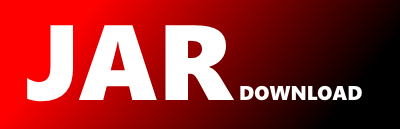
com.amazonaws.services.opsworks.model.Recipes Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.opsworks.model;
import java.io.Serializable;
/**
*
* AWS OpsWorks supports five lifecycle events, setup ,
* configuration ,
* deploy ,
* undeploy , and shutdown .
* For each layer, AWS OpsWorks runs a set of standard recipes
* for each event. In addition, you can provide custom recipes for any or
* all layers and events. AWS OpsWorks runs custom event recipes after
* the standard recipes. LayerCustomRecipes
specifies the
* custom recipes for a particular layer to be run in response to each of
* the five events.
*
*
* To specify a recipe, use the cookbook's directory name in the
* repository followed by two colons and the recipe name, which is the
* recipe's file name without the .rb extension. For example:
* phpapp2::dbsetup specifies the dbsetup.rb recipe in the repository's
* phpapp2 folder.
*
*/
public class Recipes implements Serializable {
/**
* An array of custom recipe names to be run following a
* setup
event.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag setup;
/**
* An array of custom recipe names to be run following a
* configure
event.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag configure;
/**
* An array of custom recipe names to be run following a
* deploy
event.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag deploy;
/**
* An array of custom recipe names to be run following a
* undeploy
event.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag undeploy;
/**
* An array of custom recipe names to be run following a
* shutdown
event.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag shutdown;
/**
* An array of custom recipe names to be run following a
* setup
event.
*
* @return An array of custom recipe names to be run following a
* setup
event.
*/
public java.util.List getSetup() {
if (setup == null) {
setup = new com.amazonaws.internal.ListWithAutoConstructFlag();
setup.setAutoConstruct(true);
}
return setup;
}
/**
* An array of custom recipe names to be run following a
* setup
event.
*
* @param setup An array of custom recipe names to be run following a
* setup
event.
*/
public void setSetup(java.util.Collection setup) {
if (setup == null) {
this.setup = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag setupCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(setup.size());
setupCopy.addAll(setup);
this.setup = setupCopy;
}
/**
* An array of custom recipe names to be run following a
* setup
event.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param setup An array of custom recipe names to be run following a
* setup
event.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Recipes withSetup(String... setup) {
if (getSetup() == null) setSetup(new java.util.ArrayList(setup.length));
for (String value : setup) {
getSetup().add(value);
}
return this;
}
/**
* An array of custom recipe names to be run following a
* setup
event.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param setup An array of custom recipe names to be run following a
* setup
event.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Recipes withSetup(java.util.Collection setup) {
if (setup == null) {
this.setup = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag setupCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(setup.size());
setupCopy.addAll(setup);
this.setup = setupCopy;
}
return this;
}
/**
* An array of custom recipe names to be run following a
* configure
event.
*
* @return An array of custom recipe names to be run following a
* configure
event.
*/
public java.util.List getConfigure() {
if (configure == null) {
configure = new com.amazonaws.internal.ListWithAutoConstructFlag();
configure.setAutoConstruct(true);
}
return configure;
}
/**
* An array of custom recipe names to be run following a
* configure
event.
*
* @param configure An array of custom recipe names to be run following a
* configure
event.
*/
public void setConfigure(java.util.Collection configure) {
if (configure == null) {
this.configure = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag configureCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(configure.size());
configureCopy.addAll(configure);
this.configure = configureCopy;
}
/**
* An array of custom recipe names to be run following a
* configure
event.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param configure An array of custom recipe names to be run following a
* configure
event.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Recipes withConfigure(String... configure) {
if (getConfigure() == null) setConfigure(new java.util.ArrayList(configure.length));
for (String value : configure) {
getConfigure().add(value);
}
return this;
}
/**
* An array of custom recipe names to be run following a
* configure
event.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param configure An array of custom recipe names to be run following a
* configure
event.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Recipes withConfigure(java.util.Collection configure) {
if (configure == null) {
this.configure = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag configureCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(configure.size());
configureCopy.addAll(configure);
this.configure = configureCopy;
}
return this;
}
/**
* An array of custom recipe names to be run following a
* deploy
event.
*
* @return An array of custom recipe names to be run following a
* deploy
event.
*/
public java.util.List getDeploy() {
if (deploy == null) {
deploy = new com.amazonaws.internal.ListWithAutoConstructFlag();
deploy.setAutoConstruct(true);
}
return deploy;
}
/**
* An array of custom recipe names to be run following a
* deploy
event.
*
* @param deploy An array of custom recipe names to be run following a
* deploy
event.
*/
public void setDeploy(java.util.Collection deploy) {
if (deploy == null) {
this.deploy = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag deployCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(deploy.size());
deployCopy.addAll(deploy);
this.deploy = deployCopy;
}
/**
* An array of custom recipe names to be run following a
* deploy
event.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param deploy An array of custom recipe names to be run following a
* deploy
event.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Recipes withDeploy(String... deploy) {
if (getDeploy() == null) setDeploy(new java.util.ArrayList(deploy.length));
for (String value : deploy) {
getDeploy().add(value);
}
return this;
}
/**
* An array of custom recipe names to be run following a
* deploy
event.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param deploy An array of custom recipe names to be run following a
* deploy
event.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Recipes withDeploy(java.util.Collection deploy) {
if (deploy == null) {
this.deploy = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag deployCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(deploy.size());
deployCopy.addAll(deploy);
this.deploy = deployCopy;
}
return this;
}
/**
* An array of custom recipe names to be run following a
* undeploy
event.
*
* @return An array of custom recipe names to be run following a
* undeploy
event.
*/
public java.util.List getUndeploy() {
if (undeploy == null) {
undeploy = new com.amazonaws.internal.ListWithAutoConstructFlag();
undeploy.setAutoConstruct(true);
}
return undeploy;
}
/**
* An array of custom recipe names to be run following a
* undeploy
event.
*
* @param undeploy An array of custom recipe names to be run following a
* undeploy
event.
*/
public void setUndeploy(java.util.Collection undeploy) {
if (undeploy == null) {
this.undeploy = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag undeployCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(undeploy.size());
undeployCopy.addAll(undeploy);
this.undeploy = undeployCopy;
}
/**
* An array of custom recipe names to be run following a
* undeploy
event.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param undeploy An array of custom recipe names to be run following a
* undeploy
event.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Recipes withUndeploy(String... undeploy) {
if (getUndeploy() == null) setUndeploy(new java.util.ArrayList(undeploy.length));
for (String value : undeploy) {
getUndeploy().add(value);
}
return this;
}
/**
* An array of custom recipe names to be run following a
* undeploy
event.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param undeploy An array of custom recipe names to be run following a
* undeploy
event.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Recipes withUndeploy(java.util.Collection undeploy) {
if (undeploy == null) {
this.undeploy = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag undeployCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(undeploy.size());
undeployCopy.addAll(undeploy);
this.undeploy = undeployCopy;
}
return this;
}
/**
* An array of custom recipe names to be run following a
* shutdown
event.
*
* @return An array of custom recipe names to be run following a
* shutdown
event.
*/
public java.util.List getShutdown() {
if (shutdown == null) {
shutdown = new com.amazonaws.internal.ListWithAutoConstructFlag();
shutdown.setAutoConstruct(true);
}
return shutdown;
}
/**
* An array of custom recipe names to be run following a
* shutdown
event.
*
* @param shutdown An array of custom recipe names to be run following a
* shutdown
event.
*/
public void setShutdown(java.util.Collection shutdown) {
if (shutdown == null) {
this.shutdown = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag shutdownCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(shutdown.size());
shutdownCopy.addAll(shutdown);
this.shutdown = shutdownCopy;
}
/**
* An array of custom recipe names to be run following a
* shutdown
event.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param shutdown An array of custom recipe names to be run following a
* shutdown
event.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Recipes withShutdown(String... shutdown) {
if (getShutdown() == null) setShutdown(new java.util.ArrayList(shutdown.length));
for (String value : shutdown) {
getShutdown().add(value);
}
return this;
}
/**
* An array of custom recipe names to be run following a
* shutdown
event.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param shutdown An array of custom recipe names to be run following a
* shutdown
event.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public Recipes withShutdown(java.util.Collection shutdown) {
if (shutdown == null) {
this.shutdown = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag shutdownCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(shutdown.size());
shutdownCopy.addAll(shutdown);
this.shutdown = shutdownCopy;
}
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getSetup() != null) sb.append("Setup: " + getSetup() + ",");
if (getConfigure() != null) sb.append("Configure: " + getConfigure() + ",");
if (getDeploy() != null) sb.append("Deploy: " + getDeploy() + ",");
if (getUndeploy() != null) sb.append("Undeploy: " + getUndeploy() + ",");
if (getShutdown() != null) sb.append("Shutdown: " + getShutdown() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getSetup() == null) ? 0 : getSetup().hashCode());
hashCode = prime * hashCode + ((getConfigure() == null) ? 0 : getConfigure().hashCode());
hashCode = prime * hashCode + ((getDeploy() == null) ? 0 : getDeploy().hashCode());
hashCode = prime * hashCode + ((getUndeploy() == null) ? 0 : getUndeploy().hashCode());
hashCode = prime * hashCode + ((getShutdown() == null) ? 0 : getShutdown().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof Recipes == false) return false;
Recipes other = (Recipes)obj;
if (other.getSetup() == null ^ this.getSetup() == null) return false;
if (other.getSetup() != null && other.getSetup().equals(this.getSetup()) == false) return false;
if (other.getConfigure() == null ^ this.getConfigure() == null) return false;
if (other.getConfigure() != null && other.getConfigure().equals(this.getConfigure()) == false) return false;
if (other.getDeploy() == null ^ this.getDeploy() == null) return false;
if (other.getDeploy() != null && other.getDeploy().equals(this.getDeploy()) == false) return false;
if (other.getUndeploy() == null ^ this.getUndeploy() == null) return false;
if (other.getUndeploy() != null && other.getUndeploy().equals(this.getUndeploy()) == false) return false;
if (other.getShutdown() == null ^ this.getShutdown() == null) return false;
if (other.getShutdown() != null && other.getShutdown().equals(this.getShutdown()) == false) return false;
return true;
}
}