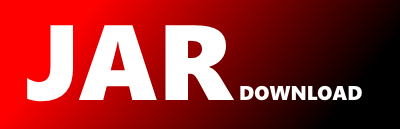
com.amazonaws.services.elastictranscoder.model.Job Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.elastictranscoder.model;
import java.io.Serializable;
/**
*
* A section of the response body that provides information about the job
* that is created.
*
*/
public class Job implements Serializable {
/**
* The identifier that Elastic Transcoder assigned to the job. You use
* this value to get settings for the job or to delete the job.
*
* Constraints:
* Pattern: ^\d{13}-\w{6}$
*/
private String id;
/**
* The Amazon Resource Name (ARN) for the job.
*/
private String arn;
/**
* The Id
of the pipeline that you want Elastic Transcoder
* to use for transcoding. The pipeline determines several settings,
* including the Amazon S3 bucket from which Elastic Transcoder gets the
* files to transcode and the bucket into which Elastic Transcoder puts
* the transcoded files.
*
* Constraints:
* Pattern: ^\d{13}-\w{6}$
*/
private String pipelineId;
/**
* A section of the request or response body that provides information
* about the file that is being transcoded.
*/
private JobInput input;
/**
* If you specified one output for a job, information about that output.
* If you specified multiple outputs for a job, the Output object lists
* information about the first output. This duplicates the information
* that is listed for the first output in the Outputs object.
*
Outputs recommended instead. A section of
* the request or response body that provides information about the
* transcoded (target) file.
*/
private JobOutput output;
/**
* Information about the output files. We recommend that you use the
* Outputs
syntax for all jobs, even when you want Elastic
* Transcoder to transcode a file into only one format. Do not use both
* the Outputs
and Output
syntaxes in the same
* request. You can create a maximum of 30 outputs per job.
If you
* specify more than one output for a job, Elastic Transcoder creates the
* files for each output in the order in which you specify them in the
* job.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag outputs;
/**
* The value, if any, that you want Elastic Transcoder to prepend to the
* names of all files that this job creates, including output files,
* thumbnails, and playlists. We recommend that you add a / or some other
* delimiter to the end of the OutputKeyPrefix
.
*
* Constraints:
* Length: 1 - 255
*/
private String outputKeyPrefix;
/**
* Outputs in MPEG-TS format only. If you specify a
* preset in PresetId
for which the value of
* Container
is ts (MPEG-TS), Playlists
* contains information about the master playlists that you want Elastic
* Transcoder to create.
We recommend that you create only one master
* playlist. The maximum number of master playlists in a job is 30.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag playlists;
/**
* The status of the job: Submitted
,
* Progressing
, Complete
,
* Canceled
, or Error
.
*
* Constraints:
* Pattern: (^Submitted$)|(^Progressing$)|(^Complete$)|(^Canceled$)|(^Error$)
*/
private String status;
/**
* The identifier that Elastic Transcoder assigned to the job. You use
* this value to get settings for the job or to delete the job.
*
* Constraints:
* Pattern: ^\d{13}-\w{6}$
*
* @return The identifier that Elastic Transcoder assigned to the job. You use
* this value to get settings for the job or to delete the job.
*/
public String getId() {
return id;
}
/**
* The identifier that Elastic Transcoder assigned to the job. You use
* this value to get settings for the job or to delete the job.
*
* Constraints:
* Pattern: ^\d{13}-\w{6}$
*
* @param id The identifier that Elastic Transcoder assigned to the job. You use
* this value to get settings for the job or to delete the job.
*/
public void setId(String id) {
this.id = id;
}
/**
* The identifier that Elastic Transcoder assigned to the job. You use
* this value to get settings for the job or to delete the job.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Pattern: ^\d{13}-\w{6}$
*
* @param id The identifier that Elastic Transcoder assigned to the job. You use
* this value to get settings for the job or to delete the job.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Job withId(String id) {
this.id = id;
return this;
}
/**
* The Amazon Resource Name (ARN) for the job.
*
* @return The Amazon Resource Name (ARN) for the job.
*/
public String getArn() {
return arn;
}
/**
* The Amazon Resource Name (ARN) for the job.
*
* @param arn The Amazon Resource Name (ARN) for the job.
*/
public void setArn(String arn) {
this.arn = arn;
}
/**
* The Amazon Resource Name (ARN) for the job.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param arn The Amazon Resource Name (ARN) for the job.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Job withArn(String arn) {
this.arn = arn;
return this;
}
/**
* The Id
of the pipeline that you want Elastic Transcoder
* to use for transcoding. The pipeline determines several settings,
* including the Amazon S3 bucket from which Elastic Transcoder gets the
* files to transcode and the bucket into which Elastic Transcoder puts
* the transcoded files.
*
* Constraints:
* Pattern: ^\d{13}-\w{6}$
*
* @return The Id
of the pipeline that you want Elastic Transcoder
* to use for transcoding. The pipeline determines several settings,
* including the Amazon S3 bucket from which Elastic Transcoder gets the
* files to transcode and the bucket into which Elastic Transcoder puts
* the transcoded files.
*/
public String getPipelineId() {
return pipelineId;
}
/**
* The Id
of the pipeline that you want Elastic Transcoder
* to use for transcoding. The pipeline determines several settings,
* including the Amazon S3 bucket from which Elastic Transcoder gets the
* files to transcode and the bucket into which Elastic Transcoder puts
* the transcoded files.
*
* Constraints:
* Pattern: ^\d{13}-\w{6}$
*
* @param pipelineId The Id
of the pipeline that you want Elastic Transcoder
* to use for transcoding. The pipeline determines several settings,
* including the Amazon S3 bucket from which Elastic Transcoder gets the
* files to transcode and the bucket into which Elastic Transcoder puts
* the transcoded files.
*/
public void setPipelineId(String pipelineId) {
this.pipelineId = pipelineId;
}
/**
* The Id
of the pipeline that you want Elastic Transcoder
* to use for transcoding. The pipeline determines several settings,
* including the Amazon S3 bucket from which Elastic Transcoder gets the
* files to transcode and the bucket into which Elastic Transcoder puts
* the transcoded files.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Pattern: ^\d{13}-\w{6}$
*
* @param pipelineId The Id
of the pipeline that you want Elastic Transcoder
* to use for transcoding. The pipeline determines several settings,
* including the Amazon S3 bucket from which Elastic Transcoder gets the
* files to transcode and the bucket into which Elastic Transcoder puts
* the transcoded files.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Job withPipelineId(String pipelineId) {
this.pipelineId = pipelineId;
return this;
}
/**
* A section of the request or response body that provides information
* about the file that is being transcoded.
*
* @return A section of the request or response body that provides information
* about the file that is being transcoded.
*/
public JobInput getInput() {
return input;
}
/**
* A section of the request or response body that provides information
* about the file that is being transcoded.
*
* @param input A section of the request or response body that provides information
* about the file that is being transcoded.
*/
public void setInput(JobInput input) {
this.input = input;
}
/**
* A section of the request or response body that provides information
* about the file that is being transcoded.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param input A section of the request or response body that provides information
* about the file that is being transcoded.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Job withInput(JobInput input) {
this.input = input;
return this;
}
/**
* If you specified one output for a job, information about that output.
* If you specified multiple outputs for a job, the Output object lists
* information about the first output. This duplicates the information
* that is listed for the first output in the Outputs object.
*
Outputs recommended instead. A section of
* the request or response body that provides information about the
* transcoded (target) file.
*
* @return If you specified one output for a job, information about that output.
* If you specified multiple outputs for a job, the Output object lists
* information about the first output. This duplicates the information
* that is listed for the first output in the Outputs object.
*
Outputs recommended instead. A section of
* the request or response body that provides information about the
* transcoded (target) file.
*/
public JobOutput getOutput() {
return output;
}
/**
* If you specified one output for a job, information about that output.
* If you specified multiple outputs for a job, the Output object lists
* information about the first output. This duplicates the information
* that is listed for the first output in the Outputs object.
*
Outputs recommended instead. A section of
* the request or response body that provides information about the
* transcoded (target) file.
*
* @param output If you specified one output for a job, information about that output.
* If you specified multiple outputs for a job, the Output object lists
* information about the first output. This duplicates the information
* that is listed for the first output in the Outputs object.
*
Outputs recommended instead. A section of
* the request or response body that provides information about the
* transcoded (target) file.
*/
public void setOutput(JobOutput output) {
this.output = output;
}
/**
* If you specified one output for a job, information about that output.
* If you specified multiple outputs for a job, the Output object lists
* information about the first output. This duplicates the information
* that is listed for the first output in the Outputs object.
*
Outputs recommended instead. A section of
* the request or response body that provides information about the
* transcoded (target) file.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param output If you specified one output for a job, information about that output.
* If you specified multiple outputs for a job, the Output object lists
* information about the first output. This duplicates the information
* that is listed for the first output in the Outputs object.
*
Outputs recommended instead. A section of
* the request or response body that provides information about the
* transcoded (target) file.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Job withOutput(JobOutput output) {
this.output = output;
return this;
}
/**
* Information about the output files. We recommend that you use the
* Outputs
syntax for all jobs, even when you want Elastic
* Transcoder to transcode a file into only one format. Do not use both
* the Outputs
and Output
syntaxes in the same
* request. You can create a maximum of 30 outputs per job.
If you
* specify more than one output for a job, Elastic Transcoder creates the
* files for each output in the order in which you specify them in the
* job.
*
* @return Information about the output files. We recommend that you use the
* Outputs
syntax for all jobs, even when you want Elastic
* Transcoder to transcode a file into only one format. Do not use both
* the Outputs
and Output
syntaxes in the same
* request. You can create a maximum of 30 outputs per job.
If you
* specify more than one output for a job, Elastic Transcoder creates the
* files for each output in the order in which you specify them in the
* job.
*/
public java.util.List getOutputs() {
if (outputs == null) {
outputs = new com.amazonaws.internal.ListWithAutoConstructFlag();
outputs.setAutoConstruct(true);
}
return outputs;
}
/**
* Information about the output files. We recommend that you use the
* Outputs
syntax for all jobs, even when you want Elastic
* Transcoder to transcode a file into only one format. Do not use both
* the Outputs
and Output
syntaxes in the same
* request. You can create a maximum of 30 outputs per job. If you
* specify more than one output for a job, Elastic Transcoder creates the
* files for each output in the order in which you specify them in the
* job.
*
* @param outputs Information about the output files. We recommend that you use the
* Outputs
syntax for all jobs, even when you want Elastic
* Transcoder to transcode a file into only one format. Do not use both
* the Outputs
and Output
syntaxes in the same
* request. You can create a maximum of 30 outputs per job.
If you
* specify more than one output for a job, Elastic Transcoder creates the
* files for each output in the order in which you specify them in the
* job.
*/
public void setOutputs(java.util.Collection outputs) {
if (outputs == null) {
this.outputs = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag outputsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(outputs.size());
outputsCopy.addAll(outputs);
this.outputs = outputsCopy;
}
/**
* Information about the output files. We recommend that you use the
* Outputs
syntax for all jobs, even when you want Elastic
* Transcoder to transcode a file into only one format. Do not use both
* the Outputs
and Output
syntaxes in the same
* request. You can create a maximum of 30 outputs per job. If you
* specify more than one output for a job, Elastic Transcoder creates the
* files for each output in the order in which you specify them in the
* job.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param outputs Information about the output files. We recommend that you use the
* Outputs
syntax for all jobs, even when you want Elastic
* Transcoder to transcode a file into only one format. Do not use both
* the Outputs
and Output
syntaxes in the same
* request. You can create a maximum of 30 outputs per job.
If you
* specify more than one output for a job, Elastic Transcoder creates the
* files for each output in the order in which you specify them in the
* job.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Job withOutputs(JobOutput... outputs) {
if (getOutputs() == null) setOutputs(new java.util.ArrayList(outputs.length));
for (JobOutput value : outputs) {
getOutputs().add(value);
}
return this;
}
/**
* Information about the output files. We recommend that you use the
* Outputs
syntax for all jobs, even when you want Elastic
* Transcoder to transcode a file into only one format. Do not use both
* the Outputs
and Output
syntaxes in the same
* request. You can create a maximum of 30 outputs per job. If you
* specify more than one output for a job, Elastic Transcoder creates the
* files for each output in the order in which you specify them in the
* job.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param outputs Information about the output files. We recommend that you use the
* Outputs
syntax for all jobs, even when you want Elastic
* Transcoder to transcode a file into only one format. Do not use both
* the Outputs
and Output
syntaxes in the same
* request. You can create a maximum of 30 outputs per job.
If you
* specify more than one output for a job, Elastic Transcoder creates the
* files for each output in the order in which you specify them in the
* job.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Job withOutputs(java.util.Collection outputs) {
if (outputs == null) {
this.outputs = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag outputsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(outputs.size());
outputsCopy.addAll(outputs);
this.outputs = outputsCopy;
}
return this;
}
/**
* The value, if any, that you want Elastic Transcoder to prepend to the
* names of all files that this job creates, including output files,
* thumbnails, and playlists. We recommend that you add a / or some other
* delimiter to the end of the OutputKeyPrefix
.
*
* Constraints:
* Length: 1 - 255
*
* @return The value, if any, that you want Elastic Transcoder to prepend to the
* names of all files that this job creates, including output files,
* thumbnails, and playlists. We recommend that you add a / or some other
* delimiter to the end of the OutputKeyPrefix
.
*/
public String getOutputKeyPrefix() {
return outputKeyPrefix;
}
/**
* The value, if any, that you want Elastic Transcoder to prepend to the
* names of all files that this job creates, including output files,
* thumbnails, and playlists. We recommend that you add a / or some other
* delimiter to the end of the OutputKeyPrefix
.
*
* Constraints:
* Length: 1 - 255
*
* @param outputKeyPrefix The value, if any, that you want Elastic Transcoder to prepend to the
* names of all files that this job creates, including output files,
* thumbnails, and playlists. We recommend that you add a / or some other
* delimiter to the end of the OutputKeyPrefix
.
*/
public void setOutputKeyPrefix(String outputKeyPrefix) {
this.outputKeyPrefix = outputKeyPrefix;
}
/**
* The value, if any, that you want Elastic Transcoder to prepend to the
* names of all files that this job creates, including output files,
* thumbnails, and playlists. We recommend that you add a / or some other
* delimiter to the end of the OutputKeyPrefix
.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 255
*
* @param outputKeyPrefix The value, if any, that you want Elastic Transcoder to prepend to the
* names of all files that this job creates, including output files,
* thumbnails, and playlists. We recommend that you add a / or some other
* delimiter to the end of the OutputKeyPrefix
.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Job withOutputKeyPrefix(String outputKeyPrefix) {
this.outputKeyPrefix = outputKeyPrefix;
return this;
}
/**
* Outputs in MPEG-TS format only. If you specify a
* preset in PresetId
for which the value of
* Container
is ts (MPEG-TS), Playlists
* contains information about the master playlists that you want Elastic
* Transcoder to create.
We recommend that you create only one master
* playlist. The maximum number of master playlists in a job is 30.
*
* @return Outputs in MPEG-TS format only. If you specify a
* preset in PresetId
for which the value of
* Container
is ts (MPEG-TS), Playlists
* contains information about the master playlists that you want Elastic
* Transcoder to create.
We recommend that you create only one master
* playlist. The maximum number of master playlists in a job is 30.
*/
public java.util.List getPlaylists() {
if (playlists == null) {
playlists = new com.amazonaws.internal.ListWithAutoConstructFlag();
playlists.setAutoConstruct(true);
}
return playlists;
}
/**
* Outputs in MPEG-TS format only. If you specify a
* preset in PresetId
for which the value of
* Container
is ts (MPEG-TS), Playlists
* contains information about the master playlists that you want Elastic
* Transcoder to create. We recommend that you create only one master
* playlist. The maximum number of master playlists in a job is 30.
*
* @param playlists Outputs in MPEG-TS format only. If you specify a
* preset in PresetId
for which the value of
* Container
is ts (MPEG-TS), Playlists
* contains information about the master playlists that you want Elastic
* Transcoder to create.
We recommend that you create only one master
* playlist. The maximum number of master playlists in a job is 30.
*/
public void setPlaylists(java.util.Collection playlists) {
if (playlists == null) {
this.playlists = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag playlistsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(playlists.size());
playlistsCopy.addAll(playlists);
this.playlists = playlistsCopy;
}
/**
* Outputs in MPEG-TS format only. If you specify a
* preset in PresetId
for which the value of
* Container
is ts (MPEG-TS), Playlists
* contains information about the master playlists that you want Elastic
* Transcoder to create. We recommend that you create only one master
* playlist. The maximum number of master playlists in a job is 30.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param playlists Outputs in MPEG-TS format only. If you specify a
* preset in PresetId
for which the value of
* Container
is ts (MPEG-TS), Playlists
* contains information about the master playlists that you want Elastic
* Transcoder to create.
We recommend that you create only one master
* playlist. The maximum number of master playlists in a job is 30.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Job withPlaylists(Playlist... playlists) {
if (getPlaylists() == null) setPlaylists(new java.util.ArrayList(playlists.length));
for (Playlist value : playlists) {
getPlaylists().add(value);
}
return this;
}
/**
* Outputs in MPEG-TS format only. If you specify a
* preset in PresetId
for which the value of
* Container
is ts (MPEG-TS), Playlists
* contains information about the master playlists that you want Elastic
* Transcoder to create. We recommend that you create only one master
* playlist. The maximum number of master playlists in a job is 30.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param playlists Outputs in MPEG-TS format only. If you specify a
* preset in PresetId
for which the value of
* Container
is ts (MPEG-TS), Playlists
* contains information about the master playlists that you want Elastic
* Transcoder to create.
We recommend that you create only one master
* playlist. The maximum number of master playlists in a job is 30.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Job withPlaylists(java.util.Collection playlists) {
if (playlists == null) {
this.playlists = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag playlistsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(playlists.size());
playlistsCopy.addAll(playlists);
this.playlists = playlistsCopy;
}
return this;
}
/**
* The status of the job: Submitted
,
* Progressing
, Complete
,
* Canceled
, or Error
.
*
* Constraints:
* Pattern: (^Submitted$)|(^Progressing$)|(^Complete$)|(^Canceled$)|(^Error$)
*
* @return The status of the job: Submitted
,
* Progressing
, Complete
,
* Canceled
, or Error
.
*/
public String getStatus() {
return status;
}
/**
* The status of the job: Submitted
,
* Progressing
, Complete
,
* Canceled
, or Error
.
*
* Constraints:
* Pattern: (^Submitted$)|(^Progressing$)|(^Complete$)|(^Canceled$)|(^Error$)
*
* @param status The status of the job: Submitted
,
* Progressing
, Complete
,
* Canceled
, or Error
.
*/
public void setStatus(String status) {
this.status = status;
}
/**
* The status of the job: Submitted
,
* Progressing
, Complete
,
* Canceled
, or Error
.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Pattern: (^Submitted$)|(^Progressing$)|(^Complete$)|(^Canceled$)|(^Error$)
*
* @param status The status of the job: Submitted
,
* Progressing
, Complete
,
* Canceled
, or Error
.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Job withStatus(String status) {
this.status = status;
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getId() != null) sb.append("Id: " + getId() + ",");
if (getArn() != null) sb.append("Arn: " + getArn() + ",");
if (getPipelineId() != null) sb.append("PipelineId: " + getPipelineId() + ",");
if (getInput() != null) sb.append("Input: " + getInput() + ",");
if (getOutput() != null) sb.append("Output: " + getOutput() + ",");
if (getOutputs() != null) sb.append("Outputs: " + getOutputs() + ",");
if (getOutputKeyPrefix() != null) sb.append("OutputKeyPrefix: " + getOutputKeyPrefix() + ",");
if (getPlaylists() != null) sb.append("Playlists: " + getPlaylists() + ",");
if (getStatus() != null) sb.append("Status: " + getStatus() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getId() == null) ? 0 : getId().hashCode());
hashCode = prime * hashCode + ((getArn() == null) ? 0 : getArn().hashCode());
hashCode = prime * hashCode + ((getPipelineId() == null) ? 0 : getPipelineId().hashCode());
hashCode = prime * hashCode + ((getInput() == null) ? 0 : getInput().hashCode());
hashCode = prime * hashCode + ((getOutput() == null) ? 0 : getOutput().hashCode());
hashCode = prime * hashCode + ((getOutputs() == null) ? 0 : getOutputs().hashCode());
hashCode = prime * hashCode + ((getOutputKeyPrefix() == null) ? 0 : getOutputKeyPrefix().hashCode());
hashCode = prime * hashCode + ((getPlaylists() == null) ? 0 : getPlaylists().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof Job == false) return false;
Job other = (Job)obj;
if (other.getId() == null ^ this.getId() == null) return false;
if (other.getId() != null && other.getId().equals(this.getId()) == false) return false;
if (other.getArn() == null ^ this.getArn() == null) return false;
if (other.getArn() != null && other.getArn().equals(this.getArn()) == false) return false;
if (other.getPipelineId() == null ^ this.getPipelineId() == null) return false;
if (other.getPipelineId() != null && other.getPipelineId().equals(this.getPipelineId()) == false) return false;
if (other.getInput() == null ^ this.getInput() == null) return false;
if (other.getInput() != null && other.getInput().equals(this.getInput()) == false) return false;
if (other.getOutput() == null ^ this.getOutput() == null) return false;
if (other.getOutput() != null && other.getOutput().equals(this.getOutput()) == false) return false;
if (other.getOutputs() == null ^ this.getOutputs() == null) return false;
if (other.getOutputs() != null && other.getOutputs().equals(this.getOutputs()) == false) return false;
if (other.getOutputKeyPrefix() == null ^ this.getOutputKeyPrefix() == null) return false;
if (other.getOutputKeyPrefix() != null && other.getOutputKeyPrefix().equals(this.getOutputKeyPrefix()) == false) return false;
if (other.getPlaylists() == null ^ this.getPlaylists() == null) return false;
if (other.getPlaylists() != null && other.getPlaylists().equals(this.getPlaylists()) == false) return false;
if (other.getStatus() == null ^ this.getStatus() == null) return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false) return false;
return true;
}
}