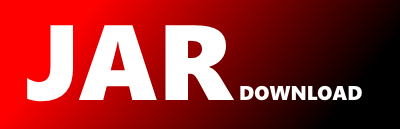
com.amazonaws.services.identitymanagement.AmazonIdentityManagementClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.identitymanagement;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import java.util.Map.Entry;
import com.amazonaws.*;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.services.identitymanagement.model.*;
import com.amazonaws.services.identitymanagement.model.transform.*;
/**
* Client for accessing AmazonIdentityManagement. All service calls made
* using this client are blocking, and will not return until the service call
* completes.
*
* AWS Identity and Access Management
* AWS Identity and Access Management (IAM) is a web service that you can
* use to manage users and user permissions under your AWS account. This
* guide provides descriptions of the IAM API. For general information
* about IAM, see
* AWS Identity and Access Management (IAM) . For the user guide for IAM, see Using IAM
* .
*
*
* NOTE: AWS provides SDKs that consist of libraries and sample
* code for various programming languages and platforms (Java, Ruby,
* .NET, iOS, Android, etc.). The SDKs provide a convenient way to create
* programmatic access to IAM and AWS. For example, the SDKs take care of
* tasks such as cryptographically signing requests (see below), managing
* errors, and retrying requests automatically. For information about the
* AWS SDKs, including how to download and install them, see the Tools
* for Amazon Web Services page.
*
*
* Using the IAM Query API, you make direct calls to the IAM web
* service. IAM supports GET and POST requests for all actions. That is,
* the API does not require you to use GET for some actions and POST for
* others. However, GET requests are subject to the limitation size of a
* URL; although this limit is browser dependent, a typical limit is 2048
* bytes. Therefore, for operations that require larger sizes, you must
* use a POST request.
*
*
* Signing Requests Requests must be signed using an access key
* ID and a secret access key. We strongly recommend that you do not use
* your AWS account access key ID and secret access key for everyday work
* with IAM. You can use the access key ID and secret access key for an
* IAM user or you can use the AWS Security Token Service to generate
* temporary security credentials and use those to sign requests.
*
*
* To sign requests, we recommend that you use
* Signature Version 4
* . If you have an existing application that uses Signature Version 2,
* you do not have to update it to use Signature Version 4. However, some
* operations now require Signature Version 4. The documentation for
* operations that require version 4 indicate this requirement.
*
*
* Additional Resources For more information, see the following:
*
*
*
* -
* AWS Security Credentials
* . This topic provides general information about the types of
* credentials used for accessing AWS.
* -
* IAM Best Practices
* . This topic presents a list of suggestions for using the IAM service
* to help secure your AWS resources.
* -
* AWS Security Token Service
* . This guide describes how to create and use temporary security
* credentials.
* -
* Signing AWS API Requests
* . This set of topics walk you through the process of signing a
* request using an access key ID and secret access key.
*
*
*/
public class AmazonIdentityManagementClient extends AmazonWebServiceClient implements AmazonIdentityManagement {
/** Provider for AWS credentials. */
private AWSCredentialsProvider awsCredentialsProvider;
/**
* List of exception unmarshallers for all AmazonIdentityManagement exceptions.
*/
protected final List> exceptionUnmarshallers
= new ArrayList>();
/**
* Constructs a new client to invoke service methods on
* AmazonIdentityManagement. A credentials provider chain will be used
* that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @see DefaultAWSCredentialsProviderChain
*/
public AmazonIdentityManagementClient() {
this(new DefaultAWSCredentialsProviderChain(), new ClientConfiguration());
}
/**
* Constructs a new client to invoke service methods on
* AmazonIdentityManagement. A credentials provider chain will be used
* that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to AmazonIdentityManagement
* (ex: proxy settings, retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
*/
public AmazonIdentityManagementClient(ClientConfiguration clientConfiguration) {
this(new DefaultAWSCredentialsProviderChain(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on
* AmazonIdentityManagement using the specified AWS account credentials.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentials The AWS credentials (access key ID and secret key) to use
* when authenticating with AWS services.
*/
public AmazonIdentityManagementClient(AWSCredentials awsCredentials) {
this(awsCredentials, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service methods on
* AmazonIdentityManagement using the specified AWS account credentials
* and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentials The AWS credentials (access key ID and secret key) to use
* when authenticating with AWS services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to AmazonIdentityManagement
* (ex: proxy settings, retry counts, etc.).
*/
public AmazonIdentityManagementClient(AWSCredentials awsCredentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.awsCredentialsProvider = new StaticCredentialsProvider(awsCredentials);
init();
}
/**
* Constructs a new client to invoke service methods on
* AmazonIdentityManagement using the specified AWS account credentials provider.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
*/
public AmazonIdentityManagementClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service methods on
* AmazonIdentityManagement using the specified AWS account credentials
* provider and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to AmazonIdentityManagement
* (ex: proxy settings, retry counts, etc.).
*/
public AmazonIdentityManagementClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on
* AmazonIdentityManagement using the specified AWS account credentials
* provider, client configuration options, and request metric collector.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to AmazonIdentityManagement
* (ex: proxy settings, retry counts, etc.).
* @param requestMetricCollector optional request metric collector
*/
public AmazonIdentityManagementClient(AWSCredentialsProvider awsCredentialsProvider,
ClientConfiguration clientConfiguration,
RequestMetricCollector requestMetricCollector) {
super(clientConfiguration, requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
init();
}
private void init() {
exceptionUnmarshallers.add(new DuplicateCertificateExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidUserTypeExceptionUnmarshaller());
exceptionUnmarshallers.add(new EntityAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new KeyPairMismatchExceptionUnmarshaller());
exceptionUnmarshallers.add(new DeleteConflictExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidAuthenticationCodeExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidInputExceptionUnmarshaller());
exceptionUnmarshallers.add(new EntityTemporarilyUnmodifiableExceptionUnmarshaller());
exceptionUnmarshallers.add(new MalformedCertificateExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidCertificateExceptionUnmarshaller());
exceptionUnmarshallers.add(new MalformedPolicyDocumentExceptionUnmarshaller());
exceptionUnmarshallers.add(new LimitExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new PasswordPolicyViolationExceptionUnmarshaller());
exceptionUnmarshallers.add(new NoSuchEntityExceptionUnmarshaller());
exceptionUnmarshallers.add(new StandardErrorUnmarshaller());
// calling this.setEndPoint(...) will also modify the signer accordingly
this.setEndpoint("iam.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain(
"/com/amazonaws/services/identitymanagement/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain(
"/com/amazonaws/services/identitymanagement/request.handler2s"));
}
/**
*
* Deletes the specified AWS account alias. For information about using
* an AWS account alias, see
* Using an Alias for Your AWS Account ID
* in Using AWS Identity and Access Management .
*
*
* @param deleteAccountAliasRequest Container for the necessary
* parameters to execute the DeleteAccountAlias service method on
* AmazonIdentityManagement.
*
*
* @throws NoSuchEntityException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteAccountAlias(DeleteAccountAliasRequest deleteAccountAliasRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAccountAliasRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteAccountAliasRequestMarshaller().marshall(deleteAccountAliasRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Lists the groups that have the specified path prefix.
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* @param listGroupsRequest Container for the necessary parameters to
* execute the ListGroups service method on AmazonIdentityManagement.
*
* @return The response from the ListGroups service method, as returned
* by AmazonIdentityManagement.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListGroupsResult listGroups(ListGroupsRequest listGroupsRequest) {
ExecutionContext executionContext = createExecutionContext(listGroupsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ListGroupsRequestMarshaller().marshall(listGroupsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ListGroupsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the access key associated with the specified user.
*
*
* If you do not specify a user name, IAM determines the user name
* implicitly based on the AWS access key ID signing the request. Because
* this action works for access keys under the AWS account, you can use
* this API to manage root credentials even if the AWS account has no
* associated users.
*
*
* @param deleteAccessKeyRequest Container for the necessary parameters
* to execute the DeleteAccessKey service method on
* AmazonIdentityManagement.
*
*
* @throws NoSuchEntityException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteAccessKey(DeleteAccessKeyRequest deleteAccessKeyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAccessKeyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteAccessKeyRequestMarshaller().marshall(deleteAccessKeyRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Deletes a virtual MFA device.
*
*
* NOTE:You must deactivate a user's virtual MFA device before you
* can delete it. For information about deactivating MFA devices, see
* DeactivateMFADevice.
*
*
* @param deleteVirtualMFADeviceRequest Container for the necessary
* parameters to execute the DeleteVirtualMFADevice service method on
* AmazonIdentityManagement.
*
*
* @throws NoSuchEntityException
* @throws DeleteConflictException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteVirtualMFADevice(DeleteVirtualMFADeviceRequest deleteVirtualMFADeviceRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVirtualMFADeviceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteVirtualMFADeviceRequestMarshaller().marshall(deleteVirtualMFADeviceRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Deletes the specified policy associated with the specified user.
*
*
* @param deleteUserPolicyRequest Container for the necessary parameters
* to execute the DeleteUserPolicy service method on
* AmazonIdentityManagement.
*
*
* @throws NoSuchEntityException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteUserPolicy(DeleteUserPolicyRequest deleteUserPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteUserPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteUserPolicyRequestMarshaller().marshall(deleteUserPolicyRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Adds (or updates) a policy document associated with the specified
* user. For information about policies, refer to
* Overview of Policies
* in Using AWS Identity and Access Management .
*
*
* For information about limits on the number of policies you can
* associate with a user, see
* Limitations on IAM Entities
* in Using AWS Identity and Access Management .
*
*
* NOTE:Because policy documents can be large, you should use POST
* rather than GET when calling PutUserPolicy. For information about
* setting up signatures and authorization through the API, go to Signing
* AWS API Requests in the AWS General Reference. For general information
* about using the Query API with IAM, go to Making Query Requests in
* Using IAM.
*
*
* @param putUserPolicyRequest Container for the necessary parameters to
* execute the PutUserPolicy service method on AmazonIdentityManagement.
*
*
* @throws MalformedPolicyDocumentException
* @throws NoSuchEntityException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void putUserPolicy(PutUserPolicyRequest putUserPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(putUserPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new PutUserPolicyRequestMarshaller().marshall(putUserPolicyRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Lists the server certificates that have the specified path prefix. If
* none exist, the action returns an empty list.
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* @param listServerCertificatesRequest Container for the necessary
* parameters to execute the ListServerCertificates service method on
* AmazonIdentityManagement.
*
* @return The response from the ListServerCertificates service method,
* as returned by AmazonIdentityManagement.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListServerCertificatesResult listServerCertificates(ListServerCertificatesRequest listServerCertificatesRequest) {
ExecutionContext executionContext = createExecutionContext(listServerCertificatesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ListServerCertificatesRequestMarshaller().marshall(listServerCertificatesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ListServerCertificatesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the SAML providers in the account.
*
*
* NOTE:This operation requires Signature Version 4.
*
*
* @param listSAMLProvidersRequest Container for the necessary parameters
* to execute the ListSAMLProviders service method on
* AmazonIdentityManagement.
*
* @return The response from the ListSAMLProviders service method, as
* returned by AmazonIdentityManagement.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListSAMLProvidersResult listSAMLProviders(ListSAMLProvidersRequest listSAMLProvidersRequest) {
ExecutionContext executionContext = createExecutionContext(listSAMLProvidersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ListSAMLProvidersRequestMarshaller().marshall(listSAMLProvidersRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ListSAMLProvidersResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the specified policy document for the specified user. The
* returned policy is URL-encoded according to RFC 3986. For more
* information about RFC 3986, go to
* http://www.faqs.org/rfcs/rfc3986.html
* .
*
*
* @param getUserPolicyRequest Container for the necessary parameters to
* execute the GetUserPolicy service method on AmazonIdentityManagement.
*
* @return The response from the GetUserPolicy service method, as
* returned by AmazonIdentityManagement.
*
* @throws NoSuchEntityException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public GetUserPolicyResult getUserPolicy(GetUserPolicyRequest getUserPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(getUserPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new GetUserPolicyRequestMarshaller().marshall(getUserPolicyRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new GetUserPolicyResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the name and/or the path of the specified server certificate.
*
*
* IMPORTANT: You should understand the implications of changing a
* server certificate's path or name. For more information, see Managing
* Server Certificates in Using AWS Identity and Access Management.
*
*
* NOTE:To change a server certificate name the requester must
* have appropriate permissions on both the source object and the target
* object. For example, to change the name from ProductionCert to
* ProdCert, the entity making the request must have permission on
* ProductionCert and ProdCert, or must have permission on all (*). For
* more information about permissions, see Permissions and Policies.
*
*
* @param updateServerCertificateRequest Container for the necessary
* parameters to execute the UpdateServerCertificate service method on
* AmazonIdentityManagement.
*
*
* @throws NoSuchEntityException
* @throws LimitExceededException
* @throws EntityAlreadyExistsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void updateServerCertificate(UpdateServerCertificateRequest updateServerCertificateRequest) {
ExecutionContext executionContext = createExecutionContext(updateServerCertificateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new UpdateServerCertificateRequestMarshaller().marshall(updateServerCertificateRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Updates the name and/or the path of the specified user.
*
*
* IMPORTANT: You should understand the implications of changing a
* user's path or name. For more information, see Renaming Users and
* Groups in Using AWS Identity and Access Management.
*
*
* NOTE:To change a user name the requester must have appropriate
* permissions on both the source object and the target object. For
* example, to change Bob to Robert, the entity making the request must
* have permission on Bob and Robert, or must have permission on all (*).
* For more information about permissions, see Permissions and Policies.
*
*
* @param updateUserRequest Container for the necessary parameters to
* execute the UpdateUser service method on AmazonIdentityManagement.
*
*
* @throws EntityTemporarilyUnmodifiableException
* @throws NoSuchEntityException
* @throws LimitExceededException
* @throws EntityAlreadyExistsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void updateUser(UpdateUserRequest updateUserRequest) {
ExecutionContext executionContext = createExecutionContext(updateUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new UpdateUserRequestMarshaller().marshall(updateUserRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Adds (or updates) a policy document associated with the specified
* role. For information about policies, go to
* Overview of Policies
* in Using AWS Identity and Access Management .
*
*
* For information about limits on the policies you can associate with a
* role, see
* Limitations on IAM Entities
* in Using AWS Identity and Access Management .
*
*
* NOTE:Because policy documents can be large, you should use POST
* rather than GET when calling PutRolePolicy. For information about
* setting up signatures and authorization through the API, go to Signing
* AWS API Requests in the AWS General Reference. For general information
* about using the Query API with IAM, go to Making Query Requests in
* Using IAM.
*
*
* @param putRolePolicyRequest Container for the necessary parameters to
* execute the PutRolePolicy service method on AmazonIdentityManagement.
*
*
* @throws MalformedPolicyDocumentException
* @throws NoSuchEntityException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void putRolePolicy(PutRolePolicyRequest putRolePolicyRequest) {
ExecutionContext executionContext = createExecutionContext(putRolePolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new PutRolePolicyRequestMarshaller().marshall(putRolePolicyRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Changes the status of the specified signing certificate from active to
* disabled, or vice versa. This action can be used to disable a user's
* signing certificate as part of a certificate rotation work flow.
*
*
* If the UserName
field is not specified, the UserName is
* determined implicitly based on the AWS access key ID used to sign the
* request. Because this action works for access keys under the AWS
* account, this API can be used to manage root credentials even if the
* AWS account has no associated users.
*
*
* For information about rotating certificates, see
* Managing Keys and Certificates
* in Using AWS Identity and Access Management .
*
*
* @param updateSigningCertificateRequest Container for the necessary
* parameters to execute the UpdateSigningCertificate service method on
* AmazonIdentityManagement.
*
*
* @throws NoSuchEntityException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void updateSigningCertificate(UpdateSigningCertificateRequest updateSigningCertificateRequest) {
ExecutionContext executionContext = createExecutionContext(updateSigningCertificateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new UpdateSigningCertificateRequestMarshaller().marshall(updateSigningCertificateRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Deletes the specified policy that is associated with the specified
* group.
*
*
* @param deleteGroupPolicyRequest Container for the necessary parameters
* to execute the DeleteGroupPolicy service method on
* AmazonIdentityManagement.
*
*
* @throws NoSuchEntityException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteGroupPolicy(DeleteGroupPolicyRequest deleteGroupPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteGroupPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteGroupPolicyRequestMarshaller().marshall(deleteGroupPolicyRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Lists the users that have the specified path prefix. If there are
* none, the action returns an empty list.
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* @param listUsersRequest Container for the necessary parameters to
* execute the ListUsers service method on AmazonIdentityManagement.
*
* @return The response from the ListUsers service method, as returned by
* AmazonIdentityManagement.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListUsersResult listUsers(ListUsersRequest listUsersRequest) {
ExecutionContext executionContext = createExecutionContext(listUsersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ListUsersRequestMarshaller().marshall(listUsersRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ListUsersResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the name and/or the path of the specified group.
*
*
* IMPORTANT: You should understand the implications of changing a
* group's path or name. For more information, see Renaming Users and
* Groups in Using AWS Identity and Access Management.
*
*
* NOTE:To change a group name the requester must have appropriate
* permissions on both the source object and the target object. For
* example, to change Managers to MGRs, the entity making the request
* must have permission on Managers and MGRs, or must have permission on
* all (*). For more information about permissions, see Permissions and
* Policies.
*
*
* @param updateGroupRequest Container for the necessary parameters to
* execute the UpdateGroup service method on AmazonIdentityManagement.
*
*
* @throws NoSuchEntityException
* @throws LimitExceededException
* @throws EntityAlreadyExistsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void updateGroup(UpdateGroupRequest updateGroupRequest) {
ExecutionContext executionContext = createExecutionContext(updateGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new UpdateGroupRequestMarshaller().marshall(updateGroupRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Creates a new user for your AWS account.
*
*
* For information about limitations on the number of users you can
* create, see
* Limitations on IAM Entities
* in Using AWS Identity and Access Management .
*
*
* @param createUserRequest Container for the necessary parameters to
* execute the CreateUser service method on AmazonIdentityManagement.
*
* @return The response from the CreateUser service method, as returned
* by AmazonIdentityManagement.
*
* @throws NoSuchEntityException
* @throws LimitExceededException
* @throws EntityAlreadyExistsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateUserResult createUser(CreateUserRequest createUserRequest) {
ExecutionContext executionContext = createExecutionContext(createUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateUserRequestMarshaller().marshall(createUserRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateUserResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a SAML provider.
*
*
* Deleting the provider does not update any roles that reference the
* SAML provider as a principal in their trust policies. Any attempt to
* assume a role that references a SAML provider that has been deleted
* will fail.
*
*
* NOTE:This operation requires Signature Version 4.
*
*
* @param deleteSAMLProviderRequest Container for the necessary
* parameters to execute the DeleteSAMLProvider service method on
* AmazonIdentityManagement.
*
*
* @throws InvalidInputException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteSAMLProvider(DeleteSAMLProviderRequest deleteSAMLProviderRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSAMLProviderRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteSAMLProviderRequestMarshaller().marshall(deleteSAMLProviderRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Enables the specified MFA device and associates it with the specified
* user name. When enabled, the MFA device is required for every
* subsequent login by the user name associated with the device.
*
*
* @param enableMFADeviceRequest Container for the necessary parameters
* to execute the EnableMFADevice service method on
* AmazonIdentityManagement.
*
*
* @throws EntityTemporarilyUnmodifiableException
* @throws NoSuchEntityException
* @throws InvalidAuthenticationCodeException
* @throws LimitExceededException
* @throws EntityAlreadyExistsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void enableMFADevice(EnableMFADeviceRequest enableMFADeviceRequest) {
ExecutionContext executionContext = createExecutionContext(enableMFADeviceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new EnableMFADeviceRequestMarshaller().marshall(enableMFADeviceRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Deletes the password policy for the AWS account.
*
*
* @param deleteAccountPasswordPolicyRequest Container for the necessary
* parameters to execute the DeleteAccountPasswordPolicy service method
* on AmazonIdentityManagement.
*
*
* @throws NoSuchEntityException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteAccountPasswordPolicy(DeleteAccountPasswordPolicyRequest deleteAccountPasswordPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAccountPasswordPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteAccountPasswordPolicyRequestMarshaller().marshall(deleteAccountPasswordPolicyRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Retrieves the user name and password-creation date for the specified
* user. If the user has not been assigned a password, the action returns
* a 404 ( NoSuchEntity
) error.
*
*
* @param getLoginProfileRequest Container for the necessary parameters
* to execute the GetLoginProfile service method on
* AmazonIdentityManagement.
*
* @return The response from the GetLoginProfile service method, as
* returned by AmazonIdentityManagement.
*
* @throws NoSuchEntityException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public GetLoginProfileResult getLoginProfile(GetLoginProfileRequest getLoginProfileRequest) {
ExecutionContext executionContext = createExecutionContext(getLoginProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new GetLoginProfileRequestMarshaller().marshall(getLoginProfileRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new GetLoginProfileResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the metadata document for an existing SAML provider.
*
*
* NOTE:This operation requires Signature Version 4.
*
*
* @param updateSAMLProviderRequest Container for the necessary
* parameters to execute the UpdateSAMLProvider service method on
* AmazonIdentityManagement.
*
* @return The response from the UpdateSAMLProvider service method, as
* returned by AmazonIdentityManagement.
*
* @throws InvalidInputException
* @throws NoSuchEntityException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public UpdateSAMLProviderResult updateSAMLProvider(UpdateSAMLProviderRequest updateSAMLProviderRequest) {
ExecutionContext executionContext = createExecutionContext(updateSAMLProviderRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new UpdateSAMLProviderRequestMarshaller().marshall(updateSAMLProviderRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new UpdateSAMLProviderResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Uploads a server certificate entity for the AWS account. The server
* certificate entity includes a public key certificate, a private key,
* and an optional certificate chain, which should all be PEM-encoded.
*
*
* For information about the number of server certificates you can
* upload, see
* Limitations on IAM Entities
* in Using AWS Identity and Access Management .
*
*
* NOTE:Because the body of the public key certificate, private
* key, and the certificate chain can be large, you should use POST
* rather than GET when calling UploadServerCertificate. For information
* about setting up signatures and authorization through the API, go to
* Signing AWS API Requests in the AWS General Reference. For general
* information about using the Query API with IAM, go to Making Query
* Requests in Using IAM.
*
*
* @param uploadServerCertificateRequest Container for the necessary
* parameters to execute the UploadServerCertificate service method on
* AmazonIdentityManagement.
*
* @return The response from the UploadServerCertificate service method,
* as returned by AmazonIdentityManagement.
*
* @throws KeyPairMismatchException
* @throws MalformedCertificateException
* @throws LimitExceededException
* @throws EntityAlreadyExistsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public UploadServerCertificateResult uploadServerCertificate(UploadServerCertificateRequest uploadServerCertificateRequest) {
ExecutionContext executionContext = createExecutionContext(uploadServerCertificateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new UploadServerCertificateRequestMarshaller().marshall(uploadServerCertificateRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new UploadServerCertificateResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new group.
*
*
* For information about the number of groups you can create, see
* Limitations on IAM Entities
* in Using AWS Identity and Access Management .
*
*
* @param createGroupRequest Container for the necessary parameters to
* execute the CreateGroup service method on AmazonIdentityManagement.
*
* @return The response from the CreateGroup service method, as returned
* by AmazonIdentityManagement.
*
* @throws NoSuchEntityException
* @throws LimitExceededException
* @throws EntityAlreadyExistsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateGroupResult createGroup(CreateGroupRequest createGroupRequest) {
ExecutionContext executionContext = createExecutionContext(createGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateGroupRequestMarshaller().marshall(createGroupRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateGroupResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This action creates an alias for your AWS account. For information
* about using an AWS account alias, see
* Using an Alias for Your AWS Account ID
* in Using AWS Identity and Access Management .
*
*
* @param createAccountAliasRequest Container for the necessary
* parameters to execute the CreateAccountAlias service method on
* AmazonIdentityManagement.
*
*
* @throws LimitExceededException
* @throws EntityAlreadyExistsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void createAccountAlias(CreateAccountAliasRequest createAccountAliasRequest) {
ExecutionContext executionContext = createExecutionContext(createAccountAliasRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateAccountAliasRequestMarshaller().marshall(createAccountAliasRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Deletes the specified user. The user must not belong to any groups,
* have any keys or signing certificates, or have any attached policies.
*
*
* @param deleteUserRequest Container for the necessary parameters to
* execute the DeleteUser service method on AmazonIdentityManagement.
*
*
* @throws NoSuchEntityException
* @throws DeleteConflictException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteUser(DeleteUserRequest deleteUserRequest) {
ExecutionContext executionContext = createExecutionContext(deleteUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteUserRequestMarshaller().marshall(deleteUserRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Deactivates the specified MFA device and removes it from association
* with the user name for which it was originally enabled.
*
*
* @param deactivateMFADeviceRequest Container for the necessary
* parameters to execute the DeactivateMFADevice service method on
* AmazonIdentityManagement.
*
*
* @throws EntityTemporarilyUnmodifiableException
* @throws NoSuchEntityException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deactivateMFADevice(DeactivateMFADeviceRequest deactivateMFADeviceRequest) {
ExecutionContext executionContext = createExecutionContext(deactivateMFADeviceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeactivateMFADeviceRequestMarshaller().marshall(deactivateMFADeviceRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Removes the specified user from the specified group.
*
*
* @param removeUserFromGroupRequest Container for the necessary
* parameters to execute the RemoveUserFromGroup service method on
* AmazonIdentityManagement.
*
*
* @throws NoSuchEntityException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void removeUserFromGroup(RemoveUserFromGroupRequest removeUserFromGroupRequest) {
ExecutionContext executionContext = createExecutionContext(removeUserFromGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new RemoveUserFromGroupRequestMarshaller().marshall(removeUserFromGroupRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Deletes the specified role. The role must not have any policies
* attached. For more information about roles, go to
* Working with Roles
* .
*
*
* IMPORTANT:Make sure you do not have any Amazon EC2 instances
* running with the role you are about to delete. Deleting a role or
* instance profile that is associated with a running instance will break
* any applications running on the instance.
*
*
* @param deleteRoleRequest Container for the necessary parameters to
* execute the DeleteRole service method on AmazonIdentityManagement.
*
*
* @throws NoSuchEntityException
* @throws DeleteConflictException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteRole(DeleteRoleRequest deleteRoleRequest) {
ExecutionContext executionContext = createExecutionContext(deleteRoleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteRoleRequestMarshaller().marshall(deleteRoleRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Deletes the specified server certificate.
*
*
* IMPORTANT:If you are using a server certificate with Elastic
* Load Balancing, deleting the certificate could have implications for
* your application. If Elastic Load Balancing doesn't detect the
* deletion of bound certificates, it may continue to use the
* certificates. This could cause Elastic Load Balancing to stop
* accepting traffic. We recommend that you remove the reference to the
* certificate from Elastic Load Balancing before using this command to
* delete the certificate. For more information, go to
* DeleteLoadBalancerListeners in the Elastic Load Balancing API
* Reference.
*
*
* @param deleteServerCertificateRequest Container for the necessary
* parameters to execute the DeleteServerCertificate service method on
* AmazonIdentityManagement.
*
*
* @throws NoSuchEntityException
* @throws DeleteConflictException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteServerCertificate(DeleteServerCertificateRequest deleteServerCertificateRequest) {
ExecutionContext executionContext = createExecutionContext(deleteServerCertificateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteServerCertificateRequestMarshaller().marshall(deleteServerCertificateRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Creates a new AWS secret access key and corresponding AWS access key
* ID for the specified user. The default status for new keys is
* Active
.
*
*
* If you do not specify a user name, IAM determines the user name
* implicitly based on the AWS access key ID signing the request. Because
* this action works for access keys under the AWS account, you can use
* this API to manage root credentials even if the AWS account has no
* associated users.
*
*
* For information about limits on the number of keys you can create, see
* Limitations on IAM Entities
* in Using AWS Identity and Access Management .
*
*
* IMPORTANT:To ensure the security of your AWS account, the
* secret access key is accessible only during key and user creation. You
* must save the key (for example, in a text file) if you want to be able
* to access it again. If a secret key is lost, you can delete the access
* keys for the associated user and then create new keys.
*
*
* @param createAccessKeyRequest Container for the necessary parameters
* to execute the CreateAccessKey service method on
* AmazonIdentityManagement.
*
* @return The response from the CreateAccessKey service method, as
* returned by AmazonIdentityManagement.
*
* @throws NoSuchEntityException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateAccessKeyResult createAccessKey(CreateAccessKeyRequest createAccessKeyRequest) {
ExecutionContext executionContext = createExecutionContext(createAccessKeyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateAccessKeyRequestMarshaller().marshall(createAccessKeyRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateAccessKeyResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about the specified user, including the user's
* path, unique ID, and ARN.
*
*
* If you do not specify a user name, IAM determines the user name
* implicitly based on the AWS access key ID signing the request.
*
*
* @param getUserRequest Container for the necessary parameters to
* execute the GetUser service method on AmazonIdentityManagement.
*
* @return The response from the GetUser service method, as returned by
* AmazonIdentityManagement.
*
* @throws NoSuchEntityException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public GetUserResult getUser(GetUserRequest getUserRequest) {
ExecutionContext executionContext = createExecutionContext(getUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new GetUserRequestMarshaller().marshall(getUserRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new GetUserResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Synchronizes the specified MFA device with AWS servers.
*
*
* @param resyncMFADeviceRequest Container for the necessary parameters
* to execute the ResyncMFADevice service method on
* AmazonIdentityManagement.
*
*
* @throws NoSuchEntityException
* @throws InvalidAuthenticationCodeException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void resyncMFADevice(ResyncMFADeviceRequest resyncMFADeviceRequest) {
ExecutionContext executionContext = createExecutionContext(resyncMFADeviceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ResyncMFADeviceRequestMarshaller().marshall(resyncMFADeviceRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Lists the MFA devices. If the request includes the user name, then
* this action lists all the MFA devices associated with the specified
* user name. If you do not specify a user name, IAM determines the user
* name implicitly based on the AWS access key ID signing the request.
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* @param listMFADevicesRequest Container for the necessary parameters to
* execute the ListMFADevices service method on AmazonIdentityManagement.
*
* @return The response from the ListMFADevices service method, as
* returned by AmazonIdentityManagement.
*
* @throws NoSuchEntityException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListMFADevicesResult listMFADevices(ListMFADevicesRequest listMFADevicesRequest) {
ExecutionContext executionContext = createExecutionContext(listMFADevicesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ListMFADevicesRequestMarshaller().marshall(listMFADevicesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ListMFADevicesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new virtual MFA device for the AWS account. After creating
* the virtual MFA, use
* EnableMFADevice to attach the MFA device to an IAM user. For more information about creating and working with virtual MFA devices, go to Using a Virtual MFA Device
* in Using AWS Identity and Access Management .
*
*
* For information about limits on the number of MFA devices you can
* create, see
* Limitations on Entities
* in Using AWS Identity and Access Management .
*
*
* IMPORTANT:The seed information contained in the QR code and the
* Base32 string should be treated like any other secret access
* information, such as your AWS access keys or your passwords. After you
* provision your virtual device, you should ensure that the information
* is destroyed following secure procedures.
*
*
* @param createVirtualMFADeviceRequest Container for the necessary
* parameters to execute the CreateVirtualMFADevice service method on
* AmazonIdentityManagement.
*
* @return The response from the CreateVirtualMFADevice service method,
* as returned by AmazonIdentityManagement.
*
* @throws LimitExceededException
* @throws EntityAlreadyExistsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateVirtualMFADeviceResult createVirtualMFADevice(CreateVirtualMFADeviceRequest createVirtualMFADeviceRequest) {
ExecutionContext executionContext = createExecutionContext(createVirtualMFADeviceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateVirtualMFADeviceRequestMarshaller().marshall(createVirtualMFADeviceRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateVirtualMFADeviceResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the instance profiles that have the specified path prefix. If
* there are none, the action returns an empty list. For more information
* about instance profiles, go to
* About Instance Profiles
* .
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* @param listInstanceProfilesRequest Container for the necessary
* parameters to execute the ListInstanceProfiles service method on
* AmazonIdentityManagement.
*
* @return The response from the ListInstanceProfiles service method, as
* returned by AmazonIdentityManagement.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListInstanceProfilesResult listInstanceProfiles(ListInstanceProfilesRequest listInstanceProfilesRequest) {
ExecutionContext executionContext = createExecutionContext(listInstanceProfilesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ListInstanceProfilesRequestMarshaller().marshall(listInstanceProfilesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ListInstanceProfilesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Changes the status of the specified access key from Active to
* Inactive, or vice versa. This action can be used to disable a user's
* key as part of a key rotation work flow.
*
*
* If the UserName
field is not specified, the UserName is
* determined implicitly based on the AWS access key ID used to sign the
* request. Because this action works for access keys under the AWS
* account, this API can be used to manage root credentials even if the
* AWS account has no associated users.
*
*
* For information about rotating keys, see
* Managing Keys and Certificates
* in Using AWS Identity and Access Management .
*
*
* @param updateAccessKeyRequest Container for the necessary parameters
* to execute the UpdateAccessKey service method on
* AmazonIdentityManagement.
*
*
* @throws NoSuchEntityException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void updateAccessKey(UpdateAccessKeyRequest updateAccessKeyRequest) {
ExecutionContext executionContext = createExecutionContext(updateAccessKeyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new UpdateAccessKeyRequestMarshaller().marshall(updateAccessKeyRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Adds the specified user to the specified group.
*
*
* @param addUserToGroupRequest Container for the necessary parameters to
* execute the AddUserToGroup service method on AmazonIdentityManagement.
*
*
* @throws NoSuchEntityException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void addUserToGroup(AddUserToGroupRequest addUserToGroupRequest) {
ExecutionContext executionContext = createExecutionContext(addUserToGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new AddUserToGroupRequestMarshaller().marshall(addUserToGroupRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Returns a list of users that are in the specified group. You can
* paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* @param getGroupRequest Container for the necessary parameters to
* execute the GetGroup service method on AmazonIdentityManagement.
*
* @return The response from the GetGroup service method, as returned by
* AmazonIdentityManagement.
*
* @throws NoSuchEntityException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public GetGroupResult getGroup(GetGroupRequest getGroupRequest) {
ExecutionContext executionContext = createExecutionContext(getGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new GetGroupRequestMarshaller().marshall(getGroupRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new GetGroupResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the account aliases associated with the account. For information
* about using an AWS account alias, see
* Using an Alias for Your AWS Account ID
* in Using AWS Identity and Access Management .
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* @param listAccountAliasesRequest Container for the necessary
* parameters to execute the ListAccountAliases service method on
* AmazonIdentityManagement.
*
* @return The response from the ListAccountAliases service method, as
* returned by AmazonIdentityManagement.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListAccountAliasesResult listAccountAliases(ListAccountAliasesRequest listAccountAliasesRequest) {
ExecutionContext executionContext = createExecutionContext(listAccountAliasesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ListAccountAliasesRequestMarshaller().marshall(listAccountAliasesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ListAccountAliasesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified group. The group must not contain any users or
* have any attached policies.
*
*
* @param deleteGroupRequest Container for the necessary parameters to
* execute the DeleteGroup service method on AmazonIdentityManagement.
*
*
* @throws NoSuchEntityException
* @throws DeleteConflictException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteGroup(DeleteGroupRequest deleteGroupRequest) {
ExecutionContext executionContext = createExecutionContext(deleteGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteGroupRequestMarshaller().marshall(deleteGroupRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Retrieves information about the specified role, including the role's
* path, GUID, ARN, and the policy granting permission to EC2 to assume
* the role. For more information about ARNs, go to ARNs. For more
* information about roles, go to
* Working with Roles
* .
*
*
* The returned policy is URL-encoded according to RFC 3986. For more
* information about RFC 3986, go to
* http://www.faqs.org/rfcs/rfc3986.html
* .
*
*
* @param getRoleRequest Container for the necessary parameters to
* execute the GetRole service method on AmazonIdentityManagement.
*
* @return The response from the GetRole service method, as returned by
* AmazonIdentityManagement.
*
* @throws NoSuchEntityException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public GetRoleResult getRole(GetRoleRequest getRoleRequest) {
ExecutionContext executionContext = createExecutionContext(getRoleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new GetRoleRequestMarshaller().marshall(getRoleRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new GetRoleResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the names of the policies associated with the specified role. If
* there are none, the action returns an empty list.
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* @param listRolePoliciesRequest Container for the necessary parameters
* to execute the ListRolePolicies service method on
* AmazonIdentityManagement.
*
* @return The response from the ListRolePolicies service method, as
* returned by AmazonIdentityManagement.
*
* @throws NoSuchEntityException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListRolePoliciesResult listRolePolicies(ListRolePoliciesRequest listRolePoliciesRequest) {
ExecutionContext executionContext = createExecutionContext(listRolePoliciesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ListRolePoliciesRequestMarshaller().marshall(listRolePoliciesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ListRolePoliciesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about the signing certificates associated with the
* specified user. If there are none, the action returns an empty list.
*
*
* Although each user is limited to a small number of signing
* certificates, you can still paginate the results using the
* MaxItems
and Marker
parameters.
*
*
* If the UserName
field is not specified, the user name is
* determined implicitly based on the AWS access key ID used to sign the
* request. Because this action works for access keys under the AWS
* account, this API can be used to manage root credentials even if the
* AWS account has no associated users.
*
*
* @param listSigningCertificatesRequest Container for the necessary
* parameters to execute the ListSigningCertificates service method on
* AmazonIdentityManagement.
*
* @return The response from the ListSigningCertificates service method,
* as returned by AmazonIdentityManagement.
*
* @throws NoSuchEntityException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListSigningCertificatesResult listSigningCertificates(ListSigningCertificatesRequest listSigningCertificatesRequest) {
ExecutionContext executionContext = createExecutionContext(listSigningCertificatesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ListSigningCertificatesRequestMarshaller().marshall(listSigningCertificatesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ListSigningCertificatesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Uploads an X.509 signing certificate and associates it with the
* specified user. Some AWS services use X.509 signing certificates to
* validate requests that are signed with a corresponding private key.
* When you upload the certificate, its default status is
* Active
.
*
*
* If the UserName
field is not specified, the user name is
* determined implicitly based on the AWS access key ID used to sign the
* request. Because this action works for access keys under the AWS
* account, this API can be used to manage root credentials even if the
* AWS account has no associated users.
*
*
* NOTE:Because the body of a X.509 certificate can be large, you
* should use POST rather than GET when calling UploadSigningCertificate.
* For information about setting up signatures and authorization through
* the API, go to Signing AWS API Requests in the AWS General Reference.
* For general information about using the Query API with IAM, go to
* Making Query Requests in Using IAM.
*
*
* @param uploadSigningCertificateRequest Container for the necessary
* parameters to execute the UploadSigningCertificate service method on
* AmazonIdentityManagement.
*
* @return The response from the UploadSigningCertificate service method,
* as returned by AmazonIdentityManagement.
*
* @throws DuplicateCertificateException
* @throws InvalidCertificateException
* @throws MalformedCertificateException
* @throws NoSuchEntityException
* @throws LimitExceededException
* @throws EntityAlreadyExistsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public UploadSigningCertificateResult uploadSigningCertificate(UploadSigningCertificateRequest uploadSigningCertificateRequest) {
ExecutionContext executionContext = createExecutionContext(uploadSigningCertificateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new UploadSigningCertificateRequestMarshaller().marshall(uploadSigningCertificateRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new UploadSigningCertificateResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified instance profile. The instance profile must not
* have an associated role.
*
*
* IMPORTANT:Make sure you do not have any Amazon EC2 instances
* running with the instance profile you are about to delete. Deleting a
* role or instance profile that is associated with a running instance
* will break any applications running on the instance.
*
*
* For more information about instance profiles, go to
* About Instance Profiles
* .
*
*
* @param deleteInstanceProfileRequest Container for the necessary
* parameters to execute the DeleteInstanceProfile service method on
* AmazonIdentityManagement.
*
*
* @throws NoSuchEntityException
* @throws DeleteConflictException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteInstanceProfile(DeleteInstanceProfileRequest deleteInstanceProfileRequest) {
ExecutionContext executionContext = createExecutionContext(deleteInstanceProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteInstanceProfileRequestMarshaller().marshall(deleteInstanceProfileRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Returns the SAML provider metadocument that was uploaded when the
* provider was created or updated.
*
*
* NOTE:This operation requires Signature Version 4.
*
*
* @param getSAMLProviderRequest Container for the necessary parameters
* to execute the GetSAMLProvider service method on
* AmazonIdentityManagement.
*
* @return The response from the GetSAMLProvider service method, as
* returned by AmazonIdentityManagement.
*
* @throws InvalidInputException
* @throws NoSuchEntityException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public GetSAMLProviderResult getSAMLProvider(GetSAMLProviderRequest getSAMLProviderRequest) {
ExecutionContext executionContext = createExecutionContext(getSAMLProviderRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new GetSAMLProviderRequestMarshaller().marshall(getSAMLProviderRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new GetSAMLProviderResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new role for your AWS account. For more information about
* roles, go to
* Working with Roles . For information about limitations on role names and the number of roles you can create, go to Limitations on IAM Entities
* in Using AWS Identity and Access Management .
*
*
* The policy grants permission to an EC2 instance to assume the role.
* The policy is URL-encoded according to RFC 3986. For more information
* about RFC 3986, go to
* http://www.faqs.org/rfcs/rfc3986.html
* . Currently, only EC2 instances can assume roles.
*
*
* @param createRoleRequest Container for the necessary parameters to
* execute the CreateRole service method on AmazonIdentityManagement.
*
* @return The response from the CreateRole service method, as returned
* by AmazonIdentityManagement.
*
* @throws MalformedPolicyDocumentException
* @throws LimitExceededException
* @throws EntityAlreadyExistsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateRoleResult createRole(CreateRoleRequest createRoleRequest) {
ExecutionContext executionContext = createExecutionContext(createRoleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateRoleRequestMarshaller().marshall(createRoleRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateRoleResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Changes the password for the specified user.
*
*
* @param updateLoginProfileRequest Container for the necessary
* parameters to execute the UpdateLoginProfile service method on
* AmazonIdentityManagement.
*
*
* @throws PasswordPolicyViolationException
* @throws EntityTemporarilyUnmodifiableException
* @throws NoSuchEntityException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void updateLoginProfile(UpdateLoginProfileRequest updateLoginProfileRequest) {
ExecutionContext executionContext = createExecutionContext(updateLoginProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new UpdateLoginProfileRequestMarshaller().marshall(updateLoginProfileRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Deletes the password for the specified user, which terminates the
* user's ability to access AWS services through the AWS Management
* Console.
*
*
* IMPORTANT:Deleting a user's password does not prevent a user
* from accessing IAM through the command line interface or the API. To
* prevent all user access you must also either make the access key
* inactive or delete it. For more information about making keys inactive
* or deleting them, see UpdateAccessKey and DeleteAccessKey.
*
*
* @param deleteLoginProfileRequest Container for the necessary
* parameters to execute the DeleteLoginProfile service method on
* AmazonIdentityManagement.
*
*
* @throws EntityTemporarilyUnmodifiableException
* @throws NoSuchEntityException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteLoginProfile(DeleteLoginProfileRequest deleteLoginProfileRequest) {
ExecutionContext executionContext = createExecutionContext(deleteLoginProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteLoginProfileRequestMarshaller().marshall(deleteLoginProfileRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Changes the password of the IAM user calling
* ChangePassword
. The root account password is not
* affected by this action. For information about modifying passwords,
* see
* Managing Passwords
* .
*
*
* @param changePasswordRequest Container for the necessary parameters to
* execute the ChangePassword service method on AmazonIdentityManagement.
*
*
* @throws EntityTemporarilyUnmodifiableException
* @throws NoSuchEntityException
* @throws LimitExceededException
* @throws InvalidUserTypeException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void changePassword(ChangePasswordRequest changePasswordRequest) {
ExecutionContext executionContext = createExecutionContext(changePasswordRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ChangePasswordRequestMarshaller().marshall(changePasswordRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Retrieves information about the specified server certificate.
*
*
* @param getServerCertificateRequest Container for the necessary
* parameters to execute the GetServerCertificate service method on
* AmazonIdentityManagement.
*
* @return The response from the GetServerCertificate service method, as
* returned by AmazonIdentityManagement.
*
* @throws NoSuchEntityException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public GetServerCertificateResult getServerCertificate(GetServerCertificateRequest getServerCertificateRequest) {
ExecutionContext executionContext = createExecutionContext(getServerCertificateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new GetServerCertificateRequestMarshaller().marshall(getServerCertificateRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new GetServerCertificateResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds (or updates) a policy document associated with the specified
* group. For information about policies, refer to
* Overview of Policies
* in Using AWS Identity and Access Management .
*
*
* For information about limits on the number of policies you can
* associate with a group, see
* Limitations on IAM Entities
* in Using AWS Identity and Access Management .
*
*
* NOTE:Because policy documents can be large, you should use POST
* rather than GET when calling PutGroupPolicy. For information about
* setting up signatures and authorization through the API, go to Signing
* AWS API Requests in the AWS General Reference. For general information
* about using the Query API with IAM, go to Making Query Requests in
* Using IAM.
*
*
* @param putGroupPolicyRequest Container for the necessary parameters to
* execute the PutGroupPolicy service method on AmazonIdentityManagement.
*
*
* @throws MalformedPolicyDocumentException
* @throws NoSuchEntityException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void putGroupPolicy(PutGroupPolicyRequest putGroupPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(putGroupPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new PutGroupPolicyRequestMarshaller().marshall(putGroupPolicyRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Deletes the specified signing certificate associated with the
* specified user.
*
*
* If you do not specify a user name, IAM determines the user name
* implicitly based on the AWS access key ID signing the request. Because
* this action works for access keys under the AWS account, you can use
* this API to manage root credentials even if the AWS account has no
* associated users.
*
*
* @param deleteSigningCertificateRequest Container for the necessary
* parameters to execute the DeleteSigningCertificate service method on
* AmazonIdentityManagement.
*
*
* @throws NoSuchEntityException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteSigningCertificate(DeleteSigningCertificateRequest deleteSigningCertificateRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSigningCertificateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteSigningCertificateRequestMarshaller().marshall(deleteSigningCertificateRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Lists the names of the policies associated with the specified user. If
* there are none, the action returns an empty list.
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* @param listUserPoliciesRequest Container for the necessary parameters
* to execute the ListUserPolicies service method on
* AmazonIdentityManagement.
*
* @return The response from the ListUserPolicies service method, as
* returned by AmazonIdentityManagement.
*
* @throws NoSuchEntityException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListUserPoliciesResult listUserPolicies(ListUserPoliciesRequest listUserPoliciesRequest) {
ExecutionContext executionContext = createExecutionContext(listUserPoliciesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ListUserPoliciesRequestMarshaller().marshall(listUserPoliciesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ListUserPoliciesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about the access key IDs associated with the
* specified user. If there are none, the action returns an empty list.
*
*
* Although each user is limited to a small number of keys, you can still
* paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* If the UserName
field is not specified, the UserName is
* determined implicitly based on the AWS access key ID used to sign the
* request. Because this action works for access keys under the AWS
* account, this API can be used to manage root credentials even if the
* AWS account has no associated users.
*
*
* NOTE:To ensure the security of your AWS account, the secret
* access key is accessible only during key and user creation.
*
*
* @param listAccessKeysRequest Container for the necessary parameters to
* execute the ListAccessKeys service method on AmazonIdentityManagement.
*
* @return The response from the ListAccessKeys service method, as
* returned by AmazonIdentityManagement.
*
* @throws NoSuchEntityException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListAccessKeysResult listAccessKeys(ListAccessKeysRequest listAccessKeysRequest) {
ExecutionContext executionContext = createExecutionContext(listAccessKeysRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ListAccessKeysRequestMarshaller().marshall(listAccessKeysRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ListAccessKeysResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the groups the specified user belongs to.
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* @param listGroupsForUserRequest Container for the necessary parameters
* to execute the ListGroupsForUser service method on
* AmazonIdentityManagement.
*
* @return The response from the ListGroupsForUser service method, as
* returned by AmazonIdentityManagement.
*
* @throws NoSuchEntityException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListGroupsForUserResult listGroupsForUser(ListGroupsForUserRequest listGroupsForUserRequest) {
ExecutionContext executionContext = createExecutionContext(listGroupsForUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ListGroupsForUserRequestMarshaller().marshall(listGroupsForUserRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ListGroupsForUserResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds the specified role to the specified instance profile. For more
* information about roles, go to
* Working with Roles . For more information about instance profiles, go to About Instance Profiles
* .
*
*
* @param addRoleToInstanceProfileRequest Container for the necessary
* parameters to execute the AddRoleToInstanceProfile service method on
* AmazonIdentityManagement.
*
*
* @throws NoSuchEntityException
* @throws LimitExceededException
* @throws EntityAlreadyExistsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void addRoleToInstanceProfile(AddRoleToInstanceProfileRequest addRoleToInstanceProfileRequest) {
ExecutionContext executionContext = createExecutionContext(addRoleToInstanceProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new AddRoleToInstanceProfileRequestMarshaller().marshall(addRoleToInstanceProfileRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Retrieves the specified policy document for the specified group. The
* returned policy is URL-encoded according to RFC 3986. For more
* information about RFC 3986, go to
* http://www.faqs.org/rfcs/rfc3986.html
* .
*
*
* @param getGroupPolicyRequest Container for the necessary parameters to
* execute the GetGroupPolicy service method on AmazonIdentityManagement.
*
* @return The response from the GetGroupPolicy service method, as
* returned by AmazonIdentityManagement.
*
* @throws NoSuchEntityException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public GetGroupPolicyResult getGroupPolicy(GetGroupPolicyRequest getGroupPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(getGroupPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new GetGroupPolicyRequestMarshaller().marshall(getGroupPolicyRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new GetGroupPolicyResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the specified policy document for the specified role. For
* more information about roles, go to
* Working with Roles
* .
*
*
* The returned policy is URL-encoded according to RFC 3986. For more
* information about RFC 3986, go to
* http://www.faqs.org/rfcs/rfc3986.html
* .
*
*
* @param getRolePolicyRequest Container for the necessary parameters to
* execute the GetRolePolicy service method on AmazonIdentityManagement.
*
* @return The response from the GetRolePolicy service method, as
* returned by AmazonIdentityManagement.
*
* @throws NoSuchEntityException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public GetRolePolicyResult getRolePolicy(GetRolePolicyRequest getRolePolicyRequest) {
ExecutionContext executionContext = createExecutionContext(getRolePolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new GetRolePolicyRequestMarshaller().marshall(getRolePolicyRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new GetRolePolicyResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the instance profiles that have the specified associated role.
* If there are none, the action returns an empty list. For more
* information about instance profiles, go to
* About Instance Profiles
* .
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* @param listInstanceProfilesForRoleRequest Container for the necessary
* parameters to execute the ListInstanceProfilesForRole service method
* on AmazonIdentityManagement.
*
* @return The response from the ListInstanceProfilesForRole service
* method, as returned by AmazonIdentityManagement.
*
* @throws NoSuchEntityException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListInstanceProfilesForRoleResult listInstanceProfilesForRole(ListInstanceProfilesForRoleRequest listInstanceProfilesForRoleRequest) {
ExecutionContext executionContext = createExecutionContext(listInstanceProfilesForRoleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ListInstanceProfilesForRoleRequestMarshaller().marshall(listInstanceProfilesForRoleRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ListInstanceProfilesForRoleResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the virtual MFA devices under the AWS account by assignment
* status. If you do not specify an assignment status, the action returns
* a list of all virtual MFA devices. Assignment status can be
* Assigned
,
* Unassigned
, or Any
.
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* @param listVirtualMFADevicesRequest Container for the necessary
* parameters to execute the ListVirtualMFADevices service method on
* AmazonIdentityManagement.
*
* @return The response from the ListVirtualMFADevices service method, as
* returned by AmazonIdentityManagement.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListVirtualMFADevicesResult listVirtualMFADevices(ListVirtualMFADevicesRequest listVirtualMFADevicesRequest) {
ExecutionContext executionContext = createExecutionContext(listVirtualMFADevicesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ListVirtualMFADevicesRequestMarshaller().marshall(listVirtualMFADevicesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ListVirtualMFADevicesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified policy associated with the specified role.
*
*
* @param deleteRolePolicyRequest Container for the necessary parameters
* to execute the DeleteRolePolicy service method on
* AmazonIdentityManagement.
*
*
* @throws NoSuchEntityException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteRolePolicy(DeleteRolePolicyRequest deleteRolePolicyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteRolePolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteRolePolicyRequestMarshaller().marshall(deleteRolePolicyRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Creates a new instance profile. For information about instance
* profiles, go to
* About Instance Profiles
* .
*
*
* For information about the number of instance profiles you can create,
* see
* Limitations on IAM Entities
* in Using AWS Identity and Access Management .
*
*
* @param createInstanceProfileRequest Container for the necessary
* parameters to execute the CreateInstanceProfile service method on
* AmazonIdentityManagement.
*
* @return The response from the CreateInstanceProfile service method, as
* returned by AmazonIdentityManagement.
*
* @throws LimitExceededException
* @throws EntityAlreadyExistsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateInstanceProfileResult createInstanceProfile(CreateInstanceProfileRequest createInstanceProfileRequest) {
ExecutionContext executionContext = createExecutionContext(createInstanceProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateInstanceProfileRequestMarshaller().marshall(createInstanceProfileRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateInstanceProfileResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the names of the policies associated with the specified group.
* If there are none, the action returns an empty list.
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* @param listGroupPoliciesRequest Container for the necessary parameters
* to execute the ListGroupPolicies service method on
* AmazonIdentityManagement.
*
* @return The response from the ListGroupPolicies service method, as
* returned by AmazonIdentityManagement.
*
* @throws NoSuchEntityException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListGroupPoliciesResult listGroupPolicies(ListGroupPoliciesRequest listGroupPoliciesRequest) {
ExecutionContext executionContext = createExecutionContext(listGroupPoliciesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ListGroupPoliciesRequestMarshaller().marshall(listGroupPoliciesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ListGroupPoliciesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a password for the specified user, giving the user the ability
* to access AWS services through the AWS Management Console. For more
* information about managing passwords, see
* Managing Passwords
* in Using IAM .
*
*
* @param createLoginProfileRequest Container for the necessary
* parameters to execute the CreateLoginProfile service method on
* AmazonIdentityManagement.
*
* @return The response from the CreateLoginProfile service method, as
* returned by AmazonIdentityManagement.
*
* @throws PasswordPolicyViolationException
* @throws NoSuchEntityException
* @throws LimitExceededException
* @throws EntityAlreadyExistsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateLoginProfileResult createLoginProfile(CreateLoginProfileRequest createLoginProfileRequest) {
ExecutionContext executionContext = createExecutionContext(createLoginProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateLoginProfileRequestMarshaller().marshall(createLoginProfileRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateLoginProfileResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes the specified role from the specified instance profile.
*
*
* IMPORTANT:Make sure you do not have any Amazon EC2 instances
* running with the role you are about to remove from the instance
* profile. Removing a role from an instance profile that is associated
* with a running instance will break any applications running on the
* instance.
*
*
* For more information about roles, go to
* Working with Roles . For more information about instance profiles, go to About Instance Profiles
* .
*
*
* @param removeRoleFromInstanceProfileRequest Container for the
* necessary parameters to execute the RemoveRoleFromInstanceProfile
* service method on AmazonIdentityManagement.
*
*
* @throws NoSuchEntityException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void removeRoleFromInstanceProfile(RemoveRoleFromInstanceProfileRequest removeRoleFromInstanceProfileRequest) {
ExecutionContext executionContext = createExecutionContext(removeRoleFromInstanceProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new RemoveRoleFromInstanceProfileRequestMarshaller().marshall(removeRoleFromInstanceProfileRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Updates the password policy settings for the account. For more
* information about using a password policy, go to
* Managing an IAM Password Policy
* .
*
*
* @param updateAccountPasswordPolicyRequest Container for the necessary
* parameters to execute the UpdateAccountPasswordPolicy service method
* on AmazonIdentityManagement.
*
*
* @throws MalformedPolicyDocumentException
* @throws NoSuchEntityException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void updateAccountPasswordPolicy(UpdateAccountPasswordPolicyRequest updateAccountPasswordPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(updateAccountPasswordPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new UpdateAccountPasswordPolicyRequestMarshaller().marshall(updateAccountPasswordPolicyRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Updates the policy that grants an entity permission to assume a role.
* Currently, only an Amazon EC2 instance can assume a role. For more
* information about roles, go to
* Working with Roles
* .
*
*
* @param updateAssumeRolePolicyRequest Container for the necessary
* parameters to execute the UpdateAssumeRolePolicy service method on
* AmazonIdentityManagement.
*
*
* @throws MalformedPolicyDocumentException
* @throws NoSuchEntityException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void updateAssumeRolePolicy(UpdateAssumeRolePolicyRequest updateAssumeRolePolicyRequest) {
ExecutionContext executionContext = createExecutionContext(updateAssumeRolePolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new UpdateAssumeRolePolicyRequestMarshaller().marshall(updateAssumeRolePolicyRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Retrieves information about the specified instance profile, including
* the instance profile's path, GUID, ARN, and role. For more information
* about instance profiles, go to
* About Instance Profiles . For more information about ARNs, go to ARNs
* .
*
*
* @param getInstanceProfileRequest Container for the necessary
* parameters to execute the GetInstanceProfile service method on
* AmazonIdentityManagement.
*
* @return The response from the GetInstanceProfile service method, as
* returned by AmazonIdentityManagement.
*
* @throws NoSuchEntityException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public GetInstanceProfileResult getInstanceProfile(GetInstanceProfileRequest getInstanceProfileRequest) {
ExecutionContext executionContext = createExecutionContext(getInstanceProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new GetInstanceProfileRequestMarshaller().marshall(getInstanceProfileRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new GetInstanceProfileResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the roles that have the specified path prefix. If there are
* none, the action returns an empty list. For more information about
* roles, go to
* Working with Roles
* .
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* The returned policy is URL-encoded according to RFC 3986. For more
* information about RFC 3986, go to
* http://www.faqs.org/rfcs/rfc3986.html
* .
*
*
* @param listRolesRequest Container for the necessary parameters to
* execute the ListRoles service method on AmazonIdentityManagement.
*
* @return The response from the ListRoles service method, as returned by
* AmazonIdentityManagement.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListRolesResult listRoles(ListRolesRequest listRolesRequest) {
ExecutionContext executionContext = createExecutionContext(listRolesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ListRolesRequestMarshaller().marshall(listRolesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ListRolesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves account level information about account entity usage and IAM
* quotas.
*
*
* For information about limitations on IAM entities, see
* Limitations on IAM Entities
* in Using AWS Identity and Access Management .
*
*
* @param getAccountSummaryRequest Container for the necessary parameters
* to execute the GetAccountSummary service method on
* AmazonIdentityManagement.
*
* @return The response from the GetAccountSummary service method, as
* returned by AmazonIdentityManagement.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public GetAccountSummaryResult getAccountSummary(GetAccountSummaryRequest getAccountSummaryRequest) {
ExecutionContext executionContext = createExecutionContext(getAccountSummaryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new GetAccountSummaryRequestMarshaller().marshall(getAccountSummaryRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new GetAccountSummaryResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an IAM entity to describe an identity provider (IdP) that
* supports SAML 2.0.
*
*
* The SAML provider that you create with this operation can be used as a
* principal in a role's trust policy to establish a trust relationship
* between AWS and a SAML identity provider. You can create an IAM role
* that supports Web-based single sign-on (SSO) to the AWS Management
* Console or one that supports API access to AWS.
*
*
* When you create the SAML provider, you upload an a SAML metadata
* document that you get from your IdP and that includes the issuer's
* name, expiration information, and keys that can be used to validate
* the SAML authentication response (assertions) that are received from
* the IdP. You must generate the metadata document using the identity
* management software that is used as your organization's IdP.
*
*
* NOTE:This operation requires Signature Version 4.
*
*
* For more information, see
* Giving Console Access Using SAML and Creating Temporary Security Credentials for SAML Federation
* in the Using Temporary Credentials guide.
*
*
* @param createSAMLProviderRequest Container for the necessary
* parameters to execute the CreateSAMLProvider service method on
* AmazonIdentityManagement.
*
* @return The response from the CreateSAMLProvider service method, as
* returned by AmazonIdentityManagement.
*
* @throws InvalidInputException
* @throws LimitExceededException
* @throws EntityAlreadyExistsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateSAMLProviderResult createSAMLProvider(CreateSAMLProviderRequest createSAMLProviderRequest) {
ExecutionContext executionContext = createExecutionContext(createSAMLProviderRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateSAMLProviderRequestMarshaller().marshall(createSAMLProviderRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateSAMLProviderResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the password policy for the AWS account. For more
* information about using a password policy, go to
* Managing an IAM Password Policy
* .
*
*
* @param getAccountPasswordPolicyRequest Container for the necessary
* parameters to execute the GetAccountPasswordPolicy service method on
* AmazonIdentityManagement.
*
* @return The response from the GetAccountPasswordPolicy service method,
* as returned by AmazonIdentityManagement.
*
* @throws NoSuchEntityException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public GetAccountPasswordPolicyResult getAccountPasswordPolicy(GetAccountPasswordPolicyRequest getAccountPasswordPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(getAccountPasswordPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new GetAccountPasswordPolicyRequestMarshaller().marshall(getAccountPasswordPolicyRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new GetAccountPasswordPolicyResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the groups that have the specified path prefix.
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* @return The response from the ListGroups service method, as returned
* by AmazonIdentityManagement.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListGroupsResult listGroups() throws AmazonServiceException, AmazonClientException {
return listGroups(new ListGroupsRequest());
}
/**
*
* Lists the server certificates that have the specified path prefix. If
* none exist, the action returns an empty list.
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* @return The response from the ListServerCertificates service method,
* as returned by AmazonIdentityManagement.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListServerCertificatesResult listServerCertificates() throws AmazonServiceException, AmazonClientException {
return listServerCertificates(new ListServerCertificatesRequest());
}
/**
*
* Lists the SAML providers in the account.
*
*
* NOTE:This operation requires Signature Version 4.
*
*
* @return The response from the ListSAMLProviders service method, as
* returned by AmazonIdentityManagement.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListSAMLProvidersResult listSAMLProviders() throws AmazonServiceException, AmazonClientException {
return listSAMLProviders(new ListSAMLProvidersRequest());
}
/**
*
* Lists the users that have the specified path prefix. If there are
* none, the action returns an empty list.
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* @return The response from the ListUsers service method, as returned by
* AmazonIdentityManagement.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListUsersResult listUsers() throws AmazonServiceException, AmazonClientException {
return listUsers(new ListUsersRequest());
}
/**
*
* Deletes the password policy for the AWS account.
*
*
*
* @throws NoSuchEntityException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteAccountPasswordPolicy() throws AmazonServiceException, AmazonClientException {
deleteAccountPasswordPolicy(new DeleteAccountPasswordPolicyRequest());
}
/**
*
* Creates a new AWS secret access key and corresponding AWS access key
* ID for the specified user. The default status for new keys is
* Active
.
*
*
* If you do not specify a user name, IAM determines the user name
* implicitly based on the AWS access key ID signing the request. Because
* this action works for access keys under the AWS account, you can use
* this API to manage root credentials even if the AWS account has no
* associated users.
*
*
* For information about limits on the number of keys you can create, see
* Limitations on IAM Entities
* in Using AWS Identity and Access Management .
*
*
* IMPORTANT:To ensure the security of your AWS account, the
* secret access key is accessible only during key and user creation. You
* must save the key (for example, in a text file) if you want to be able
* to access it again. If a secret key is lost, you can delete the access
* keys for the associated user and then create new keys.
*
*
* @return The response from the CreateAccessKey service method, as
* returned by AmazonIdentityManagement.
*
* @throws NoSuchEntityException
* @throws LimitExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateAccessKeyResult createAccessKey() throws AmazonServiceException, AmazonClientException {
return createAccessKey(new CreateAccessKeyRequest());
}
/**
*
* Retrieves information about the specified user, including the user's
* path, unique ID, and ARN.
*
*
* If you do not specify a user name, IAM determines the user name
* implicitly based on the AWS access key ID signing the request.
*
*
* @return The response from the GetUser service method, as returned by
* AmazonIdentityManagement.
*
* @throws NoSuchEntityException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public GetUserResult getUser() throws AmazonServiceException, AmazonClientException {
return getUser(new GetUserRequest());
}
/**
*
* Lists the MFA devices. If the request includes the user name, then
* this action lists all the MFA devices associated with the specified
* user name. If you do not specify a user name, IAM determines the user
* name implicitly based on the AWS access key ID signing the request.
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* @return The response from the ListMFADevices service method, as
* returned by AmazonIdentityManagement.
*
* @throws NoSuchEntityException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListMFADevicesResult listMFADevices() throws AmazonServiceException, AmazonClientException {
return listMFADevices(new ListMFADevicesRequest());
}
/**
*
* Lists the instance profiles that have the specified path prefix. If
* there are none, the action returns an empty list. For more information
* about instance profiles, go to
* About Instance Profiles
* .
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* @return The response from the ListInstanceProfiles service method, as
* returned by AmazonIdentityManagement.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListInstanceProfilesResult listInstanceProfiles() throws AmazonServiceException, AmazonClientException {
return listInstanceProfiles(new ListInstanceProfilesRequest());
}
/**
*
* Lists the account aliases associated with the account. For information
* about using an AWS account alias, see
* Using an Alias for Your AWS Account ID
* in Using AWS Identity and Access Management .
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* @return The response from the ListAccountAliases service method, as
* returned by AmazonIdentityManagement.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListAccountAliasesResult listAccountAliases() throws AmazonServiceException, AmazonClientException {
return listAccountAliases(new ListAccountAliasesRequest());
}
/**
*
* Returns information about the signing certificates associated with the
* specified user. If there are none, the action returns an empty list.
*
*
* Although each user is limited to a small number of signing
* certificates, you can still paginate the results using the
* MaxItems
and Marker
parameters.
*
*
* If the UserName
field is not specified, the user name is
* determined implicitly based on the AWS access key ID used to sign the
* request. Because this action works for access keys under the AWS
* account, this API can be used to manage root credentials even if the
* AWS account has no associated users.
*
*
* @return The response from the ListSigningCertificates service method,
* as returned by AmazonIdentityManagement.
*
* @throws NoSuchEntityException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListSigningCertificatesResult listSigningCertificates() throws AmazonServiceException, AmazonClientException {
return listSigningCertificates(new ListSigningCertificatesRequest());
}
/**
*
* Returns information about the access key IDs associated with the
* specified user. If there are none, the action returns an empty list.
*
*
* Although each user is limited to a small number of keys, you can still
* paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* If the UserName
field is not specified, the UserName is
* determined implicitly based on the AWS access key ID used to sign the
* request. Because this action works for access keys under the AWS
* account, this API can be used to manage root credentials even if the
* AWS account has no associated users.
*
*
* NOTE:To ensure the security of your AWS account, the secret
* access key is accessible only during key and user creation.
*
*
* @return The response from the ListAccessKeys service method, as
* returned by AmazonIdentityManagement.
*
* @throws NoSuchEntityException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListAccessKeysResult listAccessKeys() throws AmazonServiceException, AmazonClientException {
return listAccessKeys(new ListAccessKeysRequest());
}
/**
*
* Lists the virtual MFA devices under the AWS account by assignment
* status. If you do not specify an assignment status, the action returns
* a list of all virtual MFA devices. Assignment status can be
* Assigned
,
* Unassigned
, or Any
.
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* @return The response from the ListVirtualMFADevices service method, as
* returned by AmazonIdentityManagement.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListVirtualMFADevicesResult listVirtualMFADevices() throws AmazonServiceException, AmazonClientException {
return listVirtualMFADevices(new ListVirtualMFADevicesRequest());
}
/**
*
* Lists the roles that have the specified path prefix. If there are
* none, the action returns an empty list. For more information about
* roles, go to
* Working with Roles
* .
*
*
* You can paginate the results using the MaxItems
and
* Marker
parameters.
*
*
* The returned policy is URL-encoded according to RFC 3986. For more
* information about RFC 3986, go to
* http://www.faqs.org/rfcs/rfc3986.html
* .
*
*
* @return The response from the ListRoles service method, as returned by
* AmazonIdentityManagement.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListRolesResult listRoles() throws AmazonServiceException, AmazonClientException {
return listRoles(new ListRolesRequest());
}
/**
*
* Retrieves account level information about account entity usage and IAM
* quotas.
*
*
* For information about limitations on IAM entities, see
* Limitations on IAM Entities
* in Using AWS Identity and Access Management .
*
*
* @return The response from the GetAccountSummary service method, as
* returned by AmazonIdentityManagement.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public GetAccountSummaryResult getAccountSummary() throws AmazonServiceException, AmazonClientException {
return getAccountSummary(new GetAccountSummaryRequest());
}
/**
*
* Retrieves the password policy for the AWS account. For more
* information about using a password policy, go to
* Managing an IAM Password Policy
* .
*
*
* @return The response from the GetAccountPasswordPolicy service method,
* as returned by AmazonIdentityManagement.
*
* @throws NoSuchEntityException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonIdentityManagement indicating
* either a problem with the data in the request, or a server side issue.
*/
public GetAccountPasswordPolicyResult getAccountPasswordPolicy() throws AmazonServiceException, AmazonClientException {
return getAccountPasswordPolicy(new GetAccountPasswordPolicyRequest());
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for
* debugging issues where a service isn't acting as expected. This data isn't considered part
* of the result data returned by an operation, so it's available through this separate,
* diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access
* this extra diagnostic information for an executed request, you should use this method
* to retrieve it as soon as possible after executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none
* is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
private Response invoke(Request request,
Unmarshaller unmarshaller,
ExecutionContext executionContext)
{
request.setEndpoint(endpoint);
request.setTimeOffset(timeOffset);
AmazonWebServiceRequest originalRequest = request.getOriginalRequest();
for (Entry entry : originalRequest.copyPrivateRequestParameters().entrySet()) {
request.addParameter(entry.getKey(), entry.getValue());
}
AWSCredentials credentials = awsCredentialsProvider.getCredentials();
if (originalRequest.getRequestCredentials() != null) {
credentials = originalRequest.getRequestCredentials();
}
executionContext.setCredentials(credentials);
StaxResponseHandler responseHandler = new StaxResponseHandler(unmarshaller);
DefaultErrorResponseHandler errorResponseHandler = new DefaultErrorResponseHandler(exceptionUnmarshallers);
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
}