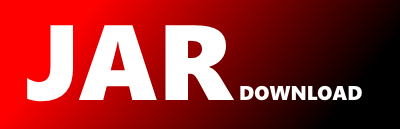
com.amazonaws.services.elastictranscoder.model.JobOutput Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.elastictranscoder.model;
import java.io.Serializable;
/**
*
*
* IMPORTANT:Outputs recommended instead.
*
* If you specified one output for a job, information about that output.
* If you specified multiple outputs for a job, the Output
* object lists information about the first output. This duplicates the
* information that is listed for the first output in the
* Outputs
object.
*
*/
public class JobOutput implements Serializable {
/**
* A sequential counter, starting with 1, that identifies an output among
* the outputs from the current job. In the Output syntax, this value is
* always 1.
*/
private String id;
/**
* The name to assign to the transcoded file. Elastic Transcoder saves
* the file in the Amazon S3 bucket specified by the
* OutputBucket
object in the pipeline that is specified by
* the pipeline ID.
*
* Constraints:
* Length: 1 - 255
*/
private String key;
/**
* Whether you want Elastic Transcoder to create thumbnails for your
* videos and, if so, how you want Elastic Transcoder to name the files.
*
If you don't want Elastic Transcoder to create thumbnails, specify
* "".
If you do want Elastic Transcoder to create thumbnails,
* specify the information that you want to include in the file name for
* each thumbnail. You can specify the following values in any sequence:
*
-
{count}
(Required): If you want to
* create thumbnails, you must include {count}
in the
* ThumbnailPattern
object. Wherever you specify
* {count}
, Elastic Transcoder adds a five-digit sequence
* number (beginning with 00001) to thumbnail file names. The
* number indicates where a given thumbnail appears in the sequence of
* thumbnails for a transcoded file. If you specify a literal
* value and/or {resolution}
but you omit
* {count}
, Elastic Transcoder returns a validation error
* and does not create the job.
-
Literal
* values (Optional): You can specify literal values anywhere in the
* ThumbnailPattern
object. For example, you can include
* them as a file name prefix or as a delimiter between
* {resolution}
and {count}
.
-
* {resolution}
(Optional): If you want Elastic
* Transcoder to include the resolution in the file name, include
* {resolution}
in the ThumbnailPattern
object.
*
When creating thumbnails, Elastic Transcoder
* automatically saves the files in the format (.jpg or .png) that
* appears in the preset that you specified in the PresetID
* value of CreateJobOutput
. Elastic Transcoder also appends
* the applicable file name extension.
*
* Constraints:
* Pattern: (^$)|(^.*\{count\}.*$)
*/
private String thumbnailPattern;
/**
* The number of degrees clockwise by which you want Elastic Transcoder
* to rotate the output relative to the input. Enter one of the following
* values:
auto
, 0
, 90
,
* 180
, 270
The value auto
* generally works only if the file that you're transcoding contains
* rotation metadata.
*
* Constraints:
* Pattern: (^auto$)|(^0$)|(^90$)|(^180$)|(^270$)
*/
private String rotate;
/**
* The value of the Id
object for the preset that you want
* to use for this job. The preset determines the audio, video, and
* thumbnail settings that Elastic Transcoder uses for transcoding. To
* use a preset that you created, specify the preset ID that Elastic
* Transcoder returned in the response when you created the preset. You
* can also use the Elastic Transcoder system presets, which you can get
* with ListPresets
.
*
* Constraints:
* Pattern: ^\d{13}-\w{6}$
*/
private String presetId;
/**
* (Outputs in MPEG-TS format only. If you specify
* a preset in PresetId
for which the value of
* Container
is ts
(MPEG-TS),
* SegmentDuration
is the maximum duration of each .ts file
* in seconds. The range of valid values is 1 to 60 seconds. If the
* duration of the video is not evenly divisible by
* SegmentDuration
, the duration of the last segment is the
* remainder of total length/SegmentDuration. Elastic Transcoder creates
* an output-specific playlist for each output that you specify in
* OutputKeys. To add an output to the master playlist for this job,
* include it in OutputKeys
.
*
* Constraints:
* Pattern: ^\d{1,5}(\.\d{0,5})?$
*/
private String segmentDuration;
/**
* The status of one output in a job. If you specified only one output
* for the job, Outputs:Status
is always the same as
* Job:Status
. If you specified more than one output:
* Job:Status
and Outputs:Status
for all of
* the outputs is Submitted until Elastic Transcoder starts to process
* the first output. - When Elastic Transcoder starts to process
* the first output,
Outputs:Status
for that output and
* Job:Status
both change to Progressing. For each output,
* the value of Outputs:Status
remains Submitted until
* Elastic Transcoder starts to process the output. - Job:Status
* remains Progressing until all of the outputs reach a terminal status,
* either Complete or Error.
- When all of the outputs reach a
* terminal status,
Job:Status
changes to Complete only if
* Outputs:Status
for all of the outputs is
* Complete
. If Outputs:Status
for one or more
* outputs is Error
, the terminal status for
* Job:Status
is also Error
.
The
* value of Status
is one of the following:
* Submitted
, Progressing
,
* Complete
, Canceled
, or Error
.
*
* Constraints:
* Pattern: (^Submitted$)|(^Progressing$)|(^Complete$)|(^Canceled$)|(^Error$)
*/
private String status;
/**
* Information that further explains Status
.
*
* Constraints:
* Length: 0 - 255
*/
private String statusDetail;
/**
* Duration of the output file, in seconds.
*/
private Long duration;
/**
* Specifies the width of the output file in pixels.
*/
private Integer width;
/**
* Height of the output file, in pixels.
*/
private Integer height;
/**
* Information about the watermarks that you want Elastic Transcoder to
* add to the video during transcoding. You can specify up to four
* watermarks for each output. Settings for each watermark must be
* defined in the preset that you specify in Preset
for the
* current output.
Watermarks are added to the output video in the
* sequence in which you list them in the job output???the first
* watermark in the list is added to the output video first, the second
* watermark in the list is added next, and so on. As a result, if the
* settings in a preset cause Elastic Transcoder to place all watermarks
* in the same location, the second watermark that you add will cover the
* first one, the third one will cover the second, and the fourth one
* will cover the third.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag watermarks;
/**
* The album art to be associated with the output file, if any.
*/
private JobAlbumArt albumArt;
/**
* You can create an output file that contains an excerpt from the input
* file. This excerpt, called a clip, can come from the beginning,
* middle, or end of the file. The Composition object contains settings
* for the clips that make up an output file. For the current release,
* you can only specify settings for a single clip per output file. The
* Composition object cannot be null.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag composition;
/**
* A sequential counter, starting with 1, that identifies an output among
* the outputs from the current job. In the Output syntax, this value is
* always 1.
*
* @return A sequential counter, starting with 1, that identifies an output among
* the outputs from the current job. In the Output syntax, this value is
* always 1.
*/
public String getId() {
return id;
}
/**
* A sequential counter, starting with 1, that identifies an output among
* the outputs from the current job. In the Output syntax, this value is
* always 1.
*
* @param id A sequential counter, starting with 1, that identifies an output among
* the outputs from the current job. In the Output syntax, this value is
* always 1.
*/
public void setId(String id) {
this.id = id;
}
/**
* A sequential counter, starting with 1, that identifies an output among
* the outputs from the current job. In the Output syntax, this value is
* always 1.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param id A sequential counter, starting with 1, that identifies an output among
* the outputs from the current job. In the Output syntax, this value is
* always 1.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public JobOutput withId(String id) {
this.id = id;
return this;
}
/**
* The name to assign to the transcoded file. Elastic Transcoder saves
* the file in the Amazon S3 bucket specified by the
* OutputBucket
object in the pipeline that is specified by
* the pipeline ID.
*
* Constraints:
* Length: 1 - 255
*
* @return The name to assign to the transcoded file. Elastic Transcoder saves
* the file in the Amazon S3 bucket specified by the
* OutputBucket
object in the pipeline that is specified by
* the pipeline ID.
*/
public String getKey() {
return key;
}
/**
* The name to assign to the transcoded file. Elastic Transcoder saves
* the file in the Amazon S3 bucket specified by the
* OutputBucket
object in the pipeline that is specified by
* the pipeline ID.
*
* Constraints:
* Length: 1 - 255
*
* @param key The name to assign to the transcoded file. Elastic Transcoder saves
* the file in the Amazon S3 bucket specified by the
* OutputBucket
object in the pipeline that is specified by
* the pipeline ID.
*/
public void setKey(String key) {
this.key = key;
}
/**
* The name to assign to the transcoded file. Elastic Transcoder saves
* the file in the Amazon S3 bucket specified by the
* OutputBucket
object in the pipeline that is specified by
* the pipeline ID.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 255
*
* @param key The name to assign to the transcoded file. Elastic Transcoder saves
* the file in the Amazon S3 bucket specified by the
* OutputBucket
object in the pipeline that is specified by
* the pipeline ID.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public JobOutput withKey(String key) {
this.key = key;
return this;
}
/**
* Whether you want Elastic Transcoder to create thumbnails for your
* videos and, if so, how you want Elastic Transcoder to name the files.
*
If you don't want Elastic Transcoder to create thumbnails, specify
* "".
If you do want Elastic Transcoder to create thumbnails,
* specify the information that you want to include in the file name for
* each thumbnail. You can specify the following values in any sequence:
*
-
{count}
(Required): If you want to
* create thumbnails, you must include {count}
in the
* ThumbnailPattern
object. Wherever you specify
* {count}
, Elastic Transcoder adds a five-digit sequence
* number (beginning with 00001) to thumbnail file names. The
* number indicates where a given thumbnail appears in the sequence of
* thumbnails for a transcoded file. If you specify a literal
* value and/or {resolution}
but you omit
* {count}
, Elastic Transcoder returns a validation error
* and does not create the job.
-
Literal
* values (Optional): You can specify literal values anywhere in the
* ThumbnailPattern
object. For example, you can include
* them as a file name prefix or as a delimiter between
* {resolution}
and {count}
.
-
* {resolution}
(Optional): If you want Elastic
* Transcoder to include the resolution in the file name, include
* {resolution}
in the ThumbnailPattern
object.
*
When creating thumbnails, Elastic Transcoder
* automatically saves the files in the format (.jpg or .png) that
* appears in the preset that you specified in the PresetID
* value of CreateJobOutput
. Elastic Transcoder also appends
* the applicable file name extension.
*
* Constraints:
* Pattern: (^$)|(^.*\{count\}.*$)
*
* @return Whether you want Elastic Transcoder to create thumbnails for your
* videos and, if so, how you want Elastic Transcoder to name the files.
*
If you don't want Elastic Transcoder to create thumbnails, specify
* "".
If you do want Elastic Transcoder to create thumbnails,
* specify the information that you want to include in the file name for
* each thumbnail. You can specify the following values in any sequence:
*
-
{count}
(Required): If you want to
* create thumbnails, you must include {count}
in the
* ThumbnailPattern
object. Wherever you specify
* {count}
, Elastic Transcoder adds a five-digit sequence
* number (beginning with 00001) to thumbnail file names. The
* number indicates where a given thumbnail appears in the sequence of
* thumbnails for a transcoded file. If you specify a literal
* value and/or {resolution}
but you omit
* {count}
, Elastic Transcoder returns a validation error
* and does not create the job.
-
Literal
* values (Optional): You can specify literal values anywhere in the
* ThumbnailPattern
object. For example, you can include
* them as a file name prefix or as a delimiter between
* {resolution}
and {count}
.
-
* {resolution}
(Optional): If you want Elastic
* Transcoder to include the resolution in the file name, include
* {resolution}
in the ThumbnailPattern
object.
*
When creating thumbnails, Elastic Transcoder
* automatically saves the files in the format (.jpg or .png) that
* appears in the preset that you specified in the PresetID
* value of CreateJobOutput
. Elastic Transcoder also appends
* the applicable file name extension.
*/
public String getThumbnailPattern() {
return thumbnailPattern;
}
/**
* Whether you want Elastic Transcoder to create thumbnails for your
* videos and, if so, how you want Elastic Transcoder to name the files.
*
If you don't want Elastic Transcoder to create thumbnails, specify
* "".
If you do want Elastic Transcoder to create thumbnails,
* specify the information that you want to include in the file name for
* each thumbnail. You can specify the following values in any sequence:
*
-
{count}
(Required): If you want to
* create thumbnails, you must include {count}
in the
* ThumbnailPattern
object. Wherever you specify
* {count}
, Elastic Transcoder adds a five-digit sequence
* number (beginning with 00001) to thumbnail file names. The
* number indicates where a given thumbnail appears in the sequence of
* thumbnails for a transcoded file. If you specify a literal
* value and/or {resolution}
but you omit
* {count}
, Elastic Transcoder returns a validation error
* and does not create the job.
-
Literal
* values (Optional): You can specify literal values anywhere in the
* ThumbnailPattern
object. For example, you can include
* them as a file name prefix or as a delimiter between
* {resolution}
and {count}
.
-
* {resolution}
(Optional): If you want Elastic
* Transcoder to include the resolution in the file name, include
* {resolution}
in the ThumbnailPattern
object.
*
When creating thumbnails, Elastic Transcoder
* automatically saves the files in the format (.jpg or .png) that
* appears in the preset that you specified in the PresetID
* value of CreateJobOutput
. Elastic Transcoder also appends
* the applicable file name extension.
*
* Constraints:
* Pattern: (^$)|(^.*\{count\}.*$)
*
* @param thumbnailPattern Whether you want Elastic Transcoder to create thumbnails for your
* videos and, if so, how you want Elastic Transcoder to name the files.
*
If you don't want Elastic Transcoder to create thumbnails, specify
* "".
If you do want Elastic Transcoder to create thumbnails,
* specify the information that you want to include in the file name for
* each thumbnail. You can specify the following values in any sequence:
*
-
{count}
(Required): If you want to
* create thumbnails, you must include {count}
in the
* ThumbnailPattern
object. Wherever you specify
* {count}
, Elastic Transcoder adds a five-digit sequence
* number (beginning with 00001) to thumbnail file names. The
* number indicates where a given thumbnail appears in the sequence of
* thumbnails for a transcoded file. If you specify a literal
* value and/or {resolution}
but you omit
* {count}
, Elastic Transcoder returns a validation error
* and does not create the job.
-
Literal
* values (Optional): You can specify literal values anywhere in the
* ThumbnailPattern
object. For example, you can include
* them as a file name prefix or as a delimiter between
* {resolution}
and {count}
.
-
* {resolution}
(Optional): If you want Elastic
* Transcoder to include the resolution in the file name, include
* {resolution}
in the ThumbnailPattern
object.
*
When creating thumbnails, Elastic Transcoder
* automatically saves the files in the format (.jpg or .png) that
* appears in the preset that you specified in the PresetID
* value of CreateJobOutput
. Elastic Transcoder also appends
* the applicable file name extension.
*/
public void setThumbnailPattern(String thumbnailPattern) {
this.thumbnailPattern = thumbnailPattern;
}
/**
* Whether you want Elastic Transcoder to create thumbnails for your
* videos and, if so, how you want Elastic Transcoder to name the files.
*
If you don't want Elastic Transcoder to create thumbnails, specify
* "".
If you do want Elastic Transcoder to create thumbnails,
* specify the information that you want to include in the file name for
* each thumbnail. You can specify the following values in any sequence:
*
-
{count}
(Required): If you want to
* create thumbnails, you must include {count}
in the
* ThumbnailPattern
object. Wherever you specify
* {count}
, Elastic Transcoder adds a five-digit sequence
* number (beginning with 00001) to thumbnail file names. The
* number indicates where a given thumbnail appears in the sequence of
* thumbnails for a transcoded file. If you specify a literal
* value and/or {resolution}
but you omit
* {count}
, Elastic Transcoder returns a validation error
* and does not create the job.
-
Literal
* values (Optional): You can specify literal values anywhere in the
* ThumbnailPattern
object. For example, you can include
* them as a file name prefix or as a delimiter between
* {resolution}
and {count}
.
-
* {resolution}
(Optional): If you want Elastic
* Transcoder to include the resolution in the file name, include
* {resolution}
in the ThumbnailPattern
object.
*
When creating thumbnails, Elastic Transcoder
* automatically saves the files in the format (.jpg or .png) that
* appears in the preset that you specified in the PresetID
* value of CreateJobOutput
. Elastic Transcoder also appends
* the applicable file name extension.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Pattern: (^$)|(^.*\{count\}.*$)
*
* @param thumbnailPattern Whether you want Elastic Transcoder to create thumbnails for your
* videos and, if so, how you want Elastic Transcoder to name the files.
*
If you don't want Elastic Transcoder to create thumbnails, specify
* "".
If you do want Elastic Transcoder to create thumbnails,
* specify the information that you want to include in the file name for
* each thumbnail. You can specify the following values in any sequence:
*
-
{count}
(Required): If you want to
* create thumbnails, you must include {count}
in the
* ThumbnailPattern
object. Wherever you specify
* {count}
, Elastic Transcoder adds a five-digit sequence
* number (beginning with 00001) to thumbnail file names. The
* number indicates where a given thumbnail appears in the sequence of
* thumbnails for a transcoded file. If you specify a literal
* value and/or {resolution}
but you omit
* {count}
, Elastic Transcoder returns a validation error
* and does not create the job.
-
Literal
* values (Optional): You can specify literal values anywhere in the
* ThumbnailPattern
object. For example, you can include
* them as a file name prefix or as a delimiter between
* {resolution}
and {count}
.
-
* {resolution}
(Optional): If you want Elastic
* Transcoder to include the resolution in the file name, include
* {resolution}
in the ThumbnailPattern
object.
*
When creating thumbnails, Elastic Transcoder
* automatically saves the files in the format (.jpg or .png) that
* appears in the preset that you specified in the PresetID
* value of CreateJobOutput
. Elastic Transcoder also appends
* the applicable file name extension.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public JobOutput withThumbnailPattern(String thumbnailPattern) {
this.thumbnailPattern = thumbnailPattern;
return this;
}
/**
* The number of degrees clockwise by which you want Elastic Transcoder
* to rotate the output relative to the input. Enter one of the following
* values:
auto
, 0
, 90
,
* 180
, 270
The value auto
* generally works only if the file that you're transcoding contains
* rotation metadata.
*
* Constraints:
* Pattern: (^auto$)|(^0$)|(^90$)|(^180$)|(^270$)
*
* @return The number of degrees clockwise by which you want Elastic Transcoder
* to rotate the output relative to the input. Enter one of the following
* values:
auto
, 0
, 90
,
* 180
, 270
The value auto
* generally works only if the file that you're transcoding contains
* rotation metadata.
*/
public String getRotate() {
return rotate;
}
/**
* The number of degrees clockwise by which you want Elastic Transcoder
* to rotate the output relative to the input. Enter one of the following
* values:
auto
, 0
, 90
,
* 180
, 270
The value auto
* generally works only if the file that you're transcoding contains
* rotation metadata.
*
* Constraints:
* Pattern: (^auto$)|(^0$)|(^90$)|(^180$)|(^270$)
*
* @param rotate The number of degrees clockwise by which you want Elastic Transcoder
* to rotate the output relative to the input. Enter one of the following
* values:
auto
, 0
, 90
,
* 180
, 270
The value auto
* generally works only if the file that you're transcoding contains
* rotation metadata.
*/
public void setRotate(String rotate) {
this.rotate = rotate;
}
/**
* The number of degrees clockwise by which you want Elastic Transcoder
* to rotate the output relative to the input. Enter one of the following
* values:
auto
, 0
, 90
,
* 180
, 270
The value auto
* generally works only if the file that you're transcoding contains
* rotation metadata.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Pattern: (^auto$)|(^0$)|(^90$)|(^180$)|(^270$)
*
* @param rotate The number of degrees clockwise by which you want Elastic Transcoder
* to rotate the output relative to the input. Enter one of the following
* values:
auto
, 0
, 90
,
* 180
, 270
The value auto
* generally works only if the file that you're transcoding contains
* rotation metadata.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public JobOutput withRotate(String rotate) {
this.rotate = rotate;
return this;
}
/**
* The value of the Id
object for the preset that you want
* to use for this job. The preset determines the audio, video, and
* thumbnail settings that Elastic Transcoder uses for transcoding. To
* use a preset that you created, specify the preset ID that Elastic
* Transcoder returned in the response when you created the preset. You
* can also use the Elastic Transcoder system presets, which you can get
* with ListPresets
.
*
* Constraints:
* Pattern: ^\d{13}-\w{6}$
*
* @return The value of the Id
object for the preset that you want
* to use for this job. The preset determines the audio, video, and
* thumbnail settings that Elastic Transcoder uses for transcoding. To
* use a preset that you created, specify the preset ID that Elastic
* Transcoder returned in the response when you created the preset. You
* can also use the Elastic Transcoder system presets, which you can get
* with ListPresets
.
*/
public String getPresetId() {
return presetId;
}
/**
* The value of the Id
object for the preset that you want
* to use for this job. The preset determines the audio, video, and
* thumbnail settings that Elastic Transcoder uses for transcoding. To
* use a preset that you created, specify the preset ID that Elastic
* Transcoder returned in the response when you created the preset. You
* can also use the Elastic Transcoder system presets, which you can get
* with ListPresets
.
*
* Constraints:
* Pattern: ^\d{13}-\w{6}$
*
* @param presetId The value of the Id
object for the preset that you want
* to use for this job. The preset determines the audio, video, and
* thumbnail settings that Elastic Transcoder uses for transcoding. To
* use a preset that you created, specify the preset ID that Elastic
* Transcoder returned in the response when you created the preset. You
* can also use the Elastic Transcoder system presets, which you can get
* with ListPresets
.
*/
public void setPresetId(String presetId) {
this.presetId = presetId;
}
/**
* The value of the Id
object for the preset that you want
* to use for this job. The preset determines the audio, video, and
* thumbnail settings that Elastic Transcoder uses for transcoding. To
* use a preset that you created, specify the preset ID that Elastic
* Transcoder returned in the response when you created the preset. You
* can also use the Elastic Transcoder system presets, which you can get
* with ListPresets
.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Pattern: ^\d{13}-\w{6}$
*
* @param presetId The value of the Id
object for the preset that you want
* to use for this job. The preset determines the audio, video, and
* thumbnail settings that Elastic Transcoder uses for transcoding. To
* use a preset that you created, specify the preset ID that Elastic
* Transcoder returned in the response when you created the preset. You
* can also use the Elastic Transcoder system presets, which you can get
* with ListPresets
.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public JobOutput withPresetId(String presetId) {
this.presetId = presetId;
return this;
}
/**
* (Outputs in MPEG-TS format only. If you specify
* a preset in PresetId
for which the value of
* Container
is ts
(MPEG-TS),
* SegmentDuration
is the maximum duration of each .ts file
* in seconds. The range of valid values is 1 to 60 seconds. If the
* duration of the video is not evenly divisible by
* SegmentDuration
, the duration of the last segment is the
* remainder of total length/SegmentDuration. Elastic Transcoder creates
* an output-specific playlist for each output that you specify in
* OutputKeys. To add an output to the master playlist for this job,
* include it in OutputKeys
.
*
* Constraints:
* Pattern: ^\d{1,5}(\.\d{0,5})?$
*
* @return (Outputs in MPEG-TS format only. If you specify
* a preset in PresetId
for which the value of
* Container
is ts
(MPEG-TS),
* SegmentDuration
is the maximum duration of each .ts file
* in seconds. The range of valid values is 1 to 60 seconds. If the
* duration of the video is not evenly divisible by
* SegmentDuration
, the duration of the last segment is the
* remainder of total length/SegmentDuration. Elastic Transcoder creates
* an output-specific playlist for each output that you specify in
* OutputKeys. To add an output to the master playlist for this job,
* include it in OutputKeys
.
*/
public String getSegmentDuration() {
return segmentDuration;
}
/**
* (Outputs in MPEG-TS format only. If you specify
* a preset in PresetId
for which the value of
* Container
is ts
(MPEG-TS),
* SegmentDuration
is the maximum duration of each .ts file
* in seconds. The range of valid values is 1 to 60 seconds. If the
* duration of the video is not evenly divisible by
* SegmentDuration
, the duration of the last segment is the
* remainder of total length/SegmentDuration. Elastic Transcoder creates
* an output-specific playlist for each output that you specify in
* OutputKeys. To add an output to the master playlist for this job,
* include it in OutputKeys
.
*
* Constraints:
* Pattern: ^\d{1,5}(\.\d{0,5})?$
*
* @param segmentDuration (Outputs in MPEG-TS format only. If you specify
* a preset in PresetId
for which the value of
* Container
is ts
(MPEG-TS),
* SegmentDuration
is the maximum duration of each .ts file
* in seconds. The range of valid values is 1 to 60 seconds. If the
* duration of the video is not evenly divisible by
* SegmentDuration
, the duration of the last segment is the
* remainder of total length/SegmentDuration. Elastic Transcoder creates
* an output-specific playlist for each output that you specify in
* OutputKeys. To add an output to the master playlist for this job,
* include it in OutputKeys
.
*/
public void setSegmentDuration(String segmentDuration) {
this.segmentDuration = segmentDuration;
}
/**
* (Outputs in MPEG-TS format only. If you specify
* a preset in PresetId
for which the value of
* Container
is ts
(MPEG-TS),
* SegmentDuration
is the maximum duration of each .ts file
* in seconds. The range of valid values is 1 to 60 seconds. If the
* duration of the video is not evenly divisible by
* SegmentDuration
, the duration of the last segment is the
* remainder of total length/SegmentDuration. Elastic Transcoder creates
* an output-specific playlist for each output that you specify in
* OutputKeys. To add an output to the master playlist for this job,
* include it in OutputKeys
.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Pattern: ^\d{1,5}(\.\d{0,5})?$
*
* @param segmentDuration (Outputs in MPEG-TS format only. If you specify
* a preset in PresetId
for which the value of
* Container
is ts
(MPEG-TS),
* SegmentDuration
is the maximum duration of each .ts file
* in seconds. The range of valid values is 1 to 60 seconds. If the
* duration of the video is not evenly divisible by
* SegmentDuration
, the duration of the last segment is the
* remainder of total length/SegmentDuration. Elastic Transcoder creates
* an output-specific playlist for each output that you specify in
* OutputKeys. To add an output to the master playlist for this job,
* include it in OutputKeys
.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public JobOutput withSegmentDuration(String segmentDuration) {
this.segmentDuration = segmentDuration;
return this;
}
/**
* The status of one output in a job. If you specified only one output
* for the job, Outputs:Status
is always the same as
* Job:Status
. If you specified more than one output:
* Job:Status
and Outputs:Status
for all of
* the outputs is Submitted until Elastic Transcoder starts to process
* the first output. - When Elastic Transcoder starts to process
* the first output,
Outputs:Status
for that output and
* Job:Status
both change to Progressing. For each output,
* the value of Outputs:Status
remains Submitted until
* Elastic Transcoder starts to process the output. - Job:Status
* remains Progressing until all of the outputs reach a terminal status,
* either Complete or Error.
- When all of the outputs reach a
* terminal status,
Job:Status
changes to Complete only if
* Outputs:Status
for all of the outputs is
* Complete
. If Outputs:Status
for one or more
* outputs is Error
, the terminal status for
* Job:Status
is also Error
.
The
* value of Status
is one of the following:
* Submitted
, Progressing
,
* Complete
, Canceled
, or Error
.
*
* Constraints:
* Pattern: (^Submitted$)|(^Progressing$)|(^Complete$)|(^Canceled$)|(^Error$)
*
* @return The status of one output in a job. If you specified only one output
* for the job, Outputs:Status
is always the same as
* Job:Status
. If you specified more than one output:
* Job:Status
and Outputs:Status
for all of
* the outputs is Submitted until Elastic Transcoder starts to process
* the first output. - When Elastic Transcoder starts to process
* the first output,
Outputs:Status
for that output and
* Job:Status
both change to Progressing. For each output,
* the value of Outputs:Status
remains Submitted until
* Elastic Transcoder starts to process the output. - Job:Status
* remains Progressing until all of the outputs reach a terminal status,
* either Complete or Error.
- When all of the outputs reach a
* terminal status,
Job:Status
changes to Complete only if
* Outputs:Status
for all of the outputs is
* Complete
. If Outputs:Status
for one or more
* outputs is Error
, the terminal status for
* Job:Status
is also Error
.
The
* value of Status
is one of the following:
* Submitted
, Progressing
,
* Complete
, Canceled
, or Error
.
*/
public String getStatus() {
return status;
}
/**
* The status of one output in a job. If you specified only one output
* for the job, Outputs:Status
is always the same as
* Job:Status
. If you specified more than one output:
* Job:Status
and Outputs:Status
for all of
* the outputs is Submitted until Elastic Transcoder starts to process
* the first output. - When Elastic Transcoder starts to process
* the first output,
Outputs:Status
for that output and
* Job:Status
both change to Progressing. For each output,
* the value of Outputs:Status
remains Submitted until
* Elastic Transcoder starts to process the output. - Job:Status
* remains Progressing until all of the outputs reach a terminal status,
* either Complete or Error.
- When all of the outputs reach a
* terminal status,
Job:Status
changes to Complete only if
* Outputs:Status
for all of the outputs is
* Complete
. If Outputs:Status
for one or more
* outputs is Error
, the terminal status for
* Job:Status
is also Error
.
The
* value of Status
is one of the following:
* Submitted
, Progressing
,
* Complete
, Canceled
, or Error
.
*
* Constraints:
* Pattern: (^Submitted$)|(^Progressing$)|(^Complete$)|(^Canceled$)|(^Error$)
*
* @param status The status of one output in a job. If you specified only one output
* for the job, Outputs:Status
is always the same as
* Job:Status
. If you specified more than one output:
* Job:Status
and Outputs:Status
for all of
* the outputs is Submitted until Elastic Transcoder starts to process
* the first output. - When Elastic Transcoder starts to process
* the first output,
Outputs:Status
for that output and
* Job:Status
both change to Progressing. For each output,
* the value of Outputs:Status
remains Submitted until
* Elastic Transcoder starts to process the output. - Job:Status
* remains Progressing until all of the outputs reach a terminal status,
* either Complete or Error.
- When all of the outputs reach a
* terminal status,
Job:Status
changes to Complete only if
* Outputs:Status
for all of the outputs is
* Complete
. If Outputs:Status
for one or more
* outputs is Error
, the terminal status for
* Job:Status
is also Error
.
The
* value of Status
is one of the following:
* Submitted
, Progressing
,
* Complete
, Canceled
, or Error
.
*/
public void setStatus(String status) {
this.status = status;
}
/**
* The status of one output in a job. If you specified only one output
* for the job, Outputs:Status
is always the same as
* Job:Status
. If you specified more than one output:
* Job:Status
and Outputs:Status
for all of
* the outputs is Submitted until Elastic Transcoder starts to process
* the first output. - When Elastic Transcoder starts to process
* the first output,
Outputs:Status
for that output and
* Job:Status
both change to Progressing. For each output,
* the value of Outputs:Status
remains Submitted until
* Elastic Transcoder starts to process the output. - Job:Status
* remains Progressing until all of the outputs reach a terminal status,
* either Complete or Error.
- When all of the outputs reach a
* terminal status,
Job:Status
changes to Complete only if
* Outputs:Status
for all of the outputs is
* Complete
. If Outputs:Status
for one or more
* outputs is Error
, the terminal status for
* Job:Status
is also Error
.
The
* value of Status
is one of the following:
* Submitted
, Progressing
,
* Complete
, Canceled
, or Error
.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Pattern: (^Submitted$)|(^Progressing$)|(^Complete$)|(^Canceled$)|(^Error$)
*
* @param status The status of one output in a job. If you specified only one output
* for the job, Outputs:Status
is always the same as
* Job:Status
. If you specified more than one output:
* Job:Status
and Outputs:Status
for all of
* the outputs is Submitted until Elastic Transcoder starts to process
* the first output. - When Elastic Transcoder starts to process
* the first output,
Outputs:Status
for that output and
* Job:Status
both change to Progressing. For each output,
* the value of Outputs:Status
remains Submitted until
* Elastic Transcoder starts to process the output. - Job:Status
* remains Progressing until all of the outputs reach a terminal status,
* either Complete or Error.
- When all of the outputs reach a
* terminal status,
Job:Status
changes to Complete only if
* Outputs:Status
for all of the outputs is
* Complete
. If Outputs:Status
for one or more
* outputs is Error
, the terminal status for
* Job:Status
is also Error
.
The
* value of Status
is one of the following:
* Submitted
, Progressing
,
* Complete
, Canceled
, or Error
.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public JobOutput withStatus(String status) {
this.status = status;
return this;
}
/**
* Information that further explains Status
.
*
* Constraints:
* Length: 0 - 255
*
* @return Information that further explains Status
.
*/
public String getStatusDetail() {
return statusDetail;
}
/**
* Information that further explains Status
.
*
* Constraints:
* Length: 0 - 255
*
* @param statusDetail Information that further explains Status
.
*/
public void setStatusDetail(String statusDetail) {
this.statusDetail = statusDetail;
}
/**
* Information that further explains Status
.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 0 - 255
*
* @param statusDetail Information that further explains Status
.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public JobOutput withStatusDetail(String statusDetail) {
this.statusDetail = statusDetail;
return this;
}
/**
* Duration of the output file, in seconds.
*
* @return Duration of the output file, in seconds.
*/
public Long getDuration() {
return duration;
}
/**
* Duration of the output file, in seconds.
*
* @param duration Duration of the output file, in seconds.
*/
public void setDuration(Long duration) {
this.duration = duration;
}
/**
* Duration of the output file, in seconds.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param duration Duration of the output file, in seconds.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public JobOutput withDuration(Long duration) {
this.duration = duration;
return this;
}
/**
* Specifies the width of the output file in pixels.
*
* @return Specifies the width of the output file in pixels.
*/
public Integer getWidth() {
return width;
}
/**
* Specifies the width of the output file in pixels.
*
* @param width Specifies the width of the output file in pixels.
*/
public void setWidth(Integer width) {
this.width = width;
}
/**
* Specifies the width of the output file in pixels.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param width Specifies the width of the output file in pixels.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public JobOutput withWidth(Integer width) {
this.width = width;
return this;
}
/**
* Height of the output file, in pixels.
*
* @return Height of the output file, in pixels.
*/
public Integer getHeight() {
return height;
}
/**
* Height of the output file, in pixels.
*
* @param height Height of the output file, in pixels.
*/
public void setHeight(Integer height) {
this.height = height;
}
/**
* Height of the output file, in pixels.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param height Height of the output file, in pixels.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public JobOutput withHeight(Integer height) {
this.height = height;
return this;
}
/**
* Information about the watermarks that you want Elastic Transcoder to
* add to the video during transcoding. You can specify up to four
* watermarks for each output. Settings for each watermark must be
* defined in the preset that you specify in Preset
for the
* current output.
Watermarks are added to the output video in the
* sequence in which you list them in the job output???the first
* watermark in the list is added to the output video first, the second
* watermark in the list is added next, and so on. As a result, if the
* settings in a preset cause Elastic Transcoder to place all watermarks
* in the same location, the second watermark that you add will cover the
* first one, the third one will cover the second, and the fourth one
* will cover the third.
*
* @return Information about the watermarks that you want Elastic Transcoder to
* add to the video during transcoding. You can specify up to four
* watermarks for each output. Settings for each watermark must be
* defined in the preset that you specify in Preset
for the
* current output.
Watermarks are added to the output video in the
* sequence in which you list them in the job output???the first
* watermark in the list is added to the output video first, the second
* watermark in the list is added next, and so on. As a result, if the
* settings in a preset cause Elastic Transcoder to place all watermarks
* in the same location, the second watermark that you add will cover the
* first one, the third one will cover the second, and the fourth one
* will cover the third.
*/
public java.util.List getWatermarks() {
if (watermarks == null) {
watermarks = new com.amazonaws.internal.ListWithAutoConstructFlag();
watermarks.setAutoConstruct(true);
}
return watermarks;
}
/**
* Information about the watermarks that you want Elastic Transcoder to
* add to the video during transcoding. You can specify up to four
* watermarks for each output. Settings for each watermark must be
* defined in the preset that you specify in Preset
for the
* current output. Watermarks are added to the output video in the
* sequence in which you list them in the job output???the first
* watermark in the list is added to the output video first, the second
* watermark in the list is added next, and so on. As a result, if the
* settings in a preset cause Elastic Transcoder to place all watermarks
* in the same location, the second watermark that you add will cover the
* first one, the third one will cover the second, and the fourth one
* will cover the third.
*
* @param watermarks Information about the watermarks that you want Elastic Transcoder to
* add to the video during transcoding. You can specify up to four
* watermarks for each output. Settings for each watermark must be
* defined in the preset that you specify in Preset
for the
* current output.
Watermarks are added to the output video in the
* sequence in which you list them in the job output???the first
* watermark in the list is added to the output video first, the second
* watermark in the list is added next, and so on. As a result, if the
* settings in a preset cause Elastic Transcoder to place all watermarks
* in the same location, the second watermark that you add will cover the
* first one, the third one will cover the second, and the fourth one
* will cover the third.
*/
public void setWatermarks(java.util.Collection watermarks) {
if (watermarks == null) {
this.watermarks = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag watermarksCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(watermarks.size());
watermarksCopy.addAll(watermarks);
this.watermarks = watermarksCopy;
}
/**
* Information about the watermarks that you want Elastic Transcoder to
* add to the video during transcoding. You can specify up to four
* watermarks for each output. Settings for each watermark must be
* defined in the preset that you specify in Preset
for the
* current output. Watermarks are added to the output video in the
* sequence in which you list them in the job output???the first
* watermark in the list is added to the output video first, the second
* watermark in the list is added next, and so on. As a result, if the
* settings in a preset cause Elastic Transcoder to place all watermarks
* in the same location, the second watermark that you add will cover the
* first one, the third one will cover the second, and the fourth one
* will cover the third.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param watermarks Information about the watermarks that you want Elastic Transcoder to
* add to the video during transcoding. You can specify up to four
* watermarks for each output. Settings for each watermark must be
* defined in the preset that you specify in Preset
for the
* current output.
Watermarks are added to the output video in the
* sequence in which you list them in the job output???the first
* watermark in the list is added to the output video first, the second
* watermark in the list is added next, and so on. As a result, if the
* settings in a preset cause Elastic Transcoder to place all watermarks
* in the same location, the second watermark that you add will cover the
* first one, the third one will cover the second, and the fourth one
* will cover the third.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public JobOutput withWatermarks(JobWatermark... watermarks) {
if (getWatermarks() == null) setWatermarks(new java.util.ArrayList(watermarks.length));
for (JobWatermark value : watermarks) {
getWatermarks().add(value);
}
return this;
}
/**
* Information about the watermarks that you want Elastic Transcoder to
* add to the video during transcoding. You can specify up to four
* watermarks for each output. Settings for each watermark must be
* defined in the preset that you specify in Preset
for the
* current output. Watermarks are added to the output video in the
* sequence in which you list them in the job output???the first
* watermark in the list is added to the output video first, the second
* watermark in the list is added next, and so on. As a result, if the
* settings in a preset cause Elastic Transcoder to place all watermarks
* in the same location, the second watermark that you add will cover the
* first one, the third one will cover the second, and the fourth one
* will cover the third.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param watermarks Information about the watermarks that you want Elastic Transcoder to
* add to the video during transcoding. You can specify up to four
* watermarks for each output. Settings for each watermark must be
* defined in the preset that you specify in Preset
for the
* current output.
Watermarks are added to the output video in the
* sequence in which you list them in the job output???the first
* watermark in the list is added to the output video first, the second
* watermark in the list is added next, and so on. As a result, if the
* settings in a preset cause Elastic Transcoder to place all watermarks
* in the same location, the second watermark that you add will cover the
* first one, the third one will cover the second, and the fourth one
* will cover the third.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public JobOutput withWatermarks(java.util.Collection watermarks) {
if (watermarks == null) {
this.watermarks = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag watermarksCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(watermarks.size());
watermarksCopy.addAll(watermarks);
this.watermarks = watermarksCopy;
}
return this;
}
/**
* The album art to be associated with the output file, if any.
*
* @return The album art to be associated with the output file, if any.
*/
public JobAlbumArt getAlbumArt() {
return albumArt;
}
/**
* The album art to be associated with the output file, if any.
*
* @param albumArt The album art to be associated with the output file, if any.
*/
public void setAlbumArt(JobAlbumArt albumArt) {
this.albumArt = albumArt;
}
/**
* The album art to be associated with the output file, if any.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param albumArt The album art to be associated with the output file, if any.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public JobOutput withAlbumArt(JobAlbumArt albumArt) {
this.albumArt = albumArt;
return this;
}
/**
* You can create an output file that contains an excerpt from the input
* file. This excerpt, called a clip, can come from the beginning,
* middle, or end of the file. The Composition object contains settings
* for the clips that make up an output file. For the current release,
* you can only specify settings for a single clip per output file. The
* Composition object cannot be null.
*
* @return You can create an output file that contains an excerpt from the input
* file. This excerpt, called a clip, can come from the beginning,
* middle, or end of the file. The Composition object contains settings
* for the clips that make up an output file. For the current release,
* you can only specify settings for a single clip per output file. The
* Composition object cannot be null.
*/
public java.util.List getComposition() {
if (composition == null) {
composition = new com.amazonaws.internal.ListWithAutoConstructFlag();
composition.setAutoConstruct(true);
}
return composition;
}
/**
* You can create an output file that contains an excerpt from the input
* file. This excerpt, called a clip, can come from the beginning,
* middle, or end of the file. The Composition object contains settings
* for the clips that make up an output file. For the current release,
* you can only specify settings for a single clip per output file. The
* Composition object cannot be null.
*
* @param composition You can create an output file that contains an excerpt from the input
* file. This excerpt, called a clip, can come from the beginning,
* middle, or end of the file. The Composition object contains settings
* for the clips that make up an output file. For the current release,
* you can only specify settings for a single clip per output file. The
* Composition object cannot be null.
*/
public void setComposition(java.util.Collection composition) {
if (composition == null) {
this.composition = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag compositionCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(composition.size());
compositionCopy.addAll(composition);
this.composition = compositionCopy;
}
/**
* You can create an output file that contains an excerpt from the input
* file. This excerpt, called a clip, can come from the beginning,
* middle, or end of the file. The Composition object contains settings
* for the clips that make up an output file. For the current release,
* you can only specify settings for a single clip per output file. The
* Composition object cannot be null.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param composition You can create an output file that contains an excerpt from the input
* file. This excerpt, called a clip, can come from the beginning,
* middle, or end of the file. The Composition object contains settings
* for the clips that make up an output file. For the current release,
* you can only specify settings for a single clip per output file. The
* Composition object cannot be null.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public JobOutput withComposition(Clip... composition) {
if (getComposition() == null) setComposition(new java.util.ArrayList(composition.length));
for (Clip value : composition) {
getComposition().add(value);
}
return this;
}
/**
* You can create an output file that contains an excerpt from the input
* file. This excerpt, called a clip, can come from the beginning,
* middle, or end of the file. The Composition object contains settings
* for the clips that make up an output file. For the current release,
* you can only specify settings for a single clip per output file. The
* Composition object cannot be null.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param composition You can create an output file that contains an excerpt from the input
* file. This excerpt, called a clip, can come from the beginning,
* middle, or end of the file. The Composition object contains settings
* for the clips that make up an output file. For the current release,
* you can only specify settings for a single clip per output file. The
* Composition object cannot be null.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public JobOutput withComposition(java.util.Collection composition) {
if (composition == null) {
this.composition = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag compositionCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(composition.size());
compositionCopy.addAll(composition);
this.composition = compositionCopy;
}
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getId() != null) sb.append("Id: " + getId() + ",");
if (getKey() != null) sb.append("Key: " + getKey() + ",");
if (getThumbnailPattern() != null) sb.append("ThumbnailPattern: " + getThumbnailPattern() + ",");
if (getRotate() != null) sb.append("Rotate: " + getRotate() + ",");
if (getPresetId() != null) sb.append("PresetId: " + getPresetId() + ",");
if (getSegmentDuration() != null) sb.append("SegmentDuration: " + getSegmentDuration() + ",");
if (getStatus() != null) sb.append("Status: " + getStatus() + ",");
if (getStatusDetail() != null) sb.append("StatusDetail: " + getStatusDetail() + ",");
if (getDuration() != null) sb.append("Duration: " + getDuration() + ",");
if (getWidth() != null) sb.append("Width: " + getWidth() + ",");
if (getHeight() != null) sb.append("Height: " + getHeight() + ",");
if (getWatermarks() != null) sb.append("Watermarks: " + getWatermarks() + ",");
if (getAlbumArt() != null) sb.append("AlbumArt: " + getAlbumArt() + ",");
if (getComposition() != null) sb.append("Composition: " + getComposition() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getId() == null) ? 0 : getId().hashCode());
hashCode = prime * hashCode + ((getKey() == null) ? 0 : getKey().hashCode());
hashCode = prime * hashCode + ((getThumbnailPattern() == null) ? 0 : getThumbnailPattern().hashCode());
hashCode = prime * hashCode + ((getRotate() == null) ? 0 : getRotate().hashCode());
hashCode = prime * hashCode + ((getPresetId() == null) ? 0 : getPresetId().hashCode());
hashCode = prime * hashCode + ((getSegmentDuration() == null) ? 0 : getSegmentDuration().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getStatusDetail() == null) ? 0 : getStatusDetail().hashCode());
hashCode = prime * hashCode + ((getDuration() == null) ? 0 : getDuration().hashCode());
hashCode = prime * hashCode + ((getWidth() == null) ? 0 : getWidth().hashCode());
hashCode = prime * hashCode + ((getHeight() == null) ? 0 : getHeight().hashCode());
hashCode = prime * hashCode + ((getWatermarks() == null) ? 0 : getWatermarks().hashCode());
hashCode = prime * hashCode + ((getAlbumArt() == null) ? 0 : getAlbumArt().hashCode());
hashCode = prime * hashCode + ((getComposition() == null) ? 0 : getComposition().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof JobOutput == false) return false;
JobOutput other = (JobOutput)obj;
if (other.getId() == null ^ this.getId() == null) return false;
if (other.getId() != null && other.getId().equals(this.getId()) == false) return false;
if (other.getKey() == null ^ this.getKey() == null) return false;
if (other.getKey() != null && other.getKey().equals(this.getKey()) == false) return false;
if (other.getThumbnailPattern() == null ^ this.getThumbnailPattern() == null) return false;
if (other.getThumbnailPattern() != null && other.getThumbnailPattern().equals(this.getThumbnailPattern()) == false) return false;
if (other.getRotate() == null ^ this.getRotate() == null) return false;
if (other.getRotate() != null && other.getRotate().equals(this.getRotate()) == false) return false;
if (other.getPresetId() == null ^ this.getPresetId() == null) return false;
if (other.getPresetId() != null && other.getPresetId().equals(this.getPresetId()) == false) return false;
if (other.getSegmentDuration() == null ^ this.getSegmentDuration() == null) return false;
if (other.getSegmentDuration() != null && other.getSegmentDuration().equals(this.getSegmentDuration()) == false) return false;
if (other.getStatus() == null ^ this.getStatus() == null) return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false) return false;
if (other.getStatusDetail() == null ^ this.getStatusDetail() == null) return false;
if (other.getStatusDetail() != null && other.getStatusDetail().equals(this.getStatusDetail()) == false) return false;
if (other.getDuration() == null ^ this.getDuration() == null) return false;
if (other.getDuration() != null && other.getDuration().equals(this.getDuration()) == false) return false;
if (other.getWidth() == null ^ this.getWidth() == null) return false;
if (other.getWidth() != null && other.getWidth().equals(this.getWidth()) == false) return false;
if (other.getHeight() == null ^ this.getHeight() == null) return false;
if (other.getHeight() != null && other.getHeight().equals(this.getHeight()) == false) return false;
if (other.getWatermarks() == null ^ this.getWatermarks() == null) return false;
if (other.getWatermarks() != null && other.getWatermarks().equals(this.getWatermarks()) == false) return false;
if (other.getAlbumArt() == null ^ this.getAlbumArt() == null) return false;
if (other.getAlbumArt() != null && other.getAlbumArt().equals(this.getAlbumArt()) == false) return false;
if (other.getComposition() == null ^ this.getComposition() == null) return false;
if (other.getComposition() != null && other.getComposition().equals(this.getComposition()) == false) return false;
return true;
}
}