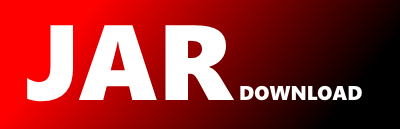
com.amazonaws.services.identitymanagement.model.ListGroupsForUserResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.identitymanagement.model;
import java.io.Serializable;
/**
*
* Contains the result of a successful invocation of the
* ListGroupsForUser action.
*
*/
public class ListGroupsForUserResult implements Serializable {
/**
* A list of groups.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag groups;
/**
* A flag that indicates whether there are more groups to list. If your
* results were truncated, you can make a subsequent pagination request
* using the Marker
request parameter to retrieve more
* groups in the list.
*/
private Boolean isTruncated;
/**
* If IsTruncated
is true
, this element is
* present and contains the value to use for the Marker
* parameter in a subsequent pagination request.
*
* Constraints:
* Length: 1 - 320
* Pattern: [\u0020-\u00FF]*
*/
private String marker;
/**
* A list of groups.
*
* @return A list of groups.
*/
public java.util.List getGroups() {
if (groups == null) {
groups = new com.amazonaws.internal.ListWithAutoConstructFlag();
groups.setAutoConstruct(true);
}
return groups;
}
/**
* A list of groups.
*
* @param groups A list of groups.
*/
public void setGroups(java.util.Collection groups) {
if (groups == null) {
this.groups = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag groupsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(groups.size());
groupsCopy.addAll(groups);
this.groups = groupsCopy;
}
/**
* A list of groups.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param groups A list of groups.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public ListGroupsForUserResult withGroups(Group... groups) {
if (getGroups() == null) setGroups(new java.util.ArrayList(groups.length));
for (Group value : groups) {
getGroups().add(value);
}
return this;
}
/**
* A list of groups.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param groups A list of groups.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public ListGroupsForUserResult withGroups(java.util.Collection groups) {
if (groups == null) {
this.groups = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag groupsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(groups.size());
groupsCopy.addAll(groups);
this.groups = groupsCopy;
}
return this;
}
/**
* A flag that indicates whether there are more groups to list. If your
* results were truncated, you can make a subsequent pagination request
* using the Marker
request parameter to retrieve more
* groups in the list.
*
* @return A flag that indicates whether there are more groups to list. If your
* results were truncated, you can make a subsequent pagination request
* using the Marker
request parameter to retrieve more
* groups in the list.
*/
public Boolean isTruncated() {
return isTruncated;
}
/**
* A flag that indicates whether there are more groups to list. If your
* results were truncated, you can make a subsequent pagination request
* using the Marker
request parameter to retrieve more
* groups in the list.
*
* @param isTruncated A flag that indicates whether there are more groups to list. If your
* results were truncated, you can make a subsequent pagination request
* using the Marker
request parameter to retrieve more
* groups in the list.
*/
public void setIsTruncated(Boolean isTruncated) {
this.isTruncated = isTruncated;
}
/**
* A flag that indicates whether there are more groups to list. If your
* results were truncated, you can make a subsequent pagination request
* using the Marker
request parameter to retrieve more
* groups in the list.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param isTruncated A flag that indicates whether there are more groups to list. If your
* results were truncated, you can make a subsequent pagination request
* using the Marker
request parameter to retrieve more
* groups in the list.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public ListGroupsForUserResult withIsTruncated(Boolean isTruncated) {
this.isTruncated = isTruncated;
return this;
}
/**
* A flag that indicates whether there are more groups to list. If your
* results were truncated, you can make a subsequent pagination request
* using the Marker
request parameter to retrieve more
* groups in the list.
*
* @return A flag that indicates whether there are more groups to list. If your
* results were truncated, you can make a subsequent pagination request
* using the Marker
request parameter to retrieve more
* groups in the list.
*/
public Boolean getIsTruncated() {
return isTruncated;
}
/**
* If IsTruncated
is true
, this element is
* present and contains the value to use for the Marker
* parameter in a subsequent pagination request.
*
* Constraints:
* Length: 1 - 320
* Pattern: [\u0020-\u00FF]*
*
* @return If IsTruncated
is true
, this element is
* present and contains the value to use for the Marker
* parameter in a subsequent pagination request.
*/
public String getMarker() {
return marker;
}
/**
* If IsTruncated
is true
, this element is
* present and contains the value to use for the Marker
* parameter in a subsequent pagination request.
*
* Constraints:
* Length: 1 - 320
* Pattern: [\u0020-\u00FF]*
*
* @param marker If IsTruncated
is true
, this element is
* present and contains the value to use for the Marker
* parameter in a subsequent pagination request.
*/
public void setMarker(String marker) {
this.marker = marker;
}
/**
* If IsTruncated
is true
, this element is
* present and contains the value to use for the Marker
* parameter in a subsequent pagination request.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 320
* Pattern: [\u0020-\u00FF]*
*
* @param marker If IsTruncated
is true
, this element is
* present and contains the value to use for the Marker
* parameter in a subsequent pagination request.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public ListGroupsForUserResult withMarker(String marker) {
this.marker = marker;
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getGroups() != null) sb.append("Groups: " + getGroups() + ",");
if (isTruncated() != null) sb.append("IsTruncated: " + isTruncated() + ",");
if (getMarker() != null) sb.append("Marker: " + getMarker() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getGroups() == null) ? 0 : getGroups().hashCode());
hashCode = prime * hashCode + ((isTruncated() == null) ? 0 : isTruncated().hashCode());
hashCode = prime * hashCode + ((getMarker() == null) ? 0 : getMarker().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof ListGroupsForUserResult == false) return false;
ListGroupsForUserResult other = (ListGroupsForUserResult)obj;
if (other.getGroups() == null ^ this.getGroups() == null) return false;
if (other.getGroups() != null && other.getGroups().equals(this.getGroups()) == false) return false;
if (other.isTruncated() == null ^ this.isTruncated() == null) return false;
if (other.isTruncated() != null && other.isTruncated().equals(this.isTruncated()) == false) return false;
if (other.getMarker() == null ^ this.getMarker() == null) return false;
if (other.getMarker() != null && other.getMarker().equals(this.getMarker()) == false) return false;
return true;
}
}