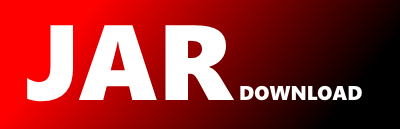
com.amazonaws.services.elastictranscoder.model.CreateJobPlaylist Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.elastictranscoder.model;
import java.io.Serializable;
/**
*
* Information about the master playlist.
*
*/
public class CreateJobPlaylist implements Serializable {
/**
* The name that you want Elastic Transcoder to assign to the master
* playlist, for example, nyc-vacation.m3u8. The name cannot include a /
* character. If you create more than one master playlist (not
* recommended), the values of all Name
objects must be
* unique. Elastic Transcoder automatically appends .m3u8 to the file
* name. If you include .m3u8 in Name
, it will appear twice
* in the file name.
*
* Constraints:
* Length: 1 - 255
*/
private String name;
/**
* This value must currently be HLSv3.
*
* Constraints:
* Pattern: (^HLSv3$)
*/
private String format;
/**
* For each output in this job that you want to include in a master
* playlist, the value of the Outputs:Key
object. If you
* include more than one output in a playlist, the value of
* SegmentDuration
for all of the outputs must be the same.
*
* Constraints:
* Length: 0 - 30
*/
private com.amazonaws.internal.ListWithAutoConstructFlag outputKeys;
/**
* The name that you want Elastic Transcoder to assign to the master
* playlist, for example, nyc-vacation.m3u8. The name cannot include a /
* character. If you create more than one master playlist (not
* recommended), the values of all Name
objects must be
* unique. Elastic Transcoder automatically appends .m3u8 to the file
* name. If you include .m3u8 in Name
, it will appear twice
* in the file name.
*
* Constraints:
* Length: 1 - 255
*
* @return The name that you want Elastic Transcoder to assign to the master
* playlist, for example, nyc-vacation.m3u8. The name cannot include a /
* character. If you create more than one master playlist (not
* recommended), the values of all Name
objects must be
* unique. Elastic Transcoder automatically appends .m3u8 to the file
* name. If you include .m3u8 in Name
, it will appear twice
* in the file name.
*/
public String getName() {
return name;
}
/**
* The name that you want Elastic Transcoder to assign to the master
* playlist, for example, nyc-vacation.m3u8. The name cannot include a /
* character. If you create more than one master playlist (not
* recommended), the values of all Name
objects must be
* unique. Elastic Transcoder automatically appends .m3u8 to the file
* name. If you include .m3u8 in Name
, it will appear twice
* in the file name.
*
* Constraints:
* Length: 1 - 255
*
* @param name The name that you want Elastic Transcoder to assign to the master
* playlist, for example, nyc-vacation.m3u8. The name cannot include a /
* character. If you create more than one master playlist (not
* recommended), the values of all Name
objects must be
* unique. Elastic Transcoder automatically appends .m3u8 to the file
* name. If you include .m3u8 in Name
, it will appear twice
* in the file name.
*/
public void setName(String name) {
this.name = name;
}
/**
* The name that you want Elastic Transcoder to assign to the master
* playlist, for example, nyc-vacation.m3u8. The name cannot include a /
* character. If you create more than one master playlist (not
* recommended), the values of all Name
objects must be
* unique. Elastic Transcoder automatically appends .m3u8 to the file
* name. If you include .m3u8 in Name
, it will appear twice
* in the file name.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 255
*
* @param name The name that you want Elastic Transcoder to assign to the master
* playlist, for example, nyc-vacation.m3u8. The name cannot include a /
* character. If you create more than one master playlist (not
* recommended), the values of all Name
objects must be
* unique. Elastic Transcoder automatically appends .m3u8 to the file
* name. If you include .m3u8 in Name
, it will appear twice
* in the file name.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateJobPlaylist withName(String name) {
this.name = name;
return this;
}
/**
* This value must currently be HLSv3.
*
* Constraints:
* Pattern: (^HLSv3$)
*
* @return This value must currently be HLSv3.
*/
public String getFormat() {
return format;
}
/**
* This value must currently be HLSv3.
*
* Constraints:
* Pattern: (^HLSv3$)
*
* @param format This value must currently be HLSv3.
*/
public void setFormat(String format) {
this.format = format;
}
/**
* This value must currently be HLSv3.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Pattern: (^HLSv3$)
*
* @param format This value must currently be HLSv3.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateJobPlaylist withFormat(String format) {
this.format = format;
return this;
}
/**
* For each output in this job that you want to include in a master
* playlist, the value of the Outputs:Key
object. If you
* include more than one output in a playlist, the value of
* SegmentDuration
for all of the outputs must be the same.
*
* Constraints:
* Length: 0 - 30
*
* @return For each output in this job that you want to include in a master
* playlist, the value of the Outputs:Key
object. If you
* include more than one output in a playlist, the value of
* SegmentDuration
for all of the outputs must be the same.
*/
public java.util.List getOutputKeys() {
if (outputKeys == null) {
outputKeys = new com.amazonaws.internal.ListWithAutoConstructFlag();
outputKeys.setAutoConstruct(true);
}
return outputKeys;
}
/**
* For each output in this job that you want to include in a master
* playlist, the value of the Outputs:Key
object. If you
* include more than one output in a playlist, the value of
* SegmentDuration
for all of the outputs must be the same.
*
* Constraints:
* Length: 0 - 30
*
* @param outputKeys For each output in this job that you want to include in a master
* playlist, the value of the Outputs:Key
object. If you
* include more than one output in a playlist, the value of
* SegmentDuration
for all of the outputs must be the same.
*/
public void setOutputKeys(java.util.Collection outputKeys) {
if (outputKeys == null) {
this.outputKeys = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag outputKeysCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(outputKeys.size());
outputKeysCopy.addAll(outputKeys);
this.outputKeys = outputKeysCopy;
}
/**
* For each output in this job that you want to include in a master
* playlist, the value of the Outputs:Key
object. If you
* include more than one output in a playlist, the value of
* SegmentDuration
for all of the outputs must be the same.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 0 - 30
*
* @param outputKeys For each output in this job that you want to include in a master
* playlist, the value of the Outputs:Key
object. If you
* include more than one output in a playlist, the value of
* SegmentDuration
for all of the outputs must be the same.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateJobPlaylist withOutputKeys(String... outputKeys) {
if (getOutputKeys() == null) setOutputKeys(new java.util.ArrayList(outputKeys.length));
for (String value : outputKeys) {
getOutputKeys().add(value);
}
return this;
}
/**
* For each output in this job that you want to include in a master
* playlist, the value of the Outputs:Key
object. If you
* include more than one output in a playlist, the value of
* SegmentDuration
for all of the outputs must be the same.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 0 - 30
*
* @param outputKeys For each output in this job that you want to include in a master
* playlist, the value of the Outputs:Key
object. If you
* include more than one output in a playlist, the value of
* SegmentDuration
for all of the outputs must be the same.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateJobPlaylist withOutputKeys(java.util.Collection outputKeys) {
if (outputKeys == null) {
this.outputKeys = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag outputKeysCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(outputKeys.size());
outputKeysCopy.addAll(outputKeys);
this.outputKeys = outputKeysCopy;
}
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null) sb.append("Name: " + getName() + ",");
if (getFormat() != null) sb.append("Format: " + getFormat() + ",");
if (getOutputKeys() != null) sb.append("OutputKeys: " + getOutputKeys() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getFormat() == null) ? 0 : getFormat().hashCode());
hashCode = prime * hashCode + ((getOutputKeys() == null) ? 0 : getOutputKeys().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof CreateJobPlaylist == false) return false;
CreateJobPlaylist other = (CreateJobPlaylist)obj;
if (other.getName() == null ^ this.getName() == null) return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false) return false;
if (other.getFormat() == null ^ this.getFormat() == null) return false;
if (other.getFormat() != null && other.getFormat().equals(this.getFormat()) == false) return false;
if (other.getOutputKeys() == null ^ this.getOutputKeys() == null) return false;
if (other.getOutputKeys() != null && other.getOutputKeys().equals(this.getOutputKeys()) == false) return false;
return true;
}
}