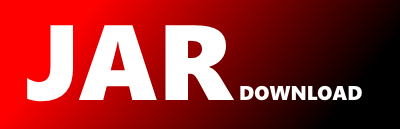
com.amazonaws.services.opsworks.AWSOpsWorks Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.opsworks;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.opsworks.model.*;
/**
* Interface for accessing AWSOpsWorks.
* AWS OpsWorks
* Welcome to the AWS OpsWorks API Reference . This guide provides
* descriptions, syntax, and usage examples about AWS OpsWorks actions
* and data types, including common parameters and error codes.
*
*
* AWS OpsWorks is an application management service that provides an
* integrated experience for overseeing the complete application
* lifecycle. For information about this product, go to the
* AWS OpsWorks
* details page.
*
*
* SDKs and CLI
*
*
* The most common way to use the AWS OpsWorks API is by using the AWS
* Command Line Interface (CLI) or by using one of the AWS SDKs to
* implement applications in your preferred language. For more
* information, see:
*
*
*
* -
* AWS CLI
*
* -
* AWS SDK for Java
*
* -
* AWS SDK for .NET
*
* -
* AWS SDK for PHP 2
*
* -
* AWS SDK for Ruby
*
* -
* AWS SDK for Node.js
*
* -
* AWS SDK for Python(Boto)
*
*
*
*
* Endpoints
*
*
* AWS OpsWorks supports only one endpoint,
* opsworks.us-east-1.amazonaws.com (HTTPS), so you must connect to that
* endpoint. You can then use the API to direct AWS OpsWorks to create
* stacks in any AWS Region.
*
*
* Chef Version
*
*
* When you call CreateStack, CloneStack, or UpdateStack we recommend you
* use the ConfigurationManager
parameter to specify the
* Chef version, 0.9 or 11.4. The default value is currently 0.9.
* However, we expect to change the default value to 11.4 in October
* 2013. For more information, see
* Using AWS OpsWorks with Chef 11
* .
*
*/
public interface AWSOpsWorks {
/**
* Overrides the default endpoint for this client ("https://opsworks.us-east-1.amazonaws.com").
* Callers can use this method to control which AWS region they want to work with.
*
* Callers can pass in just the endpoint (ex: "opsworks.us-east-1.amazonaws.com") or a full
* URL, including the protocol (ex: "https://opsworks.us-east-1.amazonaws.com"). If the
* protocol is not specified here, the default protocol from this client's
* {@link ClientConfiguration} will be used, which by default is HTTPS.
*
* For more information on using AWS regions with the AWS SDK for Java, and
* a complete list of all available endpoints for all AWS services, see:
*
* http://developer.amazonwebservices.com/connect/entry.jspa?externalID=3912
*
* This method is not threadsafe. An endpoint should be configured when the
* client is created and before any service requests are made. Changing it
* afterwards creates inevitable race conditions for any service requests in
* transit or retrying.
*
* @param endpoint
* The endpoint (ex: "opsworks.us-east-1.amazonaws.com") or a full URL,
* including the protocol (ex: "https://opsworks.us-east-1.amazonaws.com") of
* the region specific AWS endpoint this client will communicate
* with.
*
* @throws IllegalArgumentException
* If any problems are detected with the specified endpoint.
*/
public void setEndpoint(String endpoint) throws java.lang.IllegalArgumentException;
/**
* An alternative to {@link AWSOpsWorks#setEndpoint(String)}, sets the
* regional endpoint for this client's service calls. Callers can use this
* method to control which AWS region they want to work with.
*
* By default, all service endpoints in all regions use the https protocol.
* To use http instead, specify it in the {@link ClientConfiguration}
* supplied at construction.
*
* This method is not threadsafe. A region should be configured when the
* client is created and before any service requests are made. Changing it
* afterwards creates inevitable race conditions for any service requests in
* transit or retrying.
*
* @param region
* The region this client will communicate with. See
* {@link Region#getRegion(com.amazonaws.regions.Regions)} for
* accessing a given region.
* @throws java.lang.IllegalArgumentException
* If the given region is null, or if this service isn't
* available in the given region. See
* {@link Region#isServiceSupported(String)}
* @see Region#getRegion(com.amazonaws.regions.Regions)
* @see Region#createClient(Class, com.amazonaws.auth.AWSCredentialsProvider, ClientConfiguration)
*/
public void setRegion(Region region) throws java.lang.IllegalArgumentException;
/**
*
* Updates a user's SSH public key.
*
*
* Required Permissions : To use this action, an IAM user must
* have self-management enabled or an attached policy that explicitly
* grants permissions. For more information on user permissions, see
* Managing User Permissions
* .
*
*
* @param updateMyUserProfileRequest Container for the necessary
* parameters to execute the UpdateMyUserProfile service method on
* AWSOpsWorks.
*
*
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void updateMyUserProfile(UpdateMyUserProfileRequest updateMyUserProfileRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deregisters an Amazon EBS volume. The volume can then be registered by
* another stack. For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param deregisterVolumeRequest Container for the necessary parameters
* to execute the DeregisterVolume service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deregisterVolume(DeregisterVolumeRequest deregisterVolumeRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Specifies a stack's permissions. For more information, see
* Security and Permissions
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param setPermissionRequest Container for the necessary parameters to
* execute the SetPermission service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void setPermission(SetPermissionRequest setPermissionRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Requests a description of a set of instances.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeInstancesRequest Container for the necessary parameters
* to execute the DescribeInstances service method on AWSOpsWorks.
*
* @return The response from the DescribeInstances service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeInstancesResult describeInstances(DescribeInstancesRequest describeInstancesRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Describes the permissions for a specified stack.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param describePermissionsRequest Container for the necessary
* parameters to execute the DescribePermissions service method on
* AWSOpsWorks.
*
* @return The response from the DescribePermissions service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribePermissionsResult describePermissions(DescribePermissionsRequest describePermissionsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes a specified instance. You must stop an instance before you can
* delete it. For more information, see
* Deleting Instances
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param deleteInstanceRequest Container for the necessary parameters to
* execute the DeleteInstance service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteInstance(DeleteInstanceRequest deleteInstanceRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates a clone of a specified stack. For more information, see
* Clone a Stack
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have an attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param cloneStackRequest Container for the necessary parameters to
* execute the CloneStack service method on AWSOpsWorks.
*
* @return The response from the CloneStack service method, as returned
* by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public CloneStackResult cloneStack(CloneStackRequest cloneStackRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Detaches a specified Elastic Load Balancing instance from its layer.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param detachElasticLoadBalancerRequest Container for the necessary
* parameters to execute the DetachElasticLoadBalancer service method on
* AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void detachElasticLoadBalancer(DetachElasticLoadBalancerRequest detachElasticLoadBalancerRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Stops a specified instance. When you stop a standard instance, the
* data disappears and must be reinstalled when you restart the instance.
* You can stop an Amazon EBS-backed instance without losing data. For
* more information, see
* Starting, Stopping, and Rebooting Instances
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param stopInstanceRequest Container for the necessary parameters to
* execute the StopInstance service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void stopInstance(StopInstanceRequest stopInstanceRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Updates a specified app.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Deploy or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param updateAppRequest Container for the necessary parameters to
* execute the UpdateApp service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void updateApp(UpdateAppRequest updateAppRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Describes the results of specified commands.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeCommandsRequest Container for the necessary parameters
* to execute the DescribeCommands service method on AWSOpsWorks.
*
* @return The response from the DescribeCommands service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeCommandsResult describeCommands(DescribeCommandsRequest describeCommandsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Associates one of the stack's registered Elastic IP addresses with a
* specified instance. The address must first be registered with the
* stack by calling RegisterElasticIp. For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param associateElasticIpRequest Container for the necessary
* parameters to execute the AssociateElasticIp service method on
* AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void associateElasticIp(AssociateElasticIpRequest associateElasticIpRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Unassigns an assigned Amazon EBS volume. The volume remains registered
* with the stack. For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param unassignVolumeRequest Container for the necessary parameters to
* execute the UnassignVolume service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void unassignVolume(UnassignVolumeRequest unassignVolumeRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Describe an instance's RAID arrays.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeRaidArraysRequest Container for the necessary
* parameters to execute the DescribeRaidArrays service method on
* AWSOpsWorks.
*
* @return The response from the DescribeRaidArrays service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeRaidArraysResult describeRaidArrays(DescribeRaidArraysRequest describeRaidArraysRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Gets a generated host name for the specified layer, based on the
* current host name theme.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param getHostnameSuggestionRequest Container for the necessary
* parameters to execute the GetHostnameSuggestion service method on
* AWSOpsWorks.
*
* @return The response from the GetHostnameSuggestion service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public GetHostnameSuggestionResult getHostnameSuggestion(GetHostnameSuggestionRequest getHostnameSuggestionRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Specify the load-based auto scaling configuration for a specified
* layer. For more information, see
* Managing Load with Time-based and Load-based Instances
* .
*
*
* NOTE:To use load-based auto scaling, you must create a set of
* load-based auto scaling instances. Load-based auto scaling operates
* only on the instances from that set, so you must ensure that you have
* created enough instances to handle the maximum anticipated load.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param setLoadBasedAutoScalingRequest Container for the necessary
* parameters to execute the SetLoadBasedAutoScaling service method on
* AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void setLoadBasedAutoScaling(SetLoadBasedAutoScalingRequest setLoadBasedAutoScalingRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Describes an instance's Amazon EBS volumes.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeVolumesRequest Container for the necessary parameters
* to execute the DescribeVolumes service method on AWSOpsWorks.
*
* @return The response from the DescribeVolumes service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeVolumesResult describeVolumes(DescribeVolumesRequest describeVolumesRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Assigns one of the stack's registered Amazon EBS volumes to a
* specified instance. The volume must first be registered with the stack
* by calling RegisterVolume. For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param assignVolumeRequest Container for the necessary parameters to
* execute the AssignVolume service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void assignVolume(AssignVolumeRequest assignVolumeRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Describes AWS OpsWorks service errors.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeServiceErrorsRequest Container for the necessary
* parameters to execute the DescribeServiceErrors service method on
* AWSOpsWorks.
*
* @return The response from the DescribeServiceErrors service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeServiceErrorsResult describeServiceErrors(DescribeServiceErrorsRequest describeServiceErrorsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Updates a specified layer.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param updateLayerRequest Container for the necessary parameters to
* execute the UpdateLayer service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void updateLayer(UpdateLayerRequest updateLayerRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Updates a registered Elastic IP address's name. For more information,
* see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param updateElasticIpRequest Container for the necessary parameters
* to execute the UpdateElasticIp service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void updateElasticIp(UpdateElasticIpRequest updateElasticIpRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Starts a specified instance. For more information, see
* Starting, Stopping, and Rebooting Instances
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param startInstanceRequest Container for the necessary parameters to
* execute the StartInstance service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void startInstance(StartInstanceRequest startInstanceRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates a layer. For more information, see
* How to Create a Layer
* .
*
*
* NOTE:You should use CreateLayer for noncustom layer types such
* as PHP App Server only if the stack does not have an existing layer of
* that type. A stack can have at most one instance of each noncustom
* layer; if you attempt to create a second instance, CreateLayer fails.
* A stack can have an arbitrary number of custom layers, so you can call
* CreateLayer as many times as you like for that layer type.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param createLayerRequest Container for the necessary parameters to
* execute the CreateLayer service method on AWSOpsWorks.
*
* @return The response from the CreateLayer service method, as returned
* by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateLayerResult createLayer(CreateLayerRequest createLayerRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Disassociates an Elastic IP address from its instance. The address
* remains registered with the stack. For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param disassociateElasticIpRequest Container for the necessary
* parameters to execute the DisassociateElasticIp service method on
* AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void disassociateElasticIp(DisassociateElasticIpRequest disassociateElasticIpRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes a specified stack. You must first delete all instances,
* layers, and apps. For more information, see
* Shut Down a Stack
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param deleteStackRequest Container for the necessary parameters to
* execute the DeleteStack service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteStack(DeleteStackRequest deleteStackRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Starts stack's instances.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param startStackRequest Container for the necessary parameters to
* execute the StartStack service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void startStack(StartStackRequest startStackRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Registers an Amazon EBS volume with a specified stack. A volume can be
* registered with only one stack at a time. If the volume is already
* registered, you must first deregister it by calling DeregisterVolume.
* For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param registerVolumeRequest Container for the necessary parameters to
* execute the RegisterVolume service method on AWSOpsWorks.
*
* @return The response from the RegisterVolume service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public RegisterVolumeResult registerVolume(RegisterVolumeRequest registerVolumeRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Updates a specified user profile.
*
*
* Required Permissions : To use this action, an IAM user must
* have an attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param updateUserProfileRequest Container for the necessary parameters
* to execute the UpdateUserProfile service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void updateUserProfile(UpdateUserProfileRequest updateUserProfileRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Reboots a specified instance. For more information, see
* Starting, Stopping, and Rebooting Instances
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param rebootInstanceRequest Container for the necessary parameters to
* execute the RebootInstance service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void rebootInstance(RebootInstanceRequest rebootInstanceRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates a new stack. For more information, see
* Create a New Stack
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have an attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param createStackRequest Container for the necessary parameters to
* execute the CreateStack service method on AWSOpsWorks.
*
* @return The response from the CreateStack service method, as returned
* by AWSOpsWorks.
*
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateStackResult createStack(CreateStackRequest createStackRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates an instance in a specified stack. For more information, see
* Adding an Instance to a Layer
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param createInstanceRequest Container for the necessary parameters to
* execute the CreateInstance service method on AWSOpsWorks.
*
* @return The response from the CreateInstance service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateInstanceResult createInstance(CreateInstanceRequest createInstanceRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes a specified app.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param deleteAppRequest Container for the necessary parameters to
* execute the DeleteApp service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteApp(DeleteAppRequest deleteAppRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deregisters a specified Elastic IP address. The address can then be
* registered by another stack. For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param deregisterElasticIpRequest Container for the necessary
* parameters to execute the DeregisterElasticIp service method on
* AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deregisterElasticIp(DeregisterElasticIpRequest deregisterElasticIpRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Attaches an Elastic Load Balancing load balancer to a specified layer.
*
*
* NOTE:You must create the Elastic Load Balancing instance
* separately, by using the Elastic Load Balancing console, API, or CLI.
* For more information, see Elastic Load Balancing Developer Guide.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param attachElasticLoadBalancerRequest Container for the necessary
* parameters to execute the AttachElasticLoadBalancer service method on
* AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void attachElasticLoadBalancer(AttachElasticLoadBalancerRequest attachElasticLoadBalancerRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Updates a specified stack.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param updateStackRequest Container for the necessary parameters to
* execute the UpdateStack service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void updateStack(UpdateStackRequest updateStackRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates a new user profile.
*
*
* Required Permissions : To use this action, an IAM user must
* have an attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param createUserProfileRequest Container for the necessary parameters
* to execute the CreateUserProfile service method on AWSOpsWorks.
*
* @return The response from the CreateUserProfile service method, as
* returned by AWSOpsWorks.
*
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateUserProfileResult createUserProfile(CreateUserProfileRequest createUserProfileRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Requests a description of one or more layers in a specified stack.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeLayersRequest Container for the necessary parameters to
* execute the DescribeLayers service method on AWSOpsWorks.
*
* @return The response from the DescribeLayers service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeLayersResult describeLayers(DescribeLayersRequest describeLayersRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Describes load-based auto scaling configurations for specified layers.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeLoadBasedAutoScalingRequest Container for the necessary
* parameters to execute the DescribeLoadBasedAutoScaling service method
* on AWSOpsWorks.
*
* @return The response from the DescribeLoadBasedAutoScaling service
* method, as returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeLoadBasedAutoScalingResult describeLoadBasedAutoScaling(DescribeLoadBasedAutoScalingRequest describeLoadBasedAutoScalingRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Describes a stack's Elastic Load Balancing instances.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeElasticLoadBalancersRequest Container for the necessary
* parameters to execute the DescribeElasticLoadBalancers service method
* on AWSOpsWorks.
*
* @return The response from the DescribeElasticLoadBalancers service
* method, as returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeElasticLoadBalancersResult describeElasticLoadBalancers(DescribeElasticLoadBalancersRequest describeElasticLoadBalancersRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes a specified layer. You must first stop and then delete all
* associated instances. For more information, see
* How to Delete a Layer
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param deleteLayerRequest Container for the necessary parameters to
* execute the DeleteLayer service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteLayer(DeleteLayerRequest deleteLayerRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Describes a user's SSH information.
*
*
* Required Permissions : To use this action, an IAM user must
* have self-management enabled or an attached policy that explicitly
* grants permissions. For more information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeMyUserProfileRequest Container for the necessary
* parameters to execute the DescribeMyUserProfile service method on
* AWSOpsWorks.
*
* @return The response from the DescribeMyUserProfile service method, as
* returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeMyUserProfileResult describeMyUserProfile(DescribeMyUserProfileRequest describeMyUserProfileRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Specify the time-based auto scaling configuration for a specified
* instance. For more information, see
* Managing Load with Time-based and Load-based Instances
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param setTimeBasedAutoScalingRequest Container for the necessary
* parameters to execute the SetTimeBasedAutoScaling service method on
* AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void setTimeBasedAutoScaling(SetTimeBasedAutoScalingRequest setTimeBasedAutoScalingRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates an app for a specified stack. For more information, see
* Creating Apps
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param createAppRequest Container for the necessary parameters to
* execute the CreateApp service method on AWSOpsWorks.
*
* @return The response from the CreateApp service method, as returned by
* AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateAppResult createApp(CreateAppRequest createAppRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes a user profile.
*
*
* Required Permissions : To use this action, an IAM user must
* have an attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param deleteUserProfileRequest Container for the necessary parameters
* to execute the DeleteUserProfile service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteUserProfile(DeleteUserProfileRequest deleteUserProfileRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Describes time-based auto scaling configurations for specified
* instances.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeTimeBasedAutoScalingRequest Container for the necessary
* parameters to execute the DescribeTimeBasedAutoScaling service method
* on AWSOpsWorks.
*
* @return The response from the DescribeTimeBasedAutoScaling service
* method, as returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeTimeBasedAutoScalingResult describeTimeBasedAutoScaling(DescribeTimeBasedAutoScalingRequest describeTimeBasedAutoScalingRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Updates an Amazon EBS volume's name or mount point. For more
* information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param updateVolumeRequest Container for the necessary parameters to
* execute the UpdateVolume service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void updateVolume(UpdateVolumeRequest updateVolumeRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Describe specified users.
*
*
* Required Permissions : To use this action, an IAM user must
* have an attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeUserProfilesRequest Container for the necessary
* parameters to execute the DescribeUserProfiles service method on
* AWSOpsWorks.
*
* @return The response from the DescribeUserProfiles service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeUserProfilesResult describeUserProfiles(DescribeUserProfilesRequest describeUserProfilesRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Updates a specified instance.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param updateInstanceRequest Container for the necessary parameters to
* execute the UpdateInstance service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void updateInstance(UpdateInstanceRequest updateInstanceRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Requests a description of a specified set of deployments.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeDeploymentsRequest Container for the necessary
* parameters to execute the DescribeDeployments service method on
* AWSOpsWorks.
*
* @return The response from the DescribeDeployments service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeDeploymentsResult describeDeployments(DescribeDeploymentsRequest describeDeploymentsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Registers an Elastic IP address with a specified stack. An address can
* be registered with only one stack at a time. If the address is already
* registered, you must first deregister it by calling
* DeregisterElasticIp. For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param registerElasticIpRequest Container for the necessary parameters
* to execute the RegisterElasticIp service method on AWSOpsWorks.
*
* @return The response from the RegisterElasticIp service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public RegisterElasticIpResult registerElasticIp(RegisterElasticIpRequest registerElasticIpRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Describes
* Elastic IP addresses
* .
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeElasticIpsRequest Container for the necessary
* parameters to execute the DescribeElasticIps service method on
* AWSOpsWorks.
*
* @return The response from the DescribeElasticIps service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeElasticIpsResult describeElasticIps(DescribeElasticIpsRequest describeElasticIpsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Requests a description of one or more stacks.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeStacksRequest Container for the necessary parameters to
* execute the DescribeStacks service method on AWSOpsWorks.
*
* @return The response from the DescribeStacks service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeStacksResult describeStacks(DescribeStacksRequest describeStacksRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Requests a description of a specified set of apps.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeAppsRequest Container for the necessary parameters to
* execute the DescribeApps service method on AWSOpsWorks.
*
* @return The response from the DescribeApps service method, as returned
* by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeAppsResult describeApps(DescribeAppsRequest describeAppsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Describes the number of layers and apps in a specified stack, and the
* number of instances in each state, such as running_setup
* or online
.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeStackSummaryRequest Container for the necessary
* parameters to execute the DescribeStackSummary service method on
* AWSOpsWorks.
*
* @return The response from the DescribeStackSummary service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeStackSummaryResult describeStackSummary(DescribeStackSummaryRequest describeStackSummaryRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Stops a specified stack.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param stopStackRequest Container for the necessary parameters to
* execute the StopStack service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void stopStack(StopStackRequest stopStackRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deploys a stack or app.
*
*
*
* - App deployment generates a
deploy
event, which runs
* the associated recipes and passes them a JSON stack configuration
* object that includes information about the app.
* - Stack deployment runs the
deploy
recipes but does
* not raise an event.
*
*
*
* For more information, see
* Deploying Apps and Run Stack Commands
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Deploy or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param createDeploymentRequest Container for the necessary parameters
* to execute the CreateDeployment service method on AWSOpsWorks.
*
* @return The response from the CreateDeployment service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateDeploymentResult createDeployment(CreateDeploymentRequest createDeploymentRequest)
throws AmazonServiceException, AmazonClientException;
/**
* Shuts down this client object, releasing any resources that might be held
* open. This is an optional method, and callers are not expected to call
* it, but can if they want to explicitly release any open resources. Once a
* client has been shutdown, it should not be used to make any more
* requests.
*/
public void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for
* debugging issues where a service isn't acting as expected. This data isn't considered part
* of the result data returned by an operation, so it's available through this separate,
* diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access
* this extra diagnostic information for an executed request, you should use this method
* to retrieve it as soon as possible after executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none
* is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}