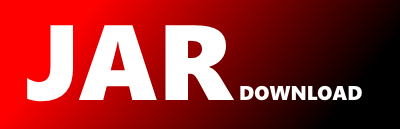
com.amazonaws.services.elasticbeanstalk.AWSElasticBeanstalk Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.elasticbeanstalk;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.elasticbeanstalk.model.*;
/**
* Interface for accessing AWSElasticBeanstalk.
* AWS Elastic Beanstalk
* This is the AWS Elastic Beanstalk API Reference. This guide provides
* detailed information about AWS Elastic Beanstalk actions, data types,
* parameters, and errors.
*
*
* AWS Elastic Beanstalk is a tool that makes it easy for you to create,
* deploy, and manage scalable, fault-tolerant applications running on
* Amazon Web Services cloud resources.
*
*
* For more information about this product, go to the
* AWS Elastic Beanstalk details page. The location of the latest AWS Elastic Beanstalk WSDL is http://elasticbeanstalk.s3.amazonaws.com/doc/2010-12-01/AWSElasticBeanstalk.wsdl
* .
*
*
* Endpoints
*
*
* For a list of region-specific endpoints that AWS Elastic Beanstalk
* supports, go to
* Regions and Endpoints
* in the Amazon Web Services Glossary .
*
*/
public interface AWSElasticBeanstalk {
/**
* Overrides the default endpoint for this client ("https://elasticbeanstalk.us-east-1.amazonaws.com").
* Callers can use this method to control which AWS region they want to work with.
*
* Callers can pass in just the endpoint (ex: "elasticbeanstalk.us-east-1.amazonaws.com") or a full
* URL, including the protocol (ex: "https://elasticbeanstalk.us-east-1.amazonaws.com"). If the
* protocol is not specified here, the default protocol from this client's
* {@link ClientConfiguration} will be used, which by default is HTTPS.
*
* For more information on using AWS regions with the AWS SDK for Java, and
* a complete list of all available endpoints for all AWS services, see:
*
* http://developer.amazonwebservices.com/connect/entry.jspa?externalID=3912
*
* This method is not threadsafe. An endpoint should be configured when the
* client is created and before any service requests are made. Changing it
* afterwards creates inevitable race conditions for any service requests in
* transit or retrying.
*
* @param endpoint
* The endpoint (ex: "elasticbeanstalk.us-east-1.amazonaws.com") or a full URL,
* including the protocol (ex: "https://elasticbeanstalk.us-east-1.amazonaws.com") of
* the region specific AWS endpoint this client will communicate
* with.
*
* @throws IllegalArgumentException
* If any problems are detected with the specified endpoint.
*/
public void setEndpoint(String endpoint) throws java.lang.IllegalArgumentException;
/**
* An alternative to {@link AWSElasticBeanstalk#setEndpoint(String)}, sets the
* regional endpoint for this client's service calls. Callers can use this
* method to control which AWS region they want to work with.
*
* By default, all service endpoints in all regions use the https protocol.
* To use http instead, specify it in the {@link ClientConfiguration}
* supplied at construction.
*
* This method is not threadsafe. A region should be configured when the
* client is created and before any service requests are made. Changing it
* afterwards creates inevitable race conditions for any service requests in
* transit or retrying.
*
* @param region
* The region this client will communicate with. See
* {@link Region#getRegion(com.amazonaws.regions.Regions)} for
* accessing a given region.
* @throws java.lang.IllegalArgumentException
* If the given region is null, or if this service isn't
* available in the given region. See
* {@link Region#isServiceSupported(String)}
* @see Region#getRegion(com.amazonaws.regions.Regions)
* @see Region#createClient(Class, com.amazonaws.auth.AWSCredentialsProvider, ClientConfiguration)
*/
public void setRegion(Region region) throws java.lang.IllegalArgumentException;
/**
*
* Checks if the specified CNAME is available.
*
*
* @param checkDNSAvailabilityRequest Container for the necessary
* parameters to execute the CheckDNSAvailability service method on
* AWSElasticBeanstalk.
*
* @return The response from the CheckDNSAvailability service method, as
* returned by AWSElasticBeanstalk.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public CheckDNSAvailabilityResult checkDNSAvailability(CheckDNSAvailabilityRequest checkDNSAvailabilityRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Describes the configuration options that are used in a particular
* configuration template or environment, or that a specified solution
* stack defines. The description includes the values the options, their
* default values, and an indication of the required action on a running
* environment if an option value is changed.
*
*
* @param describeConfigurationOptionsRequest Container for the necessary
* parameters to execute the DescribeConfigurationOptions service method
* on AWSElasticBeanstalk.
*
* @return The response from the DescribeConfigurationOptions service
* method, as returned by AWSElasticBeanstalk.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeConfigurationOptionsResult describeConfigurationOptions(DescribeConfigurationOptionsRequest describeConfigurationOptionsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes the specified configuration template.
*
*
* NOTE:When you launch an environment using a configuration
* template, the environment gets a copy of the template. You can delete
* or modify the environment's copy of the template without affecting the
* running environment.
*
*
* @param deleteConfigurationTemplateRequest Container for the necessary
* parameters to execute the DeleteConfigurationTemplate service method
* on AWSElasticBeanstalk.
*
*
* @throws OperationInProgressException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteConfigurationTemplate(DeleteConfigurationTemplateRequest deleteConfigurationTemplateRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Launches an environment for the specified application using the
* specified configuration.
*
*
* @param createEnvironmentRequest Container for the necessary parameters
* to execute the CreateEnvironment service method on
* AWSElasticBeanstalk.
*
* @return The response from the CreateEnvironment service method, as
* returned by AWSElasticBeanstalk.
*
* @throws TooManyEnvironmentsException
* @throws InsufficientPrivilegesException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateEnvironmentResult createEnvironment(CreateEnvironmentRequest createEnvironmentRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates the Amazon S3 storage location for the account.
*
*
* This location is used to store user log files.
*
*
* @param createStorageLocationRequest Container for the necessary
* parameters to execute the CreateStorageLocation service method on
* AWSElasticBeanstalk.
*
* @return The response from the CreateStorageLocation service method, as
* returned by AWSElasticBeanstalk.
*
* @throws InsufficientPrivilegesException
* @throws S3SubscriptionRequiredException
* @throws TooManyBucketsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateStorageLocationResult createStorageLocation(CreateStorageLocationRequest createStorageLocationRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Initiates a request to compile the specified type of information of
* the deployed environment.
*
*
* Setting the InfoType
to tail
compiles the
* last lines from the application server log files of every Amazon EC2
* instance in your environment. Use RetrieveEnvironmentInfo to access
* the compiled information.
*
*
* Related Topics
*
*
*
* - RetrieveEnvironmentInfo
*
*
*
* @param requestEnvironmentInfoRequest Container for the necessary
* parameters to execute the RequestEnvironmentInfo service method on
* AWSElasticBeanstalk.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public void requestEnvironmentInfo(RequestEnvironmentInfoRequest requestEnvironmentInfoRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates an application version for the specified application.
*
*
* NOTE:Once you create an application version with a specified
* Amazon S3 bucket and key location, you cannot change that Amazon S3
* location. If you change the Amazon S3 location, you receive an
* exception when you attempt to launch an environment from the
* application version.
*
*
* @param createApplicationVersionRequest Container for the necessary
* parameters to execute the CreateApplicationVersion service method on
* AWSElasticBeanstalk.
*
* @return The response from the CreateApplicationVersion service method,
* as returned by AWSElasticBeanstalk.
*
* @throws TooManyApplicationsException
* @throws InsufficientPrivilegesException
* @throws TooManyApplicationVersionsException
* @throws S3LocationNotInServiceRegionException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateApplicationVersionResult createApplicationVersion(CreateApplicationVersionRequest createApplicationVersionRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes the specified version from the specified application.
*
*
* NOTE:You cannot delete an application version that is
* associated with a running environment.
*
*
* @param deleteApplicationVersionRequest Container for the necessary
* parameters to execute the DeleteApplicationVersion service method on
* AWSElasticBeanstalk.
*
*
* @throws OperationInProgressException
* @throws InsufficientPrivilegesException
* @throws SourceBundleDeletionException
* @throws S3LocationNotInServiceRegionException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteApplicationVersion(DeleteApplicationVersionRequest deleteApplicationVersionRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns descriptions for existing application versions.
*
*
* @param describeApplicationVersionsRequest Container for the necessary
* parameters to execute the DescribeApplicationVersions service method
* on AWSElasticBeanstalk.
*
* @return The response from the DescribeApplicationVersions service
* method, as returned by AWSElasticBeanstalk.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeApplicationVersionsResult describeApplicationVersions(DescribeApplicationVersionsRequest describeApplicationVersionsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes the specified application along with all associated versions
* and configurations. The application versions will not be deleted from
* your Amazon S3 bucket.
*
*
* NOTE:You cannot delete an application that has a running
* environment.
*
*
* @param deleteApplicationRequest Container for the necessary parameters
* to execute the DeleteApplication service method on
* AWSElasticBeanstalk.
*
*
* @throws OperationInProgressException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteApplication(DeleteApplicationRequest deleteApplicationRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Updates the specified application version to have the specified
* properties.
*
*
* NOTE: If a property (for example, description) is not provided,
* the value remains unchanged. To clear properties, specify an empty
* string.
*
*
* @param updateApplicationVersionRequest Container for the necessary
* parameters to execute the UpdateApplicationVersion service method on
* AWSElasticBeanstalk.
*
* @return The response from the UpdateApplicationVersion service method,
* as returned by AWSElasticBeanstalk.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public UpdateApplicationVersionResult updateApplicationVersion(UpdateApplicationVersionRequest updateApplicationVersionRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates an application that has one configuration template named
* default
and no application versions.
*
*
* @param createApplicationRequest Container for the necessary parameters
* to execute the CreateApplication service method on
* AWSElasticBeanstalk.
*
* @return The response from the CreateApplication service method, as
* returned by AWSElasticBeanstalk.
*
* @throws TooManyApplicationsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateApplicationResult createApplication(CreateApplicationRequest createApplicationRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Swaps the CNAMEs of two environments.
*
*
* @param swapEnvironmentCNAMEsRequest Container for the necessary
* parameters to execute the SwapEnvironmentCNAMEs service method on
* AWSElasticBeanstalk.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public void swapEnvironmentCNAMEs(SwapEnvironmentCNAMEsRequest swapEnvironmentCNAMEsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Updates the specified configuration template to have the specified
* properties or configuration option values.
*
*
* NOTE: If a property (for example, ApplicationName) is not
* provided, its value remains unchanged. To clear such properties,
* specify an empty string.
*
*
* Related Topics
*
*
*
* - DescribeConfigurationOptions
*
*
*
* @param updateConfigurationTemplateRequest Container for the necessary
* parameters to execute the UpdateConfigurationTemplate service method
* on AWSElasticBeanstalk.
*
* @return The response from the UpdateConfigurationTemplate service
* method, as returned by AWSElasticBeanstalk.
*
* @throws InsufficientPrivilegesException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public UpdateConfigurationTemplateResult updateConfigurationTemplate(UpdateConfigurationTemplateRequest updateConfigurationTemplateRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Retrieves the compiled information from a RequestEnvironmentInfo
* request.
*
*
* Related Topics
*
*
*
* - RequestEnvironmentInfo
*
*
*
* @param retrieveEnvironmentInfoRequest Container for the necessary
* parameters to execute the RetrieveEnvironmentInfo service method on
* AWSElasticBeanstalk.
*
* @return The response from the RetrieveEnvironmentInfo service method,
* as returned by AWSElasticBeanstalk.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public RetrieveEnvironmentInfoResult retrieveEnvironmentInfo(RetrieveEnvironmentInfoRequest retrieveEnvironmentInfoRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a list of the available solution stack names.
*
*
* @param listAvailableSolutionStacksRequest Container for the necessary
* parameters to execute the ListAvailableSolutionStacks service method
* on AWSElasticBeanstalk.
*
* @return The response from the ListAvailableSolutionStacks service
* method, as returned by AWSElasticBeanstalk.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListAvailableSolutionStacksResult listAvailableSolutionStacks(ListAvailableSolutionStacksRequest listAvailableSolutionStacksRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Updates the specified application to have the specified properties.
*
*
* NOTE: If a property (for example, description) is not provided,
* the value remains unchanged. To clear these properties, specify an
* empty string.
*
*
* @param updateApplicationRequest Container for the necessary parameters
* to execute the UpdateApplication service method on
* AWSElasticBeanstalk.
*
* @return The response from the UpdateApplication service method, as
* returned by AWSElasticBeanstalk.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public UpdateApplicationResult updateApplication(UpdateApplicationRequest updateApplicationRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns descriptions for existing environments.
*
*
* @param describeEnvironmentsRequest Container for the necessary
* parameters to execute the DescribeEnvironments service method on
* AWSElasticBeanstalk.
*
* @return The response from the DescribeEnvironments service method, as
* returned by AWSElasticBeanstalk.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeEnvironmentsResult describeEnvironments(DescribeEnvironmentsRequest describeEnvironmentsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns AWS resources for this environment.
*
*
* @param describeEnvironmentResourcesRequest Container for the necessary
* parameters to execute the DescribeEnvironmentResources service method
* on AWSElasticBeanstalk.
*
* @return The response from the DescribeEnvironmentResources service
* method, as returned by AWSElasticBeanstalk.
*
* @throws InsufficientPrivilegesException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeEnvironmentResourcesResult describeEnvironmentResources(DescribeEnvironmentResourcesRequest describeEnvironmentResourcesRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Terminates the specified environment.
*
*
* @param terminateEnvironmentRequest Container for the necessary
* parameters to execute the TerminateEnvironment service method on
* AWSElasticBeanstalk.
*
* @return The response from the TerminateEnvironment service method, as
* returned by AWSElasticBeanstalk.
*
* @throws InsufficientPrivilegesException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public TerminateEnvironmentResult terminateEnvironment(TerminateEnvironmentRequest terminateEnvironmentRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Takes a set of configuration settings and either a configuration
* template or environment, and determines whether those values are
* valid.
*
*
* This action returns a list of messages indicating any errors or
* warnings associated with the selection of option values.
*
*
* @param validateConfigurationSettingsRequest Container for the
* necessary parameters to execute the ValidateConfigurationSettings
* service method on AWSElasticBeanstalk.
*
* @return The response from the ValidateConfigurationSettings service
* method, as returned by AWSElasticBeanstalk.
*
* @throws InsufficientPrivilegesException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public ValidateConfigurationSettingsResult validateConfigurationSettings(ValidateConfigurationSettingsRequest validateConfigurationSettingsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Causes the environment to restart the application container server
* running on each Amazon EC2 instance.
*
*
* @param restartAppServerRequest Container for the necessary parameters
* to execute the RestartAppServer service method on AWSElasticBeanstalk.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public void restartAppServer(RestartAppServerRequest restartAppServerRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes the draft configuration associated with the running
* environment.
*
*
* Updating a running environment with any configuration changes creates
* a draft configuration set. You can get the draft configuration using
* DescribeConfigurationSettings while the update is in progress or if
* the update fails. The DeploymentStatus
for the draft
* configuration indicates whether the deployment is in process or has
* failed. The draft configuration remains in existence until it is
* deleted with this action.
*
*
* @param deleteEnvironmentConfigurationRequest Container for the
* necessary parameters to execute the DeleteEnvironmentConfiguration
* service method on AWSElasticBeanstalk.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteEnvironmentConfiguration(DeleteEnvironmentConfigurationRequest deleteEnvironmentConfigurationRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Updates the environment description, deploys a new application
* version, updates the configuration settings to an entirely new
* configuration template, or updates select configuration option values
* in the running environment.
*
*
* Attempting to update both the release and configuration is not
* allowed and AWS Elastic Beanstalk returns an
* InvalidParameterCombination
error.
*
*
* When updating the configuration settings to a new template or
* individual settings, a draft configuration is created and
* DescribeConfigurationSettings for this environment returns two setting
* descriptions with different DeploymentStatus
values.
*
*
* @param updateEnvironmentRequest Container for the necessary parameters
* to execute the UpdateEnvironment service method on
* AWSElasticBeanstalk.
*
* @return The response from the UpdateEnvironment service method, as
* returned by AWSElasticBeanstalk.
*
* @throws InsufficientPrivilegesException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public UpdateEnvironmentResult updateEnvironment(UpdateEnvironmentRequest updateEnvironmentRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates a configuration template. Templates are associated with a
* specific application and are used to deploy different versions of the
* application with the same configuration settings.
*
*
* Related Topics
*
*
*
* - DescribeConfigurationOptions
* - DescribeConfigurationSettings
* - ListAvailableSolutionStacks
*
*
*
* @param createConfigurationTemplateRequest Container for the necessary
* parameters to execute the CreateConfigurationTemplate service method
* on AWSElasticBeanstalk.
*
* @return The response from the CreateConfigurationTemplate service
* method, as returned by AWSElasticBeanstalk.
*
* @throws InsufficientPrivilegesException
* @throws TooManyConfigurationTemplatesException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateConfigurationTemplateResult createConfigurationTemplate(CreateConfigurationTemplateRequest createConfigurationTemplateRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a description of the settings for the specified configuration
* set, that is, either a configuration template or the configuration set
* associated with a running environment.
*
*
* When describing the settings for the configuration set associated
* with a running environment, it is possible to receive two sets of
* setting descriptions. One is the deployed configuration set, and the
* other is a draft configuration of an environment that is either in the
* process of deployment or that failed to deploy.
*
*
* Related Topics
*
*
*
* - DeleteEnvironmentConfiguration
*
*
*
* @param describeConfigurationSettingsRequest Container for the
* necessary parameters to execute the DescribeConfigurationSettings
* service method on AWSElasticBeanstalk.
*
* @return The response from the DescribeConfigurationSettings service
* method, as returned by AWSElasticBeanstalk.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeConfigurationSettingsResult describeConfigurationSettings(DescribeConfigurationSettingsRequest describeConfigurationSettingsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns the descriptions of existing applications.
*
*
* @param describeApplicationsRequest Container for the necessary
* parameters to execute the DescribeApplications service method on
* AWSElasticBeanstalk.
*
* @return The response from the DescribeApplications service method, as
* returned by AWSElasticBeanstalk.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeApplicationsResult describeApplications(DescribeApplicationsRequest describeApplicationsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes and recreates all of the AWS resources (for example: the Auto
* Scaling group, load balancer, etc.) for a specified environment and
* forces a restart.
*
*
* @param rebuildEnvironmentRequest Container for the necessary
* parameters to execute the RebuildEnvironment service method on
* AWSElasticBeanstalk.
*
*
* @throws InsufficientPrivilegesException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public void rebuildEnvironment(RebuildEnvironmentRequest rebuildEnvironmentRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns list of event descriptions matching criteria up to the last 6
* weeks.
*
*
* NOTE: This action returns the most recent 1,000 events from the
* specified NextToken.
*
*
* @param describeEventsRequest Container for the necessary parameters to
* execute the DescribeEvents service method on AWSElasticBeanstalk.
*
* @return The response from the DescribeEvents service method, as
* returned by AWSElasticBeanstalk.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeEventsResult describeEvents(DescribeEventsRequest describeEventsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates the Amazon S3 storage location for the account.
*
*
* This location is used to store user log files.
*
*
* @return The response from the CreateStorageLocation service method, as
* returned by AWSElasticBeanstalk.
*
* @throws InsufficientPrivilegesException
* @throws S3SubscriptionRequiredException
* @throws TooManyBucketsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateStorageLocationResult createStorageLocation() throws AmazonServiceException, AmazonClientException;
/**
*
* Returns descriptions for existing application versions.
*
*
* @return The response from the DescribeApplicationVersions service
* method, as returned by AWSElasticBeanstalk.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeApplicationVersionsResult describeApplicationVersions() throws AmazonServiceException, AmazonClientException;
/**
*
* Swaps the CNAMEs of two environments.
*
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public void swapEnvironmentCNAMEs() throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a list of the available solution stack names.
*
*
* @return The response from the ListAvailableSolutionStacks service
* method, as returned by AWSElasticBeanstalk.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListAvailableSolutionStacksResult listAvailableSolutionStacks() throws AmazonServiceException, AmazonClientException;
/**
*
* Returns descriptions for existing environments.
*
*
* @return The response from the DescribeEnvironments service method, as
* returned by AWSElasticBeanstalk.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeEnvironmentsResult describeEnvironments() throws AmazonServiceException, AmazonClientException;
/**
*
* Returns the descriptions of existing applications.
*
*
* @return The response from the DescribeApplications service method, as
* returned by AWSElasticBeanstalk.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeApplicationsResult describeApplications() throws AmazonServiceException, AmazonClientException;
/**
*
* Returns list of event descriptions matching criteria up to the last 6
* weeks.
*
*
* NOTE: This action returns the most recent 1,000 events from the
* specified NextToken.
*
*
* @return The response from the DescribeEvents service method, as
* returned by AWSElasticBeanstalk.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSElasticBeanstalk indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeEventsResult describeEvents() throws AmazonServiceException, AmazonClientException;
/**
* Shuts down this client object, releasing any resources that might be held
* open. This is an optional method, and callers are not expected to call
* it, but can if they want to explicitly release any open resources. Once a
* client has been shutdown, it should not be used to make any more
* requests.
*/
public void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for
* debugging issues where a service isn't acting as expected. This data isn't considered part
* of the result data returned by an operation, so it's available through this separate,
* diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access
* this extra diagnostic information for an executed request, you should use this method
* to retrieve it as soon as possible after executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none
* is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}