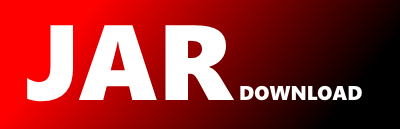
com.amazonaws.services.glacier.model.GlacierJobDescription Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.glacier.model;
import java.io.Serializable;
/**
*
* Describes an Amazon Glacier job.
*
*/
public class GlacierJobDescription implements Serializable {
/**
* An opaque string that identifies an Amazon Glacier job.
*/
private String jobId;
/**
* The job description you provided when you initiated the job.
*/
private String jobDescription;
/**
* The job type. It is either ArchiveRetrieval or InventoryRetrieval.
*
* Constraints:
* Allowed Values: ArchiveRetrieval, InventoryRetrieval
*/
private String action;
/**
* For an ArchiveRetrieval job, this is the archive ID requested for
* download. Otherwise, this field is null.
*/
private String archiveId;
/**
* The Amazon Resource Name (ARN) of the vault from which the archive
* retrieval was requested.
*/
private String vaultARN;
/**
* The UTC date when the job was created. A string representation of ISO
* 8601 date format, for example, "2012-03-20T17:03:43.221Z".
*/
private String creationDate;
/**
* The job status. When a job is completed, you get the job's output.
*/
private Boolean completed;
/**
* The status code can be InProgress, Succeeded, or Failed, and indicates
* the status of the job.
*
* Constraints:
* Allowed Values: InProgress, Succeeded, Failed
*/
private String statusCode;
/**
* A friendly message that describes the job status.
*/
private String statusMessage;
/**
* For an ArchiveRetrieval job, this is the size in bytes of the archive
* being requested for download. For the InventoryRetrieval job, the
* value is null.
*/
private Long archiveSizeInBytes;
/**
* For an InventoryRetrieval job, this is the size in bytes of the
* inventory requested for download. For the ArchiveRetrieval job, the
* value is null.
*/
private Long inventorySizeInBytes;
/**
* An Amazon Simple Notification Service (Amazon SNS) topic that receives
* notification.
*/
private String sNSTopic;
/**
* The UTC time that the archive retrieval request completed. While the
* job is in progress, the value will be null.
*/
private String completionDate;
/**
* For an ArchiveRetrieval job, it is the checksum of the archive.
* Otherwise, the value is null.
The SHA256 tree hash value for the
* requested range of an archive. If the Initiate a Job request for an
* archive specified a tree-hash aligned range, then this field returns a
* value.
For the specific case when the whole archive is retrieved,
* this value is the same as the ArchiveSHA256TreeHash value.
This
* field is null in the following situations:
Archive
* retrieval jobs that specify a range that is not tree-hash
* aligned.
Archival jobs that specify a range
* that is equal to the whole archive and the job status is
* InProgress.
Inventory jobs.
*/
private String sHA256TreeHash;
/**
* The SHA256 tree hash of the entire archive for an archive retrieval.
* For inventory retrieval jobs, this field is null.
*/
private String archiveSHA256TreeHash;
/**
* The retrieved byte range for archive retrieval jobs in the form
* "StartByteValue-EndByteValue" If no range was specified
* in the archive retrieval, then the whole archive is retrieved and
* StartByteValue equals 0 and EndByteValue equals the size
* of the archive minus 1. For inventory retrieval jobs this field is
* null.
*/
private String retrievalByteRange;
/**
* Parameters used for range inventory retrieval.
*/
private InventoryRetrievalJobDescription inventoryRetrievalParameters;
/**
* An opaque string that identifies an Amazon Glacier job.
*
* @return An opaque string that identifies an Amazon Glacier job.
*/
public String getJobId() {
return jobId;
}
/**
* An opaque string that identifies an Amazon Glacier job.
*
* @param jobId An opaque string that identifies an Amazon Glacier job.
*/
public void setJobId(String jobId) {
this.jobId = jobId;
}
/**
* An opaque string that identifies an Amazon Glacier job.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param jobId An opaque string that identifies an Amazon Glacier job.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public GlacierJobDescription withJobId(String jobId) {
this.jobId = jobId;
return this;
}
/**
* The job description you provided when you initiated the job.
*
* @return The job description you provided when you initiated the job.
*/
public String getJobDescription() {
return jobDescription;
}
/**
* The job description you provided when you initiated the job.
*
* @param jobDescription The job description you provided when you initiated the job.
*/
public void setJobDescription(String jobDescription) {
this.jobDescription = jobDescription;
}
/**
* The job description you provided when you initiated the job.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param jobDescription The job description you provided when you initiated the job.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public GlacierJobDescription withJobDescription(String jobDescription) {
this.jobDescription = jobDescription;
return this;
}
/**
* The job type. It is either ArchiveRetrieval or InventoryRetrieval.
*
* Constraints:
* Allowed Values: ArchiveRetrieval, InventoryRetrieval
*
* @return The job type. It is either ArchiveRetrieval or InventoryRetrieval.
*
* @see ActionCode
*/
public String getAction() {
return action;
}
/**
* The job type. It is either ArchiveRetrieval or InventoryRetrieval.
*
* Constraints:
* Allowed Values: ArchiveRetrieval, InventoryRetrieval
*
* @param action The job type. It is either ArchiveRetrieval or InventoryRetrieval.
*
* @see ActionCode
*/
public void setAction(String action) {
this.action = action;
}
/**
* The job type. It is either ArchiveRetrieval or InventoryRetrieval.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: ArchiveRetrieval, InventoryRetrieval
*
* @param action The job type. It is either ArchiveRetrieval or InventoryRetrieval.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see ActionCode
*/
public GlacierJobDescription withAction(String action) {
this.action = action;
return this;
}
/**
* The job type. It is either ArchiveRetrieval or InventoryRetrieval.
*
* Constraints:
* Allowed Values: ArchiveRetrieval, InventoryRetrieval
*
* @param action The job type. It is either ArchiveRetrieval or InventoryRetrieval.
*
* @see ActionCode
*/
public void setAction(ActionCode action) {
this.action = action.toString();
}
/**
* The job type. It is either ArchiveRetrieval or InventoryRetrieval.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: ArchiveRetrieval, InventoryRetrieval
*
* @param action The job type. It is either ArchiveRetrieval or InventoryRetrieval.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see ActionCode
*/
public GlacierJobDescription withAction(ActionCode action) {
this.action = action.toString();
return this;
}
/**
* For an ArchiveRetrieval job, this is the archive ID requested for
* download. Otherwise, this field is null.
*
* @return For an ArchiveRetrieval job, this is the archive ID requested for
* download. Otherwise, this field is null.
*/
public String getArchiveId() {
return archiveId;
}
/**
* For an ArchiveRetrieval job, this is the archive ID requested for
* download. Otherwise, this field is null.
*
* @param archiveId For an ArchiveRetrieval job, this is the archive ID requested for
* download. Otherwise, this field is null.
*/
public void setArchiveId(String archiveId) {
this.archiveId = archiveId;
}
/**
* For an ArchiveRetrieval job, this is the archive ID requested for
* download. Otherwise, this field is null.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param archiveId For an ArchiveRetrieval job, this is the archive ID requested for
* download. Otherwise, this field is null.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public GlacierJobDescription withArchiveId(String archiveId) {
this.archiveId = archiveId;
return this;
}
/**
* The Amazon Resource Name (ARN) of the vault from which the archive
* retrieval was requested.
*
* @return The Amazon Resource Name (ARN) of the vault from which the archive
* retrieval was requested.
*/
public String getVaultARN() {
return vaultARN;
}
/**
* The Amazon Resource Name (ARN) of the vault from which the archive
* retrieval was requested.
*
* @param vaultARN The Amazon Resource Name (ARN) of the vault from which the archive
* retrieval was requested.
*/
public void setVaultARN(String vaultARN) {
this.vaultARN = vaultARN;
}
/**
* The Amazon Resource Name (ARN) of the vault from which the archive
* retrieval was requested.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param vaultARN The Amazon Resource Name (ARN) of the vault from which the archive
* retrieval was requested.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public GlacierJobDescription withVaultARN(String vaultARN) {
this.vaultARN = vaultARN;
return this;
}
/**
* The UTC date when the job was created. A string representation of ISO
* 8601 date format, for example, "2012-03-20T17:03:43.221Z".
*
* @return The UTC date when the job was created. A string representation of ISO
* 8601 date format, for example, "2012-03-20T17:03:43.221Z".
*/
public String getCreationDate() {
return creationDate;
}
/**
* The UTC date when the job was created. A string representation of ISO
* 8601 date format, for example, "2012-03-20T17:03:43.221Z".
*
* @param creationDate The UTC date when the job was created. A string representation of ISO
* 8601 date format, for example, "2012-03-20T17:03:43.221Z".
*/
public void setCreationDate(String creationDate) {
this.creationDate = creationDate;
}
/**
* The UTC date when the job was created. A string representation of ISO
* 8601 date format, for example, "2012-03-20T17:03:43.221Z".
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param creationDate The UTC date when the job was created. A string representation of ISO
* 8601 date format, for example, "2012-03-20T17:03:43.221Z".
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public GlacierJobDescription withCreationDate(String creationDate) {
this.creationDate = creationDate;
return this;
}
/**
* The job status. When a job is completed, you get the job's output.
*
* @return The job status. When a job is completed, you get the job's output.
*/
public Boolean isCompleted() {
return completed;
}
/**
* The job status. When a job is completed, you get the job's output.
*
* @param completed The job status. When a job is completed, you get the job's output.
*/
public void setCompleted(Boolean completed) {
this.completed = completed;
}
/**
* The job status. When a job is completed, you get the job's output.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param completed The job status. When a job is completed, you get the job's output.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public GlacierJobDescription withCompleted(Boolean completed) {
this.completed = completed;
return this;
}
/**
* The job status. When a job is completed, you get the job's output.
*
* @return The job status. When a job is completed, you get the job's output.
*/
public Boolean getCompleted() {
return completed;
}
/**
* The status code can be InProgress, Succeeded, or Failed, and indicates
* the status of the job.
*
* Constraints:
* Allowed Values: InProgress, Succeeded, Failed
*
* @return The status code can be InProgress, Succeeded, or Failed, and indicates
* the status of the job.
*
* @see StatusCode
*/
public String getStatusCode() {
return statusCode;
}
/**
* The status code can be InProgress, Succeeded, or Failed, and indicates
* the status of the job.
*
* Constraints:
* Allowed Values: InProgress, Succeeded, Failed
*
* @param statusCode The status code can be InProgress, Succeeded, or Failed, and indicates
* the status of the job.
*
* @see StatusCode
*/
public void setStatusCode(String statusCode) {
this.statusCode = statusCode;
}
/**
* The status code can be InProgress, Succeeded, or Failed, and indicates
* the status of the job.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: InProgress, Succeeded, Failed
*
* @param statusCode The status code can be InProgress, Succeeded, or Failed, and indicates
* the status of the job.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see StatusCode
*/
public GlacierJobDescription withStatusCode(String statusCode) {
this.statusCode = statusCode;
return this;
}
/**
* The status code can be InProgress, Succeeded, or Failed, and indicates
* the status of the job.
*
* Constraints:
* Allowed Values: InProgress, Succeeded, Failed
*
* @param statusCode The status code can be InProgress, Succeeded, or Failed, and indicates
* the status of the job.
*
* @see StatusCode
*/
public void setStatusCode(StatusCode statusCode) {
this.statusCode = statusCode.toString();
}
/**
* The status code can be InProgress, Succeeded, or Failed, and indicates
* the status of the job.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: InProgress, Succeeded, Failed
*
* @param statusCode The status code can be InProgress, Succeeded, or Failed, and indicates
* the status of the job.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see StatusCode
*/
public GlacierJobDescription withStatusCode(StatusCode statusCode) {
this.statusCode = statusCode.toString();
return this;
}
/**
* A friendly message that describes the job status.
*
* @return A friendly message that describes the job status.
*/
public String getStatusMessage() {
return statusMessage;
}
/**
* A friendly message that describes the job status.
*
* @param statusMessage A friendly message that describes the job status.
*/
public void setStatusMessage(String statusMessage) {
this.statusMessage = statusMessage;
}
/**
* A friendly message that describes the job status.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param statusMessage A friendly message that describes the job status.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public GlacierJobDescription withStatusMessage(String statusMessage) {
this.statusMessage = statusMessage;
return this;
}
/**
* For an ArchiveRetrieval job, this is the size in bytes of the archive
* being requested for download. For the InventoryRetrieval job, the
* value is null.
*
* @return For an ArchiveRetrieval job, this is the size in bytes of the archive
* being requested for download. For the InventoryRetrieval job, the
* value is null.
*/
public Long getArchiveSizeInBytes() {
return archiveSizeInBytes;
}
/**
* For an ArchiveRetrieval job, this is the size in bytes of the archive
* being requested for download. For the InventoryRetrieval job, the
* value is null.
*
* @param archiveSizeInBytes For an ArchiveRetrieval job, this is the size in bytes of the archive
* being requested for download. For the InventoryRetrieval job, the
* value is null.
*/
public void setArchiveSizeInBytes(Long archiveSizeInBytes) {
this.archiveSizeInBytes = archiveSizeInBytes;
}
/**
* For an ArchiveRetrieval job, this is the size in bytes of the archive
* being requested for download. For the InventoryRetrieval job, the
* value is null.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param archiveSizeInBytes For an ArchiveRetrieval job, this is the size in bytes of the archive
* being requested for download. For the InventoryRetrieval job, the
* value is null.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public GlacierJobDescription withArchiveSizeInBytes(Long archiveSizeInBytes) {
this.archiveSizeInBytes = archiveSizeInBytes;
return this;
}
/**
* For an InventoryRetrieval job, this is the size in bytes of the
* inventory requested for download. For the ArchiveRetrieval job, the
* value is null.
*
* @return For an InventoryRetrieval job, this is the size in bytes of the
* inventory requested for download. For the ArchiveRetrieval job, the
* value is null.
*/
public Long getInventorySizeInBytes() {
return inventorySizeInBytes;
}
/**
* For an InventoryRetrieval job, this is the size in bytes of the
* inventory requested for download. For the ArchiveRetrieval job, the
* value is null.
*
* @param inventorySizeInBytes For an InventoryRetrieval job, this is the size in bytes of the
* inventory requested for download. For the ArchiveRetrieval job, the
* value is null.
*/
public void setInventorySizeInBytes(Long inventorySizeInBytes) {
this.inventorySizeInBytes = inventorySizeInBytes;
}
/**
* For an InventoryRetrieval job, this is the size in bytes of the
* inventory requested for download. For the ArchiveRetrieval job, the
* value is null.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param inventorySizeInBytes For an InventoryRetrieval job, this is the size in bytes of the
* inventory requested for download. For the ArchiveRetrieval job, the
* value is null.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public GlacierJobDescription withInventorySizeInBytes(Long inventorySizeInBytes) {
this.inventorySizeInBytes = inventorySizeInBytes;
return this;
}
/**
* An Amazon Simple Notification Service (Amazon SNS) topic that receives
* notification.
*
* @return An Amazon Simple Notification Service (Amazon SNS) topic that receives
* notification.
*/
public String getSNSTopic() {
return sNSTopic;
}
/**
* An Amazon Simple Notification Service (Amazon SNS) topic that receives
* notification.
*
* @param sNSTopic An Amazon Simple Notification Service (Amazon SNS) topic that receives
* notification.
*/
public void setSNSTopic(String sNSTopic) {
this.sNSTopic = sNSTopic;
}
/**
* An Amazon Simple Notification Service (Amazon SNS) topic that receives
* notification.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param sNSTopic An Amazon Simple Notification Service (Amazon SNS) topic that receives
* notification.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public GlacierJobDescription withSNSTopic(String sNSTopic) {
this.sNSTopic = sNSTopic;
return this;
}
/**
* The UTC time that the archive retrieval request completed. While the
* job is in progress, the value will be null.
*
* @return The UTC time that the archive retrieval request completed. While the
* job is in progress, the value will be null.
*/
public String getCompletionDate() {
return completionDate;
}
/**
* The UTC time that the archive retrieval request completed. While the
* job is in progress, the value will be null.
*
* @param completionDate The UTC time that the archive retrieval request completed. While the
* job is in progress, the value will be null.
*/
public void setCompletionDate(String completionDate) {
this.completionDate = completionDate;
}
/**
* The UTC time that the archive retrieval request completed. While the
* job is in progress, the value will be null.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param completionDate The UTC time that the archive retrieval request completed. While the
* job is in progress, the value will be null.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public GlacierJobDescription withCompletionDate(String completionDate) {
this.completionDate = completionDate;
return this;
}
/**
* For an ArchiveRetrieval job, it is the checksum of the archive.
* Otherwise, the value is null.
The SHA256 tree hash value for the
* requested range of an archive. If the Initiate a Job request for an
* archive specified a tree-hash aligned range, then this field returns a
* value.
For the specific case when the whole archive is retrieved,
* this value is the same as the ArchiveSHA256TreeHash value.
This
* field is null in the following situations:
Archive
* retrieval jobs that specify a range that is not tree-hash
* aligned.
Archival jobs that specify a range
* that is equal to the whole archive and the job status is
* InProgress.
Inventory jobs.
*
* @return For an ArchiveRetrieval job, it is the checksum of the archive.
* Otherwise, the value is null. The SHA256 tree hash value for the
* requested range of an archive. If the Initiate a Job request for an
* archive specified a tree-hash aligned range, then this field returns a
* value.
For the specific case when the whole archive is retrieved,
* this value is the same as the ArchiveSHA256TreeHash value.
This
* field is null in the following situations:
Archive
* retrieval jobs that specify a range that is not tree-hash
* aligned.
Archival jobs that specify a range
* that is equal to the whole archive and the job status is
* InProgress.
Inventory jobs.
*/
public String getSHA256TreeHash() {
return sHA256TreeHash;
}
/**
* For an ArchiveRetrieval job, it is the checksum of the archive.
* Otherwise, the value is null. The SHA256 tree hash value for the
* requested range of an archive. If the Initiate a Job request for an
* archive specified a tree-hash aligned range, then this field returns a
* value.
For the specific case when the whole archive is retrieved,
* this value is the same as the ArchiveSHA256TreeHash value.
This
* field is null in the following situations:
Archive
* retrieval jobs that specify a range that is not tree-hash
* aligned.
Archival jobs that specify a range
* that is equal to the whole archive and the job status is
* InProgress.
Inventory jobs.
*
* @param sHA256TreeHash For an ArchiveRetrieval job, it is the checksum of the archive.
* Otherwise, the value is null. The SHA256 tree hash value for the
* requested range of an archive. If the Initiate a Job request for an
* archive specified a tree-hash aligned range, then this field returns a
* value.
For the specific case when the whole archive is retrieved,
* this value is the same as the ArchiveSHA256TreeHash value.
This
* field is null in the following situations:
Archive
* retrieval jobs that specify a range that is not tree-hash
* aligned.
Archival jobs that specify a range
* that is equal to the whole archive and the job status is
* InProgress.
Inventory jobs.
*/
public void setSHA256TreeHash(String sHA256TreeHash) {
this.sHA256TreeHash = sHA256TreeHash;
}
/**
* For an ArchiveRetrieval job, it is the checksum of the archive.
* Otherwise, the value is null. The SHA256 tree hash value for the
* requested range of an archive. If the Initiate a Job request for an
* archive specified a tree-hash aligned range, then this field returns a
* value.
For the specific case when the whole archive is retrieved,
* this value is the same as the ArchiveSHA256TreeHash value.
This
* field is null in the following situations:
Archive
* retrieval jobs that specify a range that is not tree-hash
* aligned.
Archival jobs that specify a range
* that is equal to the whole archive and the job status is
* InProgress.
Inventory jobs.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param sHA256TreeHash For an ArchiveRetrieval job, it is the checksum of the archive.
* Otherwise, the value is null.
The SHA256 tree hash value for the
* requested range of an archive. If the Initiate a Job request for an
* archive specified a tree-hash aligned range, then this field returns a
* value.
For the specific case when the whole archive is retrieved,
* this value is the same as the ArchiveSHA256TreeHash value.
This
* field is null in the following situations:
Archive
* retrieval jobs that specify a range that is not tree-hash
* aligned.
Archival jobs that specify a range
* that is equal to the whole archive and the job status is
* InProgress.
Inventory jobs.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public GlacierJobDescription withSHA256TreeHash(String sHA256TreeHash) {
this.sHA256TreeHash = sHA256TreeHash;
return this;
}
/**
* The SHA256 tree hash of the entire archive for an archive retrieval.
* For inventory retrieval jobs, this field is null.
*
* @return The SHA256 tree hash of the entire archive for an archive retrieval.
* For inventory retrieval jobs, this field is null.
*/
public String getArchiveSHA256TreeHash() {
return archiveSHA256TreeHash;
}
/**
* The SHA256 tree hash of the entire archive for an archive retrieval.
* For inventory retrieval jobs, this field is null.
*
* @param archiveSHA256TreeHash The SHA256 tree hash of the entire archive for an archive retrieval.
* For inventory retrieval jobs, this field is null.
*/
public void setArchiveSHA256TreeHash(String archiveSHA256TreeHash) {
this.archiveSHA256TreeHash = archiveSHA256TreeHash;
}
/**
* The SHA256 tree hash of the entire archive for an archive retrieval.
* For inventory retrieval jobs, this field is null.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param archiveSHA256TreeHash The SHA256 tree hash of the entire archive for an archive retrieval.
* For inventory retrieval jobs, this field is null.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public GlacierJobDescription withArchiveSHA256TreeHash(String archiveSHA256TreeHash) {
this.archiveSHA256TreeHash = archiveSHA256TreeHash;
return this;
}
/**
* The retrieved byte range for archive retrieval jobs in the form
* "StartByteValue-EndByteValue" If no range was specified
* in the archive retrieval, then the whole archive is retrieved and
* StartByteValue equals 0 and EndByteValue equals the size
* of the archive minus 1. For inventory retrieval jobs this field is
* null.
*
* @return The retrieved byte range for archive retrieval jobs in the form
* "StartByteValue-EndByteValue" If no range was specified
* in the archive retrieval, then the whole archive is retrieved and
* StartByteValue equals 0 and EndByteValue equals the size
* of the archive minus 1. For inventory retrieval jobs this field is
* null.
*/
public String getRetrievalByteRange() {
return retrievalByteRange;
}
/**
* The retrieved byte range for archive retrieval jobs in the form
* "StartByteValue-EndByteValue" If no range was specified
* in the archive retrieval, then the whole archive is retrieved and
* StartByteValue equals 0 and EndByteValue equals the size
* of the archive minus 1. For inventory retrieval jobs this field is
* null.
*
* @param retrievalByteRange The retrieved byte range for archive retrieval jobs in the form
* "StartByteValue-EndByteValue" If no range was specified
* in the archive retrieval, then the whole archive is retrieved and
* StartByteValue equals 0 and EndByteValue equals the size
* of the archive minus 1. For inventory retrieval jobs this field is
* null.
*/
public void setRetrievalByteRange(String retrievalByteRange) {
this.retrievalByteRange = retrievalByteRange;
}
/**
* The retrieved byte range for archive retrieval jobs in the form
* "StartByteValue-EndByteValue" If no range was specified
* in the archive retrieval, then the whole archive is retrieved and
* StartByteValue equals 0 and EndByteValue equals the size
* of the archive minus 1. For inventory retrieval jobs this field is
* null.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param retrievalByteRange The retrieved byte range for archive retrieval jobs in the form
* "StartByteValue-EndByteValue" If no range was specified
* in the archive retrieval, then the whole archive is retrieved and
* StartByteValue equals 0 and EndByteValue equals the size
* of the archive minus 1. For inventory retrieval jobs this field is
* null.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public GlacierJobDescription withRetrievalByteRange(String retrievalByteRange) {
this.retrievalByteRange = retrievalByteRange;
return this;
}
/**
* Parameters used for range inventory retrieval.
*
* @return Parameters used for range inventory retrieval.
*/
public InventoryRetrievalJobDescription getInventoryRetrievalParameters() {
return inventoryRetrievalParameters;
}
/**
* Parameters used for range inventory retrieval.
*
* @param inventoryRetrievalParameters Parameters used for range inventory retrieval.
*/
public void setInventoryRetrievalParameters(InventoryRetrievalJobDescription inventoryRetrievalParameters) {
this.inventoryRetrievalParameters = inventoryRetrievalParameters;
}
/**
* Parameters used for range inventory retrieval.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param inventoryRetrievalParameters Parameters used for range inventory retrieval.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public GlacierJobDescription withInventoryRetrievalParameters(InventoryRetrievalJobDescription inventoryRetrievalParameters) {
this.inventoryRetrievalParameters = inventoryRetrievalParameters;
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getJobId() != null) sb.append("JobId: " + getJobId() + ",");
if (getJobDescription() != null) sb.append("JobDescription: " + getJobDescription() + ",");
if (getAction() != null) sb.append("Action: " + getAction() + ",");
if (getArchiveId() != null) sb.append("ArchiveId: " + getArchiveId() + ",");
if (getVaultARN() != null) sb.append("VaultARN: " + getVaultARN() + ",");
if (getCreationDate() != null) sb.append("CreationDate: " + getCreationDate() + ",");
if (isCompleted() != null) sb.append("Completed: " + isCompleted() + ",");
if (getStatusCode() != null) sb.append("StatusCode: " + getStatusCode() + ",");
if (getStatusMessage() != null) sb.append("StatusMessage: " + getStatusMessage() + ",");
if (getArchiveSizeInBytes() != null) sb.append("ArchiveSizeInBytes: " + getArchiveSizeInBytes() + ",");
if (getInventorySizeInBytes() != null) sb.append("InventorySizeInBytes: " + getInventorySizeInBytes() + ",");
if (getSNSTopic() != null) sb.append("SNSTopic: " + getSNSTopic() + ",");
if (getCompletionDate() != null) sb.append("CompletionDate: " + getCompletionDate() + ",");
if (getSHA256TreeHash() != null) sb.append("SHA256TreeHash: " + getSHA256TreeHash() + ",");
if (getArchiveSHA256TreeHash() != null) sb.append("ArchiveSHA256TreeHash: " + getArchiveSHA256TreeHash() + ",");
if (getRetrievalByteRange() != null) sb.append("RetrievalByteRange: " + getRetrievalByteRange() + ",");
if (getInventoryRetrievalParameters() != null) sb.append("InventoryRetrievalParameters: " + getInventoryRetrievalParameters() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getJobId() == null) ? 0 : getJobId().hashCode());
hashCode = prime * hashCode + ((getJobDescription() == null) ? 0 : getJobDescription().hashCode());
hashCode = prime * hashCode + ((getAction() == null) ? 0 : getAction().hashCode());
hashCode = prime * hashCode + ((getArchiveId() == null) ? 0 : getArchiveId().hashCode());
hashCode = prime * hashCode + ((getVaultARN() == null) ? 0 : getVaultARN().hashCode());
hashCode = prime * hashCode + ((getCreationDate() == null) ? 0 : getCreationDate().hashCode());
hashCode = prime * hashCode + ((isCompleted() == null) ? 0 : isCompleted().hashCode());
hashCode = prime * hashCode + ((getStatusCode() == null) ? 0 : getStatusCode().hashCode());
hashCode = prime * hashCode + ((getStatusMessage() == null) ? 0 : getStatusMessage().hashCode());
hashCode = prime * hashCode + ((getArchiveSizeInBytes() == null) ? 0 : getArchiveSizeInBytes().hashCode());
hashCode = prime * hashCode + ((getInventorySizeInBytes() == null) ? 0 : getInventorySizeInBytes().hashCode());
hashCode = prime * hashCode + ((getSNSTopic() == null) ? 0 : getSNSTopic().hashCode());
hashCode = prime * hashCode + ((getCompletionDate() == null) ? 0 : getCompletionDate().hashCode());
hashCode = prime * hashCode + ((getSHA256TreeHash() == null) ? 0 : getSHA256TreeHash().hashCode());
hashCode = prime * hashCode + ((getArchiveSHA256TreeHash() == null) ? 0 : getArchiveSHA256TreeHash().hashCode());
hashCode = prime * hashCode + ((getRetrievalByteRange() == null) ? 0 : getRetrievalByteRange().hashCode());
hashCode = prime * hashCode + ((getInventoryRetrievalParameters() == null) ? 0 : getInventoryRetrievalParameters().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof GlacierJobDescription == false) return false;
GlacierJobDescription other = (GlacierJobDescription)obj;
if (other.getJobId() == null ^ this.getJobId() == null) return false;
if (other.getJobId() != null && other.getJobId().equals(this.getJobId()) == false) return false;
if (other.getJobDescription() == null ^ this.getJobDescription() == null) return false;
if (other.getJobDescription() != null && other.getJobDescription().equals(this.getJobDescription()) == false) return false;
if (other.getAction() == null ^ this.getAction() == null) return false;
if (other.getAction() != null && other.getAction().equals(this.getAction()) == false) return false;
if (other.getArchiveId() == null ^ this.getArchiveId() == null) return false;
if (other.getArchiveId() != null && other.getArchiveId().equals(this.getArchiveId()) == false) return false;
if (other.getVaultARN() == null ^ this.getVaultARN() == null) return false;
if (other.getVaultARN() != null && other.getVaultARN().equals(this.getVaultARN()) == false) return false;
if (other.getCreationDate() == null ^ this.getCreationDate() == null) return false;
if (other.getCreationDate() != null && other.getCreationDate().equals(this.getCreationDate()) == false) return false;
if (other.isCompleted() == null ^ this.isCompleted() == null) return false;
if (other.isCompleted() != null && other.isCompleted().equals(this.isCompleted()) == false) return false;
if (other.getStatusCode() == null ^ this.getStatusCode() == null) return false;
if (other.getStatusCode() != null && other.getStatusCode().equals(this.getStatusCode()) == false) return false;
if (other.getStatusMessage() == null ^ this.getStatusMessage() == null) return false;
if (other.getStatusMessage() != null && other.getStatusMessage().equals(this.getStatusMessage()) == false) return false;
if (other.getArchiveSizeInBytes() == null ^ this.getArchiveSizeInBytes() == null) return false;
if (other.getArchiveSizeInBytes() != null && other.getArchiveSizeInBytes().equals(this.getArchiveSizeInBytes()) == false) return false;
if (other.getInventorySizeInBytes() == null ^ this.getInventorySizeInBytes() == null) return false;
if (other.getInventorySizeInBytes() != null && other.getInventorySizeInBytes().equals(this.getInventorySizeInBytes()) == false) return false;
if (other.getSNSTopic() == null ^ this.getSNSTopic() == null) return false;
if (other.getSNSTopic() != null && other.getSNSTopic().equals(this.getSNSTopic()) == false) return false;
if (other.getCompletionDate() == null ^ this.getCompletionDate() == null) return false;
if (other.getCompletionDate() != null && other.getCompletionDate().equals(this.getCompletionDate()) == false) return false;
if (other.getSHA256TreeHash() == null ^ this.getSHA256TreeHash() == null) return false;
if (other.getSHA256TreeHash() != null && other.getSHA256TreeHash().equals(this.getSHA256TreeHash()) == false) return false;
if (other.getArchiveSHA256TreeHash() == null ^ this.getArchiveSHA256TreeHash() == null) return false;
if (other.getArchiveSHA256TreeHash() != null && other.getArchiveSHA256TreeHash().equals(this.getArchiveSHA256TreeHash()) == false) return false;
if (other.getRetrievalByteRange() == null ^ this.getRetrievalByteRange() == null) return false;
if (other.getRetrievalByteRange() != null && other.getRetrievalByteRange().equals(this.getRetrievalByteRange()) == false) return false;
if (other.getInventoryRetrievalParameters() == null ^ this.getInventoryRetrievalParameters() == null) return false;
if (other.getInventoryRetrievalParameters() != null && other.getInventoryRetrievalParameters().equals(this.getInventoryRetrievalParameters()) == false) return false;
return true;
}
}