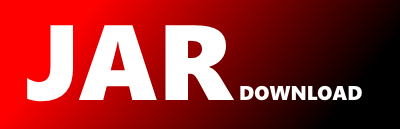
com.amazonaws.services.opsworks.model.InstancesCount Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.opsworks.model;
import java.io.Serializable;
/**
*
* Describes how many instances a stack has for each status.
*
*/
public class InstancesCount implements Serializable {
/**
* The number of instances with booting
status.
*/
private Integer booting;
/**
* The number of instances with connection_lost
status.
*/
private Integer connectionLost;
/**
* The number of instances with pending
status.
*/
private Integer pending;
/**
* The number of instances with rebooting
status.
*/
private Integer rebooting;
/**
* The number of instances with requested
status.
*/
private Integer requested;
/**
* The number of instances with running_setup
status.
*/
private Integer runningSetup;
/**
* The number of instances with setup_failed
status.
*/
private Integer setupFailed;
/**
* The number of instances with shutting_down
status.
*/
private Integer shuttingDown;
/**
* The number of instances with start_failed
status.
*/
private Integer startFailed;
/**
* The number of instances with stopped
status.
*/
private Integer stopped;
/**
* The number of instances with terminated
status.
*/
private Integer terminated;
/**
* The number of instances with terminating
status.
*/
private Integer terminating;
/**
* The number of instances with booting
status.
*
* @return The number of instances with booting
status.
*/
public Integer getBooting() {
return booting;
}
/**
* The number of instances with booting
status.
*
* @param booting The number of instances with booting
status.
*/
public void setBooting(Integer booting) {
this.booting = booting;
}
/**
* The number of instances with booting
status.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param booting The number of instances with booting
status.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public InstancesCount withBooting(Integer booting) {
this.booting = booting;
return this;
}
/**
* The number of instances with connection_lost
status.
*
* @return The number of instances with connection_lost
status.
*/
public Integer getConnectionLost() {
return connectionLost;
}
/**
* The number of instances with connection_lost
status.
*
* @param connectionLost The number of instances with connection_lost
status.
*/
public void setConnectionLost(Integer connectionLost) {
this.connectionLost = connectionLost;
}
/**
* The number of instances with connection_lost
status.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param connectionLost The number of instances with connection_lost
status.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public InstancesCount withConnectionLost(Integer connectionLost) {
this.connectionLost = connectionLost;
return this;
}
/**
* The number of instances with pending
status.
*
* @return The number of instances with pending
status.
*/
public Integer getPending() {
return pending;
}
/**
* The number of instances with pending
status.
*
* @param pending The number of instances with pending
status.
*/
public void setPending(Integer pending) {
this.pending = pending;
}
/**
* The number of instances with pending
status.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param pending The number of instances with pending
status.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public InstancesCount withPending(Integer pending) {
this.pending = pending;
return this;
}
/**
* The number of instances with rebooting
status.
*
* @return The number of instances with rebooting
status.
*/
public Integer getRebooting() {
return rebooting;
}
/**
* The number of instances with rebooting
status.
*
* @param rebooting The number of instances with rebooting
status.
*/
public void setRebooting(Integer rebooting) {
this.rebooting = rebooting;
}
/**
* The number of instances with rebooting
status.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param rebooting The number of instances with rebooting
status.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public InstancesCount withRebooting(Integer rebooting) {
this.rebooting = rebooting;
return this;
}
/**
* The number of instances with requested
status.
*
* @return The number of instances with requested
status.
*/
public Integer getRequested() {
return requested;
}
/**
* The number of instances with requested
status.
*
* @param requested The number of instances with requested
status.
*/
public void setRequested(Integer requested) {
this.requested = requested;
}
/**
* The number of instances with requested
status.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param requested The number of instances with requested
status.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public InstancesCount withRequested(Integer requested) {
this.requested = requested;
return this;
}
/**
* The number of instances with running_setup
status.
*
* @return The number of instances with running_setup
status.
*/
public Integer getRunningSetup() {
return runningSetup;
}
/**
* The number of instances with running_setup
status.
*
* @param runningSetup The number of instances with running_setup
status.
*/
public void setRunningSetup(Integer runningSetup) {
this.runningSetup = runningSetup;
}
/**
* The number of instances with running_setup
status.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param runningSetup The number of instances with running_setup
status.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public InstancesCount withRunningSetup(Integer runningSetup) {
this.runningSetup = runningSetup;
return this;
}
/**
* The number of instances with setup_failed
status.
*
* @return The number of instances with setup_failed
status.
*/
public Integer getSetupFailed() {
return setupFailed;
}
/**
* The number of instances with setup_failed
status.
*
* @param setupFailed The number of instances with setup_failed
status.
*/
public void setSetupFailed(Integer setupFailed) {
this.setupFailed = setupFailed;
}
/**
* The number of instances with setup_failed
status.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param setupFailed The number of instances with setup_failed
status.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public InstancesCount withSetupFailed(Integer setupFailed) {
this.setupFailed = setupFailed;
return this;
}
/**
* The number of instances with shutting_down
status.
*
* @return The number of instances with shutting_down
status.
*/
public Integer getShuttingDown() {
return shuttingDown;
}
/**
* The number of instances with shutting_down
status.
*
* @param shuttingDown The number of instances with shutting_down
status.
*/
public void setShuttingDown(Integer shuttingDown) {
this.shuttingDown = shuttingDown;
}
/**
* The number of instances with shutting_down
status.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param shuttingDown The number of instances with shutting_down
status.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public InstancesCount withShuttingDown(Integer shuttingDown) {
this.shuttingDown = shuttingDown;
return this;
}
/**
* The number of instances with start_failed
status.
*
* @return The number of instances with start_failed
status.
*/
public Integer getStartFailed() {
return startFailed;
}
/**
* The number of instances with start_failed
status.
*
* @param startFailed The number of instances with start_failed
status.
*/
public void setStartFailed(Integer startFailed) {
this.startFailed = startFailed;
}
/**
* The number of instances with start_failed
status.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param startFailed The number of instances with start_failed
status.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public InstancesCount withStartFailed(Integer startFailed) {
this.startFailed = startFailed;
return this;
}
/**
* The number of instances with stopped
status.
*
* @return The number of instances with stopped
status.
*/
public Integer getStopped() {
return stopped;
}
/**
* The number of instances with stopped
status.
*
* @param stopped The number of instances with stopped
status.
*/
public void setStopped(Integer stopped) {
this.stopped = stopped;
}
/**
* The number of instances with stopped
status.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param stopped The number of instances with stopped
status.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public InstancesCount withStopped(Integer stopped) {
this.stopped = stopped;
return this;
}
/**
* The number of instances with terminated
status.
*
* @return The number of instances with terminated
status.
*/
public Integer getTerminated() {
return terminated;
}
/**
* The number of instances with terminated
status.
*
* @param terminated The number of instances with terminated
status.
*/
public void setTerminated(Integer terminated) {
this.terminated = terminated;
}
/**
* The number of instances with terminated
status.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param terminated The number of instances with terminated
status.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public InstancesCount withTerminated(Integer terminated) {
this.terminated = terminated;
return this;
}
/**
* The number of instances with terminating
status.
*
* @return The number of instances with terminating
status.
*/
public Integer getTerminating() {
return terminating;
}
/**
* The number of instances with terminating
status.
*
* @param terminating The number of instances with terminating
status.
*/
public void setTerminating(Integer terminating) {
this.terminating = terminating;
}
/**
* The number of instances with terminating
status.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param terminating The number of instances with terminating
status.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public InstancesCount withTerminating(Integer terminating) {
this.terminating = terminating;
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getBooting() != null) sb.append("Booting: " + getBooting() + ",");
if (getConnectionLost() != null) sb.append("ConnectionLost: " + getConnectionLost() + ",");
if (getPending() != null) sb.append("Pending: " + getPending() + ",");
if (getRebooting() != null) sb.append("Rebooting: " + getRebooting() + ",");
if (getRequested() != null) sb.append("Requested: " + getRequested() + ",");
if (getRunningSetup() != null) sb.append("RunningSetup: " + getRunningSetup() + ",");
if (getSetupFailed() != null) sb.append("SetupFailed: " + getSetupFailed() + ",");
if (getShuttingDown() != null) sb.append("ShuttingDown: " + getShuttingDown() + ",");
if (getStartFailed() != null) sb.append("StartFailed: " + getStartFailed() + ",");
if (getStopped() != null) sb.append("Stopped: " + getStopped() + ",");
if (getTerminated() != null) sb.append("Terminated: " + getTerminated() + ",");
if (getTerminating() != null) sb.append("Terminating: " + getTerminating() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getBooting() == null) ? 0 : getBooting().hashCode());
hashCode = prime * hashCode + ((getConnectionLost() == null) ? 0 : getConnectionLost().hashCode());
hashCode = prime * hashCode + ((getPending() == null) ? 0 : getPending().hashCode());
hashCode = prime * hashCode + ((getRebooting() == null) ? 0 : getRebooting().hashCode());
hashCode = prime * hashCode + ((getRequested() == null) ? 0 : getRequested().hashCode());
hashCode = prime * hashCode + ((getRunningSetup() == null) ? 0 : getRunningSetup().hashCode());
hashCode = prime * hashCode + ((getSetupFailed() == null) ? 0 : getSetupFailed().hashCode());
hashCode = prime * hashCode + ((getShuttingDown() == null) ? 0 : getShuttingDown().hashCode());
hashCode = prime * hashCode + ((getStartFailed() == null) ? 0 : getStartFailed().hashCode());
hashCode = prime * hashCode + ((getStopped() == null) ? 0 : getStopped().hashCode());
hashCode = prime * hashCode + ((getTerminated() == null) ? 0 : getTerminated().hashCode());
hashCode = prime * hashCode + ((getTerminating() == null) ? 0 : getTerminating().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof InstancesCount == false) return false;
InstancesCount other = (InstancesCount)obj;
if (other.getBooting() == null ^ this.getBooting() == null) return false;
if (other.getBooting() != null && other.getBooting().equals(this.getBooting()) == false) return false;
if (other.getConnectionLost() == null ^ this.getConnectionLost() == null) return false;
if (other.getConnectionLost() != null && other.getConnectionLost().equals(this.getConnectionLost()) == false) return false;
if (other.getPending() == null ^ this.getPending() == null) return false;
if (other.getPending() != null && other.getPending().equals(this.getPending()) == false) return false;
if (other.getRebooting() == null ^ this.getRebooting() == null) return false;
if (other.getRebooting() != null && other.getRebooting().equals(this.getRebooting()) == false) return false;
if (other.getRequested() == null ^ this.getRequested() == null) return false;
if (other.getRequested() != null && other.getRequested().equals(this.getRequested()) == false) return false;
if (other.getRunningSetup() == null ^ this.getRunningSetup() == null) return false;
if (other.getRunningSetup() != null && other.getRunningSetup().equals(this.getRunningSetup()) == false) return false;
if (other.getSetupFailed() == null ^ this.getSetupFailed() == null) return false;
if (other.getSetupFailed() != null && other.getSetupFailed().equals(this.getSetupFailed()) == false) return false;
if (other.getShuttingDown() == null ^ this.getShuttingDown() == null) return false;
if (other.getShuttingDown() != null && other.getShuttingDown().equals(this.getShuttingDown()) == false) return false;
if (other.getStartFailed() == null ^ this.getStartFailed() == null) return false;
if (other.getStartFailed() != null && other.getStartFailed().equals(this.getStartFailed()) == false) return false;
if (other.getStopped() == null ^ this.getStopped() == null) return false;
if (other.getStopped() != null && other.getStopped().equals(this.getStopped()) == false) return false;
if (other.getTerminated() == null ^ this.getTerminated() == null) return false;
if (other.getTerminated() != null && other.getTerminated().equals(this.getTerminated()) == false) return false;
if (other.getTerminating() == null ^ this.getTerminating() == null) return false;
if (other.getTerminating() != null && other.getTerminating().equals(this.getTerminating()) == false) return false;
return true;
}
}