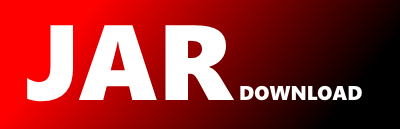
com.amazonaws.services.opsworks.AWSOpsWorksAsyncClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.opsworks;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
import com.amazonaws.AmazonClientException;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.handlers.AsyncHandler;
import com.amazonaws.ClientConfiguration;
import com.amazonaws.auth.AWSCredentials;
import com.amazonaws.auth.AWSCredentialsProvider;
import com.amazonaws.auth.DefaultAWSCredentialsProviderChain;
import com.amazonaws.services.opsworks.model.*;
/**
* Asynchronous client for accessing AWSOpsWorks.
* All asynchronous calls made using this client are non-blocking. Callers could either
* process the result and handle the exceptions in the worker thread by providing a callback handler
* when making the call, or use the returned Future object to check the result of the call in the calling thread.
* AWS OpsWorks
* Welcome to the AWS OpsWorks API Reference . This guide provides
* descriptions, syntax, and usage examples about AWS OpsWorks actions
* and data types, including common parameters and error codes.
*
*
* AWS OpsWorks is an application management service that provides an
* integrated experience for overseeing the complete application
* lifecycle. For information about this product, go to the
* AWS OpsWorks
* details page.
*
*
* SDKs and CLI
*
*
* The most common way to use the AWS OpsWorks API is by using the AWS
* Command Line Interface (CLI) or by using one of the AWS SDKs to
* implement applications in your preferred language. For more
* information, see:
*
*
*
* -
* AWS CLI
*
* -
* AWS SDK for Java
*
* -
* AWS SDK for .NET
*
* -
* AWS SDK for PHP 2
*
* -
* AWS SDK for Ruby
*
* -
* AWS SDK for Node.js
*
* -
* AWS SDK for Python(Boto)
*
*
*
*
* Endpoints
*
*
* AWS OpsWorks supports only one endpoint,
* opsworks.us-east-1.amazonaws.com (HTTPS), so you must connect to that
* endpoint. You can then use the API to direct AWS OpsWorks to create
* stacks in any AWS Region.
*
*
* Chef Version
*
*
* When you call CreateStack, CloneStack, or UpdateStack we recommend you
* use the ConfigurationManager
parameter to specify the
* Chef version, 0.9 or 11.4. The default value is currently 0.9.
* However, we expect to change the default value to 11.4 in October
* 2013. For more information, see
* Using AWS OpsWorks with Chef 11
* .
*
*/
public class AWSOpsWorksAsyncClient extends AWSOpsWorksClient
implements AWSOpsWorksAsync {
/**
* Executor service for executing asynchronous requests.
*/
private ExecutorService executorService;
private static final int DEFAULT_THREAD_POOL_SIZE = 50;
/**
* Constructs a new asynchronous client to invoke service methods on
* AWSOpsWorks. A credentials provider chain will be used
* that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @see DefaultAWSCredentialsProviderChain
*/
public AWSOpsWorksAsyncClient() {
this(new DefaultAWSCredentialsProviderChain());
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AWSOpsWorks. A credentials provider chain will be used
* that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to AWSOpsWorks
* (ex: proxy settings, retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
*/
public AWSOpsWorksAsyncClient(ClientConfiguration clientConfiguration) {
this(new DefaultAWSCredentialsProviderChain(), clientConfiguration, Executors.newFixedThreadPool(clientConfiguration.getMaxConnections()));
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AWSOpsWorks using the specified AWS account credentials.
* Default client settings will be used, and a fixed size thread pool will be
* created for executing the asynchronous tasks.
*
*
* All calls made using this new client object are non-blocking, and will immediately
* return a Java Future object that the caller can later check to see if the service
* call has actually completed.
*
* @param awsCredentials The AWS credentials (access key ID and secret key) to use
* when authenticating with AWS services.
*/
public AWSOpsWorksAsyncClient(AWSCredentials awsCredentials) {
this(awsCredentials, Executors.newFixedThreadPool(DEFAULT_THREAD_POOL_SIZE));
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AWSOpsWorks using the specified AWS account credentials
* and executor service. Default client settings will be used.
*
*
* All calls made using this new client object are non-blocking, and will immediately
* return a Java Future object that the caller can later check to see if the service
* call has actually completed.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use
* when authenticating with AWS services.
* @param executorService
* The executor service by which all asynchronous requests will
* be executed.
*/
public AWSOpsWorksAsyncClient(AWSCredentials awsCredentials, ExecutorService executorService) {
super(awsCredentials);
this.executorService = executorService;
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AWSOpsWorks using the specified AWS account credentials,
* executor service, and client configuration options.
*
*
* All calls made using this new client object are non-blocking, and will immediately
* return a Java Future object that the caller can later check to see if the service
* call has actually completed.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use
* when authenticating with AWS services.
* @param clientConfiguration
* Client configuration options (ex: max retry limit, proxy
* settings, etc).
* @param executorService
* The executor service by which all asynchronous requests will
* be executed.
*/
public AWSOpsWorksAsyncClient(AWSCredentials awsCredentials,
ClientConfiguration clientConfiguration, ExecutorService executorService) {
super(awsCredentials, clientConfiguration);
this.executorService = executorService;
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AWSOpsWorks using the specified AWS account credentials provider.
* Default client settings will be used, and a fixed size thread pool will be
* created for executing the asynchronous tasks.
*
*
* All calls made using this new client object are non-blocking, and will immediately
* return a Java Future object that the caller can later check to see if the service
* call has actually completed.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
*/
public AWSOpsWorksAsyncClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, Executors.newFixedThreadPool(DEFAULT_THREAD_POOL_SIZE));
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AWSOpsWorks using the specified AWS account credentials provider
* and executor service. Default client settings will be used.
*
*
* All calls made using this new client object are non-blocking, and will immediately
* return a Java Future object that the caller can later check to see if the service
* call has actually completed.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
* @param executorService
* The executor service by which all asynchronous requests will
* be executed.
*/
public AWSOpsWorksAsyncClient(AWSCredentialsProvider awsCredentialsProvider, ExecutorService executorService) {
this(awsCredentialsProvider, new ClientConfiguration(), executorService);
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AWSOpsWorks using the specified AWS account credentials
* provider and client configuration options.
*
*
* All calls made using this new client object are non-blocking, and will immediately
* return a Java Future object that the caller can later check to see if the service
* call has actually completed.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
* @param clientConfiguration
* Client configuration options (ex: max retry limit, proxy
* settings, etc).
*/
public AWSOpsWorksAsyncClient(AWSCredentialsProvider awsCredentialsProvider,
ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, Executors.newFixedThreadPool(clientConfiguration.getMaxConnections()));
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AWSOpsWorks using the specified AWS account credentials
* provider, executor service, and client configuration options.
*
*
* All calls made using this new client object are non-blocking, and will immediately
* return a Java Future object that the caller can later check to see if the service
* call has actually completed.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
* @param clientConfiguration
* Client configuration options (ex: max retry limit, proxy
* settings, etc).
* @param executorService
* The executor service by which all asynchronous requests will
* be executed.
*/
public AWSOpsWorksAsyncClient(AWSCredentialsProvider awsCredentialsProvider,
ClientConfiguration clientConfiguration, ExecutorService executorService) {
super(awsCredentialsProvider, clientConfiguration);
this.executorService = executorService;
}
/**
* Returns the executor service used by this async client to execute
* requests.
*
* @return The executor service used by this async client to execute
* requests.
*/
public ExecutorService getExecutorService() {
return executorService;
}
/**
* Shuts down the client, releasing all managed resources. This includes
* forcibly terminating all pending asynchronous service calls. Clients who
* wish to give pending asynchronous service calls time to complete should
* call getExecutorService().shutdown() followed by
* getExecutorService().awaitTermination() prior to calling this method.
*/
@Override
public void shutdown() {
super.shutdown();
executorService.shutdownNow();
}
/**
*
* Updates a user's SSH public key.
*
*
* Required Permissions : To use this action, an IAM user must
* have self-management enabled or an attached policy that explicitly
* grants permissions. For more information on user permissions, see
* Managing User Permissions
* .
*
*
* @param updateMyUserProfileRequest Container for the necessary
* parameters to execute the UpdateMyUserProfile operation on
* AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* UpdateMyUserProfile service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateMyUserProfileAsync(final UpdateMyUserProfileRequest updateMyUserProfileRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
updateMyUserProfile(updateMyUserProfileRequest);
return null;
}
});
}
/**
*
* Updates a user's SSH public key.
*
*
* Required Permissions : To use this action, an IAM user must
* have self-management enabled or an attached policy that explicitly
* grants permissions. For more information on user permissions, see
* Managing User Permissions
* .
*
*
* @param updateMyUserProfileRequest Container for the necessary
* parameters to execute the UpdateMyUserProfile operation on
* AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* UpdateMyUserProfile service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateMyUserProfileAsync(
final UpdateMyUserProfileRequest updateMyUserProfileRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
updateMyUserProfile(updateMyUserProfileRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(updateMyUserProfileRequest, null);
return null;
}
});
}
/**
*
* Deregisters an Amazon EBS volume. The volume can then be registered by
* another stack. For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param deregisterVolumeRequest Container for the necessary parameters
* to execute the DeregisterVolume operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* DeregisterVolume service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deregisterVolumeAsync(final DeregisterVolumeRequest deregisterVolumeRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
deregisterVolume(deregisterVolumeRequest);
return null;
}
});
}
/**
*
* Deregisters an Amazon EBS volume. The volume can then be registered by
* another stack. For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param deregisterVolumeRequest Container for the necessary parameters
* to execute the DeregisterVolume operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeregisterVolume service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deregisterVolumeAsync(
final DeregisterVolumeRequest deregisterVolumeRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
deregisterVolume(deregisterVolumeRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(deregisterVolumeRequest, null);
return null;
}
});
}
/**
*
* Specifies a stack's permissions. For more information, see
* Security and Permissions
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param setPermissionRequest Container for the necessary parameters to
* execute the SetPermission operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* SetPermission service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future setPermissionAsync(final SetPermissionRequest setPermissionRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
setPermission(setPermissionRequest);
return null;
}
});
}
/**
*
* Specifies a stack's permissions. For more information, see
* Security and Permissions
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param setPermissionRequest Container for the necessary parameters to
* execute the SetPermission operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* SetPermission service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future setPermissionAsync(
final SetPermissionRequest setPermissionRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
setPermission(setPermissionRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(setPermissionRequest, null);
return null;
}
});
}
/**
*
* Requests a description of a set of instances.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeInstancesRequest Container for the necessary parameters
* to execute the DescribeInstances operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* DescribeInstances service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeInstancesAsync(final DescribeInstancesRequest describeInstancesRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeInstancesResult call() throws Exception {
return describeInstances(describeInstancesRequest);
}
});
}
/**
*
* Requests a description of a set of instances.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeInstancesRequest Container for the necessary parameters
* to execute the DescribeInstances operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeInstances service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeInstancesAsync(
final DescribeInstancesRequest describeInstancesRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeInstancesResult call() throws Exception {
DescribeInstancesResult result;
try {
result = describeInstances(describeInstancesRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeInstancesRequest, result);
return result;
}
});
}
/**
*
* Describes the permissions for a specified stack.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param describePermissionsRequest Container for the necessary
* parameters to execute the DescribePermissions operation on
* AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* DescribePermissions service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describePermissionsAsync(final DescribePermissionsRequest describePermissionsRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribePermissionsResult call() throws Exception {
return describePermissions(describePermissionsRequest);
}
});
}
/**
*
* Describes the permissions for a specified stack.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param describePermissionsRequest Container for the necessary
* parameters to execute the DescribePermissions operation on
* AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribePermissions service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describePermissionsAsync(
final DescribePermissionsRequest describePermissionsRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribePermissionsResult call() throws Exception {
DescribePermissionsResult result;
try {
result = describePermissions(describePermissionsRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describePermissionsRequest, result);
return result;
}
});
}
/**
*
* Deletes a specified instance. You must stop an instance before you can
* delete it. For more information, see
* Deleting Instances
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param deleteInstanceRequest Container for the necessary parameters to
* execute the DeleteInstance operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* DeleteInstance service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteInstanceAsync(final DeleteInstanceRequest deleteInstanceRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
deleteInstance(deleteInstanceRequest);
return null;
}
});
}
/**
*
* Deletes a specified instance. You must stop an instance before you can
* delete it. For more information, see
* Deleting Instances
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param deleteInstanceRequest Container for the necessary parameters to
* execute the DeleteInstance operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteInstance service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteInstanceAsync(
final DeleteInstanceRequest deleteInstanceRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
deleteInstance(deleteInstanceRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(deleteInstanceRequest, null);
return null;
}
});
}
/**
*
* Creates a clone of a specified stack. For more information, see
* Clone a Stack
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have an attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param cloneStackRequest Container for the necessary parameters to
* execute the CloneStack operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* CloneStack service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future cloneStackAsync(final CloneStackRequest cloneStackRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CloneStackResult call() throws Exception {
return cloneStack(cloneStackRequest);
}
});
}
/**
*
* Creates a clone of a specified stack. For more information, see
* Clone a Stack
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have an attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param cloneStackRequest Container for the necessary parameters to
* execute the CloneStack operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CloneStack service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future cloneStackAsync(
final CloneStackRequest cloneStackRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CloneStackResult call() throws Exception {
CloneStackResult result;
try {
result = cloneStack(cloneStackRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(cloneStackRequest, result);
return result;
}
});
}
/**
*
* Detaches a specified Elastic Load Balancing instance from its layer.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param detachElasticLoadBalancerRequest Container for the necessary
* parameters to execute the DetachElasticLoadBalancer operation on
* AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* DetachElasticLoadBalancer service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future detachElasticLoadBalancerAsync(final DetachElasticLoadBalancerRequest detachElasticLoadBalancerRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
detachElasticLoadBalancer(detachElasticLoadBalancerRequest);
return null;
}
});
}
/**
*
* Detaches a specified Elastic Load Balancing instance from its layer.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param detachElasticLoadBalancerRequest Container for the necessary
* parameters to execute the DetachElasticLoadBalancer operation on
* AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DetachElasticLoadBalancer service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future detachElasticLoadBalancerAsync(
final DetachElasticLoadBalancerRequest detachElasticLoadBalancerRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
detachElasticLoadBalancer(detachElasticLoadBalancerRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(detachElasticLoadBalancerRequest, null);
return null;
}
});
}
/**
*
* Stops a specified instance. When you stop a standard instance, the
* data disappears and must be reinstalled when you restart the instance.
* You can stop an Amazon EBS-backed instance without losing data. For
* more information, see
* Starting, Stopping, and Rebooting Instances
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param stopInstanceRequest Container for the necessary parameters to
* execute the StopInstance operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* StopInstance service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future stopInstanceAsync(final StopInstanceRequest stopInstanceRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
stopInstance(stopInstanceRequest);
return null;
}
});
}
/**
*
* Stops a specified instance. When you stop a standard instance, the
* data disappears and must be reinstalled when you restart the instance.
* You can stop an Amazon EBS-backed instance without losing data. For
* more information, see
* Starting, Stopping, and Rebooting Instances
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param stopInstanceRequest Container for the necessary parameters to
* execute the StopInstance operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* StopInstance service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future stopInstanceAsync(
final StopInstanceRequest stopInstanceRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
stopInstance(stopInstanceRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(stopInstanceRequest, null);
return null;
}
});
}
/**
*
* Updates a specified app.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Deploy or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param updateAppRequest Container for the necessary parameters to
* execute the UpdateApp operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* UpdateApp service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateAppAsync(final UpdateAppRequest updateAppRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
updateApp(updateAppRequest);
return null;
}
});
}
/**
*
* Updates a specified app.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Deploy or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param updateAppRequest Container for the necessary parameters to
* execute the UpdateApp operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* UpdateApp service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateAppAsync(
final UpdateAppRequest updateAppRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
updateApp(updateAppRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(updateAppRequest, null);
return null;
}
});
}
/**
*
* Describes the results of specified commands.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeCommandsRequest Container for the necessary parameters
* to execute the DescribeCommands operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* DescribeCommands service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeCommandsAsync(final DescribeCommandsRequest describeCommandsRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeCommandsResult call() throws Exception {
return describeCommands(describeCommandsRequest);
}
});
}
/**
*
* Describes the results of specified commands.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeCommandsRequest Container for the necessary parameters
* to execute the DescribeCommands operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeCommands service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeCommandsAsync(
final DescribeCommandsRequest describeCommandsRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeCommandsResult call() throws Exception {
DescribeCommandsResult result;
try {
result = describeCommands(describeCommandsRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeCommandsRequest, result);
return result;
}
});
}
/**
*
* Associates one of the stack's registered Elastic IP addresses with a
* specified instance. The address must first be registered with the
* stack by calling RegisterElasticIp. For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param associateElasticIpRequest Container for the necessary
* parameters to execute the AssociateElasticIp operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* AssociateElasticIp service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future associateElasticIpAsync(final AssociateElasticIpRequest associateElasticIpRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
associateElasticIp(associateElasticIpRequest);
return null;
}
});
}
/**
*
* Associates one of the stack's registered Elastic IP addresses with a
* specified instance. The address must first be registered with the
* stack by calling RegisterElasticIp. For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param associateElasticIpRequest Container for the necessary
* parameters to execute the AssociateElasticIp operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* AssociateElasticIp service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future associateElasticIpAsync(
final AssociateElasticIpRequest associateElasticIpRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
associateElasticIp(associateElasticIpRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(associateElasticIpRequest, null);
return null;
}
});
}
/**
*
* Unassigns an assigned Amazon EBS volume. The volume remains registered
* with the stack. For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param unassignVolumeRequest Container for the necessary parameters to
* execute the UnassignVolume operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* UnassignVolume service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future unassignVolumeAsync(final UnassignVolumeRequest unassignVolumeRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
unassignVolume(unassignVolumeRequest);
return null;
}
});
}
/**
*
* Unassigns an assigned Amazon EBS volume. The volume remains registered
* with the stack. For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param unassignVolumeRequest Container for the necessary parameters to
* execute the UnassignVolume operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* UnassignVolume service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future unassignVolumeAsync(
final UnassignVolumeRequest unassignVolumeRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
unassignVolume(unassignVolumeRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(unassignVolumeRequest, null);
return null;
}
});
}
/**
*
* Describe an instance's RAID arrays.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeRaidArraysRequest Container for the necessary
* parameters to execute the DescribeRaidArrays operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* DescribeRaidArrays service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeRaidArraysAsync(final DescribeRaidArraysRequest describeRaidArraysRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeRaidArraysResult call() throws Exception {
return describeRaidArrays(describeRaidArraysRequest);
}
});
}
/**
*
* Describe an instance's RAID arrays.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeRaidArraysRequest Container for the necessary
* parameters to execute the DescribeRaidArrays operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeRaidArrays service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeRaidArraysAsync(
final DescribeRaidArraysRequest describeRaidArraysRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeRaidArraysResult call() throws Exception {
DescribeRaidArraysResult result;
try {
result = describeRaidArrays(describeRaidArraysRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeRaidArraysRequest, result);
return result;
}
});
}
/**
*
* Gets a generated host name for the specified layer, based on the
* current host name theme.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param getHostnameSuggestionRequest Container for the necessary
* parameters to execute the GetHostnameSuggestion operation on
* AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* GetHostnameSuggestion service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getHostnameSuggestionAsync(final GetHostnameSuggestionRequest getHostnameSuggestionRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public GetHostnameSuggestionResult call() throws Exception {
return getHostnameSuggestion(getHostnameSuggestionRequest);
}
});
}
/**
*
* Gets a generated host name for the specified layer, based on the
* current host name theme.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param getHostnameSuggestionRequest Container for the necessary
* parameters to execute the GetHostnameSuggestion operation on
* AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* GetHostnameSuggestion service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getHostnameSuggestionAsync(
final GetHostnameSuggestionRequest getHostnameSuggestionRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public GetHostnameSuggestionResult call() throws Exception {
GetHostnameSuggestionResult result;
try {
result = getHostnameSuggestion(getHostnameSuggestionRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(getHostnameSuggestionRequest, result);
return result;
}
});
}
/**
*
* Specify the load-based auto scaling configuration for a specified
* layer. For more information, see
* Managing Load with Time-based and Load-based Instances
* .
*
*
* NOTE:To use load-based auto scaling, you must create a set of
* load-based auto scaling instances. Load-based auto scaling operates
* only on the instances from that set, so you must ensure that you have
* created enough instances to handle the maximum anticipated load.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param setLoadBasedAutoScalingRequest Container for the necessary
* parameters to execute the SetLoadBasedAutoScaling operation on
* AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* SetLoadBasedAutoScaling service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future setLoadBasedAutoScalingAsync(final SetLoadBasedAutoScalingRequest setLoadBasedAutoScalingRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
setLoadBasedAutoScaling(setLoadBasedAutoScalingRequest);
return null;
}
});
}
/**
*
* Specify the load-based auto scaling configuration for a specified
* layer. For more information, see
* Managing Load with Time-based and Load-based Instances
* .
*
*
* NOTE:To use load-based auto scaling, you must create a set of
* load-based auto scaling instances. Load-based auto scaling operates
* only on the instances from that set, so you must ensure that you have
* created enough instances to handle the maximum anticipated load.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param setLoadBasedAutoScalingRequest Container for the necessary
* parameters to execute the SetLoadBasedAutoScaling operation on
* AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* SetLoadBasedAutoScaling service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future setLoadBasedAutoScalingAsync(
final SetLoadBasedAutoScalingRequest setLoadBasedAutoScalingRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
setLoadBasedAutoScaling(setLoadBasedAutoScalingRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(setLoadBasedAutoScalingRequest, null);
return null;
}
});
}
/**
*
* Describes an instance's Amazon EBS volumes.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeVolumesRequest Container for the necessary parameters
* to execute the DescribeVolumes operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* DescribeVolumes service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeVolumesAsync(final DescribeVolumesRequest describeVolumesRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeVolumesResult call() throws Exception {
return describeVolumes(describeVolumesRequest);
}
});
}
/**
*
* Describes an instance's Amazon EBS volumes.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeVolumesRequest Container for the necessary parameters
* to execute the DescribeVolumes operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeVolumes service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeVolumesAsync(
final DescribeVolumesRequest describeVolumesRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeVolumesResult call() throws Exception {
DescribeVolumesResult result;
try {
result = describeVolumes(describeVolumesRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeVolumesRequest, result);
return result;
}
});
}
/**
*
* Assigns one of the stack's registered Amazon EBS volumes to a
* specified instance. The volume must first be registered with the stack
* by calling RegisterVolume. For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param assignVolumeRequest Container for the necessary parameters to
* execute the AssignVolume operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* AssignVolume service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future assignVolumeAsync(final AssignVolumeRequest assignVolumeRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
assignVolume(assignVolumeRequest);
return null;
}
});
}
/**
*
* Assigns one of the stack's registered Amazon EBS volumes to a
* specified instance. The volume must first be registered with the stack
* by calling RegisterVolume. For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param assignVolumeRequest Container for the necessary parameters to
* execute the AssignVolume operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* AssignVolume service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future assignVolumeAsync(
final AssignVolumeRequest assignVolumeRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
assignVolume(assignVolumeRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(assignVolumeRequest, null);
return null;
}
});
}
/**
*
* Describes AWS OpsWorks service errors.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeServiceErrorsRequest Container for the necessary
* parameters to execute the DescribeServiceErrors operation on
* AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* DescribeServiceErrors service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeServiceErrorsAsync(final DescribeServiceErrorsRequest describeServiceErrorsRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeServiceErrorsResult call() throws Exception {
return describeServiceErrors(describeServiceErrorsRequest);
}
});
}
/**
*
* Describes AWS OpsWorks service errors.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeServiceErrorsRequest Container for the necessary
* parameters to execute the DescribeServiceErrors operation on
* AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeServiceErrors service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeServiceErrorsAsync(
final DescribeServiceErrorsRequest describeServiceErrorsRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeServiceErrorsResult call() throws Exception {
DescribeServiceErrorsResult result;
try {
result = describeServiceErrors(describeServiceErrorsRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeServiceErrorsRequest, result);
return result;
}
});
}
/**
*
* Updates a specified layer.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param updateLayerRequest Container for the necessary parameters to
* execute the UpdateLayer operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* UpdateLayer service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateLayerAsync(final UpdateLayerRequest updateLayerRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
updateLayer(updateLayerRequest);
return null;
}
});
}
/**
*
* Updates a specified layer.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param updateLayerRequest Container for the necessary parameters to
* execute the UpdateLayer operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* UpdateLayer service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateLayerAsync(
final UpdateLayerRequest updateLayerRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
updateLayer(updateLayerRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(updateLayerRequest, null);
return null;
}
});
}
/**
*
* Updates a registered Elastic IP address's name. For more information,
* see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param updateElasticIpRequest Container for the necessary parameters
* to execute the UpdateElasticIp operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* UpdateElasticIp service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateElasticIpAsync(final UpdateElasticIpRequest updateElasticIpRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
updateElasticIp(updateElasticIpRequest);
return null;
}
});
}
/**
*
* Updates a registered Elastic IP address's name. For more information,
* see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param updateElasticIpRequest Container for the necessary parameters
* to execute the UpdateElasticIp operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* UpdateElasticIp service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateElasticIpAsync(
final UpdateElasticIpRequest updateElasticIpRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
updateElasticIp(updateElasticIpRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(updateElasticIpRequest, null);
return null;
}
});
}
/**
*
* Starts a specified instance. For more information, see
* Starting, Stopping, and Rebooting Instances
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param startInstanceRequest Container for the necessary parameters to
* execute the StartInstance operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* StartInstance service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future startInstanceAsync(final StartInstanceRequest startInstanceRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
startInstance(startInstanceRequest);
return null;
}
});
}
/**
*
* Starts a specified instance. For more information, see
* Starting, Stopping, and Rebooting Instances
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param startInstanceRequest Container for the necessary parameters to
* execute the StartInstance operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* StartInstance service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future startInstanceAsync(
final StartInstanceRequest startInstanceRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
startInstance(startInstanceRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(startInstanceRequest, null);
return null;
}
});
}
/**
*
* Creates a layer. For more information, see
* How to Create a Layer
* .
*
*
* NOTE:You should use CreateLayer for noncustom layer types such
* as PHP App Server only if the stack does not have an existing layer of
* that type. A stack can have at most one instance of each noncustom
* layer; if you attempt to create a second instance, CreateLayer fails.
* A stack can have an arbitrary number of custom layers, so you can call
* CreateLayer as many times as you like for that layer type.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param createLayerRequest Container for the necessary parameters to
* execute the CreateLayer operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* CreateLayer service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createLayerAsync(final CreateLayerRequest createLayerRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CreateLayerResult call() throws Exception {
return createLayer(createLayerRequest);
}
});
}
/**
*
* Creates a layer. For more information, see
* How to Create a Layer
* .
*
*
* NOTE:You should use CreateLayer for noncustom layer types such
* as PHP App Server only if the stack does not have an existing layer of
* that type. A stack can have at most one instance of each noncustom
* layer; if you attempt to create a second instance, CreateLayer fails.
* A stack can have an arbitrary number of custom layers, so you can call
* CreateLayer as many times as you like for that layer type.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param createLayerRequest Container for the necessary parameters to
* execute the CreateLayer operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateLayer service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createLayerAsync(
final CreateLayerRequest createLayerRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CreateLayerResult call() throws Exception {
CreateLayerResult result;
try {
result = createLayer(createLayerRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(createLayerRequest, result);
return result;
}
});
}
/**
*
* Disassociates an Elastic IP address from its instance. The address
* remains registered with the stack. For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param disassociateElasticIpRequest Container for the necessary
* parameters to execute the DisassociateElasticIp operation on
* AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* DisassociateElasticIp service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future disassociateElasticIpAsync(final DisassociateElasticIpRequest disassociateElasticIpRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
disassociateElasticIp(disassociateElasticIpRequest);
return null;
}
});
}
/**
*
* Disassociates an Elastic IP address from its instance. The address
* remains registered with the stack. For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param disassociateElasticIpRequest Container for the necessary
* parameters to execute the DisassociateElasticIp operation on
* AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DisassociateElasticIp service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future disassociateElasticIpAsync(
final DisassociateElasticIpRequest disassociateElasticIpRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
disassociateElasticIp(disassociateElasticIpRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(disassociateElasticIpRequest, null);
return null;
}
});
}
/**
*
* Deletes a specified stack. You must first delete all instances,
* layers, and apps. For more information, see
* Shut Down a Stack
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param deleteStackRequest Container for the necessary parameters to
* execute the DeleteStack operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* DeleteStack service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteStackAsync(final DeleteStackRequest deleteStackRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
deleteStack(deleteStackRequest);
return null;
}
});
}
/**
*
* Deletes a specified stack. You must first delete all instances,
* layers, and apps. For more information, see
* Shut Down a Stack
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param deleteStackRequest Container for the necessary parameters to
* execute the DeleteStack operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteStack service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteStackAsync(
final DeleteStackRequest deleteStackRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
deleteStack(deleteStackRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(deleteStackRequest, null);
return null;
}
});
}
/**
*
* Starts stack's instances.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param startStackRequest Container for the necessary parameters to
* execute the StartStack operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* StartStack service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future startStackAsync(final StartStackRequest startStackRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
startStack(startStackRequest);
return null;
}
});
}
/**
*
* Starts stack's instances.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param startStackRequest Container for the necessary parameters to
* execute the StartStack operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* StartStack service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future startStackAsync(
final StartStackRequest startStackRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
startStack(startStackRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(startStackRequest, null);
return null;
}
});
}
/**
*
* Registers an Amazon EBS volume with a specified stack. A volume can be
* registered with only one stack at a time. If the volume is already
* registered, you must first deregister it by calling DeregisterVolume.
* For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param registerVolumeRequest Container for the necessary parameters to
* execute the RegisterVolume operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* RegisterVolume service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future registerVolumeAsync(final RegisterVolumeRequest registerVolumeRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public RegisterVolumeResult call() throws Exception {
return registerVolume(registerVolumeRequest);
}
});
}
/**
*
* Registers an Amazon EBS volume with a specified stack. A volume can be
* registered with only one stack at a time. If the volume is already
* registered, you must first deregister it by calling DeregisterVolume.
* For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param registerVolumeRequest Container for the necessary parameters to
* execute the RegisterVolume operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* RegisterVolume service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future registerVolumeAsync(
final RegisterVolumeRequest registerVolumeRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public RegisterVolumeResult call() throws Exception {
RegisterVolumeResult result;
try {
result = registerVolume(registerVolumeRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(registerVolumeRequest, result);
return result;
}
});
}
/**
*
* Updates a specified user profile.
*
*
* Required Permissions : To use this action, an IAM user must
* have an attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param updateUserProfileRequest Container for the necessary parameters
* to execute the UpdateUserProfile operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* UpdateUserProfile service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateUserProfileAsync(final UpdateUserProfileRequest updateUserProfileRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
updateUserProfile(updateUserProfileRequest);
return null;
}
});
}
/**
*
* Updates a specified user profile.
*
*
* Required Permissions : To use this action, an IAM user must
* have an attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param updateUserProfileRequest Container for the necessary parameters
* to execute the UpdateUserProfile operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* UpdateUserProfile service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateUserProfileAsync(
final UpdateUserProfileRequest updateUserProfileRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
updateUserProfile(updateUserProfileRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(updateUserProfileRequest, null);
return null;
}
});
}
/**
*
* Reboots a specified instance. For more information, see
* Starting, Stopping, and Rebooting Instances
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param rebootInstanceRequest Container for the necessary parameters to
* execute the RebootInstance operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* RebootInstance service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future rebootInstanceAsync(final RebootInstanceRequest rebootInstanceRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
rebootInstance(rebootInstanceRequest);
return null;
}
});
}
/**
*
* Reboots a specified instance. For more information, see
* Starting, Stopping, and Rebooting Instances
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param rebootInstanceRequest Container for the necessary parameters to
* execute the RebootInstance operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* RebootInstance service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future rebootInstanceAsync(
final RebootInstanceRequest rebootInstanceRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
rebootInstance(rebootInstanceRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(rebootInstanceRequest, null);
return null;
}
});
}
/**
*
* Creates a new stack. For more information, see
* Create a New Stack
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have an attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param createStackRequest Container for the necessary parameters to
* execute the CreateStack operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* CreateStack service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createStackAsync(final CreateStackRequest createStackRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CreateStackResult call() throws Exception {
return createStack(createStackRequest);
}
});
}
/**
*
* Creates a new stack. For more information, see
* Create a New Stack
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have an attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param createStackRequest Container for the necessary parameters to
* execute the CreateStack operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateStack service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createStackAsync(
final CreateStackRequest createStackRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CreateStackResult call() throws Exception {
CreateStackResult result;
try {
result = createStack(createStackRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(createStackRequest, result);
return result;
}
});
}
/**
*
* Creates an instance in a specified stack. For more information, see
* Adding an Instance to a Layer
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param createInstanceRequest Container for the necessary parameters to
* execute the CreateInstance operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* CreateInstance service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createInstanceAsync(final CreateInstanceRequest createInstanceRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CreateInstanceResult call() throws Exception {
return createInstance(createInstanceRequest);
}
});
}
/**
*
* Creates an instance in a specified stack. For more information, see
* Adding an Instance to a Layer
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param createInstanceRequest Container for the necessary parameters to
* execute the CreateInstance operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateInstance service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createInstanceAsync(
final CreateInstanceRequest createInstanceRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CreateInstanceResult call() throws Exception {
CreateInstanceResult result;
try {
result = createInstance(createInstanceRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(createInstanceRequest, result);
return result;
}
});
}
/**
*
* Deletes a specified app.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param deleteAppRequest Container for the necessary parameters to
* execute the DeleteApp operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* DeleteApp service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteAppAsync(final DeleteAppRequest deleteAppRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
deleteApp(deleteAppRequest);
return null;
}
});
}
/**
*
* Deletes a specified app.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param deleteAppRequest Container for the necessary parameters to
* execute the DeleteApp operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteApp service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteAppAsync(
final DeleteAppRequest deleteAppRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
deleteApp(deleteAppRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(deleteAppRequest, null);
return null;
}
});
}
/**
*
* Deregisters a specified Elastic IP address. The address can then be
* registered by another stack. For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param deregisterElasticIpRequest Container for the necessary
* parameters to execute the DeregisterElasticIp operation on
* AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* DeregisterElasticIp service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deregisterElasticIpAsync(final DeregisterElasticIpRequest deregisterElasticIpRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
deregisterElasticIp(deregisterElasticIpRequest);
return null;
}
});
}
/**
*
* Deregisters a specified Elastic IP address. The address can then be
* registered by another stack. For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param deregisterElasticIpRequest Container for the necessary
* parameters to execute the DeregisterElasticIp operation on
* AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeregisterElasticIp service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deregisterElasticIpAsync(
final DeregisterElasticIpRequest deregisterElasticIpRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
deregisterElasticIp(deregisterElasticIpRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(deregisterElasticIpRequest, null);
return null;
}
});
}
/**
*
* Attaches an Elastic Load Balancing load balancer to a specified layer.
*
*
* NOTE:You must create the Elastic Load Balancing instance
* separately, by using the Elastic Load Balancing console, API, or CLI.
* For more information, see Elastic Load Balancing Developer Guide.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param attachElasticLoadBalancerRequest Container for the necessary
* parameters to execute the AttachElasticLoadBalancer operation on
* AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* AttachElasticLoadBalancer service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future attachElasticLoadBalancerAsync(final AttachElasticLoadBalancerRequest attachElasticLoadBalancerRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
attachElasticLoadBalancer(attachElasticLoadBalancerRequest);
return null;
}
});
}
/**
*
* Attaches an Elastic Load Balancing load balancer to a specified layer.
*
*
* NOTE:You must create the Elastic Load Balancing instance
* separately, by using the Elastic Load Balancing console, API, or CLI.
* For more information, see Elastic Load Balancing Developer Guide.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param attachElasticLoadBalancerRequest Container for the necessary
* parameters to execute the AttachElasticLoadBalancer operation on
* AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* AttachElasticLoadBalancer service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future attachElasticLoadBalancerAsync(
final AttachElasticLoadBalancerRequest attachElasticLoadBalancerRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
attachElasticLoadBalancer(attachElasticLoadBalancerRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(attachElasticLoadBalancerRequest, null);
return null;
}
});
}
/**
*
* Updates a specified stack.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param updateStackRequest Container for the necessary parameters to
* execute the UpdateStack operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* UpdateStack service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateStackAsync(final UpdateStackRequest updateStackRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
updateStack(updateStackRequest);
return null;
}
});
}
/**
*
* Updates a specified stack.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param updateStackRequest Container for the necessary parameters to
* execute the UpdateStack operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* UpdateStack service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateStackAsync(
final UpdateStackRequest updateStackRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
updateStack(updateStackRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(updateStackRequest, null);
return null;
}
});
}
/**
*
* Creates a new user profile.
*
*
* Required Permissions : To use this action, an IAM user must
* have an attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param createUserProfileRequest Container for the necessary parameters
* to execute the CreateUserProfile operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* CreateUserProfile service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createUserProfileAsync(final CreateUserProfileRequest createUserProfileRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CreateUserProfileResult call() throws Exception {
return createUserProfile(createUserProfileRequest);
}
});
}
/**
*
* Creates a new user profile.
*
*
* Required Permissions : To use this action, an IAM user must
* have an attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param createUserProfileRequest Container for the necessary parameters
* to execute the CreateUserProfile operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateUserProfile service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createUserProfileAsync(
final CreateUserProfileRequest createUserProfileRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CreateUserProfileResult call() throws Exception {
CreateUserProfileResult result;
try {
result = createUserProfile(createUserProfileRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(createUserProfileRequest, result);
return result;
}
});
}
/**
*
* Requests a description of one or more layers in a specified stack.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeLayersRequest Container for the necessary parameters to
* execute the DescribeLayers operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* DescribeLayers service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeLayersAsync(final DescribeLayersRequest describeLayersRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeLayersResult call() throws Exception {
return describeLayers(describeLayersRequest);
}
});
}
/**
*
* Requests a description of one or more layers in a specified stack.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeLayersRequest Container for the necessary parameters to
* execute the DescribeLayers operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeLayers service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeLayersAsync(
final DescribeLayersRequest describeLayersRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeLayersResult call() throws Exception {
DescribeLayersResult result;
try {
result = describeLayers(describeLayersRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeLayersRequest, result);
return result;
}
});
}
/**
*
* Describes load-based auto scaling configurations for specified layers.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeLoadBasedAutoScalingRequest Container for the necessary
* parameters to execute the DescribeLoadBasedAutoScaling operation on
* AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* DescribeLoadBasedAutoScaling service method, as returned by
* AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeLoadBasedAutoScalingAsync(final DescribeLoadBasedAutoScalingRequest describeLoadBasedAutoScalingRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeLoadBasedAutoScalingResult call() throws Exception {
return describeLoadBasedAutoScaling(describeLoadBasedAutoScalingRequest);
}
});
}
/**
*
* Describes load-based auto scaling configurations for specified layers.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeLoadBasedAutoScalingRequest Container for the necessary
* parameters to execute the DescribeLoadBasedAutoScaling operation on
* AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeLoadBasedAutoScaling service method, as returned by
* AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeLoadBasedAutoScalingAsync(
final DescribeLoadBasedAutoScalingRequest describeLoadBasedAutoScalingRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeLoadBasedAutoScalingResult call() throws Exception {
DescribeLoadBasedAutoScalingResult result;
try {
result = describeLoadBasedAutoScaling(describeLoadBasedAutoScalingRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeLoadBasedAutoScalingRequest, result);
return result;
}
});
}
/**
*
* Describes a stack's Elastic Load Balancing instances.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeElasticLoadBalancersRequest Container for the necessary
* parameters to execute the DescribeElasticLoadBalancers operation on
* AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* DescribeElasticLoadBalancers service method, as returned by
* AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeElasticLoadBalancersAsync(final DescribeElasticLoadBalancersRequest describeElasticLoadBalancersRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeElasticLoadBalancersResult call() throws Exception {
return describeElasticLoadBalancers(describeElasticLoadBalancersRequest);
}
});
}
/**
*
* Describes a stack's Elastic Load Balancing instances.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeElasticLoadBalancersRequest Container for the necessary
* parameters to execute the DescribeElasticLoadBalancers operation on
* AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeElasticLoadBalancers service method, as returned by
* AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeElasticLoadBalancersAsync(
final DescribeElasticLoadBalancersRequest describeElasticLoadBalancersRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeElasticLoadBalancersResult call() throws Exception {
DescribeElasticLoadBalancersResult result;
try {
result = describeElasticLoadBalancers(describeElasticLoadBalancersRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeElasticLoadBalancersRequest, result);
return result;
}
});
}
/**
*
* Deletes a specified layer. You must first stop and then delete all
* associated instances. For more information, see
* How to Delete a Layer
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param deleteLayerRequest Container for the necessary parameters to
* execute the DeleteLayer operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* DeleteLayer service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteLayerAsync(final DeleteLayerRequest deleteLayerRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
deleteLayer(deleteLayerRequest);
return null;
}
});
}
/**
*
* Deletes a specified layer. You must first stop and then delete all
* associated instances. For more information, see
* How to Delete a Layer
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param deleteLayerRequest Container for the necessary parameters to
* execute the DeleteLayer operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteLayer service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteLayerAsync(
final DeleteLayerRequest deleteLayerRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
deleteLayer(deleteLayerRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(deleteLayerRequest, null);
return null;
}
});
}
/**
*
* Describes a user's SSH information.
*
*
* Required Permissions : To use this action, an IAM user must
* have self-management enabled or an attached policy that explicitly
* grants permissions. For more information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeMyUserProfileRequest Container for the necessary
* parameters to execute the DescribeMyUserProfile operation on
* AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* DescribeMyUserProfile service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeMyUserProfileAsync(final DescribeMyUserProfileRequest describeMyUserProfileRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeMyUserProfileResult call() throws Exception {
return describeMyUserProfile(describeMyUserProfileRequest);
}
});
}
/**
*
* Describes a user's SSH information.
*
*
* Required Permissions : To use this action, an IAM user must
* have self-management enabled or an attached policy that explicitly
* grants permissions. For more information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeMyUserProfileRequest Container for the necessary
* parameters to execute the DescribeMyUserProfile operation on
* AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeMyUserProfile service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeMyUserProfileAsync(
final DescribeMyUserProfileRequest describeMyUserProfileRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeMyUserProfileResult call() throws Exception {
DescribeMyUserProfileResult result;
try {
result = describeMyUserProfile(describeMyUserProfileRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeMyUserProfileRequest, result);
return result;
}
});
}
/**
*
* Specify the time-based auto scaling configuration for a specified
* instance. For more information, see
* Managing Load with Time-based and Load-based Instances
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param setTimeBasedAutoScalingRequest Container for the necessary
* parameters to execute the SetTimeBasedAutoScaling operation on
* AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* SetTimeBasedAutoScaling service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future setTimeBasedAutoScalingAsync(final SetTimeBasedAutoScalingRequest setTimeBasedAutoScalingRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
setTimeBasedAutoScaling(setTimeBasedAutoScalingRequest);
return null;
}
});
}
/**
*
* Specify the time-based auto scaling configuration for a specified
* instance. For more information, see
* Managing Load with Time-based and Load-based Instances
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param setTimeBasedAutoScalingRequest Container for the necessary
* parameters to execute the SetTimeBasedAutoScaling operation on
* AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* SetTimeBasedAutoScaling service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future setTimeBasedAutoScalingAsync(
final SetTimeBasedAutoScalingRequest setTimeBasedAutoScalingRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
setTimeBasedAutoScaling(setTimeBasedAutoScalingRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(setTimeBasedAutoScalingRequest, null);
return null;
}
});
}
/**
*
* Creates an app for a specified stack. For more information, see
* Creating Apps
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param createAppRequest Container for the necessary parameters to
* execute the CreateApp operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* CreateApp service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createAppAsync(final CreateAppRequest createAppRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CreateAppResult call() throws Exception {
return createApp(createAppRequest);
}
});
}
/**
*
* Creates an app for a specified stack. For more information, see
* Creating Apps
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param createAppRequest Container for the necessary parameters to
* execute the CreateApp operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateApp service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createAppAsync(
final CreateAppRequest createAppRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public CreateAppResult call() throws Exception {
CreateAppResult result;
try {
result = createApp(createAppRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(createAppRequest, result);
return result;
}
});
}
/**
*
* Deletes a user profile.
*
*
* Required Permissions : To use this action, an IAM user must
* have an attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param deleteUserProfileRequest Container for the necessary parameters
* to execute the DeleteUserProfile operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* DeleteUserProfile service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteUserProfileAsync(final DeleteUserProfileRequest deleteUserProfileRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
deleteUserProfile(deleteUserProfileRequest);
return null;
}
});
}
/**
*
* Deletes a user profile.
*
*
* Required Permissions : To use this action, an IAM user must
* have an attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param deleteUserProfileRequest Container for the necessary parameters
* to execute the DeleteUserProfile operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteUserProfile service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteUserProfileAsync(
final DeleteUserProfileRequest deleteUserProfileRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
deleteUserProfile(deleteUserProfileRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(deleteUserProfileRequest, null);
return null;
}
});
}
/**
*
* Describes time-based auto scaling configurations for specified
* instances.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeTimeBasedAutoScalingRequest Container for the necessary
* parameters to execute the DescribeTimeBasedAutoScaling operation on
* AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* DescribeTimeBasedAutoScaling service method, as returned by
* AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeTimeBasedAutoScalingAsync(final DescribeTimeBasedAutoScalingRequest describeTimeBasedAutoScalingRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeTimeBasedAutoScalingResult call() throws Exception {
return describeTimeBasedAutoScaling(describeTimeBasedAutoScalingRequest);
}
});
}
/**
*
* Describes time-based auto scaling configurations for specified
* instances.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeTimeBasedAutoScalingRequest Container for the necessary
* parameters to execute the DescribeTimeBasedAutoScaling operation on
* AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeTimeBasedAutoScaling service method, as returned by
* AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeTimeBasedAutoScalingAsync(
final DescribeTimeBasedAutoScalingRequest describeTimeBasedAutoScalingRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeTimeBasedAutoScalingResult call() throws Exception {
DescribeTimeBasedAutoScalingResult result;
try {
result = describeTimeBasedAutoScaling(describeTimeBasedAutoScalingRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeTimeBasedAutoScalingRequest, result);
return result;
}
});
}
/**
*
* Updates an Amazon EBS volume's name or mount point. For more
* information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param updateVolumeRequest Container for the necessary parameters to
* execute the UpdateVolume operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* UpdateVolume service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateVolumeAsync(final UpdateVolumeRequest updateVolumeRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
updateVolume(updateVolumeRequest);
return null;
}
});
}
/**
*
* Updates an Amazon EBS volume's name or mount point. For more
* information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param updateVolumeRequest Container for the necessary parameters to
* execute the UpdateVolume operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* UpdateVolume service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateVolumeAsync(
final UpdateVolumeRequest updateVolumeRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
updateVolume(updateVolumeRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(updateVolumeRequest, null);
return null;
}
});
}
/**
*
* Describe specified users.
*
*
* Required Permissions : To use this action, an IAM user must
* have an attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeUserProfilesRequest Container for the necessary
* parameters to execute the DescribeUserProfiles operation on
* AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* DescribeUserProfiles service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeUserProfilesAsync(final DescribeUserProfilesRequest describeUserProfilesRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeUserProfilesResult call() throws Exception {
return describeUserProfiles(describeUserProfilesRequest);
}
});
}
/**
*
* Describe specified users.
*
*
* Required Permissions : To use this action, an IAM user must
* have an attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeUserProfilesRequest Container for the necessary
* parameters to execute the DescribeUserProfiles operation on
* AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeUserProfiles service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeUserProfilesAsync(
final DescribeUserProfilesRequest describeUserProfilesRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeUserProfilesResult call() throws Exception {
DescribeUserProfilesResult result;
try {
result = describeUserProfiles(describeUserProfilesRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeUserProfilesRequest, result);
return result;
}
});
}
/**
*
* Updates a specified instance.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param updateInstanceRequest Container for the necessary parameters to
* execute the UpdateInstance operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* UpdateInstance service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateInstanceAsync(final UpdateInstanceRequest updateInstanceRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
updateInstance(updateInstanceRequest);
return null;
}
});
}
/**
*
* Updates a specified instance.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param updateInstanceRequest Container for the necessary parameters to
* execute the UpdateInstance operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* UpdateInstance service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future updateInstanceAsync(
final UpdateInstanceRequest updateInstanceRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
updateInstance(updateInstanceRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(updateInstanceRequest, null);
return null;
}
});
}
/**
*
* Requests a description of a specified set of deployments.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeDeploymentsRequest Container for the necessary
* parameters to execute the DescribeDeployments operation on
* AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* DescribeDeployments service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeDeploymentsAsync(final DescribeDeploymentsRequest describeDeploymentsRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeDeploymentsResult call() throws Exception {
return describeDeployments(describeDeploymentsRequest);
}
});
}
/**
*
* Requests a description of a specified set of deployments.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeDeploymentsRequest Container for the necessary
* parameters to execute the DescribeDeployments operation on
* AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeDeployments service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeDeploymentsAsync(
final DescribeDeploymentsRequest describeDeploymentsRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DescribeDeploymentsResult call() throws Exception {
DescribeDeploymentsResult result;
try {
result = describeDeployments(describeDeploymentsRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(describeDeploymentsRequest, result);
return result;
}
});
}
/**
*
* Registers an Elastic IP address with a specified stack. An address can
* be registered with only one stack at a time. If the address is already
* registered, you must first deregister it by calling
* DeregisterElasticIp. For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param registerElasticIpRequest Container for the necessary parameters
* to execute the RegisterElasticIp operation on AWSOpsWorks.
*
* @return A Java Future object containing the response from the
* RegisterElasticIp service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future registerElasticIpAsync(final RegisterElasticIpRequest registerElasticIpRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public RegisterElasticIpResult call() throws Exception {
return registerElasticIp(registerElasticIpRequest);
}
});
}
/**
*
* Registers an Elastic IP address with a specified stack. An address can
* be registered with only one stack at a time. If the address is already
* registered, you must first deregister it by calling
* DeregisterElasticIp. For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param registerElasticIpRequest Container for the necessary parameters
* to execute the RegisterElasticIp operation on AWSOpsWorks.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* RegisterElasticIp service method, as returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future registerElasticIpAsync(
final RegisterElasticIpRequest registerElasticIpRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public RegisterElasticIpResult call() throws Exception {
RegisterElasticIpResult result;
try {
result = registerElasticIp(registerElasticIpRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(registerElasticIpRequest, result);
return result;
}
});
}
/**
*