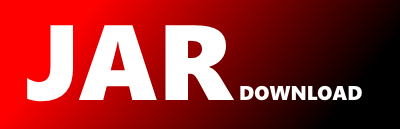
com.amazonaws.services.rds.model.RestoreDBInstanceFromDBSnapshotRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.rds.model;
import java.io.Serializable;
import com.amazonaws.AmazonWebServiceRequest;
/**
* Container for the parameters to the {@link com.amazonaws.services.rds.AmazonRDS#restoreDBInstanceFromDBSnapshot(RestoreDBInstanceFromDBSnapshotRequest) RestoreDBInstanceFromDBSnapshot operation}.
*
* Creates a new DB instance from a DB snapshot. The target database is
* created from the source database restore point with the same
* configuration as the original source database, except that the new RDS
* instance is created with the default security group.
*
*
* @see com.amazonaws.services.rds.AmazonRDS#restoreDBInstanceFromDBSnapshot(RestoreDBInstanceFromDBSnapshotRequest)
*/
public class RestoreDBInstanceFromDBSnapshotRequest extends AmazonWebServiceRequest implements Serializable {
/**
* The identifier for the DB snapshot to restore from. Constraints:
*
- Must contain from 1 to 63 alphanumeric characters or
* hyphens
- First character must be a letter
- Cannot end
* with a hyphen or contain two consecutive hyphens
*/
private String dBInstanceIdentifier;
/**
* Name of the DB instance to create from the DB snapshot. This parameter
* isn't case sensitive. Constraints:
- Must contain from 1 to
* 255 alphanumeric characters or hyphens
- First character must
* be a letter
- Cannot end with a hyphen or contain two
* consecutive hyphens
Example: my-snapshot-id
*/
private String dBSnapshotIdentifier;
/**
* The compute and memory capacity of the Amazon RDS DB instance.
*
Valid Values: db.t1.micro | db.m1.small | db.m1.medium |
* db.m1.large | db.m1.xlarge | db.m2.2xlarge | db.m2.4xlarge
*/
private String dBInstanceClass;
/**
* The port number on which the database accepts connections.
Default:
* The same port as the original DB instance
Constraints: Value must
* be 1150-65535
*/
private Integer port;
/**
* The EC2 Availability Zone that the database instance will be created
* in.
Default: A random, system-chosen Availability Zone.
*
Constraint: You cannot specify the AvailabilityZone parameter if
* the MultiAZ parameter is set to true
.
Example:
* us-east-1a
*/
private String availabilityZone;
/**
* The DB subnet group name to use for the new instance.
*/
private String dBSubnetGroupName;
/**
* Specifies if the DB instance is a Multi-AZ deployment.
Constraint:
* You cannot specify the AvailabilityZone parameter if the MultiAZ
* parameter is set to true
.
*/
private Boolean multiAZ;
/**
* Specifies the accessibility options for the DB instance. A value of
* true specifies an Internet-facing instance with a publicly resolvable
* DNS name, which resolves to a public IP address. A value of false
* specifies an internal instance with a DNS name that resolves to a
* private IP address.
Default: The default behavior varies depending
* on whether a VPC has been requested or not. The following list shows
* the default behavior in each case.
- Default
* VPC:true
- VPC:false
If no DB subnet
* group has been specified as part of the request and the
* PubliclyAccessible value has not been set, the DB instance will be
* publicly accessible. If a specific DB subnet group has been specified
* as part of the request and the PubliclyAccessible value has not been
* set, the DB instance will be private.
*/
private Boolean publiclyAccessible;
/**
* Indicates that minor version upgrades will be applied automatically to
* the DB instance during the maintenance window.
*/
private Boolean autoMinorVersionUpgrade;
/**
* License model information for the restored DB instance.
Default:
* Same as source.
Valid values: license-included
|
* bring-your-own-license
|
* general-public-license
*/
private String licenseModel;
/**
* The database name for the restored DB instance. This
* parameter doesn't apply to the MySQL engine.
*/
private String dBName;
/**
* The database engine to use for the new instance.
Default: The same
* as source
Constraint: Must be compatible with the engine of the
* source
Example: oracle-ee
*/
private String engine;
/**
* Specifies the amount of provisioned IOPS for the DB instance,
* expressed in I/O operations per second. If this parameter is not
* specified, the IOPS value will be taken from the backup. If this
* parameter is set to 0, the new instance will be converted to a
* non-PIOPS instance, which will take additional time, though your DB
* instance will be available for connections before the conversion
* starts.
Constraints: Must be an integer greater than 1000.
*/
private Integer iops;
/**
* The name of the option group to be used for the restored DB instance.
*
Permanent options, such as the TDE option for Oracle Advanced
* Security TDE, cannot be removed from an option group, and that option
* group cannot be removed from a DB instance once it is associated with
* a DB instance
*/
private String optionGroupName;
/**
* A list of tags.
*/
private com.amazonaws.internal.ListWithAutoConstructFlag tags;
/**
* Default constructor for a new RestoreDBInstanceFromDBSnapshotRequest object. Callers should use the
* setter or fluent setter (with...) methods to initialize this object after creating it.
*/
public RestoreDBInstanceFromDBSnapshotRequest() {}
/**
* Constructs a new RestoreDBInstanceFromDBSnapshotRequest object.
* Callers should use the setter or fluent setter (with...) methods to
* initialize any additional object members.
*
* @param dBInstanceIdentifier The identifier for the DB snapshot to
* restore from. Constraints:
- Must contain from 1 to 63
* alphanumeric characters or hyphens
- First character must be a
* letter
- Cannot end with a hyphen or contain two consecutive
* hyphens
* @param dBSnapshotIdentifier Name of the DB instance to create from the
* DB snapshot. This parameter isn't case sensitive. Constraints:
* - Must contain from 1 to 255 alphanumeric characters or hyphens
* - First character must be a letter
- Cannot end with a hyphen
* or contain two consecutive hyphens
Example:
* my-snapshot-id
*/
public RestoreDBInstanceFromDBSnapshotRequest(String dBInstanceIdentifier, String dBSnapshotIdentifier) {
setDBInstanceIdentifier(dBInstanceIdentifier);
setDBSnapshotIdentifier(dBSnapshotIdentifier);
}
/**
* The identifier for the DB snapshot to restore from.
Constraints:
*
- Must contain from 1 to 63 alphanumeric characters or
* hyphens
- First character must be a letter
- Cannot end
* with a hyphen or contain two consecutive hyphens
*
* @return The identifier for the DB snapshot to restore from. Constraints:
*
- Must contain from 1 to 63 alphanumeric characters or
* hyphens
- First character must be a letter
- Cannot end
* with a hyphen or contain two consecutive hyphens
*/
public String getDBInstanceIdentifier() {
return dBInstanceIdentifier;
}
/**
* The identifier for the DB snapshot to restore from. Constraints:
*
- Must contain from 1 to 63 alphanumeric characters or
* hyphens
- First character must be a letter
- Cannot end
* with a hyphen or contain two consecutive hyphens
*
* @param dBInstanceIdentifier The identifier for the DB snapshot to restore from. Constraints:
*
- Must contain from 1 to 63 alphanumeric characters or
* hyphens
- First character must be a letter
- Cannot end
* with a hyphen or contain two consecutive hyphens
*/
public void setDBInstanceIdentifier(String dBInstanceIdentifier) {
this.dBInstanceIdentifier = dBInstanceIdentifier;
}
/**
* The identifier for the DB snapshot to restore from. Constraints:
*
- Must contain from 1 to 63 alphanumeric characters or
* hyphens
- First character must be a letter
- Cannot end
* with a hyphen or contain two consecutive hyphens
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param dBInstanceIdentifier The identifier for the DB snapshot to restore from.
Constraints:
*
- Must contain from 1 to 63 alphanumeric characters or
* hyphens
- First character must be a letter
- Cannot end
* with a hyphen or contain two consecutive hyphens
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RestoreDBInstanceFromDBSnapshotRequest withDBInstanceIdentifier(String dBInstanceIdentifier) {
this.dBInstanceIdentifier = dBInstanceIdentifier;
return this;
}
/**
* Name of the DB instance to create from the DB snapshot. This parameter
* isn't case sensitive. Constraints:
- Must contain from 1 to
* 255 alphanumeric characters or hyphens
- First character must
* be a letter
- Cannot end with a hyphen or contain two
* consecutive hyphens
Example: my-snapshot-id
*
* @return Name of the DB instance to create from the DB snapshot. This parameter
* isn't case sensitive.
Constraints:
- Must contain from 1 to
* 255 alphanumeric characters or hyphens
- First character must
* be a letter
- Cannot end with a hyphen or contain two
* consecutive hyphens
Example: my-snapshot-id
*/
public String getDBSnapshotIdentifier() {
return dBSnapshotIdentifier;
}
/**
* Name of the DB instance to create from the DB snapshot. This parameter
* isn't case sensitive.
Constraints:
- Must contain from 1 to
* 255 alphanumeric characters or hyphens
- First character must
* be a letter
- Cannot end with a hyphen or contain two
* consecutive hyphens
Example: my-snapshot-id
*
* @param dBSnapshotIdentifier Name of the DB instance to create from the DB snapshot. This parameter
* isn't case sensitive.
Constraints:
- Must contain from 1 to
* 255 alphanumeric characters or hyphens
- First character must
* be a letter
- Cannot end with a hyphen or contain two
* consecutive hyphens
Example: my-snapshot-id
*/
public void setDBSnapshotIdentifier(String dBSnapshotIdentifier) {
this.dBSnapshotIdentifier = dBSnapshotIdentifier;
}
/**
* Name of the DB instance to create from the DB snapshot. This parameter
* isn't case sensitive.
Constraints:
- Must contain from 1 to
* 255 alphanumeric characters or hyphens
- First character must
* be a letter
- Cannot end with a hyphen or contain two
* consecutive hyphens
Example: my-snapshot-id
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param dBSnapshotIdentifier Name of the DB instance to create from the DB snapshot. This parameter
* isn't case sensitive.
Constraints:
- Must contain from 1 to
* 255 alphanumeric characters or hyphens
- First character must
* be a letter
- Cannot end with a hyphen or contain two
* consecutive hyphens
Example: my-snapshot-id
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RestoreDBInstanceFromDBSnapshotRequest withDBSnapshotIdentifier(String dBSnapshotIdentifier) {
this.dBSnapshotIdentifier = dBSnapshotIdentifier;
return this;
}
/**
* The compute and memory capacity of the Amazon RDS DB instance.
*
Valid Values: db.t1.micro | db.m1.small | db.m1.medium |
* db.m1.large | db.m1.xlarge | db.m2.2xlarge | db.m2.4xlarge
*
* @return The compute and memory capacity of the Amazon RDS DB instance.
*
Valid Values: db.t1.micro | db.m1.small | db.m1.medium |
* db.m1.large | db.m1.xlarge | db.m2.2xlarge | db.m2.4xlarge
*/
public String getDBInstanceClass() {
return dBInstanceClass;
}
/**
* The compute and memory capacity of the Amazon RDS DB instance.
*
Valid Values: db.t1.micro | db.m1.small | db.m1.medium |
* db.m1.large | db.m1.xlarge | db.m2.2xlarge | db.m2.4xlarge
*
* @param dBInstanceClass The compute and memory capacity of the Amazon RDS DB instance.
*
Valid Values: db.t1.micro | db.m1.small | db.m1.medium |
* db.m1.large | db.m1.xlarge | db.m2.2xlarge | db.m2.4xlarge
*/
public void setDBInstanceClass(String dBInstanceClass) {
this.dBInstanceClass = dBInstanceClass;
}
/**
* The compute and memory capacity of the Amazon RDS DB instance.
*
Valid Values: db.t1.micro | db.m1.small | db.m1.medium |
* db.m1.large | db.m1.xlarge | db.m2.2xlarge | db.m2.4xlarge
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param dBInstanceClass The compute and memory capacity of the Amazon RDS DB instance.
*
Valid Values: db.t1.micro | db.m1.small | db.m1.medium |
* db.m1.large | db.m1.xlarge | db.m2.2xlarge | db.m2.4xlarge
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RestoreDBInstanceFromDBSnapshotRequest withDBInstanceClass(String dBInstanceClass) {
this.dBInstanceClass = dBInstanceClass;
return this;
}
/**
* The port number on which the database accepts connections.
Default:
* The same port as the original DB instance
Constraints: Value must
* be 1150-65535
*
* @return The port number on which the database accepts connections.
Default:
* The same port as the original DB instance
Constraints: Value must
* be 1150-65535
*/
public Integer getPort() {
return port;
}
/**
* The port number on which the database accepts connections.
Default:
* The same port as the original DB instance
Constraints: Value must
* be 1150-65535
*
* @param port The port number on which the database accepts connections.
Default:
* The same port as the original DB instance
Constraints: Value must
* be 1150-65535
*/
public void setPort(Integer port) {
this.port = port;
}
/**
* The port number on which the database accepts connections.
Default:
* The same port as the original DB instance
Constraints: Value must
* be 1150-65535
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param port The port number on which the database accepts connections.
Default:
* The same port as the original DB instance
Constraints: Value must
* be 1150-65535
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RestoreDBInstanceFromDBSnapshotRequest withPort(Integer port) {
this.port = port;
return this;
}
/**
* The EC2 Availability Zone that the database instance will be created
* in.
Default: A random, system-chosen Availability Zone.
*
Constraint: You cannot specify the AvailabilityZone parameter if
* the MultiAZ parameter is set to true
.
Example:
* us-east-1a
*
* @return The EC2 Availability Zone that the database instance will be created
* in.
Default: A random, system-chosen Availability Zone.
*
Constraint: You cannot specify the AvailabilityZone parameter if
* the MultiAZ parameter is set to true
.
Example:
* us-east-1a
*/
public String getAvailabilityZone() {
return availabilityZone;
}
/**
* The EC2 Availability Zone that the database instance will be created
* in.
Default: A random, system-chosen Availability Zone.
*
Constraint: You cannot specify the AvailabilityZone parameter if
* the MultiAZ parameter is set to true
.
Example:
* us-east-1a
*
* @param availabilityZone The EC2 Availability Zone that the database instance will be created
* in.
Default: A random, system-chosen Availability Zone.
*
Constraint: You cannot specify the AvailabilityZone parameter if
* the MultiAZ parameter is set to true
.
Example:
* us-east-1a
*/
public void setAvailabilityZone(String availabilityZone) {
this.availabilityZone = availabilityZone;
}
/**
* The EC2 Availability Zone that the database instance will be created
* in.
Default: A random, system-chosen Availability Zone.
*
Constraint: You cannot specify the AvailabilityZone parameter if
* the MultiAZ parameter is set to true
.
Example:
* us-east-1a
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param availabilityZone The EC2 Availability Zone that the database instance will be created
* in.
Default: A random, system-chosen Availability Zone.
*
Constraint: You cannot specify the AvailabilityZone parameter if
* the MultiAZ parameter is set to true
.
Example:
* us-east-1a
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RestoreDBInstanceFromDBSnapshotRequest withAvailabilityZone(String availabilityZone) {
this.availabilityZone = availabilityZone;
return this;
}
/**
* The DB subnet group name to use for the new instance.
*
* @return The DB subnet group name to use for the new instance.
*/
public String getDBSubnetGroupName() {
return dBSubnetGroupName;
}
/**
* The DB subnet group name to use for the new instance.
*
* @param dBSubnetGroupName The DB subnet group name to use for the new instance.
*/
public void setDBSubnetGroupName(String dBSubnetGroupName) {
this.dBSubnetGroupName = dBSubnetGroupName;
}
/**
* The DB subnet group name to use for the new instance.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param dBSubnetGroupName The DB subnet group name to use for the new instance.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RestoreDBInstanceFromDBSnapshotRequest withDBSubnetGroupName(String dBSubnetGroupName) {
this.dBSubnetGroupName = dBSubnetGroupName;
return this;
}
/**
* Specifies if the DB instance is a Multi-AZ deployment.
Constraint:
* You cannot specify the AvailabilityZone parameter if the MultiAZ
* parameter is set to true
.
*
* @return Specifies if the DB instance is a Multi-AZ deployment.
Constraint:
* You cannot specify the AvailabilityZone parameter if the MultiAZ
* parameter is set to true
.
*/
public Boolean isMultiAZ() {
return multiAZ;
}
/**
* Specifies if the DB instance is a Multi-AZ deployment.
Constraint:
* You cannot specify the AvailabilityZone parameter if the MultiAZ
* parameter is set to true
.
*
* @param multiAZ Specifies if the DB instance is a Multi-AZ deployment.
Constraint:
* You cannot specify the AvailabilityZone parameter if the MultiAZ
* parameter is set to true
.
*/
public void setMultiAZ(Boolean multiAZ) {
this.multiAZ = multiAZ;
}
/**
* Specifies if the DB instance is a Multi-AZ deployment.
Constraint:
* You cannot specify the AvailabilityZone parameter if the MultiAZ
* parameter is set to true
.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param multiAZ Specifies if the DB instance is a Multi-AZ deployment.
Constraint:
* You cannot specify the AvailabilityZone parameter if the MultiAZ
* parameter is set to true
.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RestoreDBInstanceFromDBSnapshotRequest withMultiAZ(Boolean multiAZ) {
this.multiAZ = multiAZ;
return this;
}
/**
* Specifies if the DB instance is a Multi-AZ deployment.
Constraint:
* You cannot specify the AvailabilityZone parameter if the MultiAZ
* parameter is set to true
.
*
* @return Specifies if the DB instance is a Multi-AZ deployment.
Constraint:
* You cannot specify the AvailabilityZone parameter if the MultiAZ
* parameter is set to true
.
*/
public Boolean getMultiAZ() {
return multiAZ;
}
/**
* Specifies the accessibility options for the DB instance. A value of
* true specifies an Internet-facing instance with a publicly resolvable
* DNS name, which resolves to a public IP address. A value of false
* specifies an internal instance with a DNS name that resolves to a
* private IP address.
Default: The default behavior varies depending
* on whether a VPC has been requested or not. The following list shows
* the default behavior in each case.
- Default
* VPC:true
- VPC:false
If no DB subnet
* group has been specified as part of the request and the
* PubliclyAccessible value has not been set, the DB instance will be
* publicly accessible. If a specific DB subnet group has been specified
* as part of the request and the PubliclyAccessible value has not been
* set, the DB instance will be private.
*
* @return Specifies the accessibility options for the DB instance. A value of
* true specifies an Internet-facing instance with a publicly resolvable
* DNS name, which resolves to a public IP address. A value of false
* specifies an internal instance with a DNS name that resolves to a
* private IP address.
Default: The default behavior varies depending
* on whether a VPC has been requested or not. The following list shows
* the default behavior in each case.
- Default
* VPC:true
- VPC:false
If no DB subnet
* group has been specified as part of the request and the
* PubliclyAccessible value has not been set, the DB instance will be
* publicly accessible. If a specific DB subnet group has been specified
* as part of the request and the PubliclyAccessible value has not been
* set, the DB instance will be private.
*/
public Boolean isPubliclyAccessible() {
return publiclyAccessible;
}
/**
* Specifies the accessibility options for the DB instance. A value of
* true specifies an Internet-facing instance with a publicly resolvable
* DNS name, which resolves to a public IP address. A value of false
* specifies an internal instance with a DNS name that resolves to a
* private IP address.
Default: The default behavior varies depending
* on whether a VPC has been requested or not. The following list shows
* the default behavior in each case.
- Default
* VPC:true
- VPC:false
If no DB subnet
* group has been specified as part of the request and the
* PubliclyAccessible value has not been set, the DB instance will be
* publicly accessible. If a specific DB subnet group has been specified
* as part of the request and the PubliclyAccessible value has not been
* set, the DB instance will be private.
*
* @param publiclyAccessible Specifies the accessibility options for the DB instance. A value of
* true specifies an Internet-facing instance with a publicly resolvable
* DNS name, which resolves to a public IP address. A value of false
* specifies an internal instance with a DNS name that resolves to a
* private IP address.
Default: The default behavior varies depending
* on whether a VPC has been requested or not. The following list shows
* the default behavior in each case.
- Default
* VPC:true
- VPC:false
If no DB subnet
* group has been specified as part of the request and the
* PubliclyAccessible value has not been set, the DB instance will be
* publicly accessible. If a specific DB subnet group has been specified
* as part of the request and the PubliclyAccessible value has not been
* set, the DB instance will be private.
*/
public void setPubliclyAccessible(Boolean publiclyAccessible) {
this.publiclyAccessible = publiclyAccessible;
}
/**
* Specifies the accessibility options for the DB instance. A value of
* true specifies an Internet-facing instance with a publicly resolvable
* DNS name, which resolves to a public IP address. A value of false
* specifies an internal instance with a DNS name that resolves to a
* private IP address.
Default: The default behavior varies depending
* on whether a VPC has been requested or not. The following list shows
* the default behavior in each case.
- Default
* VPC:true
- VPC:false
If no DB subnet
* group has been specified as part of the request and the
* PubliclyAccessible value has not been set, the DB instance will be
* publicly accessible. If a specific DB subnet group has been specified
* as part of the request and the PubliclyAccessible value has not been
* set, the DB instance will be private.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param publiclyAccessible Specifies the accessibility options for the DB instance. A value of
* true specifies an Internet-facing instance with a publicly resolvable
* DNS name, which resolves to a public IP address. A value of false
* specifies an internal instance with a DNS name that resolves to a
* private IP address.
Default: The default behavior varies depending
* on whether a VPC has been requested or not. The following list shows
* the default behavior in each case.
- Default
* VPC:true
- VPC:false
If no DB subnet
* group has been specified as part of the request and the
* PubliclyAccessible value has not been set, the DB instance will be
* publicly accessible. If a specific DB subnet group has been specified
* as part of the request and the PubliclyAccessible value has not been
* set, the DB instance will be private.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RestoreDBInstanceFromDBSnapshotRequest withPubliclyAccessible(Boolean publiclyAccessible) {
this.publiclyAccessible = publiclyAccessible;
return this;
}
/**
* Specifies the accessibility options for the DB instance. A value of
* true specifies an Internet-facing instance with a publicly resolvable
* DNS name, which resolves to a public IP address. A value of false
* specifies an internal instance with a DNS name that resolves to a
* private IP address.
Default: The default behavior varies depending
* on whether a VPC has been requested or not. The following list shows
* the default behavior in each case.
- Default
* VPC:true
- VPC:false
If no DB subnet
* group has been specified as part of the request and the
* PubliclyAccessible value has not been set, the DB instance will be
* publicly accessible. If a specific DB subnet group has been specified
* as part of the request and the PubliclyAccessible value has not been
* set, the DB instance will be private.
*
* @return Specifies the accessibility options for the DB instance. A value of
* true specifies an Internet-facing instance with a publicly resolvable
* DNS name, which resolves to a public IP address. A value of false
* specifies an internal instance with a DNS name that resolves to a
* private IP address.
Default: The default behavior varies depending
* on whether a VPC has been requested or not. The following list shows
* the default behavior in each case.
- Default
* VPC:true
- VPC:false
If no DB subnet
* group has been specified as part of the request and the
* PubliclyAccessible value has not been set, the DB instance will be
* publicly accessible. If a specific DB subnet group has been specified
* as part of the request and the PubliclyAccessible value has not been
* set, the DB instance will be private.
*/
public Boolean getPubliclyAccessible() {
return publiclyAccessible;
}
/**
* Indicates that minor version upgrades will be applied automatically to
* the DB instance during the maintenance window.
*
* @return Indicates that minor version upgrades will be applied automatically to
* the DB instance during the maintenance window.
*/
public Boolean isAutoMinorVersionUpgrade() {
return autoMinorVersionUpgrade;
}
/**
* Indicates that minor version upgrades will be applied automatically to
* the DB instance during the maintenance window.
*
* @param autoMinorVersionUpgrade Indicates that minor version upgrades will be applied automatically to
* the DB instance during the maintenance window.
*/
public void setAutoMinorVersionUpgrade(Boolean autoMinorVersionUpgrade) {
this.autoMinorVersionUpgrade = autoMinorVersionUpgrade;
}
/**
* Indicates that minor version upgrades will be applied automatically to
* the DB instance during the maintenance window.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param autoMinorVersionUpgrade Indicates that minor version upgrades will be applied automatically to
* the DB instance during the maintenance window.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RestoreDBInstanceFromDBSnapshotRequest withAutoMinorVersionUpgrade(Boolean autoMinorVersionUpgrade) {
this.autoMinorVersionUpgrade = autoMinorVersionUpgrade;
return this;
}
/**
* Indicates that minor version upgrades will be applied automatically to
* the DB instance during the maintenance window.
*
* @return Indicates that minor version upgrades will be applied automatically to
* the DB instance during the maintenance window.
*/
public Boolean getAutoMinorVersionUpgrade() {
return autoMinorVersionUpgrade;
}
/**
* License model information for the restored DB instance.
Default:
* Same as source.
Valid values: license-included
|
* bring-your-own-license
|
* general-public-license
*
* @return License model information for the restored DB instance.
Default:
* Same as source.
Valid values: license-included
|
* bring-your-own-license
|
* general-public-license
*/
public String getLicenseModel() {
return licenseModel;
}
/**
* License model information for the restored DB instance.
Default:
* Same as source.
Valid values: license-included
|
* bring-your-own-license
|
* general-public-license
*
* @param licenseModel License model information for the restored DB instance.
Default:
* Same as source.
Valid values: license-included
|
* bring-your-own-license
|
* general-public-license
*/
public void setLicenseModel(String licenseModel) {
this.licenseModel = licenseModel;
}
/**
* License model information for the restored DB instance.
Default:
* Same as source.
Valid values: license-included
|
* bring-your-own-license
|
* general-public-license
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param licenseModel License model information for the restored DB instance.
Default:
* Same as source.
Valid values: license-included
|
* bring-your-own-license
|
* general-public-license
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RestoreDBInstanceFromDBSnapshotRequest withLicenseModel(String licenseModel) {
this.licenseModel = licenseModel;
return this;
}
/**
* The database name for the restored DB instance. This
* parameter doesn't apply to the MySQL engine.
*
* @return The database name for the restored DB instance. This
* parameter doesn't apply to the MySQL engine.
*/
public String getDBName() {
return dBName;
}
/**
* The database name for the restored DB instance. This
* parameter doesn't apply to the MySQL engine.
*
* @param dBName The database name for the restored DB instance. This
* parameter doesn't apply to the MySQL engine.
*/
public void setDBName(String dBName) {
this.dBName = dBName;
}
/**
* The database name for the restored DB instance. This
* parameter doesn't apply to the MySQL engine.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param dBName The database name for the restored DB instance. This
* parameter doesn't apply to the MySQL engine.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RestoreDBInstanceFromDBSnapshotRequest withDBName(String dBName) {
this.dBName = dBName;
return this;
}
/**
* The database engine to use for the new instance.
Default: The same
* as source
Constraint: Must be compatible with the engine of the
* source
Example: oracle-ee
*
* @return The database engine to use for the new instance.
Default: The same
* as source
Constraint: Must be compatible with the engine of the
* source
Example: oracle-ee
*/
public String getEngine() {
return engine;
}
/**
* The database engine to use for the new instance.
Default: The same
* as source
Constraint: Must be compatible with the engine of the
* source
Example: oracle-ee
*
* @param engine The database engine to use for the new instance.
Default: The same
* as source
Constraint: Must be compatible with the engine of the
* source
Example: oracle-ee
*/
public void setEngine(String engine) {
this.engine = engine;
}
/**
* The database engine to use for the new instance.
Default: The same
* as source
Constraint: Must be compatible with the engine of the
* source
Example: oracle-ee
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param engine The database engine to use for the new instance.
Default: The same
* as source
Constraint: Must be compatible with the engine of the
* source
Example: oracle-ee
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RestoreDBInstanceFromDBSnapshotRequest withEngine(String engine) {
this.engine = engine;
return this;
}
/**
* Specifies the amount of provisioned IOPS for the DB instance,
* expressed in I/O operations per second. If this parameter is not
* specified, the IOPS value will be taken from the backup. If this
* parameter is set to 0, the new instance will be converted to a
* non-PIOPS instance, which will take additional time, though your DB
* instance will be available for connections before the conversion
* starts.
Constraints: Must be an integer greater than 1000.
*
* @return Specifies the amount of provisioned IOPS for the DB instance,
* expressed in I/O operations per second. If this parameter is not
* specified, the IOPS value will be taken from the backup. If this
* parameter is set to 0, the new instance will be converted to a
* non-PIOPS instance, which will take additional time, though your DB
* instance will be available for connections before the conversion
* starts.
Constraints: Must be an integer greater than 1000.
*/
public Integer getIops() {
return iops;
}
/**
* Specifies the amount of provisioned IOPS for the DB instance,
* expressed in I/O operations per second. If this parameter is not
* specified, the IOPS value will be taken from the backup. If this
* parameter is set to 0, the new instance will be converted to a
* non-PIOPS instance, which will take additional time, though your DB
* instance will be available for connections before the conversion
* starts.
Constraints: Must be an integer greater than 1000.
*
* @param iops Specifies the amount of provisioned IOPS for the DB instance,
* expressed in I/O operations per second. If this parameter is not
* specified, the IOPS value will be taken from the backup. If this
* parameter is set to 0, the new instance will be converted to a
* non-PIOPS instance, which will take additional time, though your DB
* instance will be available for connections before the conversion
* starts.
Constraints: Must be an integer greater than 1000.
*/
public void setIops(Integer iops) {
this.iops = iops;
}
/**
* Specifies the amount of provisioned IOPS for the DB instance,
* expressed in I/O operations per second. If this parameter is not
* specified, the IOPS value will be taken from the backup. If this
* parameter is set to 0, the new instance will be converted to a
* non-PIOPS instance, which will take additional time, though your DB
* instance will be available for connections before the conversion
* starts.
Constraints: Must be an integer greater than 1000.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param iops Specifies the amount of provisioned IOPS for the DB instance,
* expressed in I/O operations per second. If this parameter is not
* specified, the IOPS value will be taken from the backup. If this
* parameter is set to 0, the new instance will be converted to a
* non-PIOPS instance, which will take additional time, though your DB
* instance will be available for connections before the conversion
* starts.
Constraints: Must be an integer greater than 1000.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RestoreDBInstanceFromDBSnapshotRequest withIops(Integer iops) {
this.iops = iops;
return this;
}
/**
* The name of the option group to be used for the restored DB instance.
*
Permanent options, such as the TDE option for Oracle Advanced
* Security TDE, cannot be removed from an option group, and that option
* group cannot be removed from a DB instance once it is associated with
* a DB instance
*
* @return The name of the option group to be used for the restored DB instance.
*
Permanent options, such as the TDE option for Oracle Advanced
* Security TDE, cannot be removed from an option group, and that option
* group cannot be removed from a DB instance once it is associated with
* a DB instance
*/
public String getOptionGroupName() {
return optionGroupName;
}
/**
* The name of the option group to be used for the restored DB instance.
*
Permanent options, such as the TDE option for Oracle Advanced
* Security TDE, cannot be removed from an option group, and that option
* group cannot be removed from a DB instance once it is associated with
* a DB instance
*
* @param optionGroupName The name of the option group to be used for the restored DB instance.
*
Permanent options, such as the TDE option for Oracle Advanced
* Security TDE, cannot be removed from an option group, and that option
* group cannot be removed from a DB instance once it is associated with
* a DB instance
*/
public void setOptionGroupName(String optionGroupName) {
this.optionGroupName = optionGroupName;
}
/**
* The name of the option group to be used for the restored DB instance.
*
Permanent options, such as the TDE option for Oracle Advanced
* Security TDE, cannot be removed from an option group, and that option
* group cannot be removed from a DB instance once it is associated with
* a DB instance
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param optionGroupName The name of the option group to be used for the restored DB instance.
*
Permanent options, such as the TDE option for Oracle Advanced
* Security TDE, cannot be removed from an option group, and that option
* group cannot be removed from a DB instance once it is associated with
* a DB instance
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RestoreDBInstanceFromDBSnapshotRequest withOptionGroupName(String optionGroupName) {
this.optionGroupName = optionGroupName;
return this;
}
/**
* A list of tags.
*
* @return A list of tags.
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.ListWithAutoConstructFlag();
tags.setAutoConstruct(true);
}
return tags;
}
/**
* A list of tags.
*
* @param tags A list of tags.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
com.amazonaws.internal.ListWithAutoConstructFlag tagsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(tags.size());
tagsCopy.addAll(tags);
this.tags = tagsCopy;
}
/**
* A list of tags.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param tags A list of tags.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RestoreDBInstanceFromDBSnapshotRequest withTags(Tag... tags) {
if (getTags() == null) setTags(new java.util.ArrayList(tags.length));
for (Tag value : tags) {
getTags().add(value);
}
return this;
}
/**
* A list of tags.
*
* Returns a reference to this object so that method calls can be chained together.
*
* @param tags A list of tags.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public RestoreDBInstanceFromDBSnapshotRequest withTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
} else {
com.amazonaws.internal.ListWithAutoConstructFlag tagsCopy = new com.amazonaws.internal.ListWithAutoConstructFlag(tags.size());
tagsCopy.addAll(tags);
this.tags = tagsCopy;
}
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDBInstanceIdentifier() != null) sb.append("DBInstanceIdentifier: " + getDBInstanceIdentifier() + ",");
if (getDBSnapshotIdentifier() != null) sb.append("DBSnapshotIdentifier: " + getDBSnapshotIdentifier() + ",");
if (getDBInstanceClass() != null) sb.append("DBInstanceClass: " + getDBInstanceClass() + ",");
if (getPort() != null) sb.append("Port: " + getPort() + ",");
if (getAvailabilityZone() != null) sb.append("AvailabilityZone: " + getAvailabilityZone() + ",");
if (getDBSubnetGroupName() != null) sb.append("DBSubnetGroupName: " + getDBSubnetGroupName() + ",");
if (isMultiAZ() != null) sb.append("MultiAZ: " + isMultiAZ() + ",");
if (isPubliclyAccessible() != null) sb.append("PubliclyAccessible: " + isPubliclyAccessible() + ",");
if (isAutoMinorVersionUpgrade() != null) sb.append("AutoMinorVersionUpgrade: " + isAutoMinorVersionUpgrade() + ",");
if (getLicenseModel() != null) sb.append("LicenseModel: " + getLicenseModel() + ",");
if (getDBName() != null) sb.append("DBName: " + getDBName() + ",");
if (getEngine() != null) sb.append("Engine: " + getEngine() + ",");
if (getIops() != null) sb.append("Iops: " + getIops() + ",");
if (getOptionGroupName() != null) sb.append("OptionGroupName: " + getOptionGroupName() + ",");
if (getTags() != null) sb.append("Tags: " + getTags() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDBInstanceIdentifier() == null) ? 0 : getDBInstanceIdentifier().hashCode());
hashCode = prime * hashCode + ((getDBSnapshotIdentifier() == null) ? 0 : getDBSnapshotIdentifier().hashCode());
hashCode = prime * hashCode + ((getDBInstanceClass() == null) ? 0 : getDBInstanceClass().hashCode());
hashCode = prime * hashCode + ((getPort() == null) ? 0 : getPort().hashCode());
hashCode = prime * hashCode + ((getAvailabilityZone() == null) ? 0 : getAvailabilityZone().hashCode());
hashCode = prime * hashCode + ((getDBSubnetGroupName() == null) ? 0 : getDBSubnetGroupName().hashCode());
hashCode = prime * hashCode + ((isMultiAZ() == null) ? 0 : isMultiAZ().hashCode());
hashCode = prime * hashCode + ((isPubliclyAccessible() == null) ? 0 : isPubliclyAccessible().hashCode());
hashCode = prime * hashCode + ((isAutoMinorVersionUpgrade() == null) ? 0 : isAutoMinorVersionUpgrade().hashCode());
hashCode = prime * hashCode + ((getLicenseModel() == null) ? 0 : getLicenseModel().hashCode());
hashCode = prime * hashCode + ((getDBName() == null) ? 0 : getDBName().hashCode());
hashCode = prime * hashCode + ((getEngine() == null) ? 0 : getEngine().hashCode());
hashCode = prime * hashCode + ((getIops() == null) ? 0 : getIops().hashCode());
hashCode = prime * hashCode + ((getOptionGroupName() == null) ? 0 : getOptionGroupName().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof RestoreDBInstanceFromDBSnapshotRequest == false) return false;
RestoreDBInstanceFromDBSnapshotRequest other = (RestoreDBInstanceFromDBSnapshotRequest)obj;
if (other.getDBInstanceIdentifier() == null ^ this.getDBInstanceIdentifier() == null) return false;
if (other.getDBInstanceIdentifier() != null && other.getDBInstanceIdentifier().equals(this.getDBInstanceIdentifier()) == false) return false;
if (other.getDBSnapshotIdentifier() == null ^ this.getDBSnapshotIdentifier() == null) return false;
if (other.getDBSnapshotIdentifier() != null && other.getDBSnapshotIdentifier().equals(this.getDBSnapshotIdentifier()) == false) return false;
if (other.getDBInstanceClass() == null ^ this.getDBInstanceClass() == null) return false;
if (other.getDBInstanceClass() != null && other.getDBInstanceClass().equals(this.getDBInstanceClass()) == false) return false;
if (other.getPort() == null ^ this.getPort() == null) return false;
if (other.getPort() != null && other.getPort().equals(this.getPort()) == false) return false;
if (other.getAvailabilityZone() == null ^ this.getAvailabilityZone() == null) return false;
if (other.getAvailabilityZone() != null && other.getAvailabilityZone().equals(this.getAvailabilityZone()) == false) return false;
if (other.getDBSubnetGroupName() == null ^ this.getDBSubnetGroupName() == null) return false;
if (other.getDBSubnetGroupName() != null && other.getDBSubnetGroupName().equals(this.getDBSubnetGroupName()) == false) return false;
if (other.isMultiAZ() == null ^ this.isMultiAZ() == null) return false;
if (other.isMultiAZ() != null && other.isMultiAZ().equals(this.isMultiAZ()) == false) return false;
if (other.isPubliclyAccessible() == null ^ this.isPubliclyAccessible() == null) return false;
if (other.isPubliclyAccessible() != null && other.isPubliclyAccessible().equals(this.isPubliclyAccessible()) == false) return false;
if (other.isAutoMinorVersionUpgrade() == null ^ this.isAutoMinorVersionUpgrade() == null) return false;
if (other.isAutoMinorVersionUpgrade() != null && other.isAutoMinorVersionUpgrade().equals(this.isAutoMinorVersionUpgrade()) == false) return false;
if (other.getLicenseModel() == null ^ this.getLicenseModel() == null) return false;
if (other.getLicenseModel() != null && other.getLicenseModel().equals(this.getLicenseModel()) == false) return false;
if (other.getDBName() == null ^ this.getDBName() == null) return false;
if (other.getDBName() != null && other.getDBName().equals(this.getDBName()) == false) return false;
if (other.getEngine() == null ^ this.getEngine() == null) return false;
if (other.getEngine() != null && other.getEngine().equals(this.getEngine()) == false) return false;
if (other.getIops() == null ^ this.getIops() == null) return false;
if (other.getIops() != null && other.getIops().equals(this.getIops()) == false) return false;
if (other.getOptionGroupName() == null ^ this.getOptionGroupName() == null) return false;
if (other.getOptionGroupName() != null && other.getOptionGroupName().equals(this.getOptionGroupName()) == false) return false;
if (other.getTags() == null ^ this.getTags() == null) return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false) return false;
return true;
}
}