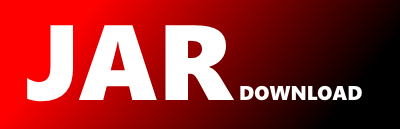
com.amazonaws.services.ec2.model.SpotPrice Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.ec2.model;
import java.io.Serializable;
/**
*
* Describes the Spot Price.
*
*/
public class SpotPrice implements Serializable {
/**
* The instance type.
*
* Constraints:
* Allowed Values: t1.micro, m1.small, m1.medium, m1.large, m1.xlarge, m3.medium, m3.large, m3.xlarge, m3.2xlarge, m2.xlarge, m2.2xlarge, m2.4xlarge, cr1.8xlarge, i2.xlarge, i2.2xlarge, i2.4xlarge, i2.8xlarge, hi1.4xlarge, hs1.8xlarge, c1.medium, c1.xlarge, c3.large, c3.xlarge, c3.2xlarge, c3.4xlarge, c3.8xlarge, cc1.4xlarge, cc2.8xlarge, g2.2xlarge, cg1.4xlarge
*/
private String instanceType;
/**
* A general description of the AMI.
*
* Constraints:
* Allowed Values: Linux/UNIX, Linux/UNIX (Amazon VPC), Windows, Windows (Amazon VPC)
*/
private String productDescription;
/**
* The maximum price you will pay to launch one or more Spot Instances.
*/
private String spotPrice;
/**
* The date and time the request was created.
*/
private java.util.Date timestamp;
/**
* The Availability Zone.
*/
private String availabilityZone;
/**
* The instance type.
*
* Constraints:
* Allowed Values: t1.micro, m1.small, m1.medium, m1.large, m1.xlarge, m3.medium, m3.large, m3.xlarge, m3.2xlarge, m2.xlarge, m2.2xlarge, m2.4xlarge, cr1.8xlarge, i2.xlarge, i2.2xlarge, i2.4xlarge, i2.8xlarge, hi1.4xlarge, hs1.8xlarge, c1.medium, c1.xlarge, c3.large, c3.xlarge, c3.2xlarge, c3.4xlarge, c3.8xlarge, cc1.4xlarge, cc2.8xlarge, g2.2xlarge, cg1.4xlarge
*
* @return The instance type.
*
* @see InstanceType
*/
public String getInstanceType() {
return instanceType;
}
/**
* The instance type.
*
* Constraints:
* Allowed Values: t1.micro, m1.small, m1.medium, m1.large, m1.xlarge, m3.medium, m3.large, m3.xlarge, m3.2xlarge, m2.xlarge, m2.2xlarge, m2.4xlarge, cr1.8xlarge, i2.xlarge, i2.2xlarge, i2.4xlarge, i2.8xlarge, hi1.4xlarge, hs1.8xlarge, c1.medium, c1.xlarge, c3.large, c3.xlarge, c3.2xlarge, c3.4xlarge, c3.8xlarge, cc1.4xlarge, cc2.8xlarge, g2.2xlarge, cg1.4xlarge
*
* @param instanceType The instance type.
*
* @see InstanceType
*/
public void setInstanceType(String instanceType) {
this.instanceType = instanceType;
}
/**
* The instance type.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: t1.micro, m1.small, m1.medium, m1.large, m1.xlarge, m3.medium, m3.large, m3.xlarge, m3.2xlarge, m2.xlarge, m2.2xlarge, m2.4xlarge, cr1.8xlarge, i2.xlarge, i2.2xlarge, i2.4xlarge, i2.8xlarge, hi1.4xlarge, hs1.8xlarge, c1.medium, c1.xlarge, c3.large, c3.xlarge, c3.2xlarge, c3.4xlarge, c3.8xlarge, cc1.4xlarge, cc2.8xlarge, g2.2xlarge, cg1.4xlarge
*
* @param instanceType The instance type.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see InstanceType
*/
public SpotPrice withInstanceType(String instanceType) {
this.instanceType = instanceType;
return this;
}
/**
* The instance type.
*
* Constraints:
* Allowed Values: t1.micro, m1.small, m1.medium, m1.large, m1.xlarge, m3.medium, m3.large, m3.xlarge, m3.2xlarge, m2.xlarge, m2.2xlarge, m2.4xlarge, cr1.8xlarge, i2.xlarge, i2.2xlarge, i2.4xlarge, i2.8xlarge, hi1.4xlarge, hs1.8xlarge, c1.medium, c1.xlarge, c3.large, c3.xlarge, c3.2xlarge, c3.4xlarge, c3.8xlarge, cc1.4xlarge, cc2.8xlarge, g2.2xlarge, cg1.4xlarge
*
* @param instanceType The instance type.
*
* @see InstanceType
*/
public void setInstanceType(InstanceType instanceType) {
this.instanceType = instanceType.toString();
}
/**
* The instance type.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: t1.micro, m1.small, m1.medium, m1.large, m1.xlarge, m3.medium, m3.large, m3.xlarge, m3.2xlarge, m2.xlarge, m2.2xlarge, m2.4xlarge, cr1.8xlarge, i2.xlarge, i2.2xlarge, i2.4xlarge, i2.8xlarge, hi1.4xlarge, hs1.8xlarge, c1.medium, c1.xlarge, c3.large, c3.xlarge, c3.2xlarge, c3.4xlarge, c3.8xlarge, cc1.4xlarge, cc2.8xlarge, g2.2xlarge, cg1.4xlarge
*
* @param instanceType The instance type.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see InstanceType
*/
public SpotPrice withInstanceType(InstanceType instanceType) {
this.instanceType = instanceType.toString();
return this;
}
/**
* A general description of the AMI.
*
* Constraints:
* Allowed Values: Linux/UNIX, Linux/UNIX (Amazon VPC), Windows, Windows (Amazon VPC)
*
* @return A general description of the AMI.
*
* @see RIProductDescription
*/
public String getProductDescription() {
return productDescription;
}
/**
* A general description of the AMI.
*
* Constraints:
* Allowed Values: Linux/UNIX, Linux/UNIX (Amazon VPC), Windows, Windows (Amazon VPC)
*
* @param productDescription A general description of the AMI.
*
* @see RIProductDescription
*/
public void setProductDescription(String productDescription) {
this.productDescription = productDescription;
}
/**
* A general description of the AMI.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: Linux/UNIX, Linux/UNIX (Amazon VPC), Windows, Windows (Amazon VPC)
*
* @param productDescription A general description of the AMI.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see RIProductDescription
*/
public SpotPrice withProductDescription(String productDescription) {
this.productDescription = productDescription;
return this;
}
/**
* A general description of the AMI.
*
* Constraints:
* Allowed Values: Linux/UNIX, Linux/UNIX (Amazon VPC), Windows, Windows (Amazon VPC)
*
* @param productDescription A general description of the AMI.
*
* @see RIProductDescription
*/
public void setProductDescription(RIProductDescription productDescription) {
this.productDescription = productDescription.toString();
}
/**
* A general description of the AMI.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: Linux/UNIX, Linux/UNIX (Amazon VPC), Windows, Windows (Amazon VPC)
*
* @param productDescription A general description of the AMI.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see RIProductDescription
*/
public SpotPrice withProductDescription(RIProductDescription productDescription) {
this.productDescription = productDescription.toString();
return this;
}
/**
* The maximum price you will pay to launch one or more Spot Instances.
*
* @return The maximum price you will pay to launch one or more Spot Instances.
*/
public String getSpotPrice() {
return spotPrice;
}
/**
* The maximum price you will pay to launch one or more Spot Instances.
*
* @param spotPrice The maximum price you will pay to launch one or more Spot Instances.
*/
public void setSpotPrice(String spotPrice) {
this.spotPrice = spotPrice;
}
/**
* The maximum price you will pay to launch one or more Spot Instances.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param spotPrice The maximum price you will pay to launch one or more Spot Instances.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public SpotPrice withSpotPrice(String spotPrice) {
this.spotPrice = spotPrice;
return this;
}
/**
* The date and time the request was created.
*
* @return The date and time the request was created.
*/
public java.util.Date getTimestamp() {
return timestamp;
}
/**
* The date and time the request was created.
*
* @param timestamp The date and time the request was created.
*/
public void setTimestamp(java.util.Date timestamp) {
this.timestamp = timestamp;
}
/**
* The date and time the request was created.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param timestamp The date and time the request was created.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public SpotPrice withTimestamp(java.util.Date timestamp) {
this.timestamp = timestamp;
return this;
}
/**
* The Availability Zone.
*
* @return The Availability Zone.
*/
public String getAvailabilityZone() {
return availabilityZone;
}
/**
* The Availability Zone.
*
* @param availabilityZone The Availability Zone.
*/
public void setAvailabilityZone(String availabilityZone) {
this.availabilityZone = availabilityZone;
}
/**
* The Availability Zone.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param availabilityZone The Availability Zone.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public SpotPrice withAvailabilityZone(String availabilityZone) {
this.availabilityZone = availabilityZone;
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getInstanceType() != null) sb.append("InstanceType: " + getInstanceType() + ",");
if (getProductDescription() != null) sb.append("ProductDescription: " + getProductDescription() + ",");
if (getSpotPrice() != null) sb.append("SpotPrice: " + getSpotPrice() + ",");
if (getTimestamp() != null) sb.append("Timestamp: " + getTimestamp() + ",");
if (getAvailabilityZone() != null) sb.append("AvailabilityZone: " + getAvailabilityZone() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getInstanceType() == null) ? 0 : getInstanceType().hashCode());
hashCode = prime * hashCode + ((getProductDescription() == null) ? 0 : getProductDescription().hashCode());
hashCode = prime * hashCode + ((getSpotPrice() == null) ? 0 : getSpotPrice().hashCode());
hashCode = prime * hashCode + ((getTimestamp() == null) ? 0 : getTimestamp().hashCode());
hashCode = prime * hashCode + ((getAvailabilityZone() == null) ? 0 : getAvailabilityZone().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof SpotPrice == false) return false;
SpotPrice other = (SpotPrice)obj;
if (other.getInstanceType() == null ^ this.getInstanceType() == null) return false;
if (other.getInstanceType() != null && other.getInstanceType().equals(this.getInstanceType()) == false) return false;
if (other.getProductDescription() == null ^ this.getProductDescription() == null) return false;
if (other.getProductDescription() != null && other.getProductDescription().equals(this.getProductDescription()) == false) return false;
if (other.getSpotPrice() == null ^ this.getSpotPrice() == null) return false;
if (other.getSpotPrice() != null && other.getSpotPrice().equals(this.getSpotPrice()) == false) return false;
if (other.getTimestamp() == null ^ this.getTimestamp() == null) return false;
if (other.getTimestamp() != null && other.getTimestamp().equals(this.getTimestamp()) == false) return false;
if (other.getAvailabilityZone() == null ^ this.getAvailabilityZone() == null) return false;
if (other.getAvailabilityZone() != null && other.getAvailabilityZone().equals(this.getAvailabilityZone()) == false) return false;
return true;
}
}