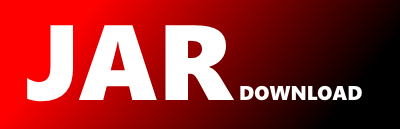
com.amazonaws.services.elasticmapreduce.AmazonElasticMapReduceAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.elasticmapreduce;
import java.util.concurrent.Future;
import com.amazonaws.AmazonClientException;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.handlers.AsyncHandler;
import com.amazonaws.services.elasticmapreduce.model.*;
/**
* Interface for accessing AmazonElasticMapReduce asynchronously.
* Each asynchronous method will return a Java Future object, and users are also allowed
* to provide a callback handler.
*
* This is the Amazon Elastic MapReduce API Reference . This
* guide provides descriptions and samples of the Amazon Elastic
* MapReduce APIs.
*
*
* Amazon Elastic MapReduce (Amazon EMR) is a web service that makes it
* easy to process large amounts of data efficiently. Amazon EMR uses
* Hadoop processing combined with several AWS products to do tasks such
* as web indexing, data mining, log file analysis, machine learning,
* scientific simulation, and data warehousing.
*
*/
public interface AmazonElasticMapReduceAsync extends AmazonElasticMapReduce {
/**
*
* Provides information about the bootstrap actions associated with a
* cluster.
*
*
* @param listBootstrapActionsRequest Container for the necessary
* parameters to execute the ListBootstrapActions operation on
* AmazonElasticMapReduce.
*
* @return A Java Future object containing the response from the
* ListBootstrapActions service method, as returned by
* AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listBootstrapActionsAsync(ListBootstrapActionsRequest listBootstrapActionsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Provides information about the bootstrap actions associated with a
* cluster.
*
*
* @param listBootstrapActionsRequest Container for the necessary
* parameters to execute the ListBootstrapActions operation on
* AmazonElasticMapReduce.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ListBootstrapActions service method, as returned by
* AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listBootstrapActionsAsync(ListBootstrapActionsRequest listBootstrapActionsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Adds tags to an Amazon EMR resource. Tags make it easier to associate
* clusters in various ways, such as grouping clusters to track your
* Amazon EMR resource allocation costs. For more information, see
* Tagging Amazon EMR Resources
* .
*
*
* @param addTagsRequest Container for the necessary parameters to
* execute the AddTags operation on AmazonElasticMapReduce.
*
* @return A Java Future object containing the response from the AddTags
* service method, as returned by AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future addTagsAsync(AddTagsRequest addTagsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Adds tags to an Amazon EMR resource. Tags make it easier to associate
* clusters in various ways, such as grouping clusters to track your
* Amazon EMR resource allocation costs. For more information, see
* Tagging Amazon EMR Resources
* .
*
*
* @param addTagsRequest Container for the necessary parameters to
* execute the AddTags operation on AmazonElasticMapReduce.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the AddTags
* service method, as returned by AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future addTagsAsync(AddTagsRequest addTagsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Sets whether all AWS Identity and Access Management (IAM) users under
* your account can access the specified job flows. This action works on
* running job flows. You can also set the visibility of a job flow when
* you launch it using the VisibleToAllUsers
parameter of
* RunJobFlow. The SetVisibleToAllUsers action can be called only by an
* IAM user who created the job flow or the AWS account that owns the job
* flow.
*
*
* @param setVisibleToAllUsersRequest Container for the necessary
* parameters to execute the SetVisibleToAllUsers operation on
* AmazonElasticMapReduce.
*
* @return A Java Future object containing the response from the
* SetVisibleToAllUsers service method, as returned by
* AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future setVisibleToAllUsersAsync(SetVisibleToAllUsersRequest setVisibleToAllUsersRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Sets whether all AWS Identity and Access Management (IAM) users under
* your account can access the specified job flows. This action works on
* running job flows. You can also set the visibility of a job flow when
* you launch it using the VisibleToAllUsers
parameter of
* RunJobFlow. The SetVisibleToAllUsers action can be called only by an
* IAM user who created the job flow or the AWS account that owns the job
* flow.
*
*
* @param setVisibleToAllUsersRequest Container for the necessary
* parameters to execute the SetVisibleToAllUsers operation on
* AmazonElasticMapReduce.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* SetVisibleToAllUsers service method, as returned by
* AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future setVisibleToAllUsersAsync(SetVisibleToAllUsersRequest setVisibleToAllUsersRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Provides a list of steps for the cluster.
*
*
* @param listStepsRequest Container for the necessary parameters to
* execute the ListSteps operation on AmazonElasticMapReduce.
*
* @return A Java Future object containing the response from the
* ListSteps service method, as returned by AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listStepsAsync(ListStepsRequest listStepsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Provides a list of steps for the cluster.
*
*
* @param listStepsRequest Container for the necessary parameters to
* execute the ListSteps operation on AmazonElasticMapReduce.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ListSteps service method, as returned by AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listStepsAsync(ListStepsRequest listStepsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* AddJobFlowSteps adds new steps to a running job flow. A maximum of
* 256 steps are allowed in each job flow.
*
*
* If your job flow is long-running (such as a Hive data warehouse) or
* complex, you may require more than 256 steps to process your data. You
* can bypass the 256-step limitation in various ways, including using
* the SSH shell to connect to the master node and submitting queries
* directly to the software running on the master node, such as Hive and
* Hadoop. For more information on how to do this, go to
* Add More than 256 Steps to a Job Flow
* in the Amazon Elastic MapReduce Developer's Guide .
*
*
* A step specifies the location of a JAR file stored either on the
* master node of the job flow or in Amazon S3. Each step is performed by
* the main function of the main class of the JAR file. The main class
* can be specified either in the manifest of the JAR or by using the
* MainFunction parameter of the step.
*
*
* Elastic MapReduce executes each step in the order listed. For a step
* to be considered complete, the main function must exit with a zero
* exit code and all Hadoop jobs started while the step was running must
* have completed and run successfully.
*
*
* You can only add steps to a job flow that is in one of the following
* states: STARTING, BOOTSTRAPPING, RUNNING, or WAITING.
*
*
* @param addJobFlowStepsRequest Container for the necessary parameters
* to execute the AddJobFlowSteps operation on AmazonElasticMapReduce.
*
* @return A Java Future object containing the response from the
* AddJobFlowSteps service method, as returned by AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future addJobFlowStepsAsync(AddJobFlowStepsRequest addJobFlowStepsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* AddJobFlowSteps adds new steps to a running job flow. A maximum of
* 256 steps are allowed in each job flow.
*
*
* If your job flow is long-running (such as a Hive data warehouse) or
* complex, you may require more than 256 steps to process your data. You
* can bypass the 256-step limitation in various ways, including using
* the SSH shell to connect to the master node and submitting queries
* directly to the software running on the master node, such as Hive and
* Hadoop. For more information on how to do this, go to
* Add More than 256 Steps to a Job Flow
* in the Amazon Elastic MapReduce Developer's Guide .
*
*
* A step specifies the location of a JAR file stored either on the
* master node of the job flow or in Amazon S3. Each step is performed by
* the main function of the main class of the JAR file. The main class
* can be specified either in the manifest of the JAR or by using the
* MainFunction parameter of the step.
*
*
* Elastic MapReduce executes each step in the order listed. For a step
* to be considered complete, the main function must exit with a zero
* exit code and all Hadoop jobs started while the step was running must
* have completed and run successfully.
*
*
* You can only add steps to a job flow that is in one of the following
* states: STARTING, BOOTSTRAPPING, RUNNING, or WAITING.
*
*
* @param addJobFlowStepsRequest Container for the necessary parameters
* to execute the AddJobFlowSteps operation on AmazonElasticMapReduce.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* AddJobFlowSteps service method, as returned by AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future addJobFlowStepsAsync(AddJobFlowStepsRequest addJobFlowStepsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Provides more detail about the cluster step.
*
*
* @param describeStepRequest Container for the necessary parameters to
* execute the DescribeStep operation on AmazonElasticMapReduce.
*
* @return A Java Future object containing the response from the
* DescribeStep service method, as returned by AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeStepAsync(DescribeStepRequest describeStepRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Provides more detail about the cluster step.
*
*
* @param describeStepRequest Container for the necessary parameters to
* execute the DescribeStep operation on AmazonElasticMapReduce.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeStep service method, as returned by AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeStepAsync(DescribeStepRequest describeStepRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Provides the status of all clusters visible to this AWS account.
* Allows you to filter the list of clusters based on certain criteria;
* for example, filtering by cluster creation date and time or by status.
* This call returns a maximum of 50 clusters per call, but returns a
* marker to track the paging of the cluster list across multiple
* ListClusters calls.
*
*
* @param listClustersRequest Container for the necessary parameters to
* execute the ListClusters operation on AmazonElasticMapReduce.
*
* @return A Java Future object containing the response from the
* ListClusters service method, as returned by AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listClustersAsync(ListClustersRequest listClustersRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Provides the status of all clusters visible to this AWS account.
* Allows you to filter the list of clusters based on certain criteria;
* for example, filtering by cluster creation date and time or by status.
* This call returns a maximum of 50 clusters per call, but returns a
* marker to track the paging of the cluster list across multiple
* ListClusters calls.
*
*
* @param listClustersRequest Container for the necessary parameters to
* execute the ListClusters operation on AmazonElasticMapReduce.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ListClusters service method, as returned by AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listClustersAsync(ListClustersRequest listClustersRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Removes tags from an Amazon EMR resource. Tags make it easier to
* associate clusters in various ways, such as grouping clusters to track
* your Amazon EMR resource allocation costs. For more information, see
* Tagging Amazon EMR Resources
* .
*
*
* @param removeTagsRequest Container for the necessary parameters to
* execute the RemoveTags operation on AmazonElasticMapReduce.
*
* @return A Java Future object containing the response from the
* RemoveTags service method, as returned by AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future removeTagsAsync(RemoveTagsRequest removeTagsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Removes tags from an Amazon EMR resource. Tags make it easier to
* associate clusters in various ways, such as grouping clusters to track
* your Amazon EMR resource allocation costs. For more information, see
* Tagging Amazon EMR Resources
* .
*
*
* @param removeTagsRequest Container for the necessary parameters to
* execute the RemoveTags operation on AmazonElasticMapReduce.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* RemoveTags service method, as returned by AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future removeTagsAsync(RemoveTagsRequest removeTagsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Provides all available details about the instance groups in a cluster.
*
*
* @param listInstanceGroupsRequest Container for the necessary
* parameters to execute the ListInstanceGroups operation on
* AmazonElasticMapReduce.
*
* @return A Java Future object containing the response from the
* ListInstanceGroups service method, as returned by
* AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listInstanceGroupsAsync(ListInstanceGroupsRequest listInstanceGroupsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Provides all available details about the instance groups in a cluster.
*
*
* @param listInstanceGroupsRequest Container for the necessary
* parameters to execute the ListInstanceGroups operation on
* AmazonElasticMapReduce.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ListInstanceGroups service method, as returned by
* AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listInstanceGroupsAsync(ListInstanceGroupsRequest listInstanceGroupsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* ModifyInstanceGroups modifies the number of nodes and configuration
* settings of an instance group. The input parameters include the new
* target instance count for the group and the instance group ID. The
* call will either succeed or fail atomically.
*
*
* @param modifyInstanceGroupsRequest Container for the necessary
* parameters to execute the ModifyInstanceGroups operation on
* AmazonElasticMapReduce.
*
* @return A Java Future object containing the response from the
* ModifyInstanceGroups service method, as returned by
* AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future modifyInstanceGroupsAsync(ModifyInstanceGroupsRequest modifyInstanceGroupsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* ModifyInstanceGroups modifies the number of nodes and configuration
* settings of an instance group. The input parameters include the new
* target instance count for the group and the instance group ID. The
* call will either succeed or fail atomically.
*
*
* @param modifyInstanceGroupsRequest Container for the necessary
* parameters to execute the ModifyInstanceGroups operation on
* AmazonElasticMapReduce.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ModifyInstanceGroups service method, as returned by
* AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future modifyInstanceGroupsAsync(ModifyInstanceGroupsRequest modifyInstanceGroupsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Provides information about the cluster instances that Amazon EMR
* provisions on behalf of a user when it creates the cluster. For
* example, this operation indicates when the EC2 instances reach the
* Ready state, when instances become available to Amazon EMR to use for
* jobs, and the IP addresses for cluster instances, etc.
*
*
* @param listInstancesRequest Container for the necessary parameters to
* execute the ListInstances operation on AmazonElasticMapReduce.
*
* @return A Java Future object containing the response from the
* ListInstances service method, as returned by AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listInstancesAsync(ListInstancesRequest listInstancesRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Provides information about the cluster instances that Amazon EMR
* provisions on behalf of a user when it creates the cluster. For
* example, this operation indicates when the EC2 instances reach the
* Ready state, when instances become available to Amazon EMR to use for
* jobs, and the IP addresses for cluster instances, etc.
*
*
* @param listInstancesRequest Container for the necessary parameters to
* execute the ListInstances operation on AmazonElasticMapReduce.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ListInstances service method, as returned by AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listInstancesAsync(ListInstancesRequest listInstancesRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* AddInstanceGroups adds an instance group to a running cluster.
*
*
* @param addInstanceGroupsRequest Container for the necessary parameters
* to execute the AddInstanceGroups operation on AmazonElasticMapReduce.
*
* @return A Java Future object containing the response from the
* AddInstanceGroups service method, as returned by
* AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future addInstanceGroupsAsync(AddInstanceGroupsRequest addInstanceGroupsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* AddInstanceGroups adds an instance group to a running cluster.
*
*
* @param addInstanceGroupsRequest Container for the necessary parameters
* to execute the AddInstanceGroups operation on AmazonElasticMapReduce.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* AddInstanceGroups service method, as returned by
* AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future addInstanceGroupsAsync(AddInstanceGroupsRequest addInstanceGroupsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* TerminateJobFlows shuts a list of job flows down. When a job flow is
* shut down, any step not yet completed is canceled and the EC2
* instances on which the job flow is running are stopped. Any log files
* not already saved are uploaded to Amazon S3 if a LogUri was specified
* when the job flow was created.
*
*
* The call to TerminateJobFlows is asynchronous. Depending on the
* configuration of the job flow, it may take up to 5-20 minutes for the
* job flow to completely terminate and release allocated resources, such
* as Amazon EC2 instances.
*
*
* @param terminateJobFlowsRequest Container for the necessary parameters
* to execute the TerminateJobFlows operation on AmazonElasticMapReduce.
*
* @return A Java Future object containing the response from the
* TerminateJobFlows service method, as returned by
* AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future terminateJobFlowsAsync(TerminateJobFlowsRequest terminateJobFlowsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* TerminateJobFlows shuts a list of job flows down. When a job flow is
* shut down, any step not yet completed is canceled and the EC2
* instances on which the job flow is running are stopped. Any log files
* not already saved are uploaded to Amazon S3 if a LogUri was specified
* when the job flow was created.
*
*
* The call to TerminateJobFlows is asynchronous. Depending on the
* configuration of the job flow, it may take up to 5-20 minutes for the
* job flow to completely terminate and release allocated resources, such
* as Amazon EC2 instances.
*
*
* @param terminateJobFlowsRequest Container for the necessary parameters
* to execute the TerminateJobFlows operation on AmazonElasticMapReduce.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* TerminateJobFlows service method, as returned by
* AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future terminateJobFlowsAsync(TerminateJobFlowsRequest terminateJobFlowsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* SetTerminationProtection locks a job flow so the Amazon EC2 instances
* in the cluster cannot be terminated by user intervention, an API call,
* or in the event of a job-flow error. The cluster still terminates upon
* successful completion of the job flow. Calling
* SetTerminationProtection on a job flow is analogous to calling the
* Amazon EC2 DisableAPITermination API on all of the EC2 instances in a
* cluster.
*
*
* SetTerminationProtection is used to prevent accidental termination of
* a job flow and to ensure that in the event of an error, the instances
* will persist so you can recover any data stored in their ephemeral
* instance storage.
*
*
* To terminate a job flow that has been locked by setting
* SetTerminationProtection to true
,
* you must first unlock the job flow by a subsequent call to
* SetTerminationProtection in which you set the value to
* false
.
*
*
* For more information, go to
* Protecting a Job Flow from Termination
* in the Amazon Elastic MapReduce Developer's Guide.
*
*
* @param setTerminationProtectionRequest Container for the necessary
* parameters to execute the SetTerminationProtection operation on
* AmazonElasticMapReduce.
*
* @return A Java Future object containing the response from the
* SetTerminationProtection service method, as returned by
* AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future setTerminationProtectionAsync(SetTerminationProtectionRequest setTerminationProtectionRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* SetTerminationProtection locks a job flow so the Amazon EC2 instances
* in the cluster cannot be terminated by user intervention, an API call,
* or in the event of a job-flow error. The cluster still terminates upon
* successful completion of the job flow. Calling
* SetTerminationProtection on a job flow is analogous to calling the
* Amazon EC2 DisableAPITermination API on all of the EC2 instances in a
* cluster.
*
*
* SetTerminationProtection is used to prevent accidental termination of
* a job flow and to ensure that in the event of an error, the instances
* will persist so you can recover any data stored in their ephemeral
* instance storage.
*
*
* To terminate a job flow that has been locked by setting
* SetTerminationProtection to true
,
* you must first unlock the job flow by a subsequent call to
* SetTerminationProtection in which you set the value to
* false
.
*
*
* For more information, go to
* Protecting a Job Flow from Termination
* in the Amazon Elastic MapReduce Developer's Guide.
*
*
* @param setTerminationProtectionRequest Container for the necessary
* parameters to execute the SetTerminationProtection operation on
* AmazonElasticMapReduce.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* SetTerminationProtection service method, as returned by
* AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future setTerminationProtectionAsync(SetTerminationProtectionRequest setTerminationProtectionRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* DescribeJobFlows returns a list of job flows that match all of the
* supplied parameters. The parameters can include a list of job flow
* IDs, job flow states, and restrictions on job flow creation date and
* time.
*
*
* Regardless of supplied parameters, only job flows created within the
* last two months are returned.
*
*
* If no parameters are supplied, then job flows matching either of the
* following criteria are returned:
*
*
*
* - Job flows created and completed in the last two weeks
* - Job flows created within the last two months that are in one of
* the following states:
RUNNING
,
* WAITING
,
* SHUTTING_DOWN
,
*
* STARTING
*
*
*
* Amazon Elastic MapReduce can return a maximum of 512 job flow
* descriptions.
*
*
* @param describeJobFlowsRequest Container for the necessary parameters
* to execute the DescribeJobFlows operation on AmazonElasticMapReduce.
*
* @return A Java Future object containing the response from the
* DescribeJobFlows service method, as returned by
* AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
@Deprecated
public Future describeJobFlowsAsync(DescribeJobFlowsRequest describeJobFlowsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* DescribeJobFlows returns a list of job flows that match all of the
* supplied parameters. The parameters can include a list of job flow
* IDs, job flow states, and restrictions on job flow creation date and
* time.
*
*
* Regardless of supplied parameters, only job flows created within the
* last two months are returned.
*
*
* If no parameters are supplied, then job flows matching either of the
* following criteria are returned:
*
*
*
* - Job flows created and completed in the last two weeks
* - Job flows created within the last two months that are in one of
* the following states:
RUNNING
,
* WAITING
,
* SHUTTING_DOWN
,
*
* STARTING
*
*
*
* Amazon Elastic MapReduce can return a maximum of 512 job flow
* descriptions.
*
*
* @param describeJobFlowsRequest Container for the necessary parameters
* to execute the DescribeJobFlows operation on AmazonElasticMapReduce.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeJobFlows service method, as returned by
* AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeJobFlowsAsync(DescribeJobFlowsRequest describeJobFlowsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* RunJobFlow creates and starts running a new job flow. The job flow
* will run the steps specified. Once the job flow completes, the cluster
* is stopped and the HDFS partition is lost. To prevent loss of data,
* configure the last step of the job flow to store results in Amazon S3.
* If the JobFlowInstancesConfig KeepJobFlowAliveWhenNoSteps
* parameter is set to TRUE
, the job flow will transition
* to the WAITING state rather than shutting down once the steps have
* completed.
*
*
* For additional protection, you can set the JobFlowInstancesConfig
* TerminationProtected
parameter to TRUE
to
* lock the job flow and prevent it from being terminated by API call,
* user intervention, or in the event of a job flow error.
*
*
* A maximum of 256 steps are allowed in each job flow.
*
*
* If your job flow is long-running (such as a Hive data warehouse) or
* complex, you may require more than 256 steps to process your data. You
* can bypass the 256-step limitation in various ways, including using
* the SSH shell to connect to the master node and submitting queries
* directly to the software running on the master node, such as Hive and
* Hadoop. For more information on how to do this, go to
* Add More than 256 Steps to a Job Flow
* in the Amazon Elastic MapReduce Developer's Guide .
*
*
* For long running job flows, we recommend that you periodically store
* your results.
*
*
* @param runJobFlowRequest Container for the necessary parameters to
* execute the RunJobFlow operation on AmazonElasticMapReduce.
*
* @return A Java Future object containing the response from the
* RunJobFlow service method, as returned by AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future runJobFlowAsync(RunJobFlowRequest runJobFlowRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* RunJobFlow creates and starts running a new job flow. The job flow
* will run the steps specified. Once the job flow completes, the cluster
* is stopped and the HDFS partition is lost. To prevent loss of data,
* configure the last step of the job flow to store results in Amazon S3.
* If the JobFlowInstancesConfig KeepJobFlowAliveWhenNoSteps
* parameter is set to TRUE
, the job flow will transition
* to the WAITING state rather than shutting down once the steps have
* completed.
*
*
* For additional protection, you can set the JobFlowInstancesConfig
* TerminationProtected
parameter to TRUE
to
* lock the job flow and prevent it from being terminated by API call,
* user intervention, or in the event of a job flow error.
*
*
* A maximum of 256 steps are allowed in each job flow.
*
*
* If your job flow is long-running (such as a Hive data warehouse) or
* complex, you may require more than 256 steps to process your data. You
* can bypass the 256-step limitation in various ways, including using
* the SSH shell to connect to the master node and submitting queries
* directly to the software running on the master node, such as Hive and
* Hadoop. For more information on how to do this, go to
* Add More than 256 Steps to a Job Flow
* in the Amazon Elastic MapReduce Developer's Guide .
*
*
* For long running job flows, we recommend that you periodically store
* your results.
*
*
* @param runJobFlowRequest Container for the necessary parameters to
* execute the RunJobFlow operation on AmazonElasticMapReduce.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* RunJobFlow service method, as returned by AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future runJobFlowAsync(RunJobFlowRequest runJobFlowRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Provides cluster-level details including status, hardware and software
* configuration, VPC settings, and so on. For information about the
* cluster steps, see ListSteps.
*
*
* @param describeClusterRequest Container for the necessary parameters
* to execute the DescribeCluster operation on AmazonElasticMapReduce.
*
* @return A Java Future object containing the response from the
* DescribeCluster service method, as returned by AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeClusterAsync(DescribeClusterRequest describeClusterRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Provides cluster-level details including status, hardware and software
* configuration, VPC settings, and so on. For information about the
* cluster steps, see ListSteps.
*
*
* @param describeClusterRequest Container for the necessary parameters
* to execute the DescribeCluster operation on AmazonElasticMapReduce.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeCluster service method, as returned by AmazonElasticMapReduce.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeClusterAsync(DescribeClusterRequest describeClusterRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
}