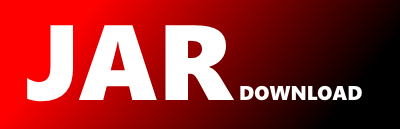
com.amazonaws.services.opsworks.model.DeploymentCommand Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.opsworks.model;
import java.io.Serializable;
/**
*
* Used to specify a deployment operation.
*
*/
public class DeploymentCommand implements Serializable {
/**
* Specifies the deployment operation. You can specify only one command.
* For stacks, the available commands are:
* execute_recipes
: Execute the recipes that are
* specified by the Args
parameter.
* install_dependencies
: Installs the stack's
* dependencies. update_custom_cookbooks
: Update
* the stack's custom cookbooks.
* update_dependencies
: Update the stack's
* dependencies.
For apps, the available commands are:
* deploy
: Deploy the app.
* rollback
Roll the app back to the previous version.
* When you update an app, AWS OpsWorks stores the previous version, up
* to a maximum of five versions. You can use this command to roll an app
* back as many as four versions. start
: Start the
* app's web or application server. stop
: Stop the
* app's web or application server. restart
:
* Restart the app's web or application server.
* undeploy
: Undeploy the app.
*
* Constraints:
* Allowed Values: install_dependencies, update_dependencies, update_custom_cookbooks, execute_recipes, deploy, rollback, start, stop, restart, undeploy
*/
private String name;
/**
* An array of command arguments. This parameter is currently used only
* to specify the list of recipes to be executed by the
* ExecuteRecipes
command.
*/
private java.util.Map> args;
/**
* Specifies the deployment operation. You can specify only one command.
* For stacks, the available commands are:
* execute_recipes
: Execute the recipes that are
* specified by the Args
parameter.
* install_dependencies
: Installs the stack's
* dependencies. update_custom_cookbooks
: Update
* the stack's custom cookbooks.
* update_dependencies
: Update the stack's
* dependencies.
For apps, the available commands are:
* deploy
: Deploy the app.
* rollback
Roll the app back to the previous version.
* When you update an app, AWS OpsWorks stores the previous version, up
* to a maximum of five versions. You can use this command to roll an app
* back as many as four versions. start
: Start the
* app's web or application server. stop
: Stop the
* app's web or application server. restart
:
* Restart the app's web or application server.
* undeploy
: Undeploy the app.
*
* Constraints:
* Allowed Values: install_dependencies, update_dependencies, update_custom_cookbooks, execute_recipes, deploy, rollback, start, stop, restart, undeploy
*
* @return Specifies the deployment operation. You can specify only one command.
*
For stacks, the available commands are:
* execute_recipes
: Execute the recipes that are
* specified by the Args
parameter.
* install_dependencies
: Installs the stack's
* dependencies. update_custom_cookbooks
: Update
* the stack's custom cookbooks.
* update_dependencies
: Update the stack's
* dependencies.
For apps, the available commands are:
* deploy
: Deploy the app.
* rollback
Roll the app back to the previous version.
* When you update an app, AWS OpsWorks stores the previous version, up
* to a maximum of five versions. You can use this command to roll an app
* back as many as four versions. start
: Start the
* app's web or application server. stop
: Stop the
* app's web or application server. restart
:
* Restart the app's web or application server.
* undeploy
: Undeploy the app.
*
* @see DeploymentCommandName
*/
public String getName() {
return name;
}
/**
* Specifies the deployment operation. You can specify only one command.
* For stacks, the available commands are:
* execute_recipes
: Execute the recipes that are
* specified by the Args
parameter.
* install_dependencies
: Installs the stack's
* dependencies. update_custom_cookbooks
: Update
* the stack's custom cookbooks.
* update_dependencies
: Update the stack's
* dependencies.
For apps, the available commands are:
* deploy
: Deploy the app.
* rollback
Roll the app back to the previous version.
* When you update an app, AWS OpsWorks stores the previous version, up
* to a maximum of five versions. You can use this command to roll an app
* back as many as four versions. start
: Start the
* app's web or application server. stop
: Stop the
* app's web or application server. restart
:
* Restart the app's web or application server.
* undeploy
: Undeploy the app.
*
* Constraints:
* Allowed Values: install_dependencies, update_dependencies, update_custom_cookbooks, execute_recipes, deploy, rollback, start, stop, restart, undeploy
*
* @param name Specifies the deployment operation. You can specify only one command.
*
For stacks, the available commands are:
* execute_recipes
: Execute the recipes that are
* specified by the Args
parameter.
* install_dependencies
: Installs the stack's
* dependencies. update_custom_cookbooks
: Update
* the stack's custom cookbooks.
* update_dependencies
: Update the stack's
* dependencies.
For apps, the available commands are:
* deploy
: Deploy the app.
* rollback
Roll the app back to the previous version.
* When you update an app, AWS OpsWorks stores the previous version, up
* to a maximum of five versions. You can use this command to roll an app
* back as many as four versions. start
: Start the
* app's web or application server. stop
: Stop the
* app's web or application server. restart
:
* Restart the app's web or application server.
* undeploy
: Undeploy the app.
*
* @see DeploymentCommandName
*/
public void setName(String name) {
this.name = name;
}
/**
* Specifies the deployment operation. You can specify only one command.
* For stacks, the available commands are:
* execute_recipes
: Execute the recipes that are
* specified by the Args
parameter.
* install_dependencies
: Installs the stack's
* dependencies. update_custom_cookbooks
: Update
* the stack's custom cookbooks.
* update_dependencies
: Update the stack's
* dependencies.
For apps, the available commands are:
* deploy
: Deploy the app.
* rollback
Roll the app back to the previous version.
* When you update an app, AWS OpsWorks stores the previous version, up
* to a maximum of five versions. You can use this command to roll an app
* back as many as four versions. start
: Start the
* app's web or application server. stop
: Stop the
* app's web or application server. restart
:
* Restart the app's web or application server.
* undeploy
: Undeploy the app.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: install_dependencies, update_dependencies, update_custom_cookbooks, execute_recipes, deploy, rollback, start, stop, restart, undeploy
*
* @param name Specifies the deployment operation. You can specify only one command.
*
For stacks, the available commands are:
* execute_recipes
: Execute the recipes that are
* specified by the Args
parameter.
* install_dependencies
: Installs the stack's
* dependencies. update_custom_cookbooks
: Update
* the stack's custom cookbooks.
* update_dependencies
: Update the stack's
* dependencies.
For apps, the available commands are:
* deploy
: Deploy the app.
* rollback
Roll the app back to the previous version.
* When you update an app, AWS OpsWorks stores the previous version, up
* to a maximum of five versions. You can use this command to roll an app
* back as many as four versions. start
: Start the
* app's web or application server. stop
: Stop the
* app's web or application server. restart
:
* Restart the app's web or application server.
* undeploy
: Undeploy the app.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see DeploymentCommandName
*/
public DeploymentCommand withName(String name) {
this.name = name;
return this;
}
/**
* Specifies the deployment operation. You can specify only one command.
* For stacks, the available commands are:
* execute_recipes
: Execute the recipes that are
* specified by the Args
parameter.
* install_dependencies
: Installs the stack's
* dependencies. update_custom_cookbooks
: Update
* the stack's custom cookbooks.
* update_dependencies
: Update the stack's
* dependencies.
For apps, the available commands are:
* deploy
: Deploy the app.
* rollback
Roll the app back to the previous version.
* When you update an app, AWS OpsWorks stores the previous version, up
* to a maximum of five versions. You can use this command to roll an app
* back as many as four versions. start
: Start the
* app's web or application server. stop
: Stop the
* app's web or application server. restart
:
* Restart the app's web or application server.
* undeploy
: Undeploy the app.
*
* Constraints:
* Allowed Values: install_dependencies, update_dependencies, update_custom_cookbooks, execute_recipes, deploy, rollback, start, stop, restart, undeploy
*
* @param name Specifies the deployment operation. You can specify only one command.
*
For stacks, the available commands are:
* execute_recipes
: Execute the recipes that are
* specified by the Args
parameter.
* install_dependencies
: Installs the stack's
* dependencies. update_custom_cookbooks
: Update
* the stack's custom cookbooks.
* update_dependencies
: Update the stack's
* dependencies.
For apps, the available commands are:
* deploy
: Deploy the app.
* rollback
Roll the app back to the previous version.
* When you update an app, AWS OpsWorks stores the previous version, up
* to a maximum of five versions. You can use this command to roll an app
* back as many as four versions. start
: Start the
* app's web or application server. stop
: Stop the
* app's web or application server. restart
:
* Restart the app's web or application server.
* undeploy
: Undeploy the app.
*
* @see DeploymentCommandName
*/
public void setName(DeploymentCommandName name) {
this.name = name.toString();
}
/**
* Specifies the deployment operation. You can specify only one command.
* For stacks, the available commands are:
* execute_recipes
: Execute the recipes that are
* specified by the Args
parameter.
* install_dependencies
: Installs the stack's
* dependencies. update_custom_cookbooks
: Update
* the stack's custom cookbooks.
* update_dependencies
: Update the stack's
* dependencies.
For apps, the available commands are:
* deploy
: Deploy the app.
* rollback
Roll the app back to the previous version.
* When you update an app, AWS OpsWorks stores the previous version, up
* to a maximum of five versions. You can use this command to roll an app
* back as many as four versions. start
: Start the
* app's web or application server. stop
: Stop the
* app's web or application server. restart
:
* Restart the app's web or application server.
* undeploy
: Undeploy the app.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Allowed Values: install_dependencies, update_dependencies, update_custom_cookbooks, execute_recipes, deploy, rollback, start, stop, restart, undeploy
*
* @param name Specifies the deployment operation. You can specify only one command.
*
For stacks, the available commands are:
* execute_recipes
: Execute the recipes that are
* specified by the Args
parameter.
* install_dependencies
: Installs the stack's
* dependencies. update_custom_cookbooks
: Update
* the stack's custom cookbooks.
* update_dependencies
: Update the stack's
* dependencies.
For apps, the available commands are:
* deploy
: Deploy the app.
* rollback
Roll the app back to the previous version.
* When you update an app, AWS OpsWorks stores the previous version, up
* to a maximum of five versions. You can use this command to roll an app
* back as many as four versions. start
: Start the
* app's web or application server. stop
: Stop the
* app's web or application server. restart
:
* Restart the app's web or application server.
* undeploy
: Undeploy the app.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*
* @see DeploymentCommandName
*/
public DeploymentCommand withName(DeploymentCommandName name) {
this.name = name.toString();
return this;
}
/**
* An array of command arguments. This parameter is currently used only
* to specify the list of recipes to be executed by the
* ExecuteRecipes
command.
*
* @return An array of command arguments. This parameter is currently used only
* to specify the list of recipes to be executed by the
* ExecuteRecipes
command.
*/
public java.util.Map> getArgs() {
if (args == null) {
args = new java.util.HashMap>();
}
return args;
}
/**
* An array of command arguments. This parameter is currently used only
* to specify the list of recipes to be executed by the
* ExecuteRecipes
command.
*
* @param args An array of command arguments. This parameter is currently used only
* to specify the list of recipes to be executed by the
* ExecuteRecipes
command.
*/
public void setArgs(java.util.Map> args) {
this.args = args;
}
/**
* An array of command arguments. This parameter is currently used only
* to specify the list of recipes to be executed by the
* ExecuteRecipes
command.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param args An array of command arguments. This parameter is currently used only
* to specify the list of recipes to be executed by the
* ExecuteRecipes
command.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public DeploymentCommand withArgs(java.util.Map> args) {
setArgs(args);
return this;
}
/**
* An array of command arguments. This parameter is currently used only
* to specify the list of recipes to be executed by the
* ExecuteRecipes
command.
*
* The method adds a new key-value pair into Args parameter, and returns
* a reference to this object so that method calls can be chained
* together.
*
* @param key The key of the entry to be added into Args.
* @param value The corresponding value of the entry to be added into Args.
*/
public DeploymentCommand addArgsEntry(String key, java.util.List value) {
if (null == this.args) {
this.args = new java.util.HashMap>();
}
if (this.args.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.args.put(key, value);
return this;
}
/**
* Removes all the entries added into Args.
*
* Returns a reference to this object so that method calls can be chained together.
*/
public DeploymentCommand clearArgsEntries() {
this.args = null;
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null) sb.append("Name: " + getName() + ",");
if (getArgs() != null) sb.append("Args: " + getArgs() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getArgs() == null) ? 0 : getArgs().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof DeploymentCommand == false) return false;
DeploymentCommand other = (DeploymentCommand)obj;
if (other.getName() == null ^ this.getName() == null) return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false) return false;
if (other.getArgs() == null ^ this.getArgs() == null) return false;
if (other.getArgs() != null && other.getArgs().equals(this.getArgs()) == false) return false;
return true;
}
}