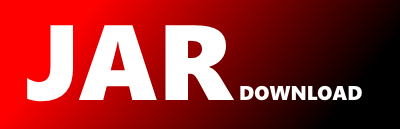
com.amazonaws.services.elastictranscoder.AmazonElasticTranscoderAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.elastictranscoder;
import java.util.concurrent.Future;
import com.amazonaws.AmazonClientException;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.handlers.AsyncHandler;
import com.amazonaws.services.elastictranscoder.model.*;
/**
* Interface for accessing AmazonElasticTranscoder asynchronously.
* Each asynchronous method will return a Java Future object, and users are also allowed
* to provide a callback handler.
* AWS Elastic Transcoder Service
* The AWS Elastic Transcoder Service.
*
*/
public interface AmazonElasticTranscoderAsync extends AmazonElasticTranscoder {
/**
*
* The UpdatePipelineStatus operation pauses or reactivates a pipeline,
* so that the pipeline stops or restarts the processing of jobs.
*
*
* Changing the pipeline status is useful if you want to cancel one or
* more jobs. You can't cancel jobs after Elastic Transcoder has started
* processing them; if you pause the pipeline to which you submitted the
* jobs, you have more time to get the job IDs for the jobs that you want
* to cancel, and to send a CancelJob request.
*
*
* @param updatePipelineStatusRequest Container for the necessary
* parameters to execute the UpdatePipelineStatus operation on
* AmazonElasticTranscoder.
*
* @return A Java Future object containing the response from the
* UpdatePipelineStatus service method, as returned by
* AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updatePipelineStatusAsync(UpdatePipelineStatusRequest updatePipelineStatusRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The UpdatePipelineStatus operation pauses or reactivates a pipeline,
* so that the pipeline stops or restarts the processing of jobs.
*
*
* Changing the pipeline status is useful if you want to cancel one or
* more jobs. You can't cancel jobs after Elastic Transcoder has started
* processing them; if you pause the pipeline to which you submitted the
* jobs, you have more time to get the job IDs for the jobs that you want
* to cancel, and to send a CancelJob request.
*
*
* @param updatePipelineStatusRequest Container for the necessary
* parameters to execute the UpdatePipelineStatus operation on
* AmazonElasticTranscoder.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* UpdatePipelineStatus service method, as returned by
* AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updatePipelineStatusAsync(UpdatePipelineStatusRequest updatePipelineStatusRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* With the UpdatePipelineNotifications operation, you can update Amazon
* Simple Notification Service (Amazon SNS) notifications for a pipeline.
*
*
* When you update notifications for a pipeline, Elastic Transcoder
* returns the values that you specified in the request.
*
*
* @param updatePipelineNotificationsRequest Container for the necessary
* parameters to execute the UpdatePipelineNotifications operation on
* AmazonElasticTranscoder.
*
* @return A Java Future object containing the response from the
* UpdatePipelineNotifications service method, as returned by
* AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updatePipelineNotificationsAsync(UpdatePipelineNotificationsRequest updatePipelineNotificationsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* With the UpdatePipelineNotifications operation, you can update Amazon
* Simple Notification Service (Amazon SNS) notifications for a pipeline.
*
*
* When you update notifications for a pipeline, Elastic Transcoder
* returns the values that you specified in the request.
*
*
* @param updatePipelineNotificationsRequest Container for the necessary
* parameters to execute the UpdatePipelineNotifications operation on
* AmazonElasticTranscoder.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* UpdatePipelineNotifications service method, as returned by
* AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updatePipelineNotificationsAsync(UpdatePipelineNotificationsRequest updatePipelineNotificationsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The ReadJob operation returns detailed information about a job.
*
*
* @param readJobRequest Container for the necessary parameters to
* execute the ReadJob operation on AmazonElasticTranscoder.
*
* @return A Java Future object containing the response from the ReadJob
* service method, as returned by AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future readJobAsync(ReadJobRequest readJobRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The ReadJob operation returns detailed information about a job.
*
*
* @param readJobRequest Container for the necessary parameters to
* execute the ReadJob operation on AmazonElasticTranscoder.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the ReadJob
* service method, as returned by AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future readJobAsync(ReadJobRequest readJobRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The ListJobsByStatus operation gets a list of jobs that have a
* specified status. The response body contains one element for each job
* that satisfies the search criteria.
*
*
* @param listJobsByStatusRequest Container for the necessary parameters
* to execute the ListJobsByStatus operation on AmazonElasticTranscoder.
*
* @return A Java Future object containing the response from the
* ListJobsByStatus service method, as returned by
* AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listJobsByStatusAsync(ListJobsByStatusRequest listJobsByStatusRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The ListJobsByStatus operation gets a list of jobs that have a
* specified status. The response body contains one element for each job
* that satisfies the search criteria.
*
*
* @param listJobsByStatusRequest Container for the necessary parameters
* to execute the ListJobsByStatus operation on AmazonElasticTranscoder.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ListJobsByStatus service method, as returned by
* AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listJobsByStatusAsync(ListJobsByStatusRequest listJobsByStatusRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The ReadPreset operation gets detailed information about a preset.
*
*
* @param readPresetRequest Container for the necessary parameters to
* execute the ReadPreset operation on AmazonElasticTranscoder.
*
* @return A Java Future object containing the response from the
* ReadPreset service method, as returned by AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future readPresetAsync(ReadPresetRequest readPresetRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The ReadPreset operation gets detailed information about a preset.
*
*
* @param readPresetRequest Container for the necessary parameters to
* execute the ReadPreset operation on AmazonElasticTranscoder.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ReadPreset service method, as returned by AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future readPresetAsync(ReadPresetRequest readPresetRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The CreatePipeline operation creates a pipeline with settings that you
* specify.
*
*
* @param createPipelineRequest Container for the necessary parameters to
* execute the CreatePipeline operation on AmazonElasticTranscoder.
*
* @return A Java Future object containing the response from the
* CreatePipeline service method, as returned by AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createPipelineAsync(CreatePipelineRequest createPipelineRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The CreatePipeline operation creates a pipeline with settings that you
* specify.
*
*
* @param createPipelineRequest Container for the necessary parameters to
* execute the CreatePipeline operation on AmazonElasticTranscoder.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreatePipeline service method, as returned by AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createPipelineAsync(CreatePipelineRequest createPipelineRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The CancelJob operation cancels an unfinished job.
*
*
* NOTE:You can only cancel a job that has a status of Submitted.
* To prevent a pipeline from starting to process a job while you're
* getting the job identifier, use UpdatePipelineStatus to temporarily
* pause the pipeline.
*
*
* @param cancelJobRequest Container for the necessary parameters to
* execute the CancelJob operation on AmazonElasticTranscoder.
*
* @return A Java Future object containing the response from the
* CancelJob service method, as returned by AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future cancelJobAsync(CancelJobRequest cancelJobRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The CancelJob operation cancels an unfinished job.
*
*
* NOTE:You can only cancel a job that has a status of Submitted.
* To prevent a pipeline from starting to process a job while you're
* getting the job identifier, use UpdatePipelineStatus to temporarily
* pause the pipeline.
*
*
* @param cancelJobRequest Container for the necessary parameters to
* execute the CancelJob operation on AmazonElasticTranscoder.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CancelJob service method, as returned by AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future cancelJobAsync(CancelJobRequest cancelJobRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Use the UpdatePipeline
operation to update settings for
* a pipeline.
* IMPORTANT:When you change pipeline settings, your changes take
* effect immediately. Jobs that you have already submitted and that
* Elastic Transcoder has not started to process are affected in addition
* to jobs that you submit after you change settings.
*
*
*
*
* @param updatePipelineRequest Container for the necessary parameters to
* execute the UpdatePipeline operation on AmazonElasticTranscoder.
*
* @return A Java Future object containing the response from the
* UpdatePipeline service method, as returned by AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updatePipelineAsync(UpdatePipelineRequest updatePipelineRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Use the UpdatePipeline
operation to update settings for
* a pipeline.
* IMPORTANT:When you change pipeline settings, your changes take
* effect immediately. Jobs that you have already submitted and that
* Elastic Transcoder has not started to process are affected in addition
* to jobs that you submit after you change settings.
*
*
*
*
* @param updatePipelineRequest Container for the necessary parameters to
* execute the UpdatePipeline operation on AmazonElasticTranscoder.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* UpdatePipeline service method, as returned by AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updatePipelineAsync(UpdatePipelineRequest updatePipelineRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The ListPresets operation gets a list of the default presets included
* with Elastic Transcoder and the presets that you've added in an AWS
* region.
*
*
* @param listPresetsRequest Container for the necessary parameters to
* execute the ListPresets operation on AmazonElasticTranscoder.
*
* @return A Java Future object containing the response from the
* ListPresets service method, as returned by AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listPresetsAsync(ListPresetsRequest listPresetsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The ListPresets operation gets a list of the default presets included
* with Elastic Transcoder and the presets that you've added in an AWS
* region.
*
*
* @param listPresetsRequest Container for the necessary parameters to
* execute the ListPresets operation on AmazonElasticTranscoder.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ListPresets service method, as returned by AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listPresetsAsync(ListPresetsRequest listPresetsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DeletePipeline operation removes a pipeline.
*
*
* You can only delete a pipeline that has never been used or that is
* not currently in use (doesn't contain any active jobs). If the
* pipeline is currently in use, DeletePipeline
returns an
* error.
*
*
* @param deletePipelineRequest Container for the necessary parameters to
* execute the DeletePipeline operation on AmazonElasticTranscoder.
*
* @return A Java Future object containing the response from the
* DeletePipeline service method, as returned by AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deletePipelineAsync(DeletePipelineRequest deletePipelineRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DeletePipeline operation removes a pipeline.
*
*
* You can only delete a pipeline that has never been used or that is
* not currently in use (doesn't contain any active jobs). If the
* pipeline is currently in use, DeletePipeline
returns an
* error.
*
*
* @param deletePipelineRequest Container for the necessary parameters to
* execute the DeletePipeline operation on AmazonElasticTranscoder.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeletePipeline service method, as returned by AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deletePipelineAsync(DeletePipelineRequest deletePipelineRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The TestRole operation tests the IAM role used to create the pipeline.
*
*
* The TestRole
action lets you determine whether the IAM
* role you are using has sufficient permissions to let Elastic
* Transcoder perform tasks associated with the transcoding process. The
* action attempts to assume the specified IAM role, checks read access
* to the input and output buckets, and tries to send a test notification
* to Amazon SNS topics that you specify.
*
*
* @param testRoleRequest Container for the necessary parameters to
* execute the TestRole operation on AmazonElasticTranscoder.
*
* @return A Java Future object containing the response from the TestRole
* service method, as returned by AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future testRoleAsync(TestRoleRequest testRoleRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The TestRole operation tests the IAM role used to create the pipeline.
*
*
* The TestRole
action lets you determine whether the IAM
* role you are using has sufficient permissions to let Elastic
* Transcoder perform tasks associated with the transcoding process. The
* action attempts to assume the specified IAM role, checks read access
* to the input and output buckets, and tries to send a test notification
* to Amazon SNS topics that you specify.
*
*
* @param testRoleRequest Container for the necessary parameters to
* execute the TestRole operation on AmazonElasticTranscoder.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the TestRole
* service method, as returned by AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future testRoleAsync(TestRoleRequest testRoleRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The ListPipelines operation gets a list of the pipelines associated
* with the current AWS account.
*
*
* @param listPipelinesRequest Container for the necessary parameters to
* execute the ListPipelines operation on AmazonElasticTranscoder.
*
* @return A Java Future object containing the response from the
* ListPipelines service method, as returned by AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listPipelinesAsync(ListPipelinesRequest listPipelinesRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The ListPipelines operation gets a list of the pipelines associated
* with the current AWS account.
*
*
* @param listPipelinesRequest Container for the necessary parameters to
* execute the ListPipelines operation on AmazonElasticTranscoder.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ListPipelines service method, as returned by AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listPipelinesAsync(ListPipelinesRequest listPipelinesRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The ReadPipeline operation gets detailed information about a pipeline.
*
*
* @param readPipelineRequest Container for the necessary parameters to
* execute the ReadPipeline operation on AmazonElasticTranscoder.
*
* @return A Java Future object containing the response from the
* ReadPipeline service method, as returned by AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future readPipelineAsync(ReadPipelineRequest readPipelineRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The ReadPipeline operation gets detailed information about a pipeline.
*
*
* @param readPipelineRequest Container for the necessary parameters to
* execute the ReadPipeline operation on AmazonElasticTranscoder.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ReadPipeline service method, as returned by AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future readPipelineAsync(ReadPipelineRequest readPipelineRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The CreatePreset operation creates a preset with settings that you
* specify.
*
*
* IMPORTANT:Elastic Transcoder checks the CreatePreset settings
* to ensure that they meet Elastic Transcoder requirements and to
* determine whether they comply with H.264 standards. If your settings
* are not valid for Elastic Transcoder, Elastic Transcoder returns an
* HTTP 400 response (ValidationException) and does not create the
* preset. If the settings are valid for Elastic Transcoder but aren't
* strictly compliant with the H.264 standard, Elastic Transcoder creates
* the preset and returns a warning message in the response. This helps
* you determine whether your settings comply with the H.264 standard
* while giving you greater flexibility with respect to the video that
* Elastic Transcoder produces.
*
*
* Elastic Transcoder uses the H.264 video-compression format. For more
* information, see the International Telecommunication Union publication
* Recommendation ITU-T H.264: Advanced video coding for generic
* audiovisual services .
*
*
* @param createPresetRequest Container for the necessary parameters to
* execute the CreatePreset operation on AmazonElasticTranscoder.
*
* @return A Java Future object containing the response from the
* CreatePreset service method, as returned by AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createPresetAsync(CreatePresetRequest createPresetRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The CreatePreset operation creates a preset with settings that you
* specify.
*
*
* IMPORTANT:Elastic Transcoder checks the CreatePreset settings
* to ensure that they meet Elastic Transcoder requirements and to
* determine whether they comply with H.264 standards. If your settings
* are not valid for Elastic Transcoder, Elastic Transcoder returns an
* HTTP 400 response (ValidationException) and does not create the
* preset. If the settings are valid for Elastic Transcoder but aren't
* strictly compliant with the H.264 standard, Elastic Transcoder creates
* the preset and returns a warning message in the response. This helps
* you determine whether your settings comply with the H.264 standard
* while giving you greater flexibility with respect to the video that
* Elastic Transcoder produces.
*
*
* Elastic Transcoder uses the H.264 video-compression format. For more
* information, see the International Telecommunication Union publication
* Recommendation ITU-T H.264: Advanced video coding for generic
* audiovisual services .
*
*
* @param createPresetRequest Container for the necessary parameters to
* execute the CreatePreset operation on AmazonElasticTranscoder.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreatePreset service method, as returned by AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createPresetAsync(CreatePresetRequest createPresetRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DeletePreset operation removes a preset that you've added in an
* AWS region.
*
*
* NOTE: You can't delete the default presets that are included
* with Elastic Transcoder.
*
*
* @param deletePresetRequest Container for the necessary parameters to
* execute the DeletePreset operation on AmazonElasticTranscoder.
*
* @return A Java Future object containing the response from the
* DeletePreset service method, as returned by AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deletePresetAsync(DeletePresetRequest deletePresetRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DeletePreset operation removes a preset that you've added in an
* AWS region.
*
*
* NOTE: You can't delete the default presets that are included
* with Elastic Transcoder.
*
*
* @param deletePresetRequest Container for the necessary parameters to
* execute the DeletePreset operation on AmazonElasticTranscoder.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeletePreset service method, as returned by AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deletePresetAsync(DeletePresetRequest deletePresetRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* When you create a job, Elastic Transcoder returns JSON data that
* includes the values that you specified plus information about the job
* that is created.
*
*
* If you have specified more than one output for your jobs (for example,
* one output for the Kindle Fire and another output for the Apple iPhone
* 4s), you currently must use the Elastic Transcoder API to list the
* jobs (as opposed to the AWS Console).
*
*
* @param createJobRequest Container for the necessary parameters to
* execute the CreateJob operation on AmazonElasticTranscoder.
*
* @return A Java Future object containing the response from the
* CreateJob service method, as returned by AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createJobAsync(CreateJobRequest createJobRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* When you create a job, Elastic Transcoder returns JSON data that
* includes the values that you specified plus information about the job
* that is created.
*
*
* If you have specified more than one output for your jobs (for example,
* one output for the Kindle Fire and another output for the Apple iPhone
* 4s), you currently must use the Elastic Transcoder API to list the
* jobs (as opposed to the AWS Console).
*
*
* @param createJobRequest Container for the necessary parameters to
* execute the CreateJob operation on AmazonElasticTranscoder.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateJob service method, as returned by AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createJobAsync(CreateJobRequest createJobRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* The ListJobsByPipeline operation gets a list of the jobs currently in
* a pipeline.
*
*
* Elastic Transcoder returns all of the jobs currently in the specified
* pipeline. The response body contains one element for each job that
* satisfies the search criteria.
*
*
* @param listJobsByPipelineRequest Container for the necessary
* parameters to execute the ListJobsByPipeline operation on
* AmazonElasticTranscoder.
*
* @return A Java Future object containing the response from the
* ListJobsByPipeline service method, as returned by
* AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listJobsByPipelineAsync(ListJobsByPipelineRequest listJobsByPipelineRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The ListJobsByPipeline operation gets a list of the jobs currently in
* a pipeline.
*
*
* Elastic Transcoder returns all of the jobs currently in the specified
* pipeline. The response body contains one element for each job that
* satisfies the search criteria.
*
*
* @param listJobsByPipelineRequest Container for the necessary
* parameters to execute the ListJobsByPipeline operation on
* AmazonElasticTranscoder.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ListJobsByPipeline service method, as returned by
* AmazonElasticTranscoder.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonElasticTranscoder indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listJobsByPipelineAsync(ListJobsByPipelineRequest listJobsByPipelineRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
}