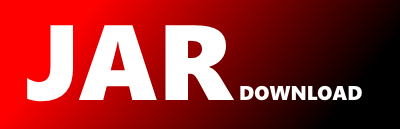
com.amazonaws.services.elasticbeanstalk.model.ConfigurationOptionDescription Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.elasticbeanstalk.model;
import java.io.Serializable;
/**
*
* Describes the possible values for a configuration option.
*
*/
public class ConfigurationOptionDescription implements Serializable {
/**
* A unique namespace identifying the option's associated AWS resource.
*/
private String namespace;
/**
* The name of the configuration option.
*/
private String name;
/**
* The default value for this configuration option.
*/
private String defaultValue;
/**
* An indication of which action is required if the value for this
* configuration option changes: NoInterruption - There is no interruption
* to the environment or application availability.
RestartEnvironment - The environment is
* restarted, all AWS resources are deleted and recreated, and the
* environment is unavailable during the process.
RestartApplicationServer - The
* environment is available the entire time. However, a short application
* outage occurs when the application servers on the running Amazon EC2
* instances are restarted.
-
*
NoInterruption
: There is no interruption to the
* environment or application availability. -
*
RestartEnvironment
: The environment is entirely
* restarted, all AWS resources are deleted and recreated, and the
* environment is unavailable during the process. -
*
RestartApplicationServer
: The environment is available
* the entire time. However, a short application outage occurs when the
* application servers on the running Amazon EC2 instances are restarted.
*
*/
private String changeSeverity;
/**
* An indication of whether the user defined this configuration option:
* true
: This
* configuration option was defined by the user. It is a valid choice for
* specifying this as an Option to Remove when updating configuration
* settings.
false
: This
* configuration was not defined by the user.
* -
true
: This configuration option was defined by
* the user. It is a valid choice for specifying if this as an
* Option to Remove
when updating configuration settings.
*
-
false
: This configuration was not defined by
* the user.
Constraint: You can remove only
* UserDefined
options from a configuration.
Valid
* Values: true
| false
*/
private Boolean userDefined;
/**
* An indication of which type of values this option has and whether it
* is allowable to select one or more than one of the possible values:
* Scalar
: Values
* for this option are a single selection from the possible values, or a
* unformatted string or numeric value governed by the MIN/MAX/Regex
* constraints:
List
:
* Values for this option are multiple selections of the possible values.
*
Boolean
: Values for
* this option are either true
or false
.
*
-
Scalar
: Values for
* this option are a single selection from the possible values, or an
* unformatted string, or numeric value governed by the
* MIN/MAX/Regex
constraints. -
List
:
* Values for this option are multiple selections from the possible
* values. -
Boolean
: Values for this option are
* either true
or false
.
*
* Constraints:
* Allowed Values: Scalar, List
*/
private String valueType;
/**
* If specified, values for the configuration option are selected from
* this list.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag valueOptions;
/**
* If specified, the configuration option must be a numeric value greater
* than this value.
*/
private Integer minValue;
/**
* If specified, the configuration option must be a numeric value less
* than this value.
*/
private Integer maxValue;
/**
* If specified, the configuration option must be a string value no
* longer than this value.
*/
private Integer maxLength;
/**
* If specified, the configuration option must be a string value that
* satisfies this regular expression.
*/
private OptionRestrictionRegex regex;
/**
* Default constructor for a new ConfigurationOptionDescription object. Callers should use the
* setter or fluent setter (with...) methods to initialize this object after creating it.
*/
public ConfigurationOptionDescription() {}
/**
* A unique namespace identifying the option's associated AWS resource.
*
* @return A unique namespace identifying the option's associated AWS resource.
*/
public String getNamespace() {
return namespace;
}
/**
* A unique namespace identifying the option's associated AWS resource.
*
* @param namespace A unique namespace identifying the option's associated AWS resource.
*/
public void setNamespace(String namespace) {
this.namespace = namespace;
}
/**
* A unique namespace identifying the option's associated AWS resource.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param namespace A unique namespace identifying the option's associated AWS resource.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public ConfigurationOptionDescription withNamespace(String namespace) {
this.namespace = namespace;
return this;
}
/**
* The name of the configuration option.
*
* @return The name of the configuration option.
*/
public String getName() {
return name;
}
/**
* The name of the configuration option.
*
* @param name The name of the configuration option.
*/
public void setName(String name) {
this.name = name;
}
/**
* The name of the configuration option.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param name The name of the configuration option.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public ConfigurationOptionDescription withName(String name) {
this.name = name;
return this;
}
/**
* The default value for this configuration option.
*
* @return The default value for this configuration option.
*/
public String getDefaultValue() {
return defaultValue;
}
/**
* The default value for this configuration option.
*
* @param defaultValue The default value for this configuration option.
*/
public void setDefaultValue(String defaultValue) {
this.defaultValue = defaultValue;
}
/**
* The default value for this configuration option.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param defaultValue The default value for this configuration option.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public ConfigurationOptionDescription withDefaultValue(String defaultValue) {
this.defaultValue = defaultValue;
return this;
}
/**
* An indication of which action is required if the value for this
* configuration option changes: NoInterruption - There is no interruption
* to the environment or application availability.
RestartEnvironment - The environment is
* restarted, all AWS resources are deleted and recreated, and the
* environment is unavailable during the process.
RestartApplicationServer - The
* environment is available the entire time. However, a short application
* outage occurs when the application servers on the running Amazon EC2
* instances are restarted.
-
*
NoInterruption
: There is no interruption to the
* environment or application availability. -
*
RestartEnvironment
: The environment is entirely
* restarted, all AWS resources are deleted and recreated, and the
* environment is unavailable during the process. -
*
RestartApplicationServer
: The environment is available
* the entire time. However, a short application outage occurs when the
* application servers on the running Amazon EC2 instances are restarted.
*
*
* @return An indication of which action is required if the value for this
* configuration option changes: NoInterruption - There is no interruption
* to the environment or application availability.
RestartEnvironment - The environment is
* restarted, all AWS resources are deleted and recreated, and the
* environment is unavailable during the process.
RestartApplicationServer - The
* environment is available the entire time. However, a short application
* outage occurs when the application servers on the running Amazon EC2
* instances are restarted.
-
*
NoInterruption
: There is no interruption to the
* environment or application availability. -
*
RestartEnvironment
: The environment is entirely
* restarted, all AWS resources are deleted and recreated, and the
* environment is unavailable during the process. -
*
RestartApplicationServer
: The environment is available
* the entire time. However, a short application outage occurs when the
* application servers on the running Amazon EC2 instances are restarted.
*
*/
public String getChangeSeverity() {
return changeSeverity;
}
/**
* An indication of which action is required if the value for this
* configuration option changes: NoInterruption - There is no interruption
* to the environment or application availability.
RestartEnvironment - The environment is
* restarted, all AWS resources are deleted and recreated, and the
* environment is unavailable during the process.
RestartApplicationServer - The
* environment is available the entire time. However, a short application
* outage occurs when the application servers on the running Amazon EC2
* instances are restarted.
-
*
NoInterruption
: There is no interruption to the
* environment or application availability. -
*
RestartEnvironment
: The environment is entirely
* restarted, all AWS resources are deleted and recreated, and the
* environment is unavailable during the process. -
*
RestartApplicationServer
: The environment is available
* the entire time. However, a short application outage occurs when the
* application servers on the running Amazon EC2 instances are restarted.
*
*
* @param changeSeverity An indication of which action is required if the value for this
* configuration option changes: NoInterruption - There is no interruption
* to the environment or application availability.
RestartEnvironment - The environment is
* restarted, all AWS resources are deleted and recreated, and the
* environment is unavailable during the process.
RestartApplicationServer - The
* environment is available the entire time. However, a short application
* outage occurs when the application servers on the running Amazon EC2
* instances are restarted.
-
*
NoInterruption
: There is no interruption to the
* environment or application availability. -
*
RestartEnvironment
: The environment is entirely
* restarted, all AWS resources are deleted and recreated, and the
* environment is unavailable during the process. -
*
RestartApplicationServer
: The environment is available
* the entire time. However, a short application outage occurs when the
* application servers on the running Amazon EC2 instances are restarted.
*
*/
public void setChangeSeverity(String changeSeverity) {
this.changeSeverity = changeSeverity;
}
/**
* An indication of which action is required if the value for this
* configuration option changes: NoInterruption - There is no interruption
* to the environment or application availability.
RestartEnvironment - The environment is
* restarted, all AWS resources are deleted and recreated, and the
* environment is unavailable during the process.
RestartApplicationServer - The
* environment is available the entire time. However, a short application
* outage occurs when the application servers on the running Amazon EC2
* instances are restarted.
-
*
NoInterruption
: There is no interruption to the
* environment or application availability. -
*
RestartEnvironment
: The environment is entirely
* restarted, all AWS resources are deleted and recreated, and the
* environment is unavailable during the process. -
*
RestartApplicationServer
: The environment is available
* the entire time. However, a short application outage occurs when the
* application servers on the running Amazon EC2 instances are restarted.
*
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param changeSeverity An indication of which action is required if the value for this
* configuration option changes: NoInterruption - There is no interruption
* to the environment or application availability.
RestartEnvironment - The environment is
* restarted, all AWS resources are deleted and recreated, and the
* environment is unavailable during the process.
RestartApplicationServer - The
* environment is available the entire time. However, a short application
* outage occurs when the application servers on the running Amazon EC2
* instances are restarted.
-
*
NoInterruption
: There is no interruption to the
* environment or application availability. -
*
RestartEnvironment
: The environment is entirely
* restarted, all AWS resources are deleted and recreated, and the
* environment is unavailable during the process. -
*
RestartApplicationServer
: The environment is available
* the entire time. However, a short application outage occurs when the
* application servers on the running Amazon EC2 instances are restarted.
*
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public ConfigurationOptionDescription withChangeSeverity(String changeSeverity) {
this.changeSeverity = changeSeverity;
return this;
}
/**
* An indication of whether the user defined this configuration option:
* true
: This
* configuration option was defined by the user. It is a valid choice for
* specifying this as an Option to Remove when updating configuration
* settings.
false
: This
* configuration was not defined by the user.
* -
true
: This configuration option was defined by
* the user. It is a valid choice for specifying if this as an
* Option to Remove
when updating configuration settings.
*
-
false
: This configuration was not defined by
* the user.
Constraint: You can remove only
* UserDefined
options from a configuration.
Valid
* Values: true
| false
*
* @return An indication of whether the user defined this configuration option:
* true
: This
* configuration option was defined by the user. It is a valid choice for
* specifying this as an Option to Remove when updating configuration
* settings.
false
: This
* configuration was not defined by the user.
* -
true
: This configuration option was defined by
* the user. It is a valid choice for specifying if this as an
* Option to Remove
when updating configuration settings.
*
-
false
: This configuration was not defined by
* the user.
Constraint: You can remove only
* UserDefined
options from a configuration.
Valid
* Values: true
| false
*/
public Boolean isUserDefined() {
return userDefined;
}
/**
* An indication of whether the user defined this configuration option:
* true
: This
* configuration option was defined by the user. It is a valid choice for
* specifying this as an Option to Remove when updating configuration
* settings.
false
: This
* configuration was not defined by the user.
* -
true
: This configuration option was defined by
* the user. It is a valid choice for specifying if this as an
* Option to Remove
when updating configuration settings.
*
-
false
: This configuration was not defined by
* the user.
Constraint: You can remove only
* UserDefined
options from a configuration.
Valid
* Values: true
| false
*
* @param userDefined An indication of whether the user defined this configuration option:
* true
: This
* configuration option was defined by the user. It is a valid choice for
* specifying this as an Option to Remove when updating configuration
* settings.
false
: This
* configuration was not defined by the user.
* -
true
: This configuration option was defined by
* the user. It is a valid choice for specifying if this as an
* Option to Remove
when updating configuration settings.
*
-
false
: This configuration was not defined by
* the user.
Constraint: You can remove only
* UserDefined
options from a configuration.
Valid
* Values: true
| false
*/
public void setUserDefined(Boolean userDefined) {
this.userDefined = userDefined;
}
/**
* An indication of whether the user defined this configuration option:
* true
: This
* configuration option was defined by the user. It is a valid choice for
* specifying this as an Option to Remove when updating configuration
* settings.
false
: This
* configuration was not defined by the user.
* -
true
: This configuration option was defined by
* the user. It is a valid choice for specifying if this as an
* Option to Remove
when updating configuration settings.
*
-
false
: This configuration was not defined by
* the user.
Constraint: You can remove only
* UserDefined
options from a configuration.
Valid
* Values: true
| false
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param userDefined An indication of whether the user defined this configuration option:
* true
: This
* configuration option was defined by the user. It is a valid choice for
* specifying this as an Option to Remove when updating configuration
* settings.
false
: This
* configuration was not defined by the user.
* -
true
: This configuration option was defined by
* the user. It is a valid choice for specifying if this as an
* Option to Remove
when updating configuration settings.
*
-
false
: This configuration was not defined by
* the user.
Constraint: You can remove only
* UserDefined
options from a configuration.
Valid
* Values: true
| false
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public ConfigurationOptionDescription withUserDefined(Boolean userDefined) {
this.userDefined = userDefined;
return this;
}
/**
* An indication of whether the user defined this configuration option:
* true
: This
* configuration option was defined by the user. It is a valid choice for
* specifying this as an Option to Remove when updating configuration
* settings.
false
: This
* configuration was not defined by the user.
* -
true
: This configuration option was defined by
* the user. It is a valid choice for specifying if this as an
* Option to Remove
when updating configuration settings.
*
-
false
: This configuration was not defined by
* the user.
Constraint: You can remove only
* UserDefined
options from a configuration.
Valid
* Values: true
| false
*
* @return An indication of whether the user defined this configuration option:
* true
: This
* configuration option was defined by the user. It is a valid choice for
* specifying this as an Option to Remove when updating configuration
* settings.
false
: This
* configuration was not defined by the user.
* -
true
: This configuration option was defined by
* the user. It is a valid choice for specifying if this as an
* Option to Remove
when updating configuration settings.
*
-
false
: This configuration was not defined by
* the user.
Constraint: You can remove only
* UserDefined
options from a configuration.
Valid
* Values: true
| false
*/
public Boolean getUserDefined() {
return userDefined;
}
/**
* An indication of which type of values this option has and whether it
* is allowable to select one or more than one of the possible values:
* Scalar
: Values
* for this option are a single selection from the possible values, or a
* unformatted string or numeric value governed by the MIN/MAX/Regex
* constraints:
List
:
* Values for this option are multiple selections of the possible values.
*
Boolean
: Values for
* this option are either true
or false
.
*
-
Scalar
: Values for
* this option are a single selection from the possible values, or an
* unformatted string, or numeric value governed by the
* MIN/MAX/Regex
constraints. -
List
:
* Values for this option are multiple selections from the possible
* values. -
Boolean
: Values for this option are
* either true
or false
.
*
* Constraints:
* Allowed Values: Scalar, List
*
* @return An indication of which type of values this option has and whether it
* is allowable to select one or more than one of the possible values:
* Scalar
: Values
* for this option are a single selection from the possible values, or a
* unformatted string or numeric value governed by the MIN/MAX/Regex
* constraints:
List
:
* Values for this option are multiple selections of the possible values.
*
Boolean
: Values for
* this option are either true
or false
.
*
-
Scalar
: Values for
* this option are a single selection from the possible values, or an
* unformatted string, or numeric value governed by the
* MIN/MAX/Regex
constraints. -
List
:
* Values for this option are multiple selections from the possible
* values. -
Boolean
: Values for this option are
* either true
or false
.
*
* @see ConfigurationOptionValueType
*/
public String getValueType() {
return valueType;
}
/**
* An indication of which type of values this option has and whether it
* is allowable to select one or more than one of the possible values:
* Scalar
: Values
* for this option are a single selection from the possible values, or a
* unformatted string or numeric value governed by the MIN/MAX/Regex
* constraints:
List
:
* Values for this option are multiple selections of the possible values.
*
Boolean
: Values for
* this option are either true
or false
.
*
-
Scalar
: Values for
* this option are a single selection from the possible values, or an
* unformatted string, or numeric value governed by the
* MIN/MAX/Regex
constraints. -
List
:
* Values for this option are multiple selections from the possible
* values. -
Boolean
: Values for this option are
* either true
or false
.
*
* Constraints:
* Allowed Values: Scalar, List
*
* @param valueType An indication of which type of values this option has and whether it
* is allowable to select one or more than one of the possible values:
* Scalar
: Values
* for this option are a single selection from the possible values, or a
* unformatted string or numeric value governed by the MIN/MAX/Regex
* constraints:
List
:
* Values for this option are multiple selections of the possible values.
*
Boolean
: Values for
* this option are either true
or false
.
*
-
Scalar
: Values for
* this option are a single selection from the possible values, or an
* unformatted string, or numeric value governed by the
* MIN/MAX/Regex
constraints. -
List
:
* Values for this option are multiple selections from the possible
* values. -
Boolean
: Values for this option are
* either true
or false
.
*
* @see ConfigurationOptionValueType
*/
public void setValueType(String valueType) {
this.valueType = valueType;
}
/**
* An indication of which type of values this option has and whether it
* is allowable to select one or more than one of the possible values:
* Scalar
: Values
* for this option are a single selection from the possible values, or a
* unformatted string or numeric value governed by the MIN/MAX/Regex
* constraints:
List
:
* Values for this option are multiple selections of the possible values.
*
Boolean
: Values for
* this option are either true
or false
.
*
-
Scalar
: Values for
* this option are a single selection from the possible values, or an
* unformatted string, or numeric value governed by the
* MIN/MAX/Regex
constraints. -
List
:
* Values for this option are multiple selections from the possible
* values. -
Boolean
: Values for this option are
* either true
or false
.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: Scalar, List
*
* @param valueType An indication of which type of values this option has and whether it
* is allowable to select one or more than one of the possible values:
* Scalar
: Values
* for this option are a single selection from the possible values, or a
* unformatted string or numeric value governed by the MIN/MAX/Regex
* constraints:
List
:
* Values for this option are multiple selections of the possible values.
*
Boolean
: Values for
* this option are either true
or false
.
*
-
Scalar
: Values for
* this option are a single selection from the possible values, or an
* unformatted string, or numeric value governed by the
* MIN/MAX/Regex
constraints. -
List
:
* Values for this option are multiple selections from the possible
* values. -
Boolean
: Values for this option are
* either true
or false
.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see ConfigurationOptionValueType
*/
public ConfigurationOptionDescription withValueType(String valueType) {
this.valueType = valueType;
return this;
}
/**
* An indication of which type of values this option has and whether it
* is allowable to select one or more than one of the possible values:
* Scalar
: Values
* for this option are a single selection from the possible values, or a
* unformatted string or numeric value governed by the MIN/MAX/Regex
* constraints:
List
:
* Values for this option are multiple selections of the possible values.
*
Boolean
: Values for
* this option are either true
or false
.
*
-
Scalar
: Values for
* this option are a single selection from the possible values, or an
* unformatted string, or numeric value governed by the
* MIN/MAX/Regex
constraints. -
List
:
* Values for this option are multiple selections from the possible
* values. -
Boolean
: Values for this option are
* either true
or false
.
*
* Constraints:
* Allowed Values: Scalar, List
*
* @param valueType An indication of which type of values this option has and whether it
* is allowable to select one or more than one of the possible values:
* Scalar
: Values
* for this option are a single selection from the possible values, or a
* unformatted string or numeric value governed by the MIN/MAX/Regex
* constraints:
List
:
* Values for this option are multiple selections of the possible values.
*
Boolean
: Values for
* this option are either true
or false
.
*
-
Scalar
: Values for
* this option are a single selection from the possible values, or an
* unformatted string, or numeric value governed by the
* MIN/MAX/Regex
constraints. -
List
:
* Values for this option are multiple selections from the possible
* values. -
Boolean
: Values for this option are
* either true
or false
.
*
* @see ConfigurationOptionValueType
*/
public void setValueType(ConfigurationOptionValueType valueType) {
this.valueType = valueType.toString();
}
/**
* An indication of which type of values this option has and whether it
* is allowable to select one or more than one of the possible values:
* Scalar
: Values
* for this option are a single selection from the possible values, or a
* unformatted string or numeric value governed by the MIN/MAX/Regex
* constraints:
List
:
* Values for this option are multiple selections of the possible values.
*
Boolean
: Values for
* this option are either true
or false
.
*
-
Scalar
: Values for
* this option are a single selection from the possible values, or an
* unformatted string, or numeric value governed by the
* MIN/MAX/Regex
constraints. -
List
:
* Values for this option are multiple selections from the possible
* values. -
Boolean
: Values for this option are
* either true
or false
.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: Scalar, List
*
* @param valueType An indication of which type of values this option has and whether it
* is allowable to select one or more than one of the possible values:
* Scalar
: Values
* for this option are a single selection from the possible values, or a
* unformatted string or numeric value governed by the MIN/MAX/Regex
* constraints:
List
:
* Values for this option are multiple selections of the possible values.
*
Boolean
: Values for
* this option are either true
or false
.
*
-
Scalar
: Values for
* this option are a single selection from the possible values, or an
* unformatted string, or numeric value governed by the
* MIN/MAX/Regex
constraints. -
List
:
* Values for this option are multiple selections from the possible
* values. -
Boolean
: Values for this option are
* either true
or false
.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see ConfigurationOptionValueType
*/
public ConfigurationOptionDescription withValueType(ConfigurationOptionValueType valueType) {
this.valueType = valueType.toString();
return this;
}
/**
* If specified, values for the configuration option are selected from
* this list.
*
* @return If specified, values for the configuration option are selected from
* this list.
*/
public java.util.List getValueOptions() {
if (valueOptions == null) {
valueOptions = new com.amazonaws.internal.ListWithAutoConstructFlag();
valueOptions.setAutoConstruct(true);
}
return valueOptions;
}
/**
* If specified, values for the configuration option are selected from
* this list.
*
* @param valueOptions If specified, values for the configuration option are selected from
* this list.
*/
public void setValueOptions(java.util.Collection valueOptions) {
if (valueOptions == null) {
this.valueOptions = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag valueOptionsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(valueOptions.size());
valueOptionsCopy.addAll(valueOptions);
this.valueOptions = valueOptionsCopy;
}
/**
* If specified, values for the configuration option are selected from
* this list.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param valueOptions If specified, values for the configuration option are selected from
* this list.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public ConfigurationOptionDescription withValueOptions(String... valueOptions) {
if (getValueOptions() == null) setValueOptions(new java.util.ArrayList(valueOptions.length));
for (String value : valueOptions) {
getValueOptions().add(value);
}
return this;
}
/**
* If specified, values for the configuration option are selected from
* this list.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param valueOptions If specified, values for the configuration option are selected from
* this list.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public ConfigurationOptionDescription withValueOptions(java.util.Collection valueOptions) {
if (valueOptions == null) {
this.valueOptions = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag valueOptionsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(valueOptions.size());
valueOptionsCopy.addAll(valueOptions);
this.valueOptions = valueOptionsCopy;
}
return this;
}
/**
* If specified, the configuration option must be a numeric value greater
* than this value.
*
* @return If specified, the configuration option must be a numeric value greater
* than this value.
*/
public Integer getMinValue() {
return minValue;
}
/**
* If specified, the configuration option must be a numeric value greater
* than this value.
*
* @param minValue If specified, the configuration option must be a numeric value greater
* than this value.
*/
public void setMinValue(Integer minValue) {
this.minValue = minValue;
}
/**
* If specified, the configuration option must be a numeric value greater
* than this value.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param minValue If specified, the configuration option must be a numeric value greater
* than this value.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public ConfigurationOptionDescription withMinValue(Integer minValue) {
this.minValue = minValue;
return this;
}
/**
* If specified, the configuration option must be a numeric value less
* than this value.
*
* @return If specified, the configuration option must be a numeric value less
* than this value.
*/
public Integer getMaxValue() {
return maxValue;
}
/**
* If specified, the configuration option must be a numeric value less
* than this value.
*
* @param maxValue If specified, the configuration option must be a numeric value less
* than this value.
*/
public void setMaxValue(Integer maxValue) {
this.maxValue = maxValue;
}
/**
* If specified, the configuration option must be a numeric value less
* than this value.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param maxValue If specified, the configuration option must be a numeric value less
* than this value.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public ConfigurationOptionDescription withMaxValue(Integer maxValue) {
this.maxValue = maxValue;
return this;
}
/**
* If specified, the configuration option must be a string value no
* longer than this value.
*
* @return If specified, the configuration option must be a string value no
* longer than this value.
*/
public Integer getMaxLength() {
return maxLength;
}
/**
* If specified, the configuration option must be a string value no
* longer than this value.
*
* @param maxLength If specified, the configuration option must be a string value no
* longer than this value.
*/
public void setMaxLength(Integer maxLength) {
this.maxLength = maxLength;
}
/**
* If specified, the configuration option must be a string value no
* longer than this value.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param maxLength If specified, the configuration option must be a string value no
* longer than this value.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public ConfigurationOptionDescription withMaxLength(Integer maxLength) {
this.maxLength = maxLength;
return this;
}
/**
* If specified, the configuration option must be a string value that
* satisfies this regular expression.
*
* @return If specified, the configuration option must be a string value that
* satisfies this regular expression.
*/
public OptionRestrictionRegex getRegex() {
return regex;
}
/**
* If specified, the configuration option must be a string value that
* satisfies this regular expression.
*
* @param regex If specified, the configuration option must be a string value that
* satisfies this regular expression.
*/
public void setRegex(OptionRestrictionRegex regex) {
this.regex = regex;
}
/**
* If specified, the configuration option must be a string value that
* satisfies this regular expression.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param regex If specified, the configuration option must be a string value that
* satisfies this regular expression.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public ConfigurationOptionDescription withRegex(OptionRestrictionRegex regex) {
this.regex = regex;
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getNamespace() != null) sb.append("Namespace: " + getNamespace() + ",");
if (getName() != null) sb.append("Name: " + getName() + ",");
if (getDefaultValue() != null) sb.append("DefaultValue: " + getDefaultValue() + ",");
if (getChangeSeverity() != null) sb.append("ChangeSeverity: " + getChangeSeverity() + ",");
if (isUserDefined() != null) sb.append("UserDefined: " + isUserDefined() + ",");
if (getValueType() != null) sb.append("ValueType: " + getValueType() + ",");
if (getValueOptions() != null) sb.append("ValueOptions: " + getValueOptions() + ",");
if (getMinValue() != null) sb.append("MinValue: " + getMinValue() + ",");
if (getMaxValue() != null) sb.append("MaxValue: " + getMaxValue() + ",");
if (getMaxLength() != null) sb.append("MaxLength: " + getMaxLength() + ",");
if (getRegex() != null) sb.append("Regex: " + getRegex() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getNamespace() == null) ? 0 : getNamespace().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDefaultValue() == null) ? 0 : getDefaultValue().hashCode());
hashCode = prime * hashCode + ((getChangeSeverity() == null) ? 0 : getChangeSeverity().hashCode());
hashCode = prime * hashCode + ((isUserDefined() == null) ? 0 : isUserDefined().hashCode());
hashCode = prime * hashCode + ((getValueType() == null) ? 0 : getValueType().hashCode());
hashCode = prime * hashCode + ((getValueOptions() == null) ? 0 : getValueOptions().hashCode());
hashCode = prime * hashCode + ((getMinValue() == null) ? 0 : getMinValue().hashCode());
hashCode = prime * hashCode + ((getMaxValue() == null) ? 0 : getMaxValue().hashCode());
hashCode = prime * hashCode + ((getMaxLength() == null) ? 0 : getMaxLength().hashCode());
hashCode = prime * hashCode + ((getRegex() == null) ? 0 : getRegex().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof ConfigurationOptionDescription == false) return false;
ConfigurationOptionDescription other = (ConfigurationOptionDescription)obj;
if (other.getNamespace() == null ^ this.getNamespace() == null) return false;
if (other.getNamespace() != null && other.getNamespace().equals(this.getNamespace()) == false) return false;
if (other.getName() == null ^ this.getName() == null) return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false) return false;
if (other.getDefaultValue() == null ^ this.getDefaultValue() == null) return false;
if (other.getDefaultValue() != null && other.getDefaultValue().equals(this.getDefaultValue()) == false) return false;
if (other.getChangeSeverity() == null ^ this.getChangeSeverity() == null) return false;
if (other.getChangeSeverity() != null && other.getChangeSeverity().equals(this.getChangeSeverity()) == false) return false;
if (other.isUserDefined() == null ^ this.isUserDefined() == null) return false;
if (other.isUserDefined() != null && other.isUserDefined().equals(this.isUserDefined()) == false) return false;
if (other.getValueType() == null ^ this.getValueType() == null) return false;
if (other.getValueType() != null && other.getValueType().equals(this.getValueType()) == false) return false;
if (other.getValueOptions() == null ^ this.getValueOptions() == null) return false;
if (other.getValueOptions() != null && other.getValueOptions().equals(this.getValueOptions()) == false) return false;
if (other.getMinValue() == null ^ this.getMinValue() == null) return false;
if (other.getMinValue() != null && other.getMinValue().equals(this.getMinValue()) == false) return false;
if (other.getMaxValue() == null ^ this.getMaxValue() == null) return false;
if (other.getMaxValue() != null && other.getMaxValue().equals(this.getMaxValue()) == false) return false;
if (other.getMaxLength() == null ^ this.getMaxLength() == null) return false;
if (other.getMaxLength() != null && other.getMaxLength().equals(this.getMaxLength()) == false) return false;
if (other.getRegex() == null ^ this.getRegex() == null) return false;
if (other.getRegex() != null && other.getRegex().equals(this.getRegex()) == false) return false;
return true;
}
}