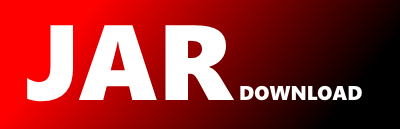
com.amazonaws.services.route53.AmazonRoute53Async Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.route53;
import java.util.concurrent.Future;
import com.amazonaws.AmazonClientException;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.handlers.AsyncHandler;
import com.amazonaws.services.route53.model.*;
/**
* Interface for accessing AmazonRoute53 asynchronously.
* Each asynchronous method will return a Java Future object, and users are also allowed
* to provide a callback handler.
*
*/
public interface AmazonRoute53Async extends AmazonRoute53 {
/**
*
* To retrieve the health check, send a GET
request to the
* 2013-04-01/healthcheck/health check ID
resource.
*
*
* @param getHealthCheckRequest Container for the necessary parameters to
* execute the GetHealthCheck operation on AmazonRoute53.
*
* @return A Java Future object containing the response from the
* GetHealthCheck service method, as returned by AmazonRoute53.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getHealthCheckAsync(GetHealthCheckRequest getHealthCheckRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* To retrieve the health check, send a GET
request to the
* 2013-04-01/healthcheck/health check ID
resource.
*
*
* @param getHealthCheckRequest Container for the necessary parameters to
* execute the GetHealthCheck operation on AmazonRoute53.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* GetHealthCheck service method, as returned by AmazonRoute53.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getHealthCheckAsync(GetHealthCheckRequest getHealthCheckRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* To retrieve the delegation set for a hosted zone, send a
* GET
request to the 2013-04-01/hostedzone/hosted
* zone ID
resource. The delegation set is the four Route 53 name
* servers that were assigned to the hosted zone when you created it.
*
*
* @param getHostedZoneRequest Container for the necessary parameters to
* execute the GetHostedZone operation on AmazonRoute53.
*
* @return A Java Future object containing the response from the
* GetHostedZone service method, as returned by AmazonRoute53.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getHostedZoneAsync(GetHostedZoneRequest getHostedZoneRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* To retrieve the delegation set for a hosted zone, send a
* GET
request to the 2013-04-01/hostedzone/hosted
* zone ID
resource. The delegation set is the four Route 53 name
* servers that were assigned to the hosted zone when you created it.
*
*
* @param getHostedZoneRequest Container for the necessary parameters to
* execute the GetHostedZone operation on AmazonRoute53.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* GetHostedZone service method, as returned by AmazonRoute53.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getHostedZoneAsync(GetHostedZoneRequest getHostedZoneRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* This action deletes a hosted zone. To delete a hosted zone, send a
* DELETE
request to the 2013-04-01/hostedzone/hosted
* zone ID
resource.
*
*
* For more information about deleting a hosted zone, see
* Deleting a Hosted Zone
* in the Amazon Route 53 Developer Guide .
*
*
* IMPORTANT: You can delete a hosted zone only if there are no
* resource record sets other than the default SOA record and NS resource
* record sets. If your hosted zone contains other resource record sets,
* you must delete them before you can delete your hosted zone. If you
* try to delete a hosted zone that contains other resource record sets,
* Route 53 will deny your request with a HostedZoneNotEmpty error. For
* information about deleting records from your hosted zone, see
* ChangeResourceRecordSets.
*
*
* @param deleteHostedZoneRequest Container for the necessary parameters
* to execute the DeleteHostedZone operation on AmazonRoute53.
*
* @return A Java Future object containing the response from the
* DeleteHostedZone service method, as returned by AmazonRoute53.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteHostedZoneAsync(DeleteHostedZoneRequest deleteHostedZoneRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* This action deletes a hosted zone. To delete a hosted zone, send a
* DELETE
request to the 2013-04-01/hostedzone/hosted
* zone ID
resource.
*
*
* For more information about deleting a hosted zone, see
* Deleting a Hosted Zone
* in the Amazon Route 53 Developer Guide .
*
*
* IMPORTANT: You can delete a hosted zone only if there are no
* resource record sets other than the default SOA record and NS resource
* record sets. If your hosted zone contains other resource record sets,
* you must delete them before you can delete your hosted zone. If you
* try to delete a hosted zone that contains other resource record sets,
* Route 53 will deny your request with a HostedZoneNotEmpty error. For
* information about deleting records from your hosted zone, see
* ChangeResourceRecordSets.
*
*
* @param deleteHostedZoneRequest Container for the necessary parameters
* to execute the DeleteHostedZone operation on AmazonRoute53.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteHostedZone service method, as returned by AmazonRoute53.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteHostedZoneAsync(DeleteHostedZoneRequest deleteHostedZoneRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* This action returns the current status of a change batch request. The
* status is one of the following values:
*
*
* - PENDING
indicates that the changes in this request have
* not replicated to all Route 53 DNS servers. This is the initial status
* of all change batch requests.
*
*
* - INSYNC
indicates that the changes have replicated to
* all Amazon Route 53 DNS servers.
*
*
* @param getChangeRequest Container for the necessary parameters to
* execute the GetChange operation on AmazonRoute53.
*
* @return A Java Future object containing the response from the
* GetChange service method, as returned by AmazonRoute53.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getChangeAsync(GetChangeRequest getChangeRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* This action returns the current status of a change batch request. The
* status is one of the following values:
*
*
* - PENDING
indicates that the changes in this request have
* not replicated to all Route 53 DNS servers. This is the initial status
* of all change batch requests.
*
*
* - INSYNC
indicates that the changes have replicated to
* all Amazon Route 53 DNS servers.
*
*
* @param getChangeRequest Container for the necessary parameters to
* execute the GetChange operation on AmazonRoute53.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* GetChange service method, as returned by AmazonRoute53.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getChangeAsync(GetChangeRequest getChangeRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* To retrieve a list of your hosted zones, send a GET
* request to the 2013-04-01/hostedzone
resource. The
* response to this request includes a HostedZones
element
* with zero, one, or multiple HostedZone
child elements. By
* default, the list of hosted zones is displayed on a single page. You
* can control the length of the page that is displayed by using the
* MaxItems
parameter. You can use the Marker
* parameter to control the hosted zone that the list begins with.
*
*
* NOTE: Amazon Route 53 returns a maximum of 100 items. If you
* set MaxItems to a value greater than 100, Amazon Route 53 returns only
* the first 100.
*
*
* @param listHostedZonesRequest Container for the necessary parameters
* to execute the ListHostedZones operation on AmazonRoute53.
*
* @return A Java Future object containing the response from the
* ListHostedZones service method, as returned by AmazonRoute53.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listHostedZonesAsync(ListHostedZonesRequest listHostedZonesRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* To retrieve a list of your hosted zones, send a GET
* request to the 2013-04-01/hostedzone
resource. The
* response to this request includes a HostedZones
element
* with zero, one, or multiple HostedZone
child elements. By
* default, the list of hosted zones is displayed on a single page. You
* can control the length of the page that is displayed by using the
* MaxItems
parameter. You can use the Marker
* parameter to control the hosted zone that the list begins with.
*
*
* NOTE: Amazon Route 53 returns a maximum of 100 items. If you
* set MaxItems to a value greater than 100, Amazon Route 53 returns only
* the first 100.
*
*
* @param listHostedZonesRequest Container for the necessary parameters
* to execute the ListHostedZones operation on AmazonRoute53.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ListHostedZones service method, as returned by AmazonRoute53.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listHostedZonesAsync(ListHostedZonesRequest listHostedZonesRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* This action creates a new hosted zone.
*
*
* To create a new hosted zone, send a POST
request to the
* 2013-04-01/hostedzone
resource. The request body must
* include an XML document with a CreateHostedZoneRequest
* element. The response returns the
* CreateHostedZoneResponse
element that contains metadata
* about the hosted zone.
*
*
* Route 53 automatically creates a default SOA record and four NS
* records for the zone. The NS records in the hosted zone are the name
* servers you give your registrar to delegate your domain to. For more
* information about SOA and NS records, see
* NS and SOA Records that Route 53 Creates for a Hosted Zone
* in the Amazon Route 53 Developer Guide .
*
*
* When you create a zone, its initial status is PENDING
.
* This means that it is not yet available on all DNS servers. The status
* of the zone changes to INSYNC
when the NS and SOA records
* are available on all Route 53 DNS servers.
*
*
* @param createHostedZoneRequest Container for the necessary parameters
* to execute the CreateHostedZone operation on AmazonRoute53.
*
* @return A Java Future object containing the response from the
* CreateHostedZone service method, as returned by AmazonRoute53.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createHostedZoneAsync(CreateHostedZoneRequest createHostedZoneRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* This action creates a new hosted zone.
*
*
* To create a new hosted zone, send a POST
request to the
* 2013-04-01/hostedzone
resource. The request body must
* include an XML document with a CreateHostedZoneRequest
* element. The response returns the
* CreateHostedZoneResponse
element that contains metadata
* about the hosted zone.
*
*
* Route 53 automatically creates a default SOA record and four NS
* records for the zone. The NS records in the hosted zone are the name
* servers you give your registrar to delegate your domain to. For more
* information about SOA and NS records, see
* NS and SOA Records that Route 53 Creates for a Hosted Zone
* in the Amazon Route 53 Developer Guide .
*
*
* When you create a zone, its initial status is PENDING
.
* This means that it is not yet available on all DNS servers. The status
* of the zone changes to INSYNC
when the NS and SOA records
* are available on all Route 53 DNS servers.
*
*
* @param createHostedZoneRequest Container for the necessary parameters
* to execute the CreateHostedZone operation on AmazonRoute53.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateHostedZone service method, as returned by AmazonRoute53.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createHostedZoneAsync(CreateHostedZoneRequest createHostedZoneRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Use this action to create or change your authoritative DNS
* information. To use this action, send a POST
request to
* the 2013-04-01/hostedzone/hosted Zone ID/rrset
resource.
* The request body must include an XML document with a
* ChangeResourceRecordSetsRequest
element.
*
*
* Changes are a list of change items and are considered transactional.
* For more information on transactional changes, also known as change
* batches, see
* Creating, Changing, and Deleting Resource Record Sets Using the Route 53 API
* in the Amazon Route 53 Developer Guide .
*
*
* IMPORTANT:Due to the nature of transactional changes, you
* cannot delete the same resource record set more than once in a single
* change batch. If you attempt to delete the same change batch more than
* once, Route 53 returns an InvalidChangeBatch error.
*
*
* In response to a ChangeResourceRecordSets
request, your
* DNS data is changed on all Route 53 DNS servers. Initially, the status
* of a change is PENDING
. This means the change has not
* yet propagated to all the authoritative Route 53 DNS servers. When the
* change is propagated to all hosts, the change returns a status of
* INSYNC
.
*
*
* Note the following limitations on a
* ChangeResourceRecordSets
request:
*
*
* - A request cannot contain more than 100 Change elements.
*
*
* - A request cannot contain more than 1000 ResourceRecord elements.
*
*
* The sum of the number of characters (including spaces) in all
* Value
elements in a request cannot exceed 32,000
* characters.
*
*
* @param changeResourceRecordSetsRequest Container for the necessary
* parameters to execute the ChangeResourceRecordSets operation on
* AmazonRoute53.
*
* @return A Java Future object containing the response from the
* ChangeResourceRecordSets service method, as returned by AmazonRoute53.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future changeResourceRecordSetsAsync(ChangeResourceRecordSetsRequest changeResourceRecordSetsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Use this action to create or change your authoritative DNS
* information. To use this action, send a POST
request to
* the 2013-04-01/hostedzone/hosted Zone ID/rrset
resource.
* The request body must include an XML document with a
* ChangeResourceRecordSetsRequest
element.
*
*
* Changes are a list of change items and are considered transactional.
* For more information on transactional changes, also known as change
* batches, see
* Creating, Changing, and Deleting Resource Record Sets Using the Route 53 API
* in the Amazon Route 53 Developer Guide .
*
*
* IMPORTANT:Due to the nature of transactional changes, you
* cannot delete the same resource record set more than once in a single
* change batch. If you attempt to delete the same change batch more than
* once, Route 53 returns an InvalidChangeBatch error.
*
*
* In response to a ChangeResourceRecordSets
request, your
* DNS data is changed on all Route 53 DNS servers. Initially, the status
* of a change is PENDING
. This means the change has not
* yet propagated to all the authoritative Route 53 DNS servers. When the
* change is propagated to all hosts, the change returns a status of
* INSYNC
.
*
*
* Note the following limitations on a
* ChangeResourceRecordSets
request:
*
*
* - A request cannot contain more than 100 Change elements.
*
*
* - A request cannot contain more than 1000 ResourceRecord elements.
*
*
* The sum of the number of characters (including spaces) in all
* Value
elements in a request cannot exceed 32,000
* characters.
*
*
* @param changeResourceRecordSetsRequest Container for the necessary
* parameters to execute the ChangeResourceRecordSets operation on
* AmazonRoute53.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ChangeResourceRecordSets service method, as returned by AmazonRoute53.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future changeResourceRecordSetsAsync(ChangeResourceRecordSetsRequest changeResourceRecordSetsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* This action creates a new health check.
*
*
* To create a new health check, send a POST
request to the
* 2013-04-01/healthcheck
resource. The request body must
* include an XML document with a CreateHealthCheckRequest
* element. The response returns the
* CreateHealthCheckResponse
element that contains metadata
* about the health check.
*
*
* @param createHealthCheckRequest Container for the necessary parameters
* to execute the CreateHealthCheck operation on AmazonRoute53.
*
* @return A Java Future object containing the response from the
* CreateHealthCheck service method, as returned by AmazonRoute53.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createHealthCheckAsync(CreateHealthCheckRequest createHealthCheckRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* This action creates a new health check.
*
*
* To create a new health check, send a POST
request to the
* 2013-04-01/healthcheck
resource. The request body must
* include an XML document with a CreateHealthCheckRequest
* element. The response returns the
* CreateHealthCheckResponse
element that contains metadata
* about the health check.
*
*
* @param createHealthCheckRequest Container for the necessary parameters
* to execute the CreateHealthCheck operation on AmazonRoute53.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CreateHealthCheck service method, as returned by AmazonRoute53.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future createHealthCheckAsync(CreateHealthCheckRequest createHealthCheckRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* To retrieve a list of your health checks, send a GET
* request to the 2013-04-01/healthcheck
resource. The
* response to this request includes a HealthChecks
element
* with zero, one, or multiple HealthCheck
child elements.
* By default, the list of health checks is displayed on a single page.
* You can control the length of the page that is displayed by using the
* MaxItems
parameter. You can use the Marker
* parameter to control the health check that the list begins with.
*
*
* NOTE: Amazon Route 53 returns a maximum of 100 items. If you
* set MaxItems to a value greater than 100, Amazon Route 53 returns only
* the first 100.
*
*
* @param listHealthChecksRequest Container for the necessary parameters
* to execute the ListHealthChecks operation on AmazonRoute53.
*
* @return A Java Future object containing the response from the
* ListHealthChecks service method, as returned by AmazonRoute53.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listHealthChecksAsync(ListHealthChecksRequest listHealthChecksRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* To retrieve a list of your health checks, send a GET
* request to the 2013-04-01/healthcheck
resource. The
* response to this request includes a HealthChecks
element
* with zero, one, or multiple HealthCheck
child elements.
* By default, the list of health checks is displayed on a single page.
* You can control the length of the page that is displayed by using the
* MaxItems
parameter. You can use the Marker
* parameter to control the health check that the list begins with.
*
*
* NOTE: Amazon Route 53 returns a maximum of 100 items. If you
* set MaxItems to a value greater than 100, Amazon Route 53 returns only
* the first 100.
*
*
* @param listHealthChecksRequest Container for the necessary parameters
* to execute the ListHealthChecks operation on AmazonRoute53.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ListHealthChecks service method, as returned by AmazonRoute53.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listHealthChecksAsync(ListHealthChecksRequest listHealthChecksRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* This action deletes a health check. To delete a health check, send a
* DELETE
request to the 2013-04-01/healthcheck/health
* check ID
resource.
*
*
* IMPORTANT: You can delete a health check only if there are no
* resource record sets associated with this health check. If resource
* record sets are associated with this health check, you must
* disassociate them before you can delete your health check. If you try
* to delete a health check that is associated with resource record sets,
* Route 53 will deny your request with a HealthCheckInUse error. For
* information about disassociating the records from your health check,
* see ChangeResourceRecordSets.
*
*
* @param deleteHealthCheckRequest Container for the necessary parameters
* to execute the DeleteHealthCheck operation on AmazonRoute53.
*
* @return A Java Future object containing the response from the
* DeleteHealthCheck service method, as returned by AmazonRoute53.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteHealthCheckAsync(DeleteHealthCheckRequest deleteHealthCheckRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* This action deletes a health check. To delete a health check, send a
* DELETE
request to the 2013-04-01/healthcheck/health
* check ID
resource.
*
*
* IMPORTANT: You can delete a health check only if there are no
* resource record sets associated with this health check. If resource
* record sets are associated with this health check, you must
* disassociate them before you can delete your health check. If you try
* to delete a health check that is associated with resource record sets,
* Route 53 will deny your request with a HealthCheckInUse error. For
* information about disassociating the records from your health check,
* see ChangeResourceRecordSets.
*
*
* @param deleteHealthCheckRequest Container for the necessary parameters
* to execute the DeleteHealthCheck operation on AmazonRoute53.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteHealthCheck service method, as returned by AmazonRoute53.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteHealthCheckAsync(DeleteHealthCheckRequest deleteHealthCheckRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Imagine all the resource record sets in a zone listed out in front of
* you. Imagine them sorted lexicographically first by DNS name (with the
* labels reversed, like "com.amazon.www" for example), and secondarily,
* lexicographically by record type. This operation retrieves at most
* MaxItems resource record sets from this list, in order, starting at a
* position specified by the Name and Type arguments:
*
*
*
* - If both Name and Type are omitted, this means start the results
* at the first RRSET in the HostedZone.
* - If Name is specified but Type is omitted, this means start the
* results at the first RRSET in the list whose name is greater than or
* equal to Name.
* - If both Name and Type are specified, this means start the results
* at the first RRSET in the list whose name is greater than or equal to
* Name and whose type is greater than or equal to Type.
* - It is an error to specify the Type but not the Name.
*
*
*
* Use ListResourceRecordSets to retrieve a single known record set by
* specifying the record set's name and type, and setting MaxItems = 1
*
*
* To retrieve all the records in a HostedZone, first pause any processes
* making calls to ChangeResourceRecordSets. Initially call
* ListResourceRecordSets without a Name and Type to get the first page
* of record sets. For subsequent calls, set Name and Type to the
* NextName and NextType values returned by the previous response.
*
*
* In the presence of concurrent ChangeResourceRecordSets calls, there is
* no consistency of results across calls to ListResourceRecordSets. The
* only way to get a consistent multi-page snapshot of all RRSETs in a
* zone is to stop making changes while pagination is in progress.
*
*
* However, the results from ListResourceRecordSets are consistent within
* a page. If MakeChange calls are taking place concurrently, the result
* of each one will either be completely visible in your results or not
* at all. You will not see partial changes, or changes that do not
* ultimately succeed. (This follows from the fact that MakeChange is
* atomic)
*
*
* The results from ListResourceRecordSets are strongly consistent with
* ChangeResourceRecordSets. To be precise, if a single process makes a
* call to ChangeResourceRecordSets and receives a successful response,
* the effects of that change will be visible in a subsequent call to
* ListResourceRecordSets by that process.
*
*
* @param listResourceRecordSetsRequest Container for the necessary
* parameters to execute the ListResourceRecordSets operation on
* AmazonRoute53.
*
* @return A Java Future object containing the response from the
* ListResourceRecordSets service method, as returned by AmazonRoute53.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listResourceRecordSetsAsync(ListResourceRecordSetsRequest listResourceRecordSetsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Imagine all the resource record sets in a zone listed out in front of
* you. Imagine them sorted lexicographically first by DNS name (with the
* labels reversed, like "com.amazon.www" for example), and secondarily,
* lexicographically by record type. This operation retrieves at most
* MaxItems resource record sets from this list, in order, starting at a
* position specified by the Name and Type arguments:
*
*
*
* - If both Name and Type are omitted, this means start the results
* at the first RRSET in the HostedZone.
* - If Name is specified but Type is omitted, this means start the
* results at the first RRSET in the list whose name is greater than or
* equal to Name.
* - If both Name and Type are specified, this means start the results
* at the first RRSET in the list whose name is greater than or equal to
* Name and whose type is greater than or equal to Type.
* - It is an error to specify the Type but not the Name.
*
*
*
* Use ListResourceRecordSets to retrieve a single known record set by
* specifying the record set's name and type, and setting MaxItems = 1
*
*
* To retrieve all the records in a HostedZone, first pause any processes
* making calls to ChangeResourceRecordSets. Initially call
* ListResourceRecordSets without a Name and Type to get the first page
* of record sets. For subsequent calls, set Name and Type to the
* NextName and NextType values returned by the previous response.
*
*
* In the presence of concurrent ChangeResourceRecordSets calls, there is
* no consistency of results across calls to ListResourceRecordSets. The
* only way to get a consistent multi-page snapshot of all RRSETs in a
* zone is to stop making changes while pagination is in progress.
*
*
* However, the results from ListResourceRecordSets are consistent within
* a page. If MakeChange calls are taking place concurrently, the result
* of each one will either be completely visible in your results or not
* at all. You will not see partial changes, or changes that do not
* ultimately succeed. (This follows from the fact that MakeChange is
* atomic)
*
*
* The results from ListResourceRecordSets are strongly consistent with
* ChangeResourceRecordSets. To be precise, if a single process makes a
* call to ChangeResourceRecordSets and receives a successful response,
* the effects of that change will be visible in a subsequent call to
* ListResourceRecordSets by that process.
*
*
* @param listResourceRecordSetsRequest Container for the necessary
* parameters to execute the ListResourceRecordSets operation on
* AmazonRoute53.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ListResourceRecordSets service method, as returned by AmazonRoute53.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listResourceRecordSetsAsync(ListResourceRecordSetsRequest listResourceRecordSetsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
}