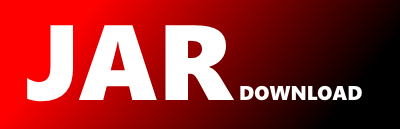
com.amazonaws.services.elastictranscoder.model.UpdatePipelineNotificationsRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.elastictranscoder.model;
import java.io.Serializable;
import com.amazonaws.AmazonWebServiceRequest;
/**
* Container for the parameters to the {@link com.amazonaws.services.elastictranscoder.AmazonElasticTranscoder#updatePipelineNotifications(UpdatePipelineNotificationsRequest) UpdatePipelineNotifications operation}.
*
* With the UpdatePipelineNotifications operation, you can update Amazon
* Simple Notification Service (Amazon SNS) notifications for a pipeline.
*
*
* When you update notifications for a pipeline, Elastic Transcoder
* returns the values that you specified in the request.
*
*
* @see com.amazonaws.services.elastictranscoder.AmazonElasticTranscoder#updatePipelineNotifications(UpdatePipelineNotificationsRequest)
*/
public class UpdatePipelineNotificationsRequest extends AmazonWebServiceRequest implements Serializable {
/**
* The identifier of the pipeline for which you want to change
* notification settings.
*
* Constraints:
* Pattern: ^\d{13}-\w{6}$
*/
private String id;
/**
* The topic ARN for the Amazon Simple Notification Service (Amazon SNS)
* topic that you want to notify to report job status. To
* receive notifications, you must also subscribe to the new topic in the
* Amazon SNS console.
- Progressing: The topic
* ARN for the Amazon Simple Notification Service (Amazon SNS) topic that
* you want to notify when Elastic Transcoder has started to process jobs
* that are added to this pipeline. This is the ARN that Amazon SNS
* returned when you created the topic.
- Completed: The
* topic ARN for the Amazon SNS topic that you want to notify when
* Elastic Transcoder has finished processing a job. This is the ARN that
* Amazon SNS returned when you created the topic.
* - Warning: The topic ARN for the Amazon SNS topic that you
* want to notify when Elastic Transcoder encounters a warning condition.
* This is the ARN that Amazon SNS returned when you created the
* topic.
- Error: The topic ARN for the Amazon SNS topic
* that you want to notify when Elastic Transcoder encounters an error
* condition. This is the ARN that Amazon SNS returned when you created
* the topic.
*/
private Notifications notifications;
/**
* The identifier of the pipeline for which you want to change
* notification settings.
*
* Constraints:
* Pattern: ^\d{13}-\w{6}$
*
* @return The identifier of the pipeline for which you want to change
* notification settings.
*/
public String getId() {
return id;
}
/**
* The identifier of the pipeline for which you want to change
* notification settings.
*
* Constraints:
* Pattern: ^\d{13}-\w{6}$
*
* @param id The identifier of the pipeline for which you want to change
* notification settings.
*/
public void setId(String id) {
this.id = id;
}
/**
* The identifier of the pipeline for which you want to change
* notification settings.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Pattern: ^\d{13}-\w{6}$
*
* @param id The identifier of the pipeline for which you want to change
* notification settings.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public UpdatePipelineNotificationsRequest withId(String id) {
this.id = id;
return this;
}
/**
* The topic ARN for the Amazon Simple Notification Service (Amazon SNS)
* topic that you want to notify to report job status. To
* receive notifications, you must also subscribe to the new topic in the
* Amazon SNS console.
- Progressing: The topic
* ARN for the Amazon Simple Notification Service (Amazon SNS) topic that
* you want to notify when Elastic Transcoder has started to process jobs
* that are added to this pipeline. This is the ARN that Amazon SNS
* returned when you created the topic.
- Completed: The
* topic ARN for the Amazon SNS topic that you want to notify when
* Elastic Transcoder has finished processing a job. This is the ARN that
* Amazon SNS returned when you created the topic.
* - Warning: The topic ARN for the Amazon SNS topic that you
* want to notify when Elastic Transcoder encounters a warning condition.
* This is the ARN that Amazon SNS returned when you created the
* topic.
- Error: The topic ARN for the Amazon SNS topic
* that you want to notify when Elastic Transcoder encounters an error
* condition. This is the ARN that Amazon SNS returned when you created
* the topic.
*
* @return The topic ARN for the Amazon Simple Notification Service (Amazon SNS)
* topic that you want to notify to report job status. To
* receive notifications, you must also subscribe to the new topic in the
* Amazon SNS console. - Progressing: The topic
* ARN for the Amazon Simple Notification Service (Amazon SNS) topic that
* you want to notify when Elastic Transcoder has started to process jobs
* that are added to this pipeline. This is the ARN that Amazon SNS
* returned when you created the topic.
- Completed: The
* topic ARN for the Amazon SNS topic that you want to notify when
* Elastic Transcoder has finished processing a job. This is the ARN that
* Amazon SNS returned when you created the topic.
* - Warning: The topic ARN for the Amazon SNS topic that you
* want to notify when Elastic Transcoder encounters a warning condition.
* This is the ARN that Amazon SNS returned when you created the
* topic.
- Error: The topic ARN for the Amazon SNS topic
* that you want to notify when Elastic Transcoder encounters an error
* condition. This is the ARN that Amazon SNS returned when you created
* the topic.
*/
public Notifications getNotifications() {
return notifications;
}
/**
* The topic ARN for the Amazon Simple Notification Service (Amazon SNS)
* topic that you want to notify to report job status. To
* receive notifications, you must also subscribe to the new topic in the
* Amazon SNS console. - Progressing: The topic
* ARN for the Amazon Simple Notification Service (Amazon SNS) topic that
* you want to notify when Elastic Transcoder has started to process jobs
* that are added to this pipeline. This is the ARN that Amazon SNS
* returned when you created the topic.
- Completed: The
* topic ARN for the Amazon SNS topic that you want to notify when
* Elastic Transcoder has finished processing a job. This is the ARN that
* Amazon SNS returned when you created the topic.
* - Warning: The topic ARN for the Amazon SNS topic that you
* want to notify when Elastic Transcoder encounters a warning condition.
* This is the ARN that Amazon SNS returned when you created the
* topic.
- Error: The topic ARN for the Amazon SNS topic
* that you want to notify when Elastic Transcoder encounters an error
* condition. This is the ARN that Amazon SNS returned when you created
* the topic.
*
* @param notifications The topic ARN for the Amazon Simple Notification Service (Amazon SNS)
* topic that you want to notify to report job status. To
* receive notifications, you must also subscribe to the new topic in the
* Amazon SNS console. - Progressing: The topic
* ARN for the Amazon Simple Notification Service (Amazon SNS) topic that
* you want to notify when Elastic Transcoder has started to process jobs
* that are added to this pipeline. This is the ARN that Amazon SNS
* returned when you created the topic.
- Completed: The
* topic ARN for the Amazon SNS topic that you want to notify when
* Elastic Transcoder has finished processing a job. This is the ARN that
* Amazon SNS returned when you created the topic.
* - Warning: The topic ARN for the Amazon SNS topic that you
* want to notify when Elastic Transcoder encounters a warning condition.
* This is the ARN that Amazon SNS returned when you created the
* topic.
- Error: The topic ARN for the Amazon SNS topic
* that you want to notify when Elastic Transcoder encounters an error
* condition. This is the ARN that Amazon SNS returned when you created
* the topic.
*/
public void setNotifications(Notifications notifications) {
this.notifications = notifications;
}
/**
* The topic ARN for the Amazon Simple Notification Service (Amazon SNS)
* topic that you want to notify to report job status. To
* receive notifications, you must also subscribe to the new topic in the
* Amazon SNS console. - Progressing: The topic
* ARN for the Amazon Simple Notification Service (Amazon SNS) topic that
* you want to notify when Elastic Transcoder has started to process jobs
* that are added to this pipeline. This is the ARN that Amazon SNS
* returned when you created the topic.
- Completed: The
* topic ARN for the Amazon SNS topic that you want to notify when
* Elastic Transcoder has finished processing a job. This is the ARN that
* Amazon SNS returned when you created the topic.
* - Warning: The topic ARN for the Amazon SNS topic that you
* want to notify when Elastic Transcoder encounters a warning condition.
* This is the ARN that Amazon SNS returned when you created the
* topic.
- Error: The topic ARN for the Amazon SNS topic
* that you want to notify when Elastic Transcoder encounters an error
* condition. This is the ARN that Amazon SNS returned when you created
* the topic.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param notifications The topic ARN for the Amazon Simple Notification Service (Amazon SNS)
* topic that you want to notify to report job status. To
* receive notifications, you must also subscribe to the new topic in the
* Amazon SNS console.
- Progressing: The topic
* ARN for the Amazon Simple Notification Service (Amazon SNS) topic that
* you want to notify when Elastic Transcoder has started to process jobs
* that are added to this pipeline. This is the ARN that Amazon SNS
* returned when you created the topic.
- Completed: The
* topic ARN for the Amazon SNS topic that you want to notify when
* Elastic Transcoder has finished processing a job. This is the ARN that
* Amazon SNS returned when you created the topic.
* - Warning: The topic ARN for the Amazon SNS topic that you
* want to notify when Elastic Transcoder encounters a warning condition.
* This is the ARN that Amazon SNS returned when you created the
* topic.
- Error: The topic ARN for the Amazon SNS topic
* that you want to notify when Elastic Transcoder encounters an error
* condition. This is the ARN that Amazon SNS returned when you created
* the topic.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public UpdatePipelineNotificationsRequest withNotifications(Notifications notifications) {
this.notifications = notifications;
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getId() != null) sb.append("Id: " + getId() + ",");
if (getNotifications() != null) sb.append("Notifications: " + getNotifications() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getId() == null) ? 0 : getId().hashCode());
hashCode = prime * hashCode + ((getNotifications() == null) ? 0 : getNotifications().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof UpdatePipelineNotificationsRequest == false) return false;
UpdatePipelineNotificationsRequest other = (UpdatePipelineNotificationsRequest)obj;
if (other.getId() == null ^ this.getId() == null) return false;
if (other.getId() != null && other.getId().equals(this.getId()) == false) return false;
if (other.getNotifications() == null ^ this.getNotifications() == null) return false;
if (other.getNotifications() != null && other.getNotifications().equals(this.getNotifications()) == false) return false;
return true;
}
}