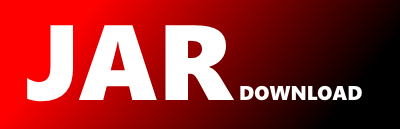
com.amazonaws.services.route53.AmazonRoute53Client Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.route53;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import java.util.Map.Entry;
import com.amazonaws.*;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.services.route53.model.*;
import com.amazonaws.services.route53.model.transform.*;
/**
* Client for accessing AmazonRoute53. All service calls made
* using this client are blocking, and will not return until the service call
* completes.
*
*
*/
public class AmazonRoute53Client extends AmazonWebServiceClient implements AmazonRoute53 {
/** Provider for AWS credentials. */
private AWSCredentialsProvider awsCredentialsProvider;
/**
* List of exception unmarshallers for all AmazonRoute53 exceptions.
*/
protected final List> exceptionUnmarshallers
= new ArrayList>();
/**
* Constructs a new client to invoke service methods on
* AmazonRoute53. A credentials provider chain will be used
* that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @see DefaultAWSCredentialsProviderChain
*/
public AmazonRoute53Client() {
this(new DefaultAWSCredentialsProviderChain(), new ClientConfiguration());
}
/**
* Constructs a new client to invoke service methods on
* AmazonRoute53. A credentials provider chain will be used
* that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to AmazonRoute53
* (ex: proxy settings, retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
*/
public AmazonRoute53Client(ClientConfiguration clientConfiguration) {
this(new DefaultAWSCredentialsProviderChain(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on
* AmazonRoute53 using the specified AWS account credentials.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentials The AWS credentials (access key ID and secret key) to use
* when authenticating with AWS services.
*/
public AmazonRoute53Client(AWSCredentials awsCredentials) {
this(awsCredentials, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service methods on
* AmazonRoute53 using the specified AWS account credentials
* and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentials The AWS credentials (access key ID and secret key) to use
* when authenticating with AWS services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to AmazonRoute53
* (ex: proxy settings, retry counts, etc.).
*/
public AmazonRoute53Client(AWSCredentials awsCredentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.awsCredentialsProvider = new StaticCredentialsProvider(awsCredentials);
init();
}
/**
* Constructs a new client to invoke service methods on
* AmazonRoute53 using the specified AWS account credentials provider.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
*/
public AmazonRoute53Client(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, new ClientConfiguration());
}
/**
* Constructs a new client to invoke service methods on
* AmazonRoute53 using the specified AWS account credentials
* provider and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to AmazonRoute53
* (ex: proxy settings, retry counts, etc.).
*/
public AmazonRoute53Client(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on
* AmazonRoute53 using the specified AWS account credentials
* provider, client configuration options, and request metric collector.
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to AmazonRoute53
* (ex: proxy settings, retry counts, etc.).
* @param requestMetricCollector optional request metric collector
*/
public AmazonRoute53Client(AWSCredentialsProvider awsCredentialsProvider,
ClientConfiguration clientConfiguration,
RequestMetricCollector requestMetricCollector) {
super(clientConfiguration, requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
init();
}
private void init() {
exceptionUnmarshallers.add(new NoSuchChangeExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyHealthChecksExceptionUnmarshaller());
exceptionUnmarshallers.add(new HostedZoneAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new TooManyHostedZonesExceptionUnmarshaller());
exceptionUnmarshallers.add(new HostedZoneNotEmptyExceptionUnmarshaller());
exceptionUnmarshallers.add(new IncompatibleVersionExceptionUnmarshaller());
exceptionUnmarshallers.add(new HealthCheckAlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers.add(new NoSuchHealthCheckExceptionUnmarshaller());
exceptionUnmarshallers.add(new PriorRequestNotCompleteExceptionUnmarshaller());
exceptionUnmarshallers.add(new HealthCheckInUseExceptionUnmarshaller());
exceptionUnmarshallers.add(new DelegationSetNotAvailableExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidInputExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidChangeBatchExceptionUnmarshaller());
exceptionUnmarshallers.add(new NoSuchHostedZoneExceptionUnmarshaller());
exceptionUnmarshallers.add(new InvalidDomainNameExceptionUnmarshaller());
exceptionUnmarshallers.add(new StandardErrorUnmarshaller());
// calling this.setEndPoint(...) will also modify the signer accordingly
this.setEndpoint("route53.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain(
"/com/amazonaws/services/route53/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain(
"/com/amazonaws/services/route53/request.handler2s"));
}
/**
*
* To retrieve the health check, send a GET
request to the
* 2013-04-01/healthcheck/health check ID
resource.
*
*
* @param getHealthCheckRequest Container for the necessary parameters to
* execute the GetHealthCheck service method on AmazonRoute53.
*
* @return The response from the GetHealthCheck service method, as
* returned by AmazonRoute53.
*
* @throws NoSuchHealthCheckException
* @throws IncompatibleVersionException
* @throws InvalidInputException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public GetHealthCheckResult getHealthCheck(GetHealthCheckRequest getHealthCheckRequest) {
ExecutionContext executionContext = createExecutionContext(getHealthCheckRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new GetHealthCheckRequestMarshaller().marshall(getHealthCheckRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new GetHealthCheckResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* To retrieve the delegation set for a hosted zone, send a
* GET
request to the 2013-04-01/hostedzone/hosted
* zone ID
resource. The delegation set is the four Route 53 name
* servers that were assigned to the hosted zone when you created it.
*
*
* @param getHostedZoneRequest Container for the necessary parameters to
* execute the GetHostedZone service method on AmazonRoute53.
*
* @return The response from the GetHostedZone service method, as
* returned by AmazonRoute53.
*
* @throws NoSuchHostedZoneException
* @throws InvalidInputException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public GetHostedZoneResult getHostedZone(GetHostedZoneRequest getHostedZoneRequest) {
ExecutionContext executionContext = createExecutionContext(getHostedZoneRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new GetHostedZoneRequestMarshaller().marshall(getHostedZoneRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new GetHostedZoneResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This action deletes a hosted zone. To delete a hosted zone, send a
* DELETE
request to the 2013-04-01/hostedzone/hosted
* zone ID
resource.
*
*
* For more information about deleting a hosted zone, see
* Deleting a Hosted Zone
* in the Amazon Route 53 Developer Guide .
*
*
* IMPORTANT: You can delete a hosted zone only if there are no
* resource record sets other than the default SOA record and NS resource
* record sets. If your hosted zone contains other resource record sets,
* you must delete them before you can delete your hosted zone. If you
* try to delete a hosted zone that contains other resource record sets,
* Route 53 will deny your request with a HostedZoneNotEmpty error. For
* information about deleting records from your hosted zone, see
* ChangeResourceRecordSets.
*
*
* @param deleteHostedZoneRequest Container for the necessary parameters
* to execute the DeleteHostedZone service method on AmazonRoute53.
*
* @return The response from the DeleteHostedZone service method, as
* returned by AmazonRoute53.
*
* @throws PriorRequestNotCompleteException
* @throws HostedZoneNotEmptyException
* @throws NoSuchHostedZoneException
* @throws InvalidInputException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DeleteHostedZoneResult deleteHostedZone(DeleteHostedZoneRequest deleteHostedZoneRequest) {
ExecutionContext executionContext = createExecutionContext(deleteHostedZoneRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteHostedZoneRequestMarshaller().marshall(deleteHostedZoneRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DeleteHostedZoneResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This action returns the current status of a change batch request. The
* status is one of the following values:
*
*
* - PENDING
indicates that the changes in this request have
* not replicated to all Route 53 DNS servers. This is the initial status
* of all change batch requests.
*
*
* - INSYNC
indicates that the changes have replicated to
* all Amazon Route 53 DNS servers.
*
*
* @param getChangeRequest Container for the necessary parameters to
* execute the GetChange service method on AmazonRoute53.
*
* @return The response from the GetChange service method, as returned by
* AmazonRoute53.
*
* @throws NoSuchChangeException
* @throws InvalidInputException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public GetChangeResult getChange(GetChangeRequest getChangeRequest) {
ExecutionContext executionContext = createExecutionContext(getChangeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new GetChangeRequestMarshaller().marshall(getChangeRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new GetChangeResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* To retrieve a list of your hosted zones, send a GET
* request to the 2013-04-01/hostedzone
resource. The
* response to this request includes a HostedZones
element
* with zero, one, or multiple HostedZone
child elements. By
* default, the list of hosted zones is displayed on a single page. You
* can control the length of the page that is displayed by using the
* MaxItems
parameter. You can use the Marker
* parameter to control the hosted zone that the list begins with.
*
*
* NOTE: Amazon Route 53 returns a maximum of 100 items. If you
* set MaxItems to a value greater than 100, Amazon Route 53 returns only
* the first 100.
*
*
* @param listHostedZonesRequest Container for the necessary parameters
* to execute the ListHostedZones service method on AmazonRoute53.
*
* @return The response from the ListHostedZones service method, as
* returned by AmazonRoute53.
*
* @throws InvalidInputException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListHostedZonesResult listHostedZones(ListHostedZonesRequest listHostedZonesRequest) {
ExecutionContext executionContext = createExecutionContext(listHostedZonesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ListHostedZonesRequestMarshaller().marshall(listHostedZonesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ListHostedZonesResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This action creates a new hosted zone.
*
*
* To create a new hosted zone, send a POST
request to the
* 2013-04-01/hostedzone
resource. The request body must
* include an XML document with a CreateHostedZoneRequest
* element. The response returns the
* CreateHostedZoneResponse
element that contains metadata
* about the hosted zone.
*
*
* Route 53 automatically creates a default SOA record and four NS
* records for the zone. The NS records in the hosted zone are the name
* servers you give your registrar to delegate your domain to. For more
* information about SOA and NS records, see
* NS and SOA Records that Route 53 Creates for a Hosted Zone
* in the Amazon Route 53 Developer Guide .
*
*
* When you create a zone, its initial status is PENDING
.
* This means that it is not yet available on all DNS servers. The status
* of the zone changes to INSYNC
when the NS and SOA records
* are available on all Route 53 DNS servers.
*
*
* @param createHostedZoneRequest Container for the necessary parameters
* to execute the CreateHostedZone service method on AmazonRoute53.
*
* @return The response from the CreateHostedZone service method, as
* returned by AmazonRoute53.
*
* @throws TooManyHostedZonesException
* @throws DelegationSetNotAvailableException
* @throws InvalidDomainNameException
* @throws InvalidInputException
* @throws HostedZoneAlreadyExistsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateHostedZoneResult createHostedZone(CreateHostedZoneRequest createHostedZoneRequest) {
ExecutionContext executionContext = createExecutionContext(createHostedZoneRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateHostedZoneRequestMarshaller().marshall(createHostedZoneRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateHostedZoneResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Use this action to create or change your authoritative DNS
* information. To use this action, send a POST
request to
* the 2013-04-01/hostedzone/hosted Zone ID/rrset
resource.
* The request body must include an XML document with a
* ChangeResourceRecordSetsRequest
element.
*
*
* Changes are a list of change items and are considered transactional.
* For more information on transactional changes, also known as change
* batches, see
* Creating, Changing, and Deleting Resource Record Sets Using the Route 53 API
* in the Amazon Route 53 Developer Guide .
*
*
* IMPORTANT:Due to the nature of transactional changes, you
* cannot delete the same resource record set more than once in a single
* change batch. If you attempt to delete the same change batch more than
* once, Route 53 returns an InvalidChangeBatch error.
*
*
* In response to a ChangeResourceRecordSets
request, your
* DNS data is changed on all Route 53 DNS servers. Initially, the status
* of a change is PENDING
. This means the change has not
* yet propagated to all the authoritative Route 53 DNS servers. When the
* change is propagated to all hosts, the change returns a status of
* INSYNC
.
*
*
* Note the following limitations on a
* ChangeResourceRecordSets
request:
*
*
* - A request cannot contain more than 100 Change elements.
*
*
* - A request cannot contain more than 1000 ResourceRecord elements.
*
*
* The sum of the number of characters (including spaces) in all
* Value
elements in a request cannot exceed 32,000
* characters.
*
*
* @param changeResourceRecordSetsRequest Container for the necessary
* parameters to execute the ChangeResourceRecordSets service method on
* AmazonRoute53.
*
* @return The response from the ChangeResourceRecordSets service method,
* as returned by AmazonRoute53.
*
* @throws PriorRequestNotCompleteException
* @throws NoSuchHealthCheckException
* @throws NoSuchHostedZoneException
* @throws InvalidInputException
* @throws InvalidChangeBatchException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public ChangeResourceRecordSetsResult changeResourceRecordSets(ChangeResourceRecordSetsRequest changeResourceRecordSetsRequest) {
ExecutionContext executionContext = createExecutionContext(changeResourceRecordSetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ChangeResourceRecordSetsRequestMarshaller().marshall(changeResourceRecordSetsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ChangeResourceRecordSetsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This action creates a new health check.
*
*
* To create a new health check, send a POST
request to the
* 2013-04-01/healthcheck
resource. The request body must
* include an XML document with a CreateHealthCheckRequest
* element. The response returns the
* CreateHealthCheckResponse
element that contains metadata
* about the health check.
*
*
* @param createHealthCheckRequest Container for the necessary parameters
* to execute the CreateHealthCheck service method on AmazonRoute53.
*
* @return The response from the CreateHealthCheck service method, as
* returned by AmazonRoute53.
*
* @throws HealthCheckAlreadyExistsException
* @throws InvalidInputException
* @throws TooManyHealthChecksException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public CreateHealthCheckResult createHealthCheck(CreateHealthCheckRequest createHealthCheckRequest) {
ExecutionContext executionContext = createExecutionContext(createHealthCheckRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new CreateHealthCheckRequestMarshaller().marshall(createHealthCheckRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new CreateHealthCheckResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* To retrieve a list of your health checks, send a GET
* request to the 2013-04-01/healthcheck
resource. The
* response to this request includes a HealthChecks
element
* with zero, one, or multiple HealthCheck
child elements.
* By default, the list of health checks is displayed on a single page.
* You can control the length of the page that is displayed by using the
* MaxItems
parameter. You can use the Marker
* parameter to control the health check that the list begins with.
*
*
* NOTE: Amazon Route 53 returns a maximum of 100 items. If you
* set MaxItems to a value greater than 100, Amazon Route 53 returns only
* the first 100.
*
*
* @param listHealthChecksRequest Container for the necessary parameters
* to execute the ListHealthChecks service method on AmazonRoute53.
*
* @return The response from the ListHealthChecks service method, as
* returned by AmazonRoute53.
*
* @throws IncompatibleVersionException
* @throws InvalidInputException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListHealthChecksResult listHealthChecks(ListHealthChecksRequest listHealthChecksRequest) {
ExecutionContext executionContext = createExecutionContext(listHealthChecksRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ListHealthChecksRequestMarshaller().marshall(listHealthChecksRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ListHealthChecksResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* This action deletes a health check. To delete a health check, send a
* DELETE
request to the 2013-04-01/healthcheck/health
* check ID
resource.
*
*
* IMPORTANT: You can delete a health check only if there are no
* resource record sets associated with this health check. If resource
* record sets are associated with this health check, you must
* disassociate them before you can delete your health check. If you try
* to delete a health check that is associated with resource record sets,
* Route 53 will deny your request with a HealthCheckInUse error. For
* information about disassociating the records from your health check,
* see ChangeResourceRecordSets.
*
*
* @param deleteHealthCheckRequest Container for the necessary parameters
* to execute the DeleteHealthCheck service method on AmazonRoute53.
*
* @return The response from the DeleteHealthCheck service method, as
* returned by AmazonRoute53.
*
* @throws NoSuchHealthCheckException
* @throws HealthCheckInUseException
* @throws InvalidInputException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public DeleteHealthCheckResult deleteHealthCheck(DeleteHealthCheckRequest deleteHealthCheckRequest) {
ExecutionContext executionContext = createExecutionContext(deleteHealthCheckRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new DeleteHealthCheckRequestMarshaller().marshall(deleteHealthCheckRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new DeleteHealthCheckResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Imagine all the resource record sets in a zone listed out in front of
* you. Imagine them sorted lexicographically first by DNS name (with the
* labels reversed, like "com.amazon.www" for example), and secondarily,
* lexicographically by record type. This operation retrieves at most
* MaxItems resource record sets from this list, in order, starting at a
* position specified by the Name and Type arguments:
*
*
*
* - If both Name and Type are omitted, this means start the results
* at the first RRSET in the HostedZone.
* - If Name is specified but Type is omitted, this means start the
* results at the first RRSET in the list whose name is greater than or
* equal to Name.
* - If both Name and Type are specified, this means start the results
* at the first RRSET in the list whose name is greater than or equal to
* Name and whose type is greater than or equal to Type.
* - It is an error to specify the Type but not the Name.
*
*
*
* Use ListResourceRecordSets to retrieve a single known record set by
* specifying the record set's name and type, and setting MaxItems = 1
*
*
* To retrieve all the records in a HostedZone, first pause any processes
* making calls to ChangeResourceRecordSets. Initially call
* ListResourceRecordSets without a Name and Type to get the first page
* of record sets. For subsequent calls, set Name and Type to the
* NextName and NextType values returned by the previous response.
*
*
* In the presence of concurrent ChangeResourceRecordSets calls, there is
* no consistency of results across calls to ListResourceRecordSets. The
* only way to get a consistent multi-page snapshot of all RRSETs in a
* zone is to stop making changes while pagination is in progress.
*
*
* However, the results from ListResourceRecordSets are consistent within
* a page. If MakeChange calls are taking place concurrently, the result
* of each one will either be completely visible in your results or not
* at all. You will not see partial changes, or changes that do not
* ultimately succeed. (This follows from the fact that MakeChange is
* atomic)
*
*
* The results from ListResourceRecordSets are strongly consistent with
* ChangeResourceRecordSets. To be precise, if a single process makes a
* call to ChangeResourceRecordSets and receives a successful response,
* the effects of that change will be visible in a subsequent call to
* ListResourceRecordSets by that process.
*
*
* @param listResourceRecordSetsRequest Container for the necessary
* parameters to execute the ListResourceRecordSets service method on
* AmazonRoute53.
*
* @return The response from the ListResourceRecordSets service method,
* as returned by AmazonRoute53.
*
* @throws NoSuchHostedZoneException
* @throws InvalidInputException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListResourceRecordSetsResult listResourceRecordSets(ListResourceRecordSetsRequest listResourceRecordSetsRequest) {
ExecutionContext executionContext = createExecutionContext(listResourceRecordSetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
Request request = null;
Response response = null;
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
try {
request = new ListResourceRecordSetsRequestMarshaller().marshall(listResourceRecordSetsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
response = invoke(request, new ListResourceRecordSetsResultStaxUnmarshaller(), executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* To retrieve a list of your hosted zones, send a GET
* request to the 2013-04-01/hostedzone
resource. The
* response to this request includes a HostedZones
element
* with zero, one, or multiple HostedZone
child elements. By
* default, the list of hosted zones is displayed on a single page. You
* can control the length of the page that is displayed by using the
* MaxItems
parameter. You can use the Marker
* parameter to control the hosted zone that the list begins with.
*
*
* NOTE: Amazon Route 53 returns a maximum of 100 items. If you
* set MaxItems to a value greater than 100, Amazon Route 53 returns only
* the first 100.
*
*
* @return The response from the ListHostedZones service method, as
* returned by AmazonRoute53.
*
* @throws InvalidInputException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListHostedZonesResult listHostedZones() throws AmazonServiceException, AmazonClientException {
return listHostedZones(new ListHostedZonesRequest());
}
/**
*
* To retrieve a list of your health checks, send a GET
* request to the 2013-04-01/healthcheck
resource. The
* response to this request includes a HealthChecks
element
* with zero, one, or multiple HealthCheck
child elements.
* By default, the list of health checks is displayed on a single page.
* You can control the length of the page that is displayed by using the
* MaxItems
parameter. You can use the Marker
* parameter to control the health check that the list begins with.
*
*
* NOTE: Amazon Route 53 returns a maximum of 100 items. If you
* set MaxItems to a value greater than 100, Amazon Route 53 returns only
* the first 100.
*
*
* @return The response from the ListHealthChecks service method, as
* returned by AmazonRoute53.
*
* @throws IncompatibleVersionException
* @throws InvalidInputException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonRoute53 indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListHealthChecksResult listHealthChecks() throws AmazonServiceException, AmazonClientException {
return listHealthChecks(new ListHealthChecksRequest());
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for
* debugging issues where a service isn't acting as expected. This data isn't considered part
* of the result data returned by an operation, so it's available through this separate,
* diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access
* this extra diagnostic information for an executed request, you should use this method
* to retrieve it as soon as possible after executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none
* is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
private Response invoke(Request request,
Unmarshaller unmarshaller,
ExecutionContext executionContext)
{
request.setEndpoint(endpoint);
request.setTimeOffset(timeOffset);
AmazonWebServiceRequest originalRequest = request.getOriginalRequest();
for (Entry entry : originalRequest.copyPrivateRequestParameters().entrySet()) {
request.addParameter(entry.getKey(), entry.getValue());
}
AWSCredentials credentials = awsCredentialsProvider.getCredentials();
if (originalRequest.getRequestCredentials() != null) {
credentials = originalRequest.getRequestCredentials();
}
executionContext.setCredentials(credentials);
StaxResponseHandler responseHandler = new StaxResponseHandler(unmarshaller);
DefaultErrorResponseHandler errorResponseHandler = new DefaultErrorResponseHandler(exceptionUnmarshallers);
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
}