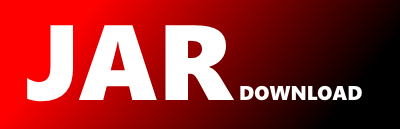
com.amazonaws.services.elasticbeanstalk.model.EnvironmentResourceDescription Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.elasticbeanstalk.model;
import java.io.Serializable;
/**
*
* Describes the AWS resources in use by this environment. This data is
* live.
*
*/
public class EnvironmentResourceDescription implements Serializable {
/**
* The name of the environment.
*
* Constraints:
* Length: 4 - 23
*/
private String environmentName;
/**
* The AutoScalingGroups
used by this environment.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag autoScalingGroups;
/**
* The Amazon EC2 instances used by this environment.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag instances;
/**
* The Auto Scaling launch configurations in use by this environment.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag launchConfigurations;
/**
* The LoadBalancers in use by this environment.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag loadBalancers;
/**
* The AutoScaling
triggers in use by this environment.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag triggers;
/**
* The queues used by this environment.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag queues;
/**
* Default constructor for a new EnvironmentResourceDescription object. Callers should use the
* setter or fluent setter (with...) methods to initialize this object after creating it.
*/
public EnvironmentResourceDescription() {}
/**
* The name of the environment.
*
* Constraints:
* Length: 4 - 23
*
* @return The name of the environment.
*/
public String getEnvironmentName() {
return environmentName;
}
/**
* The name of the environment.
*
* Constraints:
* Length: 4 - 23
*
* @param environmentName The name of the environment.
*/
public void setEnvironmentName(String environmentName) {
this.environmentName = environmentName;
}
/**
* The name of the environment.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 4 - 23
*
* @param environmentName The name of the environment.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public EnvironmentResourceDescription withEnvironmentName(String environmentName) {
this.environmentName = environmentName;
return this;
}
/**
* The AutoScalingGroups
used by this environment.
*
* @return The AutoScalingGroups
used by this environment.
*/
public java.util.List getAutoScalingGroups() {
if (autoScalingGroups == null) {
autoScalingGroups = new com.amazonaws.internal.ListWithAutoConstructFlag();
autoScalingGroups.setAutoConstruct(true);
}
return autoScalingGroups;
}
/**
* The AutoScalingGroups
used by this environment.
*
* @param autoScalingGroups The AutoScalingGroups
used by this environment.
*/
public void setAutoScalingGroups(java.util.Collection autoScalingGroups) {
if (autoScalingGroups == null) {
this.autoScalingGroups = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag autoScalingGroupsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(autoScalingGroups.size());
autoScalingGroupsCopy.addAll(autoScalingGroups);
this.autoScalingGroups = autoScalingGroupsCopy;
}
/**
* The AutoScalingGroups
used by this environment.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param autoScalingGroups The AutoScalingGroups
used by this environment.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public EnvironmentResourceDescription withAutoScalingGroups(AutoScalingGroup... autoScalingGroups) {
if (getAutoScalingGroups() == null) setAutoScalingGroups(new java.util.ArrayList(autoScalingGroups.length));
for (AutoScalingGroup value : autoScalingGroups) {
getAutoScalingGroups().add(value);
}
return this;
}
/**
* The AutoScalingGroups
used by this environment.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param autoScalingGroups The AutoScalingGroups
used by this environment.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public EnvironmentResourceDescription withAutoScalingGroups(java.util.Collection autoScalingGroups) {
if (autoScalingGroups == null) {
this.autoScalingGroups = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag autoScalingGroupsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(autoScalingGroups.size());
autoScalingGroupsCopy.addAll(autoScalingGroups);
this.autoScalingGroups = autoScalingGroupsCopy;
}
return this;
}
/**
* The Amazon EC2 instances used by this environment.
*
* @return The Amazon EC2 instances used by this environment.
*/
public java.util.List getInstances() {
if (instances == null) {
instances = new com.amazonaws.internal.ListWithAutoConstructFlag();
instances.setAutoConstruct(true);
}
return instances;
}
/**
* The Amazon EC2 instances used by this environment.
*
* @param instances The Amazon EC2 instances used by this environment.
*/
public void setInstances(java.util.Collection instances) {
if (instances == null) {
this.instances = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag instancesCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(instances.size());
instancesCopy.addAll(instances);
this.instances = instancesCopy;
}
/**
* The Amazon EC2 instances used by this environment.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param instances The Amazon EC2 instances used by this environment.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public EnvironmentResourceDescription withInstances(Instance... instances) {
if (getInstances() == null) setInstances(new java.util.ArrayList(instances.length));
for (Instance value : instances) {
getInstances().add(value);
}
return this;
}
/**
* The Amazon EC2 instances used by this environment.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param instances The Amazon EC2 instances used by this environment.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public EnvironmentResourceDescription withInstances(java.util.Collection instances) {
if (instances == null) {
this.instances = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag instancesCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(instances.size());
instancesCopy.addAll(instances);
this.instances = instancesCopy;
}
return this;
}
/**
* The Auto Scaling launch configurations in use by this environment.
*
* @return The Auto Scaling launch configurations in use by this environment.
*/
public java.util.List getLaunchConfigurations() {
if (launchConfigurations == null) {
launchConfigurations = new com.amazonaws.internal.ListWithAutoConstructFlag();
launchConfigurations.setAutoConstruct(true);
}
return launchConfigurations;
}
/**
* The Auto Scaling launch configurations in use by this environment.
*
* @param launchConfigurations The Auto Scaling launch configurations in use by this environment.
*/
public void setLaunchConfigurations(java.util.Collection launchConfigurations) {
if (launchConfigurations == null) {
this.launchConfigurations = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag launchConfigurationsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(launchConfigurations.size());
launchConfigurationsCopy.addAll(launchConfigurations);
this.launchConfigurations = launchConfigurationsCopy;
}
/**
* The Auto Scaling launch configurations in use by this environment.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param launchConfigurations The Auto Scaling launch configurations in use by this environment.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public EnvironmentResourceDescription withLaunchConfigurations(LaunchConfiguration... launchConfigurations) {
if (getLaunchConfigurations() == null) setLaunchConfigurations(new java.util.ArrayList(launchConfigurations.length));
for (LaunchConfiguration value : launchConfigurations) {
getLaunchConfigurations().add(value);
}
return this;
}
/**
* The Auto Scaling launch configurations in use by this environment.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param launchConfigurations The Auto Scaling launch configurations in use by this environment.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public EnvironmentResourceDescription withLaunchConfigurations(java.util.Collection launchConfigurations) {
if (launchConfigurations == null) {
this.launchConfigurations = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag launchConfigurationsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(launchConfigurations.size());
launchConfigurationsCopy.addAll(launchConfigurations);
this.launchConfigurations = launchConfigurationsCopy;
}
return this;
}
/**
* The LoadBalancers in use by this environment.
*
* @return The LoadBalancers in use by this environment.
*/
public java.util.List getLoadBalancers() {
if (loadBalancers == null) {
loadBalancers = new com.amazonaws.internal.ListWithAutoConstructFlag();
loadBalancers.setAutoConstruct(true);
}
return loadBalancers;
}
/**
* The LoadBalancers in use by this environment.
*
* @param loadBalancers The LoadBalancers in use by this environment.
*/
public void setLoadBalancers(java.util.Collection loadBalancers) {
if (loadBalancers == null) {
this.loadBalancers = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag loadBalancersCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(loadBalancers.size());
loadBalancersCopy.addAll(loadBalancers);
this.loadBalancers = loadBalancersCopy;
}
/**
* The LoadBalancers in use by this environment.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param loadBalancers The LoadBalancers in use by this environment.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public EnvironmentResourceDescription withLoadBalancers(LoadBalancer... loadBalancers) {
if (getLoadBalancers() == null) setLoadBalancers(new java.util.ArrayList(loadBalancers.length));
for (LoadBalancer value : loadBalancers) {
getLoadBalancers().add(value);
}
return this;
}
/**
* The LoadBalancers in use by this environment.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param loadBalancers The LoadBalancers in use by this environment.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public EnvironmentResourceDescription withLoadBalancers(java.util.Collection loadBalancers) {
if (loadBalancers == null) {
this.loadBalancers = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag loadBalancersCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(loadBalancers.size());
loadBalancersCopy.addAll(loadBalancers);
this.loadBalancers = loadBalancersCopy;
}
return this;
}
/**
* The AutoScaling
triggers in use by this environment.
*
* @return The AutoScaling
triggers in use by this environment.
*/
public java.util.List getTriggers() {
if (triggers == null) {
triggers = new com.amazonaws.internal.ListWithAutoConstructFlag();
triggers.setAutoConstruct(true);
}
return triggers;
}
/**
* The AutoScaling
triggers in use by this environment.
*
* @param triggers The AutoScaling
triggers in use by this environment.
*/
public void setTriggers(java.util.Collection triggers) {
if (triggers == null) {
this.triggers = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag triggersCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(triggers.size());
triggersCopy.addAll(triggers);
this.triggers = triggersCopy;
}
/**
* The AutoScaling
triggers in use by this environment.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param triggers The AutoScaling
triggers in use by this environment.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public EnvironmentResourceDescription withTriggers(Trigger... triggers) {
if (getTriggers() == null) setTriggers(new java.util.ArrayList(triggers.length));
for (Trigger value : triggers) {
getTriggers().add(value);
}
return this;
}
/**
* The AutoScaling
triggers in use by this environment.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param triggers The AutoScaling
triggers in use by this environment.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public EnvironmentResourceDescription withTriggers(java.util.Collection triggers) {
if (triggers == null) {
this.triggers = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag triggersCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(triggers.size());
triggersCopy.addAll(triggers);
this.triggers = triggersCopy;
}
return this;
}
/**
* The queues used by this environment.
*
* @return The queues used by this environment.
*/
public java.util.List getQueues() {
if (queues == null) {
queues = new com.amazonaws.internal.ListWithAutoConstructFlag();
queues.setAutoConstruct(true);
}
return queues;
}
/**
* The queues used by this environment.
*
* @param queues The queues used by this environment.
*/
public void setQueues(java.util.Collection queues) {
if (queues == null) {
this.queues = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag queuesCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(queues.size());
queuesCopy.addAll(queues);
this.queues = queuesCopy;
}
/**
* The queues used by this environment.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param queues The queues used by this environment.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public EnvironmentResourceDescription withQueues(Queue... queues) {
if (getQueues() == null) setQueues(new java.util.ArrayList(queues.length));
for (Queue value : queues) {
getQueues().add(value);
}
return this;
}
/**
* The queues used by this environment.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param queues The queues used by this environment.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public EnvironmentResourceDescription withQueues(java.util.Collection queues) {
if (queues == null) {
this.queues = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag queuesCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(queues.size());
queuesCopy.addAll(queues);
this.queues = queuesCopy;
}
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getEnvironmentName() != null) sb.append("EnvironmentName: " + getEnvironmentName() + ",");
if (getAutoScalingGroups() != null) sb.append("AutoScalingGroups: " + getAutoScalingGroups() + ",");
if (getInstances() != null) sb.append("Instances: " + getInstances() + ",");
if (getLaunchConfigurations() != null) sb.append("LaunchConfigurations: " + getLaunchConfigurations() + ",");
if (getLoadBalancers() != null) sb.append("LoadBalancers: " + getLoadBalancers() + ",");
if (getTriggers() != null) sb.append("Triggers: " + getTriggers() + ",");
if (getQueues() != null) sb.append("Queues: " + getQueues() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getEnvironmentName() == null) ? 0 : getEnvironmentName().hashCode());
hashCode = prime * hashCode + ((getAutoScalingGroups() == null) ? 0 : getAutoScalingGroups().hashCode());
hashCode = prime * hashCode + ((getInstances() == null) ? 0 : getInstances().hashCode());
hashCode = prime * hashCode + ((getLaunchConfigurations() == null) ? 0 : getLaunchConfigurations().hashCode());
hashCode = prime * hashCode + ((getLoadBalancers() == null) ? 0 : getLoadBalancers().hashCode());
hashCode = prime * hashCode + ((getTriggers() == null) ? 0 : getTriggers().hashCode());
hashCode = prime * hashCode + ((getQueues() == null) ? 0 : getQueues().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof EnvironmentResourceDescription == false) return false;
EnvironmentResourceDescription other = (EnvironmentResourceDescription)obj;
if (other.getEnvironmentName() == null ^ this.getEnvironmentName() == null) return false;
if (other.getEnvironmentName() != null && other.getEnvironmentName().equals(this.getEnvironmentName()) == false) return false;
if (other.getAutoScalingGroups() == null ^ this.getAutoScalingGroups() == null) return false;
if (other.getAutoScalingGroups() != null && other.getAutoScalingGroups().equals(this.getAutoScalingGroups()) == false) return false;
if (other.getInstances() == null ^ this.getInstances() == null) return false;
if (other.getInstances() != null && other.getInstances().equals(this.getInstances()) == false) return false;
if (other.getLaunchConfigurations() == null ^ this.getLaunchConfigurations() == null) return false;
if (other.getLaunchConfigurations() != null && other.getLaunchConfigurations().equals(this.getLaunchConfigurations()) == false) return false;
if (other.getLoadBalancers() == null ^ this.getLoadBalancers() == null) return false;
if (other.getLoadBalancers() != null && other.getLoadBalancers().equals(this.getLoadBalancers()) == false) return false;
if (other.getTriggers() == null ^ this.getTriggers() == null) return false;
if (other.getTriggers() != null && other.getTriggers().equals(this.getTriggers()) == false) return false;
if (other.getQueues() == null ^ this.getQueues() == null) return false;
if (other.getQueues() != null && other.getQueues().equals(this.getQueues()) == false) return false;
return true;
}
}