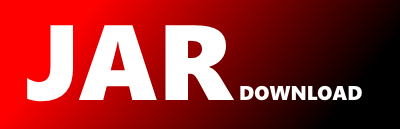
com.amazonaws.services.opsworks.AWSOpsWorksClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.opsworks;
import java.net.*;
import java.util.*;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.regions.*;
import com.amazonaws.internal.*;
import com.amazonaws.metrics.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.util.json.*;
import com.amazonaws.services.opsworks.model.*;
import com.amazonaws.services.opsworks.model.transform.*;
/**
* Client for accessing AWSOpsWorks. All service calls made
* using this client are blocking, and will not return until the service call
* completes.
*
* AWS OpsWorks
* Welcome to the AWS OpsWorks API Reference . This guide provides
* descriptions, syntax, and usage examples about AWS OpsWorks actions
* and data types, including common parameters and error codes.
*
*
* AWS OpsWorks is an application management service that provides an
* integrated experience for overseeing the complete application
* lifecycle. For information about this product, go to the
* AWS OpsWorks
* details page.
*
*
* SDKs and CLI
*
*
* The most common way to use the AWS OpsWorks API is by using the AWS
* Command Line Interface (CLI) or by using one of the AWS SDKs to
* implement applications in your preferred language. For more
* information, see:
*
*
*
* -
* AWS CLI
*
* -
* AWS SDK for Java
*
* -
* AWS SDK for .NET
*
* -
* AWS SDK for PHP 2
*
* -
* AWS SDK for Ruby
*
* -
* AWS SDK for Node.js
*
* -
* AWS SDK for Python(Boto)
*
*
*
*
* Endpoints
*
*
* AWS OpsWorks supports only one endpoint,
* opsworks.us-east-1.amazonaws.com (HTTPS), so you must connect to that
* endpoint. You can then use the API to direct AWS OpsWorks to create
* stacks in any AWS Region.
*
*
* Chef Version
*
*
* When you call CreateStack, CloneStack, or UpdateStack we recommend you
* use the ConfigurationManager
parameter to specify the
* Chef version, 0.9 or 11.4. The default value is currently 0.9.
* However, we expect to change the default value to 11.4 in October
* 2013. For more information, see
* Using AWS OpsWorks with Chef 11
* .
*
*/
public class AWSOpsWorksClient extends AmazonWebServiceClient implements AWSOpsWorks {
/** Provider for AWS credentials. */
private AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSOpsWorks.class);
/**
* List of exception unmarshallers for all AWSOpsWorks exceptions.
*/
protected List jsonErrorUnmarshallers;
/**
* Constructs a new client to invoke service methods on
* AWSOpsWorks. A credentials provider chain will be used
* that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @see DefaultAWSCredentialsProviderChain
*/
public AWSOpsWorksClient() {
this(new DefaultAWSCredentialsProviderChain(), new ClientConfiguration());
}
/**
* Constructs a new client to invoke service methods on
* AWSOpsWorks. A credentials provider chain will be used
* that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to AWSOpsWorks
* (ex: proxy settings, retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
*/
public AWSOpsWorksClient(ClientConfiguration clientConfiguration) {
this(new DefaultAWSCredentialsProviderChain(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on
* AWSOpsWorks using the specified AWS account credentials.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentials The AWS credentials (access key ID and secret key) to use
* when authenticating with AWS services.
*/
public AWSOpsWorksClient(AWSCredentials awsCredentials) {
this(awsCredentials, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service methods on
* AWSOpsWorks using the specified AWS account credentials
* and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentials The AWS credentials (access key ID and secret key) to use
* when authenticating with AWS services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to AWSOpsWorks
* (ex: proxy settings, retry counts, etc.).
*/
public AWSOpsWorksClient(AWSCredentials awsCredentials, ClientConfiguration clientConfiguration) {
super(adjustClientConfiguration(clientConfiguration));
this.awsCredentialsProvider = new StaticCredentialsProvider(awsCredentials);
init();
}
/**
* Constructs a new client to invoke service methods on
* AWSOpsWorks using the specified AWS account credentials provider.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
*/
public AWSOpsWorksClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service methods on
* AWSOpsWorks using the specified AWS account credentials
* provider and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to AWSOpsWorks
* (ex: proxy settings, retry counts, etc.).
*/
public AWSOpsWorksClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on
* AWSOpsWorks using the specified AWS account credentials
* provider, client configuration options and request metric collector.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to AWSOpsWorks
* (ex: proxy settings, retry counts, etc.).
* @param requestMetricCollector optional request metric collector
*/
public AWSOpsWorksClient(AWSCredentialsProvider awsCredentialsProvider,
ClientConfiguration clientConfiguration,
RequestMetricCollector requestMetricCollector) {
super(adjustClientConfiguration(clientConfiguration), requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
init();
}
private void init() {
jsonErrorUnmarshallers = new ArrayList();
jsonErrorUnmarshallers.add(new ValidationExceptionUnmarshaller());
jsonErrorUnmarshallers.add(new ResourceNotFoundExceptionUnmarshaller());
jsonErrorUnmarshallers.add(new JsonErrorUnmarshaller());
// calling this.setEndPoint(...) will also modify the signer accordingly
this.setEndpoint("opsworks.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain(
"/com/amazonaws/services/opsworks/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain(
"/com/amazonaws/services/opsworks/request.handler2s"));
}
private static ClientConfiguration adjustClientConfiguration(ClientConfiguration orig) {
ClientConfiguration config = orig;
return config;
}
/**
*
* Updates a user's SSH public key.
*
*
* Required Permissions : To use this action, an IAM user must
* have self-management enabled or an attached policy that explicitly
* grants permissions. For more information on user permissions, see
* Managing User Permissions
* .
*
*
* @param updateMyUserProfileRequest Container for the necessary
* parameters to execute the UpdateMyUserProfile service method on
* AWSOpsWorks.
*
*
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void updateMyUserProfile(UpdateMyUserProfileRequest updateMyUserProfileRequest) {
ExecutionContext executionContext = createExecutionContext(updateMyUserProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateMyUserProfileRequestMarshaller().marshall(updateMyUserProfileRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Deregisters an Amazon EBS volume. The volume can then be registered by
* another stack. For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param deregisterVolumeRequest Container for the necessary parameters
* to execute the DeregisterVolume service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deregisterVolume(DeregisterVolumeRequest deregisterVolumeRequest) {
ExecutionContext executionContext = createExecutionContext(deregisterVolumeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeregisterVolumeRequestMarshaller().marshall(deregisterVolumeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Specifies a stack's permissions. For more information, see
* Security and Permissions
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param setPermissionRequest Container for the necessary parameters to
* execute the SetPermission service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void setPermission(SetPermissionRequest setPermissionRequest) {
ExecutionContext executionContext = createExecutionContext(setPermissionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SetPermissionRequestMarshaller().marshall(setPermissionRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Requests a description of a set of instances.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeInstancesRequest Container for the necessary parameters
* to execute the DescribeInstances service method on AWSOpsWorks.
*
* @return The response from the DescribeInstances service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeInstancesResult describeInstances(DescribeInstancesRequest describeInstancesRequest) {
ExecutionContext executionContext = createExecutionContext(describeInstancesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeInstancesRequestMarshaller().marshall(describeInstancesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new DescribeInstancesResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Describes the permissions for a specified stack.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param describePermissionsRequest Container for the necessary
* parameters to execute the DescribePermissions service method on
* AWSOpsWorks.
*
* @return The response from the DescribePermissions service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribePermissionsResult describePermissions(DescribePermissionsRequest describePermissionsRequest) {
ExecutionContext executionContext = createExecutionContext(describePermissionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribePermissionsRequestMarshaller().marshall(describePermissionsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new DescribePermissionsResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Deletes a specified instance. You must stop an instance before you can
* delete it. For more information, see
* Deleting Instances
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param deleteInstanceRequest Container for the necessary parameters to
* execute the DeleteInstance service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteInstance(DeleteInstanceRequest deleteInstanceRequest) {
ExecutionContext executionContext = createExecutionContext(deleteInstanceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteInstanceRequestMarshaller().marshall(deleteInstanceRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Creates a clone of a specified stack. For more information, see
* Clone a Stack
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have an attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param cloneStackRequest Container for the necessary parameters to
* execute the CloneStack service method on AWSOpsWorks.
*
* @return The response from the CloneStack service method, as returned
* by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public CloneStackResult cloneStack(CloneStackRequest cloneStackRequest) {
ExecutionContext executionContext = createExecutionContext(cloneStackRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CloneStackRequestMarshaller().marshall(cloneStackRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new CloneStackResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Detaches a specified Elastic Load Balancing instance from its layer.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param detachElasticLoadBalancerRequest Container for the necessary
* parameters to execute the DetachElasticLoadBalancer service method on
* AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void detachElasticLoadBalancer(DetachElasticLoadBalancerRequest detachElasticLoadBalancerRequest) {
ExecutionContext executionContext = createExecutionContext(detachElasticLoadBalancerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DetachElasticLoadBalancerRequestMarshaller().marshall(detachElasticLoadBalancerRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Stops a specified instance. When you stop a standard instance, the
* data disappears and must be reinstalled when you restart the instance.
* You can stop an Amazon EBS-backed instance without losing data. For
* more information, see
* Starting, Stopping, and Rebooting Instances
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param stopInstanceRequest Container for the necessary parameters to
* execute the StopInstance service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void stopInstance(StopInstanceRequest stopInstanceRequest) {
ExecutionContext executionContext = createExecutionContext(stopInstanceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StopInstanceRequestMarshaller().marshall(stopInstanceRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Updates a specified app.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Deploy or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param updateAppRequest Container for the necessary parameters to
* execute the UpdateApp service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void updateApp(UpdateAppRequest updateAppRequest) {
ExecutionContext executionContext = createExecutionContext(updateAppRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateAppRequestMarshaller().marshall(updateAppRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Describes the results of specified commands.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeCommandsRequest Container for the necessary parameters
* to execute the DescribeCommands service method on AWSOpsWorks.
*
* @return The response from the DescribeCommands service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeCommandsResult describeCommands(DescribeCommandsRequest describeCommandsRequest) {
ExecutionContext executionContext = createExecutionContext(describeCommandsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeCommandsRequestMarshaller().marshall(describeCommandsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new DescribeCommandsResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Associates one of the stack's registered Elastic IP addresses with a
* specified instance. The address must first be registered with the
* stack by calling RegisterElasticIp. For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param associateElasticIpRequest Container for the necessary
* parameters to execute the AssociateElasticIp service method on
* AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void associateElasticIp(AssociateElasticIpRequest associateElasticIpRequest) {
ExecutionContext executionContext = createExecutionContext(associateElasticIpRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateElasticIpRequestMarshaller().marshall(associateElasticIpRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Unassigns an assigned Amazon EBS volume. The volume remains registered
* with the stack. For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param unassignVolumeRequest Container for the necessary parameters to
* execute the UnassignVolume service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void unassignVolume(UnassignVolumeRequest unassignVolumeRequest) {
ExecutionContext executionContext = createExecutionContext(unassignVolumeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UnassignVolumeRequestMarshaller().marshall(unassignVolumeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Describe an instance's RAID arrays.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeRaidArraysRequest Container for the necessary
* parameters to execute the DescribeRaidArrays service method on
* AWSOpsWorks.
*
* @return The response from the DescribeRaidArrays service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeRaidArraysResult describeRaidArrays(DescribeRaidArraysRequest describeRaidArraysRequest) {
ExecutionContext executionContext = createExecutionContext(describeRaidArraysRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeRaidArraysRequestMarshaller().marshall(describeRaidArraysRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new DescribeRaidArraysResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Gets a generated host name for the specified layer, based on the
* current host name theme.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param getHostnameSuggestionRequest Container for the necessary
* parameters to execute the GetHostnameSuggestion service method on
* AWSOpsWorks.
*
* @return The response from the GetHostnameSuggestion service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public GetHostnameSuggestionResult getHostnameSuggestion(GetHostnameSuggestionRequest getHostnameSuggestionRequest) {
ExecutionContext executionContext = createExecutionContext(getHostnameSuggestionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetHostnameSuggestionRequestMarshaller().marshall(getHostnameSuggestionRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new GetHostnameSuggestionResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Specify the load-based auto scaling configuration for a specified
* layer. For more information, see
* Managing Load with Time-based and Load-based Instances
* .
*
*
* NOTE:To use load-based auto scaling, you must create a set of
* load-based auto scaling instances. Load-based auto scaling operates
* only on the instances from that set, so you must ensure that you have
* created enough instances to handle the maximum anticipated load.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param setLoadBasedAutoScalingRequest Container for the necessary
* parameters to execute the SetLoadBasedAutoScaling service method on
* AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void setLoadBasedAutoScaling(SetLoadBasedAutoScalingRequest setLoadBasedAutoScalingRequest) {
ExecutionContext executionContext = createExecutionContext(setLoadBasedAutoScalingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SetLoadBasedAutoScalingRequestMarshaller().marshall(setLoadBasedAutoScalingRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Describes an instance's Amazon EBS volumes.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeVolumesRequest Container for the necessary parameters
* to execute the DescribeVolumes service method on AWSOpsWorks.
*
* @return The response from the DescribeVolumes service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeVolumesResult describeVolumes(DescribeVolumesRequest describeVolumesRequest) {
ExecutionContext executionContext = createExecutionContext(describeVolumesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeVolumesRequestMarshaller().marshall(describeVolumesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new DescribeVolumesResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Assigns one of the stack's registered Amazon EBS volumes to a
* specified instance. The volume must first be registered with the stack
* by calling RegisterVolume. For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param assignVolumeRequest Container for the necessary parameters to
* execute the AssignVolume service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void assignVolume(AssignVolumeRequest assignVolumeRequest) {
ExecutionContext executionContext = createExecutionContext(assignVolumeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssignVolumeRequestMarshaller().marshall(assignVolumeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Describes AWS OpsWorks service errors.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeServiceErrorsRequest Container for the necessary
* parameters to execute the DescribeServiceErrors service method on
* AWSOpsWorks.
*
* @return The response from the DescribeServiceErrors service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeServiceErrorsResult describeServiceErrors(DescribeServiceErrorsRequest describeServiceErrorsRequest) {
ExecutionContext executionContext = createExecutionContext(describeServiceErrorsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeServiceErrorsRequestMarshaller().marshall(describeServiceErrorsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new DescribeServiceErrorsResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Updates a specified layer.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param updateLayerRequest Container for the necessary parameters to
* execute the UpdateLayer service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void updateLayer(UpdateLayerRequest updateLayerRequest) {
ExecutionContext executionContext = createExecutionContext(updateLayerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateLayerRequestMarshaller().marshall(updateLayerRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Updates a registered Elastic IP address's name. For more information,
* see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param updateElasticIpRequest Container for the necessary parameters
* to execute the UpdateElasticIp service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void updateElasticIp(UpdateElasticIpRequest updateElasticIpRequest) {
ExecutionContext executionContext = createExecutionContext(updateElasticIpRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateElasticIpRequestMarshaller().marshall(updateElasticIpRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Starts a specified instance. For more information, see
* Starting, Stopping, and Rebooting Instances
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param startInstanceRequest Container for the necessary parameters to
* execute the StartInstance service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void startInstance(StartInstanceRequest startInstanceRequest) {
ExecutionContext executionContext = createExecutionContext(startInstanceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartInstanceRequestMarshaller().marshall(startInstanceRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Creates a layer. For more information, see
* How to Create a Layer
* .
*
*
* NOTE:You should use CreateLayer for noncustom layer types such
* as PHP App Server only if the stack does not have an existing layer of
* that type. A stack can have at most one instance of each noncustom
* layer; if you attempt to create a second instance, CreateLayer fails.
* A stack can have an arbitrary number of custom layers, so you can call
* CreateLayer as many times as you like for that layer type.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param createLayerRequest Container for the necessary parameters to
* execute the CreateLayer service method on AWSOpsWorks.
*
* @return The response from the CreateLayer service method, as returned
* by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateLayerResult createLayer(CreateLayerRequest createLayerRequest) {
ExecutionContext executionContext = createExecutionContext(createLayerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateLayerRequestMarshaller().marshall(createLayerRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new CreateLayerResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Disassociates an Elastic IP address from its instance. The address
* remains registered with the stack. For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param disassociateElasticIpRequest Container for the necessary
* parameters to execute the DisassociateElasticIp service method on
* AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void disassociateElasticIp(DisassociateElasticIpRequest disassociateElasticIpRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateElasticIpRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateElasticIpRequestMarshaller().marshall(disassociateElasticIpRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Deletes a specified stack. You must first delete all instances,
* layers, and apps. For more information, see
* Shut Down a Stack
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param deleteStackRequest Container for the necessary parameters to
* execute the DeleteStack service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteStack(DeleteStackRequest deleteStackRequest) {
ExecutionContext executionContext = createExecutionContext(deleteStackRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteStackRequestMarshaller().marshall(deleteStackRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Starts stack's instances.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param startStackRequest Container for the necessary parameters to
* execute the StartStack service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void startStack(StartStackRequest startStackRequest) {
ExecutionContext executionContext = createExecutionContext(startStackRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartStackRequestMarshaller().marshall(startStackRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Registers an Amazon EBS volume with a specified stack. A volume can be
* registered with only one stack at a time. If the volume is already
* registered, you must first deregister it by calling DeregisterVolume.
* For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param registerVolumeRequest Container for the necessary parameters to
* execute the RegisterVolume service method on AWSOpsWorks.
*
* @return The response from the RegisterVolume service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public RegisterVolumeResult registerVolume(RegisterVolumeRequest registerVolumeRequest) {
ExecutionContext executionContext = createExecutionContext(registerVolumeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RegisterVolumeRequestMarshaller().marshall(registerVolumeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new RegisterVolumeResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Updates a specified user profile.
*
*
* Required Permissions : To use this action, an IAM user must
* have an attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param updateUserProfileRequest Container for the necessary parameters
* to execute the UpdateUserProfile service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void updateUserProfile(UpdateUserProfileRequest updateUserProfileRequest) {
ExecutionContext executionContext = createExecutionContext(updateUserProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateUserProfileRequestMarshaller().marshall(updateUserProfileRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Reboots a specified instance. For more information, see
* Starting, Stopping, and Rebooting Instances
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param rebootInstanceRequest Container for the necessary parameters to
* execute the RebootInstance service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void rebootInstance(RebootInstanceRequest rebootInstanceRequest) {
ExecutionContext executionContext = createExecutionContext(rebootInstanceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RebootInstanceRequestMarshaller().marshall(rebootInstanceRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Creates a new stack. For more information, see
* Create a New Stack
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have an attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param createStackRequest Container for the necessary parameters to
* execute the CreateStack service method on AWSOpsWorks.
*
* @return The response from the CreateStack service method, as returned
* by AWSOpsWorks.
*
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateStackResult createStack(CreateStackRequest createStackRequest) {
ExecutionContext executionContext = createExecutionContext(createStackRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateStackRequestMarshaller().marshall(createStackRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new CreateStackResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Creates an instance in a specified stack. For more information, see
* Adding an Instance to a Layer
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param createInstanceRequest Container for the necessary parameters to
* execute the CreateInstance service method on AWSOpsWorks.
*
* @return The response from the CreateInstance service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateInstanceResult createInstance(CreateInstanceRequest createInstanceRequest) {
ExecutionContext executionContext = createExecutionContext(createInstanceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateInstanceRequestMarshaller().marshall(createInstanceRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new CreateInstanceResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Deletes a specified app.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param deleteAppRequest Container for the necessary parameters to
* execute the DeleteApp service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteApp(DeleteAppRequest deleteAppRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAppRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAppRequestMarshaller().marshall(deleteAppRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Deregisters a specified Elastic IP address. The address can then be
* registered by another stack. For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param deregisterElasticIpRequest Container for the necessary
* parameters to execute the DeregisterElasticIp service method on
* AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deregisterElasticIp(DeregisterElasticIpRequest deregisterElasticIpRequest) {
ExecutionContext executionContext = createExecutionContext(deregisterElasticIpRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeregisterElasticIpRequestMarshaller().marshall(deregisterElasticIpRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Attaches an Elastic Load Balancing load balancer to a specified layer.
*
*
* NOTE:You must create the Elastic Load Balancing instance
* separately, by using the Elastic Load Balancing console, API, or CLI.
* For more information, see Elastic Load Balancing Developer Guide.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param attachElasticLoadBalancerRequest Container for the necessary
* parameters to execute the AttachElasticLoadBalancer service method on
* AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void attachElasticLoadBalancer(AttachElasticLoadBalancerRequest attachElasticLoadBalancerRequest) {
ExecutionContext executionContext = createExecutionContext(attachElasticLoadBalancerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AttachElasticLoadBalancerRequestMarshaller().marshall(attachElasticLoadBalancerRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Updates a specified stack.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param updateStackRequest Container for the necessary parameters to
* execute the UpdateStack service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void updateStack(UpdateStackRequest updateStackRequest) {
ExecutionContext executionContext = createExecutionContext(updateStackRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateStackRequestMarshaller().marshall(updateStackRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Creates a new user profile.
*
*
* Required Permissions : To use this action, an IAM user must
* have an attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param createUserProfileRequest Container for the necessary parameters
* to execute the CreateUserProfile service method on AWSOpsWorks.
*
* @return The response from the CreateUserProfile service method, as
* returned by AWSOpsWorks.
*
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateUserProfileResult createUserProfile(CreateUserProfileRequest createUserProfileRequest) {
ExecutionContext executionContext = createExecutionContext(createUserProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateUserProfileRequestMarshaller().marshall(createUserProfileRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new CreateUserProfileResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Requests a description of one or more layers in a specified stack.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeLayersRequest Container for the necessary parameters to
* execute the DescribeLayers service method on AWSOpsWorks.
*
* @return The response from the DescribeLayers service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeLayersResult describeLayers(DescribeLayersRequest describeLayersRequest) {
ExecutionContext executionContext = createExecutionContext(describeLayersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeLayersRequestMarshaller().marshall(describeLayersRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new DescribeLayersResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Describes load-based auto scaling configurations for specified layers.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeLoadBasedAutoScalingRequest Container for the necessary
* parameters to execute the DescribeLoadBasedAutoScaling service method
* on AWSOpsWorks.
*
* @return The response from the DescribeLoadBasedAutoScaling service
* method, as returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeLoadBasedAutoScalingResult describeLoadBasedAutoScaling(DescribeLoadBasedAutoScalingRequest describeLoadBasedAutoScalingRequest) {
ExecutionContext executionContext = createExecutionContext(describeLoadBasedAutoScalingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeLoadBasedAutoScalingRequestMarshaller().marshall(describeLoadBasedAutoScalingRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new DescribeLoadBasedAutoScalingResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Describes a stack's Elastic Load Balancing instances.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeElasticLoadBalancersRequest Container for the necessary
* parameters to execute the DescribeElasticLoadBalancers service method
* on AWSOpsWorks.
*
* @return The response from the DescribeElasticLoadBalancers service
* method, as returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeElasticLoadBalancersResult describeElasticLoadBalancers(DescribeElasticLoadBalancersRequest describeElasticLoadBalancersRequest) {
ExecutionContext executionContext = createExecutionContext(describeElasticLoadBalancersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeElasticLoadBalancersRequestMarshaller().marshall(describeElasticLoadBalancersRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new DescribeElasticLoadBalancersResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Deletes a specified layer. You must first stop and then delete all
* associated instances. For more information, see
* How to Delete a Layer
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param deleteLayerRequest Container for the necessary parameters to
* execute the DeleteLayer service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteLayer(DeleteLayerRequest deleteLayerRequest) {
ExecutionContext executionContext = createExecutionContext(deleteLayerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteLayerRequestMarshaller().marshall(deleteLayerRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Describes a user's SSH information.
*
*
* Required Permissions : To use this action, an IAM user must
* have self-management enabled or an attached policy that explicitly
* grants permissions. For more information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeMyUserProfileRequest Container for the necessary
* parameters to execute the DescribeMyUserProfile service method on
* AWSOpsWorks.
*
* @return The response from the DescribeMyUserProfile service method, as
* returned by AWSOpsWorks.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeMyUserProfileResult describeMyUserProfile(DescribeMyUserProfileRequest describeMyUserProfileRequest) {
ExecutionContext executionContext = createExecutionContext(describeMyUserProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeMyUserProfileRequestMarshaller().marshall(describeMyUserProfileRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new DescribeMyUserProfileResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Specify the time-based auto scaling configuration for a specified
* instance. For more information, see
* Managing Load with Time-based and Load-based Instances
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param setTimeBasedAutoScalingRequest Container for the necessary
* parameters to execute the SetTimeBasedAutoScaling service method on
* AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void setTimeBasedAutoScaling(SetTimeBasedAutoScalingRequest setTimeBasedAutoScalingRequest) {
ExecutionContext executionContext = createExecutionContext(setTimeBasedAutoScalingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SetTimeBasedAutoScalingRequestMarshaller().marshall(setTimeBasedAutoScalingRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Creates an app for a specified stack. For more information, see
* Creating Apps
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param createAppRequest Container for the necessary parameters to
* execute the CreateApp service method on AWSOpsWorks.
*
* @return The response from the CreateApp service method, as returned by
* AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateAppResult createApp(CreateAppRequest createAppRequest) {
ExecutionContext executionContext = createExecutionContext(createAppRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAppRequestMarshaller().marshall(createAppRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new CreateAppResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Deletes a user profile.
*
*
* Required Permissions : To use this action, an IAM user must
* have an attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param deleteUserProfileRequest Container for the necessary parameters
* to execute the DeleteUserProfile service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteUserProfile(DeleteUserProfileRequest deleteUserProfileRequest) {
ExecutionContext executionContext = createExecutionContext(deleteUserProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteUserProfileRequestMarshaller().marshall(deleteUserProfileRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Describes time-based auto scaling configurations for specified
* instances.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeTimeBasedAutoScalingRequest Container for the necessary
* parameters to execute the DescribeTimeBasedAutoScaling service method
* on AWSOpsWorks.
*
* @return The response from the DescribeTimeBasedAutoScaling service
* method, as returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeTimeBasedAutoScalingResult describeTimeBasedAutoScaling(DescribeTimeBasedAutoScalingRequest describeTimeBasedAutoScalingRequest) {
ExecutionContext executionContext = createExecutionContext(describeTimeBasedAutoScalingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeTimeBasedAutoScalingRequestMarshaller().marshall(describeTimeBasedAutoScalingRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new DescribeTimeBasedAutoScalingResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Updates an Amazon EBS volume's name or mount point. For more
* information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param updateVolumeRequest Container for the necessary parameters to
* execute the UpdateVolume service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void updateVolume(UpdateVolumeRequest updateVolumeRequest) {
ExecutionContext executionContext = createExecutionContext(updateVolumeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateVolumeRequestMarshaller().marshall(updateVolumeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Describe specified users.
*
*
* Required Permissions : To use this action, an IAM user must
* have an attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeUserProfilesRequest Container for the necessary
* parameters to execute the DescribeUserProfiles service method on
* AWSOpsWorks.
*
* @return The response from the DescribeUserProfiles service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeUserProfilesResult describeUserProfiles(DescribeUserProfilesRequest describeUserProfilesRequest) {
ExecutionContext executionContext = createExecutionContext(describeUserProfilesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeUserProfilesRequestMarshaller().marshall(describeUserProfilesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new DescribeUserProfilesResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Updates a specified instance.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param updateInstanceRequest Container for the necessary parameters to
* execute the UpdateInstance service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void updateInstance(UpdateInstanceRequest updateInstanceRequest) {
ExecutionContext executionContext = createExecutionContext(updateInstanceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateInstanceRequestMarshaller().marshall(updateInstanceRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Requests a description of a specified set of deployments.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeDeploymentsRequest Container for the necessary
* parameters to execute the DescribeDeployments service method on
* AWSOpsWorks.
*
* @return The response from the DescribeDeployments service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeDeploymentsResult describeDeployments(DescribeDeploymentsRequest describeDeploymentsRequest) {
ExecutionContext executionContext = createExecutionContext(describeDeploymentsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDeploymentsRequestMarshaller().marshall(describeDeploymentsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new DescribeDeploymentsResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Registers an Elastic IP address with a specified stack. An address can
* be registered with only one stack at a time. If the address is already
* registered, you must first deregister it by calling
* DeregisterElasticIp. For more information, see
* Resource Management
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param registerElasticIpRequest Container for the necessary parameters
* to execute the RegisterElasticIp service method on AWSOpsWorks.
*
* @return The response from the RegisterElasticIp service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public RegisterElasticIpResult registerElasticIp(RegisterElasticIpRequest registerElasticIpRequest) {
ExecutionContext executionContext = createExecutionContext(registerElasticIpRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RegisterElasticIpRequestMarshaller().marshall(registerElasticIpRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new RegisterElasticIpResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Describes
* Elastic IP addresses
* .
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeElasticIpsRequest Container for the necessary
* parameters to execute the DescribeElasticIps service method on
* AWSOpsWorks.
*
* @return The response from the DescribeElasticIps service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeElasticIpsResult describeElasticIps(DescribeElasticIpsRequest describeElasticIpsRequest) {
ExecutionContext executionContext = createExecutionContext(describeElasticIpsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeElasticIpsRequestMarshaller().marshall(describeElasticIpsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new DescribeElasticIpsResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Requests a description of one or more stacks.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeStacksRequest Container for the necessary parameters to
* execute the DescribeStacks service method on AWSOpsWorks.
*
* @return The response from the DescribeStacks service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeStacksResult describeStacks(DescribeStacksRequest describeStacksRequest) {
ExecutionContext executionContext = createExecutionContext(describeStacksRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeStacksRequestMarshaller().marshall(describeStacksRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new DescribeStacksResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Requests a description of a specified set of apps.
*
*
* NOTE:You must specify at least one of the parameters.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeAppsRequest Container for the necessary parameters to
* execute the DescribeApps service method on AWSOpsWorks.
*
* @return The response from the DescribeApps service method, as returned
* by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeAppsResult describeApps(DescribeAppsRequest describeAppsRequest) {
ExecutionContext executionContext = createExecutionContext(describeAppsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAppsRequestMarshaller().marshall(describeAppsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new DescribeAppsResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Describes the number of layers and apps in a specified stack, and the
* number of instances in each state, such as running_setup
* or online
.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Show, Deploy, or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param describeStackSummaryRequest Container for the necessary
* parameters to execute the DescribeStackSummary service method on
* AWSOpsWorks.
*
* @return The response from the DescribeStackSummary service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeStackSummaryResult describeStackSummary(DescribeStackSummaryRequest describeStackSummaryRequest) {
ExecutionContext executionContext = createExecutionContext(describeStackSummaryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeStackSummaryRequestMarshaller().marshall(describeStackSummaryRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new DescribeStackSummaryResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
/**
*
* Stops a specified stack.
*
*
* Required Permissions : To use this action, an IAM user must
* have a Manage permissions level for the stack, or an attached policy
* that explicitly grants permissions. For more information on user
* permissions, see
* Managing User Permissions
* .
*
*
* @param stopStackRequest Container for the necessary parameters to
* execute the StopStack service method on AWSOpsWorks.
*
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public void stopStack(StopStackRequest stopStackRequest) {
ExecutionContext executionContext = createExecutionContext(stopStackRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request;
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StopStackRequestMarshaller().marshall(stopStackRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = new JsonResponseHandler(null);
invoke(request, responseHandler, executionContext);
}
/**
*
* Deploys a stack or app.
*
*
*
* - App deployment generates a
deploy
event, which runs
* the associated recipes and passes them a JSON stack configuration
* object that includes information about the app.
* - Stack deployment runs the
deploy
recipes but does
* not raise an event.
*
*
*
* For more information, see
* Deploying Apps and Run Stack Commands
* .
*
*
* Required Permissions : To use this action, an IAM user must
* have a Deploy or Manage permissions level for the stack, or an
* attached policy that explicitly grants permissions. For more
* information on user permissions, see
* Managing User Permissions
* .
*
*
* @param createDeploymentRequest Container for the necessary parameters
* to execute the CreateDeployment service method on AWSOpsWorks.
*
* @return The response from the CreateDeployment service method, as
* returned by AWSOpsWorks.
*
* @throws ResourceNotFoundException
* @throws ValidationException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AWSOpsWorks indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateDeploymentResult createDeployment(CreateDeploymentRequest createDeploymentRequest) {
ExecutionContext executionContext = createExecutionContext(createDeploymentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDeploymentRequestMarshaller().marshall(createDeploymentRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
Unmarshaller unmarshaller = new CreateDeploymentResultJsonUnmarshaller();
JsonResponseHandler responseHandler = new JsonResponseHandler(unmarshaller);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response, LOGGING_AWS_REQUEST_METRIC);
}
}
@Override
public void setEndpoint(String endpoint) {
super.setEndpoint(endpoint);
}
@Override
public void setEndpoint(String endpoint, String serviceName, String regionId) throws IllegalArgumentException {
super.setEndpoint(endpoint, serviceName, regionId);
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for
* debugging issues where a service isn't acting as expected. This data isn't considered part
* of the result data returned by an operation, so it's available through this separate,
* diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access
* this extra diagnostic information for an executed request, you should use this method
* to retrieve it as soon as possible after executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none
* is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
private Response invoke(Request request,
HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
request.setEndpoint(endpoint);
request.setTimeOffset(timeOffset);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
AWSCredentials credentials;
awsRequestMetrics.startEvent(Field.CredentialsRequestTime);
try {
credentials = awsCredentialsProvider.getCredentials();
} finally {
awsRequestMetrics.endEvent(Field.CredentialsRequestTime);
}
AmazonWebServiceRequest originalRequest = request.getOriginalRequest();
if (originalRequest != null && originalRequest.getRequestCredentials() != null) {
credentials = originalRequest.getRequestCredentials();
}
executionContext.setCredentials(credentials);
JsonErrorResponseHandler errorResponseHandler = new JsonErrorResponseHandler(jsonErrorUnmarshallers);
Response result = client.execute(request, responseHandler,
errorResponseHandler, executionContext);
return result;
}
}