amazon Kernel Image in the Amazon Elastic
* Compute Cloud User Guide.
*/
public void setKernelId(String kernelId) {
this.kernelId = kernelId;
}
/**
* The ID of the kernel. We recommend that you use PV-GRUB
* instead of kernels and RAM disks. For more information, see
* PV-GRUB: A New Amazon Kernel Image in the Amazon Elastic
* Compute Cloud User Guide.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param kernelId The ID of the kernel. We recommend that you use PV-GRUB
* instead of kernels and RAM disks. For more information, see
* PV-GRUB: A New Amazon Kernel Image in the Amazon Elastic
* Compute Cloud User Guide.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RunInstancesRequest withKernelId(String kernelId) {
this.kernelId = kernelId;
return this;
}
/**
* The ID of the RAM disk.
*
* @return The ID of the RAM disk.
*/
public String getRamdiskId() {
return ramdiskId;
}
/**
* The ID of the RAM disk.
*
* @param ramdiskId The ID of the RAM disk.
*/
public void setRamdiskId(String ramdiskId) {
this.ramdiskId = ramdiskId;
}
/**
* The ID of the RAM disk.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param ramdiskId The ID of the RAM disk.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RunInstancesRequest withRamdiskId(String ramdiskId) {
this.ramdiskId = ramdiskId;
return this;
}
/**
* The block device mapping.
*
* @return The block device mapping.
*/
public java.util.List getBlockDeviceMappings() {
if (blockDeviceMappings == null) {
blockDeviceMappings = new com.amazonaws.internal.ListWithAutoConstructFlag();
blockDeviceMappings.setAutoConstruct(true);
}
return blockDeviceMappings;
}
/**
* The block device mapping.
*
* @param blockDeviceMappings The block device mapping.
*/
public void setBlockDeviceMappings(java.util.Collection blockDeviceMappings) {
if (blockDeviceMappings == null) {
this.blockDeviceMappings = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag blockDeviceMappingsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(blockDeviceMappings.size());
blockDeviceMappingsCopy.addAll(blockDeviceMappings);
this.blockDeviceMappings = blockDeviceMappingsCopy;
}
/**
* The block device mapping.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param blockDeviceMappings The block device mapping.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RunInstancesRequest withBlockDeviceMappings(BlockDeviceMapping... blockDeviceMappings) {
if (getBlockDeviceMappings() == null) setBlockDeviceMappings(new java.util.ArrayList(blockDeviceMappings.length));
for (BlockDeviceMapping value : blockDeviceMappings) {
getBlockDeviceMappings().add(value);
}
return this;
}
/**
* The block device mapping.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param blockDeviceMappings The block device mapping.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RunInstancesRequest withBlockDeviceMappings(java.util.Collection blockDeviceMappings) {
if (blockDeviceMappings == null) {
this.blockDeviceMappings = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag blockDeviceMappingsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(blockDeviceMappings.size());
blockDeviceMappingsCopy.addAll(blockDeviceMappings);
this.blockDeviceMappings = blockDeviceMappingsCopy;
}
return this;
}
/**
* The monitoring for the instance.
*
* @return The monitoring for the instance.
*/
public Boolean isMonitoring() {
return monitoring;
}
/**
* The monitoring for the instance.
*
* @param monitoring The monitoring for the instance.
*/
public void setMonitoring(Boolean monitoring) {
this.monitoring = monitoring;
}
/**
* The monitoring for the instance.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param monitoring The monitoring for the instance.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RunInstancesRequest withMonitoring(Boolean monitoring) {
this.monitoring = monitoring;
return this;
}
/**
* The monitoring for the instance.
*
* @return The monitoring for the instance.
*/
public Boolean getMonitoring() {
return monitoring;
}
/**
* [EC2-VPC] The ID of the subnet to launch the instance into.
*
* @return [EC2-VPC] The ID of the subnet to launch the instance into.
*/
public String getSubnetId() {
return subnetId;
}
/**
* [EC2-VPC] The ID of the subnet to launch the instance into.
*
* @param subnetId [EC2-VPC] The ID of the subnet to launch the instance into.
*/
public void setSubnetId(String subnetId) {
this.subnetId = subnetId;
}
/**
* [EC2-VPC] The ID of the subnet to launch the instance into.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param subnetId [EC2-VPC] The ID of the subnet to launch the instance into.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RunInstancesRequest withSubnetId(String subnetId) {
this.subnetId = subnetId;
return this;
}
/**
* If you set this parameter to true
, you can't terminate
* the instance using the Amazon EC2 console, CLI, or API; otherwise, you
* can. If you set this parameter to true
and then later
* want to be able to terminate the instance, you must first change the
* value of the disableApiTermination
attribute to
* false
using ModifyInstanceAttribute.
* Alternatively, if you set
* InstanceInitiatedShutdownBehavior
to
* terminate
, you can terminate the instance by running the
* shutdown command from the instance.
Default: false
*
* @return If you set this parameter to true
, you can't terminate
* the instance using the Amazon EC2 console, CLI, or API; otherwise, you
* can. If you set this parameter to true
and then later
* want to be able to terminate the instance, you must first change the
* value of the disableApiTermination
attribute to
* false
using ModifyInstanceAttribute.
* Alternatively, if you set
* InstanceInitiatedShutdownBehavior
to
* terminate
, you can terminate the instance by running the
* shutdown command from the instance.
Default: false
*/
public Boolean isDisableApiTermination() {
return disableApiTermination;
}
/**
* If you set this parameter to true
, you can't terminate
* the instance using the Amazon EC2 console, CLI, or API; otherwise, you
* can. If you set this parameter to true
and then later
* want to be able to terminate the instance, you must first change the
* value of the disableApiTermination
attribute to
* false
using ModifyInstanceAttribute.
* Alternatively, if you set
* InstanceInitiatedShutdownBehavior
to
* terminate
, you can terminate the instance by running the
* shutdown command from the instance.
Default: false
*
* @param disableApiTermination If you set this parameter to true
, you can't terminate
* the instance using the Amazon EC2 console, CLI, or API; otherwise, you
* can. If you set this parameter to true
and then later
* want to be able to terminate the instance, you must first change the
* value of the disableApiTermination
attribute to
* false
using ModifyInstanceAttribute.
* Alternatively, if you set
* InstanceInitiatedShutdownBehavior
to
* terminate
, you can terminate the instance by running the
* shutdown command from the instance.
Default: false
*/
public void setDisableApiTermination(Boolean disableApiTermination) {
this.disableApiTermination = disableApiTermination;
}
/**
* If you set this parameter to true
, you can't terminate
* the instance using the Amazon EC2 console, CLI, or API; otherwise, you
* can. If you set this parameter to true
and then later
* want to be able to terminate the instance, you must first change the
* value of the disableApiTermination
attribute to
* false
using ModifyInstanceAttribute.
* Alternatively, if you set
* InstanceInitiatedShutdownBehavior
to
* terminate
, you can terminate the instance by running the
* shutdown command from the instance.
Default: false
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param disableApiTermination If you set this parameter to true
, you can't terminate
* the instance using the Amazon EC2 console, CLI, or API; otherwise, you
* can. If you set this parameter to true
and then later
* want to be able to terminate the instance, you must first change the
* value of the disableApiTermination
attribute to
* false
using ModifyInstanceAttribute.
* Alternatively, if you set
* InstanceInitiatedShutdownBehavior
to
* terminate
, you can terminate the instance by running the
* shutdown command from the instance.
Default: false
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RunInstancesRequest withDisableApiTermination(Boolean disableApiTermination) {
this.disableApiTermination = disableApiTermination;
return this;
}
/**
* If you set this parameter to true
, you can't terminate
* the instance using the Amazon EC2 console, CLI, or API; otherwise, you
* can. If you set this parameter to true
and then later
* want to be able to terminate the instance, you must first change the
* value of the disableApiTermination
attribute to
* false
using ModifyInstanceAttribute.
* Alternatively, if you set
* InstanceInitiatedShutdownBehavior
to
* terminate
, you can terminate the instance by running the
* shutdown command from the instance.
Default: false
*
* @return If you set this parameter to true
, you can't terminate
* the instance using the Amazon EC2 console, CLI, or API; otherwise, you
* can. If you set this parameter to true
and then later
* want to be able to terminate the instance, you must first change the
* value of the disableApiTermination
attribute to
* false
using ModifyInstanceAttribute.
* Alternatively, if you set
* InstanceInitiatedShutdownBehavior
to
* terminate
, you can terminate the instance by running the
* shutdown command from the instance.
Default: false
*/
public Boolean getDisableApiTermination() {
return disableApiTermination;
}
/**
* Indicates whether an instance stops or terminates when you initiate
* shutdown from the instance (using the operating system command for
* system shutdown).
Default: stop
*
* Constraints:
* Allowed Values: stop, terminate
*
* @return Indicates whether an instance stops or terminates when you initiate
* shutdown from the instance (using the operating system command for
* system shutdown).
Default: stop
*
* @see ShutdownBehavior
*/
public String getInstanceInitiatedShutdownBehavior() {
return instanceInitiatedShutdownBehavior;
}
/**
* Indicates whether an instance stops or terminates when you initiate
* shutdown from the instance (using the operating system command for
* system shutdown).
Default: stop
*
* Constraints:
* Allowed Values: stop, terminate
*
* @param instanceInitiatedShutdownBehavior Indicates whether an instance stops or terminates when you initiate
* shutdown from the instance (using the operating system command for
* system shutdown).
Default: stop
*
* @see ShutdownBehavior
*/
public void setInstanceInitiatedShutdownBehavior(String instanceInitiatedShutdownBehavior) {
this.instanceInitiatedShutdownBehavior = instanceInitiatedShutdownBehavior;
}
/**
* Indicates whether an instance stops or terminates when you initiate
* shutdown from the instance (using the operating system command for
* system shutdown).
Default: stop
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: stop, terminate
*
* @param instanceInitiatedShutdownBehavior Indicates whether an instance stops or terminates when you initiate
* shutdown from the instance (using the operating system command for
* system shutdown).
Default: stop
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see ShutdownBehavior
*/
public RunInstancesRequest withInstanceInitiatedShutdownBehavior(String instanceInitiatedShutdownBehavior) {
this.instanceInitiatedShutdownBehavior = instanceInitiatedShutdownBehavior;
return this;
}
/**
* Indicates whether an instance stops or terminates when you initiate
* shutdown from the instance (using the operating system command for
* system shutdown).
Default: stop
*
* Constraints:
* Allowed Values: stop, terminate
*
* @param instanceInitiatedShutdownBehavior Indicates whether an instance stops or terminates when you initiate
* shutdown from the instance (using the operating system command for
* system shutdown).
Default: stop
*
* @see ShutdownBehavior
*/
public void setInstanceInitiatedShutdownBehavior(ShutdownBehavior instanceInitiatedShutdownBehavior) {
this.instanceInitiatedShutdownBehavior = instanceInitiatedShutdownBehavior.toString();
}
/**
* Indicates whether an instance stops or terminates when you initiate
* shutdown from the instance (using the operating system command for
* system shutdown).
Default: stop
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: stop, terminate
*
* @param instanceInitiatedShutdownBehavior Indicates whether an instance stops or terminates when you initiate
* shutdown from the instance (using the operating system command for
* system shutdown).
Default: stop
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see ShutdownBehavior
*/
public RunInstancesRequest withInstanceInitiatedShutdownBehavior(ShutdownBehavior instanceInitiatedShutdownBehavior) {
this.instanceInitiatedShutdownBehavior = instanceInitiatedShutdownBehavior.toString();
return this;
}
/**
* [EC2-VPC] The primary IP address. You must specify a value from the IP
* address range of the subnet.
Only one private IP address can be
* designated as primary. Therefore, you can't specify this parameter if
* PrivateIpAddresses.n.Primary
is set to true
* and PrivateIpAddresses.n.PrivateIpAddress
is set to an IP
* address.
Default: We select an IP address from the IP address range
* of the subnet.
*
* @return [EC2-VPC] The primary IP address. You must specify a value from the IP
* address range of the subnet.
Only one private IP address can be
* designated as primary. Therefore, you can't specify this parameter if
* PrivateIpAddresses.n.Primary
is set to true
* and PrivateIpAddresses.n.PrivateIpAddress
is set to an IP
* address.
Default: We select an IP address from the IP address range
* of the subnet.
*/
public String getPrivateIpAddress() {
return privateIpAddress;
}
/**
* [EC2-VPC] The primary IP address. You must specify a value from the IP
* address range of the subnet.
Only one private IP address can be
* designated as primary. Therefore, you can't specify this parameter if
* PrivateIpAddresses.n.Primary
is set to true
* and PrivateIpAddresses.n.PrivateIpAddress
is set to an IP
* address.
Default: We select an IP address from the IP address range
* of the subnet.
*
* @param privateIpAddress [EC2-VPC] The primary IP address. You must specify a value from the IP
* address range of the subnet.
Only one private IP address can be
* designated as primary. Therefore, you can't specify this parameter if
* PrivateIpAddresses.n.Primary
is set to true
* and PrivateIpAddresses.n.PrivateIpAddress
is set to an IP
* address.
Default: We select an IP address from the IP address range
* of the subnet.
*/
public void setPrivateIpAddress(String privateIpAddress) {
this.privateIpAddress = privateIpAddress;
}
/**
* [EC2-VPC] The primary IP address. You must specify a value from the IP
* address range of the subnet.
Only one private IP address can be
* designated as primary. Therefore, you can't specify this parameter if
* PrivateIpAddresses.n.Primary
is set to true
* and PrivateIpAddresses.n.PrivateIpAddress
is set to an IP
* address.
Default: We select an IP address from the IP address range
* of the subnet.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param privateIpAddress [EC2-VPC] The primary IP address. You must specify a value from the IP
* address range of the subnet.
Only one private IP address can be
* designated as primary. Therefore, you can't specify this parameter if
* PrivateIpAddresses.n.Primary
is set to true
* and PrivateIpAddresses.n.PrivateIpAddress
is set to an IP
* address.
Default: We select an IP address from the IP address range
* of the subnet.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RunInstancesRequest withPrivateIpAddress(String privateIpAddress) {
this.privateIpAddress = privateIpAddress;
return this;
}
/**
* Unique, case-sensitive identifier you provide to ensure the
* idempotency of the request. For more information, see How
* to Ensure Idempotency in the Amazon Elastic Compute Cloud User
* Guide.
Constraints: Maximum 64 ASCII characters
*
* @return Unique, case-sensitive identifier you provide to ensure the
* idempotency of the request. For more information, see How
* to Ensure Idempotency in the Amazon Elastic Compute Cloud User
* Guide.
Constraints: Maximum 64 ASCII characters
*/
public String getClientToken() {
return clientToken;
}
/**
* Unique, case-sensitive identifier you provide to ensure the
* idempotency of the request. For more information, see How
* to Ensure Idempotency in the Amazon Elastic Compute Cloud User
* Guide.
Constraints: Maximum 64 ASCII characters
*
* @param clientToken Unique, case-sensitive identifier you provide to ensure the
* idempotency of the request. For more information, see How
* to Ensure Idempotency in the Amazon Elastic Compute Cloud User
* Guide.
Constraints: Maximum 64 ASCII characters
*/
public void setClientToken(String clientToken) {
this.clientToken = clientToken;
}
/**
* Unique, case-sensitive identifier you provide to ensure the
* idempotency of the request. For more information, see How
* to Ensure Idempotency in the Amazon Elastic Compute Cloud User
* Guide.
Constraints: Maximum 64 ASCII characters
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param clientToken Unique, case-sensitive identifier you provide to ensure the
* idempotency of the request. For more information, see How
* to Ensure Idempotency in the Amazon Elastic Compute Cloud User
* Guide.
Constraints: Maximum 64 ASCII characters
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RunInstancesRequest withClientToken(String clientToken) {
this.clientToken = clientToken;
return this;
}
/**
*
*
* @return
*/
public String getAdditionalInfo() {
return additionalInfo;
}
/**
*
*
* @param additionalInfo
*/
public void setAdditionalInfo(String additionalInfo) {
this.additionalInfo = additionalInfo;
}
/**
*
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param additionalInfo
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RunInstancesRequest withAdditionalInfo(String additionalInfo) {
this.additionalInfo = additionalInfo;
return this;
}
/**
* One or more network interfaces.
*
* @return One or more network interfaces.
*/
public java.util.List getNetworkInterfaces() {
if (networkInterfaces == null) {
networkInterfaces = new com.amazonaws.internal.ListWithAutoConstructFlag();
networkInterfaces.setAutoConstruct(true);
}
return networkInterfaces;
}
/**
* One or more network interfaces.
*
* @param networkInterfaces One or more network interfaces.
*/
public void setNetworkInterfaces(java.util.Collection networkInterfaces) {
if (networkInterfaces == null) {
this.networkInterfaces = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag networkInterfacesCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(networkInterfaces.size());
networkInterfacesCopy.addAll(networkInterfaces);
this.networkInterfaces = networkInterfacesCopy;
}
/**
* One or more network interfaces.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param networkInterfaces One or more network interfaces.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RunInstancesRequest withNetworkInterfaces(InstanceNetworkInterfaceSpecification... networkInterfaces) {
if (getNetworkInterfaces() == null) setNetworkInterfaces(new java.util.ArrayList(networkInterfaces.length));
for (InstanceNetworkInterfaceSpecification value : networkInterfaces) {
getNetworkInterfaces().add(value);
}
return this;
}
/**
* One or more network interfaces.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param networkInterfaces One or more network interfaces.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RunInstancesRequest withNetworkInterfaces(java.util.Collection networkInterfaces) {
if (networkInterfaces == null) {
this.networkInterfaces = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag networkInterfacesCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(networkInterfaces.size());
networkInterfacesCopy.addAll(networkInterfaces);
this.networkInterfaces = networkInterfacesCopy;
}
return this;
}
/**
* The IAM instance profile.
*
* @return The IAM instance profile.
*/
public IamInstanceProfileSpecification getIamInstanceProfile() {
return iamInstanceProfile;
}
/**
* The IAM instance profile.
*
* @param iamInstanceProfile The IAM instance profile.
*/
public void setIamInstanceProfile(IamInstanceProfileSpecification iamInstanceProfile) {
this.iamInstanceProfile = iamInstanceProfile;
}
/**
* The IAM instance profile.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param iamInstanceProfile The IAM instance profile.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RunInstancesRequest withIamInstanceProfile(IamInstanceProfileSpecification iamInstanceProfile) {
this.iamInstanceProfile = iamInstanceProfile;
return this;
}
/**
* Indicates whether the instance is optimized for EBS I/O. This
* optimization provides dedicated throughput to Amazon EBS and an
* optimized configuration stack to provide optimal Amazon EBS I/O
* performance. This optimization isn't available with all instance
* types. Additional usage charges apply when using an EBS-optimized
* instance.
Default: false
*
* @return Indicates whether the instance is optimized for EBS I/O. This
* optimization provides dedicated throughput to Amazon EBS and an
* optimized configuration stack to provide optimal Amazon EBS I/O
* performance. This optimization isn't available with all instance
* types. Additional usage charges apply when using an EBS-optimized
* instance.
Default: false
*/
public Boolean isEbsOptimized() {
return ebsOptimized;
}
/**
* Indicates whether the instance is optimized for EBS I/O. This
* optimization provides dedicated throughput to Amazon EBS and an
* optimized configuration stack to provide optimal Amazon EBS I/O
* performance. This optimization isn't available with all instance
* types. Additional usage charges apply when using an EBS-optimized
* instance.
Default: false
*
* @param ebsOptimized Indicates whether the instance is optimized for EBS I/O. This
* optimization provides dedicated throughput to Amazon EBS and an
* optimized configuration stack to provide optimal Amazon EBS I/O
* performance. This optimization isn't available with all instance
* types. Additional usage charges apply when using an EBS-optimized
* instance.
Default: false
*/
public void setEbsOptimized(Boolean ebsOptimized) {
this.ebsOptimized = ebsOptimized;
}
/**
* Indicates whether the instance is optimized for EBS I/O. This
* optimization provides dedicated throughput to Amazon EBS and an
* optimized configuration stack to provide optimal Amazon EBS I/O
* performance. This optimization isn't available with all instance
* types. Additional usage charges apply when using an EBS-optimized
* instance.
Default: false
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param ebsOptimized Indicates whether the instance is optimized for EBS I/O. This
* optimization provides dedicated throughput to Amazon EBS and an
* optimized configuration stack to provide optimal Amazon EBS I/O
* performance. This optimization isn't available with all instance
* types. Additional usage charges apply when using an EBS-optimized
* instance.
Default: false
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RunInstancesRequest withEbsOptimized(Boolean ebsOptimized) {
this.ebsOptimized = ebsOptimized;
return this;
}
/**
* Indicates whether the instance is optimized for EBS I/O. This
* optimization provides dedicated throughput to Amazon EBS and an
* optimized configuration stack to provide optimal Amazon EBS I/O
* performance. This optimization isn't available with all instance
* types. Additional usage charges apply when using an EBS-optimized
* instance.
Default: false
*
* @return Indicates whether the instance is optimized for EBS I/O. This
* optimization provides dedicated throughput to Amazon EBS and an
* optimized configuration stack to provide optimal Amazon EBS I/O
* performance. This optimization isn't available with all instance
* types. Additional usage charges apply when using an EBS-optimized
* instance.
Default: false
*/
public Boolean getEbsOptimized() {
return ebsOptimized;
}
/**
* This method is intended for internal use only.
* Returns the marshaled request configured with additional parameters to
* enable operation dry-run.
*/
@Override
public Request getDryRunRequest() {
Request request = new RunInstancesRequestMarshaller().marshall(this);
request.addParameter("DryRun", Boolean.toString(true));
return request;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getImageId() != null) sb.append("ImageId: " + getImageId() + ",");
if (getMinCount() != null) sb.append("MinCount: " + getMinCount() + ",");
if (getMaxCount() != null) sb.append("MaxCount: " + getMaxCount() + ",");
if (getKeyName() != null) sb.append("KeyName: " + getKeyName() + ",");
if (getSecurityGroups() != null) sb.append("SecurityGroups: " + getSecurityGroups() + ",");
if (getSecurityGroupIds() != null) sb.append("SecurityGroupIds: " + getSecurityGroupIds() + ",");
if (getUserData() != null) sb.append("UserData: " + getUserData() + ",");
if (getInstanceType() != null) sb.append("InstanceType: " + getInstanceType() + ",");
if (getPlacement() != null) sb.append("Placement: " + getPlacement() + ",");
if (getKernelId() != null) sb.append("KernelId: " + getKernelId() + ",");
if (getRamdiskId() != null) sb.append("RamdiskId: " + getRamdiskId() + ",");
if (getBlockDeviceMappings() != null) sb.append("BlockDeviceMappings: " + getBlockDeviceMappings() + ",");
if (isMonitoring() != null) sb.append("Monitoring: " + isMonitoring() + ",");
if (getSubnetId() != null) sb.append("SubnetId: " + getSubnetId() + ",");
if (isDisableApiTermination() != null) sb.append("DisableApiTermination: " + isDisableApiTermination() + ",");
if (getInstanceInitiatedShutdownBehavior() != null) sb.append("InstanceInitiatedShutdownBehavior: " + getInstanceInitiatedShutdownBehavior() + ",");
if (getPrivateIpAddress() != null) sb.append("PrivateIpAddress: " + getPrivateIpAddress() + ",");
if (getClientToken() != null) sb.append("ClientToken: " + getClientToken() + ",");
if (getAdditionalInfo() != null) sb.append("AdditionalInfo: " + getAdditionalInfo() + ",");
if (getNetworkInterfaces() != null) sb.append("NetworkInterfaces: " + getNetworkInterfaces() + ",");
if (getIamInstanceProfile() != null) sb.append("IamInstanceProfile: " + getIamInstanceProfile() + ",");
if (isEbsOptimized() != null) sb.append("EbsOptimized: " + isEbsOptimized() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getImageId() == null) ? 0 : getImageId().hashCode());
hashCode = prime * hashCode + ((getMinCount() == null) ? 0 : getMinCount().hashCode());
hashCode = prime * hashCode + ((getMaxCount() == null) ? 0 : getMaxCount().hashCode());
hashCode = prime * hashCode + ((getKeyName() == null) ? 0 : getKeyName().hashCode());
hashCode = prime * hashCode + ((getSecurityGroups() == null) ? 0 : getSecurityGroups().hashCode());
hashCode = prime * hashCode + ((getSecurityGroupIds() == null) ? 0 : getSecurityGroupIds().hashCode());
hashCode = prime * hashCode + ((getUserData() == null) ? 0 : getUserData().hashCode());
hashCode = prime * hashCode + ((getInstanceType() == null) ? 0 : getInstanceType().hashCode());
hashCode = prime * hashCode + ((getPlacement() == null) ? 0 : getPlacement().hashCode());
hashCode = prime * hashCode + ((getKernelId() == null) ? 0 : getKernelId().hashCode());
hashCode = prime * hashCode + ((getRamdiskId() == null) ? 0 : getRamdiskId().hashCode());
hashCode = prime * hashCode + ((getBlockDeviceMappings() == null) ? 0 : getBlockDeviceMappings().hashCode());
hashCode = prime * hashCode + ((isMonitoring() == null) ? 0 : isMonitoring().hashCode());
hashCode = prime * hashCode + ((getSubnetId() == null) ? 0 : getSubnetId().hashCode());
hashCode = prime * hashCode + ((isDisableApiTermination() == null) ? 0 : isDisableApiTermination().hashCode());
hashCode = prime * hashCode + ((getInstanceInitiatedShutdownBehavior() == null) ? 0 : getInstanceInitiatedShutdownBehavior().hashCode());
hashCode = prime * hashCode + ((getPrivateIpAddress() == null) ? 0 : getPrivateIpAddress().hashCode());
hashCode = prime * hashCode + ((getClientToken() == null) ? 0 : getClientToken().hashCode());
hashCode = prime * hashCode + ((getAdditionalInfo() == null) ? 0 : getAdditionalInfo().hashCode());
hashCode = prime * hashCode + ((getNetworkInterfaces() == null) ? 0 : getNetworkInterfaces().hashCode());
hashCode = prime * hashCode + ((getIamInstanceProfile() == null) ? 0 : getIamInstanceProfile().hashCode());
hashCode = prime * hashCode + ((isEbsOptimized() == null) ? 0 : isEbsOptimized().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof RunInstancesRequest == false) return false;
RunInstancesRequest other = (RunInstancesRequest)obj;
if (other.getImageId() == null ^ this.getImageId() == null) return false;
if (other.getImageId() != null && other.getImageId().equals(this.getImageId()) == false) return false;
if (other.getMinCount() == null ^ this.getMinCount() == null) return false;
if (other.getMinCount() != null && other.getMinCount().equals(this.getMinCount()) == false) return false;
if (other.getMaxCount() == null ^ this.getMaxCount() == null) return false;
if (other.getMaxCount() != null && other.getMaxCount().equals(this.getMaxCount()) == false) return false;
if (other.getKeyName() == null ^ this.getKeyName() == null) return false;
if (other.getKeyName() != null && other.getKeyName().equals(this.getKeyName()) == false) return false;
if (other.getSecurityGroups() == null ^ this.getSecurityGroups() == null) return false;
if (other.getSecurityGroups() != null && other.getSecurityGroups().equals(this.getSecurityGroups()) == false) return false;
if (other.getSecurityGroupIds() == null ^ this.getSecurityGroupIds() == null) return false;
if (other.getSecurityGroupIds() != null && other.getSecurityGroupIds().equals(this.getSecurityGroupIds()) == false) return false;
if (other.getUserData() == null ^ this.getUserData() == null) return false;
if (other.getUserData() != null && other.getUserData().equals(this.getUserData()) == false) return false;
if (other.getInstanceType() == null ^ this.getInstanceType() == null) return false;
if (other.getInstanceType() != null && other.getInstanceType().equals(this.getInstanceType()) == false) return false;
if (other.getPlacement() == null ^ this.getPlacement() == null) return false;
if (other.getPlacement() != null && other.getPlacement().equals(this.getPlacement()) == false) return false;
if (other.getKernelId() == null ^ this.getKernelId() == null) return false;
if (other.getKernelId() != null && other.getKernelId().equals(this.getKernelId()) == false) return false;
if (other.getRamdiskId() == null ^ this.getRamdiskId() == null) return false;
if (other.getRamdiskId() != null && other.getRamdiskId().equals(this.getRamdiskId()) == false) return false;
if (other.getBlockDeviceMappings() == null ^ this.getBlockDeviceMappings() == null) return false;
if (other.getBlockDeviceMappings() != null && other.getBlockDeviceMappings().equals(this.getBlockDeviceMappings()) == false) return false;
if (other.isMonitoring() == null ^ this.isMonitoring() == null) return false;
if (other.isMonitoring() != null && other.isMonitoring().equals(this.isMonitoring()) == false) return false;
if (other.getSubnetId() == null ^ this.getSubnetId() == null) return false;
if (other.getSubnetId() != null && other.getSubnetId().equals(this.getSubnetId()) == false) return false;
if (other.isDisableApiTermination() == null ^ this.isDisableApiTermination() == null) return false;
if (other.isDisableApiTermination() != null && other.isDisableApiTermination().equals(this.isDisableApiTermination()) == false) return false;
if (other.getInstanceInitiatedShutdownBehavior() == null ^ this.getInstanceInitiatedShutdownBehavior() == null) return false;
if (other.getInstanceInitiatedShutdownBehavior() != null && other.getInstanceInitiatedShutdownBehavior().equals(this.getInstanceInitiatedShutdownBehavior()) == false) return false;
if (other.getPrivateIpAddress() == null ^ this.getPrivateIpAddress() == null) return false;
if (other.getPrivateIpAddress() != null && other.getPrivateIpAddress().equals(this.getPrivateIpAddress()) == false) return false;
if (other.getClientToken() == null ^ this.getClientToken() == null) return false;
if (other.getClientToken() != null && other.getClientToken().equals(this.getClientToken()) == false) return false;
if (other.getAdditionalInfo() == null ^ this.getAdditionalInfo() == null) return false;
if (other.getAdditionalInfo() != null && other.getAdditionalInfo().equals(this.getAdditionalInfo()) == false) return false;
if (other.getNetworkInterfaces() == null ^ this.getNetworkInterfaces() == null) return false;
if (other.getNetworkInterfaces() != null && other.getNetworkInterfaces().equals(this.getNetworkInterfaces()) == false) return false;
if (other.getIamInstanceProfile() == null ^ this.getIamInstanceProfile() == null) return false;
if (other.getIamInstanceProfile() != null && other.getIamInstanceProfile().equals(this.getIamInstanceProfile()) == false) return false;
if (other.isEbsOptimized() == null ^ this.isEbsOptimized() == null) return false;
if (other.isEbsOptimized() != null && other.isEbsOptimized().equals(this.isEbsOptimized()) == false) return false;
return true;
}
}