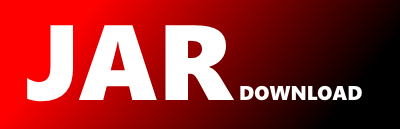
com.amazonaws.services.rds.model.PurchaseReservedDBInstancesOfferingRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.rds.model;
import java.io.Serializable;
import com.amazonaws.AmazonWebServiceRequest;
/**
* Container for the parameters to the {@link com.amazonaws.services.rds.AmazonRDS#purchaseReservedDBInstancesOffering(PurchaseReservedDBInstancesOfferingRequest) PurchaseReservedDBInstancesOffering operation}.
*
* Purchases a reserved DB instance offering.
*
*
* @see com.amazonaws.services.rds.AmazonRDS#purchaseReservedDBInstancesOffering(PurchaseReservedDBInstancesOfferingRequest)
*/
public class PurchaseReservedDBInstancesOfferingRequest extends AmazonWebServiceRequest implements Serializable {
/**
* The ID of the Reserved DB instance offering to purchase. Example:
* 438012d3-4052-4cc7-b2e3-8d3372e0e706
*/
private String reservedDBInstancesOfferingId;
/**
* Customer-specified identifier to track this reservation.
Example:
* myreservationID
*/
private String reservedDBInstanceId;
/**
* The number of instances to reserve.
Default: 1
*/
private Integer dBInstanceCount;
/**
* A list of tags.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag tags;
/**
* Default constructor for a new PurchaseReservedDBInstancesOfferingRequest object. Callers should use the
* setter or fluent setter (with...) methods to initialize this object after creating it.
*/
public PurchaseReservedDBInstancesOfferingRequest() {}
/**
* Constructs a new PurchaseReservedDBInstancesOfferingRequest object.
* Callers should use the setter or fluent setter (with...) methods to
* initialize any additional object members.
*
* @param reservedDBInstancesOfferingId The ID of the Reserved DB
* instance offering to purchase. Example:
* 438012d3-4052-4cc7-b2e3-8d3372e0e706
*/
public PurchaseReservedDBInstancesOfferingRequest(String reservedDBInstancesOfferingId) {
setReservedDBInstancesOfferingId(reservedDBInstancesOfferingId);
}
/**
* The ID of the Reserved DB instance offering to purchase.
Example:
* 438012d3-4052-4cc7-b2e3-8d3372e0e706
*
* @return The ID of the Reserved DB instance offering to purchase.
Example:
* 438012d3-4052-4cc7-b2e3-8d3372e0e706
*/
public String getReservedDBInstancesOfferingId() {
return reservedDBInstancesOfferingId;
}
/**
* The ID of the Reserved DB instance offering to purchase.
Example:
* 438012d3-4052-4cc7-b2e3-8d3372e0e706
*
* @param reservedDBInstancesOfferingId The ID of the Reserved DB instance offering to purchase.
Example:
* 438012d3-4052-4cc7-b2e3-8d3372e0e706
*/
public void setReservedDBInstancesOfferingId(String reservedDBInstancesOfferingId) {
this.reservedDBInstancesOfferingId = reservedDBInstancesOfferingId;
}
/**
* The ID of the Reserved DB instance offering to purchase.
Example:
* 438012d3-4052-4cc7-b2e3-8d3372e0e706
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param reservedDBInstancesOfferingId The ID of the Reserved DB instance offering to purchase.
Example:
* 438012d3-4052-4cc7-b2e3-8d3372e0e706
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public PurchaseReservedDBInstancesOfferingRequest withReservedDBInstancesOfferingId(String reservedDBInstancesOfferingId) {
this.reservedDBInstancesOfferingId = reservedDBInstancesOfferingId;
return this;
}
/**
* Customer-specified identifier to track this reservation.
Example:
* myreservationID
*
* @return Customer-specified identifier to track this reservation.
Example:
* myreservationID
*/
public String getReservedDBInstanceId() {
return reservedDBInstanceId;
}
/**
* Customer-specified identifier to track this reservation.
Example:
* myreservationID
*
* @param reservedDBInstanceId Customer-specified identifier to track this reservation.
Example:
* myreservationID
*/
public void setReservedDBInstanceId(String reservedDBInstanceId) {
this.reservedDBInstanceId = reservedDBInstanceId;
}
/**
* Customer-specified identifier to track this reservation.
Example:
* myreservationID
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param reservedDBInstanceId Customer-specified identifier to track this reservation.
Example:
* myreservationID
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public PurchaseReservedDBInstancesOfferingRequest withReservedDBInstanceId(String reservedDBInstanceId) {
this.reservedDBInstanceId = reservedDBInstanceId;
return this;
}
/**
* The number of instances to reserve.
Default: 1
*
* @return The number of instances to reserve.
Default: 1
*/
public Integer getDBInstanceCount() {
return dBInstanceCount;
}
/**
* The number of instances to reserve.
Default: 1
*
* @param dBInstanceCount The number of instances to reserve.
Default: 1
*/
public void setDBInstanceCount(Integer dBInstanceCount) {
this.dBInstanceCount = dBInstanceCount;
}
/**
* The number of instances to reserve.
Default: 1
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param dBInstanceCount The number of instances to reserve.
Default: 1
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public PurchaseReservedDBInstancesOfferingRequest withDBInstanceCount(Integer dBInstanceCount) {
this.dBInstanceCount = dBInstanceCount;
return this;
}
/**
* A list of tags.
*
* @return A list of tags.
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.ListWithAutoConstructFlag();
tags.setAutoConstruct(true);
}
return tags;
}
/**
* A list of tags.
*
* @param tags A list of tags.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag tagsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(tags.size());
tagsCopy.addAll(tags);
this.tags = tagsCopy;
}
/**
* A list of tags.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param tags A list of tags.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public PurchaseReservedDBInstancesOfferingRequest withTags(Tag... tags) {
if (getTags() == null) setTags(new java.util.ArrayList(tags.length));
for (Tag value : tags) {
getTags().add(value);
}
return this;
}
/**
* A list of tags.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param tags A list of tags.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public PurchaseReservedDBInstancesOfferingRequest withTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag tagsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(tags.size());
tagsCopy.addAll(tags);
this.tags = tagsCopy;
}
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getReservedDBInstancesOfferingId() != null) sb.append("ReservedDBInstancesOfferingId: " + getReservedDBInstancesOfferingId() + ",");
if (getReservedDBInstanceId() != null) sb.append("ReservedDBInstanceId: " + getReservedDBInstanceId() + ",");
if (getDBInstanceCount() != null) sb.append("DBInstanceCount: " + getDBInstanceCount() + ",");
if (getTags() != null) sb.append("Tags: " + getTags() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getReservedDBInstancesOfferingId() == null) ? 0 : getReservedDBInstancesOfferingId().hashCode());
hashCode = prime * hashCode + ((getReservedDBInstanceId() == null) ? 0 : getReservedDBInstanceId().hashCode());
hashCode = prime * hashCode + ((getDBInstanceCount() == null) ? 0 : getDBInstanceCount().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof PurchaseReservedDBInstancesOfferingRequest == false) return false;
PurchaseReservedDBInstancesOfferingRequest other = (PurchaseReservedDBInstancesOfferingRequest)obj;
if (other.getReservedDBInstancesOfferingId() == null ^ this.getReservedDBInstancesOfferingId() == null) return false;
if (other.getReservedDBInstancesOfferingId() != null && other.getReservedDBInstancesOfferingId().equals(this.getReservedDBInstancesOfferingId()) == false) return false;
if (other.getReservedDBInstanceId() == null ^ this.getReservedDBInstanceId() == null) return false;
if (other.getReservedDBInstanceId() != null && other.getReservedDBInstanceId().equals(this.getReservedDBInstanceId()) == false) return false;
if (other.getDBInstanceCount() == null ^ this.getDBInstanceCount() == null) return false;
if (other.getDBInstanceCount() != null && other.getDBInstanceCount().equals(this.getDBInstanceCount()) == false) return false;
if (other.getTags() == null ^ this.getTags() == null) return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false) return false;
return true;
}
}