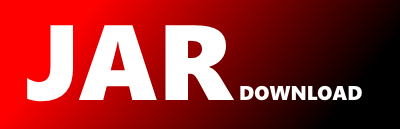
com.amazonaws.services.elasticmapreduce.AmazonElasticMapReduceClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.elasticmapreduce;
import java.net.*;
import java.util.*;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.regions.*;
import com.amazonaws.internal.*;
import com.amazonaws.metrics.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.util.json.*;
import com.amazonaws.services.elasticmapreduce.model.*;
import com.amazonaws.services.elasticmapreduce.model.transform.*;
/**
* Client for accessing AmazonElasticMapReduce. All service calls made
* using this client are blocking, and will not return until the service call
* completes.
*
*
* This is the Amazon Elastic MapReduce API Reference . This
* guide provides descriptions and samples of the Amazon Elastic
* MapReduce APIs.
*
*
* Amazon Elastic MapReduce (Amazon EMR) is a web service that makes it
* easy to process large amounts of data efficiently. Amazon EMR uses
* Hadoop processing combined with several AWS products to do tasks such
* as web indexing, data mining, log file analysis, machine learning,
* scientific simulation, and data warehousing.
*
*/
public class AmazonElasticMapReduceClient extends AmazonWebServiceClient implements AmazonElasticMapReduce {
/** Provider for AWS credentials. */
private AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AmazonElasticMapReduce.class);
/**
* List of exception unmarshallers for all AmazonElasticMapReduce exceptions.
*/
protected List jsonErrorUnmarshallers;
/**
* Constructs a new client to invoke service methods on
* AmazonElasticMapReduce. A credentials provider chain will be used
* that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @see DefaultAWSCredentialsProviderChain
*/
public AmazonElasticMapReduceClient() {
this(new DefaultAWSCredentialsProviderChain(), new ClientConfiguration());
}
/**
* Constructs a new client to invoke service methods on
* AmazonElasticMapReduce. A credentials provider chain will be used
* that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to AmazonElasticMapReduce
* (ex: proxy settings, retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
*/
public AmazonElasticMapReduceClient(ClientConfiguration clientConfiguration) {
this(new DefaultAWSCredentialsProviderChain(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on
* AmazonElasticMapReduce using the specified AWS account credentials.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentials The AWS credentials (access key ID and secret key) to use
* when authenticating with AWS services.
*/
public AmazonElasticMapReduceClient(AWSCredentials awsCredentials) {
this(awsCredentials, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service methods on
* AmazonElasticMapReduce using the specified AWS account credentials
* and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentials The AWS credentials (access key ID and secret key) to use
* when authenticating with AWS services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to AmazonElasticMapReduce
* (ex: proxy settings, retry counts, etc.).
*/
public AmazonElasticMapReduceClient(AWSCredentials awsCredentials, ClientConfiguration clientConfiguration) {
super(adjustClientConfiguration(clientConfiguration));
this.awsCredentialsProvider = new StaticCredentialsProvider(awsCredentials);
init();
}
/**
* Constructs a new client to invoke service methods on
* AmazonElasticMapReduce using the specified AWS account credentials provider.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
*/
public AmazonElasticMapReduceClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service methods on
* AmazonElasticMapReduce using the specified AWS account credentials
* provider and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to AmazonElasticMapReduce
* (ex: proxy settings, retry counts, etc.).
*/
public AmazonElasticMapReduceClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on
* AmazonElasticMapReduce using the specified AWS account credentials
* provider, client configuration options and request metric collector.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to AmazonElasticMapReduce
* (ex: proxy settings, retry counts, etc.).
* @param requestMetricCollector optional request metric collector
*/
public AmazonElasticMapReduceClient(AWSCredentialsProvider awsCredentialsProvider,
ClientConfiguration clientConfiguration,
RequestMetricCollector requestMetricCollector) {
super(adjustClientConfiguration(clientConfiguration), requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
init();
}
private void init() {
jsonErrorUnmarshallers = new ArrayList();
jsonErrorUnmarshallers.add(new InternalServerExceptionUnmarshaller());
jsonErrorUnmarshallers.add(new InternalServerErrorExceptionUnmarshaller());
jsonErrorUnmarshallers.add(new InvalidRequestExceptionUnmarshaller());
jsonErrorUnmarshallers.add(new JsonErrorUnmarshaller());
// calling this.setEndPoint(...) will also modify the signer accordingly
this.setEndpoint("elasticmapreduce.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain(
"/com/amazonaws/services/elasticmapreduce/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain(
"/com/amazonaws/services/elasticmapreduce/request.handler2s"));
}
private static ClientConfiguration adjustClientConfiguration(ClientConfiguration orig) {
ClientConfiguration config = orig;
return config;
}
/**
*
* Provides information about the bootstrap actions associated with a
* cluster.
*
*
* @param listBootstrapActionsRequest Container for the necessary
* parameters to execute the ListBootstrapActions service method on
* AmazonElasticMapReduce.
*
* @return The response from the ListBootstrapActions service method, as
* returned by AmazonElasticMapReduce.
*
* @throws InternalServerException
* @throws InvalidRequestException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListBootstrapActionsResult listBootstrapActions(ListBootstrapActionsRequest listBootstrapActionsRequest) {
ExecutionContext executionContext = createExecutionContext(listBootstrapActionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListBootstrapActionsRequestMarshaller().marshall(listBootstrapActionsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new ListBootstrapActionsResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Adds tags to an Amazon EMR resource. Tags make it easier to associate
* clusters in various ways, such as grouping clusters to track your
* Amazon EMR resource allocation costs. For more information, see
* Tagging Amazon EMR Resources
* .
*
*
* @param addTagsRequest Container for the necessary parameters to
* execute the AddTags service method on AmazonElasticMapReduce.
*
* @return The response from the AddTags service method, as returned by
* AmazonElasticMapReduce.
*
* @throws InternalServerException
* @throws InvalidRequestException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public AddTagsResult addTags(AddTagsRequest addTagsRequest) {
ExecutionContext executionContext = createExecutionContext(addTagsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AddTagsRequestMarshaller().marshall(addTagsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new AddTagsResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Sets whether all AWS Identity and Access Management (IAM) users under
* your account can access the specified job flows. This action works on
* running job flows. You can also set the visibility of a job flow when
* you launch it using the VisibleToAllUsers
parameter of
* RunJobFlow. The SetVisibleToAllUsers action can be called only by an
* IAM user who created the job flow or the AWS account that owns the job
* flow.
*
*
* @param setVisibleToAllUsersRequest Container for the necessary
* parameters to execute the SetVisibleToAllUsers service method on
* AmazonElasticMapReduce.
*
*
* @throws InternalServerErrorException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public void setVisibleToAllUsers(SetVisibleToAllUsersRequest setVisibleToAllUsersRequest) {
ExecutionContext executionContext = createExecutionContext(setVisibleToAllUsersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SetVisibleToAllUsersRequestMarshaller().marshall(setVisibleToAllUsersRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Provides a list of steps for the cluster.
*
*
* @param listStepsRequest Container for the necessary parameters to
* execute the ListSteps service method on AmazonElasticMapReduce.
*
* @return The response from the ListSteps service method, as returned by
* AmazonElasticMapReduce.
*
* @throws InternalServerException
* @throws InvalidRequestException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListStepsResult listSteps(ListStepsRequest listStepsRequest) {
ExecutionContext executionContext = createExecutionContext(listStepsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListStepsRequestMarshaller().marshall(listStepsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new ListStepsResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* AddJobFlowSteps adds new steps to a running job flow. A maximum of
* 256 steps are allowed in each job flow.
*
*
* If your job flow is long-running (such as a Hive data warehouse) or
* complex, you may require more than 256 steps to process your data. You
* can bypass the 256-step limitation in various ways, including using
* the SSH shell to connect to the master node and submitting queries
* directly to the software running on the master node, such as Hive and
* Hadoop. For more information on how to do this, go to
* Add More than 256 Steps to a Job Flow
* in the Amazon Elastic MapReduce Developer's Guide .
*
*
* A step specifies the location of a JAR file stored either on the
* master node of the job flow or in Amazon S3. Each step is performed by
* the main function of the main class of the JAR file. The main class
* can be specified either in the manifest of the JAR or by using the
* MainFunction parameter of the step.
*
*
* Elastic MapReduce executes each step in the order listed. For a step
* to be considered complete, the main function must exit with a zero
* exit code and all Hadoop jobs started while the step was running must
* have completed and run successfully.
*
*
* You can only add steps to a job flow that is in one of the following
* states: STARTING, BOOTSTRAPPING, RUNNING, or WAITING.
*
*
* @param addJobFlowStepsRequest Container for the necessary parameters
* to execute the AddJobFlowSteps service method on
* AmazonElasticMapReduce.
*
* @return The response from the AddJobFlowSteps service method, as
* returned by AmazonElasticMapReduce.
*
* @throws InternalServerErrorException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public AddJobFlowStepsResult addJobFlowSteps(AddJobFlowStepsRequest addJobFlowStepsRequest) {
ExecutionContext executionContext = createExecutionContext(addJobFlowStepsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AddJobFlowStepsRequestMarshaller().marshall(addJobFlowStepsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new AddJobFlowStepsResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Provides more detail about the cluster step.
*
*
* @param describeStepRequest Container for the necessary parameters to
* execute the DescribeStep service method on AmazonElasticMapReduce.
*
* @return The response from the DescribeStep service method, as returned
* by AmazonElasticMapReduce.
*
* @throws InternalServerException
* @throws InvalidRequestException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeStepResult describeStep(DescribeStepRequest describeStepRequest) {
ExecutionContext executionContext = createExecutionContext(describeStepRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeStepRequestMarshaller().marshall(describeStepRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new DescribeStepResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Provides the status of all clusters visible to this AWS account.
* Allows you to filter the list of clusters based on certain criteria;
* for example, filtering by cluster creation date and time or by status.
* This call returns a maximum of 50 clusters per call, but returns a
* marker to track the paging of the cluster list across multiple
* ListClusters calls.
*
*
* @param listClustersRequest Container for the necessary parameters to
* execute the ListClusters service method on AmazonElasticMapReduce.
*
* @return The response from the ListClusters service method, as returned
* by AmazonElasticMapReduce.
*
* @throws InternalServerException
* @throws InvalidRequestException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListClustersResult listClusters(ListClustersRequest listClustersRequest) {
ExecutionContext executionContext = createExecutionContext(listClustersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListClustersRequestMarshaller().marshall(listClustersRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new ListClustersResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Removes tags from an Amazon EMR resource. Tags make it easier to
* associate clusters in various ways, such as grouping clusters to track
* your Amazon EMR resource allocation costs. For more information, see
* Tagging Amazon EMR Resources
* .
*
*
* @param removeTagsRequest Container for the necessary parameters to
* execute the RemoveTags service method on AmazonElasticMapReduce.
*
* @return The response from the RemoveTags service method, as returned
* by AmazonElasticMapReduce.
*
* @throws InternalServerException
* @throws InvalidRequestException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public RemoveTagsResult removeTags(RemoveTagsRequest removeTagsRequest) {
ExecutionContext executionContext = createExecutionContext(removeTagsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RemoveTagsRequestMarshaller().marshall(removeTagsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new RemoveTagsResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Provides all available details about the instance groups in a cluster.
*
*
* @param listInstanceGroupsRequest Container for the necessary
* parameters to execute the ListInstanceGroups service method on
* AmazonElasticMapReduce.
*
* @return The response from the ListInstanceGroups service method, as
* returned by AmazonElasticMapReduce.
*
* @throws InternalServerException
* @throws InvalidRequestException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListInstanceGroupsResult listInstanceGroups(ListInstanceGroupsRequest listInstanceGroupsRequest) {
ExecutionContext executionContext = createExecutionContext(listInstanceGroupsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListInstanceGroupsRequestMarshaller().marshall(listInstanceGroupsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new ListInstanceGroupsResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* ModifyInstanceGroups modifies the number of nodes and configuration
* settings of an instance group. The input parameters include the new
* target instance count for the group and the instance group ID. The
* call will either succeed or fail atomically.
*
*
* @param modifyInstanceGroupsRequest Container for the necessary
* parameters to execute the ModifyInstanceGroups service method on
* AmazonElasticMapReduce.
*
*
* @throws InternalServerErrorException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public void modifyInstanceGroups(ModifyInstanceGroupsRequest modifyInstanceGroupsRequest) {
ExecutionContext executionContext = createExecutionContext(modifyInstanceGroupsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ModifyInstanceGroupsRequestMarshaller().marshall(modifyInstanceGroupsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Provides information about the cluster instances that Amazon EMR
* provisions on behalf of a user when it creates the cluster. For
* example, this operation indicates when the EC2 instances reach the
* Ready state, when instances become available to Amazon EMR to use for
* jobs, and the IP addresses for cluster instances, etc.
*
*
* @param listInstancesRequest Container for the necessary parameters to
* execute the ListInstances service method on AmazonElasticMapReduce.
*
* @return The response from the ListInstances service method, as
* returned by AmazonElasticMapReduce.
*
* @throws InternalServerException
* @throws InvalidRequestException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListInstancesResult listInstances(ListInstancesRequest listInstancesRequest) {
ExecutionContext executionContext = createExecutionContext(listInstancesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListInstancesRequestMarshaller().marshall(listInstancesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new ListInstancesResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* AddInstanceGroups adds an instance group to a running cluster.
*
*
* @param addInstanceGroupsRequest Container for the necessary parameters
* to execute the AddInstanceGroups service method on
* AmazonElasticMapReduce.
*
* @return The response from the AddInstanceGroups service method, as
* returned by AmazonElasticMapReduce.
*
* @throws InternalServerErrorException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public AddInstanceGroupsResult addInstanceGroups(AddInstanceGroupsRequest addInstanceGroupsRequest) {
ExecutionContext executionContext = createExecutionContext(addInstanceGroupsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AddInstanceGroupsRequestMarshaller().marshall(addInstanceGroupsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new AddInstanceGroupsResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* TerminateJobFlows shuts a list of job flows down. When a job flow is
* shut down, any step not yet completed is canceled and the EC2
* instances on which the job flow is running are stopped. Any log files
* not already saved are uploaded to Amazon S3 if a LogUri was specified
* when the job flow was created.
*
*
* The call to TerminateJobFlows is asynchronous. Depending on the
* configuration of the job flow, it may take up to 5-20 minutes for the
* job flow to completely terminate and release allocated resources, such
* as Amazon EC2 instances.
*
*
* @param terminateJobFlowsRequest Container for the necessary parameters
* to execute the TerminateJobFlows service method on
* AmazonElasticMapReduce.
*
*
* @throws InternalServerErrorException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public void terminateJobFlows(TerminateJobFlowsRequest terminateJobFlowsRequest) {
ExecutionContext executionContext = createExecutionContext(terminateJobFlowsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TerminateJobFlowsRequestMarshaller().marshall(terminateJobFlowsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* SetTerminationProtection locks a job flow so the Amazon EC2 instances
* in the cluster cannot be terminated by user intervention, an API call,
* or in the event of a job-flow error. The cluster still terminates upon
* successful completion of the job flow. Calling
* SetTerminationProtection on a job flow is analogous to calling the
* Amazon EC2 DisableAPITermination API on all of the EC2 instances in a
* cluster.
*
*
* SetTerminationProtection is used to prevent accidental termination of
* a job flow and to ensure that in the event of an error, the instances
* will persist so you can recover any data stored in their ephemeral
* instance storage.
*
*
* To terminate a job flow that has been locked by setting
* SetTerminationProtection to true
,
* you must first unlock the job flow by a subsequent call to
* SetTerminationProtection in which you set the value to
* false
.
*
*
* For more information, go to
* Protecting a Job Flow from Termination
* in the Amazon Elastic MapReduce Developer's Guide.
*
*
* @param setTerminationProtectionRequest Container for the necessary
* parameters to execute the SetTerminationProtection service method on
* AmazonElasticMapReduce.
*
*
* @throws InternalServerErrorException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public void setTerminationProtection(SetTerminationProtectionRequest setTerminationProtectionRequest) {
ExecutionContext executionContext = createExecutionContext(setTerminationProtectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SetTerminationProtectionRequestMarshaller().marshall(setTerminationProtectionRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* DescribeJobFlows returns a list of job flows that match all of the
* supplied parameters. The parameters can include a list of job flow
* IDs, job flow states, and restrictions on job flow creation date and
* time.
*
*
* Regardless of supplied parameters, only job flows created within the
* last two months are returned.
*
*
* If no parameters are supplied, then job flows matching either of the
* following criteria are returned:
*
*
*
* - Job flows created and completed in the last two weeks
* - Job flows created within the last two months that are in one of
* the following states:
RUNNING
,
* WAITING
,
* SHUTTING_DOWN
,
*
* STARTING
*
*
*
* Amazon Elastic MapReduce can return a maximum of 512 job flow
* descriptions.
*
*
* @param describeJobFlowsRequest Container for the necessary parameters
* to execute the DescribeJobFlows service method on
* AmazonElasticMapReduce.
*
* @return The response from the DescribeJobFlows service method, as
* returned by AmazonElasticMapReduce.
*
* @throws InternalServerErrorException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
@Deprecated
public DescribeJobFlowsResult describeJobFlows(DescribeJobFlowsRequest describeJobFlowsRequest) {
ExecutionContext executionContext = createExecutionContext(describeJobFlowsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeJobFlowsRequestMarshaller().marshall(describeJobFlowsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new DescribeJobFlowsResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* RunJobFlow creates and starts running a new job flow. The job flow
* will run the steps specified. Once the job flow completes, the cluster
* is stopped and the HDFS partition is lost. To prevent loss of data,
* configure the last step of the job flow to store results in Amazon S3.
* If the JobFlowInstancesConfig KeepJobFlowAliveWhenNoSteps
* parameter is set to TRUE
, the job flow will transition
* to the WAITING state rather than shutting down once the steps have
* completed.
*
*
* For additional protection, you can set the JobFlowInstancesConfig
* TerminationProtected
parameter to TRUE
to
* lock the job flow and prevent it from being terminated by API call,
* user intervention, or in the event of a job flow error.
*
*
* A maximum of 256 steps are allowed in each job flow.
*
*
* If your job flow is long-running (such as a Hive data warehouse) or
* complex, you may require more than 256 steps to process your data. You
* can bypass the 256-step limitation in various ways, including using
* the SSH shell to connect to the master node and submitting queries
* directly to the software running on the master node, such as Hive and
* Hadoop. For more information on how to do this, go to
* Add More than 256 Steps to a Job Flow
* in the Amazon Elastic MapReduce Developer's Guide .
*
*
* For long running job flows, we recommend that you periodically store
* your results.
*
*
* @param runJobFlowRequest Container for the necessary parameters to
* execute the RunJobFlow service method on AmazonElasticMapReduce.
*
* @return The response from the RunJobFlow service method, as returned
* by AmazonElasticMapReduce.
*
* @throws InternalServerErrorException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public RunJobFlowResult runJobFlow(RunJobFlowRequest runJobFlowRequest) {
ExecutionContext executionContext = createExecutionContext(runJobFlowRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RunJobFlowRequestMarshaller().marshall(runJobFlowRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new RunJobFlowResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Provides cluster-level details including status, hardware and software
* configuration, VPC settings, and so on. For information about the
* cluster steps, see ListSteps.
*
*
* @param describeClusterRequest Container for the necessary parameters
* to execute the DescribeCluster service method on
* AmazonElasticMapReduce.
*
* @return The response from the DescribeCluster service method, as
* returned by AmazonElasticMapReduce.
*
* @throws InternalServerException
* @throws InvalidRequestException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeClusterResult describeCluster(DescribeClusterRequest describeClusterRequest) {
ExecutionContext executionContext = createExecutionContext(describeClusterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeClusterRequestMarshaller().marshall(describeClusterRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new DescribeClusterResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Provides information about the bootstrap actions associated with a
* cluster.
*
*
* @return The response from the ListBootstrapActions service method, as
* returned by AmazonElasticMapReduce.
*
* @throws InternalServerException
* @throws InvalidRequestException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListBootstrapActionsResult listBootstrapActions() throws AmazonServiceException, AmazonClientException {
return listBootstrapActions(new ListBootstrapActionsRequest());
}
/**
*
* Adds tags to an Amazon EMR resource. Tags make it easier to associate
* clusters in various ways, such as grouping clusters to track your
* Amazon EMR resource allocation costs. For more information, see
* Tagging Amazon EMR Resources
* .
*
*
* @return The response from the AddTags service method, as returned by
* AmazonElasticMapReduce.
*
* @throws InternalServerException
* @throws InvalidRequestException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public AddTagsResult addTags() throws AmazonServiceException, AmazonClientException {
return addTags(new AddTagsRequest());
}
/**
*
* Provides a list of steps for the cluster.
*
*
* @return The response from the ListSteps service method, as returned by
* AmazonElasticMapReduce.
*
* @throws InternalServerException
* @throws InvalidRequestException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListStepsResult listSteps() throws AmazonServiceException, AmazonClientException {
return listSteps(new ListStepsRequest());
}
/**
*
* Provides more detail about the cluster step.
*
*
* @return The response from the DescribeStep service method, as returned
* by AmazonElasticMapReduce.
*
* @throws InternalServerException
* @throws InvalidRequestException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeStepResult describeStep() throws AmazonServiceException, AmazonClientException {
return describeStep(new DescribeStepRequest());
}
/**
*
* Provides the status of all clusters visible to this AWS account.
* Allows you to filter the list of clusters based on certain criteria;
* for example, filtering by cluster creation date and time or by status.
* This call returns a maximum of 50 clusters per call, but returns a
* marker to track the paging of the cluster list across multiple
* ListClusters calls.
*
*
* @return The response from the ListClusters service method, as returned
* by AmazonElasticMapReduce.
*
* @throws InternalServerException
* @throws InvalidRequestException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListClustersResult listClusters() throws AmazonServiceException, AmazonClientException {
return listClusters(new ListClustersRequest());
}
/**
*
* Removes tags from an Amazon EMR resource. Tags make it easier to
* associate clusters in various ways, such as grouping clusters to track
* your Amazon EMR resource allocation costs. For more information, see
* Tagging Amazon EMR Resources
* .
*
*
* @return The response from the RemoveTags service method, as returned
* by AmazonElasticMapReduce.
*
* @throws InternalServerException
* @throws InvalidRequestException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public RemoveTagsResult removeTags() throws AmazonServiceException, AmazonClientException {
return removeTags(new RemoveTagsRequest());
}
/**
*
* Provides all available details about the instance groups in a cluster.
*
*
* @return The response from the ListInstanceGroups service method, as
* returned by AmazonElasticMapReduce.
*
* @throws InternalServerException
* @throws InvalidRequestException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListInstanceGroupsResult listInstanceGroups() throws AmazonServiceException, AmazonClientException {
return listInstanceGroups(new ListInstanceGroupsRequest());
}
/**
*
* ModifyInstanceGroups modifies the number of nodes and configuration
* settings of an instance group. The input parameters include the new
* target instance count for the group and the instance group ID. The
* call will either succeed or fail atomically.
*
*
*
* @throws InternalServerErrorException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public void modifyInstanceGroups() throws AmazonServiceException, AmazonClientException {
modifyInstanceGroups(new ModifyInstanceGroupsRequest());
}
/**
*
* Provides information about the cluster instances that Amazon EMR
* provisions on behalf of a user when it creates the cluster. For
* example, this operation indicates when the EC2 instances reach the
* Ready state, when instances become available to Amazon EMR to use for
* jobs, and the IP addresses for cluster instances, etc.
*
*
* @return The response from the ListInstances service method, as
* returned by AmazonElasticMapReduce.
*
* @throws InternalServerException
* @throws InvalidRequestException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListInstancesResult listInstances() throws AmazonServiceException, AmazonClientException {
return listInstances(new ListInstancesRequest());
}
/**
*
* DescribeJobFlows returns a list of job flows that match all of the
* supplied parameters. The parameters can include a list of job flow
* IDs, job flow states, and restrictions on job flow creation date and
* time.
*
*
* Regardless of supplied parameters, only job flows created within the
* last two months are returned.
*
*
* If no parameters are supplied, then job flows matching either of the
* following criteria are returned:
*
*
*
* - Job flows created and completed in the last two weeks
* - Job flows created within the last two months that are in one of
* the following states:
RUNNING
,
* WAITING
,
* SHUTTING_DOWN
,
*
* STARTING
*
*
*
* Amazon Elastic MapReduce can return a maximum of 512 job flow
* descriptions.
*
*
* @return The response from the DescribeJobFlows service method, as
* returned by AmazonElasticMapReduce.
*
* @throws InternalServerErrorException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
@Deprecated
public DescribeJobFlowsResult describeJobFlows() throws AmazonServiceException, AmazonClientException {
return describeJobFlows(new DescribeJobFlowsRequest());
}
/**
*
* Provides cluster-level details including status, hardware and software
* configuration, VPC settings, and so on. For information about the
* cluster steps, see ListSteps.
*
*
* @return The response from the DescribeCluster service method, as
* returned by AmazonElasticMapReduce.
*
* @throws InternalServerException
* @throws InvalidRequestException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticMapReduce indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeClusterResult describeCluster() throws AmazonServiceException, AmazonClientException {
return describeCluster(new DescribeClusterRequest());
}
@Override
public void setEndpoint(String endpoint) {
super.setEndpoint(endpoint);
}
@Override
public void setEndpoint(String endpoint, String serviceName, String regionId) throws IllegalArgumentException {
super.setEndpoint(endpoint, serviceName, regionId);
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for
* debugging issues where a service isn't acting as expected. This data isn't considered part
* of the result data returned by an operation, so it's available through this separate,
* diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access
* this extra diagnostic information for an executed request, you should use this method
* to retrieve it as soon as possible after executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none
* is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
private Response invoke(Request request,
HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
request.setEndpoint(endpoint);
request.setTimeOffset(timeOffset);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
AWSCredentials credentials;
awsRequestMetrics.startEvent(Field.CredentialsRequestTime);
try {
credentials = awsCredentialsProvider.getCredentials();
} finally {
awsRequestMetrics.endEvent(Field.CredentialsRequestTime);
}
AmazonWebServiceRequest originalRequest = request.getOriginalRequest();
if (originalRequest != null && originalRequest.getRequestCredentials() != null) {
credentials = originalRequest.getRequestCredentials();
}
executionContext.setCredentials(credentials);
JsonErrorResponseHandler errorResponseHandler = new JsonErrorResponseHandler(jsonErrorUnmarshallers);
Response result = client.execute(request, responseHandler,
errorResponseHandler, executionContext);
return result;
}
}