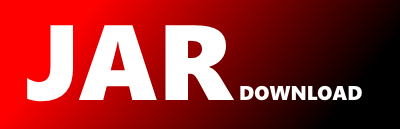
com.amazonaws.services.redshift.AmazonRedshiftClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.redshift;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import java.util.Map.Entry;
import com.amazonaws.*;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.services.redshift.model.*;
import com.amazonaws.services.redshift.model.transform.*;
/**
* Client for accessing AmazonRedshift. All service calls made
* using this client are blocking, and will not return until the service call
* completes.
*
* Amazon Redshift Overview
* This is an interface reference for Amazon Redshift. It contains
* documentation for one of the programming or command line interfaces
* you can use to manage Amazon Redshift clusters. Note that Amazon
* Redshift is asynchronous, which means that some interfaces may require
* techniques, such as polling or asynchronous callback handlers, to
* determine when a command has been applied. In this reference, the
* parameter descriptions indicate whether a change is applied
* immediately, on the next instance reboot, or during the next
* maintenance window. For a summary of the Amazon Redshift cluster
* management interfaces, go to
* Using the Amazon Redshift Management Interfaces
* .
*
*
* Amazon Redshift manages all the work of setting up, operating, and
* scaling a data warehouse: provisioning capacity, monitoring and
* backing up the cluster, and applying patches and upgrades to the
* Amazon Redshift engine. You can focus on using your data to acquire
* new insights for your business and customers.
*
*
* If you are a first-time user of Amazon Redshift, we recommend that you
* begin by reading the The
* Amazon Redshift Getting Started Guide
*
*
*
* If you are a database developer, the
* Amazon Redshift Database Developer Guide
* explains how to design, build, query, and maintain the databases that
* make up your data warehouse.
*
*/
public class AmazonRedshiftClient extends AmazonWebServiceClient implements AmazonRedshift {
/** Provider for AWS credentials. */
private AWSCredentialsProvider awsCredentialsProvider;
/**
* List of exception unmarshallers for all AmazonRedshift exceptions.
*/
protected final List> exceptionUnmarshallers
= new ArrayList>();
/**
* Constructs a new client to invoke service methods on
* AmazonRedshift. A credentials provider chain will be used
* that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @see DefaultAWSCredentialsProviderChain
*/
public AmazonRedshiftClient() {
this(new DefaultAWSCredentialsProviderChain(), new ClientConfiguration());
}
/**
* Constructs a new client to invoke service methods on
* AmazonRedshift. A credentials provider chain will be used
* that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to AmazonRedshift
* (ex: proxy settings, retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
*/
public AmazonRedshiftClient(ClientConfiguration clientConfiguration) {
this(new DefaultAWSCredentialsProviderChain(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on
* AmazonRedshift using the specified AWS account credentials.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentials The AWS credentials (access key ID and secret key) to use
* when authenticating with AWS services.
*/
public AmazonRedshiftClient(AWSCredentials awsCredentials) {
this(awsCredentials, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service methods on
* AmazonRedshift using the specified AWS account credentials
* and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentials The AWS credentials (access key ID and secret key) to use
* when authenticating with AWS services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to AmazonRedshift
* (ex: proxy settings, retry counts, etc.).
*/
public AmazonRedshiftClient(AWSCredentials awsCredentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.awsCredentialsProvider = new StaticCredentialsProvider(awsCredentials);
init();
}
/**
* Constructs a new client to invoke service methods on
* AmazonRedshift using the specified AWS account credentials provider.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
*/
public AmazonRedshiftClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service methods on
* AmazonRedshift using the specified AWS account credentials
* provider and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to AmazonRedshift
* (ex: proxy settings, retry counts, etc.).
*/
public AmazonRedshiftClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on
* AmazonRedshift using the specified AWS account credentials
* provider, client configuration options, and request metric collector.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to AmazonRedshift
* (ex: proxy settings, retry counts, etc.).
* @param requestMetricCollector optional request metric collector
*/
public AmazonRedshiftClient(AWSCredentialsProvider awsCredentialsProvider,
ClientConfiguration clientConfiguration,
RequestMetricCollector requestMetricCollector) {
super(clientConfiguration, requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
init();
}
private void init() {
exceptionUnmarshallers.add(new ReservedNodeAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new AuthorizationAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidHsmConfigurationStateExceptionUnmarshaller());
exceptionUnmarshallers.add(new NumberOfNodesQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new ClusterNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new SubscriptionNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new HsmClientCertificateQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new SnapshotCopyDisabledExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidRestoreExceptionUnmarshaller());
exceptionUnmarshallers.add(new ClusterSnapshotNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new SNSInvalidTopicExceptionUnmarshaller());
exceptionUnmarshallers.add(new HsmClientCertificateAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new SNSNoAuthorizationExceptionUnmarshaller());
exceptionUnmarshallers.add(new ClusterSecurityGroupAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new ReservedNodeQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new HsmConfigurationAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new EventSubscriptionQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new ReservedNodeOfferingNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new ClusterSecurityGroupQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new SubnetAlreadyInUseExceptionUnmarshaller());
exceptionUnmarshallers.add(new HsmConfigurationQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new ReservedNodeNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new ClusterSnapshotAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new SnapshotCopyAlreadyEnabledExceptionUnmarshaller());
exceptionUnmarshallers.add(new CopyToRegionDisabledExceptionUnmarshaller());
exceptionUnmarshallers.add(new NumberOfNodesPerClusterLimitExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new HsmClientCertificateNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new SubscriptionCategoryNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidClusterStateExceptionUnmarshaller());
exceptionUnmarshallers.add(new ClusterParameterGroupAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidClusterParameterGroupStateExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidHsmClientCertificateStateExceptionUnmarshaller());
exceptionUnmarshallers.add(new ClusterSecurityGroupNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new ResizeNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new SnapshotCopyAlreadyDisabledExceptionUnmarshaller());
exceptionUnmarshallers.add(new ClusterParameterGroupQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new ClusterSubnetQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new SubscriptionSeverityNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new SubscriptionAlreadyExistExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidClusterSubnetStateExceptionUnmarshaller());
exceptionUnmarshallers.add(new AuthorizationNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new ClusterAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new AccessToSnapshotDeniedExceptionUnmarshaller());
exceptionUnmarshallers.add(new UnknownSnapshotCopyRegionExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidS3BucketNameExceptionUnmarshaller());
exceptionUnmarshallers.add(new ClusterSnapshotQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new IncompatibleOrderableOptionsExceptionUnmarshaller());
exceptionUnmarshallers.add(new InsufficientS3BucketPolicyExceptionUnmarshaller());
exceptionUnmarshallers.add(new InsufficientClusterCapacityExceptionUnmarshaller());
exceptionUnmarshallers.add(new ClusterQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidSubnetExceptionUnmarshaller());
exceptionUnmarshallers.add(new HsmConfigurationNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new BucketNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new ClusterSubnetGroupQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidVPCNetworkStateExceptionUnmarshaller());
exceptionUnmarshallers.add(new ClusterParameterGroupNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidClusterSubnetGroupStateExceptionUnmarshaller());
exceptionUnmarshallers.add(new AuthorizationQuotaExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new SNSTopicArnNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new UnsupportedOptionExceptionUnmarshaller());
exceptionUnmarshallers.add(new UnauthorizedOperationExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidElasticIpExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidClusterSnapshotStateExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidS3KeyPrefixExceptionUnmarshaller());
exceptionUnmarshallers.add(new SourceNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidClusterSecurityGroupStateExceptionUnmarshaller());
exceptionUnmarshallers.add(new SubscriptionEventIdNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new ClusterSubnetGroupNotFoundExceptionUnmarshaller());
exceptionUnmarshallers.add(new ClusterSubnetGroupAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new StandardErrorUnmarshaller());
// calling this.setEndPoint(...) will also modify the signer accordingly
this.setEndpoint("redshift.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain(
"/com/amazonaws/services/redshift/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain(
"/com/amazonaws/services/redshift/request.handler2s"));
}
/**
*
* Removes the ability of the specified AWS customer account to restore
* the specified snapshot. If the account is currently restoring the
* snapshot, the restore will run to completion.
*
*
* For more information about working with snapshots, go to
* Amazon Redshift Snapshots
* in the Amazon Redshift Management Guide .
*
*
* @param revokeSnapshotAccessRequest Container for the necessary
* parameters to execute the RevokeSnapshotAccess service method on
* AmazonRedshift.
*
* @return The response from the RevokeSnapshotAccess service method, as
* returned by AmazonRedshift.
*
* @throws AccessToSnapshotDeniedException
* @throws ClusterSnapshotNotFoundException
* @throws AuthorizationNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Snapshot revokeSnapshotAccess(RevokeSnapshotAccessRequest revokeSnapshotAccessRequest) {
ExecutionContext executionContext = createExecutionContext(revokeSnapshotAccessRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new RevokeSnapshotAccessRequestMarshaller().marshall(revokeSnapshotAccessRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new SnapshotStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Modifies the number of days to retain automated snapshots in the
* destination region after they are copied from the source region.
*
*
* @param modifySnapshotCopyRetentionPeriodRequest Container for the
* necessary parameters to execute the ModifySnapshotCopyRetentionPeriod
* service method on AmazonRedshift.
*
* @return The response from the ModifySnapshotCopyRetentionPeriod
* service method, as returned by AmazonRedshift.
*
* @throws SnapshotCopyDisabledException
* @throws UnauthorizedOperationException
* @throws InvalidClusterStateException
* @throws ClusterNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Cluster modifySnapshotCopyRetentionPeriod(ModifySnapshotCopyRetentionPeriodRequest modifySnapshotCopyRetentionPeriodRequest) {
ExecutionContext executionContext = createExecutionContext(modifySnapshotCopyRetentionPeriodRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ModifySnapshotCopyRetentionPeriodRequestMarshaller().marshall(modifySnapshotCopyRetentionPeriodRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ClusterStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Modifies a cluster subnet group to include the specified list of VPC
* subnets. The operation replaces the existing list of subnets with the
* new list of subnets.
*
*
* @param modifyClusterSubnetGroupRequest Container for the necessary
* parameters to execute the ModifyClusterSubnetGroup service method on
* AmazonRedshift.
*
* @return The response from the ModifyClusterSubnetGroup service method,
* as returned by AmazonRedshift.
*
* @throws SubnetAlreadyInUseException
* @throws InvalidSubnetException
* @throws UnauthorizedOperationException
* @throws ClusterSubnetQuotaExceededException
* @throws ClusterSubnetGroupNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public ClusterSubnetGroup modifyClusterSubnetGroup(ModifyClusterSubnetGroupRequest modifyClusterSubnetGroupRequest) {
ExecutionContext executionContext = createExecutionContext(modifyClusterSubnetGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ModifyClusterSubnetGroupRequestMarshaller().marshall(modifyClusterSubnetGroupRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ClusterSubnetGroupStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified Amazon Redshift HSM configuration.
*
*
* @param deleteHsmConfigurationRequest Container for the necessary
* parameters to execute the DeleteHsmConfiguration service method on
* AmazonRedshift.
*
*
* @throws HsmConfigurationNotFoundException
* @throws InvalidHsmConfigurationStateException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteHsmConfiguration(DeleteHsmConfigurationRequest deleteHsmConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteHsmConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteHsmConfigurationRequestMarshaller().marshall(deleteHsmConfigurationRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Allows you to purchase reserved nodes. Amazon Redshift offers a
* predefined set of reserved node offerings. You can purchase one of the
* offerings. You can call the DescribeReservedNodeOfferings API to
* obtain the available reserved node offerings. You can call this API by
* providing a specific reserved node offering and the number of nodes
* you want to reserve.
*
*
* For more information about managing parameter groups, go to
* Purchasing Reserved Nodes
* in the Amazon Redshift Management Guide .
*
*
*
*
* @param purchaseReservedNodeOfferingRequest Container for the necessary
* parameters to execute the PurchaseReservedNodeOffering service method
* on AmazonRedshift.
*
* @return The response from the PurchaseReservedNodeOffering service
* method, as returned by AmazonRedshift.
*
* @throws ReservedNodeAlreadyExistsException
* @throws ReservedNodeOfferingNotFoundException
* @throws ReservedNodeQuotaExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public ReservedNode purchaseReservedNodeOffering(PurchaseReservedNodeOfferingRequest purchaseReservedNodeOfferingRequest) {
ExecutionContext executionContext = createExecutionContext(purchaseReservedNodeOfferingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new PurchaseReservedNodeOfferingRequestMarshaller().marshall(purchaseReservedNodeOfferingRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ReservedNodeStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Stops logging information, such as queries and connection attempts,
* for the specified Amazon Redshift cluster.
*
*
* @param disableLoggingRequest Container for the necessary parameters to
* execute the DisableLogging service method on AmazonRedshift.
*
* @return The response from the DisableLogging service method, as
* returned by AmazonRedshift.
*
* @throws ClusterNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DisableLoggingResult disableLogging(DisableLoggingRequest disableLoggingRequest) {
ExecutionContext executionContext = createExecutionContext(disableLoggingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DisableLoggingRequestMarshaller().marshall(disableLoggingRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DisableLoggingResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Modifies the settings for a cluster. For example, you can add another
* security or parameter group, update the preferred maintenance window,
* or change the master user password. Resetting a cluster password or
* modifying the security groups associated with a cluster do not need a
* reboot. However, modifying parameter group requires a reboot for
* parameters to take effect. For more information about managing
* clusters, go to
* Amazon Redshift Clusters
* in the Amazon Redshift Management Guide
*
*
* You can also change node type and the number of nodes to scale up or
* down the cluster. When resizing a cluster, you must specify both the
* number of nodes and the node type even if one of the parameters does
* not change. If you specify the same number of nodes and node type that
* are already configured for the cluster, an error is returned.
*
*
* @param modifyClusterRequest Container for the necessary parameters to
* execute the ModifyCluster service method on AmazonRedshift.
*
* @return The response from the ModifyCluster service method, as
* returned by AmazonRedshift.
*
* @throws InvalidClusterSecurityGroupStateException
* @throws HsmConfigurationNotFoundException
* @throws InsufficientClusterCapacityException
* @throws UnauthorizedOperationException
* @throws InvalidClusterStateException
* @throws NumberOfNodesQuotaExceededException
* @throws ClusterNotFoundException
* @throws UnsupportedOptionException
* @throws ClusterSecurityGroupNotFoundException
* @throws HsmClientCertificateNotFoundException
* @throws ClusterParameterGroupNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Cluster modifyCluster(ModifyClusterRequest modifyClusterRequest) {
ExecutionContext executionContext = createExecutionContext(modifyClusterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ModifyClusterRequestMarshaller().marshall(modifyClusterRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ClusterStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Copies the specified automated cluster snapshot to a new manual
* cluster snapshot. The source must be an automated snapshot and it must
* be in the available state.
*
*
* When you delete a cluster, Amazon Redshift deletes any automated
* snapshots of the cluster. Also, when the retention period of the
* snapshot expires, Amazon Redshift automatically deletes it. If you
* want to keep an automated snapshot for a longer period, you can make a
* manual copy of the snapshot. Manual snapshots are retained until you
* delete them.
*
*
* For more information about working with snapshots, go to
* Amazon Redshift Snapshots
* in the Amazon Redshift Management Guide .
*
*
* @param copyClusterSnapshotRequest Container for the necessary
* parameters to execute the CopyClusterSnapshot service method on
* AmazonRedshift.
*
* @return The response from the CopyClusterSnapshot service method, as
* returned by AmazonRedshift.
*
* @throws ClusterSnapshotAlreadyExistsException
* @throws ClusterSnapshotNotFoundException
* @throws ClusterSnapshotQuotaExceededException
* @throws InvalidClusterSnapshotStateException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Snapshot copyClusterSnapshot(CopyClusterSnapshotRequest copyClusterSnapshotRequest) {
ExecutionContext executionContext = createExecutionContext(copyClusterSnapshotRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CopyClusterSnapshotRequestMarshaller().marshall(copyClusterSnapshotRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new SnapshotStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of orderable cluster options. Before you create a new
* cluster you can use this operation to find what options are available,
* such as the EC2 Availability Zones (AZ) in the specific AWS region
* that you can specify, and the node types you can request. The node
* types differ by available storage, memory, CPU and price. With the
* cost involved you might want to obtain a list of cluster options in
* the specific region and specify values when creating a cluster. For
* more information about managing clusters, go to
* Amazon Redshift Clusters
* in the Amazon Redshift Management Guide
*
*
* @param describeOrderableClusterOptionsRequest Container for the
* necessary parameters to execute the DescribeOrderableClusterOptions
* service method on AmazonRedshift.
*
* @return The response from the DescribeOrderableClusterOptions service
* method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeOrderableClusterOptionsResult describeOrderableClusterOptions(DescribeOrderableClusterOptionsRequest describeOrderableClusterOptionsRequest) {
ExecutionContext executionContext = createExecutionContext(describeOrderableClusterOptionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeOrderableClusterOptionsRequestMarshaller().marshall(describeOrderableClusterOptionsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeOrderableClusterOptionsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Starts logging information, such as queries and connection attempts,
* for the specified Amazon Redshift cluster.
*
*
* @param enableLoggingRequest Container for the necessary parameters to
* execute the EnableLogging service method on AmazonRedshift.
*
* @return The response from the EnableLogging service method, as
* returned by AmazonRedshift.
*
* @throws InvalidS3KeyPrefixException
* @throws InvalidS3BucketNameException
* @throws BucketNotFoundException
* @throws ClusterNotFoundException
* @throws InsufficientS3BucketPolicyException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public EnableLoggingResult enableLogging(EnableLoggingRequest enableLoggingRequest) {
ExecutionContext executionContext = createExecutionContext(enableLoggingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new EnableLoggingRequestMarshaller().marshall(enableLoggingRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new EnableLoggingResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new Amazon Redshift subnet group. You must provide a list
* of one or more subnets in your existing Amazon Virtual Private Cloud
* (Amazon VPC) when creating Amazon Redshift subnet group.
*
*
* For information about subnet groups, go to
* Amazon Redshift Cluster Subnet Groups
* in the Amazon Redshift Management Guide .
*
*
*
* @param createClusterSubnetGroupRequest Container for the necessary
* parameters to execute the CreateClusterSubnetGroup service method on
* AmazonRedshift.
*
* @return The response from the CreateClusterSubnetGroup service method,
* as returned by AmazonRedshift.
*
* @throws InvalidSubnetException
* @throws UnauthorizedOperationException
* @throws ClusterSubnetQuotaExceededException
* @throws ClusterSubnetGroupAlreadyExistsException
* @throws ClusterSubnetGroupQuotaExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public ClusterSubnetGroup createClusterSubnetGroup(CreateClusterSubnetGroupRequest createClusterSubnetGroupRequest) {
ExecutionContext executionContext = createExecutionContext(createClusterSubnetGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateClusterSubnetGroupRequestMarshaller().marshall(createClusterSubnetGroupRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ClusterSubnetGroupStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified HSM client certificate.
*
*
* @param deleteHsmClientCertificateRequest Container for the necessary
* parameters to execute the DeleteHsmClientCertificate service method on
* AmazonRedshift.
*
*
* @throws InvalidHsmClientCertificateStateException
* @throws HsmClientCertificateNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteHsmClientCertificate(DeleteHsmClientCertificateRequest deleteHsmClientCertificateRequest) {
ExecutionContext executionContext = createExecutionContext(deleteHsmClientCertificateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteHsmClientCertificateRequestMarshaller().marshall(deleteHsmClientCertificateRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Reboots a cluster. This action is taken as soon as possible. It
* results in a momentary outage to the cluster, during which the cluster
* status is set to rebooting
. A cluster event is created
* when the reboot is completed. Any pending cluster modifications (see
* ModifyCluster) are applied at this reboot. For more information about
* managing clusters, go to
* Amazon Redshift Clusters
* in the Amazon Redshift Management Guide
*
*
* @param rebootClusterRequest Container for the necessary parameters to
* execute the RebootCluster service method on AmazonRedshift.
*
* @return The response from the RebootCluster service method, as
* returned by AmazonRedshift.
*
* @throws InvalidClusterStateException
* @throws ClusterNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Cluster rebootCluster(RebootClusterRequest rebootClusterRequest) {
ExecutionContext executionContext = createExecutionContext(rebootClusterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new RebootClusterRequestMarshaller().marshall(rebootClusterRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ClusterStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified cluster subnet group.
*
*
* @param deleteClusterSubnetGroupRequest Container for the necessary
* parameters to execute the DeleteClusterSubnetGroup service method on
* AmazonRedshift.
*
*
* @throws InvalidClusterSubnetStateException
* @throws ClusterSubnetGroupNotFoundException
* @throws InvalidClusterSubnetGroupStateException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteClusterSubnetGroup(DeleteClusterSubnetGroupRequest deleteClusterSubnetGroupRequest) {
ExecutionContext executionContext = createExecutionContext(deleteClusterSubnetGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteClusterSubnetGroupRequestMarshaller().marshall(deleteClusterSubnetGroupRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Deletes a previously provisioned cluster. A successful response from
* the web service indicates that the request was received correctly. If
* a final cluster snapshot is requested the status of the cluster will
* be "final-snapshot" while the snapshot is being taken, then it's
* "deleting" once Amazon Redshift begins deleting the cluster. Use
* DescribeClusters to monitor the status of the deletion. The delete
* operation cannot be canceled or reverted once submitted. For more
* information about managing clusters, go to
* Amazon Redshift Clusters
* in the Amazon Redshift Management Guide .
*
*
*
* @param deleteClusterRequest Container for the necessary parameters to
* execute the DeleteCluster service method on AmazonRedshift.
*
* @return The response from the DeleteCluster service method, as
* returned by AmazonRedshift.
*
* @throws ClusterSnapshotAlreadyExistsException
* @throws InvalidClusterStateException
* @throws ClusterNotFoundException
* @throws ClusterSnapshotQuotaExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Cluster deleteCluster(DeleteClusterRequest deleteClusterRequest) {
ExecutionContext executionContext = createExecutionContext(deleteClusterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteClusterRequestMarshaller().marshall(deleteClusterRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ClusterStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a manual snapshot of the specified cluster. The cluster must
* be in the "available" state.
*
*
* For more information about working with snapshots, go to
* Amazon Redshift Snapshots
* in the Amazon Redshift Management Guide .
*
*
* @param createClusterSnapshotRequest Container for the necessary
* parameters to execute the CreateClusterSnapshot service method on
* AmazonRedshift.
*
* @return The response from the CreateClusterSnapshot service method, as
* returned by AmazonRedshift.
*
* @throws ClusterSnapshotAlreadyExistsException
* @throws InvalidClusterStateException
* @throws ClusterNotFoundException
* @throws ClusterSnapshotQuotaExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Snapshot createClusterSnapshot(CreateClusterSnapshotRequest createClusterSnapshotRequest) {
ExecutionContext executionContext = createExecutionContext(createClusterSnapshotRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateClusterSnapshotRequestMarshaller().marshall(createClusterSnapshotRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new SnapshotStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new cluster. To create the cluster in virtual private cloud
* (VPC), you must provide cluster subnet group name. If you don't
* provide a cluster subnet group name or the cluster security group
* parameter, Amazon Redshift creates a non-VPC cluster, it associates
* the default cluster security group with the cluster. For more
* information about managing clusters, go to
* Amazon Redshift Clusters
* in the Amazon Redshift Management Guide .
*
*
*
* @param createClusterRequest Container for the necessary parameters to
* execute the CreateCluster service method on AmazonRedshift.
*
* @return The response from the CreateCluster service method, as
* returned by AmazonRedshift.
*
* @throws InvalidElasticIpException
* @throws InvalidSubnetException
* @throws HsmConfigurationNotFoundException
* @throws ClusterSubnetGroupNotFoundException
* @throws InvalidClusterSubnetGroupStateException
* @throws ClusterAlreadyExistsException
* @throws InvalidVPCNetworkStateException
* @throws ClusterParameterGroupNotFoundException
* @throws InsufficientClusterCapacityException
* @throws UnauthorizedOperationException
* @throws NumberOfNodesQuotaExceededException
* @throws NumberOfNodesPerClusterLimitExceededException
* @throws HsmClientCertificateNotFoundException
* @throws ClusterQuotaExceededException
* @throws ClusterSecurityGroupNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Cluster createCluster(CreateClusterRequest createClusterRequest) {
ExecutionContext executionContext = createExecutionContext(createClusterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateClusterRequestMarshaller().marshall(createClusterRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ClusterStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns events related to clusters, security groups, snapshots, and
* parameter groups for the past 14 days. Events specific to a particular
* cluster, security group, snapshot or parameter group can be obtained
* by providing the name as a parameter. By default, the past hour of
* events are returned.
*
*
* @param describeEventsRequest Container for the necessary parameters to
* execute the DescribeEvents service method on AmazonRedshift.
*
* @return The response from the DescribeEvents service method, as
* returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeEventsResult describeEvents(DescribeEventsRequest describeEventsRequest) {
ExecutionContext executionContext = createExecutionContext(describeEventsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeEventsRequestMarshaller().marshall(describeEventsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeEventsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds an inbound (ingress) rule to an Amazon Redshift security group.
* Depending on whether the application accessing your cluster is running
* on the Internet or an EC2 instance, you can authorize inbound access
* to either a Classless Interdomain Routing (CIDR) IP address range or
* an EC2 security group. You can add as many as 20 ingress rules to an
* Amazon Redshift security group.
*
*
* NOTE: The EC2 security group must be defined in the AWS region
* where the cluster resides.
*
*
* For an overview of CIDR blocks, see the Wikipedia article on
* Classless Inter-Domain Routing
* .
*
*
* You must also associate the security group with a cluster so that
* clients running on these IP addresses or the EC2 instance are
* authorized to connect to the cluster. For information about managing
* security groups, go to
* Working with Security Groups
* in the Amazon Redshift Management Guide .
*
*
* @param authorizeClusterSecurityGroupIngressRequest Container for the
* necessary parameters to execute the
* AuthorizeClusterSecurityGroupIngress service method on AmazonRedshift.
*
* @return The response from the AuthorizeClusterSecurityGroupIngress
* service method, as returned by AmazonRedshift.
*
* @throws InvalidClusterSecurityGroupStateException
* @throws AuthorizationAlreadyExistsException
* @throws AuthorizationQuotaExceededException
* @throws ClusterSecurityGroupNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public ClusterSecurityGroup authorizeClusterSecurityGroupIngress(AuthorizeClusterSecurityGroupIngressRequest authorizeClusterSecurityGroupIngressRequest) {
ExecutionContext executionContext = createExecutionContext(authorizeClusterSecurityGroupIngressRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new AuthorizeClusterSecurityGroupIngressRequestMarshaller().marshall(authorizeClusterSecurityGroupIngressRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ClusterSecurityGroupStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Rotates the encryption keys for a cluster.
*
*
* @param rotateEncryptionKeyRequest Container for the necessary
* parameters to execute the RotateEncryptionKey service method on
* AmazonRedshift.
*
* @return The response from the RotateEncryptionKey service method, as
* returned by AmazonRedshift.
*
* @throws InvalidClusterStateException
* @throws ClusterNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Cluster rotateEncryptionKey(RotateEncryptionKeyRequest rotateEncryptionKeyRequest) {
ExecutionContext executionContext = createExecutionContext(rotateEncryptionKeyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new RotateEncryptionKeyRequestMarshaller().marshall(rotateEncryptionKeyRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ClusterStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an Amazon Redshift security group.
*
*
* NOTE:You cannot delete a security group that is associated with
* any clusters. You cannot delete the default security group.
*
*
* For information about managing security groups, go to
* Amazon Redshift Cluster Security Groups
* in the Amazon Redshift Management Guide .
*
*
* @param deleteClusterSecurityGroupRequest Container for the necessary
* parameters to execute the DeleteClusterSecurityGroup service method on
* AmazonRedshift.
*
*
* @throws InvalidClusterSecurityGroupStateException
* @throws ClusterSecurityGroupNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteClusterSecurityGroup(DeleteClusterSecurityGroupRequest deleteClusterSecurityGroupRequest) {
ExecutionContext executionContext = createExecutionContext(deleteClusterSecurityGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteClusterSecurityGroupRequestMarshaller().marshall(deleteClusterSecurityGroupRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Returns the descriptions of the reserved nodes.
*
*
* @param describeReservedNodesRequest Container for the necessary
* parameters to execute the DescribeReservedNodes service method on
* AmazonRedshift.
*
* @return The response from the DescribeReservedNodes service method, as
* returned by AmazonRedshift.
*
* @throws ReservedNodeNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeReservedNodesResult describeReservedNodes(DescribeReservedNodesRequest describeReservedNodesRequest) {
ExecutionContext executionContext = createExecutionContext(describeReservedNodesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeReservedNodesRequestMarshaller().marshall(describeReservedNodesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeReservedNodesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of parameter settings for the specified parameter
* group family.
*
*
* For more information about managing parameter groups, go to
* Amazon Redshift Parameter Groups
* in the Amazon Redshift Management Guide .
*
*
* @param describeDefaultClusterParametersRequest Container for the
* necessary parameters to execute the DescribeDefaultClusterParameters
* service method on AmazonRedshift.
*
* @return The response from the DescribeDefaultClusterParameters service
* method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DefaultClusterParameters describeDefaultClusterParameters(DescribeDefaultClusterParametersRequest describeDefaultClusterParametersRequest) {
ExecutionContext executionContext = createExecutionContext(describeDefaultClusterParametersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeDefaultClusterParametersRequestMarshaller().marshall(describeDefaultClusterParametersRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DefaultClusterParametersStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Modifies an existing Amazon Redshift event notification subscription.
*
*
* @param modifyEventSubscriptionRequest Container for the necessary
* parameters to execute the ModifyEventSubscription service method on
* AmazonRedshift.
*
* @return The response from the ModifyEventSubscription service method,
* as returned by AmazonRedshift.
*
* @throws SubscriptionCategoryNotFoundException
* @throws SubscriptionEventIdNotFoundException
* @throws SubscriptionSeverityNotFoundException
* @throws SourceNotFoundException
* @throws SNSNoAuthorizationException
* @throws SNSTopicArnNotFoundException
* @throws SubscriptionNotFoundException
* @throws SNSInvalidTopicException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public EventSubscription modifyEventSubscription(ModifyEventSubscriptionRequest modifyEventSubscriptionRequest) {
ExecutionContext executionContext = createExecutionContext(modifyEventSubscriptionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ModifyEventSubscriptionRequestMarshaller().marshall(modifyEventSubscriptionRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new EventSubscriptionStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new Amazon Redshift security group. You use security groups
* to control access to non-VPC clusters.
*
*
* For information about managing security groups, go to
* Amazon Redshift Cluster Security Groups
* in the Amazon Redshift Management Guide .
*
*
*
* @param createClusterSecurityGroupRequest Container for the necessary
* parameters to execute the CreateClusterSecurityGroup service method on
* AmazonRedshift.
*
* @return The response from the CreateClusterSecurityGroup service
* method, as returned by AmazonRedshift.
*
* @throws ClusterSecurityGroupQuotaExceededException
* @throws ClusterSecurityGroupAlreadyExistsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public ClusterSecurityGroup createClusterSecurityGroup(CreateClusterSecurityGroupRequest createClusterSecurityGroupRequest) {
ExecutionContext executionContext = createExecutionContext(createClusterSecurityGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateClusterSecurityGroupRequestMarshaller().marshall(createClusterSecurityGroupRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ClusterSecurityGroupStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about the last resize operation for the specified
* cluster. If no resize operation has ever been initiated for the
* specified cluster, a HTTP 404
error is returned. If a
* resize operation was initiated and completed, the status of the resize
* remains as SUCCEEDED
until the next resize.
*
*
* A resize operation can be requested using ModifyCluster and
* specifying a different number or type of nodes for the cluster.
*
*
* @param describeResizeRequest Container for the necessary parameters to
* execute the DescribeResize service method on AmazonRedshift.
*
* @return The response from the DescribeResize service method, as
* returned by AmazonRedshift.
*
* @throws ClusterNotFoundException
* @throws ResizeNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeResizeResult describeResize(DescribeResizeRequest describeResizeRequest) {
ExecutionContext executionContext = createExecutionContext(describeResizeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeResizeRequestMarshaller().marshall(describeResizeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeResizeResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns descriptions of the available Amazon Redshift cluster
* versions. You can call this operation even before creating any
* clusters to learn more about the Amazon Redshift versions. For more
* information about managing clusters, go to
* Amazon Redshift Clusters
* in the Amazon Redshift Management Guide
*
*
* @param describeClusterVersionsRequest Container for the necessary
* parameters to execute the DescribeClusterVersions service method on
* AmazonRedshift.
*
* @return The response from the DescribeClusterVersions service method,
* as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeClusterVersionsResult describeClusterVersions(DescribeClusterVersionsRequest describeClusterVersionsRequest) {
ExecutionContext executionContext = createExecutionContext(describeClusterVersionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeClusterVersionsRequestMarshaller().marshall(describeClusterVersionsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeClusterVersionsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new cluster from a snapshot. Amazon Redshift creates the
* resulting cluster with the same configuration as the original cluster
* from which the snapshot was created, except that the new cluster is
* created with the default cluster security and parameter group. After
* Amazon Redshift creates the cluster you can use the ModifyCluster API
* to associate a different security group and different parameter group
* with the restored cluster.
*
*
* If a snapshot is taken of a cluster in VPC, you can restore it only
* in VPC. In this case, you must provide a cluster subnet group where
* you want the cluster restored. If snapshot is taken of a cluster
* outside VPC, then you can restore it only outside VPC.
*
*
* For more information about working with snapshots, go to
* Amazon Redshift Snapshots
* in the Amazon Redshift Management Guide .
*
*
* @param restoreFromClusterSnapshotRequest Container for the necessary
* parameters to execute the RestoreFromClusterSnapshot service method on
* AmazonRedshift.
*
* @return The response from the RestoreFromClusterSnapshot service
* method, as returned by AmazonRedshift.
*
* @throws InvalidElasticIpException
* @throws InvalidSubnetException
* @throws HsmConfigurationNotFoundException
* @throws ClusterSubnetGroupNotFoundException
* @throws InvalidClusterSubnetGroupStateException
* @throws ClusterAlreadyExistsException
* @throws InvalidVPCNetworkStateException
* @throws InvalidClusterSnapshotStateException
* @throws AccessToSnapshotDeniedException
* @throws InvalidRestoreException
* @throws UnauthorizedOperationException
* @throws InsufficientClusterCapacityException
* @throws NumberOfNodesQuotaExceededException
* @throws NumberOfNodesPerClusterLimitExceededException
* @throws ClusterSnapshotNotFoundException
* @throws ClusterQuotaExceededException
* @throws HsmClientCertificateNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Cluster restoreFromClusterSnapshot(RestoreFromClusterSnapshotRequest restoreFromClusterSnapshotRequest) {
ExecutionContext executionContext = createExecutionContext(restoreFromClusterSnapshotRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new RestoreFromClusterSnapshotRequestMarshaller().marshall(restoreFromClusterSnapshotRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ClusterStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Modifies the parameters of a parameter group.
*
*
* For more information about managing parameter groups, go to
* Amazon Redshift Parameter Groups
* in the Amazon Redshift Management Guide .
*
*
* @param modifyClusterParameterGroupRequest Container for the necessary
* parameters to execute the ModifyClusterParameterGroup service method
* on AmazonRedshift.
*
* @return The response from the ModifyClusterParameterGroup service
* method, as returned by AmazonRedshift.
*
* @throws InvalidClusterParameterGroupStateException
* @throws ClusterParameterGroupNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public ModifyClusterParameterGroupResult modifyClusterParameterGroup(ModifyClusterParameterGroupRequest modifyClusterParameterGroupRequest) {
ExecutionContext executionContext = createExecutionContext(modifyClusterParameterGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ModifyClusterParameterGroupRequestMarshaller().marshall(modifyClusterParameterGroupRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ModifyClusterParameterGroupResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Displays a list of event categories for all event source types, or for
* a specified source type. For a list of the event categories and source
* types, go to
* Amazon Redshift Event Notifications
* .
*
*
* @param describeEventCategoriesRequest Container for the necessary
* parameters to execute the DescribeEventCategories service method on
* AmazonRedshift.
*
* @return The response from the DescribeEventCategories service method,
* as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeEventCategoriesResult describeEventCategories(DescribeEventCategoriesRequest describeEventCategoriesRequest) {
ExecutionContext executionContext = createExecutionContext(describeEventCategoriesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeEventCategoriesRequestMarshaller().marshall(describeEventCategoriesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeEventCategoriesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about Amazon Redshift security groups. If the
* name of a security group is specified, the response will contain only
* information about only that security group.
*
*
* For information about managing security groups, go to
* Amazon Redshift Cluster Security Groups
* in the Amazon Redshift Management Guide .
*
*
* @param describeClusterSecurityGroupsRequest Container for the
* necessary parameters to execute the DescribeClusterSecurityGroups
* service method on AmazonRedshift.
*
* @return The response from the DescribeClusterSecurityGroups service
* method, as returned by AmazonRedshift.
*
* @throws ClusterSecurityGroupNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeClusterSecurityGroupsResult describeClusterSecurityGroups(DescribeClusterSecurityGroupsRequest describeClusterSecurityGroupsRequest) {
ExecutionContext executionContext = createExecutionContext(describeClusterSecurityGroupsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeClusterSecurityGroupsRequestMarshaller().marshall(describeClusterSecurityGroupsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeClusterSecurityGroupsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns one or more cluster subnet group objects, which contain
* metadata about your cluster subnet groups. By default, this operation
* returns information about all cluster subnet groups that are defined
* in you AWS account.
*
*
* @param describeClusterSubnetGroupsRequest Container for the necessary
* parameters to execute the DescribeClusterSubnetGroups service method
* on AmazonRedshift.
*
* @return The response from the DescribeClusterSubnetGroups service
* method, as returned by AmazonRedshift.
*
* @throws ClusterSubnetGroupNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeClusterSubnetGroupsResult describeClusterSubnetGroups(DescribeClusterSubnetGroupsRequest describeClusterSubnetGroupsRequest) {
ExecutionContext executionContext = createExecutionContext(describeClusterSubnetGroupsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeClusterSubnetGroupsRequestMarshaller().marshall(describeClusterSubnetGroupsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeClusterSubnetGroupsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about the specified Amazon Redshift HSM
* configuration. If no configuration ID is specified, returns
* information about all the HSM configurations owned by your AWS
* customer account.
*
*
* @param describeHsmConfigurationsRequest Container for the necessary
* parameters to execute the DescribeHsmConfigurations service method on
* AmazonRedshift.
*
* @return The response from the DescribeHsmConfigurations service
* method, as returned by AmazonRedshift.
*
* @throws HsmConfigurationNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeHsmConfigurationsResult describeHsmConfigurations(DescribeHsmConfigurationsRequest describeHsmConfigurationsRequest) {
ExecutionContext executionContext = createExecutionContext(describeHsmConfigurationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeHsmConfigurationsRequestMarshaller().marshall(describeHsmConfigurationsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeHsmConfigurationsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified manual snapshot. The snapshot must be in the
* "available" state, with no other users authorized to access the
* snapshot.
*
*
* Unlike automated snapshots, manual snapshots are retained even after
* you delete your cluster. Amazon Redshift does not delete your manual
* snapshots. You must delete manual snapshot explicitly to avoid getting
* charged. If other accounts are authorized to access the snapshot, you
* must revoke all of the authorizations before you can delete the
* snapshot.
*
*
* @param deleteClusterSnapshotRequest Container for the necessary
* parameters to execute the DeleteClusterSnapshot service method on
* AmazonRedshift.
*
* @return The response from the DeleteClusterSnapshot service method, as
* returned by AmazonRedshift.
*
* @throws ClusterSnapshotNotFoundException
* @throws InvalidClusterSnapshotStateException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Snapshot deleteClusterSnapshot(DeleteClusterSnapshotRequest deleteClusterSnapshotRequest) {
ExecutionContext executionContext = createExecutionContext(deleteClusterSnapshotRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteClusterSnapshotRequestMarshaller().marshall(deleteClusterSnapshotRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new SnapshotStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a specified Amazon Redshift parameter group.
* NOTE:You cannot delete a parameter group if it is associated
* with a cluster.
*
*
*
*
* @param deleteClusterParameterGroupRequest Container for the necessary
* parameters to execute the DeleteClusterParameterGroup service method
* on AmazonRedshift.
*
*
* @throws InvalidClusterParameterGroupStateException
* @throws ClusterParameterGroupNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteClusterParameterGroup(DeleteClusterParameterGroupRequest deleteClusterParameterGroupRequest) {
ExecutionContext executionContext = createExecutionContext(deleteClusterParameterGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteClusterParameterGroupRequestMarshaller().marshall(deleteClusterParameterGroupRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Disables the automatic copying of snapshots from one region to another
* region for a specified cluster.
*
*
* @param disableSnapshotCopyRequest Container for the necessary
* parameters to execute the DisableSnapshotCopy service method on
* AmazonRedshift.
*
* @return The response from the DisableSnapshotCopy service method, as
* returned by AmazonRedshift.
*
* @throws SnapshotCopyAlreadyDisabledException
* @throws UnauthorizedOperationException
* @throws InvalidClusterStateException
* @throws ClusterNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Cluster disableSnapshotCopy(DisableSnapshotCopyRequest disableSnapshotCopyRequest) {
ExecutionContext executionContext = createExecutionContext(disableSnapshotCopyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DisableSnapshotCopyRequestMarshaller().marshall(disableSnapshotCopyRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ClusterStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Amazon Redshift event notification subscription. This
* action requires an ARN (Amazon Resource Name) of an Amazon SNS topic
* created by either the Amazon Redshift console, the Amazon SNS console,
* or the Amazon SNS API. To obtain an ARN with Amazon SNS, you must
* create a topic in Amazon SNS and subscribe to the topic. The ARN is
* displayed in the SNS console.
*
*
* You can specify the source type, and lists of Amazon Redshift source
* IDs, event categories, and event severities. Notifications will be
* sent for all events you want that match those criteria. For example,
* you can specify source type = cluster, source ID = my-cluster-1 and
* mycluster2, event categories = Availability, Backup, and severity =
* ERROR. The subscription will only send notifications for those ERROR
* events in the Availability and Backup categories for the specified
* clusters.
*
*
* If you specify both the source type and source IDs, such as source
* type = cluster and source identifier = my-cluster-1, notifications
* will be sent for all the cluster events for my-cluster-1. If you
* specify a source type but do not specify a source identifier, you will
* receive notice of the events for the objects of that type in your AWS
* account. If you do not specify either the SourceType nor the
* SourceIdentifier, you will be notified of events generated from all
* Amazon Redshift sources belonging to your AWS account. You must
* specify a source type if you specify a source ID.
*
*
* @param createEventSubscriptionRequest Container for the necessary
* parameters to execute the CreateEventSubscription service method on
* AmazonRedshift.
*
* @return The response from the CreateEventSubscription service method,
* as returned by AmazonRedshift.
*
* @throws SubscriptionCategoryNotFoundException
* @throws SubscriptionAlreadyExistException
* @throws EventSubscriptionQuotaExceededException
* @throws SubscriptionEventIdNotFoundException
* @throws SubscriptionSeverityNotFoundException
* @throws SourceNotFoundException
* @throws SNSNoAuthorizationException
* @throws SNSTopicArnNotFoundException
* @throws SNSInvalidTopicException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public EventSubscription createEventSubscription(CreateEventSubscriptionRequest createEventSubscriptionRequest) {
ExecutionContext executionContext = createExecutionContext(createEventSubscriptionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateEventSubscriptionRequestMarshaller().marshall(createEventSubscriptionRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new EventSubscriptionStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Sets one or more parameters of the specified parameter group to their
* default values and sets the source values of the parameters to
* "engine-default". To reset the entire parameter group specify the
* ResetAllParameters parameter. For parameter changes to take
* effect you must reboot any associated clusters.
*
*
* @param resetClusterParameterGroupRequest Container for the necessary
* parameters to execute the ResetClusterParameterGroup service method on
* AmazonRedshift.
*
* @return The response from the ResetClusterParameterGroup service
* method, as returned by AmazonRedshift.
*
* @throws InvalidClusterParameterGroupStateException
* @throws ClusterParameterGroupNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public ResetClusterParameterGroupResult resetClusterParameterGroup(ResetClusterParameterGroupRequest resetClusterParameterGroupRequest) {
ExecutionContext executionContext = createExecutionContext(resetClusterParameterGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ResetClusterParameterGroupRequestMarshaller().marshall(resetClusterParameterGroupRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ResetClusterParameterGroupResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Authorizes the specified AWS customer account to restore the
* specified snapshot.
*
*
* For more information about working with snapshots, go to
* Amazon Redshift Snapshots
* in the Amazon Redshift Management Guide .
*
*
* @param authorizeSnapshotAccessRequest Container for the necessary
* parameters to execute the AuthorizeSnapshotAccess service method on
* AmazonRedshift.
*
* @return The response from the AuthorizeSnapshotAccess service method,
* as returned by AmazonRedshift.
*
* @throws AuthorizationAlreadyExistsException
* @throws ClusterSnapshotNotFoundException
* @throws AuthorizationQuotaExceededException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Snapshot authorizeSnapshotAccess(AuthorizeSnapshotAccessRequest authorizeSnapshotAccessRequest) {
ExecutionContext executionContext = createExecutionContext(authorizeSnapshotAccessRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new AuthorizeSnapshotAccessRequestMarshaller().marshall(authorizeSnapshotAccessRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new SnapshotStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Enables the automatic copy of snapshots from one region to another
* region for a specified cluster.
*
*
* @param enableSnapshotCopyRequest Container for the necessary
* parameters to execute the EnableSnapshotCopy service method on
* AmazonRedshift.
*
* @return The response from the EnableSnapshotCopy service method, as
* returned by AmazonRedshift.
*
* @throws CopyToRegionDisabledException
* @throws UnauthorizedOperationException
* @throws InvalidClusterStateException
* @throws ClusterNotFoundException
* @throws IncompatibleOrderableOptionsException
* @throws SnapshotCopyAlreadyEnabledException
* @throws UnknownSnapshotCopyRegionException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public Cluster enableSnapshotCopy(EnableSnapshotCopyRequest enableSnapshotCopyRequest) {
ExecutionContext executionContext = createExecutionContext(enableSnapshotCopyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new EnableSnapshotCopyRequestMarshaller().marshall(enableSnapshotCopyRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ClusterStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of the available reserved node offerings by Amazon
* Redshift with their descriptions including the node type, the fixed
* and recurring costs of reserving the node and duration the node will
* be reserved for you. These descriptions help you determine which
* reserve node offering you want to purchase. You then use the unique
* offering ID in you call to PurchaseReservedNodeOffering to reserve one
* or more nodes for your Amazon Redshift cluster.
*
*
* For more information about managing parameter groups, go to
* Purchasing Reserved Nodes
* in the Amazon Redshift Management Guide .
*
*
*
*
* @param describeReservedNodeOfferingsRequest Container for the
* necessary parameters to execute the DescribeReservedNodeOfferings
* service method on AmazonRedshift.
*
* @return The response from the DescribeReservedNodeOfferings service
* method, as returned by AmazonRedshift.
*
* @throws ReservedNodeOfferingNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeReservedNodeOfferingsResult describeReservedNodeOfferings(DescribeReservedNodeOfferingsRequest describeReservedNodeOfferingsRequest) {
ExecutionContext executionContext = createExecutionContext(describeReservedNodeOfferingsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeReservedNodeOfferingsRequestMarshaller().marshall(describeReservedNodeOfferingsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeReservedNodeOfferingsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists descriptions of all the Amazon Redshift event notifications
* subscription for a customer account. If you specify a subscription
* name, lists the description for that subscription.
*
*
* @param describeEventSubscriptionsRequest Container for the necessary
* parameters to execute the DescribeEventSubscriptions service method on
* AmazonRedshift.
*
* @return The response from the DescribeEventSubscriptions service
* method, as returned by AmazonRedshift.
*
* @throws SubscriptionNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeEventSubscriptionsResult describeEventSubscriptions(DescribeEventSubscriptionsRequest describeEventSubscriptionsRequest) {
ExecutionContext executionContext = createExecutionContext(describeEventSubscriptionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeEventSubscriptionsRequestMarshaller().marshall(describeEventSubscriptionsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeEventSubscriptionsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes whether information, such as queries and connection
* attempts, is being logged for the specified Amazon Redshift cluster.
*
*
* @param describeLoggingStatusRequest Container for the necessary
* parameters to execute the DescribeLoggingStatus service method on
* AmazonRedshift.
*
* @return The response from the DescribeLoggingStatus service method, as
* returned by AmazonRedshift.
*
* @throws ClusterNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeLoggingStatusResult describeLoggingStatus(DescribeLoggingStatusRequest describeLoggingStatusRequest) {
ExecutionContext executionContext = createExecutionContext(describeLoggingStatusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeLoggingStatusRequestMarshaller().marshall(describeLoggingStatusRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeLoggingStatusResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an Amazon Redshift event notification subscription.
*
*
* @param deleteEventSubscriptionRequest Container for the necessary
* parameters to execute the DeleteEventSubscription service method on
* AmazonRedshift.
*
*
* @throws SubscriptionNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteEventSubscription(DeleteEventSubscriptionRequest deleteEventSubscriptionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteEventSubscriptionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteEventSubscriptionRequestMarshaller().marshall(deleteEventSubscriptionRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
invoke(request, null, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, null);
}
}
/**
*
* Creates an HSM client certificate that an Amazon Redshift cluster will
* use to connect to the client's HSM in order to store and retrieve the
* keys used to encrypt the cluster databases.
*
*
* The command returns a public key, which you must store in the HSM. In
* addition to creating the HSM certificate, you must create an Amazon
* Redshift HSM configuration that provides a cluster the information
* needed to store and use encryption keys in the HSM. For more
* information, go to
* Hardware Security Modules
* in the Amazon Redshift Management Guide.
*
*
* @param createHsmClientCertificateRequest Container for the necessary
* parameters to execute the CreateHsmClientCertificate service method on
* AmazonRedshift.
*
* @return The response from the CreateHsmClientCertificate service
* method, as returned by AmazonRedshift.
*
* @throws HsmClientCertificateQuotaExceededException
* @throws HsmClientCertificateAlreadyExistsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public HsmClientCertificate createHsmClientCertificate(CreateHsmClientCertificateRequest createHsmClientCertificateRequest) {
ExecutionContext executionContext = createExecutionContext(createHsmClientCertificateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateHsmClientCertificateRequestMarshaller().marshall(createHsmClientCertificateRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new HsmClientCertificateStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Revokes an ingress rule in an Amazon Redshift security group for a
* previously authorized IP range or Amazon EC2 security group. To add an
* ingress rule, see AuthorizeClusterSecurityGroupIngress. For
* information about managing security groups, go to
* Amazon Redshift Cluster Security Groups
* in the Amazon Redshift Management Guide .
*
*
*
* @param revokeClusterSecurityGroupIngressRequest Container for the
* necessary parameters to execute the RevokeClusterSecurityGroupIngress
* service method on AmazonRedshift.
*
* @return The response from the RevokeClusterSecurityGroupIngress
* service method, as returned by AmazonRedshift.
*
* @throws InvalidClusterSecurityGroupStateException
* @throws AuthorizationNotFoundException
* @throws ClusterSecurityGroupNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public ClusterSecurityGroup revokeClusterSecurityGroupIngress(RevokeClusterSecurityGroupIngressRequest revokeClusterSecurityGroupIngressRequest) {
ExecutionContext executionContext = createExecutionContext(revokeClusterSecurityGroupIngressRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new RevokeClusterSecurityGroupIngressRequestMarshaller().marshall(revokeClusterSecurityGroupIngressRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ClusterSecurityGroupStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a detailed list of parameters contained within the specified
* Amazon Redshift parameter group. For each parameter the response
* includes information such as parameter name, description, data type,
* value, whether the parameter value is modifiable, and so on.
*
*
* You can specify source filter to retrieve parameters of only
* specific type. For example, to retrieve parameters that were modified
* by a user action such as from ModifyClusterParameterGroup, you can
* specify source equal to user .
*
*
* For more information about managing parameter groups, go to
* Amazon Redshift Parameter Groups
* in the Amazon Redshift Management Guide .
*
*
* @param describeClusterParametersRequest Container for the necessary
* parameters to execute the DescribeClusterParameters service method on
* AmazonRedshift.
*
* @return The response from the DescribeClusterParameters service
* method, as returned by AmazonRedshift.
*
* @throws ClusterParameterGroupNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeClusterParametersResult describeClusterParameters(DescribeClusterParametersRequest describeClusterParametersRequest) {
ExecutionContext executionContext = createExecutionContext(describeClusterParametersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeClusterParametersRequestMarshaller().marshall(describeClusterParametersRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeClusterParametersResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about the specified HSM client certificate. If no
* certificate ID is specified, returns information about all the HSM
* certificates owned by your AWS customer account.
*
*
* @param describeHsmClientCertificatesRequest Container for the
* necessary parameters to execute the DescribeHsmClientCertificates
* service method on AmazonRedshift.
*
* @return The response from the DescribeHsmClientCertificates service
* method, as returned by AmazonRedshift.
*
* @throws HsmClientCertificateNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeHsmClientCertificatesResult describeHsmClientCertificates(DescribeHsmClientCertificatesRequest describeHsmClientCertificatesRequest) {
ExecutionContext executionContext = createExecutionContext(describeHsmClientCertificatesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeHsmClientCertificatesRequestMarshaller().marshall(describeHsmClientCertificatesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeHsmClientCertificatesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an HSM configuration that contains the information required by
* an Amazon Redshift cluster to store and use database encryption keys
* in a Hardware Security Module (HSM). After creating the HSM
* configuration, you can specify it as a parameter when creating a
* cluster. The cluster will then store its encryption keys in the HSM.
*
*
* In addition to creating an HSM configuration, you must also create an
* HSM client certificate. For more information, go to
* Hardware Security Modules
* in the Amazon Redshift Management Guide.
*
*
* @param createHsmConfigurationRequest Container for the necessary
* parameters to execute the CreateHsmConfiguration service method on
* AmazonRedshift.
*
* @return The response from the CreateHsmConfiguration service method,
* as returned by AmazonRedshift.
*
* @throws HsmConfigurationQuotaExceededException
* @throws HsmConfigurationAlreadyExistsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public HsmConfiguration createHsmConfiguration(CreateHsmConfigurationRequest createHsmConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(createHsmConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateHsmConfigurationRequestMarshaller().marshall(createHsmConfigurationRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new HsmConfigurationStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns properties of provisioned clusters including general cluster
* properties, cluster database properties, maintenance and backup
* properties, and security and access properties. This operation
* supports pagination. For more information about managing clusters, go
* to
* Amazon Redshift Clusters
* in the Amazon Redshift Management Guide .
*
*
*
* @param describeClustersRequest Container for the necessary parameters
* to execute the DescribeClusters service method on AmazonRedshift.
*
* @return The response from the DescribeClusters service method, as
* returned by AmazonRedshift.
*
* @throws ClusterNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeClustersResult describeClusters(DescribeClustersRequest describeClustersRequest) {
ExecutionContext executionContext = createExecutionContext(describeClustersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeClustersRequestMarshaller().marshall(describeClustersRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeClustersResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns one or more snapshot objects, which contain metadata about
* your cluster snapshots. By default, this operation returns information
* about all snapshots of all clusters that are owned by you AWS customer
* account. No information is returned for snapshots owned by inactive
* AWS customer accounts.
*
*
* @param describeClusterSnapshotsRequest Container for the necessary
* parameters to execute the DescribeClusterSnapshots service method on
* AmazonRedshift.
*
* @return The response from the DescribeClusterSnapshots service method,
* as returned by AmazonRedshift.
*
* @throws ClusterSnapshotNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeClusterSnapshotsResult describeClusterSnapshots(DescribeClusterSnapshotsRequest describeClusterSnapshotsRequest) {
ExecutionContext executionContext = createExecutionContext(describeClusterSnapshotsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeClusterSnapshotsRequestMarshaller().marshall(describeClusterSnapshotsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeClusterSnapshotsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Amazon Redshift parameter group.
*
*
* Creating parameter groups is independent of creating clusters. You can
* associate a cluster with a parameter group when you create the
* cluster. You can also associate an existing cluster with a parameter
* group after the cluster is created by using ModifyCluster.
*
*
* Parameters in the parameter group define specific behavior that
* applies to the databases you create on the cluster. For more
* information about managing parameter groups, go to
* Amazon Redshift Parameter Groups
* in the Amazon Redshift Management Guide .
*
*
*
* @param createClusterParameterGroupRequest Container for the necessary
* parameters to execute the CreateClusterParameterGroup service method
* on AmazonRedshift.
*
* @return The response from the CreateClusterParameterGroup service
* method, as returned by AmazonRedshift.
*
* @throws ClusterParameterGroupQuotaExceededException
* @throws ClusterParameterGroupAlreadyExistsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public ClusterParameterGroup createClusterParameterGroup(CreateClusterParameterGroupRequest createClusterParameterGroupRequest) {
ExecutionContext executionContext = createExecutionContext(createClusterParameterGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateClusterParameterGroupRequestMarshaller().marshall(createClusterParameterGroupRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ClusterParameterGroupStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of Amazon Redshift parameter groups, including
* parameter groups you created and the default parameter group. For each
* parameter group, the response includes the parameter group name,
* description, and parameter group family name. You can optionally
* specify a name to retrieve the description of a specific parameter
* group.
*
*
* For more information about managing parameter groups, go to
* Amazon Redshift Parameter Groups
* in the Amazon Redshift Management Guide .
*
*
* @param describeClusterParameterGroupsRequest Container for the
* necessary parameters to execute the DescribeClusterParameterGroups
* service method on AmazonRedshift.
*
* @return The response from the DescribeClusterParameterGroups service
* method, as returned by AmazonRedshift.
*
* @throws ClusterParameterGroupNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeClusterParameterGroupsResult describeClusterParameterGroups(DescribeClusterParameterGroupsRequest describeClusterParameterGroupsRequest) {
ExecutionContext executionContext = createExecutionContext(describeClusterParameterGroupsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DescribeClusterParameterGroupsRequestMarshaller().marshall(describeClusterParameterGroupsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DescribeClusterParameterGroupsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of orderable cluster options. Before you create a new
* cluster you can use this operation to find what options are available,
* such as the EC2 Availability Zones (AZ) in the specific AWS region
* that you can specify, and the node types you can request. The node
* types differ by available storage, memory, CPU and price. With the
* cost involved you might want to obtain a list of cluster options in
* the specific region and specify values when creating a cluster. For
* more information about managing clusters, go to
* Amazon Redshift Clusters
* in the Amazon Redshift Management Guide
*
*
* @return The response from the DescribeOrderableClusterOptions service
* method, as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeOrderableClusterOptionsResult describeOrderableClusterOptions() throws AmazonServiceException, AmazonClientException {
return describeOrderableClusterOptions(new DescribeOrderableClusterOptionsRequest());
}
/**
*
* Returns events related to clusters, security groups, snapshots, and
* parameter groups for the past 14 days. Events specific to a particular
* cluster, security group, snapshot or parameter group can be obtained
* by providing the name as a parameter. By default, the past hour of
* events are returned.
*
*
* @return The response from the DescribeEvents service method, as
* returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeEventsResult describeEvents() throws AmazonServiceException, AmazonClientException {
return describeEvents(new DescribeEventsRequest());
}
/**
*
* Returns the descriptions of the reserved nodes.
*
*
* @return The response from the DescribeReservedNodes service method, as
* returned by AmazonRedshift.
*
* @throws ReservedNodeNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeReservedNodesResult describeReservedNodes() throws AmazonServiceException, AmazonClientException {
return describeReservedNodes(new DescribeReservedNodesRequest());
}
/**
*
* Returns descriptions of the available Amazon Redshift cluster
* versions. You can call this operation even before creating any
* clusters to learn more about the Amazon Redshift versions. For more
* information about managing clusters, go to
* Amazon Redshift Clusters
* in the Amazon Redshift Management Guide
*
*
* @return The response from the DescribeClusterVersions service method,
* as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeClusterVersionsResult describeClusterVersions() throws AmazonServiceException, AmazonClientException {
return describeClusterVersions(new DescribeClusterVersionsRequest());
}
/**
*
* Displays a list of event categories for all event source types, or for
* a specified source type. For a list of the event categories and source
* types, go to
* Amazon Redshift Event Notifications
* .
*
*
* @return The response from the DescribeEventCategories service method,
* as returned by AmazonRedshift.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeEventCategoriesResult describeEventCategories() throws AmazonServiceException, AmazonClientException {
return describeEventCategories(new DescribeEventCategoriesRequest());
}
/**
*
* Returns information about Amazon Redshift security groups. If the
* name of a security group is specified, the response will contain only
* information about only that security group.
*
*
* For information about managing security groups, go to
* Amazon Redshift Cluster Security Groups
* in the Amazon Redshift Management Guide .
*
*
* @return The response from the DescribeClusterSecurityGroups service
* method, as returned by AmazonRedshift.
*
* @throws ClusterSecurityGroupNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeClusterSecurityGroupsResult describeClusterSecurityGroups() throws AmazonServiceException, AmazonClientException {
return describeClusterSecurityGroups(new DescribeClusterSecurityGroupsRequest());
}
/**
*
* Returns one or more cluster subnet group objects, which contain
* metadata about your cluster subnet groups. By default, this operation
* returns information about all cluster subnet groups that are defined
* in you AWS account.
*
*
* @return The response from the DescribeClusterSubnetGroups service
* method, as returned by AmazonRedshift.
*
* @throws ClusterSubnetGroupNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeClusterSubnetGroupsResult describeClusterSubnetGroups() throws AmazonServiceException, AmazonClientException {
return describeClusterSubnetGroups(new DescribeClusterSubnetGroupsRequest());
}
/**
*
* Returns information about the specified Amazon Redshift HSM
* configuration. If no configuration ID is specified, returns
* information about all the HSM configurations owned by your AWS
* customer account.
*
*
* @return The response from the DescribeHsmConfigurations service
* method, as returned by AmazonRedshift.
*
* @throws HsmConfigurationNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeHsmConfigurationsResult describeHsmConfigurations() throws AmazonServiceException, AmazonClientException {
return describeHsmConfigurations(new DescribeHsmConfigurationsRequest());
}
/**
*
* Returns a list of the available reserved node offerings by Amazon
* Redshift with their descriptions including the node type, the fixed
* and recurring costs of reserving the node and duration the node will
* be reserved for you. These descriptions help you determine which
* reserve node offering you want to purchase. You then use the unique
* offering ID in you call to PurchaseReservedNodeOffering to reserve one
* or more nodes for your Amazon Redshift cluster.
*
*
* For more information about managing parameter groups, go to
* Purchasing Reserved Nodes
* in the Amazon Redshift Management Guide .
*
*
*
*
* @return The response from the DescribeReservedNodeOfferings service
* method, as returned by AmazonRedshift.
*
* @throws ReservedNodeOfferingNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeReservedNodeOfferingsResult describeReservedNodeOfferings() throws AmazonServiceException, AmazonClientException {
return describeReservedNodeOfferings(new DescribeReservedNodeOfferingsRequest());
}
/**
*
* Lists descriptions of all the Amazon Redshift event notifications
* subscription for a customer account. If you specify a subscription
* name, lists the description for that subscription.
*
*
* @return The response from the DescribeEventSubscriptions service
* method, as returned by AmazonRedshift.
*
* @throws SubscriptionNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeEventSubscriptionsResult describeEventSubscriptions() throws AmazonServiceException, AmazonClientException {
return describeEventSubscriptions(new DescribeEventSubscriptionsRequest());
}
/**
*
* Returns information about the specified HSM client certificate. If no
* certificate ID is specified, returns information about all the HSM
* certificates owned by your AWS customer account.
*
*
* @return The response from the DescribeHsmClientCertificates service
* method, as returned by AmazonRedshift.
*
* @throws HsmClientCertificateNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeHsmClientCertificatesResult describeHsmClientCertificates() throws AmazonServiceException, AmazonClientException {
return describeHsmClientCertificates(new DescribeHsmClientCertificatesRequest());
}
/**
*
* Returns properties of provisioned clusters including general cluster
* properties, cluster database properties, maintenance and backup
* properties, and security and access properties. This operation
* supports pagination. For more information about managing clusters, go
* to
* Amazon Redshift Clusters
* in the Amazon Redshift Management Guide .
*
*
*
* @return The response from the DescribeClusters service method, as
* returned by AmazonRedshift.
*
* @throws ClusterNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeClustersResult describeClusters() throws AmazonServiceException, AmazonClientException {
return describeClusters(new DescribeClustersRequest());
}
/**
*
* Returns one or more snapshot objects, which contain metadata about
* your cluster snapshots. By default, this operation returns information
* about all snapshots of all clusters that are owned by you AWS customer
* account. No information is returned for snapshots owned by inactive
* AWS customer accounts.
*
*
* @return The response from the DescribeClusterSnapshots service method,
* as returned by AmazonRedshift.
*
* @throws ClusterSnapshotNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeClusterSnapshotsResult describeClusterSnapshots() throws AmazonServiceException, AmazonClientException {
return describeClusterSnapshots(new DescribeClusterSnapshotsRequest());
}
/**
*
* Returns a list of Amazon Redshift parameter groups, including
* parameter groups you created and the default parameter group. For each
* parameter group, the response includes the parameter group name,
* description, and parameter group family name. You can optionally
* specify a name to retrieve the description of a specific parameter
* group.
*
*
* For more information about managing parameter groups, go to
* Amazon Redshift Parameter Groups
* in the Amazon Redshift Management Guide .
*
*
* @return The response from the DescribeClusterParameterGroups service
* method, as returned by AmazonRedshift.
*
* @throws ClusterParameterGroupNotFoundException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRedshift indicating
* either a problem with the data in the request, or a server side issue.
*/
public DescribeClusterParameterGroupsResult describeClusterParameterGroups() throws AmazonServiceException, AmazonClientException {
return describeClusterParameterGroups(new DescribeClusterParameterGroupsRequest());
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for
* debugging issues where a service isn't acting as expected. This data isn't considered part
* of the result data returned by an operation, so it's available through this separate,
* diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access
* this extra diagnostic information for an executed request, you should use this method
* to retrieve it as soon as possible after executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none
* is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
private Response invoke(Request request,
Unmarshaller unmarshaller,
ExecutionContext executionContext)
{
request.setEndpoint(endpoint);
request.setTimeOffset(timeOffset);
AmazonWebServiceRequest originalRequest = request.getOriginalRequest();
for (Entry entry : originalRequest.copyPrivateRequestParameters().entrySet()) {
request.addParameter(entry.getKey(), entry.getValue());
}
AWSCredentials credentials = awsCredentialsProvider.getCredentials();
if (originalRequest.getRequestCredentials() != null) {
credentials = originalRequest.getRequestCredentials();
}
executionContext.setCredentials(credentials);
StaxResponseHandler responseHandler = new StaxResponseHandler(unmarshaller);
DefaultErrorResponseHandler errorResponseHandler = new DefaultErrorResponseHandler(exceptionUnmarshallers);
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
}