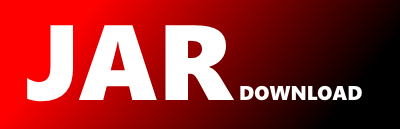
com.amazonaws.services.redshift.AmazonRedshiftAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.redshift;
import java.util.concurrent.Future;
import com.amazonaws.AmazonClientException;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.handlers.AsyncHandler;
import com.amazonaws.services.redshift.model.*;
/**
* Interface for accessing AmazonRedshift asynchronously.
* Each asynchronous method will return a Java Future object, and users are also allowed
* to provide a callback handler.
* Amazon Redshift Overview
* This is an interface reference for Amazon Redshift. It contains
* documentation for one of the programming or command line interfaces
* you can use to manage Amazon Redshift clusters. Note that Amazon
* Redshift is asynchronous, which means that some interfaces may require
* techniques, such as polling or asynchronous callback handlers, to
* determine when a command has been applied. In this reference, the
* parameter descriptions indicate whether a change is applied
* immediately, on the next instance reboot, or during the next
* maintenance window. For a summary of the Amazon Redshift cluster
* management interfaces, go to
* Using the Amazon Redshift Management Interfaces
* .
*
*
* Amazon Redshift manages all the work of setting up, operating, and
* scaling a data warehouse: provisioning capacity, monitoring and
* backing up the cluster, and applying patches and upgrades to the
* Amazon Redshift engine. You can focus on using your data to acquire
* new insights for your business and customers.
*
*
* If you are a first-time user of Amazon Redshift, we recommend that you
* begin by reading the The
* Amazon Redshift Getting Started Guide
*
*
*
* If you are a database developer, the
* Amazon Redshift Database Developer Guide
* explains how to design, build, query, and maintain the databases that
* make up your data warehouse.
*
*/
public interface AmazonRedshiftAsync extends AmazonRedshift {
/**
*
* Removes the ability of the specified AWS customer account to restore
* the specified snapshot. If the account is currently restoring the
* snapshot, the restore will run to completion.
*
*
* For more information about working with snapshots, go to
* Amazon Redshift Snapshots
* in the Amazon Redshift Management Guide .
*
*
* @param revokeSnapshotAccessRequest Container for the necessary
* parameters to execute the RevokeSnapshotAccess operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* RevokeSnapshotAccess service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future revokeSnapshotAccessAsync(RevokeSnapshotAccessRequest revokeSnapshotAccessRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Removes the ability of the specified AWS customer account to restore
* the specified snapshot. If the account is currently restoring the
* snapshot, the restore will run to completion.
*
*
* For more information about working with snapshots, go to
* Amazon Redshift Snapshots
* in the Amazon Redshift Management Guide .
*
*
* @param revokeSnapshotAccessRequest Container for the necessary
* parameters to execute the RevokeSnapshotAccess operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* RevokeSnapshotAccess service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future revokeSnapshotAccessAsync(RevokeSnapshotAccessRequest revokeSnapshotAccessRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Modifies the number of days to retain automated snapshots in the
* destination region after they are copied from the source region.
*
*
* @param modifySnapshotCopyRetentionPeriodRequest Container for the
* necessary parameters to execute the ModifySnapshotCopyRetentionPeriod
* operation on AmazonRedshift.
*
* @return A Java Future object containing the response from the
* ModifySnapshotCopyRetentionPeriod service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future modifySnapshotCopyRetentionPeriodAsync(ModifySnapshotCopyRetentionPeriodRequest modifySnapshotCopyRetentionPeriodRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Modifies the number of days to retain automated snapshots in the
* destination region after they are copied from the source region.
*
*
* @param modifySnapshotCopyRetentionPeriodRequest Container for the
* necessary parameters to execute the ModifySnapshotCopyRetentionPeriod
* operation on AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ModifySnapshotCopyRetentionPeriod service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future modifySnapshotCopyRetentionPeriodAsync(ModifySnapshotCopyRetentionPeriodRequest modifySnapshotCopyRetentionPeriodRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Modifies a cluster subnet group to include the specified list of VPC
* subnets. The operation replaces the existing list of subnets with the
* new list of subnets.
*
*
* @param modifyClusterSubnetGroupRequest Container for the necessary
* parameters to execute the ModifyClusterSubnetGroup operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* ModifyClusterSubnetGroup service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future modifyClusterSubnetGroupAsync(ModifyClusterSubnetGroupRequest modifyClusterSubnetGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Modifies a cluster subnet group to include the specified list of VPC
* subnets. The operation replaces the existing list of subnets with the
* new list of subnets.
*
*
* @param modifyClusterSubnetGroupRequest Container for the necessary
* parameters to execute the ModifyClusterSubnetGroup operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ModifyClusterSubnetGroup service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future modifyClusterSubnetGroupAsync(ModifyClusterSubnetGroupRequest modifyClusterSubnetGroupRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes the specified Amazon Redshift HSM configuration.
*
*
* @param deleteHsmConfigurationRequest Container for the necessary
* parameters to execute the DeleteHsmConfiguration operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* DeleteHsmConfiguration service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteHsmConfigurationAsync(DeleteHsmConfigurationRequest deleteHsmConfigurationRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes the specified Amazon Redshift HSM configuration.
*
*
* @param deleteHsmConfigurationRequest Container for the necessary
* parameters to execute the DeleteHsmConfiguration operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteHsmConfiguration service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteHsmConfigurationAsync(DeleteHsmConfigurationRequest deleteHsmConfigurationRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Allows you to purchase reserved nodes. Amazon Redshift offers a
* predefined set of reserved node offerings. You can purchase one of the
* offerings. You can call the DescribeReservedNodeOfferings API to
* obtain the available reserved node offerings. You can call this API by
* providing a specific reserved node offering and the number of nodes
* you want to reserve.
*
*
* For more information about managing parameter groups, go to
* Purchasing Reserved Nodes
* in the Amazon Redshift Management Guide .
*
*
*
*
* @param purchaseReservedNodeOfferingRequest Container for the necessary
* parameters to execute the PurchaseReservedNodeOffering operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* PurchaseReservedNodeOffering service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future purchaseReservedNodeOfferingAsync(PurchaseReservedNodeOfferingRequest purchaseReservedNodeOfferingRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Allows you to purchase reserved nodes. Amazon Redshift offers a
* predefined set of reserved node offerings. You can purchase one of the
* offerings. You can call the DescribeReservedNodeOfferings API to
* obtain the available reserved node offerings. You can call this API by
* providing a specific reserved node offering and the number of nodes
* you want to reserve.
*
*
* For more information about managing parameter groups, go to
* Purchasing Reserved Nodes
* in the Amazon Redshift Management Guide .
*
*
*
*
* @param purchaseReservedNodeOfferingRequest Container for the necessary
* parameters to execute the PurchaseReservedNodeOffering operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* PurchaseReservedNodeOffering service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future purchaseReservedNodeOfferingAsync(PurchaseReservedNodeOfferingRequest purchaseReservedNodeOfferingRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Stops logging information, such as queries and connection attempts,
* for the specified Amazon Redshift cluster.
*
*
* @param disableLoggingRequest Container for the necessary parameters to
* execute the DisableLogging operation on AmazonRedshift.
*
* @return A Java Future object containing the response from the
* DisableLogging service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future disableLoggingAsync(DisableLoggingRequest disableLoggingRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Stops logging information, such as queries and connection attempts,
* for the specified Amazon Redshift cluster.
*
*
* @param disableLoggingRequest Container for the necessary parameters to
* execute the DisableLogging operation on AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DisableLogging service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future disableLoggingAsync(DisableLoggingRequest disableLoggingRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Modifies the settings for a cluster. For example, you can add another
* security or parameter group, update the preferred maintenance window,
* or change the master user password. Resetting a cluster password or
* modifying the security groups associated with a cluster do not need a
* reboot. However, modifying parameter group requires a reboot for
* parameters to take effect. For more information about managing
* clusters, go to
* Amazon Redshift Clusters
* in the Amazon Redshift Management Guide
*
*
* You can also change node type and the number of nodes to scale up or
* down the cluster. When resizing a cluster, you must specify both the
* number of nodes and the node type even if one of the parameters does
* not change. If you specify the same number of nodes and node type that
* are already configured for the cluster, an error is returned.
*
*
* @param modifyClusterRequest Container for the necessary parameters to
* execute the ModifyCluster operation on AmazonRedshift.
*
* @return A Java Future object containing the response from the
* ModifyCluster service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future modifyClusterAsync(ModifyClusterRequest modifyClusterRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Modifies the settings for a cluster. For example, you can add another
* security or parameter group, update the preferred maintenance window,
* or change the master user password. Resetting a cluster password or
* modifying the security groups associated with a cluster do not need a
* reboot. However, modifying parameter group requires a reboot for
* parameters to take effect. For more information about managing
* clusters, go to
* Amazon Redshift Clusters
* in the Amazon Redshift Management Guide
*
*
* You can also change node type and the number of nodes to scale up or
* down the cluster. When resizing a cluster, you must specify both the
* number of nodes and the node type even if one of the parameters does
* not change. If you specify the same number of nodes and node type that
* are already configured for the cluster, an error is returned.
*
*
* @param modifyClusterRequest Container for the necessary parameters to
* execute the ModifyCluster operation on AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ModifyCluster service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future modifyClusterAsync(ModifyClusterRequest modifyClusterRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Copies the specified automated cluster snapshot to a new manual
* cluster snapshot. The source must be an automated snapshot and it must
* be in the available state.
*
*
* When you delete a cluster, Amazon Redshift deletes any automated
* snapshots of the cluster. Also, when the retention period of the
* snapshot expires, Amazon Redshift automatically deletes it. If you
* want to keep an automated snapshot for a longer period, you can make a
* manual copy of the snapshot. Manual snapshots are retained until you
* delete them.
*
*
* For more information about working with snapshots, go to
* Amazon Redshift Snapshots
* in the Amazon Redshift Management Guide .
*
*
* @param copyClusterSnapshotRequest Container for the necessary
* parameters to execute the CopyClusterSnapshot operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* CopyClusterSnapshot service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future copyClusterSnapshotAsync(CopyClusterSnapshotRequest copyClusterSnapshotRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Copies the specified automated cluster snapshot to a new manual
* cluster snapshot. The source must be an automated snapshot and it must
* be in the available state.
*
*
* When you delete a cluster, Amazon Redshift deletes any automated
* snapshots of the cluster. Also, when the retention period of the
* snapshot expires, Amazon Redshift automatically deletes it. If you
* want to keep an automated snapshot for a longer period, you can make a
* manual copy of the snapshot. Manual snapshots are retained until you
* delete them.
*
*
* For more information about working with snapshots, go to
* Amazon Redshift Snapshots
* in the Amazon Redshift Management Guide .
*
*
* @param copyClusterSnapshotRequest Container for the necessary
* parameters to execute the CopyClusterSnapshot operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CopyClusterSnapshot service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future copyClusterSnapshotAsync(CopyClusterSnapshotRequest copyClusterSnapshotRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a list of orderable cluster options. Before you create a new
* cluster you can use this operation to find what options are available,
* such as the EC2 Availability Zones (AZ) in the specific AWS region
* that you can specify, and the node types you can request. The node
* types differ by available storage, memory, CPU and price. With the
* cost involved you might want to obtain a list of cluster options in
* the specific region and specify values when creating a cluster. For
* more information about managing clusters, go to
* Amazon Redshift Clusters
* in the Amazon Redshift Management Guide
*
*
* @param describeOrderableClusterOptionsRequest Container for the
* necessary parameters to execute the DescribeOrderableClusterOptions
* operation on AmazonRedshift.
*
* @return A Java Future object containing the response from the
* DescribeOrderableClusterOptions service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeOrderableClusterOptionsAsync(DescribeOrderableClusterOptionsRequest describeOrderableClusterOptionsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a list of orderable cluster options. Before you create a new
* cluster you can use this operation to find what options are available,
* such as the EC2 Availability Zones (AZ) in the specific AWS region
* that you can specify, and the node types you can request. The node
* types differ by available storage, memory, CPU and price. With the
* cost involved you might want to obtain a list of cluster options in
* the specific region and specify values when creating a cluster. For
* more information about managing clusters, go to
* Amazon Redshift Clusters
* in the Amazon Redshift Management Guide
*
*
* @param describeOrderableClusterOptionsRequest Container for the
* necessary parameters to execute the DescribeOrderableClusterOptions
* operation on AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeOrderableClusterOptions service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeOrderableClusterOptionsAsync(DescribeOrderableClusterOptionsRequest describeOrderableClusterOptionsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Starts logging information, such as queries and connection attempts,
* for the specified Amazon Redshift cluster.
*
*
* @param enableLoggingRequest Container for the necessary parameters to
* execute the EnableLogging operation on AmazonRedshift.
*
* @return A Java Future object containing the response from the
* EnableLogging service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future enableLoggingAsync(EnableLoggingRequest enableLoggingRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Starts logging information, such as queries and connection attempts,
* for the specified Amazon Redshift cluster.
*
*
* @param enableLoggingRequest Container for the necessary parameters to
* execute the EnableLogging operation on AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* EnableLogging service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future enableLoggingAsync(EnableLoggingRequest enableLoggingRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates a new Amazon Redshift subnet group. You must provide a list
* of one or more subnets in your existing Amazon Virtual Private Cloud
* (Amazon VPC) when creating Amazon Redshift subnet group.
*
*
* For information about subnet groups, go to
* Amazon Redshift Cluster Subnet Groups
* in the Amazon Redshift Management Guide .
*
*
*
* @param createClusterSubnetGroupRequest Container for the necessary
* parameters to execute the CreateClusterSubnetGroup operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* CreateClusterSubnetGroup service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createClusterSubnetGroupAsync(CreateClusterSubnetGroupRequest createClusterSubnetGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates a new Amazon Redshift subnet group. You must provide a list
* of one or more subnets in your existing Amazon Virtual Private Cloud
* (Amazon VPC) when creating Amazon Redshift subnet group.
*
*
* For information about subnet groups, go to
* Amazon Redshift Cluster Subnet Groups
* in the Amazon Redshift Management Guide .
*
*
*
* @param createClusterSubnetGroupRequest Container for the necessary
* parameters to execute the CreateClusterSubnetGroup operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateClusterSubnetGroup service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createClusterSubnetGroupAsync(CreateClusterSubnetGroupRequest createClusterSubnetGroupRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes the specified HSM client certificate.
*
*
* @param deleteHsmClientCertificateRequest Container for the necessary
* parameters to execute the DeleteHsmClientCertificate operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* DeleteHsmClientCertificate service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteHsmClientCertificateAsync(DeleteHsmClientCertificateRequest deleteHsmClientCertificateRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes the specified HSM client certificate.
*
*
* @param deleteHsmClientCertificateRequest Container for the necessary
* parameters to execute the DeleteHsmClientCertificate operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteHsmClientCertificate service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteHsmClientCertificateAsync(DeleteHsmClientCertificateRequest deleteHsmClientCertificateRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Reboots a cluster. This action is taken as soon as possible. It
* results in a momentary outage to the cluster, during which the cluster
* status is set to rebooting
. A cluster event is created
* when the reboot is completed. Any pending cluster modifications (see
* ModifyCluster) are applied at this reboot. For more information about
* managing clusters, go to
* Amazon Redshift Clusters
* in the Amazon Redshift Management Guide
*
*
* @param rebootClusterRequest Container for the necessary parameters to
* execute the RebootCluster operation on AmazonRedshift.
*
* @return A Java Future object containing the response from the
* RebootCluster service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future rebootClusterAsync(RebootClusterRequest rebootClusterRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Reboots a cluster. This action is taken as soon as possible. It
* results in a momentary outage to the cluster, during which the cluster
* status is set to rebooting
. A cluster event is created
* when the reboot is completed. Any pending cluster modifications (see
* ModifyCluster) are applied at this reboot. For more information about
* managing clusters, go to
* Amazon Redshift Clusters
* in the Amazon Redshift Management Guide
*
*
* @param rebootClusterRequest Container for the necessary parameters to
* execute the RebootCluster operation on AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* RebootCluster service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future rebootClusterAsync(RebootClusterRequest rebootClusterRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes the specified cluster subnet group.
*
*
* @param deleteClusterSubnetGroupRequest Container for the necessary
* parameters to execute the DeleteClusterSubnetGroup operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* DeleteClusterSubnetGroup service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteClusterSubnetGroupAsync(DeleteClusterSubnetGroupRequest deleteClusterSubnetGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes the specified cluster subnet group.
*
*
* @param deleteClusterSubnetGroupRequest Container for the necessary
* parameters to execute the DeleteClusterSubnetGroup operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteClusterSubnetGroup service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteClusterSubnetGroupAsync(DeleteClusterSubnetGroupRequest deleteClusterSubnetGroupRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes a previously provisioned cluster. A successful response from
* the web service indicates that the request was received correctly. If
* a final cluster snapshot is requested the status of the cluster will
* be "final-snapshot" while the snapshot is being taken, then it's
* "deleting" once Amazon Redshift begins deleting the cluster. Use
* DescribeClusters to monitor the status of the deletion. The delete
* operation cannot be canceled or reverted once submitted. For more
* information about managing clusters, go to
* Amazon Redshift Clusters
* in the Amazon Redshift Management Guide .
*
*
*
* @param deleteClusterRequest Container for the necessary parameters to
* execute the DeleteCluster operation on AmazonRedshift.
*
* @return A Java Future object containing the response from the
* DeleteCluster service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteClusterAsync(DeleteClusterRequest deleteClusterRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes a previously provisioned cluster. A successful response from
* the web service indicates that the request was received correctly. If
* a final cluster snapshot is requested the status of the cluster will
* be "final-snapshot" while the snapshot is being taken, then it's
* "deleting" once Amazon Redshift begins deleting the cluster. Use
* DescribeClusters to monitor the status of the deletion. The delete
* operation cannot be canceled or reverted once submitted. For more
* information about managing clusters, go to
* Amazon Redshift Clusters
* in the Amazon Redshift Management Guide .
*
*
*
* @param deleteClusterRequest Container for the necessary parameters to
* execute the DeleteCluster operation on AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteCluster service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteClusterAsync(DeleteClusterRequest deleteClusterRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates a manual snapshot of the specified cluster. The cluster must
* be in the "available" state.
*
*
* For more information about working with snapshots, go to
* Amazon Redshift Snapshots
* in the Amazon Redshift Management Guide .
*
*
* @param createClusterSnapshotRequest Container for the necessary
* parameters to execute the CreateClusterSnapshot operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* CreateClusterSnapshot service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createClusterSnapshotAsync(CreateClusterSnapshotRequest createClusterSnapshotRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates a manual snapshot of the specified cluster. The cluster must
* be in the "available" state.
*
*
* For more information about working with snapshots, go to
* Amazon Redshift Snapshots
* in the Amazon Redshift Management Guide .
*
*
* @param createClusterSnapshotRequest Container for the necessary
* parameters to execute the CreateClusterSnapshot operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateClusterSnapshot service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createClusterSnapshotAsync(CreateClusterSnapshotRequest createClusterSnapshotRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates a new cluster. To create the cluster in virtual private cloud
* (VPC), you must provide cluster subnet group name. If you don't
* provide a cluster subnet group name or the cluster security group
* parameter, Amazon Redshift creates a non-VPC cluster, it associates
* the default cluster security group with the cluster. For more
* information about managing clusters, go to
* Amazon Redshift Clusters
* in the Amazon Redshift Management Guide .
*
*
*
* @param createClusterRequest Container for the necessary parameters to
* execute the CreateCluster operation on AmazonRedshift.
*
* @return A Java Future object containing the response from the
* CreateCluster service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createClusterAsync(CreateClusterRequest createClusterRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates a new cluster. To create the cluster in virtual private cloud
* (VPC), you must provide cluster subnet group name. If you don't
* provide a cluster subnet group name or the cluster security group
* parameter, Amazon Redshift creates a non-VPC cluster, it associates
* the default cluster security group with the cluster. For more
* information about managing clusters, go to
* Amazon Redshift Clusters
* in the Amazon Redshift Management Guide .
*
*
*
* @param createClusterRequest Container for the necessary parameters to
* execute the CreateCluster operation on AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateCluster service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createClusterAsync(CreateClusterRequest createClusterRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns events related to clusters, security groups, snapshots, and
* parameter groups for the past 14 days. Events specific to a particular
* cluster, security group, snapshot or parameter group can be obtained
* by providing the name as a parameter. By default, the past hour of
* events are returned.
*
*
* @param describeEventsRequest Container for the necessary parameters to
* execute the DescribeEvents operation on AmazonRedshift.
*
* @return A Java Future object containing the response from the
* DescribeEvents service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeEventsAsync(DescribeEventsRequest describeEventsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns events related to clusters, security groups, snapshots, and
* parameter groups for the past 14 days. Events specific to a particular
* cluster, security group, snapshot or parameter group can be obtained
* by providing the name as a parameter. By default, the past hour of
* events are returned.
*
*
* @param describeEventsRequest Container for the necessary parameters to
* execute the DescribeEvents operation on AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeEvents service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeEventsAsync(DescribeEventsRequest describeEventsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Adds an inbound (ingress) rule to an Amazon Redshift security group.
* Depending on whether the application accessing your cluster is running
* on the Internet or an EC2 instance, you can authorize inbound access
* to either a Classless Interdomain Routing (CIDR) IP address range or
* an EC2 security group. You can add as many as 20 ingress rules to an
* Amazon Redshift security group.
*
*
* NOTE: The EC2 security group must be defined in the AWS region
* where the cluster resides.
*
*
* For an overview of CIDR blocks, see the Wikipedia article on
* Classless Inter-Domain Routing
* .
*
*
* You must also associate the security group with a cluster so that
* clients running on these IP addresses or the EC2 instance are
* authorized to connect to the cluster. For information about managing
* security groups, go to
* Working with Security Groups
* in the Amazon Redshift Management Guide .
*
*
* @param authorizeClusterSecurityGroupIngressRequest Container for the
* necessary parameters to execute the
* AuthorizeClusterSecurityGroupIngress operation on AmazonRedshift.
*
* @return A Java Future object containing the response from the
* AuthorizeClusterSecurityGroupIngress service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future authorizeClusterSecurityGroupIngressAsync(AuthorizeClusterSecurityGroupIngressRequest authorizeClusterSecurityGroupIngressRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Adds an inbound (ingress) rule to an Amazon Redshift security group.
* Depending on whether the application accessing your cluster is running
* on the Internet or an EC2 instance, you can authorize inbound access
* to either a Classless Interdomain Routing (CIDR) IP address range or
* an EC2 security group. You can add as many as 20 ingress rules to an
* Amazon Redshift security group.
*
*
* NOTE: The EC2 security group must be defined in the AWS region
* where the cluster resides.
*
*
* For an overview of CIDR blocks, see the Wikipedia article on
* Classless Inter-Domain Routing
* .
*
*
* You must also associate the security group with a cluster so that
* clients running on these IP addresses or the EC2 instance are
* authorized to connect to the cluster. For information about managing
* security groups, go to
* Working with Security Groups
* in the Amazon Redshift Management Guide .
*
*
* @param authorizeClusterSecurityGroupIngressRequest Container for the
* necessary parameters to execute the
* AuthorizeClusterSecurityGroupIngress operation on AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* AuthorizeClusterSecurityGroupIngress service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future authorizeClusterSecurityGroupIngressAsync(AuthorizeClusterSecurityGroupIngressRequest authorizeClusterSecurityGroupIngressRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Rotates the encryption keys for a cluster.
*
*
* @param rotateEncryptionKeyRequest Container for the necessary
* parameters to execute the RotateEncryptionKey operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* RotateEncryptionKey service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future rotateEncryptionKeyAsync(RotateEncryptionKeyRequest rotateEncryptionKeyRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Rotates the encryption keys for a cluster.
*
*
* @param rotateEncryptionKeyRequest Container for the necessary
* parameters to execute the RotateEncryptionKey operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* RotateEncryptionKey service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future rotateEncryptionKeyAsync(RotateEncryptionKeyRequest rotateEncryptionKeyRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes an Amazon Redshift security group.
*
*
* NOTE:You cannot delete a security group that is associated with
* any clusters. You cannot delete the default security group.
*
*
* For information about managing security groups, go to
* Amazon Redshift Cluster Security Groups
* in the Amazon Redshift Management Guide .
*
*
* @param deleteClusterSecurityGroupRequest Container for the necessary
* parameters to execute the DeleteClusterSecurityGroup operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* DeleteClusterSecurityGroup service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteClusterSecurityGroupAsync(DeleteClusterSecurityGroupRequest deleteClusterSecurityGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes an Amazon Redshift security group.
*
*
* NOTE:You cannot delete a security group that is associated with
* any clusters. You cannot delete the default security group.
*
*
* For information about managing security groups, go to
* Amazon Redshift Cluster Security Groups
* in the Amazon Redshift Management Guide .
*
*
* @param deleteClusterSecurityGroupRequest Container for the necessary
* parameters to execute the DeleteClusterSecurityGroup operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteClusterSecurityGroup service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteClusterSecurityGroupAsync(DeleteClusterSecurityGroupRequest deleteClusterSecurityGroupRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns the descriptions of the reserved nodes.
*
*
* @param describeReservedNodesRequest Container for the necessary
* parameters to execute the DescribeReservedNodes operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* DescribeReservedNodes service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeReservedNodesAsync(DescribeReservedNodesRequest describeReservedNodesRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns the descriptions of the reserved nodes.
*
*
* @param describeReservedNodesRequest Container for the necessary
* parameters to execute the DescribeReservedNodes operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeReservedNodes service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeReservedNodesAsync(DescribeReservedNodesRequest describeReservedNodesRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a list of parameter settings for the specified parameter
* group family.
*
*
* For more information about managing parameter groups, go to
* Amazon Redshift Parameter Groups
* in the Amazon Redshift Management Guide .
*
*
* @param describeDefaultClusterParametersRequest Container for the
* necessary parameters to execute the DescribeDefaultClusterParameters
* operation on AmazonRedshift.
*
* @return A Java Future object containing the response from the
* DescribeDefaultClusterParameters service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeDefaultClusterParametersAsync(DescribeDefaultClusterParametersRequest describeDefaultClusterParametersRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a list of parameter settings for the specified parameter
* group family.
*
*
* For more information about managing parameter groups, go to
* Amazon Redshift Parameter Groups
* in the Amazon Redshift Management Guide .
*
*
* @param describeDefaultClusterParametersRequest Container for the
* necessary parameters to execute the DescribeDefaultClusterParameters
* operation on AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeDefaultClusterParameters service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeDefaultClusterParametersAsync(DescribeDefaultClusterParametersRequest describeDefaultClusterParametersRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Modifies an existing Amazon Redshift event notification subscription.
*
*
* @param modifyEventSubscriptionRequest Container for the necessary
* parameters to execute the ModifyEventSubscription operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* ModifyEventSubscription service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future modifyEventSubscriptionAsync(ModifyEventSubscriptionRequest modifyEventSubscriptionRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Modifies an existing Amazon Redshift event notification subscription.
*
*
* @param modifyEventSubscriptionRequest Container for the necessary
* parameters to execute the ModifyEventSubscription operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ModifyEventSubscription service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future modifyEventSubscriptionAsync(ModifyEventSubscriptionRequest modifyEventSubscriptionRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates a new Amazon Redshift security group. You use security groups
* to control access to non-VPC clusters.
*
*
* For information about managing security groups, go to
* Amazon Redshift Cluster Security Groups
* in the Amazon Redshift Management Guide .
*
*
*
* @param createClusterSecurityGroupRequest Container for the necessary
* parameters to execute the CreateClusterSecurityGroup operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* CreateClusterSecurityGroup service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createClusterSecurityGroupAsync(CreateClusterSecurityGroupRequest createClusterSecurityGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates a new Amazon Redshift security group. You use security groups
* to control access to non-VPC clusters.
*
*
* For information about managing security groups, go to
* Amazon Redshift Cluster Security Groups
* in the Amazon Redshift Management Guide .
*
*
*
* @param createClusterSecurityGroupRequest Container for the necessary
* parameters to execute the CreateClusterSecurityGroup operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateClusterSecurityGroup service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createClusterSecurityGroupAsync(CreateClusterSecurityGroupRequest createClusterSecurityGroupRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns information about the last resize operation for the specified
* cluster. If no resize operation has ever been initiated for the
* specified cluster, a HTTP 404
error is returned. If a
* resize operation was initiated and completed, the status of the resize
* remains as SUCCEEDED
until the next resize.
*
*
* A resize operation can be requested using ModifyCluster and
* specifying a different number or type of nodes for the cluster.
*
*
* @param describeResizeRequest Container for the necessary parameters to
* execute the DescribeResize operation on AmazonRedshift.
*
* @return A Java Future object containing the response from the
* DescribeResize service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeResizeAsync(DescribeResizeRequest describeResizeRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns information about the last resize operation for the specified
* cluster. If no resize operation has ever been initiated for the
* specified cluster, a HTTP 404
error is returned. If a
* resize operation was initiated and completed, the status of the resize
* remains as SUCCEEDED
until the next resize.
*
*
* A resize operation can be requested using ModifyCluster and
* specifying a different number or type of nodes for the cluster.
*
*
* @param describeResizeRequest Container for the necessary parameters to
* execute the DescribeResize operation on AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeResize service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeResizeAsync(DescribeResizeRequest describeResizeRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns descriptions of the available Amazon Redshift cluster
* versions. You can call this operation even before creating any
* clusters to learn more about the Amazon Redshift versions. For more
* information about managing clusters, go to
* Amazon Redshift Clusters
* in the Amazon Redshift Management Guide
*
*
* @param describeClusterVersionsRequest Container for the necessary
* parameters to execute the DescribeClusterVersions operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* DescribeClusterVersions service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeClusterVersionsAsync(DescribeClusterVersionsRequest describeClusterVersionsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns descriptions of the available Amazon Redshift cluster
* versions. You can call this operation even before creating any
* clusters to learn more about the Amazon Redshift versions. For more
* information about managing clusters, go to
* Amazon Redshift Clusters
* in the Amazon Redshift Management Guide
*
*
* @param describeClusterVersionsRequest Container for the necessary
* parameters to execute the DescribeClusterVersions operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeClusterVersions service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeClusterVersionsAsync(DescribeClusterVersionsRequest describeClusterVersionsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates a new cluster from a snapshot. Amazon Redshift creates the
* resulting cluster with the same configuration as the original cluster
* from which the snapshot was created, except that the new cluster is
* created with the default cluster security and parameter group. After
* Amazon Redshift creates the cluster you can use the ModifyCluster API
* to associate a different security group and different parameter group
* with the restored cluster.
*
*
* If a snapshot is taken of a cluster in VPC, you can restore it only
* in VPC. In this case, you must provide a cluster subnet group where
* you want the cluster restored. If snapshot is taken of a cluster
* outside VPC, then you can restore it only outside VPC.
*
*
* For more information about working with snapshots, go to
* Amazon Redshift Snapshots
* in the Amazon Redshift Management Guide .
*
*
* @param restoreFromClusterSnapshotRequest Container for the necessary
* parameters to execute the RestoreFromClusterSnapshot operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* RestoreFromClusterSnapshot service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future restoreFromClusterSnapshotAsync(RestoreFromClusterSnapshotRequest restoreFromClusterSnapshotRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates a new cluster from a snapshot. Amazon Redshift creates the
* resulting cluster with the same configuration as the original cluster
* from which the snapshot was created, except that the new cluster is
* created with the default cluster security and parameter group. After
* Amazon Redshift creates the cluster you can use the ModifyCluster API
* to associate a different security group and different parameter group
* with the restored cluster.
*
*
* If a snapshot is taken of a cluster in VPC, you can restore it only
* in VPC. In this case, you must provide a cluster subnet group where
* you want the cluster restored. If snapshot is taken of a cluster
* outside VPC, then you can restore it only outside VPC.
*
*
* For more information about working with snapshots, go to
* Amazon Redshift Snapshots
* in the Amazon Redshift Management Guide .
*
*
* @param restoreFromClusterSnapshotRequest Container for the necessary
* parameters to execute the RestoreFromClusterSnapshot operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* RestoreFromClusterSnapshot service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future restoreFromClusterSnapshotAsync(RestoreFromClusterSnapshotRequest restoreFromClusterSnapshotRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Modifies the parameters of a parameter group.
*
*
* For more information about managing parameter groups, go to
* Amazon Redshift Parameter Groups
* in the Amazon Redshift Management Guide .
*
*
* @param modifyClusterParameterGroupRequest Container for the necessary
* parameters to execute the ModifyClusterParameterGroup operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* ModifyClusterParameterGroup service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future modifyClusterParameterGroupAsync(ModifyClusterParameterGroupRequest modifyClusterParameterGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Modifies the parameters of a parameter group.
*
*
* For more information about managing parameter groups, go to
* Amazon Redshift Parameter Groups
* in the Amazon Redshift Management Guide .
*
*
* @param modifyClusterParameterGroupRequest Container for the necessary
* parameters to execute the ModifyClusterParameterGroup operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ModifyClusterParameterGroup service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future modifyClusterParameterGroupAsync(ModifyClusterParameterGroupRequest modifyClusterParameterGroupRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Displays a list of event categories for all event source types, or for
* a specified source type. For a list of the event categories and source
* types, go to
* Amazon Redshift Event Notifications
* .
*
*
* @param describeEventCategoriesRequest Container for the necessary
* parameters to execute the DescribeEventCategories operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* DescribeEventCategories service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeEventCategoriesAsync(DescribeEventCategoriesRequest describeEventCategoriesRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Displays a list of event categories for all event source types, or for
* a specified source type. For a list of the event categories and source
* types, go to
* Amazon Redshift Event Notifications
* .
*
*
* @param describeEventCategoriesRequest Container for the necessary
* parameters to execute the DescribeEventCategories operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeEventCategories service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeEventCategoriesAsync(DescribeEventCategoriesRequest describeEventCategoriesRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns information about Amazon Redshift security groups. If the
* name of a security group is specified, the response will contain only
* information about only that security group.
*
*
* For information about managing security groups, go to
* Amazon Redshift Cluster Security Groups
* in the Amazon Redshift Management Guide .
*
*
* @param describeClusterSecurityGroupsRequest Container for the
* necessary parameters to execute the DescribeClusterSecurityGroups
* operation on AmazonRedshift.
*
* @return A Java Future object containing the response from the
* DescribeClusterSecurityGroups service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeClusterSecurityGroupsAsync(DescribeClusterSecurityGroupsRequest describeClusterSecurityGroupsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns information about Amazon Redshift security groups. If the
* name of a security group is specified, the response will contain only
* information about only that security group.
*
*
* For information about managing security groups, go to
* Amazon Redshift Cluster Security Groups
* in the Amazon Redshift Management Guide .
*
*
* @param describeClusterSecurityGroupsRequest Container for the
* necessary parameters to execute the DescribeClusterSecurityGroups
* operation on AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeClusterSecurityGroups service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeClusterSecurityGroupsAsync(DescribeClusterSecurityGroupsRequest describeClusterSecurityGroupsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns one or more cluster subnet group objects, which contain
* metadata about your cluster subnet groups. By default, this operation
* returns information about all cluster subnet groups that are defined
* in you AWS account.
*
*
* @param describeClusterSubnetGroupsRequest Container for the necessary
* parameters to execute the DescribeClusterSubnetGroups operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* DescribeClusterSubnetGroups service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeClusterSubnetGroupsAsync(DescribeClusterSubnetGroupsRequest describeClusterSubnetGroupsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns one or more cluster subnet group objects, which contain
* metadata about your cluster subnet groups. By default, this operation
* returns information about all cluster subnet groups that are defined
* in you AWS account.
*
*
* @param describeClusterSubnetGroupsRequest Container for the necessary
* parameters to execute the DescribeClusterSubnetGroups operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeClusterSubnetGroups service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeClusterSubnetGroupsAsync(DescribeClusterSubnetGroupsRequest describeClusterSubnetGroupsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns information about the specified Amazon Redshift HSM
* configuration. If no configuration ID is specified, returns
* information about all the HSM configurations owned by your AWS
* customer account.
*
*
* @param describeHsmConfigurationsRequest Container for the necessary
* parameters to execute the DescribeHsmConfigurations operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* DescribeHsmConfigurations service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeHsmConfigurationsAsync(DescribeHsmConfigurationsRequest describeHsmConfigurationsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns information about the specified Amazon Redshift HSM
* configuration. If no configuration ID is specified, returns
* information about all the HSM configurations owned by your AWS
* customer account.
*
*
* @param describeHsmConfigurationsRequest Container for the necessary
* parameters to execute the DescribeHsmConfigurations operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeHsmConfigurations service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeHsmConfigurationsAsync(DescribeHsmConfigurationsRequest describeHsmConfigurationsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes the specified manual snapshot. The snapshot must be in the
* "available" state, with no other users authorized to access the
* snapshot.
*
*
* Unlike automated snapshots, manual snapshots are retained even after
* you delete your cluster. Amazon Redshift does not delete your manual
* snapshots. You must delete manual snapshot explicitly to avoid getting
* charged. If other accounts are authorized to access the snapshot, you
* must revoke all of the authorizations before you can delete the
* snapshot.
*
*
* @param deleteClusterSnapshotRequest Container for the necessary
* parameters to execute the DeleteClusterSnapshot operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* DeleteClusterSnapshot service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteClusterSnapshotAsync(DeleteClusterSnapshotRequest deleteClusterSnapshotRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes the specified manual snapshot. The snapshot must be in the
* "available" state, with no other users authorized to access the
* snapshot.
*
*
* Unlike automated snapshots, manual snapshots are retained even after
* you delete your cluster. Amazon Redshift does not delete your manual
* snapshots. You must delete manual snapshot explicitly to avoid getting
* charged. If other accounts are authorized to access the snapshot, you
* must revoke all of the authorizations before you can delete the
* snapshot.
*
*
* @param deleteClusterSnapshotRequest Container for the necessary
* parameters to execute the DeleteClusterSnapshot operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteClusterSnapshot service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteClusterSnapshotAsync(DeleteClusterSnapshotRequest deleteClusterSnapshotRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes a specified Amazon Redshift parameter group.
* NOTE:You cannot delete a parameter group if it is associated
* with a cluster.
*
*
*
*
* @param deleteClusterParameterGroupRequest Container for the necessary
* parameters to execute the DeleteClusterParameterGroup operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* DeleteClusterParameterGroup service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteClusterParameterGroupAsync(DeleteClusterParameterGroupRequest deleteClusterParameterGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes a specified Amazon Redshift parameter group.
* NOTE:You cannot delete a parameter group if it is associated
* with a cluster.
*
*
*
*
* @param deleteClusterParameterGroupRequest Container for the necessary
* parameters to execute the DeleteClusterParameterGroup operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteClusterParameterGroup service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteClusterParameterGroupAsync(DeleteClusterParameterGroupRequest deleteClusterParameterGroupRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Disables the automatic copying of snapshots from one region to another
* region for a specified cluster.
*
*
* @param disableSnapshotCopyRequest Container for the necessary
* parameters to execute the DisableSnapshotCopy operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* DisableSnapshotCopy service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future disableSnapshotCopyAsync(DisableSnapshotCopyRequest disableSnapshotCopyRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Disables the automatic copying of snapshots from one region to another
* region for a specified cluster.
*
*
* @param disableSnapshotCopyRequest Container for the necessary
* parameters to execute the DisableSnapshotCopy operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DisableSnapshotCopy service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future disableSnapshotCopyAsync(DisableSnapshotCopyRequest disableSnapshotCopyRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates an Amazon Redshift event notification subscription. This
* action requires an ARN (Amazon Resource Name) of an Amazon SNS topic
* created by either the Amazon Redshift console, the Amazon SNS console,
* or the Amazon SNS API. To obtain an ARN with Amazon SNS, you must
* create a topic in Amazon SNS and subscribe to the topic. The ARN is
* displayed in the SNS console.
*
*
* You can specify the source type, and lists of Amazon Redshift source
* IDs, event categories, and event severities. Notifications will be
* sent for all events you want that match those criteria. For example,
* you can specify source type = cluster, source ID = my-cluster-1 and
* mycluster2, event categories = Availability, Backup, and severity =
* ERROR. The subscription will only send notifications for those ERROR
* events in the Availability and Backup categories for the specified
* clusters.
*
*
* If you specify both the source type and source IDs, such as source
* type = cluster and source identifier = my-cluster-1, notifications
* will be sent for all the cluster events for my-cluster-1. If you
* specify a source type but do not specify a source identifier, you will
* receive notice of the events for the objects of that type in your AWS
* account. If you do not specify either the SourceType nor the
* SourceIdentifier, you will be notified of events generated from all
* Amazon Redshift sources belonging to your AWS account. You must
* specify a source type if you specify a source ID.
*
*
* @param createEventSubscriptionRequest Container for the necessary
* parameters to execute the CreateEventSubscription operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* CreateEventSubscription service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createEventSubscriptionAsync(CreateEventSubscriptionRequest createEventSubscriptionRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates an Amazon Redshift event notification subscription. This
* action requires an ARN (Amazon Resource Name) of an Amazon SNS topic
* created by either the Amazon Redshift console, the Amazon SNS console,
* or the Amazon SNS API. To obtain an ARN with Amazon SNS, you must
* create a topic in Amazon SNS and subscribe to the topic. The ARN is
* displayed in the SNS console.
*
*
* You can specify the source type, and lists of Amazon Redshift source
* IDs, event categories, and event severities. Notifications will be
* sent for all events you want that match those criteria. For example,
* you can specify source type = cluster, source ID = my-cluster-1 and
* mycluster2, event categories = Availability, Backup, and severity =
* ERROR. The subscription will only send notifications for those ERROR
* events in the Availability and Backup categories for the specified
* clusters.
*
*
* If you specify both the source type and source IDs, such as source
* type = cluster and source identifier = my-cluster-1, notifications
* will be sent for all the cluster events for my-cluster-1. If you
* specify a source type but do not specify a source identifier, you will
* receive notice of the events for the objects of that type in your AWS
* account. If you do not specify either the SourceType nor the
* SourceIdentifier, you will be notified of events generated from all
* Amazon Redshift sources belonging to your AWS account. You must
* specify a source type if you specify a source ID.
*
*
* @param createEventSubscriptionRequest Container for the necessary
* parameters to execute the CreateEventSubscription operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateEventSubscription service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createEventSubscriptionAsync(CreateEventSubscriptionRequest createEventSubscriptionRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Sets one or more parameters of the specified parameter group to their
* default values and sets the source values of the parameters to
* "engine-default". To reset the entire parameter group specify the
* ResetAllParameters parameter. For parameter changes to take
* effect you must reboot any associated clusters.
*
*
* @param resetClusterParameterGroupRequest Container for the necessary
* parameters to execute the ResetClusterParameterGroup operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* ResetClusterParameterGroup service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future resetClusterParameterGroupAsync(ResetClusterParameterGroupRequest resetClusterParameterGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Sets one or more parameters of the specified parameter group to their
* default values and sets the source values of the parameters to
* "engine-default". To reset the entire parameter group specify the
* ResetAllParameters parameter. For parameter changes to take
* effect you must reboot any associated clusters.
*
*
* @param resetClusterParameterGroupRequest Container for the necessary
* parameters to execute the ResetClusterParameterGroup operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ResetClusterParameterGroup service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future resetClusterParameterGroupAsync(ResetClusterParameterGroupRequest resetClusterParameterGroupRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Authorizes the specified AWS customer account to restore the
* specified snapshot.
*
*
* For more information about working with snapshots, go to
* Amazon Redshift Snapshots
* in the Amazon Redshift Management Guide .
*
*
* @param authorizeSnapshotAccessRequest Container for the necessary
* parameters to execute the AuthorizeSnapshotAccess operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* AuthorizeSnapshotAccess service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future authorizeSnapshotAccessAsync(AuthorizeSnapshotAccessRequest authorizeSnapshotAccessRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Authorizes the specified AWS customer account to restore the
* specified snapshot.
*
*
* For more information about working with snapshots, go to
* Amazon Redshift Snapshots
* in the Amazon Redshift Management Guide .
*
*
* @param authorizeSnapshotAccessRequest Container for the necessary
* parameters to execute the AuthorizeSnapshotAccess operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* AuthorizeSnapshotAccess service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future authorizeSnapshotAccessAsync(AuthorizeSnapshotAccessRequest authorizeSnapshotAccessRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Enables the automatic copy of snapshots from one region to another
* region for a specified cluster.
*
*
* @param enableSnapshotCopyRequest Container for the necessary
* parameters to execute the EnableSnapshotCopy operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* EnableSnapshotCopy service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future enableSnapshotCopyAsync(EnableSnapshotCopyRequest enableSnapshotCopyRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Enables the automatic copy of snapshots from one region to another
* region for a specified cluster.
*
*
* @param enableSnapshotCopyRequest Container for the necessary
* parameters to execute the EnableSnapshotCopy operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* EnableSnapshotCopy service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future enableSnapshotCopyAsync(EnableSnapshotCopyRequest enableSnapshotCopyRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a list of the available reserved node offerings by Amazon
* Redshift with their descriptions including the node type, the fixed
* and recurring costs of reserving the node and duration the node will
* be reserved for you. These descriptions help you determine which
* reserve node offering you want to purchase. You then use the unique
* offering ID in you call to PurchaseReservedNodeOffering to reserve one
* or more nodes for your Amazon Redshift cluster.
*
*
* For more information about managing parameter groups, go to
* Purchasing Reserved Nodes
* in the Amazon Redshift Management Guide .
*
*
*
*
* @param describeReservedNodeOfferingsRequest Container for the
* necessary parameters to execute the DescribeReservedNodeOfferings
* operation on AmazonRedshift.
*
* @return A Java Future object containing the response from the
* DescribeReservedNodeOfferings service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeReservedNodeOfferingsAsync(DescribeReservedNodeOfferingsRequest describeReservedNodeOfferingsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a list of the available reserved node offerings by Amazon
* Redshift with their descriptions including the node type, the fixed
* and recurring costs of reserving the node and duration the node will
* be reserved for you. These descriptions help you determine which
* reserve node offering you want to purchase. You then use the unique
* offering ID in you call to PurchaseReservedNodeOffering to reserve one
* or more nodes for your Amazon Redshift cluster.
*
*
* For more information about managing parameter groups, go to
* Purchasing Reserved Nodes
* in the Amazon Redshift Management Guide .
*
*
*
*
* @param describeReservedNodeOfferingsRequest Container for the
* necessary parameters to execute the DescribeReservedNodeOfferings
* operation on AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeReservedNodeOfferings service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeReservedNodeOfferingsAsync(DescribeReservedNodeOfferingsRequest describeReservedNodeOfferingsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Lists descriptions of all the Amazon Redshift event notifications
* subscription for a customer account. If you specify a subscription
* name, lists the description for that subscription.
*
*
* @param describeEventSubscriptionsRequest Container for the necessary
* parameters to execute the DescribeEventSubscriptions operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* DescribeEventSubscriptions service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeEventSubscriptionsAsync(DescribeEventSubscriptionsRequest describeEventSubscriptionsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Lists descriptions of all the Amazon Redshift event notifications
* subscription for a customer account. If you specify a subscription
* name, lists the description for that subscription.
*
*
* @param describeEventSubscriptionsRequest Container for the necessary
* parameters to execute the DescribeEventSubscriptions operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeEventSubscriptions service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeEventSubscriptionsAsync(DescribeEventSubscriptionsRequest describeEventSubscriptionsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Describes whether information, such as queries and connection
* attempts, is being logged for the specified Amazon Redshift cluster.
*
*
* @param describeLoggingStatusRequest Container for the necessary
* parameters to execute the DescribeLoggingStatus operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* DescribeLoggingStatus service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeLoggingStatusAsync(DescribeLoggingStatusRequest describeLoggingStatusRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Describes whether information, such as queries and connection
* attempts, is being logged for the specified Amazon Redshift cluster.
*
*
* @param describeLoggingStatusRequest Container for the necessary
* parameters to execute the DescribeLoggingStatus operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeLoggingStatus service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeLoggingStatusAsync(DescribeLoggingStatusRequest describeLoggingStatusRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes an Amazon Redshift event notification subscription.
*
*
* @param deleteEventSubscriptionRequest Container for the necessary
* parameters to execute the DeleteEventSubscription operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* DeleteEventSubscription service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteEventSubscriptionAsync(DeleteEventSubscriptionRequest deleteEventSubscriptionRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes an Amazon Redshift event notification subscription.
*
*
* @param deleteEventSubscriptionRequest Container for the necessary
* parameters to execute the DeleteEventSubscription operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteEventSubscription service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteEventSubscriptionAsync(DeleteEventSubscriptionRequest deleteEventSubscriptionRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates an HSM client certificate that an Amazon Redshift cluster will
* use to connect to the client's HSM in order to store and retrieve the
* keys used to encrypt the cluster databases.
*
*
* The command returns a public key, which you must store in the HSM. In
* addition to creating the HSM certificate, you must create an Amazon
* Redshift HSM configuration that provides a cluster the information
* needed to store and use encryption keys in the HSM. For more
* information, go to
* Hardware Security Modules
* in the Amazon Redshift Management Guide.
*
*
* @param createHsmClientCertificateRequest Container for the necessary
* parameters to execute the CreateHsmClientCertificate operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* CreateHsmClientCertificate service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createHsmClientCertificateAsync(CreateHsmClientCertificateRequest createHsmClientCertificateRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates an HSM client certificate that an Amazon Redshift cluster will
* use to connect to the client's HSM in order to store and retrieve the
* keys used to encrypt the cluster databases.
*
*
* The command returns a public key, which you must store in the HSM. In
* addition to creating the HSM certificate, you must create an Amazon
* Redshift HSM configuration that provides a cluster the information
* needed to store and use encryption keys in the HSM. For more
* information, go to
* Hardware Security Modules
* in the Amazon Redshift Management Guide.
*
*
* @param createHsmClientCertificateRequest Container for the necessary
* parameters to execute the CreateHsmClientCertificate operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateHsmClientCertificate service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createHsmClientCertificateAsync(CreateHsmClientCertificateRequest createHsmClientCertificateRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Revokes an ingress rule in an Amazon Redshift security group for a
* previously authorized IP range or Amazon EC2 security group. To add an
* ingress rule, see AuthorizeClusterSecurityGroupIngress. For
* information about managing security groups, go to
* Amazon Redshift Cluster Security Groups
* in the Amazon Redshift Management Guide .
*
*
*
* @param revokeClusterSecurityGroupIngressRequest Container for the
* necessary parameters to execute the RevokeClusterSecurityGroupIngress
* operation on AmazonRedshift.
*
* @return A Java Future object containing the response from the
* RevokeClusterSecurityGroupIngress service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future revokeClusterSecurityGroupIngressAsync(RevokeClusterSecurityGroupIngressRequest revokeClusterSecurityGroupIngressRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Revokes an ingress rule in an Amazon Redshift security group for a
* previously authorized IP range or Amazon EC2 security group. To add an
* ingress rule, see AuthorizeClusterSecurityGroupIngress. For
* information about managing security groups, go to
* Amazon Redshift Cluster Security Groups
* in the Amazon Redshift Management Guide .
*
*
*
* @param revokeClusterSecurityGroupIngressRequest Container for the
* necessary parameters to execute the RevokeClusterSecurityGroupIngress
* operation on AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* RevokeClusterSecurityGroupIngress service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future revokeClusterSecurityGroupIngressAsync(RevokeClusterSecurityGroupIngressRequest revokeClusterSecurityGroupIngressRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a detailed list of parameters contained within the specified
* Amazon Redshift parameter group. For each parameter the response
* includes information such as parameter name, description, data type,
* value, whether the parameter value is modifiable, and so on.
*
*
* You can specify source filter to retrieve parameters of only
* specific type. For example, to retrieve parameters that were modified
* by a user action such as from ModifyClusterParameterGroup, you can
* specify source equal to user .
*
*
* For more information about managing parameter groups, go to
* Amazon Redshift Parameter Groups
* in the Amazon Redshift Management Guide .
*
*
* @param describeClusterParametersRequest Container for the necessary
* parameters to execute the DescribeClusterParameters operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* DescribeClusterParameters service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeClusterParametersAsync(DescribeClusterParametersRequest describeClusterParametersRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a detailed list of parameters contained within the specified
* Amazon Redshift parameter group. For each parameter the response
* includes information such as parameter name, description, data type,
* value, whether the parameter value is modifiable, and so on.
*
*
* You can specify source filter to retrieve parameters of only
* specific type. For example, to retrieve parameters that were modified
* by a user action such as from ModifyClusterParameterGroup, you can
* specify source equal to user .
*
*
* For more information about managing parameter groups, go to
* Amazon Redshift Parameter Groups
* in the Amazon Redshift Management Guide .
*
*
* @param describeClusterParametersRequest Container for the necessary
* parameters to execute the DescribeClusterParameters operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeClusterParameters service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeClusterParametersAsync(DescribeClusterParametersRequest describeClusterParametersRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns information about the specified HSM client certificate. If no
* certificate ID is specified, returns information about all the HSM
* certificates owned by your AWS customer account.
*
*
* @param describeHsmClientCertificatesRequest Container for the
* necessary parameters to execute the DescribeHsmClientCertificates
* operation on AmazonRedshift.
*
* @return A Java Future object containing the response from the
* DescribeHsmClientCertificates service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeHsmClientCertificatesAsync(DescribeHsmClientCertificatesRequest describeHsmClientCertificatesRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns information about the specified HSM client certificate. If no
* certificate ID is specified, returns information about all the HSM
* certificates owned by your AWS customer account.
*
*
* @param describeHsmClientCertificatesRequest Container for the
* necessary parameters to execute the DescribeHsmClientCertificates
* operation on AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeHsmClientCertificates service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeHsmClientCertificatesAsync(DescribeHsmClientCertificatesRequest describeHsmClientCertificatesRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates an HSM configuration that contains the information required by
* an Amazon Redshift cluster to store and use database encryption keys
* in a Hardware Security Module (HSM). After creating the HSM
* configuration, you can specify it as a parameter when creating a
* cluster. The cluster will then store its encryption keys in the HSM.
*
*
* In addition to creating an HSM configuration, you must also create an
* HSM client certificate. For more information, go to
* Hardware Security Modules
* in the Amazon Redshift Management Guide.
*
*
* @param createHsmConfigurationRequest Container for the necessary
* parameters to execute the CreateHsmConfiguration operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* CreateHsmConfiguration service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createHsmConfigurationAsync(CreateHsmConfigurationRequest createHsmConfigurationRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates an HSM configuration that contains the information required by
* an Amazon Redshift cluster to store and use database encryption keys
* in a Hardware Security Module (HSM). After creating the HSM
* configuration, you can specify it as a parameter when creating a
* cluster. The cluster will then store its encryption keys in the HSM.
*
*
* In addition to creating an HSM configuration, you must also create an
* HSM client certificate. For more information, go to
* Hardware Security Modules
* in the Amazon Redshift Management Guide.
*
*
* @param createHsmConfigurationRequest Container for the necessary
* parameters to execute the CreateHsmConfiguration operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateHsmConfiguration service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createHsmConfigurationAsync(CreateHsmConfigurationRequest createHsmConfigurationRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns properties of provisioned clusters including general cluster
* properties, cluster database properties, maintenance and backup
* properties, and security and access properties. This operation
* supports pagination. For more information about managing clusters, go
* to
* Amazon Redshift Clusters
* in the Amazon Redshift Management Guide .
*
*
*
* @param describeClustersRequest Container for the necessary parameters
* to execute the DescribeClusters operation on AmazonRedshift.
*
* @return A Java Future object containing the response from the
* DescribeClusters service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeClustersAsync(DescribeClustersRequest describeClustersRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns properties of provisioned clusters including general cluster
* properties, cluster database properties, maintenance and backup
* properties, and security and access properties. This operation
* supports pagination. For more information about managing clusters, go
* to
* Amazon Redshift Clusters
* in the Amazon Redshift Management Guide .
*
*
*
* @param describeClustersRequest Container for the necessary parameters
* to execute the DescribeClusters operation on AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeClusters service method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeClustersAsync(DescribeClustersRequest describeClustersRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns one or more snapshot objects, which contain metadata about
* your cluster snapshots. By default, this operation returns information
* about all snapshots of all clusters that are owned by you AWS customer
* account. No information is returned for snapshots owned by inactive
* AWS customer accounts.
*
*
* @param describeClusterSnapshotsRequest Container for the necessary
* parameters to execute the DescribeClusterSnapshots operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* DescribeClusterSnapshots service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeClusterSnapshotsAsync(DescribeClusterSnapshotsRequest describeClusterSnapshotsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns one or more snapshot objects, which contain metadata about
* your cluster snapshots. By default, this operation returns information
* about all snapshots of all clusters that are owned by you AWS customer
* account. No information is returned for snapshots owned by inactive
* AWS customer accounts.
*
*
* @param describeClusterSnapshotsRequest Container for the necessary
* parameters to execute the DescribeClusterSnapshots operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeClusterSnapshots service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeClusterSnapshotsAsync(DescribeClusterSnapshotsRequest describeClusterSnapshotsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates an Amazon Redshift parameter group.
*
*
* Creating parameter groups is independent of creating clusters. You can
* associate a cluster with a parameter group when you create the
* cluster. You can also associate an existing cluster with a parameter
* group after the cluster is created by using ModifyCluster.
*
*
* Parameters in the parameter group define specific behavior that
* applies to the databases you create on the cluster. For more
* information about managing parameter groups, go to
* Amazon Redshift Parameter Groups
* in the Amazon Redshift Management Guide .
*
*
*
* @param createClusterParameterGroupRequest Container for the necessary
* parameters to execute the CreateClusterParameterGroup operation on
* AmazonRedshift.
*
* @return A Java Future object containing the response from the
* CreateClusterParameterGroup service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createClusterParameterGroupAsync(CreateClusterParameterGroupRequest createClusterParameterGroupRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Creates an Amazon Redshift parameter group.
*
*
* Creating parameter groups is independent of creating clusters. You can
* associate a cluster with a parameter group when you create the
* cluster. You can also associate an existing cluster with a parameter
* group after the cluster is created by using ModifyCluster.
*
*
* Parameters in the parameter group define specific behavior that
* applies to the databases you create on the cluster. For more
* information about managing parameter groups, go to
* Amazon Redshift Parameter Groups
* in the Amazon Redshift Management Guide .
*
*
*
* @param createClusterParameterGroupRequest Container for the necessary
* parameters to execute the CreateClusterParameterGroup operation on
* AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateClusterParameterGroup service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createClusterParameterGroupAsync(CreateClusterParameterGroupRequest createClusterParameterGroupRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a list of Amazon Redshift parameter groups, including
* parameter groups you created and the default parameter group. For each
* parameter group, the response includes the parameter group name,
* description, and parameter group family name. You can optionally
* specify a name to retrieve the description of a specific parameter
* group.
*
*
* For more information about managing parameter groups, go to
* Amazon Redshift Parameter Groups
* in the Amazon Redshift Management Guide .
*
*
* @param describeClusterParameterGroupsRequest Container for the
* necessary parameters to execute the DescribeClusterParameterGroups
* operation on AmazonRedshift.
*
* @return A Java Future object containing the response from the
* DescribeClusterParameterGroups service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeClusterParameterGroupsAsync(DescribeClusterParameterGroupsRequest describeClusterParameterGroupsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a list of Amazon Redshift parameter groups, including
* parameter groups you created and the default parameter group. For each
* parameter group, the response includes the parameter group name,
* description, and parameter group family name. You can optionally
* specify a name to retrieve the description of a specific parameter
* group.
*
*
* For more information about managing parameter groups, go to
* Amazon Redshift Parameter Groups
* in the Amazon Redshift Management Guide .
*
*
* @param describeClusterParameterGroupsRequest Container for the
* necessary parameters to execute the DescribeClusterParameterGroups
* operation on AmazonRedshift.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeClusterParameterGroups service method, as returned by
* AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeClusterParameterGroupsAsync(DescribeClusterParameterGroupsRequest describeClusterParameterGroupsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
}