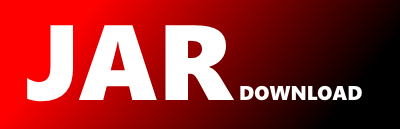
com.amazonaws.services.kinesis.model.GetRecordsRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.kinesis.model;
import java.io.Serializable;
import com.amazonaws.AmazonWebServiceRequest;
/**
* Container for the parameters to the {@link com.amazonaws.services.kinesis.AmazonKinesis#getRecords(GetRecordsRequest) GetRecords operation}.
*
* This operation returns one or more data records from a shard. A
* GetRecords
operation request can retrieve up to 10 MB of
* data.
*
*
* You specify a shard iterator for the shard that you want to read data
* from in the ShardIterator
parameter. The shard iterator
* specifies the position in the shard from which you want to start
* reading data records sequentially. A shard iterator specifies this
* position using the sequence number of a data record in the shard. For
* more information about the shard iterator, see GetShardIterator.
*
*
* GetRecords
may return a partial result if the response
* size limit is exceeded. You will get an error, but not a partial
* result if the shard's provisioned throughput is exceeded, the shard
* iterator has expired, or an internal processing failure has occurred.
* Clients can request a smaller amount of data by specifying a maximum
* number of returned records using the Limit
parameter. The
* Limit
parameter can be set to an integer value of up to
* 10,000. If you set the value to an integer greater than 10,000, you
* will receive InvalidArgumentException
.
*
*
* A new shard iterator is returned by every GetRecords
* request in NextShardIterator
,
* which you use in the ShardIterator
parameter
* of the next GetRecords
request. When you repeatedly read
* from an Amazon Kinesis stream use a GetShardIterator request to get
* the first shard iterator to use in your first GetRecords
* request and then use the shard iterator returned in
* NextShardIterator
for subsequent reads.
*
*
* GetRecords
can return null
for the
* NextShardIterator
to reflect that the shard has been
* closed and that the requested shard iterator would never have returned
* more data.
*
*
* If no items can be processed because of insufficient provisioned
* throughput on the shard involved in the request,
* GetRecords
throws
* ProvisionedThroughputExceededException
.
*
*
* @see com.amazonaws.services.kinesis.AmazonKinesis#getRecords(GetRecordsRequest)
*/
public class GetRecordsRequest extends AmazonWebServiceRequest implements Serializable {
/**
* The position in the shard from which you want to start sequentially
* reading data records.
*
* Constraints:
* Length: 1 - 512
*/
private String shardIterator;
/**
* The maximum number of records to return, which can be set to a value
* of up to 10,000.
*
* Constraints:
* Range: 1 - 10000
*/
private Integer limit;
/**
* The position in the shard from which you want to start sequentially
* reading data records.
*
* Constraints:
* Length: 1 - 512
*
* @return The position in the shard from which you want to start sequentially
* reading data records.
*/
public String getShardIterator() {
return shardIterator;
}
/**
* The position in the shard from which you want to start sequentially
* reading data records.
*
* Constraints:
* Length: 1 - 512
*
* @param shardIterator The position in the shard from which you want to start sequentially
* reading data records.
*/
public void setShardIterator(String shardIterator) {
this.shardIterator = shardIterator;
}
/**
* The position in the shard from which you want to start sequentially
* reading data records.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 512
*
* @param shardIterator The position in the shard from which you want to start sequentially
* reading data records.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public GetRecordsRequest withShardIterator(String shardIterator) {
this.shardIterator = shardIterator;
return this;
}
/**
* The maximum number of records to return, which can be set to a value
* of up to 10,000.
*
* Constraints:
* Range: 1 - 10000
*
* @return The maximum number of records to return, which can be set to a value
* of up to 10,000.
*/
public Integer getLimit() {
return limit;
}
/**
* The maximum number of records to return, which can be set to a value
* of up to 10,000.
*
* Constraints:
* Range: 1 - 10000
*
* @param limit The maximum number of records to return, which can be set to a value
* of up to 10,000.
*/
public void setLimit(Integer limit) {
this.limit = limit;
}
/**
* The maximum number of records to return, which can be set to a value
* of up to 10,000.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Range: 1 - 10000
*
* @param limit The maximum number of records to return, which can be set to a value
* of up to 10,000.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public GetRecordsRequest withLimit(Integer limit) {
this.limit = limit;
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getShardIterator() != null) sb.append("ShardIterator: " + getShardIterator() + ",");
if (getLimit() != null) sb.append("Limit: " + getLimit() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getShardIterator() == null) ? 0 : getShardIterator().hashCode());
hashCode = prime * hashCode + ((getLimit() == null) ? 0 : getLimit().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof GetRecordsRequest == false) return false;
GetRecordsRequest other = (GetRecordsRequest)obj;
if (other.getShardIterator() == null ^ this.getShardIterator() == null) return false;
if (other.getShardIterator() != null && other.getShardIterator().equals(this.getShardIterator()) == false) return false;
if (other.getLimit() == null ^ this.getLimit() == null) return false;
if (other.getLimit() != null && other.getLimit().equals(this.getLimit()) == false) return false;
return true;
}
}