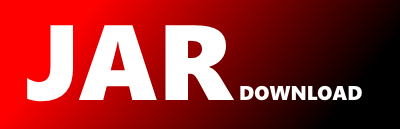
com.amazonaws.services.identitymanagement.model.Role Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.identitymanagement.model;
import java.io.Serializable;
/**
*
* The Role data type contains information about a role.
*
*
* This data type is used as a response element in the following
* actions:
*
*
*
* -
* CreateRole
*
*
* -
* GetRole
*
*
* -
* ListRoles
*
*
*
*
*/
public class Role implements Serializable {
/**
* Path to the role. For more information about paths, see Identifiers for IAM Entities in Using AWS
* Identity and Access Management.
*
* Constraints:
* Length: 1 - 512
* Pattern: (\u002F)|(\u002F[\u0021-\u007F]+\u002F)
*/
private String path;
/**
* The name identifying the role.
*
* Constraints:
* Length: 1 - 64
* Pattern: [\w+=,.@-]*
*/
private String roleName;
/**
* The stable and unique string identifying the role. For more
* information about IDs, see Identifiers for IAM Entities in Using AWS
* Identity and Access Management.
*
* Constraints:
* Length: 16 - 32
* Pattern: [\w]*
*/
private String roleId;
/**
* The Amazon Resource Name (ARN) specifying the role. For more
* information about ARNs and how to use them in policies, see Identifiers for IAM Entities in Using AWS
* Identity and Access Management.
*
* Constraints:
* Length: 20 - 2048
*/
private String arn;
/**
* The date when the role was created.
*/
private java.util.Date createDate;
/**
* The policy that grants an entity permission to assume the role.
The
* returned policy is URL-encoded according to RFC 3986. For more
* information about RFC 3986, go to http://www.faqs.org/rfcs/rfc3986.html.
*
* Constraints:
* Length: 1 - 131072
* Pattern: [\u0009\u000A\u000D\u0020-\u00FF]+
*/
private String assumeRolePolicyDocument;
/**
* Path to the role. For more information about paths, see Identifiers for IAM Entities in Using AWS
* Identity and Access Management.
*
* Constraints:
* Length: 1 - 512
* Pattern: (\u002F)|(\u002F[\u0021-\u007F]+\u002F)
*
* @return Path to the role. For more information about paths, see Identifiers for IAM Entities in Using AWS
* Identity and Access Management.
*/
public String getPath() {
return path;
}
/**
* Path to the role. For more information about paths, see Identifiers for IAM Entities in Using AWS
* Identity and Access Management.
*
* Constraints:
* Length: 1 - 512
* Pattern: (\u002F)|(\u002F[\u0021-\u007F]+\u002F)
*
* @param path Path to the role. For more information about paths, see Identifiers for IAM Entities in Using AWS
* Identity and Access Management.
*/
public void setPath(String path) {
this.path = path;
}
/**
* Path to the role. For more information about paths, see Identifiers for IAM Entities in Using AWS
* Identity and Access Management.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 512
* Pattern: (\u002F)|(\u002F[\u0021-\u007F]+\u002F)
*
* @param path Path to the role. For more information about paths, see Identifiers for IAM Entities in Using AWS
* Identity and Access Management.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Role withPath(String path) {
this.path = path;
return this;
}
/**
* The name identifying the role.
*
* Constraints:
* Length: 1 - 64
* Pattern: [\w+=,.@-]*
*
* @return The name identifying the role.
*/
public String getRoleName() {
return roleName;
}
/**
* The name identifying the role.
*
* Constraints:
* Length: 1 - 64
* Pattern: [\w+=,.@-]*
*
* @param roleName The name identifying the role.
*/
public void setRoleName(String roleName) {
this.roleName = roleName;
}
/**
* The name identifying the role.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 64
* Pattern: [\w+=,.@-]*
*
* @param roleName The name identifying the role.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Role withRoleName(String roleName) {
this.roleName = roleName;
return this;
}
/**
* The stable and unique string identifying the role. For more
* information about IDs, see Identifiers for IAM Entities in Using AWS
* Identity and Access Management.
*
* Constraints:
* Length: 16 - 32
* Pattern: [\w]*
*
* @return The stable and unique string identifying the role. For more
* information about IDs, see Identifiers for IAM Entities in Using AWS
* Identity and Access Management.
*/
public String getRoleId() {
return roleId;
}
/**
* The stable and unique string identifying the role. For more
* information about IDs, see Identifiers for IAM Entities in Using AWS
* Identity and Access Management.
*
* Constraints:
* Length: 16 - 32
* Pattern: [\w]*
*
* @param roleId The stable and unique string identifying the role. For more
* information about IDs, see Identifiers for IAM Entities in Using AWS
* Identity and Access Management.
*/
public void setRoleId(String roleId) {
this.roleId = roleId;
}
/**
* The stable and unique string identifying the role. For more
* information about IDs, see Identifiers for IAM Entities in Using AWS
* Identity and Access Management.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 16 - 32
* Pattern: [\w]*
*
* @param roleId The stable and unique string identifying the role. For more
* information about IDs, see Identifiers for IAM Entities in Using AWS
* Identity and Access Management.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Role withRoleId(String roleId) {
this.roleId = roleId;
return this;
}
/**
* The Amazon Resource Name (ARN) specifying the role. For more
* information about ARNs and how to use them in policies, see Identifiers for IAM Entities in Using AWS
* Identity and Access Management.
*
* Constraints:
* Length: 20 - 2048
*
* @return The Amazon Resource Name (ARN) specifying the role. For more
* information about ARNs and how to use them in policies, see Identifiers for IAM Entities in Using AWS
* Identity and Access Management.
*/
public String getArn() {
return arn;
}
/**
* The Amazon Resource Name (ARN) specifying the role. For more
* information about ARNs and how to use them in policies, see Identifiers for IAM Entities in Using AWS
* Identity and Access Management.
*
* Constraints:
* Length: 20 - 2048
*
* @param arn The Amazon Resource Name (ARN) specifying the role. For more
* information about ARNs and how to use them in policies, see Identifiers for IAM Entities in Using AWS
* Identity and Access Management.
*/
public void setArn(String arn) {
this.arn = arn;
}
/**
* The Amazon Resource Name (ARN) specifying the role. For more
* information about ARNs and how to use them in policies, see Identifiers for IAM Entities in Using AWS
* Identity and Access Management.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 20 - 2048
*
* @param arn The Amazon Resource Name (ARN) specifying the role. For more
* information about ARNs and how to use them in policies, see Identifiers for IAM Entities in Using AWS
* Identity and Access Management.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Role withArn(String arn) {
this.arn = arn;
return this;
}
/**
* The date when the role was created.
*
* @return The date when the role was created.
*/
public java.util.Date getCreateDate() {
return createDate;
}
/**
* The date when the role was created.
*
* @param createDate The date when the role was created.
*/
public void setCreateDate(java.util.Date createDate) {
this.createDate = createDate;
}
/**
* The date when the role was created.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param createDate The date when the role was created.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Role withCreateDate(java.util.Date createDate) {
this.createDate = createDate;
return this;
}
/**
* The policy that grants an entity permission to assume the role.
The
* returned policy is URL-encoded according to RFC 3986. For more
* information about RFC 3986, go to http://www.faqs.org/rfcs/rfc3986.html.
*
* Constraints:
* Length: 1 - 131072
* Pattern: [\u0009\u000A\u000D\u0020-\u00FF]+
*
* @return The policy that grants an entity permission to assume the role.
The
* returned policy is URL-encoded according to RFC 3986. For more
* information about RFC 3986, go to http://www.faqs.org/rfcs/rfc3986.html.
*/
public String getAssumeRolePolicyDocument() {
return assumeRolePolicyDocument;
}
/**
* The policy that grants an entity permission to assume the role.
The
* returned policy is URL-encoded according to RFC 3986. For more
* information about RFC 3986, go to http://www.faqs.org/rfcs/rfc3986.html.
*
* Constraints:
* Length: 1 - 131072
* Pattern: [\u0009\u000A\u000D\u0020-\u00FF]+
*
* @param assumeRolePolicyDocument The policy that grants an entity permission to assume the role.
The
* returned policy is URL-encoded according to RFC 3986. For more
* information about RFC 3986, go to http://www.faqs.org/rfcs/rfc3986.html.
*/
public void setAssumeRolePolicyDocument(String assumeRolePolicyDocument) {
this.assumeRolePolicyDocument = assumeRolePolicyDocument;
}
/**
* The policy that grants an entity permission to assume the role.
The
* returned policy is URL-encoded according to RFC 3986. For more
* information about RFC 3986, go to http://www.faqs.org/rfcs/rfc3986.html.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 131072
* Pattern: [\u0009\u000A\u000D\u0020-\u00FF]+
*
* @param assumeRolePolicyDocument The policy that grants an entity permission to assume the role.
The
* returned policy is URL-encoded according to RFC 3986. For more
* information about RFC 3986, go to http://www.faqs.org/rfcs/rfc3986.html.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Role withAssumeRolePolicyDocument(String assumeRolePolicyDocument) {
this.assumeRolePolicyDocument = assumeRolePolicyDocument;
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getPath() != null) sb.append("Path: " + getPath() + ",");
if (getRoleName() != null) sb.append("RoleName: " + getRoleName() + ",");
if (getRoleId() != null) sb.append("RoleId: " + getRoleId() + ",");
if (getArn() != null) sb.append("Arn: " + getArn() + ",");
if (getCreateDate() != null) sb.append("CreateDate: " + getCreateDate() + ",");
if (getAssumeRolePolicyDocument() != null) sb.append("AssumeRolePolicyDocument: " + getAssumeRolePolicyDocument() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getPath() == null) ? 0 : getPath().hashCode());
hashCode = prime * hashCode + ((getRoleName() == null) ? 0 : getRoleName().hashCode());
hashCode = prime * hashCode + ((getRoleId() == null) ? 0 : getRoleId().hashCode());
hashCode = prime * hashCode + ((getArn() == null) ? 0 : getArn().hashCode());
hashCode = prime * hashCode + ((getCreateDate() == null) ? 0 : getCreateDate().hashCode());
hashCode = prime * hashCode + ((getAssumeRolePolicyDocument() == null) ? 0 : getAssumeRolePolicyDocument().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof Role == false) return false;
Role other = (Role)obj;
if (other.getPath() == null ^ this.getPath() == null) return false;
if (other.getPath() != null && other.getPath().equals(this.getPath()) == false) return false;
if (other.getRoleName() == null ^ this.getRoleName() == null) return false;
if (other.getRoleName() != null && other.getRoleName().equals(this.getRoleName()) == false) return false;
if (other.getRoleId() == null ^ this.getRoleId() == null) return false;
if (other.getRoleId() != null && other.getRoleId().equals(this.getRoleId()) == false) return false;
if (other.getArn() == null ^ this.getArn() == null) return false;
if (other.getArn() != null && other.getArn().equals(this.getArn()) == false) return false;
if (other.getCreateDate() == null ^ this.getCreateDate() == null) return false;
if (other.getCreateDate() != null && other.getCreateDate().equals(this.getCreateDate()) == false) return false;
if (other.getAssumeRolePolicyDocument() == null ^ this.getAssumeRolePolicyDocument() == null) return false;
if (other.getAssumeRolePolicyDocument() != null && other.getAssumeRolePolicyDocument().equals(this.getAssumeRolePolicyDocument()) == false) return false;
return true;
}
}