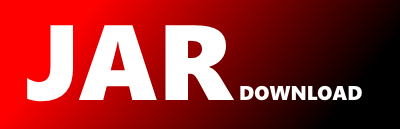
com.amazonaws.services.opsworks.model.Permission Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.opsworks.model;
import java.io.Serializable;
/**
*
* Describes stack or user permissions.
*
*/
public class Permission implements Serializable {
/**
* A stack ID.
*/
private String stackId;
/**
* The Amazon Resource Name (ARN) for an AWS Identity and Access
* Management (IAM) role. For more information about IAM ARNs, see Using
* Identifiers.
*/
private String iamUserArn;
/**
* Whether the user can use SSH.
*/
private Boolean allowSsh;
/**
* Whether the user can use sudo.
*/
private Boolean allowSudo;
/**
* The user's permission level, which must be the following:
* deny
show
* deploy
manage
* iam_only
For more information on the
* permissions associated with these levels, see Managing
* User Permissions
*/
private String level;
/**
* A stack ID.
*
* @return A stack ID.
*/
public String getStackId() {
return stackId;
}
/**
* A stack ID.
*
* @param stackId A stack ID.
*/
public void setStackId(String stackId) {
this.stackId = stackId;
}
/**
* A stack ID.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param stackId A stack ID.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Permission withStackId(String stackId) {
this.stackId = stackId;
return this;
}
/**
* The Amazon Resource Name (ARN) for an AWS Identity and Access
* Management (IAM) role. For more information about IAM ARNs, see Using
* Identifiers.
*
* @return The Amazon Resource Name (ARN) for an AWS Identity and Access
* Management (IAM) role. For more information about IAM ARNs, see Using
* Identifiers.
*/
public String getIamUserArn() {
return iamUserArn;
}
/**
* The Amazon Resource Name (ARN) for an AWS Identity and Access
* Management (IAM) role. For more information about IAM ARNs, see Using
* Identifiers.
*
* @param iamUserArn The Amazon Resource Name (ARN) for an AWS Identity and Access
* Management (IAM) role. For more information about IAM ARNs, see Using
* Identifiers.
*/
public void setIamUserArn(String iamUserArn) {
this.iamUserArn = iamUserArn;
}
/**
* The Amazon Resource Name (ARN) for an AWS Identity and Access
* Management (IAM) role. For more information about IAM ARNs, see Using
* Identifiers.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param iamUserArn The Amazon Resource Name (ARN) for an AWS Identity and Access
* Management (IAM) role. For more information about IAM ARNs, see Using
* Identifiers.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Permission withIamUserArn(String iamUserArn) {
this.iamUserArn = iamUserArn;
return this;
}
/**
* Whether the user can use SSH.
*
* @return Whether the user can use SSH.
*/
public Boolean isAllowSsh() {
return allowSsh;
}
/**
* Whether the user can use SSH.
*
* @param allowSsh Whether the user can use SSH.
*/
public void setAllowSsh(Boolean allowSsh) {
this.allowSsh = allowSsh;
}
/**
* Whether the user can use SSH.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param allowSsh Whether the user can use SSH.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Permission withAllowSsh(Boolean allowSsh) {
this.allowSsh = allowSsh;
return this;
}
/**
* Whether the user can use SSH.
*
* @return Whether the user can use SSH.
*/
public Boolean getAllowSsh() {
return allowSsh;
}
/**
* Whether the user can use sudo.
*
* @return Whether the user can use sudo.
*/
public Boolean isAllowSudo() {
return allowSudo;
}
/**
* Whether the user can use sudo.
*
* @param allowSudo Whether the user can use sudo.
*/
public void setAllowSudo(Boolean allowSudo) {
this.allowSudo = allowSudo;
}
/**
* Whether the user can use sudo.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param allowSudo Whether the user can use sudo.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Permission withAllowSudo(Boolean allowSudo) {
this.allowSudo = allowSudo;
return this;
}
/**
* Whether the user can use sudo.
*
* @return Whether the user can use sudo.
*/
public Boolean getAllowSudo() {
return allowSudo;
}
/**
* The user's permission level, which must be the following:
* deny
show
* deploy
manage
* iam_only
For more information on the
* permissions associated with these levels, see Managing
* User Permissions
*
* @return The user's permission level, which must be the following:
* deny
show
* deploy
manage
* iam_only
For more information on the
* permissions associated with these levels, see Managing
* User Permissions
*/
public String getLevel() {
return level;
}
/**
* The user's permission level, which must be the following:
* deny
show
* deploy
manage
* iam_only
For more information on the
* permissions associated with these levels, see Managing
* User Permissions
*
* @param level The user's permission level, which must be the following:
* deny
show
* deploy
manage
* iam_only
For more information on the
* permissions associated with these levels, see Managing
* User Permissions
*/
public void setLevel(String level) {
this.level = level;
}
/**
* The user's permission level, which must be the following:
* deny
show
* deploy
manage
* iam_only
For more information on the
* permissions associated with these levels, see Managing
* User Permissions
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param level The user's permission level, which must be the following:
* deny
show
* deploy
manage
* iam_only
For more information on the
* permissions associated with these levels, see Managing
* User Permissions
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Permission withLevel(String level) {
this.level = level;
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getStackId() != null) sb.append("StackId: " + getStackId() + ",");
if (getIamUserArn() != null) sb.append("IamUserArn: " + getIamUserArn() + ",");
if (isAllowSsh() != null) sb.append("AllowSsh: " + isAllowSsh() + ",");
if (isAllowSudo() != null) sb.append("AllowSudo: " + isAllowSudo() + ",");
if (getLevel() != null) sb.append("Level: " + getLevel() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getStackId() == null) ? 0 : getStackId().hashCode());
hashCode = prime * hashCode + ((getIamUserArn() == null) ? 0 : getIamUserArn().hashCode());
hashCode = prime * hashCode + ((isAllowSsh() == null) ? 0 : isAllowSsh().hashCode());
hashCode = prime * hashCode + ((isAllowSudo() == null) ? 0 : isAllowSudo().hashCode());
hashCode = prime * hashCode + ((getLevel() == null) ? 0 : getLevel().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof Permission == false) return false;
Permission other = (Permission)obj;
if (other.getStackId() == null ^ this.getStackId() == null) return false;
if (other.getStackId() != null && other.getStackId().equals(this.getStackId()) == false) return false;
if (other.getIamUserArn() == null ^ this.getIamUserArn() == null) return false;
if (other.getIamUserArn() != null && other.getIamUserArn().equals(this.getIamUserArn()) == false) return false;
if (other.isAllowSsh() == null ^ this.isAllowSsh() == null) return false;
if (other.isAllowSsh() != null && other.isAllowSsh().equals(this.isAllowSsh()) == false) return false;
if (other.isAllowSudo() == null ^ this.isAllowSudo() == null) return false;
if (other.isAllowSudo() != null && other.isAllowSudo().equals(this.isAllowSudo()) == false) return false;
if (other.getLevel() == null ^ this.getLevel() == null) return false;
if (other.getLevel() != null && other.getLevel().equals(this.getLevel()) == false) return false;
return true;
}
}