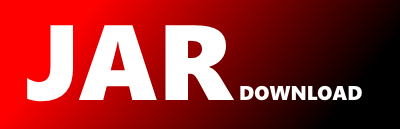
com.amazonaws.services.elastictranscoder.model.PresetWatermark Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.elastictranscoder.model;
import java.io.Serializable;
/**
*
* Settings for the size, location, and opacity of graphics that you want
* Elastic Transcoder to overlay over videos that are transcoded using
* this preset. You can specify settings for up to four watermarks.
* Watermarks appear in the specified size and location, and with the
* specified opacity for the duration of the transcoded video.
*
*
* Watermarks can be in .png or .jpg format. If you want to display a
* watermark that is not rectangular, use the .png format, which supports
* transparency.
*
*
* When you create a job that uses this preset, you specify the .png or
* .jpg graphics that you want Elastic Transcoder to include in the
* transcoded videos. You can specify fewer graphics in the job than you
* specify watermark settings in the preset, which allows you to use the
* same preset for up to four watermarks that have different dimensions.
*
*/
public class PresetWatermark implements Serializable {
/**
* A unique identifier for the settings for one watermark. The value of
* Id
can be up to 40 characters long.
*
* Constraints:
* Length: 1 - 40
*/
private String id;
/**
* The maximum width of the watermark in one of the following formats:
*
- number of pixels (px): The minimum value is 16 pixels, and
* the maximum value is the value of
MaxWidth
.
* - integer percentage (%): The range of valid values is 0 to 100. Use
* the value of
Target
to specify whether you want Elastic
* Transcoder to include the black bars that are added by Elastic
* Transcoder, if any, in the calculation. If you specify the value
* in pixels, it must be less than or equal to the value of
* MaxWidth
.
*
* Constraints:
* Pattern: (^\d{1,3}(\.\d{0,5})?%$)|(^\d{2,4}?px$)
*/
private String maxWidth;
/**
* The maximum height of the watermark in one of the following formats:
*
- number of pixels (px): The minimum value is 16 pixels, and
* the maximum value is the value of
MaxHeight
.
* - integer percentage (%): The range of valid values is 0 to 100. Use
* the value of
Target
to specify whether you want Elastic
* Transcoder to include the black bars that are added by Elastic
* Transcoder, if any, in the calculation.
If you specify the
* value in pixels, it must be less than or equal to the value of
* MaxHeight
.
*
* Constraints:
* Pattern: (^\d{1,3}(\.\d{0,5})?%$)|(^\d{2,4}?px$)
*/
private String maxHeight;
/**
* A value that controls scaling of the watermark:
- Fit:
* Elastic Transcoder scales the watermark so it matches the value that
* you specified in either
MaxWidth
or
* MaxHeight
without exceeding the other value.
* - Stretch: Elastic Transcoder stretches the watermark to
* match the values that you specified for
MaxWidth
and
* MaxHeight
. If the relative proportions of the watermark
* and the values of MaxWidth
and MaxHeight
are
* different, the watermark will be distorted.
* - ShrinkToFit: Elastic Transcoder scales the watermark down
* so that its dimensions match the values that you specified for at
* least one of
MaxWidth
and MaxHeight
without
* exceeding either value. If you specify this option, Elastic Transcoder
* does not scale the watermark up.
*
* Constraints:
* Pattern: (^Fit$)|(^Stretch$)|(^ShrinkToFit$)
*/
private String sizingPolicy;
/**
* The horizontal position of the watermark unless you specify a non-zero
* value for HorizontalOffset
:
- Left: The
* left edge of the watermark is aligned with the left border of the
* video.
- Right: The right edge of the watermark is
* aligned with the right border of the video.
- Center:
* The watermark is centered between the left and right
* borders.
*
* Constraints:
* Pattern: (^Left$)|(^Right$)|(^Center$)
*/
private String horizontalAlign;
/**
* The amount by which you want the horizontal position of the watermark
* to be offset from the position specified by HorizontalAlign:
* - number of pixels (px): The minimum value is 0 pixels, and the
* maximum value is the value of MaxWidth.
- integer percentage
* (%): The range of valid values is 0 to 100.
For example, if
* you specify Left for HorizontalAlign
and 5px for
* HorizontalOffset
, the left side of the watermark appears
* 5 pixels from the left border of the output video.
* HorizontalOffset
is only valid when the value of
* HorizontalAlign
is Left
or
* Right
. If you specify an offset that causes the watermark
* to extend beyond the left or right border and Elastic Transcoder has
* not added black bars, the watermark is cropped. If Elastic Transcoder
* has added black bars, the watermark extends into the black bars. If
* the watermark extends beyond the black bars, it is cropped.
Use the
* value of Target
to specify whether you want to include
* the black bars that are added by Elastic Transcoder, if any, in the
* offset calculation.
*
* Constraints:
* Pattern: (^\d{1,3}(\.\d{0,5})?%$)|(^\d{2,4}?px$)
*/
private String horizontalOffset;
/**
* The vertical position of the watermark unless you specify a non-zero
* value for VerticalOffset
:
- Top: The top
* edge of the watermark is aligned with the top border of the
* video.
- Bottom: The bottom edge of the watermark is
* aligned with the bottom border of the video.
- Center:
* The watermark is centered between the top and bottom
* borders.
*
* Constraints:
* Pattern: (^Top$)|(^Bottom$)|(^Center$)
*/
private String verticalAlign;
/**
* VerticalOffset
The amount by which you want the
* vertical position of the watermark to be offset from the position
* specified by VerticalAlign:
- number of pixels (px): The minimum
* value is 0 pixels, and the maximum value is the value of
*
MaxHeight
. - integer percentage (%): The range of
* valid values is 0 to 100.
For example, if you specify
* Top
for VerticalAlign
and 5px
* for VerticalOffset
, the top of the watermark appears 5
* pixels from the top border of the output video.
* VerticalOffset
is only valid when the value of
* VerticalAlign is Top or Bottom.
If you specify an offset that
* causes the watermark to extend beyond the top or bottom border and
* Elastic Transcoder has not added black bars, the watermark is cropped.
* If Elastic Transcoder has added black bars, the watermark extends into
* the black bars. If the watermark extends beyond the black bars, it is
* cropped.
Use the value of Target
to specify whether
* you want Elastic Transcoder to include the black bars that are added
* by Elastic Transcoder, if any, in the offset calculation.
*
* Constraints:
* Pattern: (^\d{1,3}(\.\d{0,5})?%$)|(^\d{2,4}?px$)
*/
private String verticalOffset;
/**
* A percentage that indicates how much you want a watermark to obscure
* the video in the location where it appears. Valid values are 0 (the
* watermark is invisible) to 100 (the watermark completely obscures the
* video in the specified location). The datatype of Opacity
* is float.
Elastic Transcoder supports transparent .png graphics. If
* you use a transparent .png, the transparent portion of the video
* appears as if you had specified a value of 0 for Opacity
.
* The .jpg file format doesn't support transparency.
*
* Constraints:
* Pattern: ^\d{1,3}(\.\d{0,20})?$
*/
private String opacity;
/**
* A value that determines how Elastic Transcoder interprets values that
* you specified for HorizontalOffset
,
* VerticalOffset
, MaxWidth
, and
* MaxHeight
:
- Content:
*
HorizontalOffset
and VerticalOffset
values
* are calculated based on the borders of the video excluding black bars
* added by Elastic Transcoder, if any. In addition,
* MaxWidth
and MaxHeight
, if specified as a
* percentage, are calculated based on the borders of the video excluding
* black bars added by Elastic Transcoder, if any. - Frame:
*
HorizontalOffset
and VerticalOffset
values
* are calculated based on the borders of the video including black bars
* added by Elastic Transcoder, if any. In addition,
* MaxWidth
and MaxHeight
, if specified as a
* percentage, are calculated based on the borders of the video including
* black bars added by Elastic Transcoder, if any.
*
* Constraints:
* Pattern: (^Content$)|(^Frame$)
*/
private String target;
/**
* A unique identifier for the settings for one watermark. The value of
* Id
can be up to 40 characters long.
*
* Constraints:
* Length: 1 - 40
*
* @return A unique identifier for the settings for one watermark. The value of
* Id
can be up to 40 characters long.
*/
public String getId() {
return id;
}
/**
* A unique identifier for the settings for one watermark. The value of
* Id
can be up to 40 characters long.
*
* Constraints:
* Length: 1 - 40
*
* @param id A unique identifier for the settings for one watermark. The value of
* Id
can be up to 40 characters long.
*/
public void setId(String id) {
this.id = id;
}
/**
* A unique identifier for the settings for one watermark. The value of
* Id
can be up to 40 characters long.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 40
*
* @param id A unique identifier for the settings for one watermark. The value of
* Id
can be up to 40 characters long.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public PresetWatermark withId(String id) {
this.id = id;
return this;
}
/**
* The maximum width of the watermark in one of the following formats:
*
- number of pixels (px): The minimum value is 16 pixels, and
* the maximum value is the value of
MaxWidth
.
* - integer percentage (%): The range of valid values is 0 to 100. Use
* the value of
Target
to specify whether you want Elastic
* Transcoder to include the black bars that are added by Elastic
* Transcoder, if any, in the calculation. If you specify the value
* in pixels, it must be less than or equal to the value of
* MaxWidth
.
*
* Constraints:
* Pattern: (^\d{1,3}(\.\d{0,5})?%$)|(^\d{2,4}?px$)
*
* @return The maximum width of the watermark in one of the following formats:
*
- number of pixels (px): The minimum value is 16 pixels, and
* the maximum value is the value of
MaxWidth
.
* - integer percentage (%): The range of valid values is 0 to 100. Use
* the value of
Target
to specify whether you want Elastic
* Transcoder to include the black bars that are added by Elastic
* Transcoder, if any, in the calculation. If you specify the value
* in pixels, it must be less than or equal to the value of
* MaxWidth
.
*/
public String getMaxWidth() {
return maxWidth;
}
/**
* The maximum width of the watermark in one of the following formats:
* - number of pixels (px): The minimum value is 16 pixels, and
* the maximum value is the value of
MaxWidth
.
* - integer percentage (%): The range of valid values is 0 to 100. Use
* the value of
Target
to specify whether you want Elastic
* Transcoder to include the black bars that are added by Elastic
* Transcoder, if any, in the calculation. If you specify the value
* in pixels, it must be less than or equal to the value of
* MaxWidth
.
*
* Constraints:
* Pattern: (^\d{1,3}(\.\d{0,5})?%$)|(^\d{2,4}?px$)
*
* @param maxWidth The maximum width of the watermark in one of the following formats:
*
- number of pixels (px): The minimum value is 16 pixels, and
* the maximum value is the value of
MaxWidth
.
* - integer percentage (%): The range of valid values is 0 to 100. Use
* the value of
Target
to specify whether you want Elastic
* Transcoder to include the black bars that are added by Elastic
* Transcoder, if any, in the calculation. If you specify the value
* in pixels, it must be less than or equal to the value of
* MaxWidth
.
*/
public void setMaxWidth(String maxWidth) {
this.maxWidth = maxWidth;
}
/**
* The maximum width of the watermark in one of the following formats:
* - number of pixels (px): The minimum value is 16 pixels, and
* the maximum value is the value of
MaxWidth
.
* - integer percentage (%): The range of valid values is 0 to 100. Use
* the value of
Target
to specify whether you want Elastic
* Transcoder to include the black bars that are added by Elastic
* Transcoder, if any, in the calculation. If you specify the value
* in pixels, it must be less than or equal to the value of
* MaxWidth
.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Pattern: (^\d{1,3}(\.\d{0,5})?%$)|(^\d{2,4}?px$)
*
* @param maxWidth The maximum width of the watermark in one of the following formats:
*
- number of pixels (px): The minimum value is 16 pixels, and
* the maximum value is the value of
MaxWidth
.
* - integer percentage (%): The range of valid values is 0 to 100. Use
* the value of
Target
to specify whether you want Elastic
* Transcoder to include the black bars that are added by Elastic
* Transcoder, if any, in the calculation. If you specify the value
* in pixels, it must be less than or equal to the value of
* MaxWidth
.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public PresetWatermark withMaxWidth(String maxWidth) {
this.maxWidth = maxWidth;
return this;
}
/**
* The maximum height of the watermark in one of the following formats:
* - number of pixels (px): The minimum value is 16 pixels, and
* the maximum value is the value of
MaxHeight
.
* - integer percentage (%): The range of valid values is 0 to 100. Use
* the value of
Target
to specify whether you want Elastic
* Transcoder to include the black bars that are added by Elastic
* Transcoder, if any, in the calculation.
If you specify the
* value in pixels, it must be less than or equal to the value of
* MaxHeight
.
*
* Constraints:
* Pattern: (^\d{1,3}(\.\d{0,5})?%$)|(^\d{2,4}?px$)
*
* @return The maximum height of the watermark in one of the following formats:
*
- number of pixels (px): The minimum value is 16 pixels, and
* the maximum value is the value of
MaxHeight
.
* - integer percentage (%): The range of valid values is 0 to 100. Use
* the value of
Target
to specify whether you want Elastic
* Transcoder to include the black bars that are added by Elastic
* Transcoder, if any, in the calculation.
If you specify the
* value in pixels, it must be less than or equal to the value of
* MaxHeight
.
*/
public String getMaxHeight() {
return maxHeight;
}
/**
* The maximum height of the watermark in one of the following formats:
* - number of pixels (px): The minimum value is 16 pixels, and
* the maximum value is the value of
MaxHeight
.
* - integer percentage (%): The range of valid values is 0 to 100. Use
* the value of
Target
to specify whether you want Elastic
* Transcoder to include the black bars that are added by Elastic
* Transcoder, if any, in the calculation.
If you specify the
* value in pixels, it must be less than or equal to the value of
* MaxHeight
.
*
* Constraints:
* Pattern: (^\d{1,3}(\.\d{0,5})?%$)|(^\d{2,4}?px$)
*
* @param maxHeight The maximum height of the watermark in one of the following formats:
*
- number of pixels (px): The minimum value is 16 pixels, and
* the maximum value is the value of
MaxHeight
.
* - integer percentage (%): The range of valid values is 0 to 100. Use
* the value of
Target
to specify whether you want Elastic
* Transcoder to include the black bars that are added by Elastic
* Transcoder, if any, in the calculation.
If you specify the
* value in pixels, it must be less than or equal to the value of
* MaxHeight
.
*/
public void setMaxHeight(String maxHeight) {
this.maxHeight = maxHeight;
}
/**
* The maximum height of the watermark in one of the following formats:
* - number of pixels (px): The minimum value is 16 pixels, and
* the maximum value is the value of
MaxHeight
.
* - integer percentage (%): The range of valid values is 0 to 100. Use
* the value of
Target
to specify whether you want Elastic
* Transcoder to include the black bars that are added by Elastic
* Transcoder, if any, in the calculation.
If you specify the
* value in pixels, it must be less than or equal to the value of
* MaxHeight
.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Pattern: (^\d{1,3}(\.\d{0,5})?%$)|(^\d{2,4}?px$)
*
* @param maxHeight The maximum height of the watermark in one of the following formats:
*
- number of pixels (px): The minimum value is 16 pixels, and
* the maximum value is the value of
MaxHeight
.
* - integer percentage (%): The range of valid values is 0 to 100. Use
* the value of
Target
to specify whether you want Elastic
* Transcoder to include the black bars that are added by Elastic
* Transcoder, if any, in the calculation.
If you specify the
* value in pixels, it must be less than or equal to the value of
* MaxHeight
.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public PresetWatermark withMaxHeight(String maxHeight) {
this.maxHeight = maxHeight;
return this;
}
/**
* A value that controls scaling of the watermark: - Fit:
* Elastic Transcoder scales the watermark so it matches the value that
* you specified in either
MaxWidth
or
* MaxHeight
without exceeding the other value.
* - Stretch: Elastic Transcoder stretches the watermark to
* match the values that you specified for
MaxWidth
and
* MaxHeight
. If the relative proportions of the watermark
* and the values of MaxWidth
and MaxHeight
are
* different, the watermark will be distorted.
* - ShrinkToFit: Elastic Transcoder scales the watermark down
* so that its dimensions match the values that you specified for at
* least one of
MaxWidth
and MaxHeight
without
* exceeding either value. If you specify this option, Elastic Transcoder
* does not scale the watermark up.
*
* Constraints:
* Pattern: (^Fit$)|(^Stretch$)|(^ShrinkToFit$)
*
* @return A value that controls scaling of the watermark:
- Fit:
* Elastic Transcoder scales the watermark so it matches the value that
* you specified in either
MaxWidth
or
* MaxHeight
without exceeding the other value.
* - Stretch: Elastic Transcoder stretches the watermark to
* match the values that you specified for
MaxWidth
and
* MaxHeight
. If the relative proportions of the watermark
* and the values of MaxWidth
and MaxHeight
are
* different, the watermark will be distorted.
* - ShrinkToFit: Elastic Transcoder scales the watermark down
* so that its dimensions match the values that you specified for at
* least one of
MaxWidth
and MaxHeight
without
* exceeding either value. If you specify this option, Elastic Transcoder
* does not scale the watermark up.
*/
public String getSizingPolicy() {
return sizingPolicy;
}
/**
* A value that controls scaling of the watermark: - Fit:
* Elastic Transcoder scales the watermark so it matches the value that
* you specified in either
MaxWidth
or
* MaxHeight
without exceeding the other value.
* - Stretch: Elastic Transcoder stretches the watermark to
* match the values that you specified for
MaxWidth
and
* MaxHeight
. If the relative proportions of the watermark
* and the values of MaxWidth
and MaxHeight
are
* different, the watermark will be distorted.
* - ShrinkToFit: Elastic Transcoder scales the watermark down
* so that its dimensions match the values that you specified for at
* least one of
MaxWidth
and MaxHeight
without
* exceeding either value. If you specify this option, Elastic Transcoder
* does not scale the watermark up.
*
* Constraints:
* Pattern: (^Fit$)|(^Stretch$)|(^ShrinkToFit$)
*
* @param sizingPolicy A value that controls scaling of the watermark:
- Fit:
* Elastic Transcoder scales the watermark so it matches the value that
* you specified in either
MaxWidth
or
* MaxHeight
without exceeding the other value.
* - Stretch: Elastic Transcoder stretches the watermark to
* match the values that you specified for
MaxWidth
and
* MaxHeight
. If the relative proportions of the watermark
* and the values of MaxWidth
and MaxHeight
are
* different, the watermark will be distorted.
* - ShrinkToFit: Elastic Transcoder scales the watermark down
* so that its dimensions match the values that you specified for at
* least one of
MaxWidth
and MaxHeight
without
* exceeding either value. If you specify this option, Elastic Transcoder
* does not scale the watermark up.
*/
public void setSizingPolicy(String sizingPolicy) {
this.sizingPolicy = sizingPolicy;
}
/**
* A value that controls scaling of the watermark: - Fit:
* Elastic Transcoder scales the watermark so it matches the value that
* you specified in either
MaxWidth
or
* MaxHeight
without exceeding the other value.
* - Stretch: Elastic Transcoder stretches the watermark to
* match the values that you specified for
MaxWidth
and
* MaxHeight
. If the relative proportions of the watermark
* and the values of MaxWidth
and MaxHeight
are
* different, the watermark will be distorted.
* - ShrinkToFit: Elastic Transcoder scales the watermark down
* so that its dimensions match the values that you specified for at
* least one of
MaxWidth
and MaxHeight
without
* exceeding either value. If you specify this option, Elastic Transcoder
* does not scale the watermark up.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Pattern: (^Fit$)|(^Stretch$)|(^ShrinkToFit$)
*
* @param sizingPolicy A value that controls scaling of the watermark:
- Fit:
* Elastic Transcoder scales the watermark so it matches the value that
* you specified in either
MaxWidth
or
* MaxHeight
without exceeding the other value.
* - Stretch: Elastic Transcoder stretches the watermark to
* match the values that you specified for
MaxWidth
and
* MaxHeight
. If the relative proportions of the watermark
* and the values of MaxWidth
and MaxHeight
are
* different, the watermark will be distorted.
* - ShrinkToFit: Elastic Transcoder scales the watermark down
* so that its dimensions match the values that you specified for at
* least one of
MaxWidth
and MaxHeight
without
* exceeding either value. If you specify this option, Elastic Transcoder
* does not scale the watermark up.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public PresetWatermark withSizingPolicy(String sizingPolicy) {
this.sizingPolicy = sizingPolicy;
return this;
}
/**
* The horizontal position of the watermark unless you specify a non-zero
* value for HorizontalOffset
: - Left: The
* left edge of the watermark is aligned with the left border of the
* video.
- Right: The right edge of the watermark is
* aligned with the right border of the video.
- Center:
* The watermark is centered between the left and right
* borders.
*
* Constraints:
* Pattern: (^Left$)|(^Right$)|(^Center$)
*
* @return The horizontal position of the watermark unless you specify a non-zero
* value for HorizontalOffset
:
- Left: The
* left edge of the watermark is aligned with the left border of the
* video.
- Right: The right edge of the watermark is
* aligned with the right border of the video.
- Center:
* The watermark is centered between the left and right
* borders.
*/
public String getHorizontalAlign() {
return horizontalAlign;
}
/**
* The horizontal position of the watermark unless you specify a non-zero
* value for HorizontalOffset
: - Left: The
* left edge of the watermark is aligned with the left border of the
* video.
- Right: The right edge of the watermark is
* aligned with the right border of the video.
- Center:
* The watermark is centered between the left and right
* borders.
*
* Constraints:
* Pattern: (^Left$)|(^Right$)|(^Center$)
*
* @param horizontalAlign The horizontal position of the watermark unless you specify a non-zero
* value for HorizontalOffset
:
- Left: The
* left edge of the watermark is aligned with the left border of the
* video.
- Right: The right edge of the watermark is
* aligned with the right border of the video.
- Center:
* The watermark is centered between the left and right
* borders.
*/
public void setHorizontalAlign(String horizontalAlign) {
this.horizontalAlign = horizontalAlign;
}
/**
* The horizontal position of the watermark unless you specify a non-zero
* value for HorizontalOffset
: - Left: The
* left edge of the watermark is aligned with the left border of the
* video.
- Right: The right edge of the watermark is
* aligned with the right border of the video.
- Center:
* The watermark is centered between the left and right
* borders.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Pattern: (^Left$)|(^Right$)|(^Center$)
*
* @param horizontalAlign The horizontal position of the watermark unless you specify a non-zero
* value for HorizontalOffset
:
- Left: The
* left edge of the watermark is aligned with the left border of the
* video.
- Right: The right edge of the watermark is
* aligned with the right border of the video.
- Center:
* The watermark is centered between the left and right
* borders.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public PresetWatermark withHorizontalAlign(String horizontalAlign) {
this.horizontalAlign = horizontalAlign;
return this;
}
/**
* The amount by which you want the horizontal position of the watermark
* to be offset from the position specified by HorizontalAlign:
* - number of pixels (px): The minimum value is 0 pixels, and the
* maximum value is the value of MaxWidth.
- integer percentage
* (%): The range of valid values is 0 to 100.
For example, if
* you specify Left for HorizontalAlign
and 5px for
* HorizontalOffset
, the left side of the watermark appears
* 5 pixels from the left border of the output video.
* HorizontalOffset
is only valid when the value of
* HorizontalAlign
is Left
or
* Right
. If you specify an offset that causes the watermark
* to extend beyond the left or right border and Elastic Transcoder has
* not added black bars, the watermark is cropped. If Elastic Transcoder
* has added black bars, the watermark extends into the black bars. If
* the watermark extends beyond the black bars, it is cropped.
Use the
* value of Target
to specify whether you want to include
* the black bars that are added by Elastic Transcoder, if any, in the
* offset calculation.
*
* Constraints:
* Pattern: (^\d{1,3}(\.\d{0,5})?%$)|(^\d{2,4}?px$)
*
* @return The amount by which you want the horizontal position of the watermark
* to be offset from the position specified by HorizontalAlign:
* - number of pixels (px): The minimum value is 0 pixels, and the
* maximum value is the value of MaxWidth.
- integer percentage
* (%): The range of valid values is 0 to 100.
For example, if
* you specify Left for HorizontalAlign
and 5px for
* HorizontalOffset
, the left side of the watermark appears
* 5 pixels from the left border of the output video.
* HorizontalOffset
is only valid when the value of
* HorizontalAlign
is Left
or
* Right
. If you specify an offset that causes the watermark
* to extend beyond the left or right border and Elastic Transcoder has
* not added black bars, the watermark is cropped. If Elastic Transcoder
* has added black bars, the watermark extends into the black bars. If
* the watermark extends beyond the black bars, it is cropped.
Use the
* value of Target
to specify whether you want to include
* the black bars that are added by Elastic Transcoder, if any, in the
* offset calculation.
*/
public String getHorizontalOffset() {
return horizontalOffset;
}
/**
* The amount by which you want the horizontal position of the watermark
* to be offset from the position specified by HorizontalAlign:
* - number of pixels (px): The minimum value is 0 pixels, and the
* maximum value is the value of MaxWidth.
- integer percentage
* (%): The range of valid values is 0 to 100.
For example, if
* you specify Left for HorizontalAlign
and 5px for
* HorizontalOffset
, the left side of the watermark appears
* 5 pixels from the left border of the output video.
* HorizontalOffset
is only valid when the value of
* HorizontalAlign
is Left
or
* Right
. If you specify an offset that causes the watermark
* to extend beyond the left or right border and Elastic Transcoder has
* not added black bars, the watermark is cropped. If Elastic Transcoder
* has added black bars, the watermark extends into the black bars. If
* the watermark extends beyond the black bars, it is cropped.
Use the
* value of Target
to specify whether you want to include
* the black bars that are added by Elastic Transcoder, if any, in the
* offset calculation.
*
* Constraints:
* Pattern: (^\d{1,3}(\.\d{0,5})?%$)|(^\d{2,4}?px$)
*
* @param horizontalOffset The amount by which you want the horizontal position of the watermark
* to be offset from the position specified by HorizontalAlign:
* - number of pixels (px): The minimum value is 0 pixels, and the
* maximum value is the value of MaxWidth.
- integer percentage
* (%): The range of valid values is 0 to 100.
For example, if
* you specify Left for HorizontalAlign
and 5px for
* HorizontalOffset
, the left side of the watermark appears
* 5 pixels from the left border of the output video.
* HorizontalOffset
is only valid when the value of
* HorizontalAlign
is Left
or
* Right
. If you specify an offset that causes the watermark
* to extend beyond the left or right border and Elastic Transcoder has
* not added black bars, the watermark is cropped. If Elastic Transcoder
* has added black bars, the watermark extends into the black bars. If
* the watermark extends beyond the black bars, it is cropped.
Use the
* value of Target
to specify whether you want to include
* the black bars that are added by Elastic Transcoder, if any, in the
* offset calculation.
*/
public void setHorizontalOffset(String horizontalOffset) {
this.horizontalOffset = horizontalOffset;
}
/**
* The amount by which you want the horizontal position of the watermark
* to be offset from the position specified by HorizontalAlign:
* - number of pixels (px): The minimum value is 0 pixels, and the
* maximum value is the value of MaxWidth.
- integer percentage
* (%): The range of valid values is 0 to 100.
For example, if
* you specify Left for HorizontalAlign
and 5px for
* HorizontalOffset
, the left side of the watermark appears
* 5 pixels from the left border of the output video.
* HorizontalOffset
is only valid when the value of
* HorizontalAlign
is Left
or
* Right
. If you specify an offset that causes the watermark
* to extend beyond the left or right border and Elastic Transcoder has
* not added black bars, the watermark is cropped. If Elastic Transcoder
* has added black bars, the watermark extends into the black bars. If
* the watermark extends beyond the black bars, it is cropped.
Use the
* value of Target
to specify whether you want to include
* the black bars that are added by Elastic Transcoder, if any, in the
* offset calculation.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Pattern: (^\d{1,3}(\.\d{0,5})?%$)|(^\d{2,4}?px$)
*
* @param horizontalOffset The amount by which you want the horizontal position of the watermark
* to be offset from the position specified by HorizontalAlign:
* - number of pixels (px): The minimum value is 0 pixels, and the
* maximum value is the value of MaxWidth.
- integer percentage
* (%): The range of valid values is 0 to 100.
For example, if
* you specify Left for HorizontalAlign
and 5px for
* HorizontalOffset
, the left side of the watermark appears
* 5 pixels from the left border of the output video.
* HorizontalOffset
is only valid when the value of
* HorizontalAlign
is Left
or
* Right
. If you specify an offset that causes the watermark
* to extend beyond the left or right border and Elastic Transcoder has
* not added black bars, the watermark is cropped. If Elastic Transcoder
* has added black bars, the watermark extends into the black bars. If
* the watermark extends beyond the black bars, it is cropped.
Use the
* value of Target
to specify whether you want to include
* the black bars that are added by Elastic Transcoder, if any, in the
* offset calculation.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public PresetWatermark withHorizontalOffset(String horizontalOffset) {
this.horizontalOffset = horizontalOffset;
return this;
}
/**
* The vertical position of the watermark unless you specify a non-zero
* value for VerticalOffset
:
- Top: The top
* edge of the watermark is aligned with the top border of the
* video.
- Bottom: The bottom edge of the watermark is
* aligned with the bottom border of the video.
- Center:
* The watermark is centered between the top and bottom
* borders.
*
* Constraints:
* Pattern: (^Top$)|(^Bottom$)|(^Center$)
*
* @return The vertical position of the watermark unless you specify a non-zero
* value for VerticalOffset
:
- Top: The top
* edge of the watermark is aligned with the top border of the
* video.
- Bottom: The bottom edge of the watermark is
* aligned with the bottom border of the video.
- Center:
* The watermark is centered between the top and bottom
* borders.
*/
public String getVerticalAlign() {
return verticalAlign;
}
/**
* The vertical position of the watermark unless you specify a non-zero
* value for VerticalOffset
: - Top: The top
* edge of the watermark is aligned with the top border of the
* video.
- Bottom: The bottom edge of the watermark is
* aligned with the bottom border of the video.
- Center:
* The watermark is centered between the top and bottom
* borders.
*
* Constraints:
* Pattern: (^Top$)|(^Bottom$)|(^Center$)
*
* @param verticalAlign The vertical position of the watermark unless you specify a non-zero
* value for VerticalOffset
:
- Top: The top
* edge of the watermark is aligned with the top border of the
* video.
- Bottom: The bottom edge of the watermark is
* aligned with the bottom border of the video.
- Center:
* The watermark is centered between the top and bottom
* borders.
*/
public void setVerticalAlign(String verticalAlign) {
this.verticalAlign = verticalAlign;
}
/**
* The vertical position of the watermark unless you specify a non-zero
* value for VerticalOffset
: - Top: The top
* edge of the watermark is aligned with the top border of the
* video.
- Bottom: The bottom edge of the watermark is
* aligned with the bottom border of the video.
- Center:
* The watermark is centered between the top and bottom
* borders.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Pattern: (^Top$)|(^Bottom$)|(^Center$)
*
* @param verticalAlign The vertical position of the watermark unless you specify a non-zero
* value for VerticalOffset
:
- Top: The top
* edge of the watermark is aligned with the top border of the
* video.
- Bottom: The bottom edge of the watermark is
* aligned with the bottom border of the video.
- Center:
* The watermark is centered between the top and bottom
* borders.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public PresetWatermark withVerticalAlign(String verticalAlign) {
this.verticalAlign = verticalAlign;
return this;
}
/**
* VerticalOffset
The amount by which you want the
* vertical position of the watermark to be offset from the position
* specified by VerticalAlign:
- number of pixels (px): The minimum
* value is 0 pixels, and the maximum value is the value of
*
MaxHeight
. - integer percentage (%): The range of
* valid values is 0 to 100.
For example, if you specify
* Top
for VerticalAlign
and 5px
* for VerticalOffset
, the top of the watermark appears 5
* pixels from the top border of the output video.
* VerticalOffset
is only valid when the value of
* VerticalAlign is Top or Bottom.
If you specify an offset that
* causes the watermark to extend beyond the top or bottom border and
* Elastic Transcoder has not added black bars, the watermark is cropped.
* If Elastic Transcoder has added black bars, the watermark extends into
* the black bars. If the watermark extends beyond the black bars, it is
* cropped.
Use the value of Target
to specify whether
* you want Elastic Transcoder to include the black bars that are added
* by Elastic Transcoder, if any, in the offset calculation.
*
* Constraints:
* Pattern: (^\d{1,3}(\.\d{0,5})?%$)|(^\d{2,4}?px$)
*
* @return VerticalOffset
The amount by which you want the
* vertical position of the watermark to be offset from the position
* specified by VerticalAlign:
- number of pixels (px): The minimum
* value is 0 pixels, and the maximum value is the value of
*
MaxHeight
. - integer percentage (%): The range of
* valid values is 0 to 100.
For example, if you specify
* Top
for VerticalAlign
and 5px
* for VerticalOffset
, the top of the watermark appears 5
* pixels from the top border of the output video.
* VerticalOffset
is only valid when the value of
* VerticalAlign is Top or Bottom.
If you specify an offset that
* causes the watermark to extend beyond the top or bottom border and
* Elastic Transcoder has not added black bars, the watermark is cropped.
* If Elastic Transcoder has added black bars, the watermark extends into
* the black bars. If the watermark extends beyond the black bars, it is
* cropped.
Use the value of Target
to specify whether
* you want Elastic Transcoder to include the black bars that are added
* by Elastic Transcoder, if any, in the offset calculation.
*/
public String getVerticalOffset() {
return verticalOffset;
}
/**
* VerticalOffset
The amount by which you want the
* vertical position of the watermark to be offset from the position
* specified by VerticalAlign:
- number of pixels (px): The minimum
* value is 0 pixels, and the maximum value is the value of
*
MaxHeight
. - integer percentage (%): The range of
* valid values is 0 to 100.
For example, if you specify
* Top
for VerticalAlign
and 5px
* for VerticalOffset
, the top of the watermark appears 5
* pixels from the top border of the output video.
* VerticalOffset
is only valid when the value of
* VerticalAlign is Top or Bottom.
If you specify an offset that
* causes the watermark to extend beyond the top or bottom border and
* Elastic Transcoder has not added black bars, the watermark is cropped.
* If Elastic Transcoder has added black bars, the watermark extends into
* the black bars. If the watermark extends beyond the black bars, it is
* cropped.
Use the value of Target
to specify whether
* you want Elastic Transcoder to include the black bars that are added
* by Elastic Transcoder, if any, in the offset calculation.
*
* Constraints:
* Pattern: (^\d{1,3}(\.\d{0,5})?%$)|(^\d{2,4}?px$)
*
* @param verticalOffset VerticalOffset
The amount by which you want the
* vertical position of the watermark to be offset from the position
* specified by VerticalAlign:
- number of pixels (px): The minimum
* value is 0 pixels, and the maximum value is the value of
*
MaxHeight
. - integer percentage (%): The range of
* valid values is 0 to 100.
For example, if you specify
* Top
for VerticalAlign
and 5px
* for VerticalOffset
, the top of the watermark appears 5
* pixels from the top border of the output video.
* VerticalOffset
is only valid when the value of
* VerticalAlign is Top or Bottom.
If you specify an offset that
* causes the watermark to extend beyond the top or bottom border and
* Elastic Transcoder has not added black bars, the watermark is cropped.
* If Elastic Transcoder has added black bars, the watermark extends into
* the black bars. If the watermark extends beyond the black bars, it is
* cropped.
Use the value of Target
to specify whether
* you want Elastic Transcoder to include the black bars that are added
* by Elastic Transcoder, if any, in the offset calculation.
*/
public void setVerticalOffset(String verticalOffset) {
this.verticalOffset = verticalOffset;
}
/**
* VerticalOffset
The amount by which you want the
* vertical position of the watermark to be offset from the position
* specified by VerticalAlign:
- number of pixels (px): The minimum
* value is 0 pixels, and the maximum value is the value of
*
MaxHeight
. - integer percentage (%): The range of
* valid values is 0 to 100.
For example, if you specify
* Top
for VerticalAlign
and 5px
* for VerticalOffset
, the top of the watermark appears 5
* pixels from the top border of the output video.
* VerticalOffset
is only valid when the value of
* VerticalAlign is Top or Bottom.
If you specify an offset that
* causes the watermark to extend beyond the top or bottom border and
* Elastic Transcoder has not added black bars, the watermark is cropped.
* If Elastic Transcoder has added black bars, the watermark extends into
* the black bars. If the watermark extends beyond the black bars, it is
* cropped.
Use the value of Target
to specify whether
* you want Elastic Transcoder to include the black bars that are added
* by Elastic Transcoder, if any, in the offset calculation.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Pattern: (^\d{1,3}(\.\d{0,5})?%$)|(^\d{2,4}?px$)
*
* @param verticalOffset VerticalOffset
The amount by which you want the
* vertical position of the watermark to be offset from the position
* specified by VerticalAlign:
- number of pixels (px): The minimum
* value is 0 pixels, and the maximum value is the value of
*
MaxHeight
. - integer percentage (%): The range of
* valid values is 0 to 100.
For example, if you specify
* Top
for VerticalAlign
and 5px
* for VerticalOffset
, the top of the watermark appears 5
* pixels from the top border of the output video.
* VerticalOffset
is only valid when the value of
* VerticalAlign is Top or Bottom.
If you specify an offset that
* causes the watermark to extend beyond the top or bottom border and
* Elastic Transcoder has not added black bars, the watermark is cropped.
* If Elastic Transcoder has added black bars, the watermark extends into
* the black bars. If the watermark extends beyond the black bars, it is
* cropped.
Use the value of Target
to specify whether
* you want Elastic Transcoder to include the black bars that are added
* by Elastic Transcoder, if any, in the offset calculation.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public PresetWatermark withVerticalOffset(String verticalOffset) {
this.verticalOffset = verticalOffset;
return this;
}
/**
* A percentage that indicates how much you want a watermark to obscure
* the video in the location where it appears. Valid values are 0 (the
* watermark is invisible) to 100 (the watermark completely obscures the
* video in the specified location). The datatype of Opacity
* is float.
Elastic Transcoder supports transparent .png graphics. If
* you use a transparent .png, the transparent portion of the video
* appears as if you had specified a value of 0 for Opacity
.
* The .jpg file format doesn't support transparency.
*
* Constraints:
* Pattern: ^\d{1,3}(\.\d{0,20})?$
*
* @return A percentage that indicates how much you want a watermark to obscure
* the video in the location where it appears. Valid values are 0 (the
* watermark is invisible) to 100 (the watermark completely obscures the
* video in the specified location). The datatype of Opacity
* is float.
Elastic Transcoder supports transparent .png graphics. If
* you use a transparent .png, the transparent portion of the video
* appears as if you had specified a value of 0 for Opacity
.
* The .jpg file format doesn't support transparency.
*/
public String getOpacity() {
return opacity;
}
/**
* A percentage that indicates how much you want a watermark to obscure
* the video in the location where it appears. Valid values are 0 (the
* watermark is invisible) to 100 (the watermark completely obscures the
* video in the specified location). The datatype of Opacity
* is float.
Elastic Transcoder supports transparent .png graphics. If
* you use a transparent .png, the transparent portion of the video
* appears as if you had specified a value of 0 for Opacity
.
* The .jpg file format doesn't support transparency.
*
* Constraints:
* Pattern: ^\d{1,3}(\.\d{0,20})?$
*
* @param opacity A percentage that indicates how much you want a watermark to obscure
* the video in the location where it appears. Valid values are 0 (the
* watermark is invisible) to 100 (the watermark completely obscures the
* video in the specified location). The datatype of Opacity
* is float.
Elastic Transcoder supports transparent .png graphics. If
* you use a transparent .png, the transparent portion of the video
* appears as if you had specified a value of 0 for Opacity
.
* The .jpg file format doesn't support transparency.
*/
public void setOpacity(String opacity) {
this.opacity = opacity;
}
/**
* A percentage that indicates how much you want a watermark to obscure
* the video in the location where it appears. Valid values are 0 (the
* watermark is invisible) to 100 (the watermark completely obscures the
* video in the specified location). The datatype of Opacity
* is float.
Elastic Transcoder supports transparent .png graphics. If
* you use a transparent .png, the transparent portion of the video
* appears as if you had specified a value of 0 for Opacity
.
* The .jpg file format doesn't support transparency.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Pattern: ^\d{1,3}(\.\d{0,20})?$
*
* @param opacity A percentage that indicates how much you want a watermark to obscure
* the video in the location where it appears. Valid values are 0 (the
* watermark is invisible) to 100 (the watermark completely obscures the
* video in the specified location). The datatype of Opacity
* is float.
Elastic Transcoder supports transparent .png graphics. If
* you use a transparent .png, the transparent portion of the video
* appears as if you had specified a value of 0 for Opacity
.
* The .jpg file format doesn't support transparency.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public PresetWatermark withOpacity(String opacity) {
this.opacity = opacity;
return this;
}
/**
* A value that determines how Elastic Transcoder interprets values that
* you specified for HorizontalOffset
,
* VerticalOffset
, MaxWidth
, and
* MaxHeight
:
- Content:
*
HorizontalOffset
and VerticalOffset
values
* are calculated based on the borders of the video excluding black bars
* added by Elastic Transcoder, if any. In addition,
* MaxWidth
and MaxHeight
, if specified as a
* percentage, are calculated based on the borders of the video excluding
* black bars added by Elastic Transcoder, if any. - Frame:
*
HorizontalOffset
and VerticalOffset
values
* are calculated based on the borders of the video including black bars
* added by Elastic Transcoder, if any. In addition,
* MaxWidth
and MaxHeight
, if specified as a
* percentage, are calculated based on the borders of the video including
* black bars added by Elastic Transcoder, if any.
*
* Constraints:
* Pattern: (^Content$)|(^Frame$)
*
* @return A value that determines how Elastic Transcoder interprets values that
* you specified for HorizontalOffset
,
* VerticalOffset
, MaxWidth
, and
* MaxHeight
:
- Content:
*
HorizontalOffset
and VerticalOffset
values
* are calculated based on the borders of the video excluding black bars
* added by Elastic Transcoder, if any. In addition,
* MaxWidth
and MaxHeight
, if specified as a
* percentage, are calculated based on the borders of the video excluding
* black bars added by Elastic Transcoder, if any. - Frame:
*
HorizontalOffset
and VerticalOffset
values
* are calculated based on the borders of the video including black bars
* added by Elastic Transcoder, if any. In addition,
* MaxWidth
and MaxHeight
, if specified as a
* percentage, are calculated based on the borders of the video including
* black bars added by Elastic Transcoder, if any.
*/
public String getTarget() {
return target;
}
/**
* A value that determines how Elastic Transcoder interprets values that
* you specified for HorizontalOffset
,
* VerticalOffset
, MaxWidth
, and
* MaxHeight
: - Content:
*
HorizontalOffset
and VerticalOffset
values
* are calculated based on the borders of the video excluding black bars
* added by Elastic Transcoder, if any. In addition,
* MaxWidth
and MaxHeight
, if specified as a
* percentage, are calculated based on the borders of the video excluding
* black bars added by Elastic Transcoder, if any. - Frame:
*
HorizontalOffset
and VerticalOffset
values
* are calculated based on the borders of the video including black bars
* added by Elastic Transcoder, if any. In addition,
* MaxWidth
and MaxHeight
, if specified as a
* percentage, are calculated based on the borders of the video including
* black bars added by Elastic Transcoder, if any.
*
* Constraints:
* Pattern: (^Content$)|(^Frame$)
*
* @param target A value that determines how Elastic Transcoder interprets values that
* you specified for HorizontalOffset
,
* VerticalOffset
, MaxWidth
, and
* MaxHeight
:
- Content:
*
HorizontalOffset
and VerticalOffset
values
* are calculated based on the borders of the video excluding black bars
* added by Elastic Transcoder, if any. In addition,
* MaxWidth
and MaxHeight
, if specified as a
* percentage, are calculated based on the borders of the video excluding
* black bars added by Elastic Transcoder, if any. - Frame:
*
HorizontalOffset
and VerticalOffset
values
* are calculated based on the borders of the video including black bars
* added by Elastic Transcoder, if any. In addition,
* MaxWidth
and MaxHeight
, if specified as a
* percentage, are calculated based on the borders of the video including
* black bars added by Elastic Transcoder, if any.
*/
public void setTarget(String target) {
this.target = target;
}
/**
* A value that determines how Elastic Transcoder interprets values that
* you specified for HorizontalOffset
,
* VerticalOffset
, MaxWidth
, and
* MaxHeight
: - Content:
*
HorizontalOffset
and VerticalOffset
values
* are calculated based on the borders of the video excluding black bars
* added by Elastic Transcoder, if any. In addition,
* MaxWidth
and MaxHeight
, if specified as a
* percentage, are calculated based on the borders of the video excluding
* black bars added by Elastic Transcoder, if any. - Frame:
*
HorizontalOffset
and VerticalOffset
values
* are calculated based on the borders of the video including black bars
* added by Elastic Transcoder, if any. In addition,
* MaxWidth
and MaxHeight
, if specified as a
* percentage, are calculated based on the borders of the video including
* black bars added by Elastic Transcoder, if any.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Pattern: (^Content$)|(^Frame$)
*
* @param target A value that determines how Elastic Transcoder interprets values that
* you specified for HorizontalOffset
,
* VerticalOffset
, MaxWidth
, and
* MaxHeight
:
- Content:
*
HorizontalOffset
and VerticalOffset
values
* are calculated based on the borders of the video excluding black bars
* added by Elastic Transcoder, if any. In addition,
* MaxWidth
and MaxHeight
, if specified as a
* percentage, are calculated based on the borders of the video excluding
* black bars added by Elastic Transcoder, if any. - Frame:
*
HorizontalOffset
and VerticalOffset
values
* are calculated based on the borders of the video including black bars
* added by Elastic Transcoder, if any. In addition,
* MaxWidth
and MaxHeight
, if specified as a
* percentage, are calculated based on the borders of the video including
* black bars added by Elastic Transcoder, if any.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public PresetWatermark withTarget(String target) {
this.target = target;
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getId() != null) sb.append("Id: " + getId() + ",");
if (getMaxWidth() != null) sb.append("MaxWidth: " + getMaxWidth() + ",");
if (getMaxHeight() != null) sb.append("MaxHeight: " + getMaxHeight() + ",");
if (getSizingPolicy() != null) sb.append("SizingPolicy: " + getSizingPolicy() + ",");
if (getHorizontalAlign() != null) sb.append("HorizontalAlign: " + getHorizontalAlign() + ",");
if (getHorizontalOffset() != null) sb.append("HorizontalOffset: " + getHorizontalOffset() + ",");
if (getVerticalAlign() != null) sb.append("VerticalAlign: " + getVerticalAlign() + ",");
if (getVerticalOffset() != null) sb.append("VerticalOffset: " + getVerticalOffset() + ",");
if (getOpacity() != null) sb.append("Opacity: " + getOpacity() + ",");
if (getTarget() != null) sb.append("Target: " + getTarget() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getId() == null) ? 0 : getId().hashCode());
hashCode = prime * hashCode + ((getMaxWidth() == null) ? 0 : getMaxWidth().hashCode());
hashCode = prime * hashCode + ((getMaxHeight() == null) ? 0 : getMaxHeight().hashCode());
hashCode = prime * hashCode + ((getSizingPolicy() == null) ? 0 : getSizingPolicy().hashCode());
hashCode = prime * hashCode + ((getHorizontalAlign() == null) ? 0 : getHorizontalAlign().hashCode());
hashCode = prime * hashCode + ((getHorizontalOffset() == null) ? 0 : getHorizontalOffset().hashCode());
hashCode = prime * hashCode + ((getVerticalAlign() == null) ? 0 : getVerticalAlign().hashCode());
hashCode = prime * hashCode + ((getVerticalOffset() == null) ? 0 : getVerticalOffset().hashCode());
hashCode = prime * hashCode + ((getOpacity() == null) ? 0 : getOpacity().hashCode());
hashCode = prime * hashCode + ((getTarget() == null) ? 0 : getTarget().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof PresetWatermark == false) return false;
PresetWatermark other = (PresetWatermark)obj;
if (other.getId() == null ^ this.getId() == null) return false;
if (other.getId() != null && other.getId().equals(this.getId()) == false) return false;
if (other.getMaxWidth() == null ^ this.getMaxWidth() == null) return false;
if (other.getMaxWidth() != null && other.getMaxWidth().equals(this.getMaxWidth()) == false) return false;
if (other.getMaxHeight() == null ^ this.getMaxHeight() == null) return false;
if (other.getMaxHeight() != null && other.getMaxHeight().equals(this.getMaxHeight()) == false) return false;
if (other.getSizingPolicy() == null ^ this.getSizingPolicy() == null) return false;
if (other.getSizingPolicy() != null && other.getSizingPolicy().equals(this.getSizingPolicy()) == false) return false;
if (other.getHorizontalAlign() == null ^ this.getHorizontalAlign() == null) return false;
if (other.getHorizontalAlign() != null && other.getHorizontalAlign().equals(this.getHorizontalAlign()) == false) return false;
if (other.getHorizontalOffset() == null ^ this.getHorizontalOffset() == null) return false;
if (other.getHorizontalOffset() != null && other.getHorizontalOffset().equals(this.getHorizontalOffset()) == false) return false;
if (other.getVerticalAlign() == null ^ this.getVerticalAlign() == null) return false;
if (other.getVerticalAlign() != null && other.getVerticalAlign().equals(this.getVerticalAlign()) == false) return false;
if (other.getVerticalOffset() == null ^ this.getVerticalOffset() == null) return false;
if (other.getVerticalOffset() != null && other.getVerticalOffset().equals(this.getVerticalOffset()) == false) return false;
if (other.getOpacity() == null ^ this.getOpacity() == null) return false;
if (other.getOpacity() != null && other.getOpacity().equals(this.getOpacity()) == false) return false;
if (other.getTarget() == null ^ this.getTarget() == null) return false;
if (other.getTarget() != null && other.getTarget().equals(this.getTarget()) == false) return false;
return true;
}
}